Radix sort is a non-comparative integer sorting algorithm that processes each digit of the numbers in a specific base (usually decimal or binary) to achieve efficient sorting.
Here's a simple implementation of Radix Sort in C++:
#include <iostream>
#include <vector>
using namespace std;
// Function to get the maximum value in the array
int getMax(const vector<int>& arr) {
return *max_element(arr.begin(), arr.end());
}
// A function to do counting sort of arr[] according to the digit represented by exp
void countingSort(vector<int>& arr, int exp) {
vector<int> output(arr.size());
int count[10] = {0};
// Store count of occurrences in count[]
for (int i = 0; i < arr.size(); i++)
count[(arr[i] / exp) % 10]++;
// Change count[i] so that it contains the actual position of this digit in output[]
for (int i = 1; i < 10; i++)
count[i] += count[i - 1];
// Build the output array
for (int i = arr.size() - 1; i >= 0; i--) {
output[count[(arr[i] / exp) % 10] - 1] = arr[i];
count[(arr[i] / exp) % 10]--;
}
// Copy the output array to arr[], so that arr[] now contains sorted numbers
for (int i = 0; i < arr.size(); i++)
arr[i] = output[i];
}
// The main function to implement radix sort
void radixSort(vector<int>& arr) {
// Find the maximum number to know the number of digits
int max = getMax(arr);
// Do counting sort for every digit. The exp is 10^i where i is the current digit number
for (int exp = 1; max / exp > 0; exp *= 10)
countingSort(arr, exp);
}
int main() {
vector<int> arr = {170, 45, 75, 90, 802, 24, 2, 66};
radixSort(arr);
cout << "Sorted array: ";
for (int num : arr)
cout << num << " ";
return 0;
}
What is Radix Sort?
Radix Sort is a non-comparative integer sorting algorithm that sorts numbers by processing individual digits. Rather than comparing entire numbers directly, it sorts them based on their individual digits from the least significant to the most significant. This process is repeated for each digit using a stable sorting algorithm—in most implementations, counting sort is used as a subroutine.
One of the key features that distinguishes Radix Sort from traditional sorting algorithms like Quick Sort and Merge Sort is that it can achieve performance that is linear relative to the number of items being sorted, given that the maximum number of digits (or the range of the data) is limited.
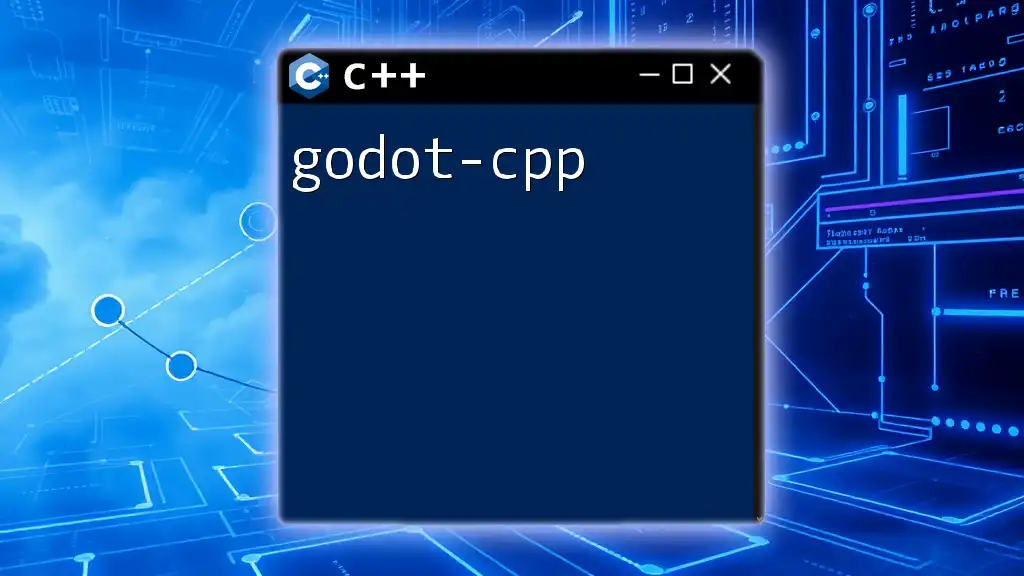
Key Characteristics of Radix Sort
Stability: Radix Sort is a stable sort, meaning that if two elements have the same key value, they will maintain their relative order in the sorted output. This characteristic is particularly important for multi-key sorting.
