A C++ code checker is a tool that analyzes your C++ code for syntax errors, coding standards, and potential bugs, ensuring that your program runs smoothly and efficiently.
Here's a simple example of a code snippet you might use to check for syntax using a common compiler:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is a C++ Code Checker?
A C++ code checker is a tool designed to analyze C++ source code for potential errors and code quality issues before compilation. Its primary goal is to improve the reliability and maintainability of software by identifying bugs, inefficiencies, and stylistic discrepancies in the code.
Code checkers can detect a variety of issues, categorized as follows:
- Syntax Errors: These are violations of the C++ language rules that can cause compilation failures.
- Semantic Errors: Issues that occur when statements are syntactically correct but logically flawed, leading to incorrect behavior in execution.
- Logical Errors: Errors that arise when the code compiles and runs without crashing but produces unintended outcomes.
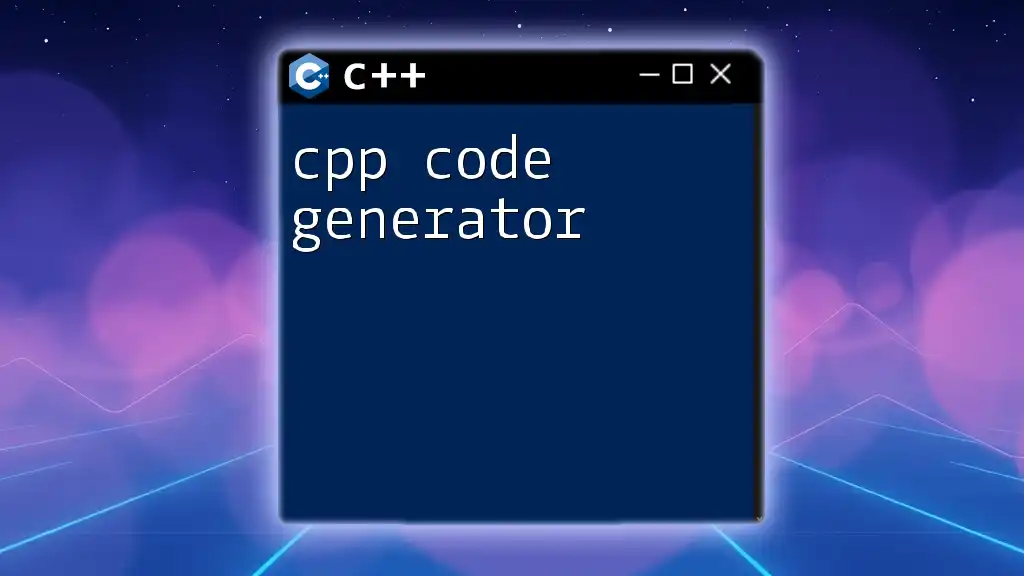
Why Use a C++ Code Checker?
Using a C++ code checker offers several invaluable benefits that enhance the development process:
- Improved Code Quality: Code checkers help ensure that your code complies with best practices and style guidelines, resulting in cleaner and more readable code that is easier to maintain.
- Faster Debugging Process: By identifying issues early in the development cycle, debugging time is significantly reduced. This leads to quicker iterations and timely project completions.
- Consistency in Coding Practices: Teams can benefit from having a standardized codebase. A code checker enforces rules across all contributions, leading to uniformity in how code is written and organized.
Consider the common pitfalls in C++ coding, such as memory leaks, uninitialized variables, and improper use of pointers. A cpp code checker can help catch these errors before they escalate into major issues.
