The Firebase C++ SDK enables developers to integrate Firebase services into their C++ applications, providing powerful features like real-time databases, authentication, and cloud storage.
Here’s a simple code snippet to demonstrate how to initialize Firebase in a C++ application:
#include "firebase/app.h"
#include "firebase/auth.h"
int main() {
// Initialize the Firebase App
firebase::App* app = firebase::App::Create(firebase::AppOptions());
// Initialize Firebase Authentication
firebase::auth::Auth* auth = firebase::auth::Auth::GetAuth(app);
// Your further implementation goes here...
return 0;
}
Understanding Firebase C++ SDK
What is Firebase? Firebase is a comprehensive app development platform created by Google, designed to help developers build high-quality mobile and web applications. It provides a wide variety of services, including real-time databases, authentication, analytics, cloud storage, and messaging.
Why use C++ for Firebase? Utilizing C++ for Firebase development offers several advantages. C++ is known for its performance and efficiency, making it a suitable choice for applications that require fast processing and seamless interaction with back-end services. C++ also allows developers to integrate Firebase functionalities into applications across multiple platforms, including mobile and desktop applications.
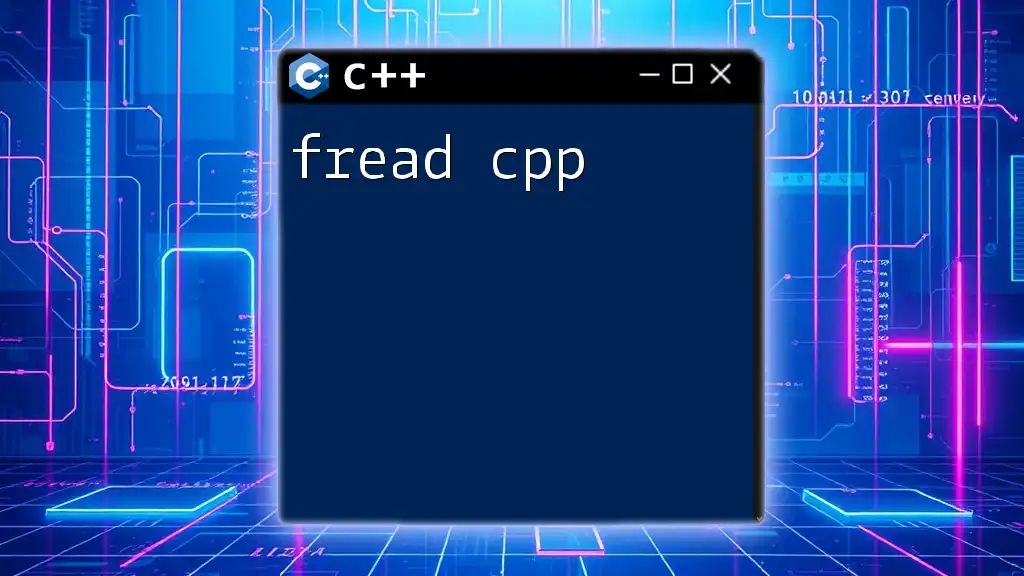
Components of Firebase C++ SDK
What is Firebase C++ SDK? The Firebase C++ SDK is a set of tools and libraries that enable C++ developers to integrate Firebase services into their applications. It enables developers to leverage powerful Firebase features while utilizing the versatility and performance of C++.
Core Services provided by the Firebase C++ SDK include:
- Realtime Database: A cloud-hosted NoSQL database that allows data to be synced in real time across all clients.
- Authentication: Simplifies managing user authentication with various methods including email/password, phone numbers, and third-party providers like Google and Facebook.
- Cloud Storage: Offers a robust solution for storing and serving user-generated content, such as images and videos.
- Messaging: A service for sending notifications and messages to users through Firebase Cloud Messaging (FCM).
Additional Features that come with the SDK are:
- Analytics: Provides insights into user behavior and engagement within your application.
- Remote Config: Allows for dynamic changes in your app’s configuration, enabling A/B testing and feature flags.
- Crashlytics: Helps in tracking crashes and performance issues, providing detailed reports to improve app stability.