Time Complexity: The time complexity of Radix Sort is *O(d(n + k))**, where:
- n is the number of elements to be sorted.
- d is the number of digits in the longest number.
- k is the range of the input.
In practice, if d and k are small compared to n, Radix Sort can perform exceedingly well.
Space Complexity: The space complexity of the algorithm is O(n + k), where the additional space is primarily used for the counting sort subroutine.
Data Types Supported: Mainly integers, but Radix Sort can be adapted to other base representations—it's advisable to use it when the data is uniformly distributed.
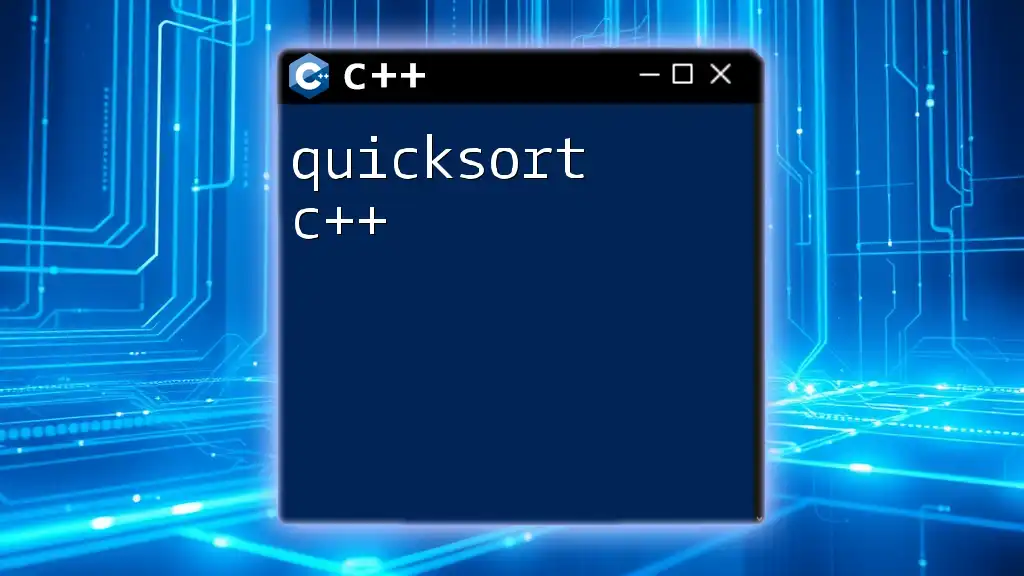
The Process of Radix Sort
Understanding the Basics of Digits Processing
Radix Sort sorts integers digit by digit. It starts with the least significant digit (LSD) and progresses to the most significant digit (MSD). The method relies on the idea that sorting by the lowest digit first will yield a complete sorted order after all digits have been processed.
Steps Involved in Radix Sort
- Identify the maximum number in the array to determine the number of digits for sorting.
- Perform a stable sort (using counting sort) on each digit, starting with the least significant digit.
For example, consider the following list of numbers: 170, 45, 75, 90, 802, 24, 2, 66. The sorting process will operate as follows:
- Sort using 0's: Result remains the same.
- Sort using 1's: (170 remains at place).
- Sort using 2's: (2 moves to its place).
- Sort using 3's and 4's: (order fixes 24, 45).
- Finally, sort using the 8's: (separate 802, and so forth until sorted).
