C++ threads allow you to perform concurrent execution in your applications, enabling multiple operations to run simultaneously for improved efficiency.
Here's a simple example of using C++ threads:
#include <iostream>
#include <thread>
void greet() {
std::cout << "Hello from the thread!" << std::endl;
}
int main() {
std::thread t(greet); // Create a thread that runs the greet function
t.join(); // Wait for the thread to finish
return 0;
}
What is Threading in C++?
Threading allows multiple threads to run concurrently within a single process, enabling the execution of tasks in parallel. This is essential for utilizing multi-core processor capabilities and improving application performance. Understanding threading in C++ is critical for developers who want to build responsive and efficient applications.
Difference Between Multi-threading and Single-threading
- Single-threading: In a single-threaded application, tasks are performed sequentially. This means that one task must complete before the next begins, which can lead to inefficiencies, especially with I/O operations or long-running computations.
- Multi-threading: In contrast, multi-threading allows multiple threads to run simultaneously, handling multiple tasks concurrently. This is particularly beneficial in I/O-bound applications, where one thread can wait for I/O operations while other threads continue executing.
Key Concepts
To grasp the concept of C++ threads, it’s important to distinguish between threads and processes. A thread is a lightweight subprocess that shares the same memory space with other threads within the same process, which allows for faster communication and data sharing.
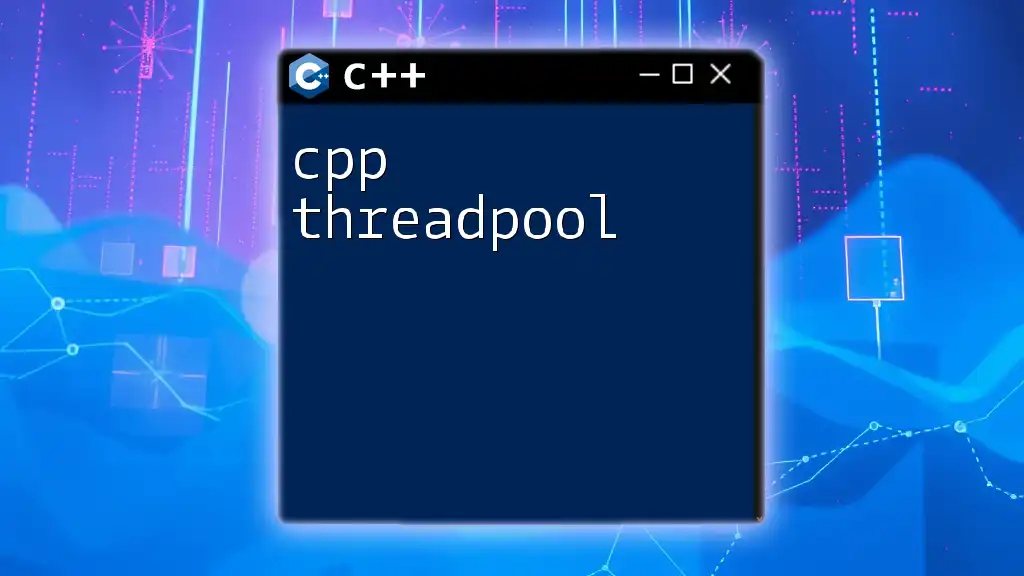
Creating Threads in C++
The C++11 thread library introduces powerful threading capabilities via the `<thread>` header.
Basic Thread Creation
With the `std::thread` class, threads can be created easily. When a thread is spawned, it can run a function or method.
Code Example: Creating a Simple Thread
#include <iostream>
#include <thread>
void sayHello() {
std::cout << "Hello from thread!" << std::endl;
}
int main() {
std::thread t(sayHello);
t.join(); // Wait for thread to finish
return 0;
}
In this example, the `sayHello` function is executed by a separate thread created in `main()`. The `join()` method ensures that the main thread waits for `t` to complete before exiting.
Passing Parameters to Threads
Threads can also accept parameters to be passed to the functions they execute.