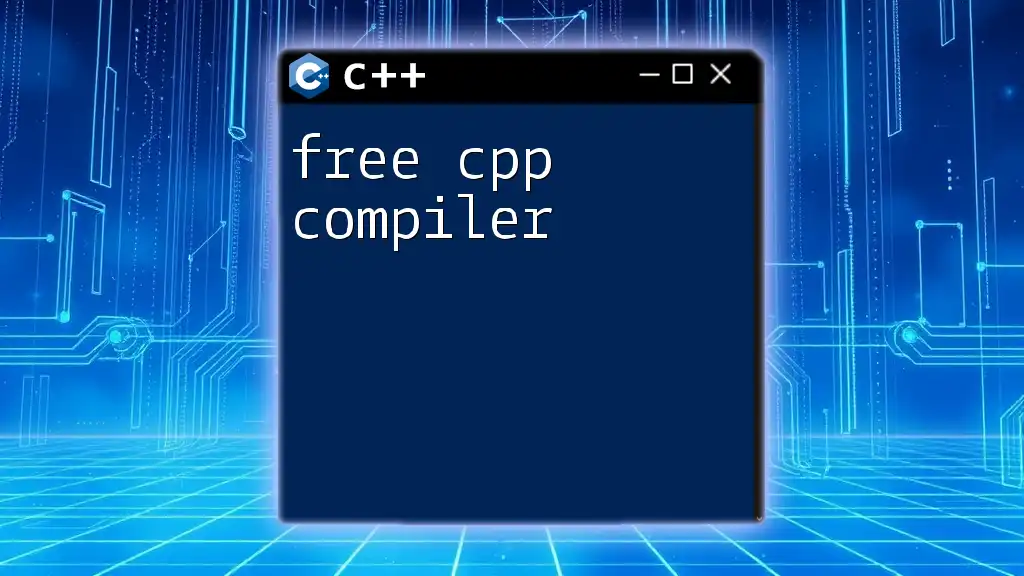
Setting Up Firebase C++ SDK
System Requirements: Before getting started with the Firebase C++ SDK, ensure that your development environment meets the following requirements:
- Compatible operating systems: Windows, macOS, or Linux.
- Additional tools: CMake or Android Studio, based on the platform of your choice.
Installation Guide
-
Step 1: Create a Firebase Project
Navigate to the Firebase Console and create a new project. Provide a name and other relevant details to set up your project. -
Step 2: Download Firebase C++ SDK
Access the official Firebase repository on GitHub and download the C++ SDK. Extract the files to your preferred directory for easy access. -
Step 3: Integrating Firebase SDK into Your C++ Project
After setting up your development environment, add Firebase to your project using CMake. This can be done by including the following configuration in your CMakeLists.txt file:
cmake_minimum_required(VERSION 3.10)
project(MyFirebaseApp)
# Add Firebase C++ SDK as a subdirectory
add_subdirectory(path/to/firebase)
This setup ensures that the Firebase libraries are correctly included in your build process.
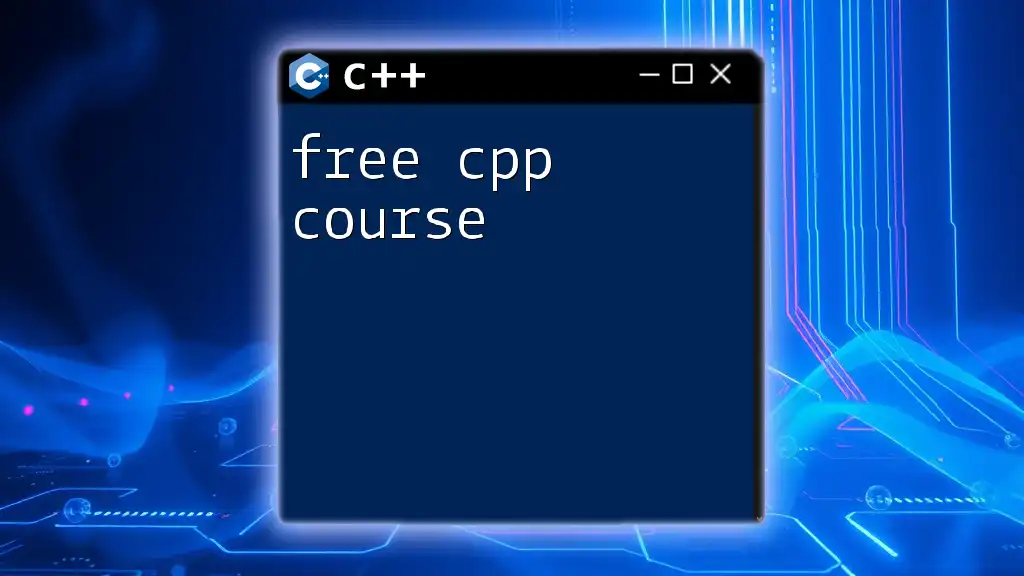
Initializing Firebase in Your C++ Application
Setting Up Firebase App: The first step in your application is to initialize the Firebase app to gain access to its services.