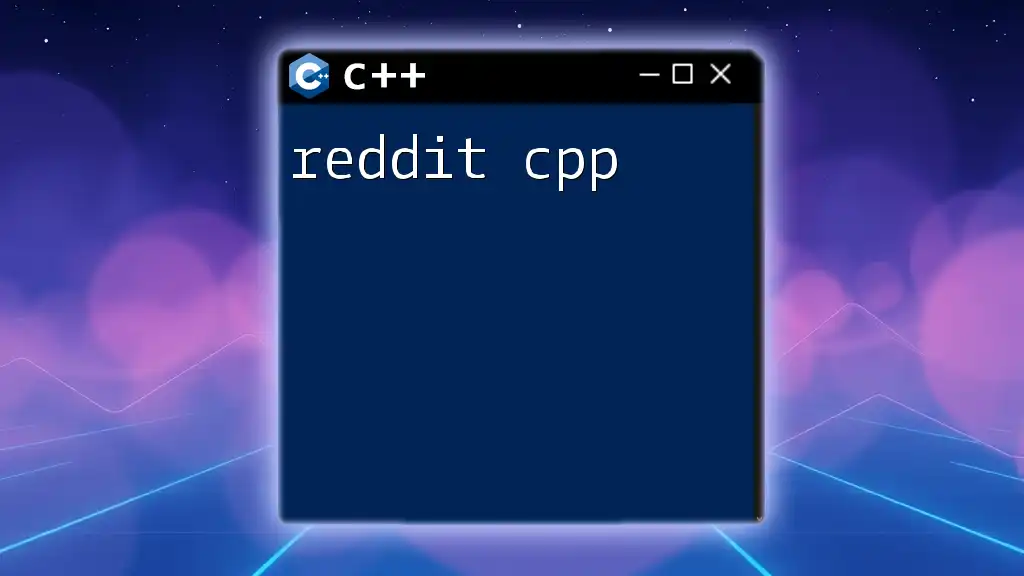
Implementing Radix Sort in C++
Basic Implementation of Radix Sort
Here's a simple implementation of Radix Sort in C++:
#include <iostream>
#include <vector>
using namespace std;
void countingSort(vector<int>& arr, int exp) {
int n = arr.size();
vector<int> output(n); // Output array
int count[10] = {0}; // Count array initialized to zero
// Count occurrences of each digit
for (int i = 0; i < n; i++) {
count[(arr[i] / exp) % 10]++;
}
// Update count[i] so that it contains actual position of this digit in output[]
for (int i = 1; i < 10; i++) {
count[i] += count[i - 1];
}
// Build the output array
for (int i = n - 1; i >= 0; i--) {
output[count[(arr[i] / exp) % 10] - 1] = arr[i];
count[(arr[i] / exp) % 10]--;
}
// Copy the output array to arr[]
for (int i = 0; i < n; i++) {
arr[i] = output[i];
}
}
void radixSort(vector<int>& arr) {
// Find the maximum number to determine the number of digits
int max_val = *max_element(arr.begin(), arr.end());
// Do counting sort for every digit
for (int exp = 1; max_val / exp > 0; exp *= 10) {
countingSort(arr, exp);
}
}
Explanation of Code Components
-
Main Function: This serves as the entry point of the program. It initializes an array of integers, calls the radixSort function to sort the array, and prints the sorted results.
-
Counting Sort Function: This function implements counting sort based on the digit defined by `exp`. It counts occurrences of each digit, computes the actual positions, and builds the output array accordingly.
-
Radix Sort Function: This function is responsible for the overall operation of Radix Sort. It determines the maximum value in the input array to find out the number of digits. It then iteratively calls the counting sort for each digit (represented by `exp`).
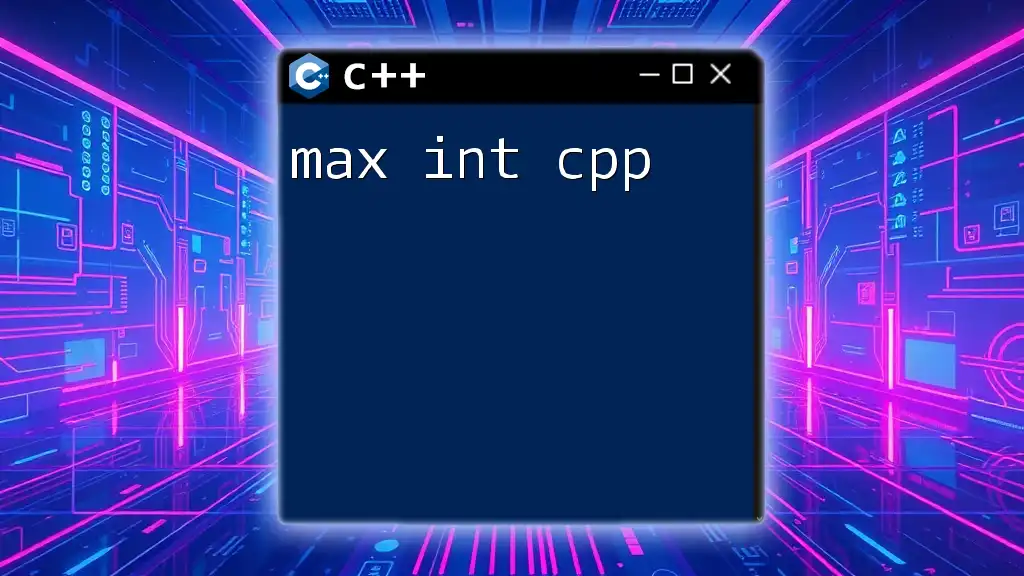
Performance Analysis of Radix Sort
Comparing with Other Sorting Algorithms
Radix Sort is particularly efficient for sorting large datasets with small digit lengths. For instance, when sorting 100,000 integers that only range up to 1,000, Radix Sort tends to outperform comparison-based sorting algorithms like Quick Sort or Merge Sort, which have an average time complexity of O(n log n).
