`jwt-cpp` is a lightweight C++ library for creating and verifying JSON Web Tokens (JWT) efficiently and securely.
Here's a simple example of how to create a JWT using `jwt-cpp`:
#include <jwt-cpp/jwt.h>
int main() {
// Create a JWT token
auto token = jwt::create()
.set_issuer("auth.server.com")
.set_subject("user@example.com")
.set_claim("role", "user")
.set_expires_at(std::chrono::system_clock::now() + std::chrono::seconds{3600})
.sign(jwt::algorithm::hs256{"your-secret-key"});
// Output the generated token
std::cout << "Generated JWT: " << token << std::endl;
return 0;
}
What is JWT?
JWT, or JSON Web Token, is a compact and self-contained way for securely transmitting information between parties as a JSON object. It can be used to verify the authenticity of the transmitted information, allowing for secure data exchanges.
JWTs are often used for authentication and information exchange. They facilitate stateless communication, meaning that the server does not need to maintain a session for every user. Instead, a JWT can be sent with each request and can contain all the information necessary to authenticate the user.
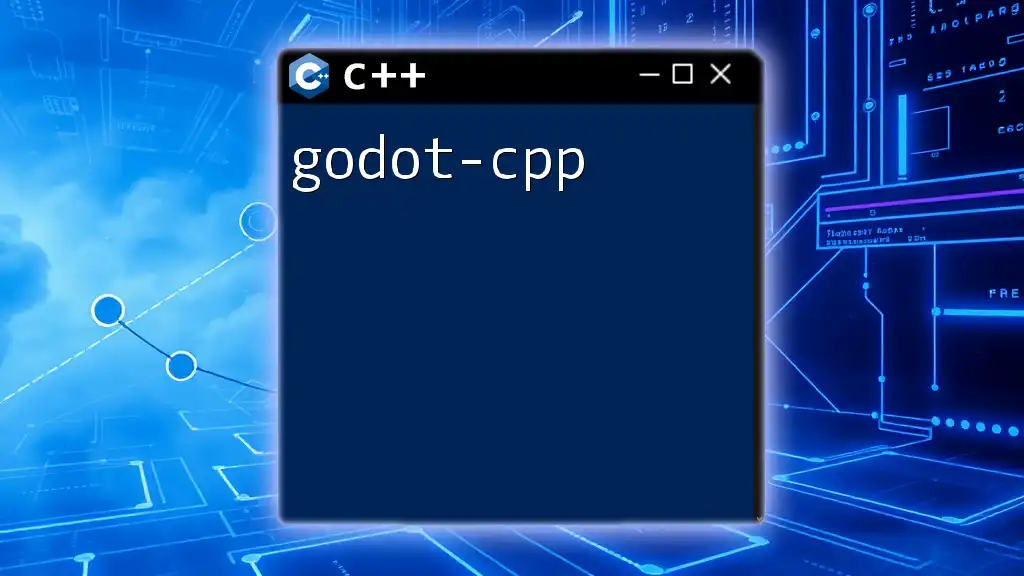
Why Use jwt-cpp?
jwt-cpp is a powerful C++ library designed specifically for handling JWTs. Its lightweight construction allows developers to easily create, parse, and verify JWTs without hassle.
Key features and benefits of using jwt-cpp include:
- Ease of use: Designed with simplicity in mind, it allows developers to integrate JWT functionality quickly and efficiently.
- Flexibility: Supports various signing algorithms including HMAC and RSA.
- Lightweight and efficient: Minimal overhead, making it suitable for high-performance applications.
