A C++ debugger online is a web-based tool that allows users to write, compile, and debug C++ code in real-time without the need for local software installation.
Here's a simple example that demonstrates a basic C++ program using a debugger:
#include <iostream>
int main() {
int a = 5;
int b = 0;
std::cout << "Enter a number: ";
std::cin >> b;
std::cout << "The result of a divided by b is: " << a / b << std::endl; // Debug here to check division
return 0;
}
In this example, you can set a breakpoint on the division line to inspect the values of `a` and `b` as you debug the program.
Introduction to C++ Debugging
Debugging is an essential aspect of programming that involves identifying and fixing errors within code. In C++, debugging allows developers to ensure their programs run correctly and efficiently. The importance of debugging cannot be overstated, as even minor errors can lead to significant problems, including crashes or unexpected behavior.
Common errors in C++ programming can range from syntax issues to logical errors, each requiring different approaches to resolve. This is where utilizing a C++ debugger online becomes vital. An online debugger provides developers with a way to test and troubleshoot their code without the need for local setup, making it accessible for learning and experimentation.
What is an Online Debugger?
An online debugger is a web-based tool that allows developers to write, compile, run, and debug their code directly in a browser. The advantages of using online debuggers include:
- Accessibility: Since they are browser-based, any device with internet access can run them.
- No Installation Required: Users can immediately start coding without needing to set up a local development environment.
- Collaboration: Online debuggers often come with features that allow sharing code snippets with peers for collaboration and feedback.
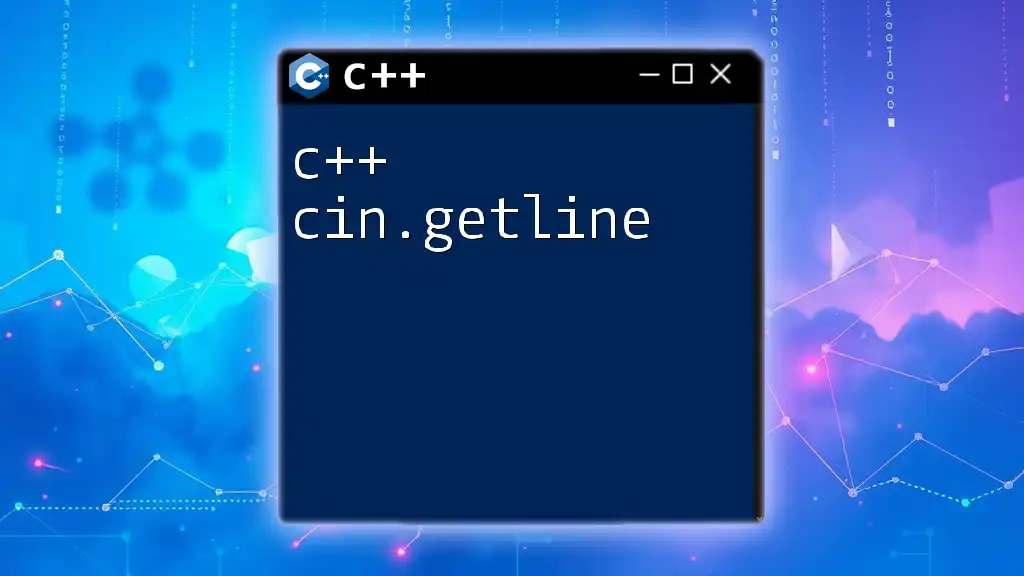
Choosing the Right Online Debugger for C++
When searching for a C++ debugger online, it's essential to consider particular characteristics that will suit your needs.
Characteristics of an Effective Online Debugger
User Interface and Ease of Use: A clear, intuitive interface can significantly enhance your coding experience. Look for debuggers that are easy to navigate, with user-friendly tools.
Supported C++ Standards: Not all online debuggers support the latest C++ standards. Ensure the debugger you choose aligns with the version of C++ you are using.
Additional Features: Features like code highlighting and auto-completion can vastly improve productivity and code quality.
Popular Online Debuggers for C++
Several online debuggers stand out in the market:
- Tool A: Known for its clean interface and efficient error reporting, Tool A allows users to test code quickly and provides excellent support for different C++ versions.
- Tool B: This online debugger offers collaborative editing features and a built-in community for sharing code examples, which is beneficial for learning.
- Tool C: Featuring extensive documentation, Tool C helps both novice and experienced programmers with educational resources.