Practical Applications of Radix Sort
Radix Sort is frequently used in applications involving sorting large volumes of integers or when input data is structured with fixed sizes and ranges. Examples include sorting IP addresses, IDs, or timestamps, where Radix Sort can efficiently manage digit-based ordering.
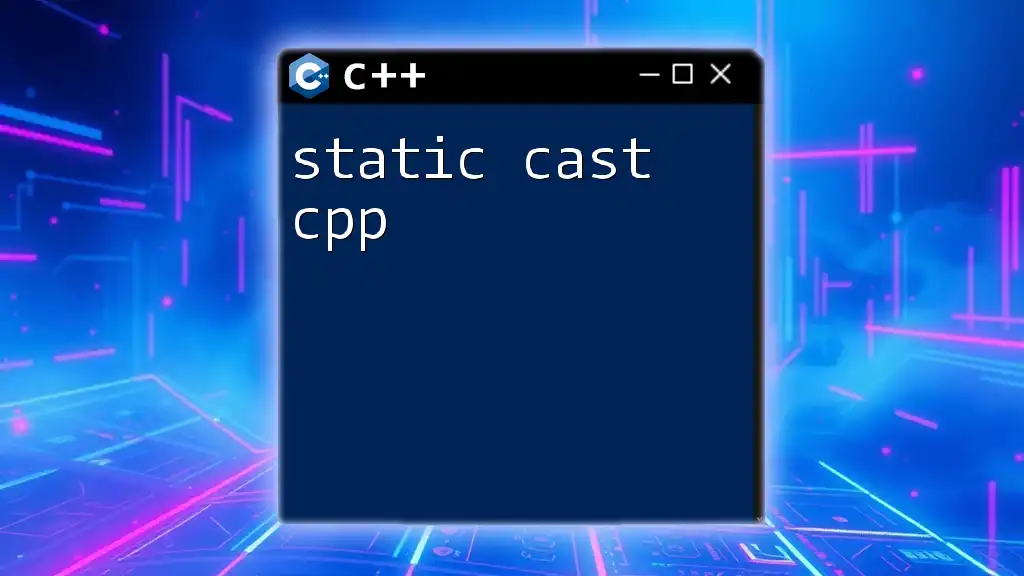
Tips for Implementing Radix Sort in C++
Common Pitfalls
-
Negative Numbers: If the dataset contains negative integers, adjustments to the algorithm will be necessary, as Radix Sort typically handles only non-negative integers.
-
Data Type Limitations: Observing the limits of integers is crucial. For large datasets, consider using data representations that can accommodate the range effectively.
Best Practices
-
Always validate the input array to ensure it meets the expected formats, especially when handling raw data that may not be sanitized.
-
Comment your code for clarity, explaining each step of the process to make it easy for others (and your future self) to understand the logic behind your implementation.