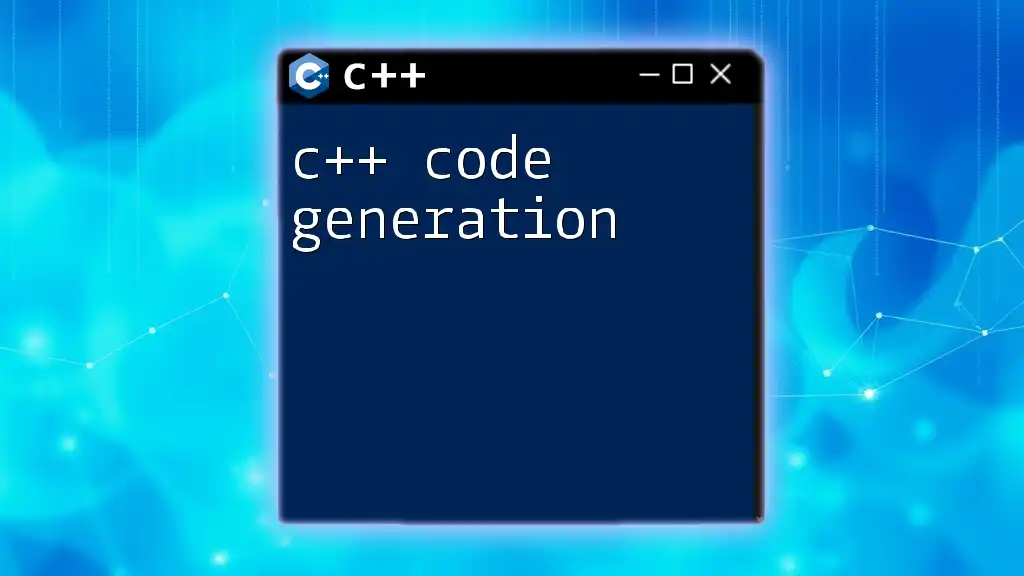
Types of C++ Code Checkers
Static Code Analysis Tools
Static code analysis tools analyze the code without executing it. They perform a variety of checks to catch potential issues early in the development cycle.
Popular static code analysis tools include:
- CPPcheck: A versatile and widely used static analyzer that focuses on code quality, and can handle various C++ versions.
- Clang Static Analyzer: This tool is part of the Clang project and provides valuable diagnostic capabilities, particularly around safety and performance issues.
Here is how you can use CPPcheck:
cppcheck --enable=all your_code.cpp
This command enables all checks, providing a comprehensive analysis of your C++ file.
Dynamic Code Analysis Tools
Dynamic code analysis tools, unlike static ones, analyze the program while it is running to catch runtime errors and memory issues.
Notable dynamic code analysis tools include:
- Valgrind: Primarily focused on memory debugging, this tool is extremely effective in identifying memory leaks and improper memory access.
- GDB (GNU Debugger): While primarily a debugger, GDB can also perform dynamic checks that help in tracking down runtime issues.
Using Valgrind for analyzing memory usage can be done like this:
valgrind ./your_executable
This command will execute your program under Valgrind’s supervision, producing a detailed report of any memory issues encountered.
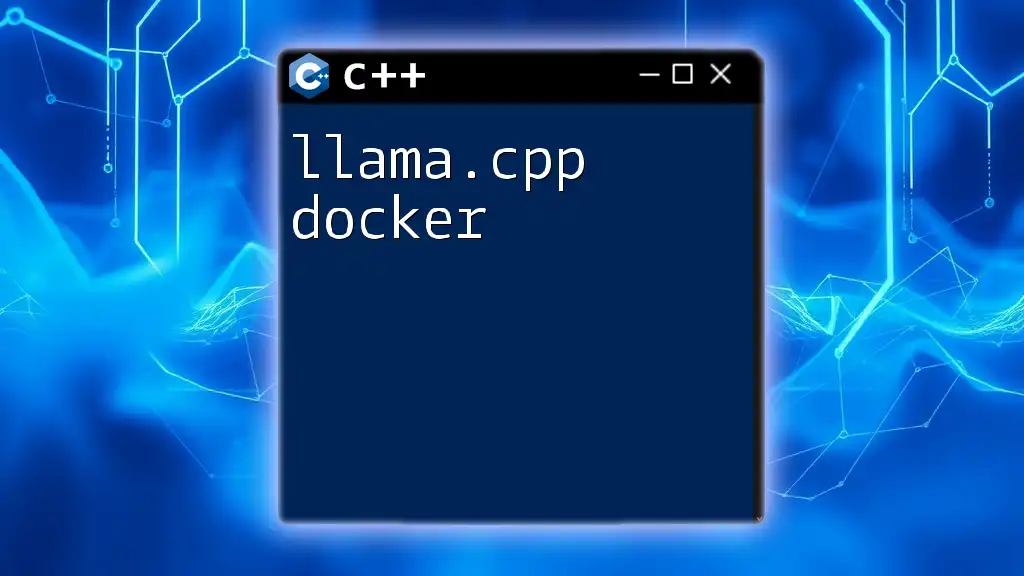
Popular C++ Code Checkers
CPPcheck
CPPcheck is one of the most popular static analysis tools for C++. It checks for various issues ranging from simple stylistic requirements to more complex logical errors.
