The Eigen library in C++ provides a powerful, high-performance template library for linear algebra, including matrix and vector operations.
Here’s a simple example of using Eigen to perform matrix addition:
#include <iostream>
#include <Eigen/Dense>
using namespace Eigen;
int main() {
Matrix2d mat1;
Matrix2d mat2;
mat1 << 1, 2,
3, 4;
mat2 << 5, 6,
7, 8;
Matrix2d result = mat1 + mat2;
std::cout << "Result of matrix addition:\n" << result << std::endl;
return 0;
}
Getting Started with Eigen
Installing Eigen
To start using Eigen in your C++ projects, you'll first need to install the library. Here’s how you can do it step-by-step:
-
Download Eigen: Visit the official Eigen website and download the latest version of the library. Eigen is header-only, which means you only need to include the headers in your project.
-
Extract and Include: After downloading, extract the contents of the .zip or .tar archive. You will find a folder named `Eigen`. This folder contains all the header files.
-
Integrate into Your Project: To use Eigen, include the path to the Eigen folder in your project's settings as follows:
- For Visual Studio: Go to Project Properties → C/C++ → General → Additional Include Directories, and add the path.
- For GCC or Clang: Use the `-I` flag to include the path in your terminal/IDE.
Basic Concepts of Eigen
Eigen provides several matrix and vector types that are essential for linear algebra operations. Two fundamental types in Eigen are `Matrix` and `Vector`.
-
Matrix Types:
- `MatrixXd`: Represents a dynamic-size matrix with double precision.
- `Matrix2d`: Represents a fixed-size 2x2 matrix.
-
Vector Types:
- `VectorXd`: Represents a dynamic-size vector with double precision.
- `Vector2d`: Represents a fixed-size 2D vector.
Here is an example to illustrate how to create matrices and vectors:
#include <Eigen/Dense>
#include <iostream>
using namespace Eigen;
int main() {
MatrixXd mat(2, 2);
mat << 1, 2,
3, 4;
VectorXd vec(2);
vec << 5, 6;
std::cout << "Matrix:\n" << mat << std::endl;
std::cout << "Vector:\n" << vec << std::endl;
return 0;
}
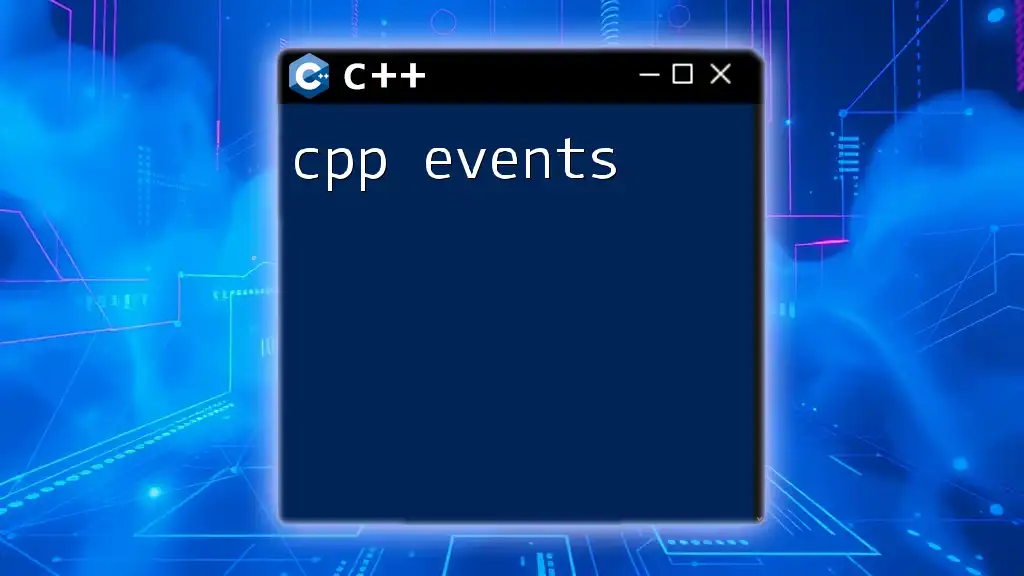
Performing Basic Matrix Operations
Addition and Subtraction
Eigen makes it easy to perform element-wise operations on matrices and vectors. Here’s how you can add and subtract matrices:
MatrixXd matA(2, 2);
matA << 1, 2,
3, 4;
MatrixXd matB(2, 2);
matB << 5, 6,
7, 8;
MatrixXd resultAdd = matA + matB;
MatrixXd resultSub = matA - matB;
In this example, `resultAdd` contains the sum of `matA` and `matB`, while `resultSub` contains their difference.