Code Example: Passing Parameters to a Thread
void greet(std::string name) {
std::cout << "Hello, " << name << "!" << std::endl;
}
int main() {
std::thread t(greet, "Alice");
t.join();
return 0;
}
Here, the `greet` function takes a string parameter that is passed when creating the thread.
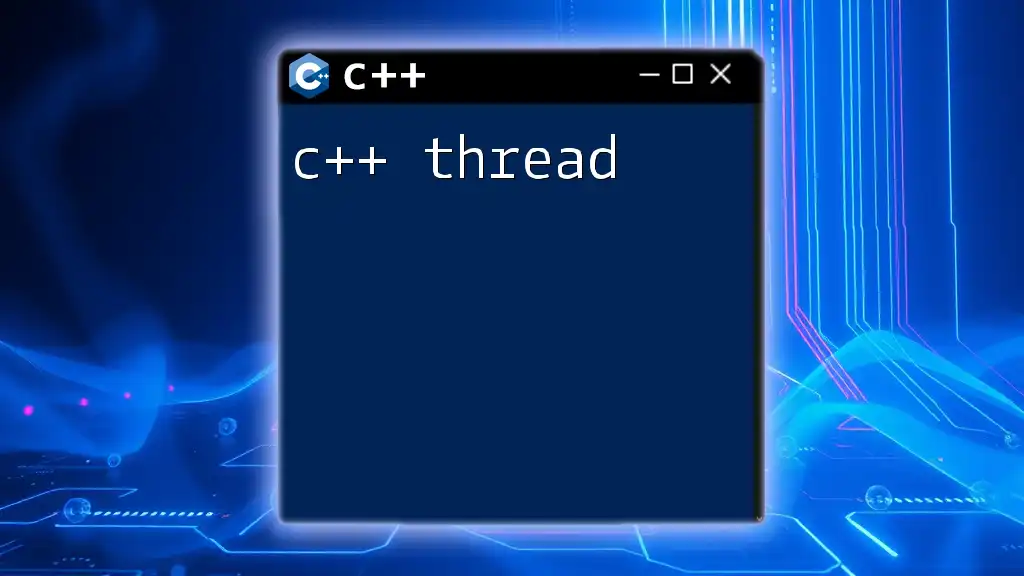
Managing Threads
Efficient management of threads is crucial for robust applications.
Joining vs Detaching Threads
Understanding when to join or detach threads is key to managing their lifecycle.
- Joining: The `join()` method blocks the calling thread until the thread identified by `std::thread` completes execution.
- Detaching: The `detach()` method allows the thread to execute independently. It’s essential to ensure that the thread’s resources are appropriately handled to avoid memory leaks or undefined behavior.
Code Example: Joining and Detaching Threads
std::thread t(sayHello);
t.detach(); // Thread runs independently
After calling `detach()`, the main thread does not wait for `t` to complete.
Checking Thread Status
To check whether a thread is still active, you can use `std::thread::joinable()`. This method returns `true` if the thread is joinable.
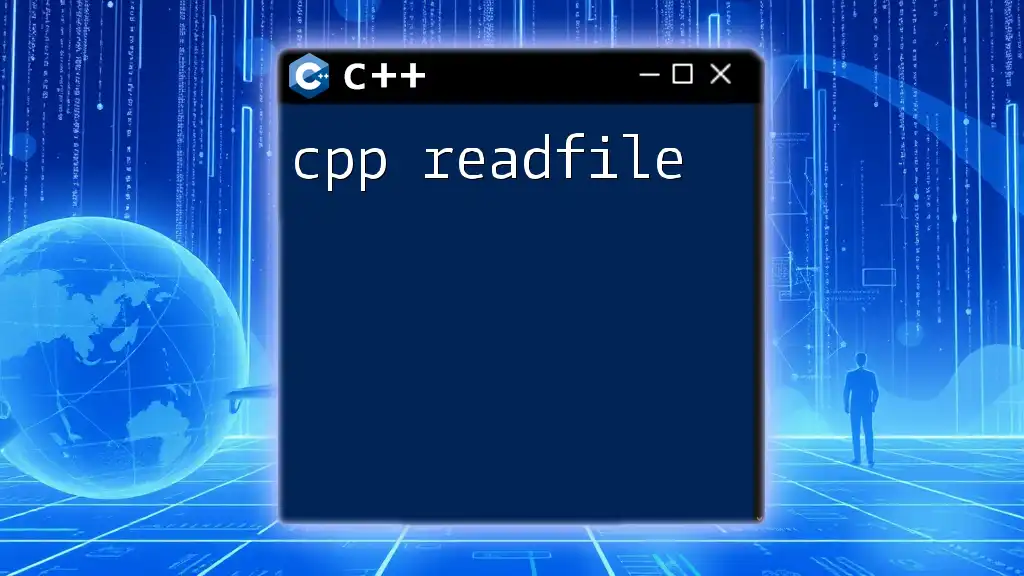
Thread Safety in C++
As multiple threads can potentially access shared resources simultaneously, thread safety becomes a crucial consideration.