#include "firebase/app.h"
// Create a Firebase app instance
firebase::App* app = firebase::App::Create(firebase::AppOptions());
Configuring Services: After initializing the Firebase app, you can configure various services, like the Realtime Database or Authentication, to integrate them into your application.
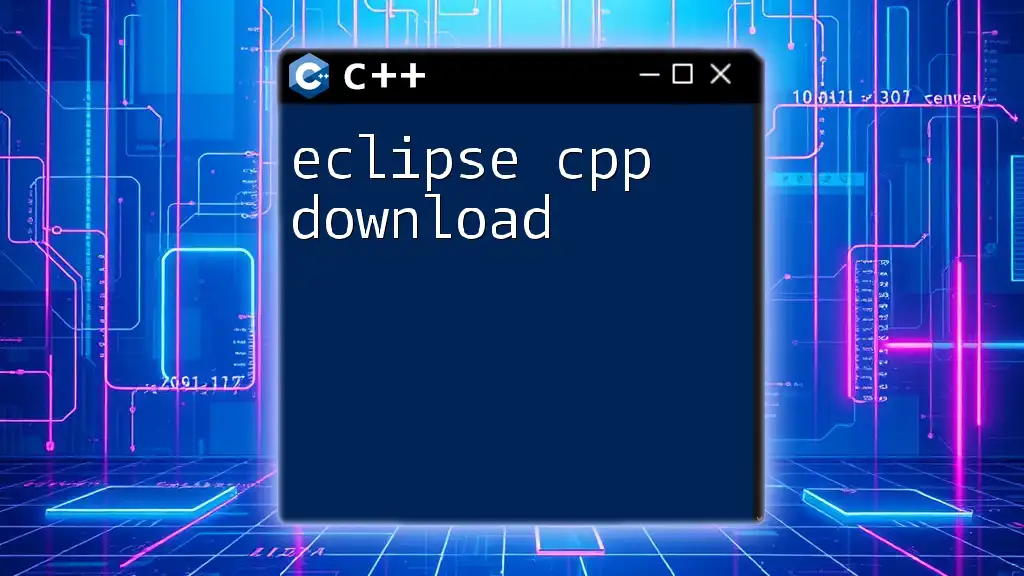
Using Firebase C++ SDK Features
Realtime Database
-
Setting Up the Database: Create a Realtime Database through the Firebase Console and configure the necessary security rules for data access.
-
Code Snippet for Reading/Writing Data: Here’s an example of how to write data to the Realtime Database:
#include "firebase/database.h"
firebase::database::Database* database = firebase::database::Database::GetInstance(app);
firebase::database::DatabaseReference ref = database->GetReference("example/path");
// Write data to the database
ref.SetValue("Hello, Firebase!");
This simple example shows how to structure and manipulate data efficiently.
User Authentication
-
Methods of Authentication: Firebase supports various authentication methods. This can include email/password, phone number verification, and third-party providers like Google and Facebook.
-
Code Snippet for User Authentication: Below is a code snippet to create a user account with an email and password:
#include "firebase/auth.h"
firebase::auth::Auth* auth = firebase::auth::Auth::GetAuth(app);
auth->CreateUserWithEmailAndPassword("user@example.com", "password123");
This powerful feature streamlines the user sign-up process, enhancing user experience.
