The C++ debugger is a powerful tool that allows developers to inspect their code, set breakpoints, and step through execution to identify and fix issues efficiently.
Here's a simple example of using a debug command such as setting a breakpoint:
#include <iostream>
int main() {
int a = 5;
int b = 10;
// Set a breakpoint on the next line
int sum = a + b; // Use debugger to inspect 'a' and 'b' values before this line
std::cout << "Sum: " << sum << std::endl;
return 0;
}
What is a Debugger?
A debugger is a powerful tool in programming that allows developers to inspect and manipulate the execution of their code. It helps in identifying and resolving issues known as bugs, which can arise from syntax errors, logical errors, or unexpected behavior. The role of a debugger is crucial in software development, as it saves time and resources by enabling efficient troubleshooting.

Why Use a C++ Debugger?
Using a C++ debugger streamlines the debugging process, allowing developers to focus on fixing issues rather than searching for them. Here are a few reasons why employing a C++ debugger is beneficial:
-
Reduced Time on Bug Fixing: The ability to step through code and examine variable states can significantly decrease the time spent on identifying issues.
-
Improved Understanding: Debuggers provide insights into the program's flow and logic, enhancing your overall understanding of C++ and its nuances.
-
Practical Application: Consider a scenario where an application crashes due to a segmentation fault. A debugger allows you to pinpoint exactly where in the code this fault occurs, drastically simplifying the resolution process.
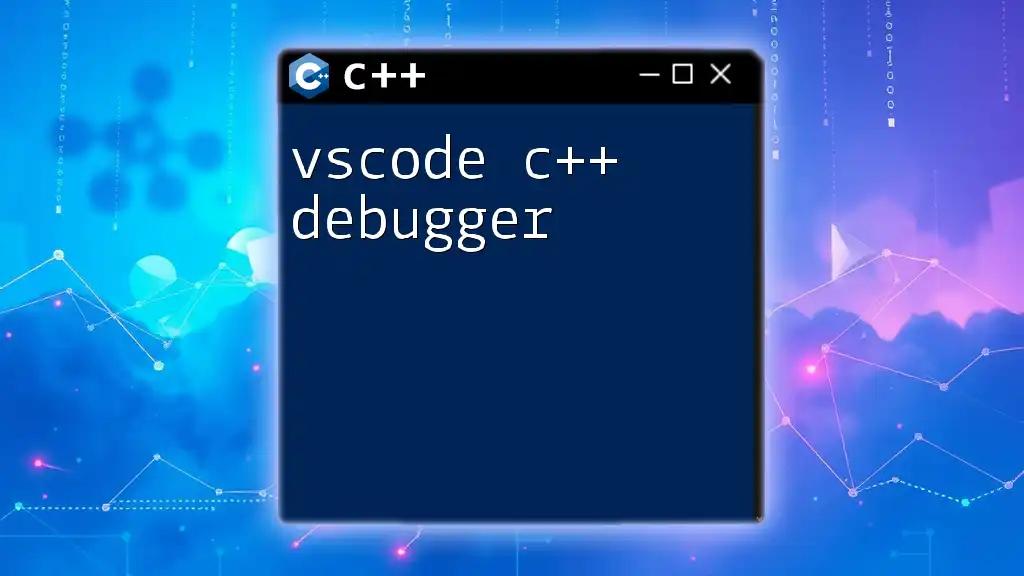
Understanding the Basics of Debugging
What Does Debugging Mean?
Debugging is the comprehensive process of identifying, isolating, and correcting bugs or errors in computer programs. It ensures that the code works as intended and produces the expected outcomes. Debugging is often viewed as an integral part of the development lifecycle, rather than a separate, burdensome task.
Steps in the Debugging Process
The debugging process generally consists of several key steps:
-
Reproducing the Bug: Determine how to trigger the bug by recreating the conditions under which it occurs.
-
Diagnosing the Issue: Utilize debugging tools to analyze the code and identify potential sources of the bug.
-
Fixing the Bug: Apply the necessary corrections to the code to resolve the identified issue.
-
Verifying Fixes: Test the application to ensure that the bug has been resolved and that no new issues were introduced.
A comprehensive understanding of these steps ultimately leads to a more systematic and less frustrating debugging experience.
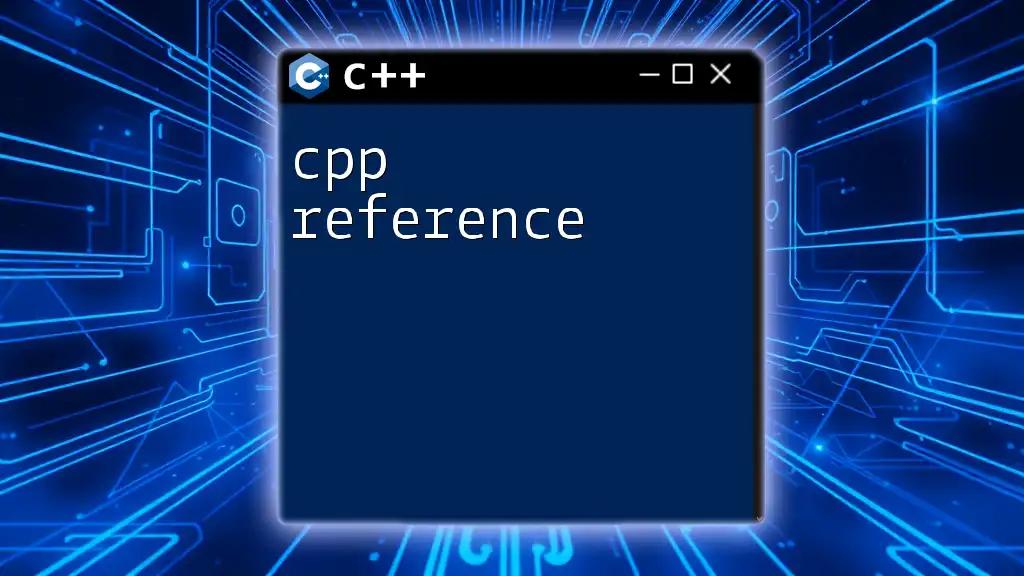
Types of C++ Debuggers
Command-Line Debuggers
Command-line debuggers, like GDB (GNU Debugger), are essential for developers who prefer a text-based approach to debugging. Below is a simple example demonstrating how to start debugging a basic C++ program using GDB:
