A CPP file reader is a program that reads contents from a file using C++ file handling techniques to demonstrate data input and processing.
Here's a simple example code snippet to read from a file in C++:
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream file("example.txt");
std::string line;
if (file.is_open()) {
while (getline(file, line)) {
std::cout << line << '\n';
}
file.close();
} else {
std::cout << "Unable to open file";
}
return 0;
}
Understanding File Streams in C++
In C++, file reading is primarily handled through the concept of file streams. File streams are objects that facilitate the reading and writing of files. In C++, there are three primary types of file streams that you need to be familiar with:
- `ifstream` (Input File Stream) is used for reading files.
- `ofstream` (Output File Stream) is used for writing to files.
- `fstream` (File Stream) can be used for both reading and writing.
These streams form the backbone of C++ file handling and allow the programmer to perform various operations on files seamlessly. Understanding how these streams work involves concepts of streams and buffers, where data flows in and out of the program through an abstraction provided by these classes.
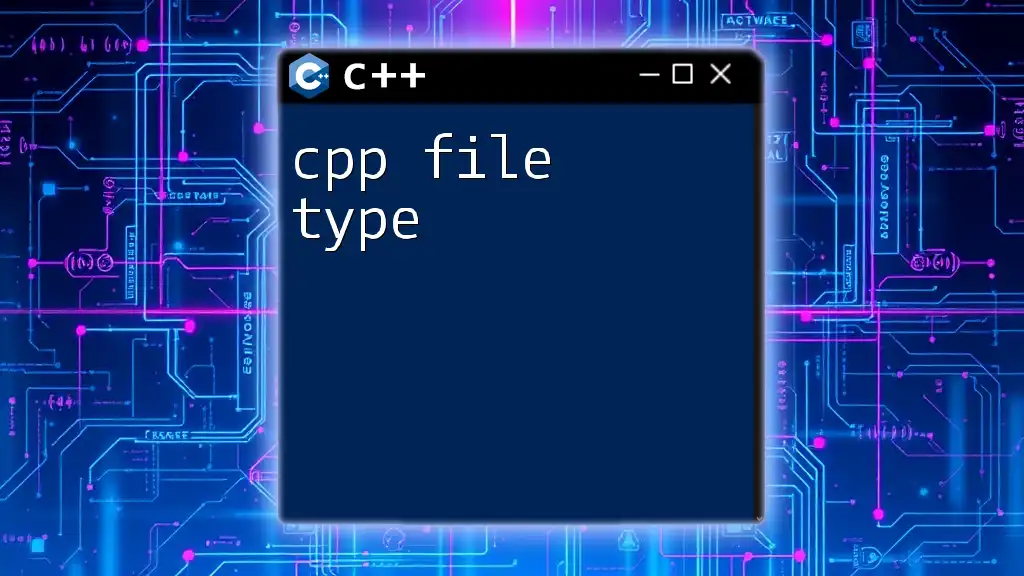
Setting Up Your Development Environment
Before diving into coding, it’s essential to have the right tools at your disposal. You will need a C++ compiler and an Integrated Development Environment (IDE) to write and test your code. Popular options include Visual Studio, Code::Blocks, or lightweight editors like Visual Studio Code.
To write your first C++ program to read a file, ensure you've included the necessary C++ libraries, particularly `<fstream>`, which contains the functionality required for file handling.