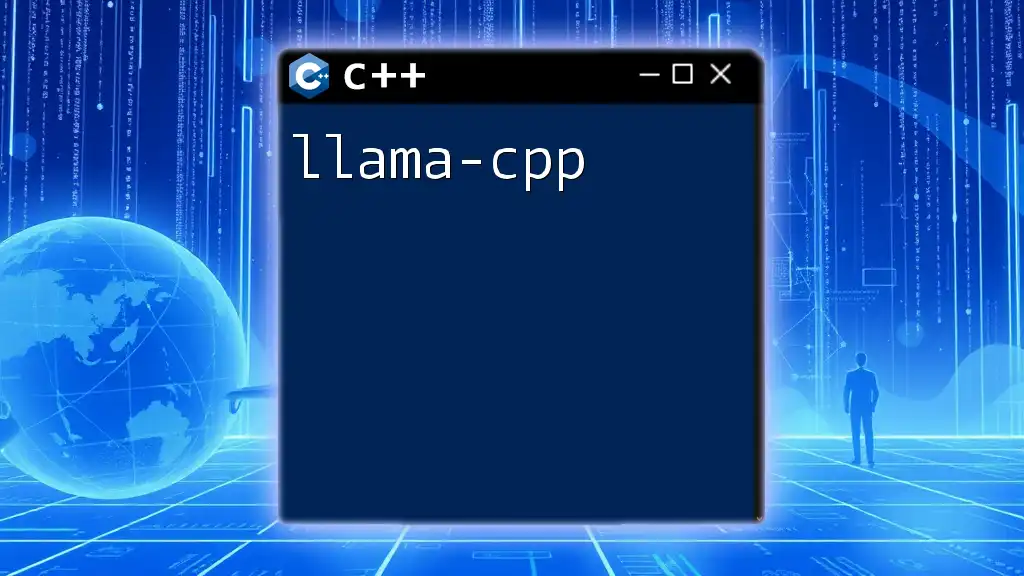
Installation
How to Install jwt-cpp
You can install jwt-cpp using a package manager, or you can manually install it from the source.
For Linux users utilizing vcpkg:
vcpkg install jwt-cpp
For manual installation, clone the repository from GitHub and build it:
git clone https://github.com/Thalhammer/jwt-cpp.git
cd jwt-cpp
mkdir build
cd build
cmake ..
make
Setting Up Your Development Environment
Before diving into coding, ensure that you have the necessary dependencies installed. You will generally need:
- A C++ compiler that supports C++11 or later.
- CMake for building your project.
For example, a basic CMake configuration to include jwt-cpp might look like this:
cmake_minimum_required(VERSION 3.10)
project(MyJwtCPPExample)
add_executable(my_jwt_example main.cpp)
find_package(jwt-cpp REQUIRED)
target_link_libraries(my_jwt_example jwt-cpp)
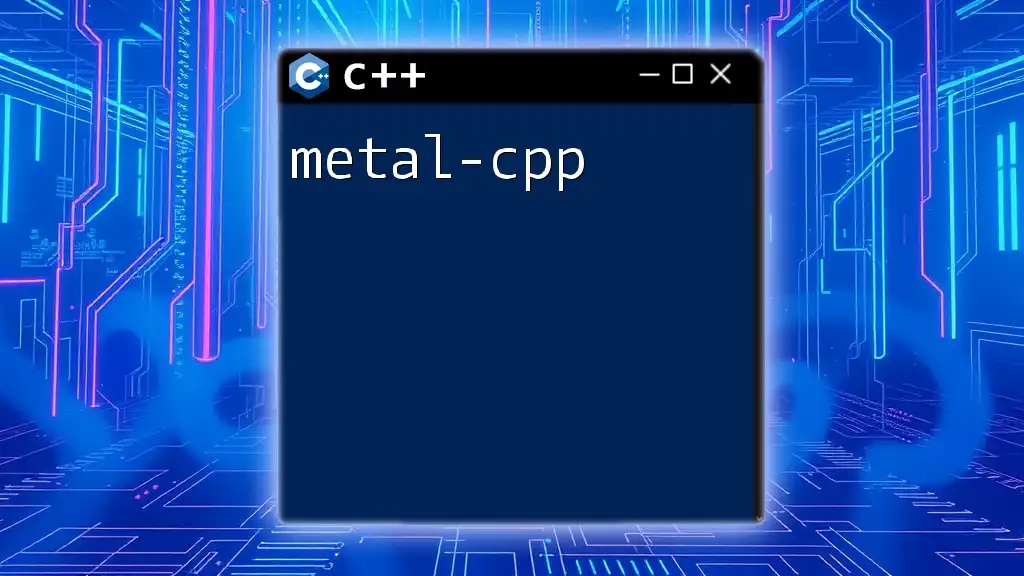
Core Concepts of JWT
Structure of a JWT
A JWT consists of three parts: Header, Payload, and Signature.