Common Pitfalls
- Race Conditions: This occurs when two or more threads access shared data and try to change it at the same time. Typically, the last thread to finish may overwrite data, leading to inconsistent results.
- Deadlocks: This situation arises when two or more threads are waiting for each other to release resources, causing them all to be blocked indefinitely.
Using Mutexes for Synchronization
To prevent data races, synchronization mechanisms like mutexes can be employed. A mutex allows only one thread to access a resource at a time, providing a safeguard against concurrent modifications.
Code Example: Using a Mutex
#include <iostream>
#include <thread>
#include <mutex>
std::mutex mtx; // Mutex for critical section
void printHello() {
mtx.lock(); // Lock the mutex
std::cout << "Hello from thread!" << std::endl;
mtx.unlock(); // Unlock the mutex
}
In this example, the mutex protects the critical section where the thread is printing.
Using lock_guard for Automatic Mutex Management
Instead of manually locking and unlocking, it’s safer to use a `std::lock_guard`, which automatically manages the mutex's lifecycle.
Code Example: Using lock_guard
void printHello() {
std::lock_guard<std::mutex> lock(mtx); // Mutex automatically locked
std::cout << "Hello from thread!" << std::endl;
} // Mutex automatically unlocked when lock_guard goes out of scope
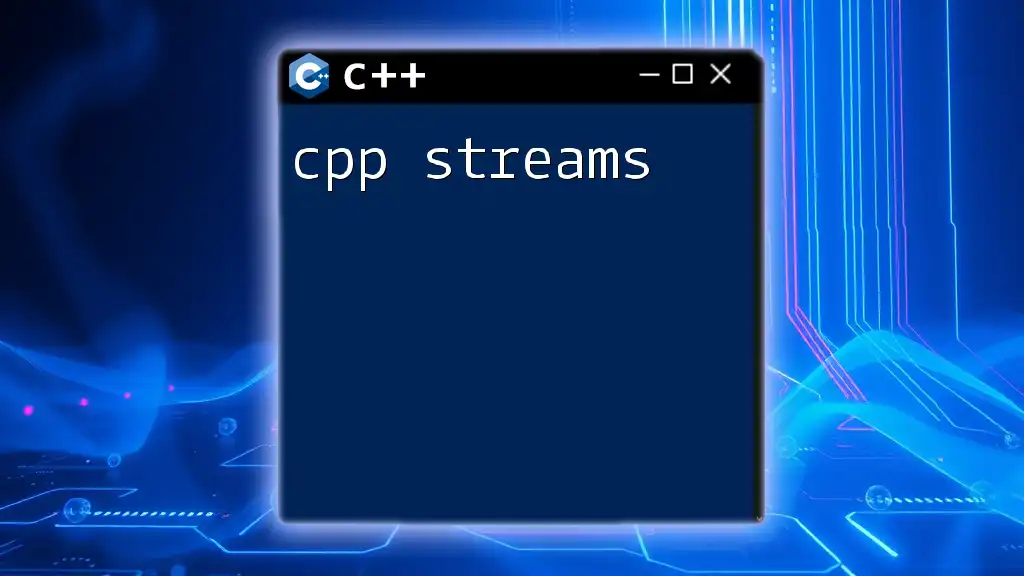
Advanced Threading Concepts
For more complex applications, advanced threading concepts can be utilized.