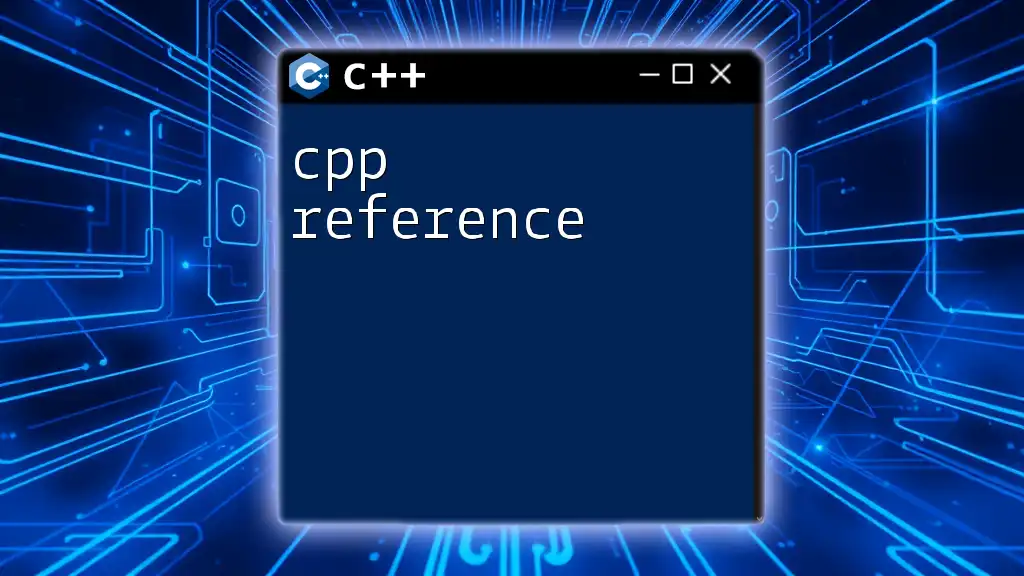
Basic File Reading in C++
When working with a C++ file reader, the first step is to include the necessary headers at the beginning of your program:
#include <iostream>
#include <fstream>
#include <string>
Then, you'll open a file for reading:
std::ifstream file("filename.txt");
By default, files are opened in read mode, and if the specified file does not exist, it will result in an error.
Handling Opening Errors
Error handling is crucial in file operations. You should always check if the file has successfully opened:
if (!file) {
std::cerr << "Error opening file!" << std::endl;
return 1; // Exit the program
}
Checking for errors immediately after attempting to open the file helps prevent undefined behavior in your program.
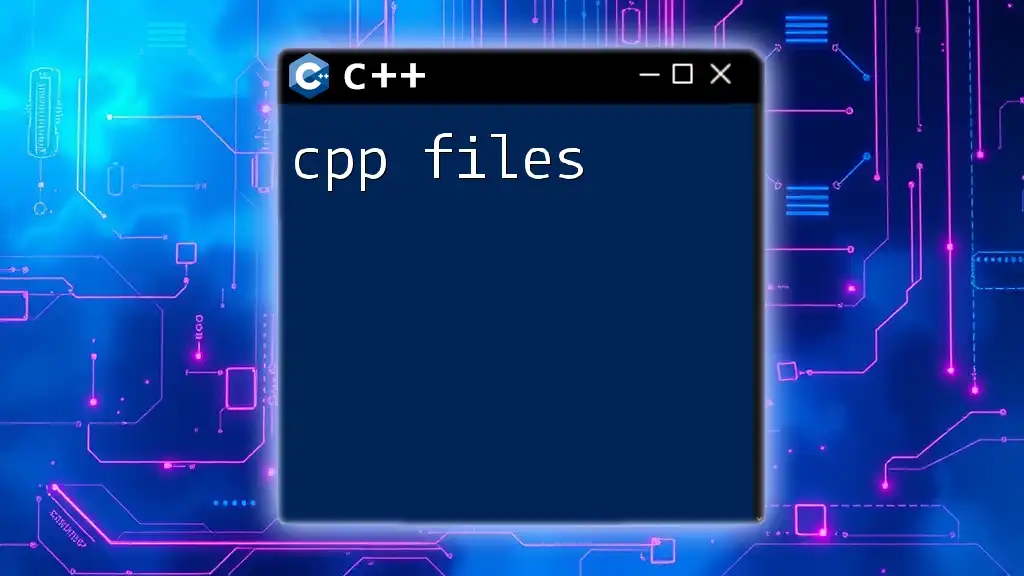
Reading Data From a File
Now that you know how to open a file, let’s read its contents. You can read a file either line by line or word by word.
Reading Line by Line
To read a file line by line, you can use the `getline()` function. Here’s a straightforward approach to read lines from a file:
std::string line;
while (getline(file, line)) {
std::cout << line << std::endl;
}
This loop continues until all lines in the file have been read. It’s effective for processing larger text and allows you to handle each line independently.