Scalar Multiplication
Scalar multiplication is straightforward in Eigen. You can easily multiply a matrix or vector by a scalar value. Here’s a code snippet to demonstrate:
MatrixXd mat(2, 2);
mat << 1, 2,
3, 4;
double scalar = 2.0;
MatrixXd result = scalar * mat;
The variable `result` now contains each element of `mat`, multiplied by `2.0`.
Matrix Multiplication
Matrix multiplication differs from element-wise addition and subtraction, as it requires conforming dimensions. Here’s how to perform matrix multiplication in Eigen:
MatrixXd matA(2, 3), matB(3, 2);
matA << 1, 2, 3,
4, 5, 6;
matB << 7, 8,
9, 10,
11, 12;
MatrixXd result = matA * matB;
The `result` matrix contains the product of `matA` and `matB`.
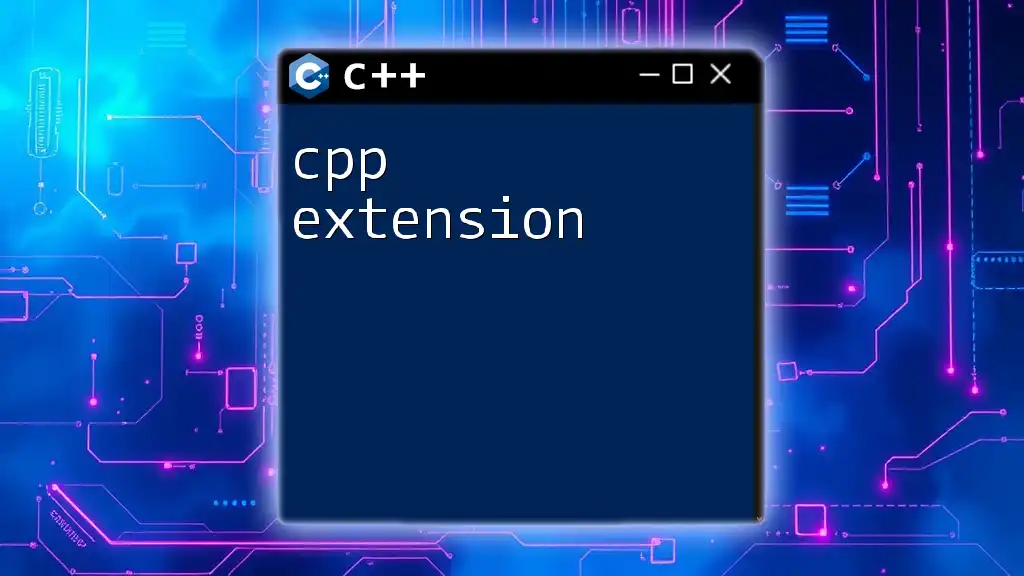
Advanced Operations with Eigen
Inversion
Matrix inversion is an important operation, especially when solving linear systems. Here’s how you can invert a matrix using Eigen:
MatrixXd mat(2, 2);
mat << 1, 2,
3, 4;
MatrixXd invMat = mat.inverse();
The `invMat` now contains the inverse of `mat`. Ensure that the matrix is non-singular, as attempting to invert a singular matrix will result in an error.
Eigenvalues and Eigenvectors
Eigenvalues and eigenvectors are crucial in various scientific computations. You can compute these entities in Eigen as follows:
Eigen::EigenSolver<MatrixXd> eigSolver(mat);
std::cout << "Eigenvalues:\n" << eigSolver.eigenvalues() << std::endl;
std::cout << "Eigenvectors:\n" << eigSolver.eigenvectors() << std::endl;
This example extracts the eigenvalues and eigenvectors from `mat`, allowing you to analyze its properties further.