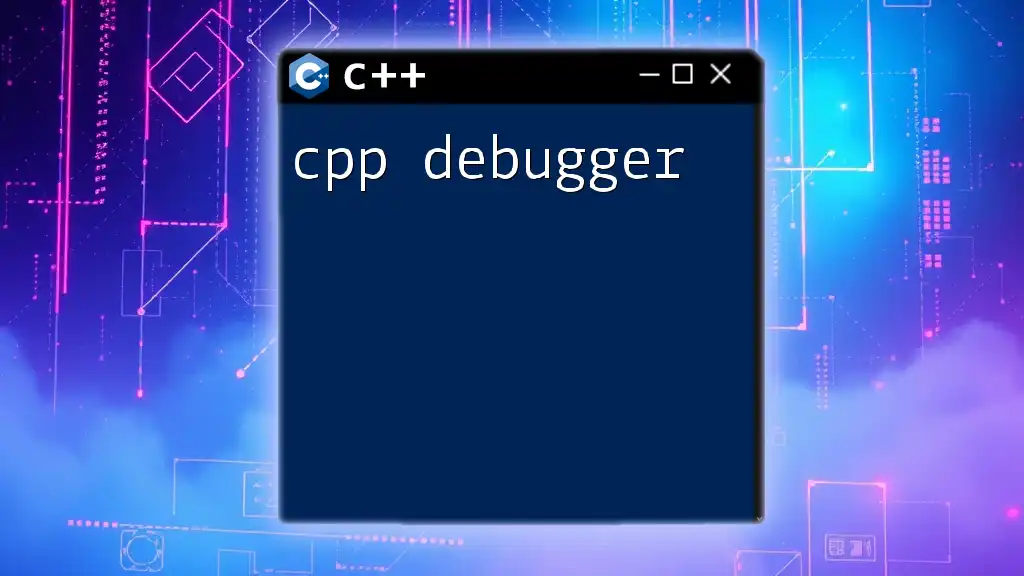
Getting Started with an Online C++ Debugger
Ready to dive into debugging? Here’s how to get started with an online C++ debugger.
Step-by-Step Setup Guide
Accessing the Online Debugger: Choose your preferred online debugger from the list above and navigate to its website.
Creating Your First Project: Most online debuggers will have a "New Project" feature. Select this option to begin working on your code.
Writing Sample Code: Let’s create a simple program to illustrate debugging. Here is a basic code snippet that attempts to divide two numbers:
#include <iostream>
using namespace std;
int main() {
int a = 10;
int b = 0;
cout << "Enter a number: ";
cin >> b;
cout << "Result: " << (a / b) << endl; // Potential division by zero
return 0;
}
Compiling and Running Your Code
Compile your code after writing it. Compiling translates your code into machine language, while running executes the code. Understanding the difference will help you utilize online debuggers more effectively. If there are compilation errors, the debugger will notify you, often detailing the nature of the error.
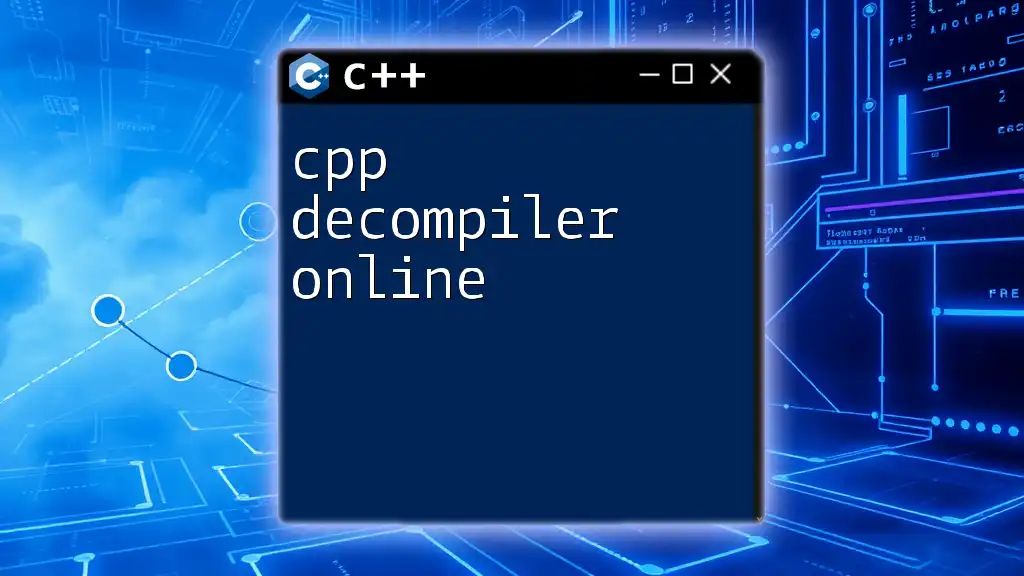
Debugging Techniques in C++
Learning effective debugging techniques will streamline your coding process significantly.