Reading Word by Word
If your goal is to read the file word by word, you can use the extraction operator (`>>`). This operator reads formatted data, making it ideal for parsing words. Here’s how you can achieve that:
std::string word;
while (file >> word) {
std::cout << word << std::endl;
}
This method works well for files containing space-separated values and allows you to easily extract individual words.
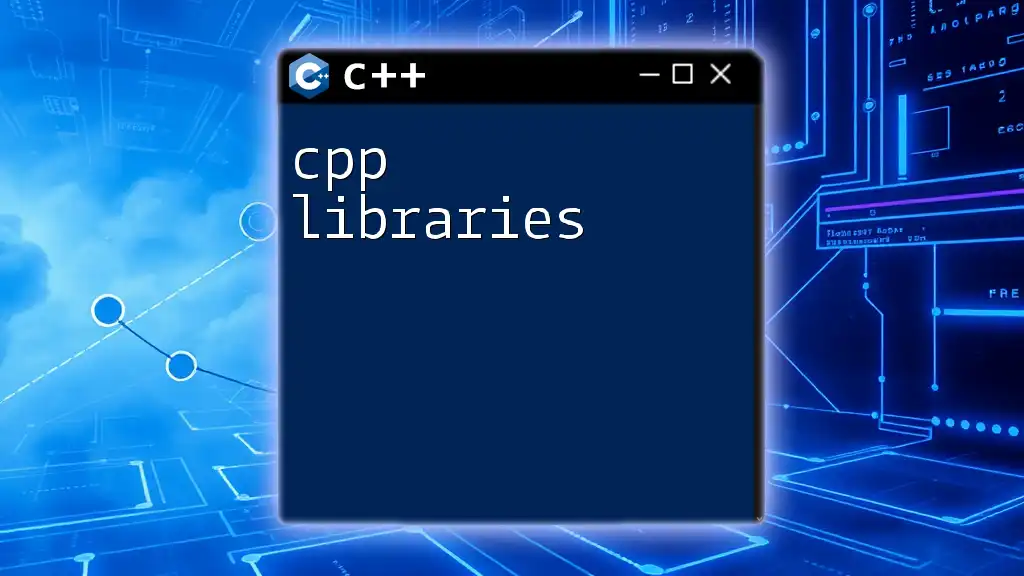
Reading Different Data Types from a File
Not all files contain textual data. Sometimes, you'll need to read numeric values or other data types from a file. C++ makes it effortless to convert strings to other types, such as integers or floats.
Example: Reading Numbers
When dealing with files containing numbers, you can simply extract them just like words:
int number;
while (file >> number) {
std::cout << "Number: " << number << std::endl;
}
This method assumes that the file contains integers formatted in a way that C++ can interpret. Be cautious with data types and ensure that the file follows the expected format to prevent runtime errors.
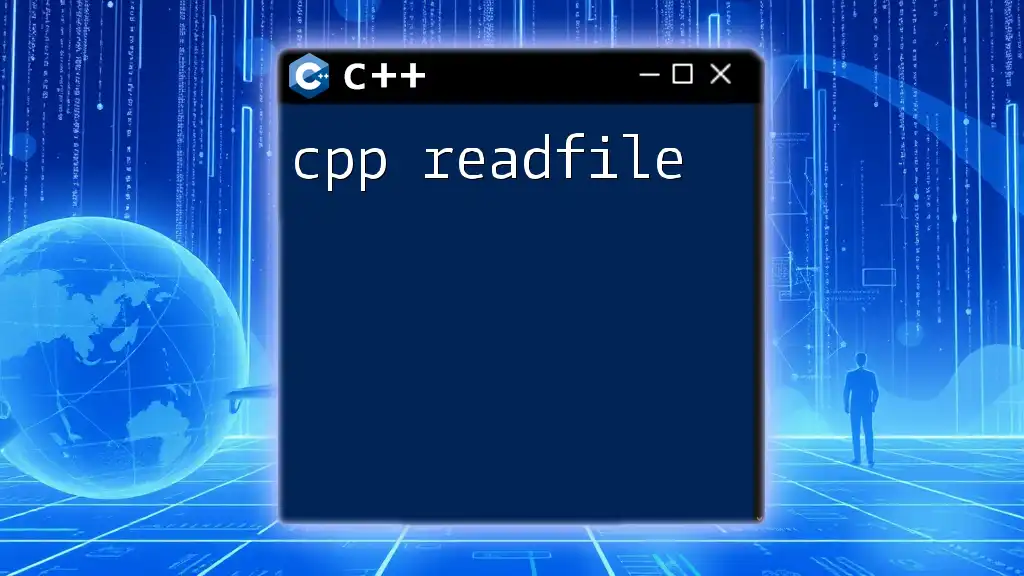
Practical Example: A Complete C++ File Reader Program
To consolidate your understanding, let’s put together a complete C++ program that demonstrates reading from a file. Below is an example that encompasses error handling, line-by-line reading, and displaying its contents.