Cloud Storage
- Uploading and Downloading Files: Firebase Storage provides an easy way to store and retrieve user-generated content. Below is a sample of how to upload a file:
#include "firebase/storage.h"
firebase::storage::Storage* storage = firebase::storage::Storage::GetInstance(app);
firebase::storage::StorageReference storage_ref = storage->GetReference("uploads/file.txt");
// Upload a file to cloud storage
storage_ref.PutFile("local/path/to/file.txt");
This allows developers to manage user data efficiently and securely.
Messaging
- Setting Up Notifications: Utilize Firebase Cloud Messaging (FCM) to send notifications to users. This can enhance user engagement by keeping them informed of important updates and messages.
// FCM initialization code would be placed here to handle message sending
In this section, ensure you include information on configuring FCM in the Firebase Console and implementing the appropriate handlers within your application logic.
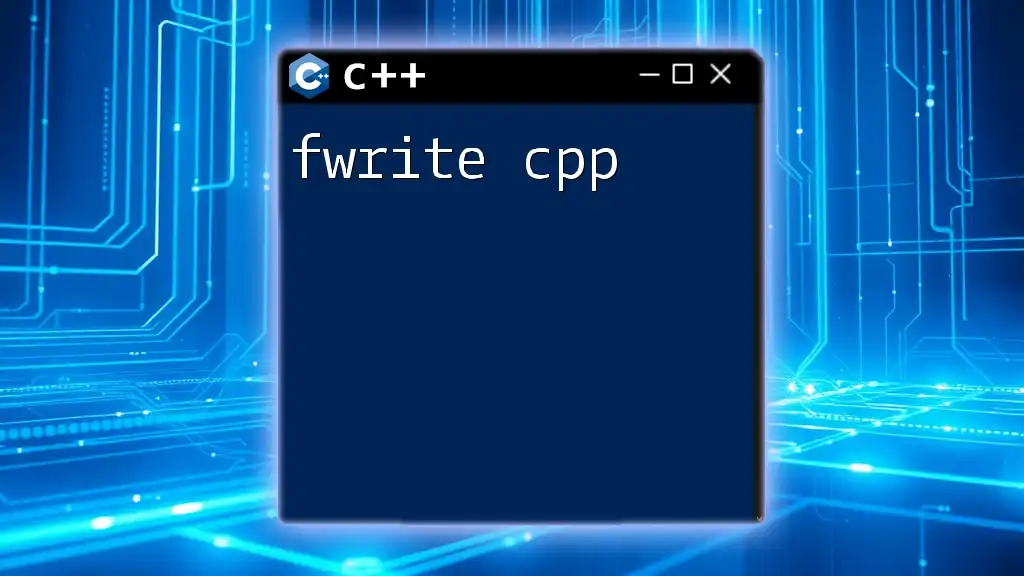
Best Practices for Using Firebase C++ SDK
Security Rules: Implement appropriate security rules for your database and storage. Properly configured rules ensure that data is only accessible by authorized entities, protecting user privacy and data integrity.
Efficient Data Handling: Optimize your data reads and writes. For example, batch your database writes when possible to minimize the number of round trips to the server and improve app performance.
Error Handling in Firebase: Implement robust error handling throughout your application. Here’s how to check for errors when reading or writing data:
if (result.error() != 0) {
std::cerr << "Error occurred: " << result.error_message() << std::endl;
}
Properly handling errors not only improves user experience but also facilitates easier debugging as you develop your application.