Thread Pools
Thread pools are collections of pre-instantiated threads ready to execute tasks. This can significantly reduce the overhead of thread creation and destruction, enhancing performance.
Future and Promise
To manage values computed asynchronously, `std::promise` and `std::future` can be used.
Code Example of Using std::Promise and std::Future
#include <future>
#include <iostream>
void calculate(std::promise<int>&& p) {
// Perform some calculations
p.set_value(123); // Set the value to pass to future
}
int main() {
std::promise<int> prom; // A promise
std::future<int> fut = prom.get_future(); // Get future from promise
std::thread t(calculate, std::move(prom));
std::cout << "Calculated value: " << fut.get() << std::endl; // Get value from future
t.join();
return 0;
}
This example shows how `std::promise` allows a thread to send a value back to the main thread via `std::future`.
Condition Variables
Using condition variables is another advanced technique for thread synchronization, allowing threads to block until a certain condition is met.
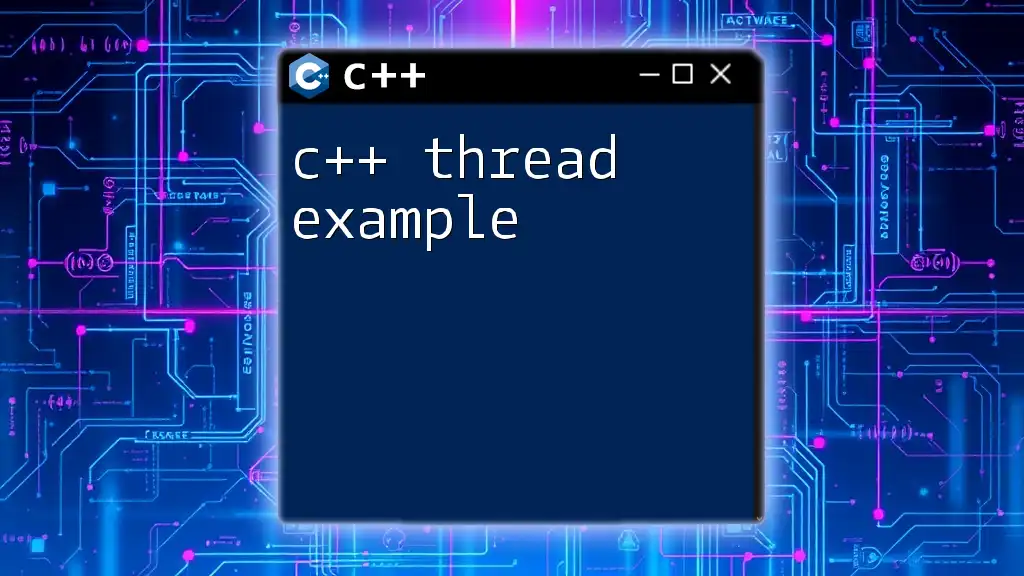
Example C++ Threading Applications
C++ threading can be applied to various real-world scenarios:
- Server Applications: Multi-threading allows a server to handle multiple client requests concurrently, improving responsiveness and throughput.
- Multicore Processing: CPU-intensive tasks can be divided across multiple threads, leveraging the power of multicore processors to reduce execution time.
- Real-World Applications: Projects such as game engines, data processing applications, or user interface frameworks frequently utilize threading to enhance performance and user experience.