g++ -g my_program.cpp -o my_program
gdb ./my_program
In this example, the `-g` option includes debugging information in the compiled output, making it easier to trace errors.
Graphical Debuggers
Graphical debuggers, such as Visual Studio, Code::Blocks, and Eclipse, offer a more user-friendly approach, featuring intuitive interfaces that make it easier to set breakpoints, examine variables, and view call stacks. The visual representation simplifies the debugging process, making it less overwhelming, especially for beginners.
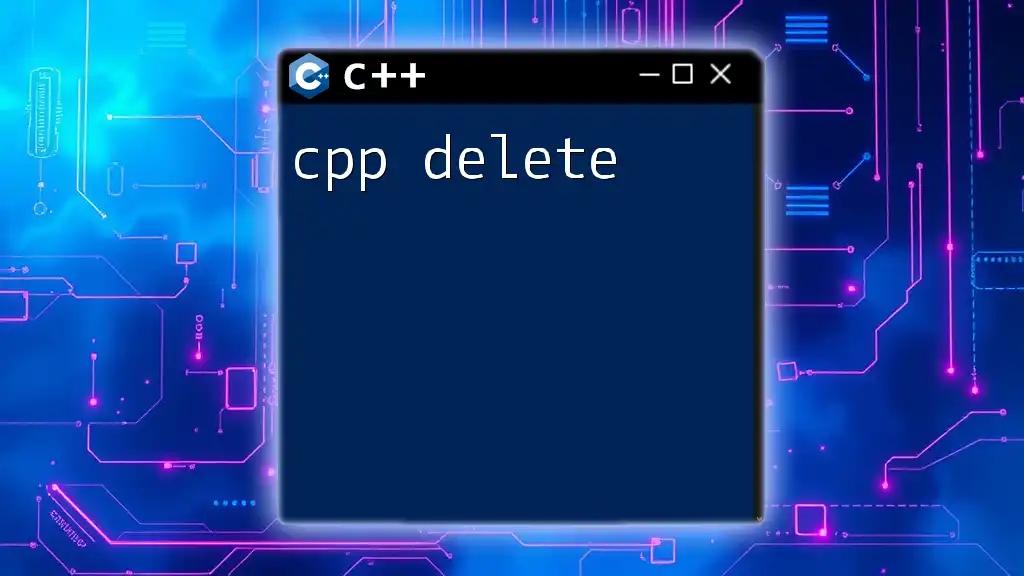
Key Features of C++ Debuggers
Breakpoints
Breakpoints are an essential feature of any C++ debugger, allowing developers to pause program execution at designated lines of code. By setting breakpoints, you can inspect variable states and program flow right before an error occurs. Here's a simple code snippet to visualize how breakpoints can be set in GDB:
#include <iostream>
void faultyFunction() {
int x = 10;
int y = 0;
std::cout << x / y << std::endl; // This will trigger a division by zero error
}
int main() {
faultyFunction();
return 0;
}
By placing a breakpoint at the line with `x / y`, you can evaluate the state of the program just before the crash.
Watch Variables
Watch variables are used to monitor specific variables throughout the execution of your program. They allow you to see how variables change over time and can be invaluable for tracking the source of issues. When debugging, you can add watch variables to inspect their values at any point during execution.
For example, if you wanted to track the value of `x` in the previous snippet, simply add a watch for `x`, and you'll receive updates every time `x` is modified.
Stepping Through Code
Stepping through code is a crucial feature that allows developers to execute their programs line by line. The main commands for stepping through code are:
- Step Over: Executes the next line of code but doesn't enter into function calls.
- Step Into: Executes the next line of code and enters into any function calls.
- Step Out: Completes the execution of the current function and returns to the calling function.
To demonstrate, if you step into the `faultyFunction` from the main function, the debugger will take you directly into its execution, allowing you to analyze calculations and flow.
Call Stack Examination
The call stack is a foundational concept in debugging. It represents the stack of function calls made by your program and can be viewed in most debugging tools. Understanding the call stack's structure can help you trace the flow of execution and identify where things might have gone wrong.
Consider a scenario with nested function calls. If an error occurs, inspecting the call stack helps determine the chain of events leading to the error. Each entry shows which function was called, in what order, and the arguments passed, thus enabling you to backtrack effectively.