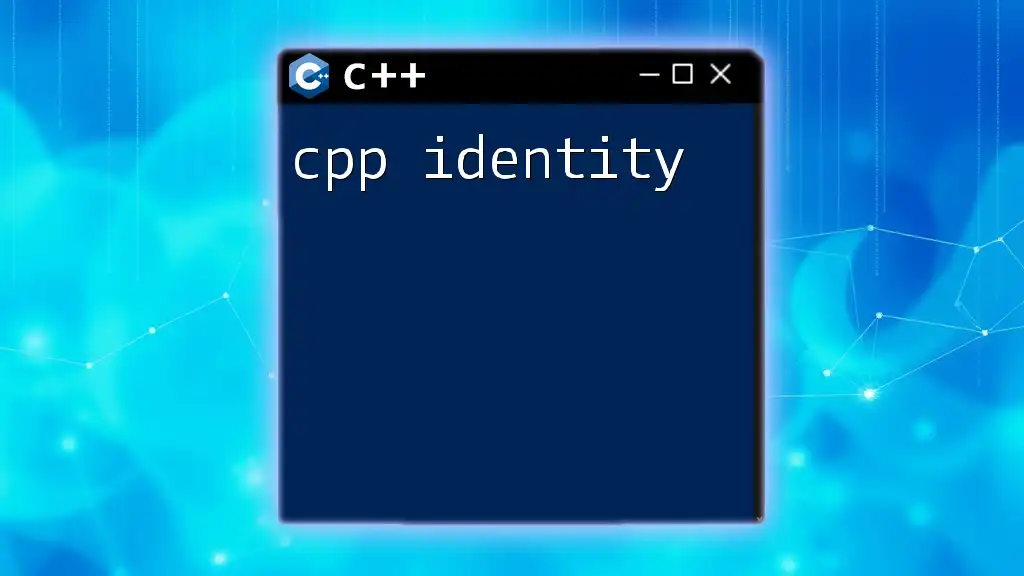
Practical Applications of Eigen
Solving Linear Systems
One of the significant advantages of using Eigen is the ability to solve linear systems efficiently. Given a linear system in the form of \( Ax = b \), you can find the value of \( x \) with the following code:
MatrixXd A(2, 2);
A << 3, 2,
1, 4;
VectorXd b(2);
b << 5, 6;
VectorXd x = A.colPivHouseholderQr().solve(b);
After running this code, the variable `x` will contain the solution for the system.
Optimization Problems
Eigen can also be employed to tackle optimization problems, especially in fields like machine learning and operations research. You can implement gradient descent, least squares fitting, and other optimization techniques utilizing Eigen’s matrix operations.
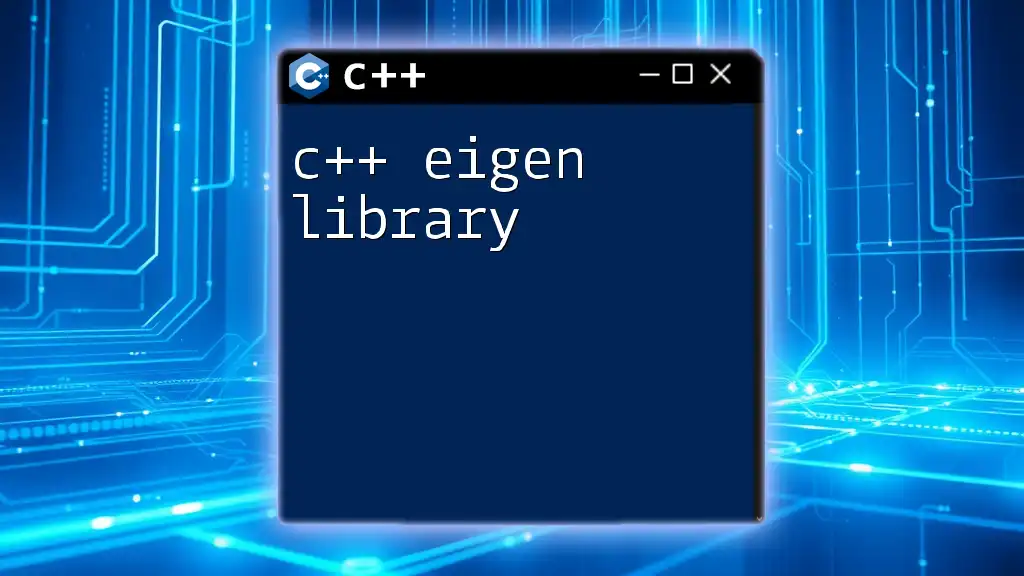
Performance Considerations
Compiler Optimizations
To maximize performance, make sure to enable optimization flags while compiling your code. Common flags include `-O2` or `-O3` when using GCC or Clang. These optimizations can significantly speed up execution without altering the program's output.
Memory Management
Eigen implements efficient memory management strategies. You can choose between static-sized and dynamic-sized matrices. Static matrices are faster but have fixed sizes, while dynamic matrices allow for more flexibility. It’s essential to choose the right type based on your application's needs to optimize resource utilization.
