"Qt C++ is a powerful framework for developing cross-platform applications using C++, combining an intuitive API with excellent GUI capabilities."
Here’s a simple code snippet demonstrating the creation of a basic Qt application window:
#include <QApplication>
#include <QPushButton>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QPushButton button("Hello, Qt!");
button.resize(200, 100);
button.show();
return app.exec();
}
Getting Started with Qt
What is Qt?
Qt is a powerful cross-platform framework designed for developing applications with a rich graphical user interface. It offers a comprehensive set of libraries and tools to make application development faster and more efficient. Since its inception, Qt has evolved to support a wide array of platforms, allowing developers to create applications that run seamlessly on Windows, macOS, Linux, and even mobile devices.
Setting Up the Development Environment
Before diving into Qt development, it's vital to set up your development environment. This typically includes the following steps:
- Requirements for Qt Development: You will need to install Qt Creator as your Integrated Development Environment (IDE), along with the necessary C++ compilers suitable for your platform.
- Installation Steps: Download and install the Qt Installer from the official Qt website. During installation, select the components you need, including the version of Qt and the toolchains for your targeted platforms.
- Creating Your First Project: Once the installation is complete, open Qt Creator, create a new project, and choose a Qt Widgets Application. This will set the foundation for your first application.
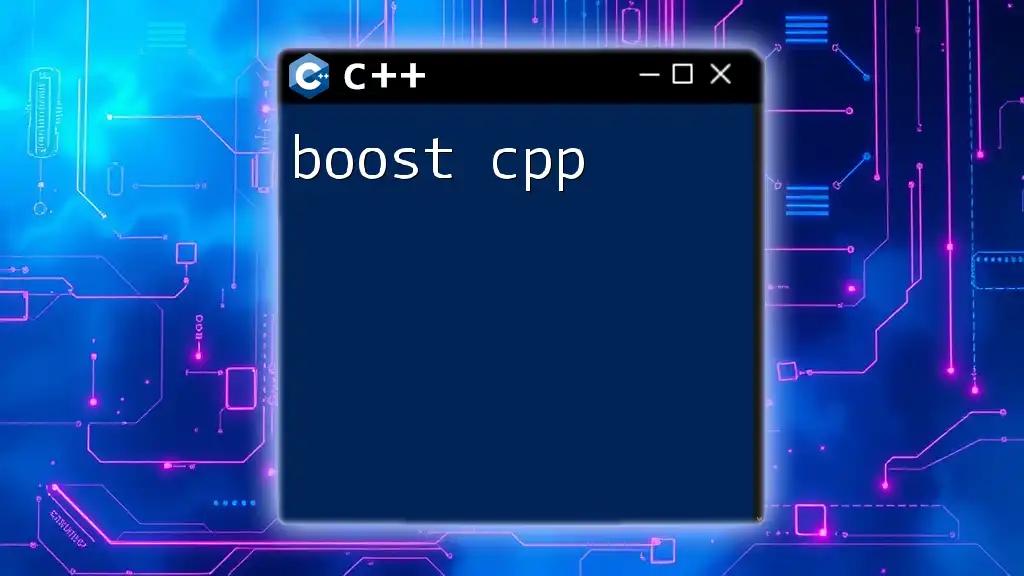
Understanding Qt's Structure
Core Components of Qt
The Qt framework is structured around several key modules, each serving a specific purpose:
- Core Module: Provides the foundational classes for managing events, data types, and the application's main loop.
- GUI Module: Contains classes for creating graphical user interfaces, including widgets, dialogs, and more.
- Widgets Module: Offers a vast array of user interface elements that make it easier to build interactive applications.
A crucial feature of Qt is the signal and slot mechanism, which facilitates communication between objects. When a signal is emitted, the connected slot is invoked, allowing developers to create interactive UI components effortlessly.
Qt’s Object Model
At the heart of Qt is the QObject class, which forms the basis for all Qt objects. Understanding this object model is central to using Qt effectively.
- Properties: Qt objects can have properties, which are variables that you can access and modify.
- Signals and Slots: Custom signals can be defined alongside standard ones, allowing for intricate event handling.
Here's an example of how to create a simple QObject-derived class:
#include <QObject>
class MyObject : public QObject {
Q_OBJECT
public:
MyObject(QObject *parent = nullptr) : QObject(parent) {
// Constructor implementation
}
signals:
void mySignal();
public slots:
void mySlot() {
// Slot implementation
}
};

Building a Basic Qt Application
Designing the User Interface
Qt Designer is a powerful tool for designing user interfaces. By leveraging drag-and-drop capabilities, you can create visually appealing layouts without needing to write extensive code upfront.