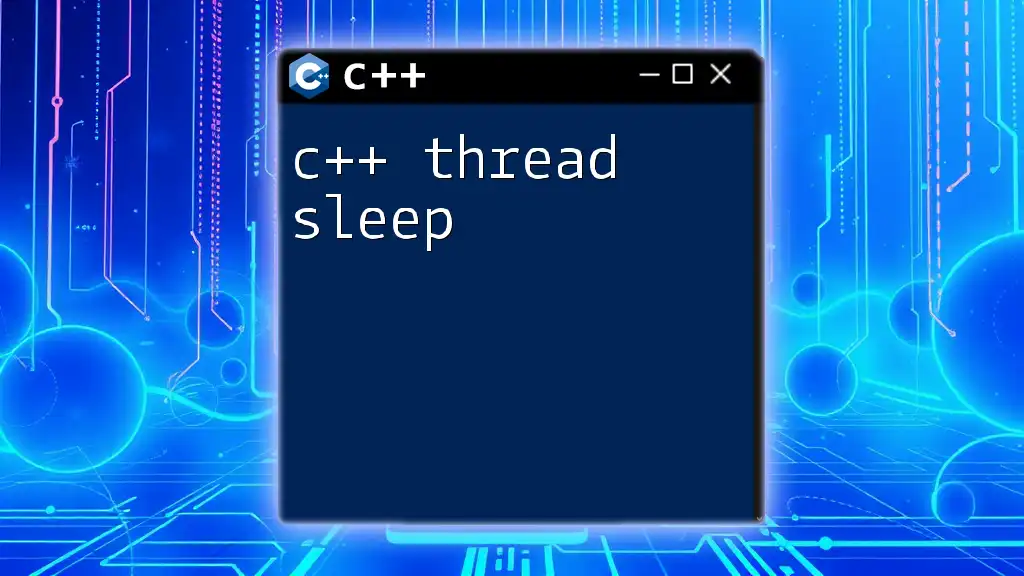
Conclusion
Understanding C++ threading is essential for developers aiming to create efficient, responsive applications that leverage the full power of modern hardware. By mastering the creation, management, and synchronization of threads, programmers can significantly enhance their application's performance. Experimenting with thread examples, practicing synchronization techniques, and learning from advanced concepts will prepare you to tackle threading challenges in your future projects.
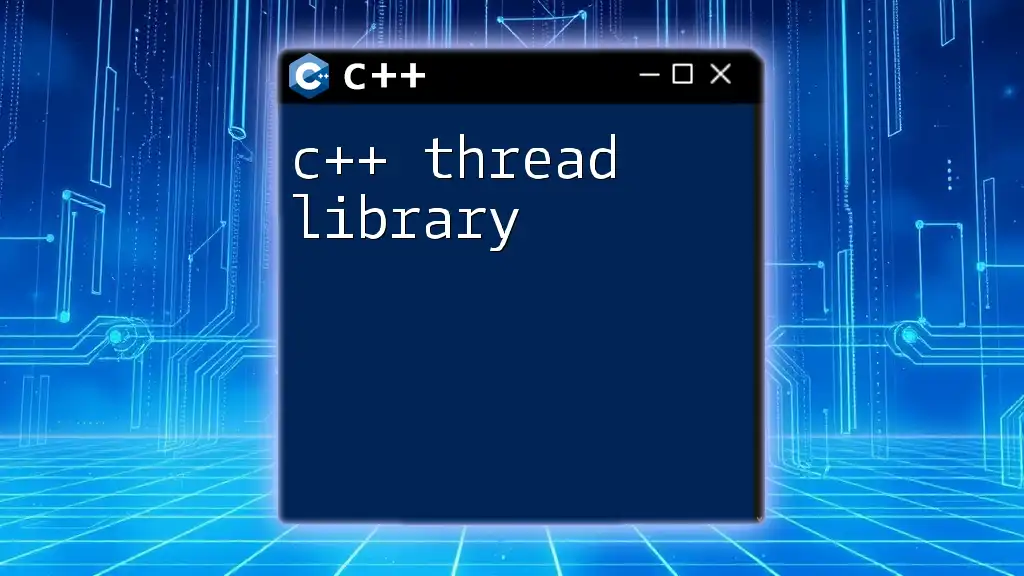
Additional Resources
For those keen to deepen their knowledge of threading in C++, recommended books, online tutorials, and community forums can serve as valuable resources for continued learning. Engaging with fellow developers will also provide insights and practical advice to enhance your threading skills.