- Installation Process: CPPcheck can be installed via package managers on Linux or downloaded from its official website for Windows and macOS.
To check for errors in your code, you can run:
cppcheck --enable=all your_code.cpp
The output will provide you with insights into potential issues such as unused variables, out-of-bounds accesses, and other coding inefficiencies, ensuring that you're aware of problems before they turn into bugs.
Clang Static Analyzer
The Clang Static Analyzer is another robust tool in the C++ toolbox. It is particularly known for its powerful diagnostics and ease of integration with the LLVM compiler infrastructure.
To utilize the analyzer, you can incorporate it into your build process with:
scan-build make
This command compiles your code while generating a static analysis report that highlights potential problems like memory leaks and null pointer dereferences.
Valgrind
When it comes to runtime checking, Valgrind shines as a powerful tool for discovering memory-related errors. It’s particularly useful for debugging complex C++ applications where memory management is crucial.
By running:
valgrind ./your_executable
you will receive an output that highlights memory leaks, invalid read or write access, and misuse of pointers, allowing you to address these underlying issues effectively.
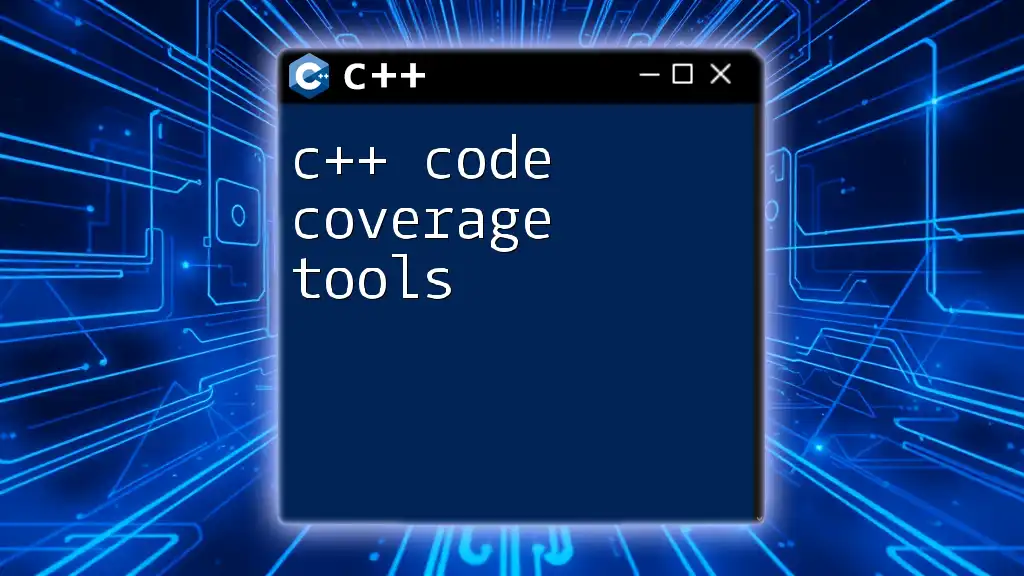
Integrating C++ Code Checkers into Your Workflow
CI/CD Integration
Integrating a C++ code checker into your Continuous Integration/Continuous Deployment (CI/CD) workflow is essential for ensuring ongoing code quality. Setting up code checkers during the CI process allows automatic analysis for every change made in the codebase.