When creating your UI, consider the layout management options available:
- Horizontal Layouts: Align child widgets horizontally.
- Vertical Layouts: Align child widgets vertically.
- Grid Layouts: Arrange widgets in a grid format, optimizing space usage.
By organizing your UI effectively, you can create a more user-friendly experience.
Writing the Application Logic
After designing the interface, it’s time to write the application logic. A fundamental aspect of Qt programming is connecting signals and slots.
Here's an example of connecting a button click signal to a slot that handles the event:
connect(button, &QPushButton::clicked, this, &MainWindow::onButtonClicked);
In the above code, when the button is clicked, the `onButtonClicked` method will be executed.
Handling Events
Event handling is another critical aspect of Qt applications. You can override event handlers to customize program behavior. For instance, if you wanted to create a custom mouse click event, you could do so with the following example:
void MyWidget::mousePressEvent(QMouseEvent *event) {
// Custom behavior implementation
}
This functionality allows for a more interactive and responsive user interface.
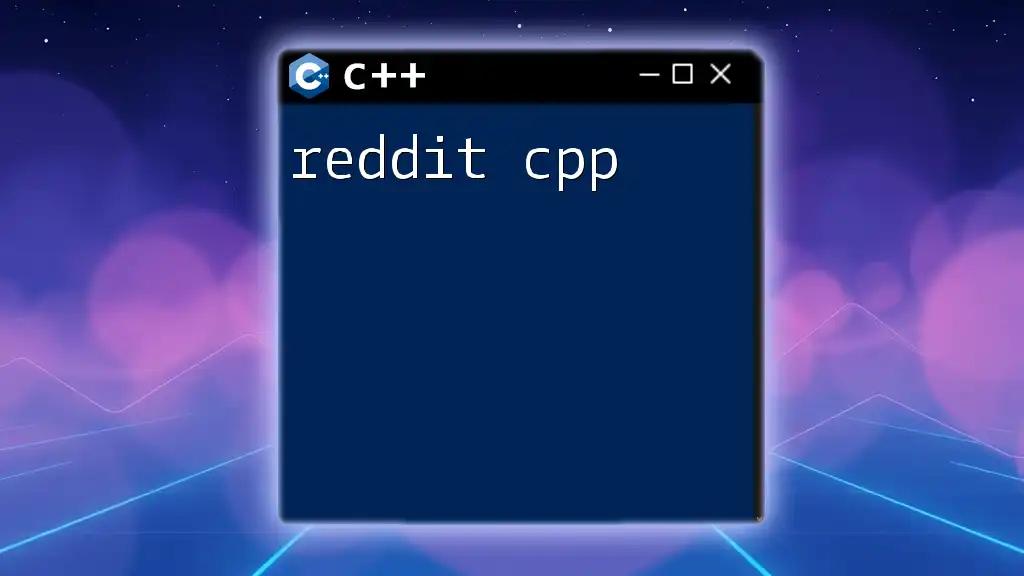
Advanced Qt Features
Working with Qt Widgets
Qt provides a rich variety of widgets ranging from simple buttons to complex input forms. Familiarity with these widgets can significantly expedite your development process. You can create custom widgets by subclassing existing ones, allowing for tailored functionality to meet your application's needs.
Multithreading with Qt
In modern applications, performance is paramount. Multithreading provides a way to execute multiple operations concurrently. The `QThread` class is essential for creating and managing threads in a Qt application.
Here’s a brief example of starting a thread in Qt:
MyThread *thread = new MyThread();
thread->start();
This allows for long-running tasks to be executed without freezing the user interface, creating a smoother user experience.
Database Interaction
With the Qt SQL module, accessing and manipulating databases becomes straightforward. Qt provides a unified API to work with different databases. Here’s how to connect to a database and fetch data:
- Include the necessary headers.
- Use `QSqlDatabase` to define your database connection.
- Execute SQL queries to fetch data.
Example snippet for fetching data:
QSqlDatabase db = QSqlDatabase::addDatabase("QSQLITE");
db.setDatabaseName("mydatabase.db");
if (db.open()) {
QSqlQuery query("SELECT * FROM tablename");
while (query.next()) {
QString data = query.value(0).toString();
// Process data
}
}
This approach allows seamless integration of data management into your applications.

Best Practices in Qt Development
Code Organization and Management
Following a clean architecture promotes efficient development. Implementing the Model/View architecture is highly encouraged in Qt applications. This approach helps separate user interface concerns from business logic, making your application more modular and easier to maintain.
Debugging and Optimization
Debugging is essential to any software development process. Qt Creator includes powerful debugging tools allowing you to track down and fix issues efficiently. Optimize your applications by profiling them and identifying bottlenecks, ensuring they run smoothly across all platforms.