Basic Debugging Techniques
Using Print Statements: A straightforward approach for beginners is inserting print statements to track the value of variables at various points in the code.
Step-by-Step Execution: Many online debuggers allow you to run code line by line. This feature helps in pinpointing where the error occurs.
Advanced Debugging Techniques
- Setting Breakpoints: Breakpoints let you pause execution at specific points in your program, allowing for in-depth inspection.
- Using Watch Variables: This feature enables you to monitor variable changes over time, providing insight into how data is manipulated.
- Inspecting Call Stack: Understanding the call stack can help identify not just where but how your program reached a particular state.
Practical Debugging Example
Let's analyze the sample code provided earlier. When you run this, you'll likely encounter a runtime error due to division by zero if `b` is set to zero. Using the techniques mentioned, you can set a breakpoint before the division and inspect the value of `b`. This enables you to catch the error before it causes a crash.
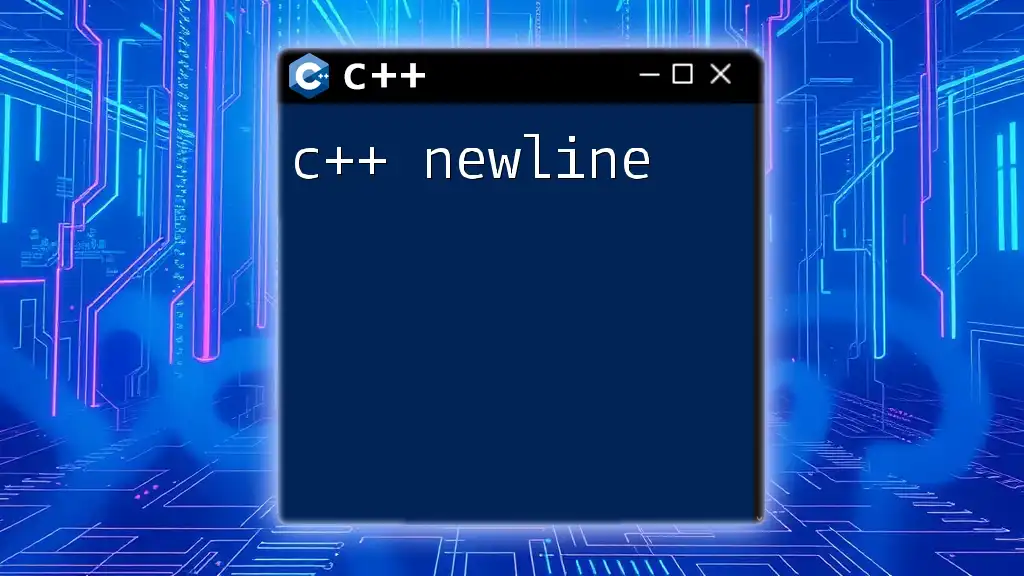
Utilizing Online Debugger Features
Effective use of the features available in an online debugger can greatly enhance your debugging efficiency.
Code Syntax Highlighting
Syntax highlighting is crucial for readability. By distinguishing keywords, variables, and comments through color coding, you can quickly spot errors or areas needing attention.
Error Reporting and Suggestions
When coding, you’ll often receive messages indicating what's wrong. Learning to interpret these messages is crucial. Most online debuggers provide suggestions to help fix errors, which can be a valuable learning tool.
Collaboration Features
Many online debuggers facilitate code sharing, fostering peer collaboration. Utilize these tools to request feedback or assistance, especially when tackling complex problems.
