A `.cpp` file is a source code file written in C++ that contains a combination of declarations, definitions, and executable code, compiled by a C++ compiler to create an executable program.
Here's an example of a simple C++ syntax in a `.cpp` file:
#include <iostream>
int main() {
std::cout << "Hello, world!" << std::endl;
return 0;
}
Understanding CPP Files
What is a CPP File?
A CPP file, denoted by the `.cpp` extension, is a source code file written in the C++ programming language. These files are crucial components of C++ programs where developers write the instructions that tell the computer what to do. The significance of the `.cpp` extension lies not only in its identification as a C++ source file but also in its compatibility with various compilers that process and execute the code.
Role of CPP Files in C++
CPP files play an essential role in C++ programming. They encapsulate the logic and functionality of applications. Often, CPP files are used in conjunction with header files (which have the `.h` or `.hpp` extension) that declare function prototypes and classes utilized in the CPP code. This separation aids in organizing code and ensuring that different parts of a program can be compiled independently.
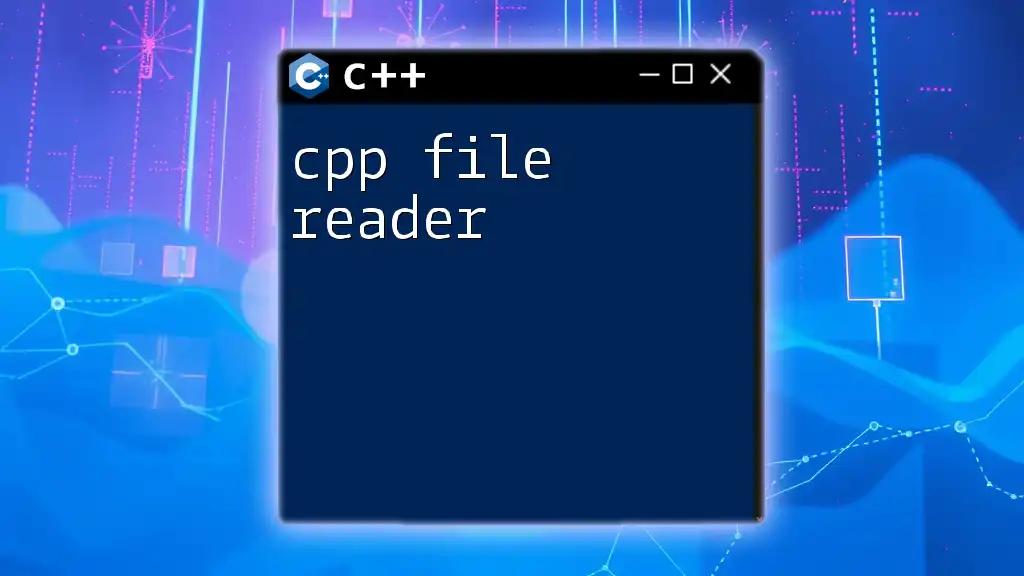
Structure of a CPP File
Basic Components of a CPP File
A typical CPP file comprises several key components that together form a functional C++ program.
-
Preprocessor Directives: These commands are processed before the actual compilation begins. The most common preprocessor directives include `#include` for importing libraries, and the `using namespace std;` directive which allows the use of elements from the standard C++ library without the `std::` prefix.
-
Function Definitions: Every C++ program must have a `main()` function where execution begins. Additional user-defined functions may also be included to modularize the code.
-
Variable Declarations: Variables of different data types must be declared and initialized before use. This includes fundamental data types such as `int`, `char`, `float`, and more.
Example CPP File Structure
To illustrate these components, consider the following example of a basic CPP file structure:
#include <iostream>
using namespace std; // Example of using a directive
int main() {
// Code execution starts here
cout << "Hello, World!" << endl; // Output example
return 0; // Return statement
}
In this example, the preprocessor directive `#include <iostream>` includes the iostream library which provides functionalities for input and output operations. The `main()` function serves as the entry point, and `cout` outputs the message "Hello, World!" to the console.

Working With CPP Files
Compiling CPP Files
The compilation process converts the human-readable C++ code into machine code that the computer can execute. To compile a CPP file, developers commonly use command-line tools such as `g++`, part of the GNU Compiler Collection.
For instance, to compile a CPP file named `myProgram.cpp`, the command would be as follows:
g++ myProgram.cpp -o myProgram
This command compiles `myProgram.cpp` and outputs an executable file named `myProgram`.
Running CPP Files
Once compiled, CPP programs can be executed. Using the terminal, one can run the program created from the previous step by entering:
./myProgram
Alternatively, when using an Integrated Development Environment (IDE) like Visual Studio or Code::Blocks, the IDE often provides built-in buttons to compile and run the CPP code, simplifying the process for the developer.