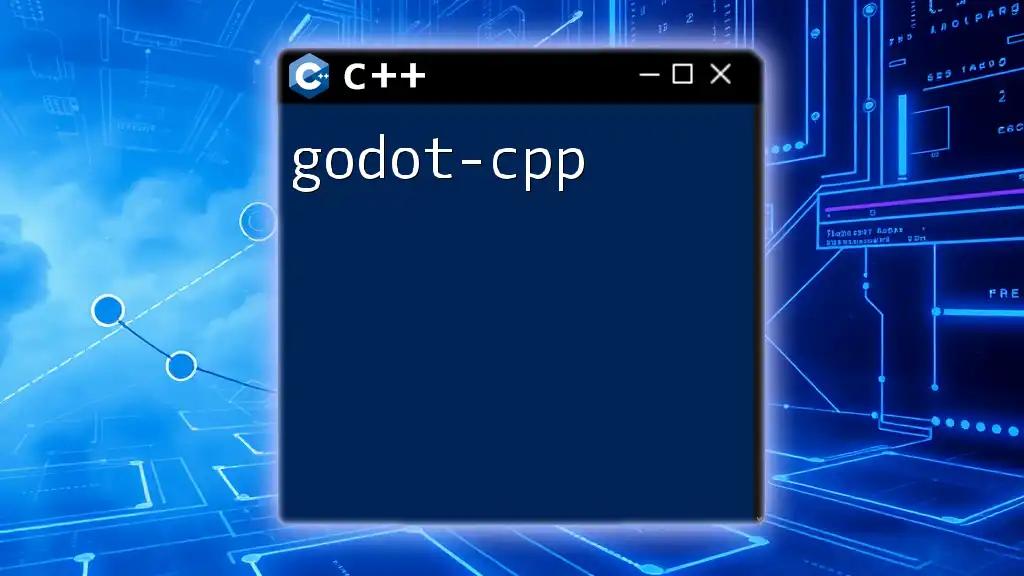
Resources for Further Learning
Official Documentation
The Qt documentation is comprehensive, providing detailed explanations of each module and functionality. It’s advisable to reference the documentation during development to enhance your understanding and solve any challenges you encounter.
Community and Support
Leverage forums like the Qt Forum and Stack Overflow for community support. These platforms are excellent resources for troubleshooting, gathering insights, and connecting with other developers. Additionally, consider contributing to open-source Qt projects to further deepen your skill set.
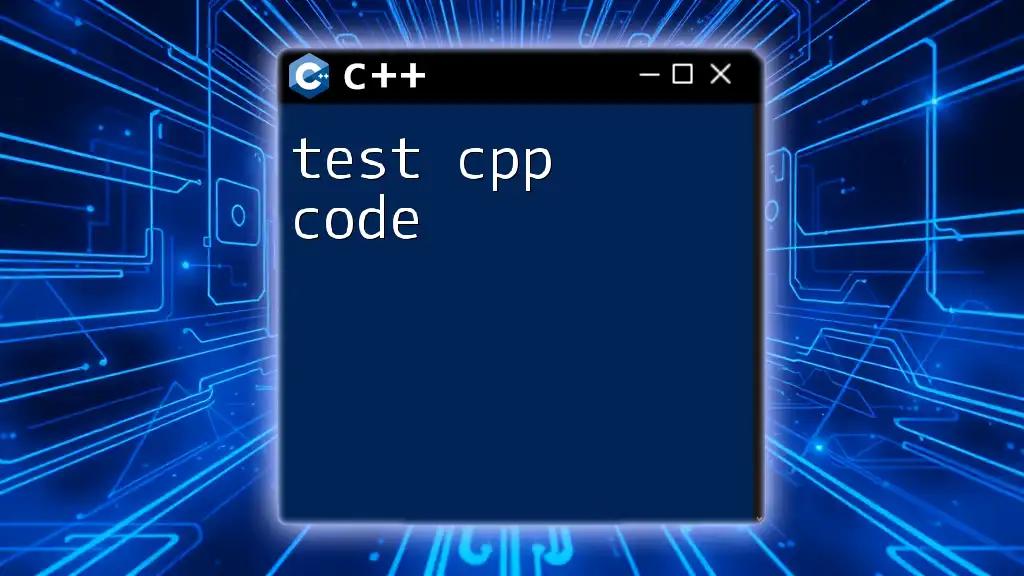
Conclusion
Qt CPP is a robust framework, providing developers with the tools they need to create sophisticated applications. With its extensive features and capabilities, it’s an essential skill for modern developers. Embrace your learning journey, explore advanced topics, and enhance your understanding of Qt. Stay tuned for more engaging tutorials and articles on C++ programming!