- Header: Typically consists of two parts: the type of the token (JWT) and the signing algorithm being used.
- Payload: Contains the claims, which can be predefined or custom. These claims contain the actual data that is being transmitted.
- Signature: To create the signature part, you need to have the encoded header, the encoded payload, a secret, and the algorithm specified in the header.
Signing and Verifying JWTs
Signing and verifying JWTs is crucial for maintaining data integrity and authenticity.
HMAC and RSA are two common methods:
- HMAC (symmetric): The same secret key is used for both signing and verifying the JWT.
- RSA (asymmetric): A pair of public and private keys is used. The private key signs the token, while the public key is used to verify it.
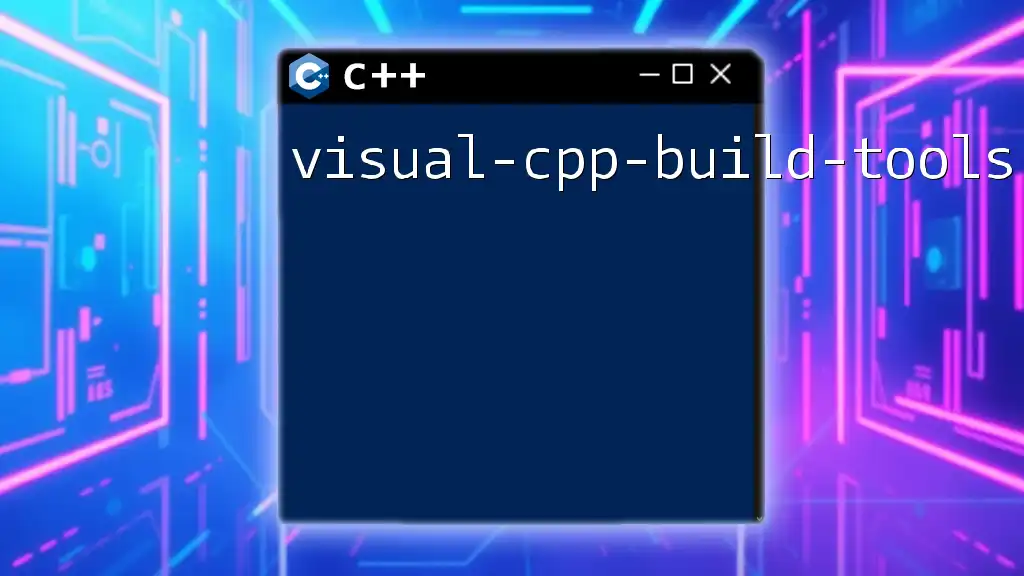
Using jwt-cpp for JWT Creation
Creating JWTs
Creating a JWT with jwt-cpp is straightforward. Here’s a code snippet to demonstrate how to create one:
#include <jwt-cpp/jwt.h>
std::string createJwt(const std::string& secret) {
auto token = jwt::create()
.set_issuer("auth.example.com")
.set_subject("user@example.com")
.set_expires_at(std::chrono::system_clock::now() + std::chrono::hours(1))
.sign(jwt::algorithm::hs256{secret});
return token;
}
Explanation of the Code:
- `set_issuer`: Specifies who issued the token.
- `set_subject`: Indicates the subject of the token, usually representing the user.
- `set_expires_at`: Determines when the token will expire.
- `sign`: Signs the token with the chosen algorithm and secret key.