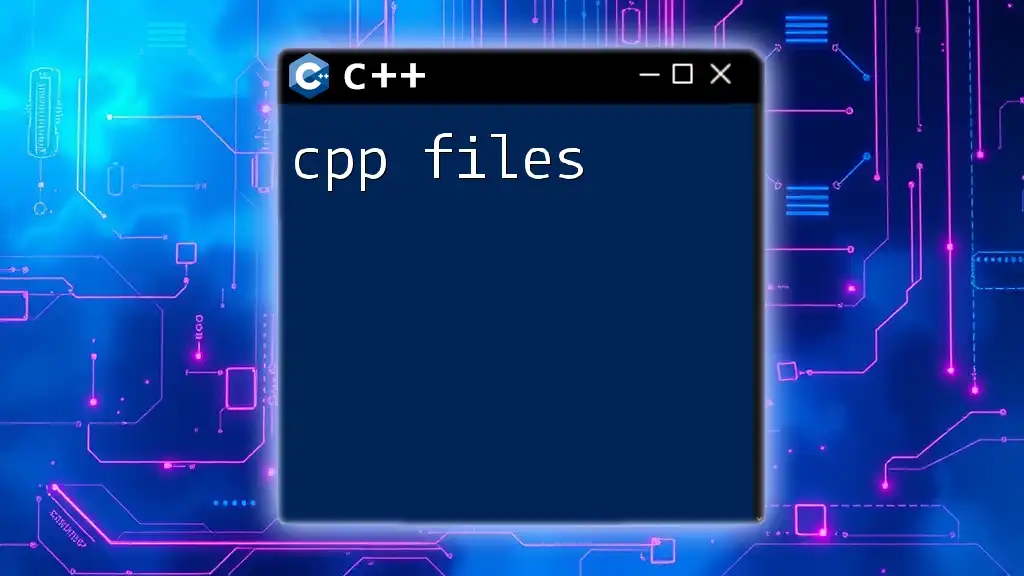
Debugging Eigen Code
While using Eigen, you may encounter some pitfalls. Here are a few common issues and how to resolve them:
- Matrix Size Mismatches: Ensure that your matrices conform to the rules of matrix operations (e.g., correct dimensions for multiplication).
- Using Uninitialized Variables: Always initialize your matrices and vectors before usage to prevent undefined behavior.
- Check for Singular Matrices: Before inverting a matrix, verify that it is non-singular to avoid errors.
Utilizing debugging tools like `gdb` or IDE-integrated debuggers can help trace and resolve these issues efficiently.
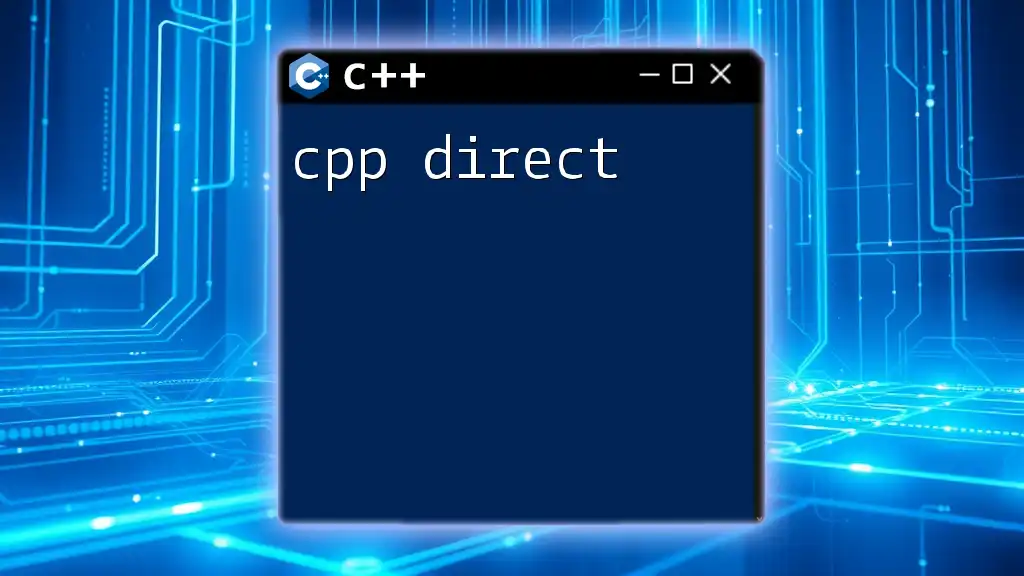
Conclusion
In summary, the CPP Eigen library is a powerful tool for performing linear algebra computations with ease and efficiency. By mastering its core concepts such as matrix operations, inversions, and solving linear systems, you can leverage Eigen in various applications, from basic projects to complex scientific calculations.