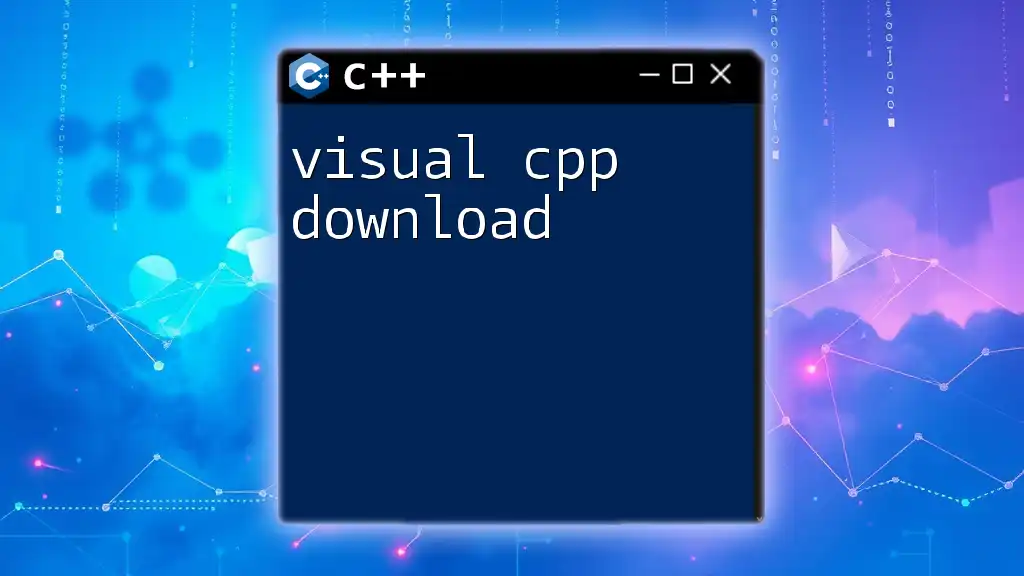
Troubleshooting Common Issues
Common Installation Problems: Some developers might face installation issues. Ensure that you have the correct version of all necessary dependencies as specified in the Firebase documentation.
Debugging Firebase Interactions: It’s critical to have reliable logging in place to capture interaction issues with Firebase services. Use built-in logging frameworks or custom console output to trace problems effectively.
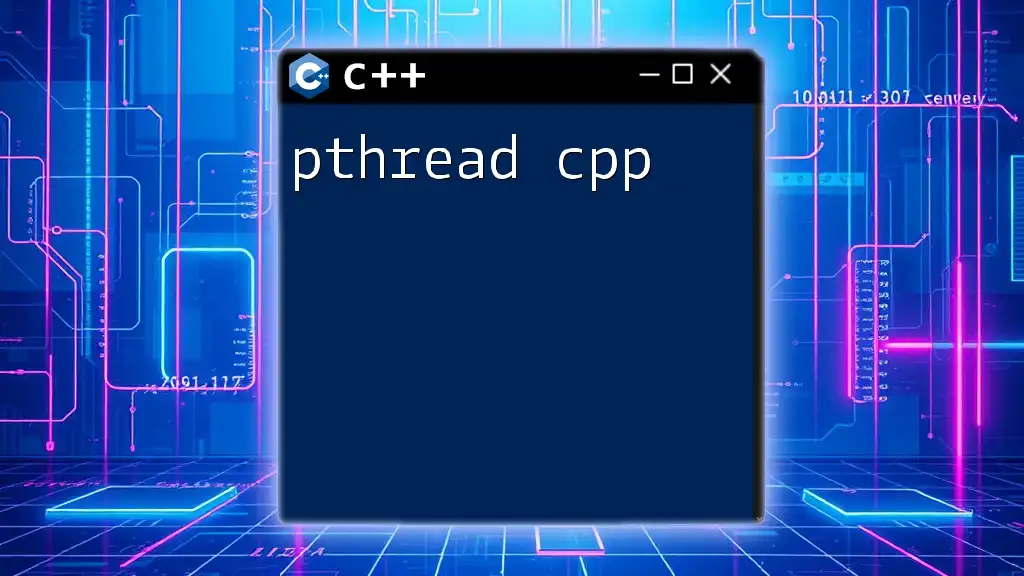
Conclusion
In this guide, we have explored the Firebase C++ SDK, delving into its powerful features, installation procedures, and practical code snippets for various functionalities. By effectively leveraging the Firebase SDK in your C++ applications, you can accelerate development and enhance the overall user experience. As you continue your journey with Firebase, consider further resources such as official documentation and community forums to continue learning and improving your skills.