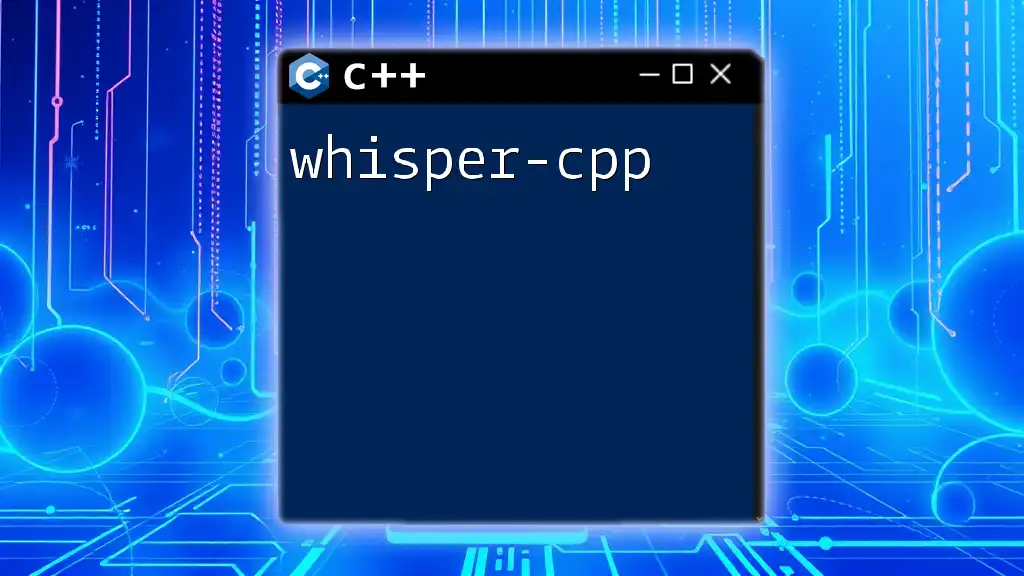
Parsing and Verifying JWTs
Extracting Information from JWTs
Once you’ve created a JWT, you can extract its contents. Here’s how to verify and decode the JWT:
#include <jwt-cpp/jwt.h>
void verifyJwt(const std::string& token, const std::string& secret) {
try {
auto decoded = jwt::decode(token);
auto verifier = jwt::verify()
.allow_secret_from(secret)
.with_issuer("auth.example.com");
verifier.verify(decoded);
// Extract payload data
std::string subject = decoded.get_payload_claim("sub").as_string();
} catch (const std::exception& e) {
// handle error
}
}
Explanation of the Code:
- `jwt::decode`: Decodes the JWT and retrieves the data.
- `jwt::verify`: Configures the verification process using the secret and issuer.
- `get_payload_claim`: Allows you to extract specific claims from the payload.
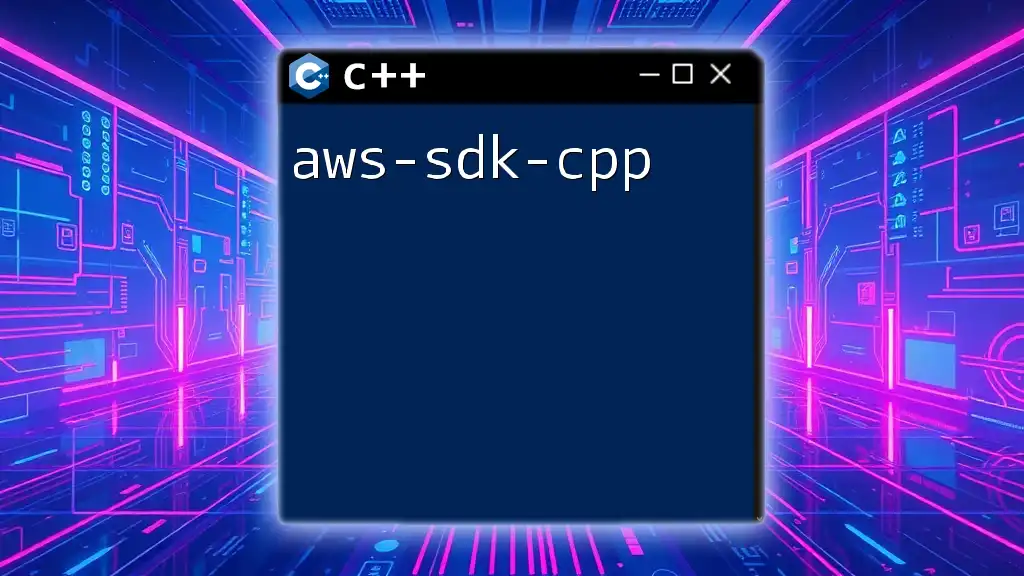
Error Handling in jwt-cpp
Common Errors and Exceptions
While working with JWTs, it is important to handle errors effectively. Common scenarios include:
- Expired tokens: When a token is used after its expiration time.