Common Debugging Techniques in C++
Using Print Statements
Though not a replacement for dedicated debugging tools, using print statements can often provide quick feedback during the development process. Implementing simple output statements can help identify when and where certain conditions occur in your code.
Example:
int main() {
int total = 0;
for (int i = 0; i < 10; ++i) {
total += i;
std::cout << "Current total: " << total << std::endl; // Print statement for tracking
}
return 0;
}
Automated Testing and Debugging
Automated testing frameworks, such as Google Test, facilitate debugging through structured testing of your functions. By writing unit tests, you can verify each component of your software independently, making it easier to pinpoint where bugs might reside.
Incorporating automated tests complements the debugging process, ensuring that as you fix bugs, you do not inadvertently introduce new ones.

Best Practices for Debugging C++ Code
Code Organization and Readability
Writing clean, organized code is a fundamental practice for reducing debugging complexity. Clear code reduces cognitive load and enhances maintainability. To achieve this, consider:
- Maintaining consistent naming conventions.
- Structuring code with coherent indentation and spacing.
- Using comments to clarify complex logic.
Embracing the Debugging Process
Instead of viewing debugging as a chore, consider it an opportunity for growth. Each debugging session can deepen your understanding of C++ programming and system behavior. Famous developers often share insights about their debugging experiences, highlighting its role in their professional development.

Conclusion
The path to mastering the C++ debugger is paved with exploration and practice. Investing time into understanding debugging tools and strategies is invaluable for any programmer. Debugging isn’t just about fixing issues—it's about improving the quality of your code and becoming a more effective developer.

Further Resources for Aspiring Debuggers
To enhance your debugging skills, consider exploring online courses, forums, and tutorials specialized in C++ debugging. Engaging with community resources can broaden your understanding and offer new techniques.

Encourage Engagement
We invite you to share your debugging experiences and challenges. What techniques have you found most helpful? Let us know what topics you’re interested in exploring next concerning C++ debugging!