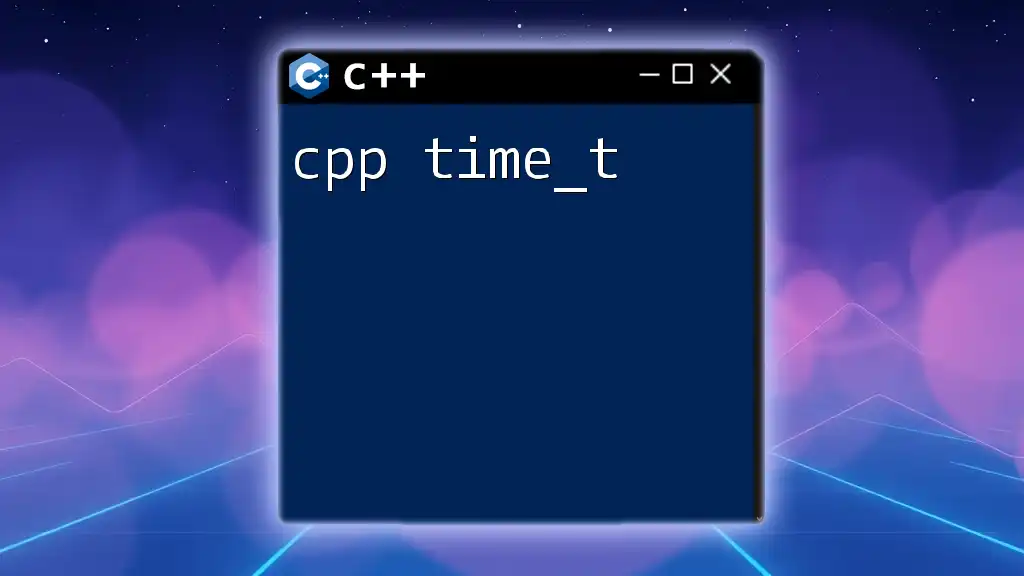
Further Resources
For additional learning, refer to the official Eigen documentation, which provides extensive details and examples for all functionalities. Consider exploring recommended books or online courses that delve deeper into linear algebra and optimization techniques, as well as participating in community forums for collaborative learning and support.
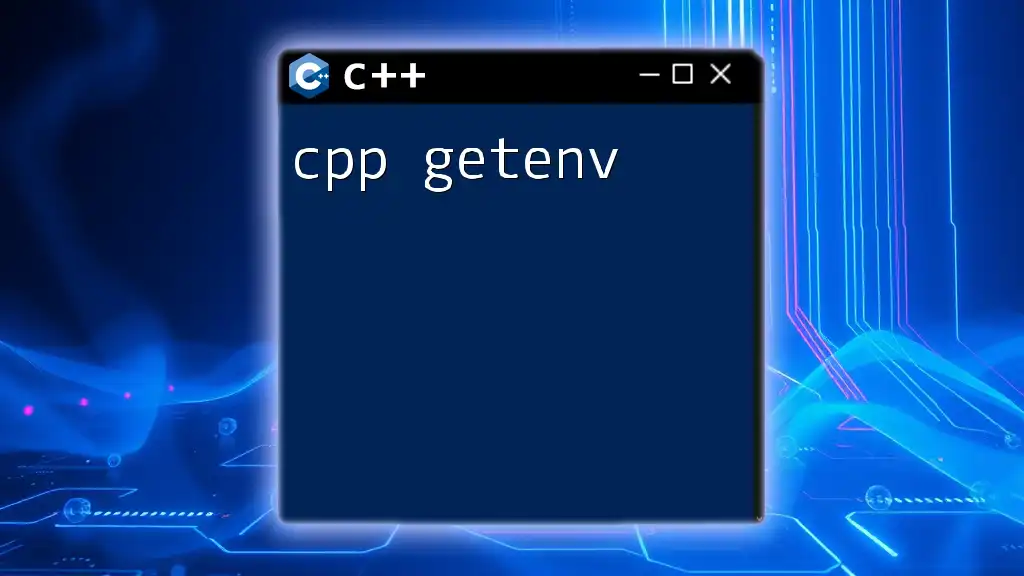
Additional Examples and Use Cases
Finally, applying what you've learned in real-world problems can significantly strengthen your understanding of CPP Eigen. Examples include using Eigen in graphical applications for transformations or implementing machine learning algorithms where efficient linear algebra is crucial. Engage with projects that challenge your skills and push you to apply Eigen in innovative ways.