A C++ CSV reader parses Comma-Separated Values (CSV) files, allowing users to extract data from the file in a structured manner. Here’s a simple example of a C++ CSV reader:
#include <iostream>
#include <fstream>
#include <sstream>
#include <vector>
void readCSV(const std::string& filename) {
std::ifstream file(filename);
std::string line;
while (std::getline(file, line)) {
std::stringstream ss(line);
std::string item;
std::vector<std::string> row;
while (std::getline(ss, item, ',')) {
row.push_back(item);
}
for (const auto& cell : row) {
std::cout << cell << " ";
}
std::cout << std::endl;
}
}
int main() {
readCSV("data.csv");
return 0;
}
What is a CSV File?
CSV stands for Comma-Separated Values. It is a widely-used format for storing tabular data in plain text, where each line corresponds to a record, and each field within the record is separated by a comma (or other delimiters such as semicolons). The basic structure consists of rows and columns where:
- Rows represent individual records.
- Columns represent attributes or fields of the data.
The simplicity of the CSV format makes it popular for data exchange between different applications, such as databases, spreadsheets, and statistical software. Here are some common use cases for CSV files:
- Data Transfer: CSV files are frequently used to transfer data between applications that may not use the same database formats.
- Data Storage: CSV files can serve as a lightweight database, especially for small datasets or for exporting data from larger systems.
- Data Analysis: Many data analysis and machine learning libraries can directly read CSV formatted data, making it easy to gather and manipulate large sets of information.
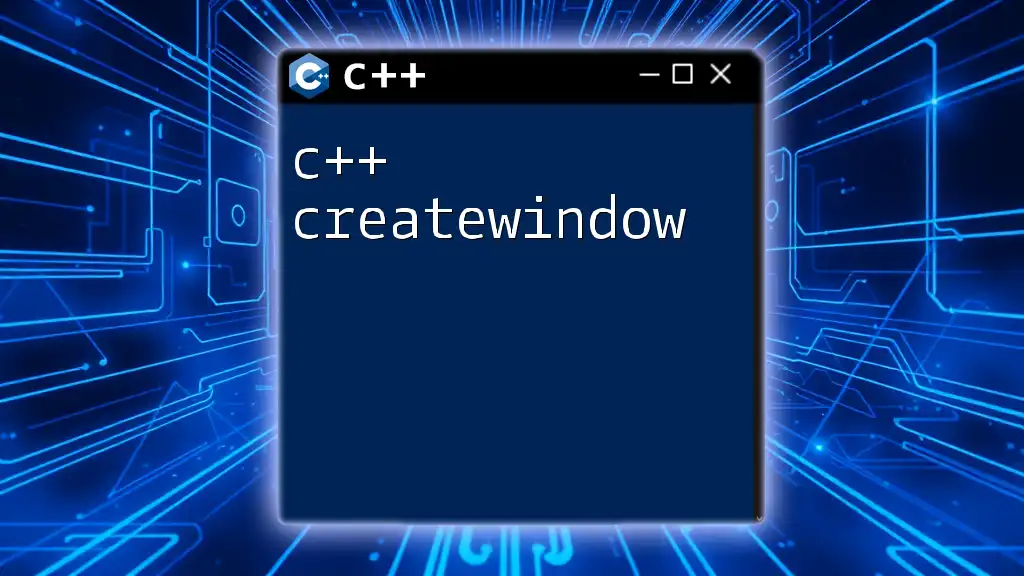
Understanding the C++ CSV Reader
A C++ CSV reader is designed to read CSV files in a structured manner, extracting data from them so that they can be processed or analyzed. Unlike a generic CSV parser, which may provide functionalities for altering or manipulating data, a C++ CSV reader primarily focuses on reading data efficiently and effectively.
Libraries for Reading CSV in C++
When it comes to reading CSV files in C++, you can choose between using the Standard Library or third-party libraries. Each option offers unique benefits and drawbacks:
- Standard Library: Provides a lightweight and native way to read files. However, it requires more manual effort to parse the data.
- Third-party Libraries: Offer pre-built functions and capabilities, easing the process of reading and parsing data from CSV files. However, they may require additional setup and learning.