Organizing CPP Projects
File Naming Conventions
When engaging in C++ programming, it's essential to employ consistent naming conventions for clarity and maintainability. By adopting descriptive file names that represent the contents or functionalities—for example, using `bankAccount.cpp` for a file that manages bank transactions—you can make the codebase easier to navigate.
Directory Structure
Properly organizing your CPP projects enhances efficiency in development. A well-structured project often includes folders for:
- Source Files: This directory can house all of your CPP files.
- Header Files: Store associated header files with function declarations here.
- Assets: Any additional files like images, documentation, or configuration files can be kept in this directory.
A recommended structure might look like this:
/MyProject
/src
main.cpp
utils.cpp
/include
utils.h
/assets
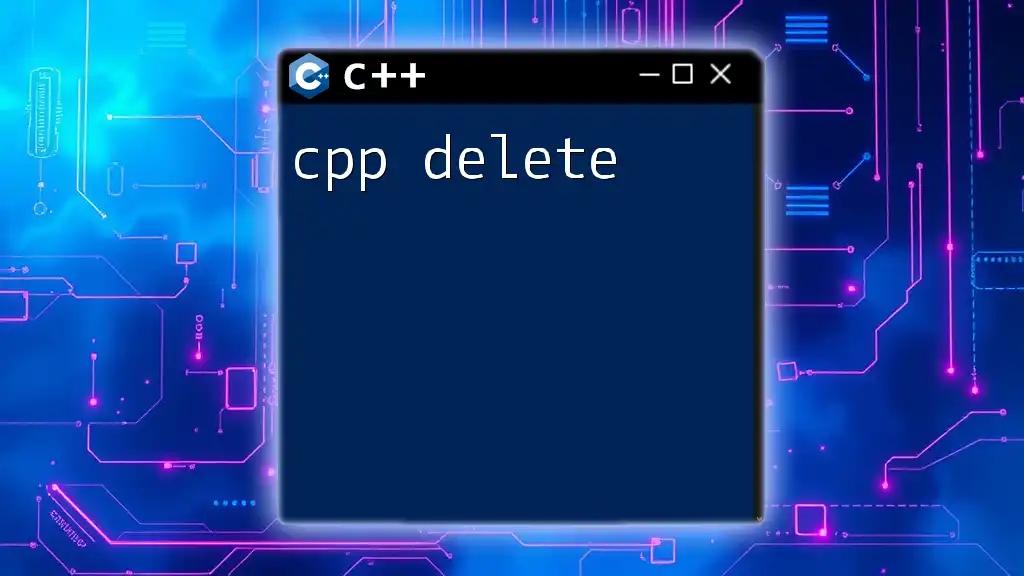
Advanced CPP File Concepts
CPP File vs. Header Files
CPP files differ fundamentally from header files. While CPP files contain the definitions of classes and functions, header files are used to declare these entities. This separation allows for improved organization and makes it easier to manage dependencies between files.
Using Multiple CPP Files
As projects scale, the organization of code into multiple CPP files becomes imperative. Using multiple source files can avoid overly complex files and enhance code reusability. For example, one might separate out utility functions into a dedicated `utils.cpp` file:
// main.cpp
#include "utils.cpp"
int main() {
greet(); // Call a function defined in utils.cpp
return 0;
}
// utils.cpp
#include <iostream>
void greet() {
std::cout << "Welcome to our C++ program!" << std::endl;
}
In the example above, the `greet()` function, defined in `utils.cpp`, is used in the `main.cpp` file, enabling a modular approach to coding.