- Invalid tokens: Tokens that fail signature verification.
Best Practices for Error Management
Implementing robust error handling ensures that you can address issues proactively. Strategies include:
- Logging all errors for debugging purposes.
- Providing user-friendly messages when authentication fails.
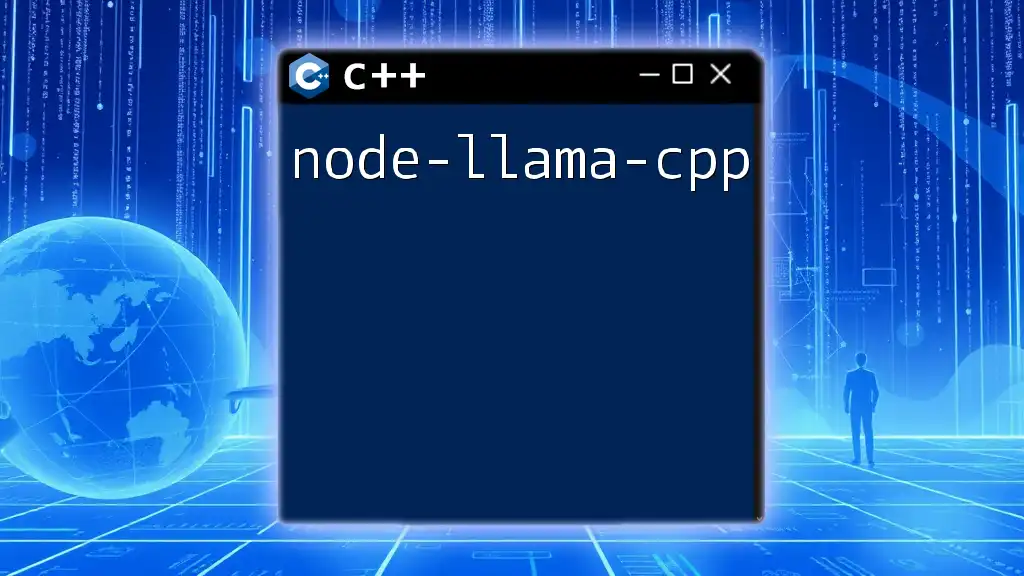
Advanced Features of jwt-cpp
Custom Claims
You can add custom claims to a JWT, extending its functionality:
auto token = jwt::create()
.set_issuer("auth.example.com")
.set_subject("user@example.com")
.set_claim("role", jwt::claim(std::string{"admin"}))
.sign(jwt::algorithm::hs256{secret});
This capability allows for further customization based on your application's specific needs.
Token Refresh Mechanism
For enhancing user experience, consider implementing a refresh token mechanism. This involves issuing short-lived access tokens complemented by long-lived refresh tokens, allowing users to obtain new access tokens without re-authentication.
Asynchronous Operations
In modern applications, JWT handling might require asynchronous operations. Libraries like Boost can be integrated with jwt-cpp to handle JWTs without blocking the main thread, improving responsiveness.