Popular Libraries
Boost: The Boost Library is one of the most widely-used libraries in C++. It includes powerful tools for file and data manipulation, including reading CSV files.
To implement a C++ CSV reader using Boost, you can install the Boost libraries and include necessary headers. An example code snippet for reading a CSV file using Boost is as follows:
#include <boost/tokenizer.hpp>
#include <fstream>
#include <iostream>
#include <string>
#include <vector>
void readCSV(const std::string &filename) {
std::ifstream file(filename);
if (!file.is_open()) {
std::cerr << "Error: Could not open the file!" << std::endl;
return;
}
std::string line;
while (std::getline(file, line)) {
boost::tokenizer<boost::escaped_list_separator<char>> tok(line);
std::vector<std::string> fields(tok.begin(), tok.end());
for (const auto &field : fields) {
std::cout << field << " ";
}
std::cout << std::endl; // End of line
}
file.close();
}
libcsv: Another excellent choice for reading CSV files in C++ is libcsv, a lightweight, fast CSV parser. The basic operations in libcsv can handle complex CSV formats, inclusively managing quoted fields.
To use libcsv, you need to include it in your project. Here is a code snippet that showcases how to read a CSV file:
#include <stdio.h>
#include <csv.h>
void readCSV(const std::string &filename) {
struct csv_parser p;
csv_init(&p, 0);
FILE *file = fopen(filename.c_str(), "r");
if (file == NULL) {
std::cerr << "Couldn't open the file." << std::endl;
return;
}
char buf[1024];
size_t bytes_read;
while ((bytes_read = fread(buf, 1, sizeof(buf), file)) > 0) {
csv_parse(&p, buf, bytes_read, NULL, NULL, NULL);
}
csv_fini(&p, NULL, NULL, NULL);
csv_free(&p);
fclose(file);
}
csv-parser: For those looking for a more user-friendly, modern approach, the csv-parser library offers a powerful and easy-to-use interface for reading CSV files.
Here is a quick example of using csv-parser:
#include "csv.hpp"
void readCSV(const std::string &filename) {
csv::Parser file(filename);
for (int i = 0; i < file.rowCount(); ++i) {
for (int j = 0; j < file.columnCount(); ++j) {
std::cout << file[i][j] << " ";
}
std::cout << std::endl;
}
}
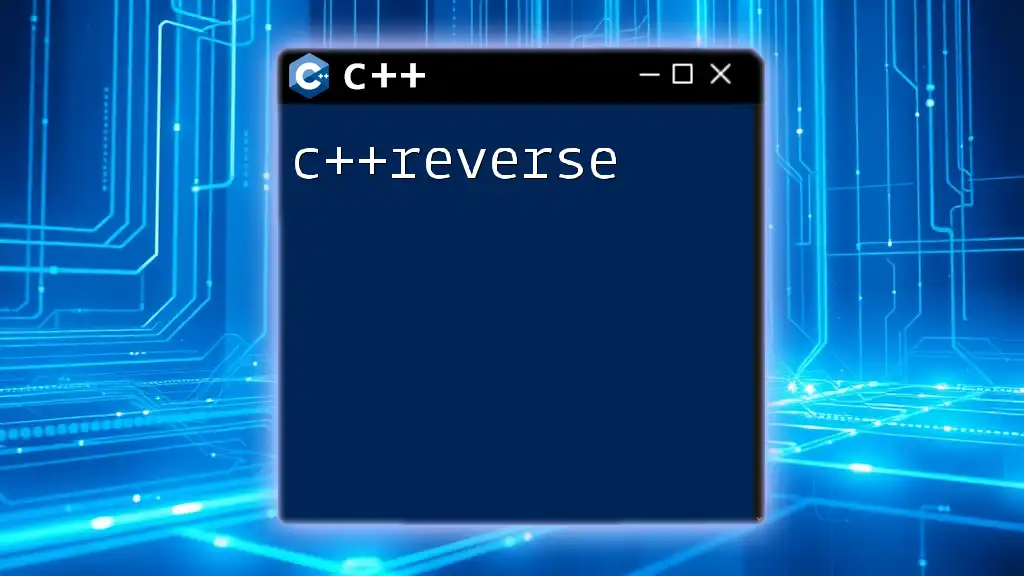
Building a Simple C++ CSV Reader
Now that we have an understanding of libraries available for reading CSV files, let’s build a simple C++ CSV reader using the C++ standard library.