Example integration using GitHub Actions might look like this:
name: C++ Code Checker
on: [push]
jobs:
cppcheck:
runs-on: ubuntu-latest
steps:
- name: Check out code
uses: actions/checkout@v2
- name: Install CPPcheck
run: sudo apt-get install cppcheck
- name: Run CPPcheck
run: cppcheck --enable=all src/
This configuration checks C++ code for errors every time changes are pushed to the repository.
Code Review Practices
Code checkers can significantly enhance team code reviews. Before a pull request is reviewed, running a code checker ensures that the code adheres to standards and is free of errors. This practice not only improves code quality but also fosters a learning environment where team members can understand common coding mistakes.
When conducting code reviews, highlight instances where the code checker flagged issues. This educates the team on best practices and drives home the importance of leveraging tools effectively.
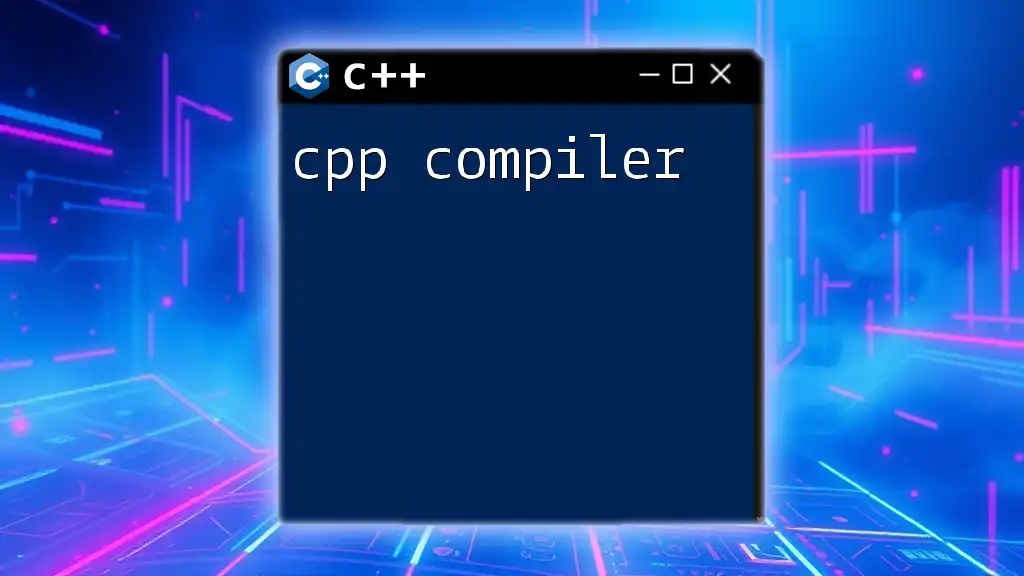
Common Challenges and How to Overcome Them
False Positives
One of the challenges with using any automated tool is the occurrence of false positives—instances where the tool flags code that is, in fact, correct. This can lead to unnecessary work being done to "fix" non-existent issues.
To mitigate false positives:
- Review the documentation of the tool to understand the common warnings it generates.
- Customize the tool's settings to focus on the most relevant checks for your project.
- Communicate with your team to ensure everyone understands which warnings are acceptable in certain contexts.
Tool Limitations
Each tool has its limitations, and it's essential to be aware that no single checker can cover all conceivable issues. For example, static code checkers may miss runtime errors, while dynamic tools are ineffective in discovering certain syntax errors.
Choosing the right tool involves understanding the specific needs of your project, including the complexity of the codebase and the types of issues you anticipate facing. Often, a combination of tools will yield the best results.