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream file("data.txt");
if (!file) {
std::cerr << "Error opening file!" << std::endl;
return 1;
}
std::string line;
while (getline(file, line)) {
std::cout << line << std::endl;
}
file.close();
return 0;
}
In this code, you'll see that we check if the file opens successfully, read the file line by line, and print each line to the console. Finally, we ensure to close the file to prevent memory leaks.
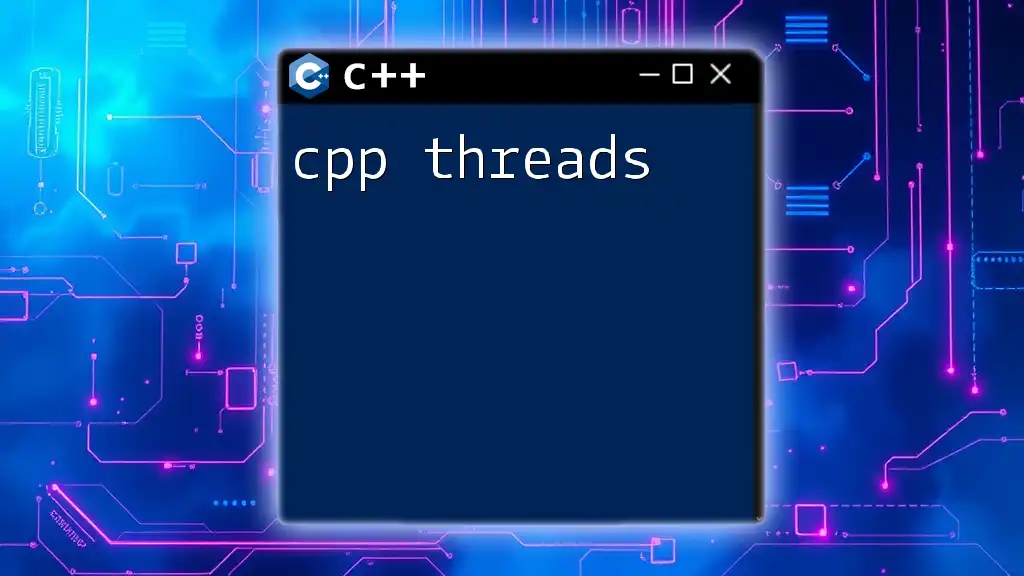
Closing the File
Closing a file is just as important as opening it. It releases the resources associated with that file. You can close a file as follows:
file.close();
This function ensures that all operations on the file are finalized before the program ends. It’s a good habit to always close your files, typically at the end of your reading or writing operations.