Opening a CSV File
Start by creating a new C++ project. You will need to include the `<fstream>`, `<iostream>`, and `<sstream>` headers for file handling and string manipulation.
Begin by opening a CSV file for reading:
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream file("data.csv");
if (!file.is_open()) {
std::cerr << "Error: Could not open the file!" << std::endl;
return 1;
}
// Further code goes here.
}
Reading Lines from the File
Once the file is successfully opened, the next step is to read the lines from the file in a loop. Use `std::getline` to read each line individually:
std::string line;
while (std::getline(file, line)) {
// Process each line
}
Parsing CSV Data
To transform the lines into individual fields, we can split them by commas. The following function utilizes `std::stringstream` to achieve this:
#include <sstream>
#include <vector>
std::vector<std::string> parseLine(const std::string &line) {
std::vector<std::string> fields;
std::stringstream ss(line);
std::string field;
while (std::getline(ss, field, ',')) {
fields.push_back(field);
}
return fields;
}
You can then integrate this function within your main loop to process each line accordingly.
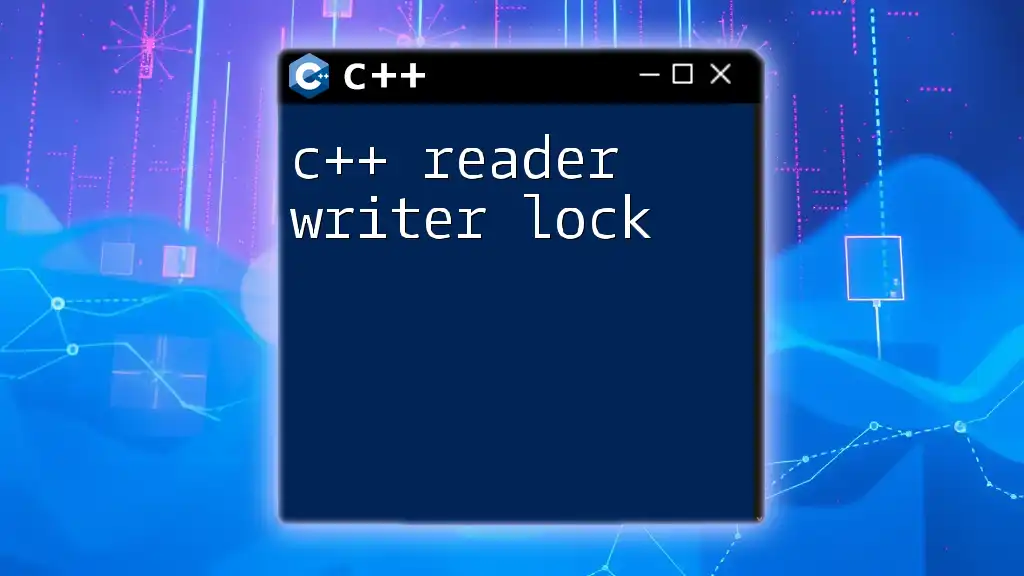
Error Handling in C++ CSV Reader
Robust error handling is essential in any application handling file I/O operations. Common scenarios include files that cannot be opened or existing data that does not conform to the expected CSV format.
To illustrate error handling, consider the following example that gracefully handles these situations:
if (!file) {
std::cerr << "Error: Could not read the file." << std::endl;
}
Adding checks when parsing data can also help to catch formatting errors, ensuring you can inform the user if the CSV data is inconsistent or malformed.