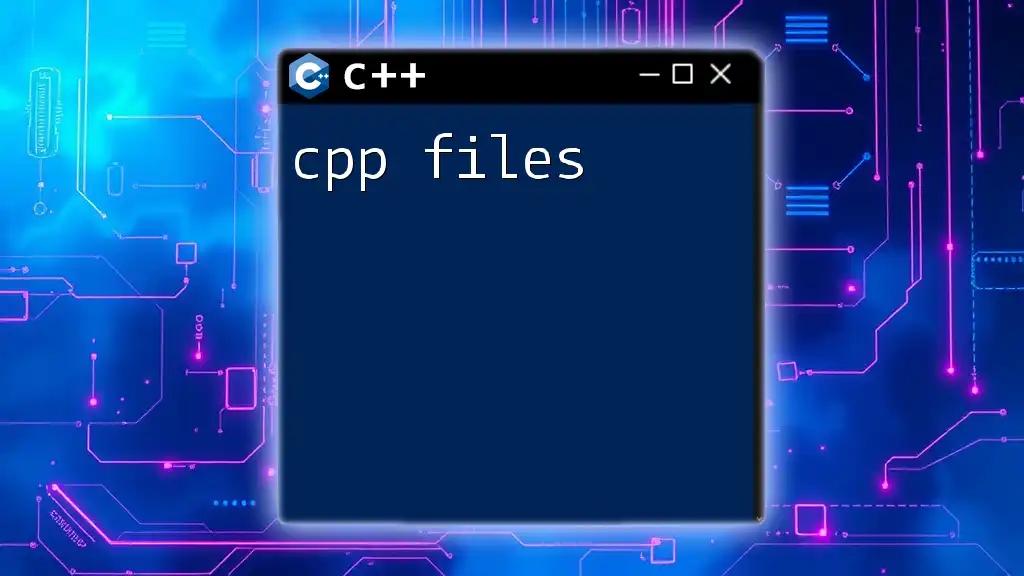
Best Practices for Working with CPP Files
Code Comments and Documentation
In C++ programming, clear documentation through code comments can significantly improve future readability and maintainability. Developers should utilize comments to explain complex segments of code, outline function purposes, and provide abridged descriptions of logical flows.
Error Handling
Common errors in CPP files can arise from syntax mistakes, type mismatches, or logical inconsistencies. To identify and resolve these issues, developers should employ effective debugging techniques, such as using built-in debugger tools in IDEs or inserting print statements throughout the code to trace execution.
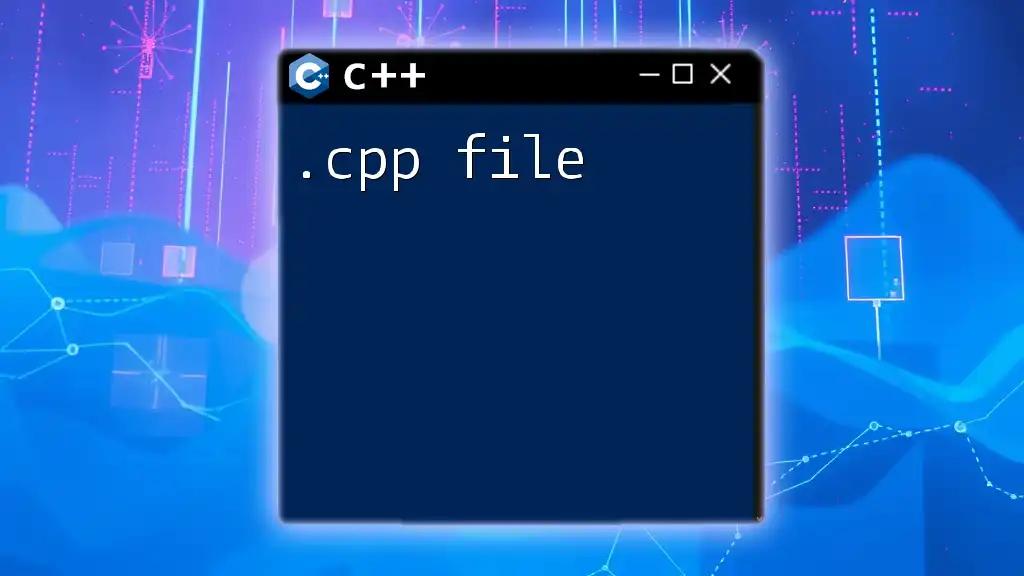
Common Questions About CPP Files
FAQs
-
What does a .cpp file contain?
A `.cpp` file typically contains C++ code, which includes variable declarations, function definitions, and user-defined classes. -
Can CPP files include other CPP files?
Yes, CPP files can include other CPP files, but it's a common and better practice to include header files to avoid potential issues with multiple definitions. -
How do I change the file extension?
To change a file’s extension, simply rename the file in your operating system or IDE and modify it accordingly.
Troubleshooting Common Issues
In the development of C++ applications, encountering errors is inevitable. Understanding basic troubleshooting—including checking for missing semicolons, ensuring proper data type usage, and verifying function calls—can reduce frustration and expedite issue resolution.

Conclusion
In summary, CPP files are essential in C++ programming, encapsulating code that drives the functionality of software applications. By understanding the structure, organization, and best practices related to the CPP file type, developers can significantly enhance their coding efficiency and project clarity. Emphasizing the importance of continual learning and hands-on practice will pave the way for mastery in using CPP files effectively.

Additional Resources
Recommended Books and Online Courses
Consider exploring notable resources to deepen your understanding of C++ programming, including classic textbooks and online platforms offering structured courses.
Useful Websites and Community Forums
Community forums such as Stack Overflow and GitHub serve as excellent resources for collaboration, troubleshooting, and knowledge sharing among C++ practitioners.