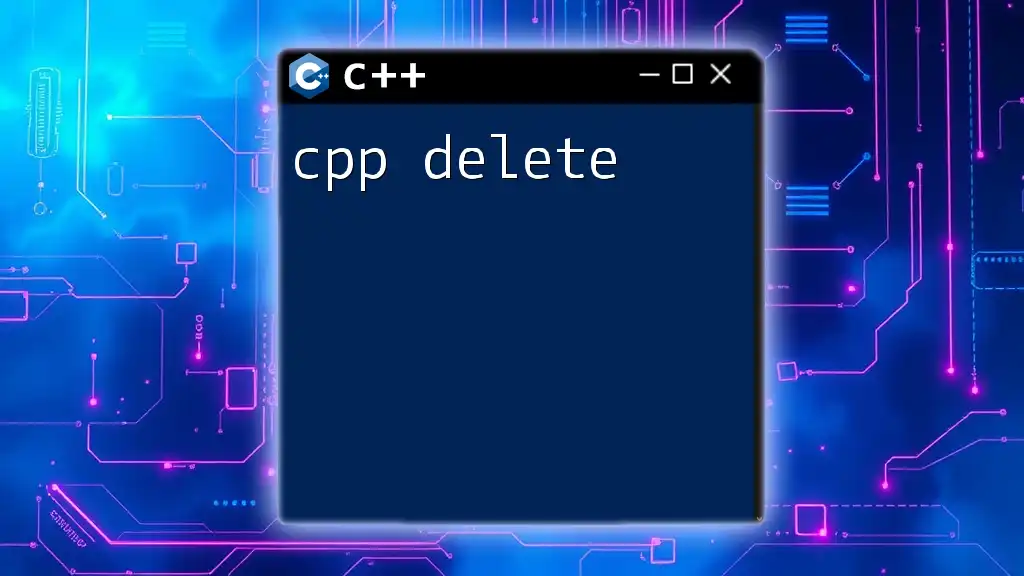
Conclusion
Incorporating a C++ code checker into your development workflow is not just about spotting issues—it's about fostering a culture of code quality and continuous improvement. By adopting best practices and leveraging various tools, you can significantly enhance the reliability and maintainability of your C++ projects. Start using a code checker today and take a step towards writing cleaner, more efficient code!
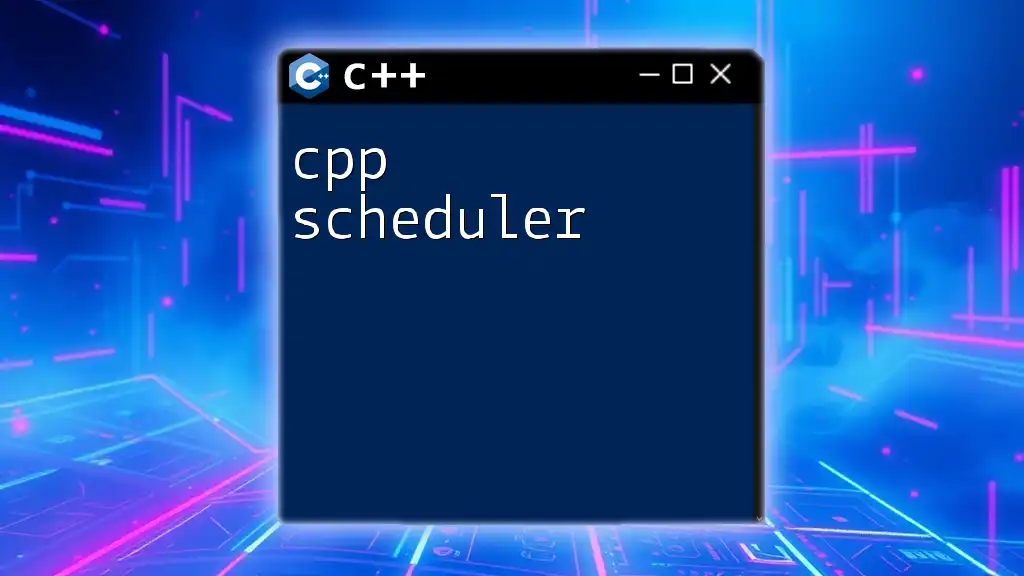
Additional Resources
For more in-depth knowledge and tools, consider exploring the official documentation of the mentioned tools, online courses focusing on C++ development, and forums for community-driven discussions. These resources will help reinforce your understanding and skill in using C++ effectively.