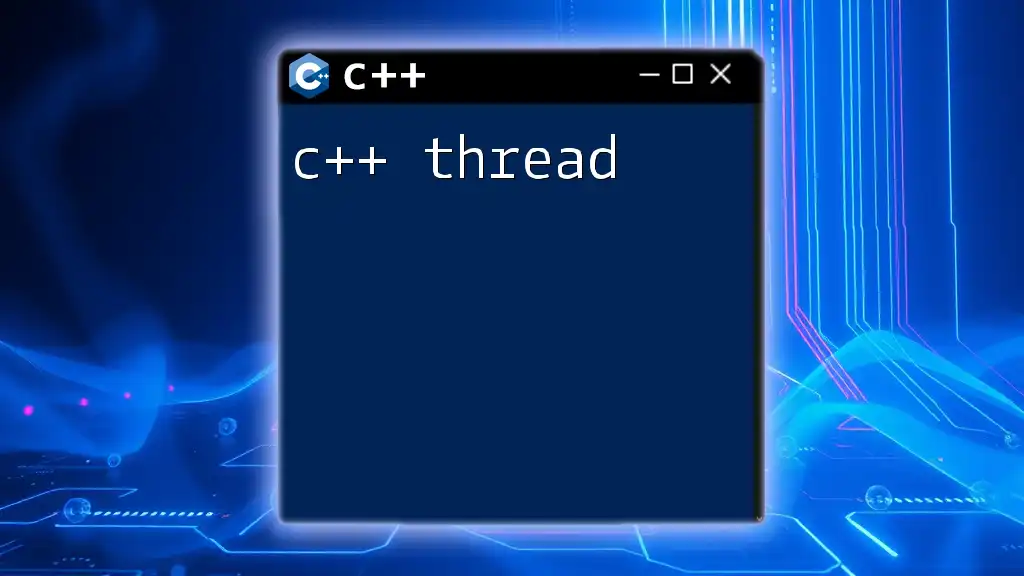
Testing Your C++ CSV Reader
It’s vital to test your C++ CSV reader with various sample CSV files to ensure robustness. Create test files with diverse data sets, including:
- Missing Values: Some rows don’t contain data for certain fields.
- Varying Column Counts: Examples where not all rows have the same number of fields.
Testing will help you debug and refine your reader, making it more resilient for varied data.
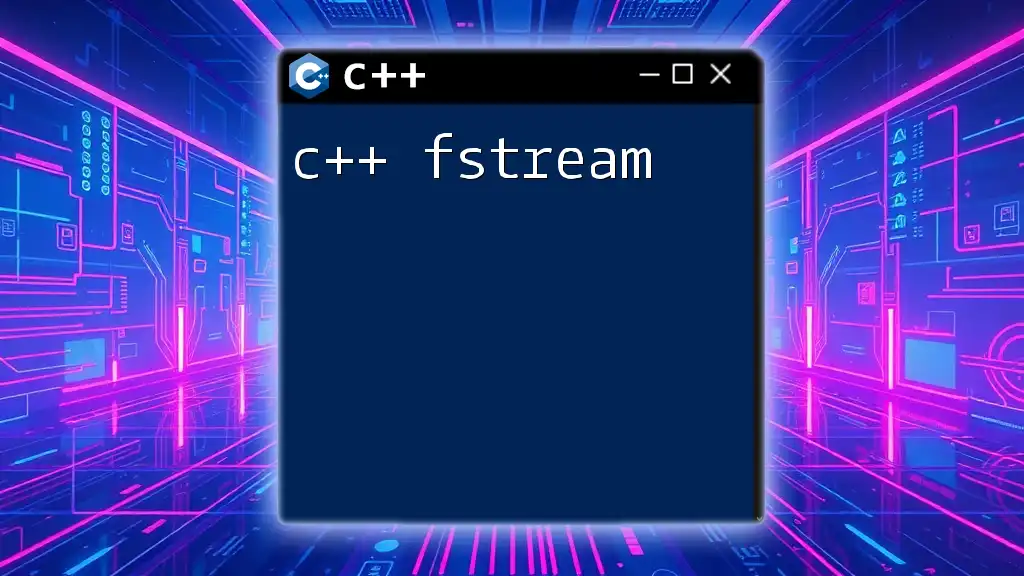
Conclusion
In this comprehensive guide, you have learned how to create a C++ CSV reader from scratch. We covered the fundamentals of CSV files, explored libraries for reading CSV data, and provided clear code examples to help you build your own reader. With this knowledge, you can confidently handle CSV files in your C++ applications.
To enhance your skills further, delve into more complex CSV manipulation techniques, and don't hesitate to explore different libraries that can assist with more advanced parsing operations.