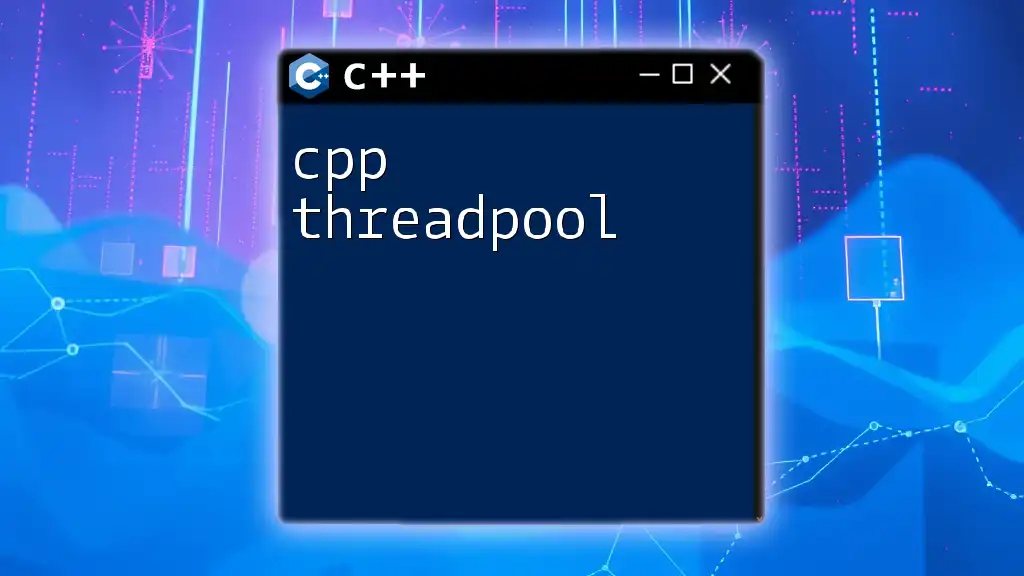
Error Handling in File Reading
While reading files, there are common errors that you can encounter. Examples include trying to read from a file that doesn’t exist, having permission issues, or even attempting to read from an empty file.
Using `try-catch` blocks can be an effective way to handle exceptions. Here’s an example of implementing error handling:
try {
// Code to open and read the file
} catch (const std::exception& e) {
std::cerr << "Exception: " << e.what() << std::endl;
}
This approach allows your program to handle unexpected behaviors gracefully and provides clear feedback about what went wrong.
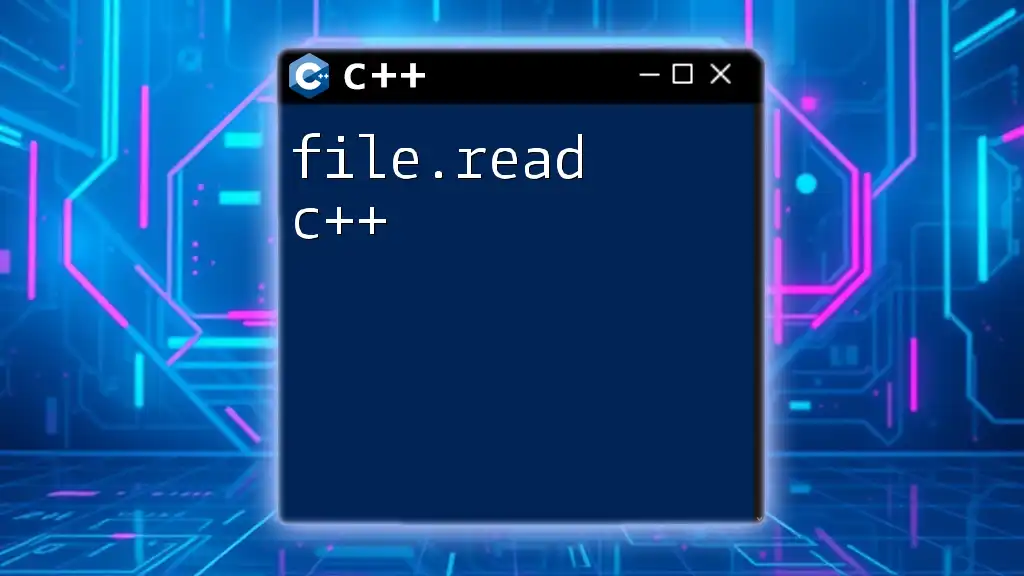
Conclusion
In this guide, we explored the realm of file handling in C++, focusing on the essential concepts necessary for building a competent C++ file reader. We covered how to open files, read their contents line by line or by words, handle different data types, and implement robust error handling.
With practice and experimentation, you can harness the full potential of C++ file I/O operations to enhance your programming capabilities. Don't hesitate to apply these techniques in your projects, and continue learning to perfect your skills in file management!