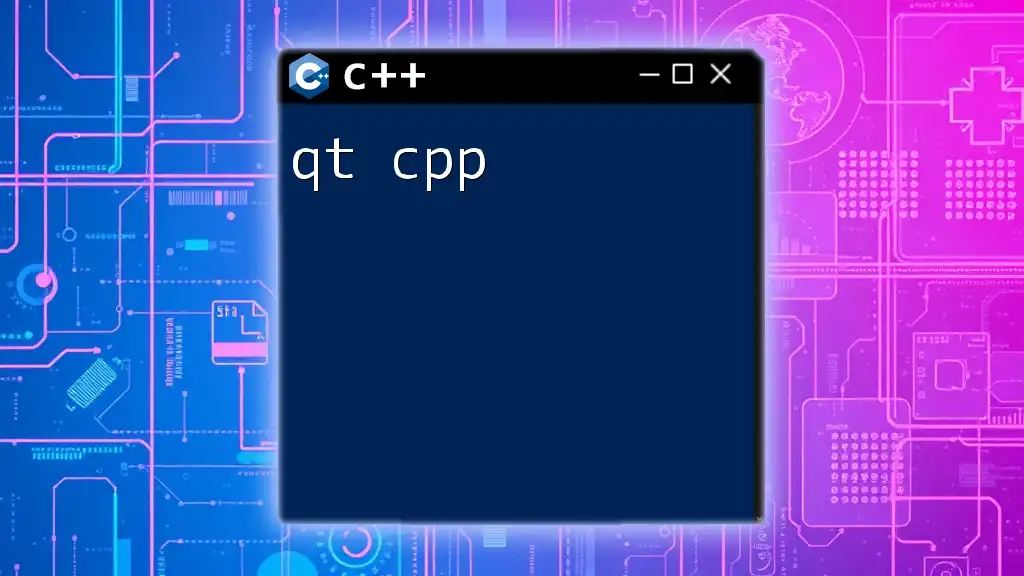
Real-World Applications of jwt-cpp
Use Cases in Authentication Systems
JWTs can be an excellent choice for API security. They can be included in HTTP headers when making requests to protected resources, enabling secure user authentication without server state.
Best Practices in Production Environments
Consider the following to ensure security while using JWTs:
- Use strong, random secrets.
- Store tokens securely on the client-side (e.g., HTTP-only cookies).
- Regularly rotate keys and implement token revocation mechanisms.
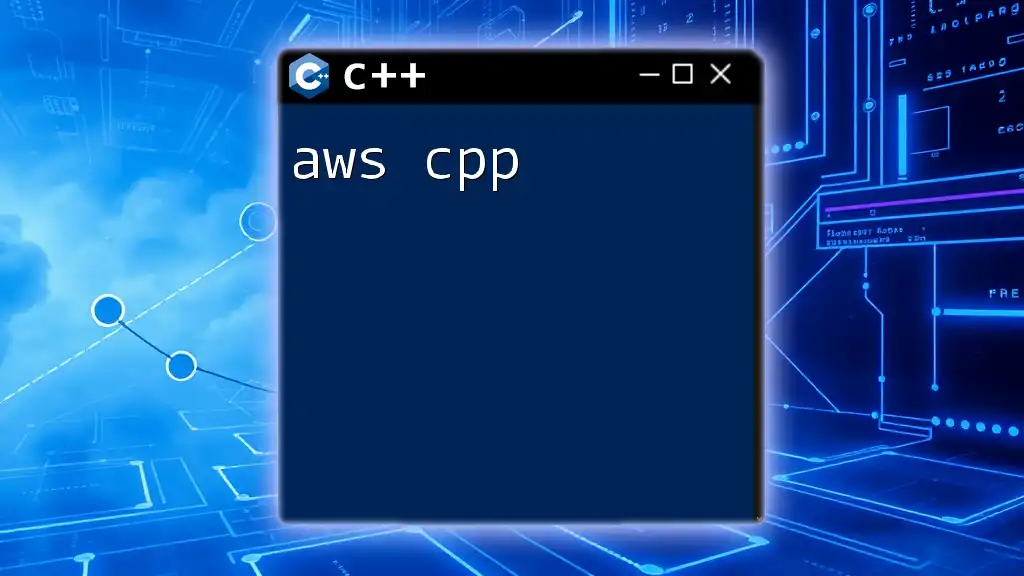
Conclusion
In summary, jwt-cpp serves as a powerful library for managing JSON Web Tokens in C++. Its ease of use combined with the flexible features it offers makes it a valuable tool in modern authentication systems. By understanding the structure of JWTs, using jwt-cpp effectively, and adhering to best practices, developers can create robust authentication solutions.