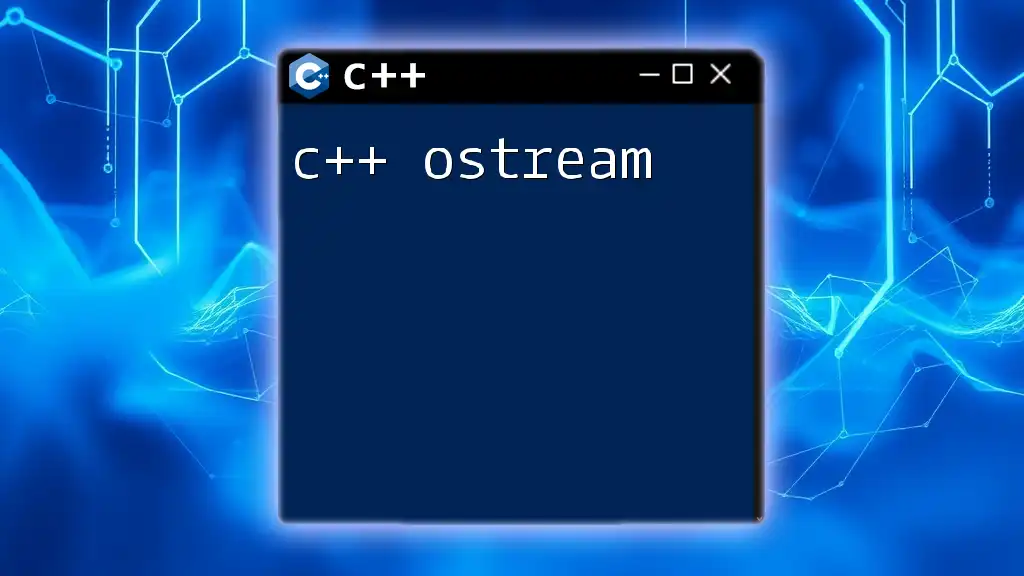
Call to Action
Share your experiences and insights on handling CSV files in C++. If you have questions or topics you want to explore further, feel free to leave a comment! Also, subscribe to our updates for more concise and effective C++ command guides and tutorials.
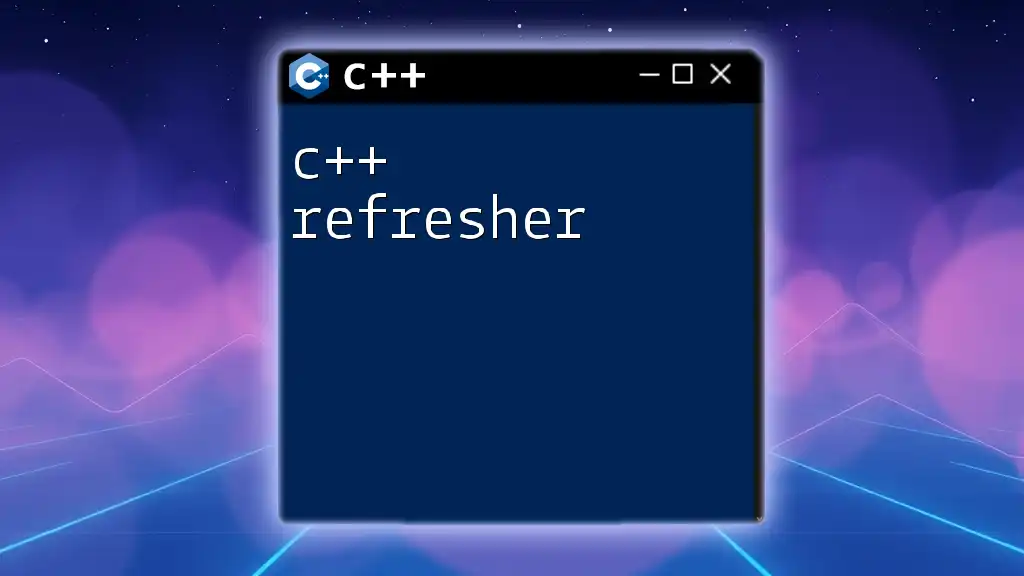
Additional Resources
For further reading, consider looking into books and websites focused on C++ and CSV handling techniques. Check out available GitHub repositories to find source code examples that can enhance your understanding and implementation of CSV processing in C++.