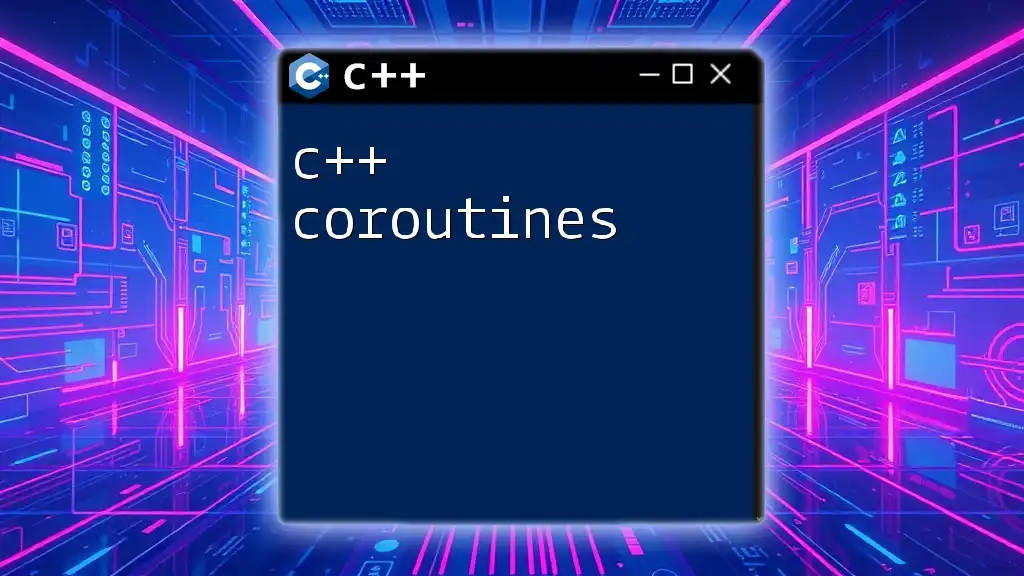
Troubleshooting Common Issues
Even with excellent tools, challenges may arise when using an online debugger.
Common Challenges with Online Debuggers
Browser Compatibility Issues: Some features may not function as intended on all browsers, which can impede the debugging process.
Code Compatibility with Different Platforms: Ensure that your code conforms to the specific features of the online debugger, as discrepancies in C++ standards can lead to errors.
Tips to Overcome These Issues
- Stay updated with the latest versions of browsers to ensure compatibility with online tools.
- Adhere to standardized coding practices, ensuring compatibility across various debugging platforms.
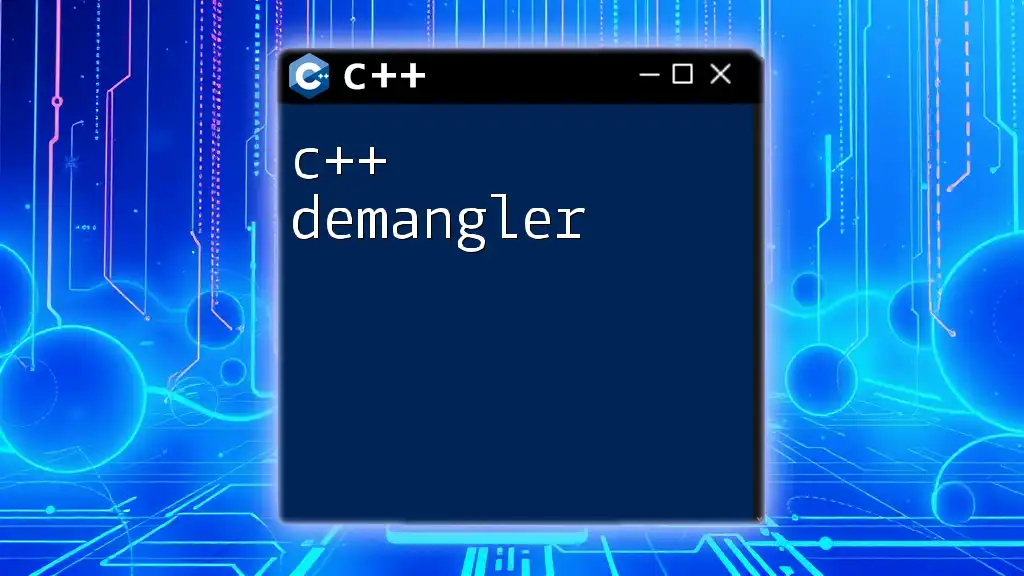
Best Practices for Effective C++ Debugging
Implementing best practices will boost your debugging efficiency.
Maintain Clean Code
Writing clean, organized code is foundational for effective debugging. Structuring your code logically helps quickly spot errors and understand your design.
Organizing Your Code
Use functions and classes to break down complex programs into manageable sections. This approach not only simplifies debugging but also promotes reusability.
Testing Your Code Regularly
Incorporating unit tests into your workflow enables early error detection. Regular testing fosters a deeper understanding of your code’s behavior and reduces the time spent debugging.
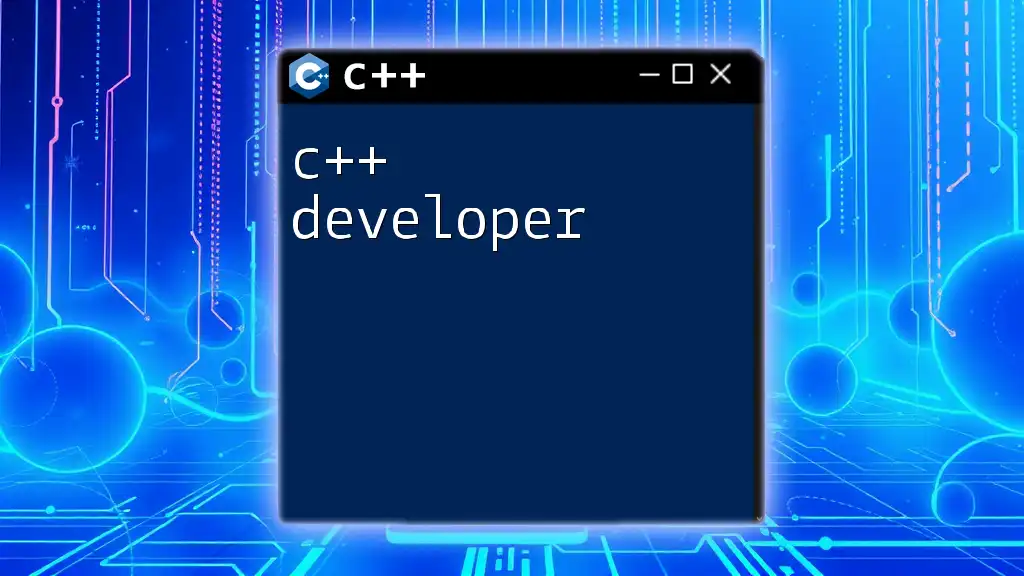
Conclusion
Utilizing a C++ debugger online is an essential part of error management in programming. With the right tools and techniques, you can enhance your debugging skills significantly. Regular practice, along with collaboration and adherence to coding best practices, will establish a strong foundation for your programming journey. Explore the various online debuggers available, and don’t hesitate to harness their power for effective and efficient code debugging!
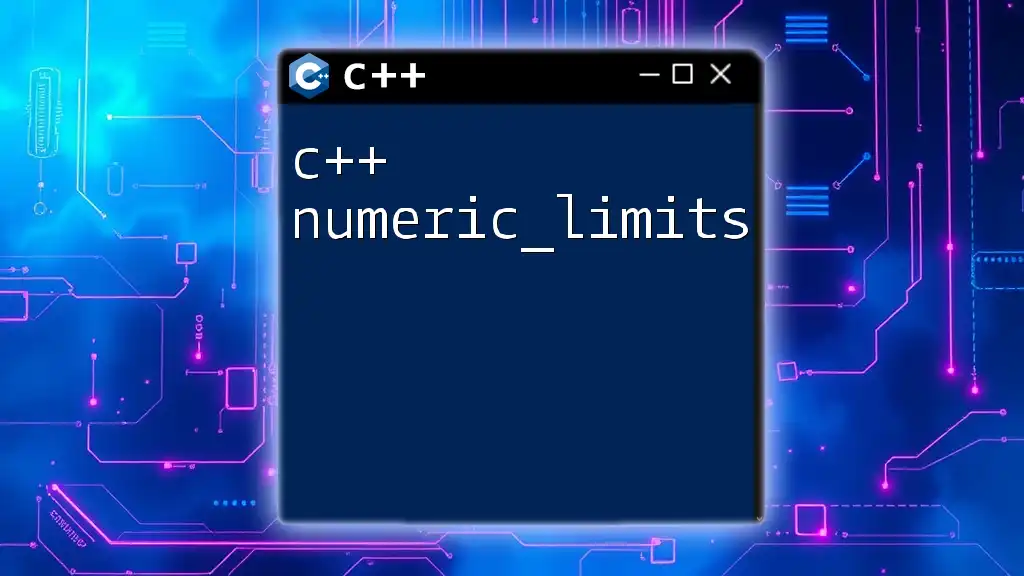
Additional Resources
To further enhance your understanding and skills:
- Explore links to top online debuggers tailored for C++.
- Consider reading recommended C++ books and tutorials.
- Engage with coding communities and forums to stay updated and seek assistance.