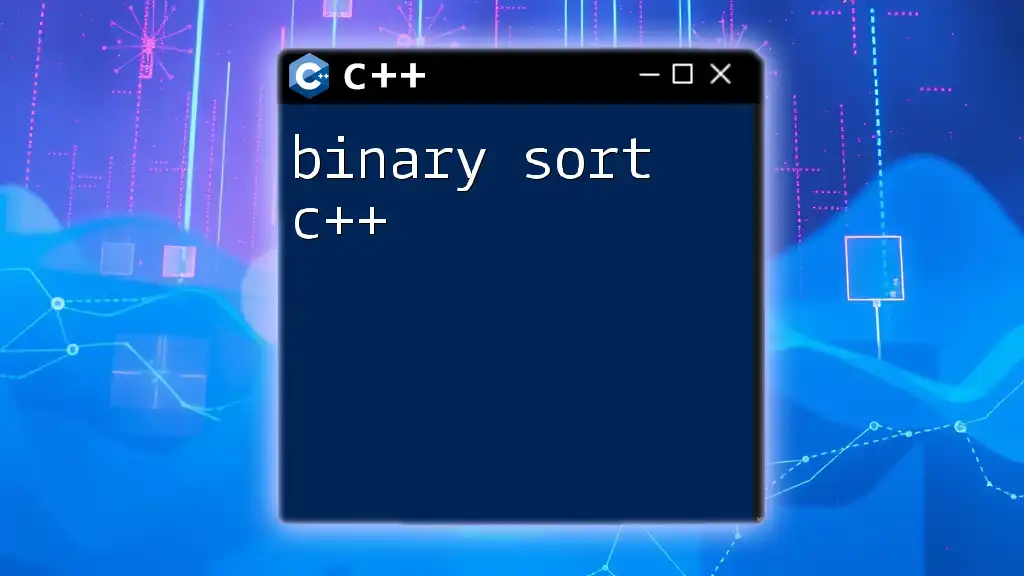
Conclusion
Radix Sort in C++ showcases the elegance of sorting without direct comparisons. By emphasizing digit-based sequencing, it affords notable speed advantages when employed in the right contexts. As with all algorithms, understanding the underlying principles is key to applying them effectively in various programming challenges.
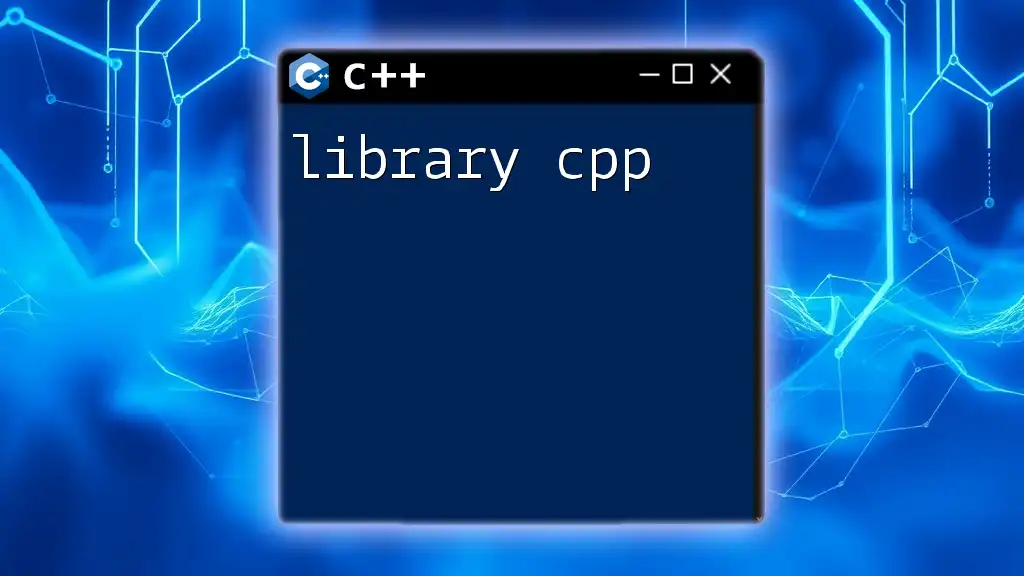
Additional Resources
To deepen your understanding of Radix Sort and sorting algorithms, consider exploring some of these resources:
- Books: Look for algorithms textbooks that cover sorting in detail.
- Online Courses: Sites like Coursera and Udacity often have courses on data structures and algorithms.
- Documentation: Familiarize with C++ libraries and their implementations of sorting algorithms.
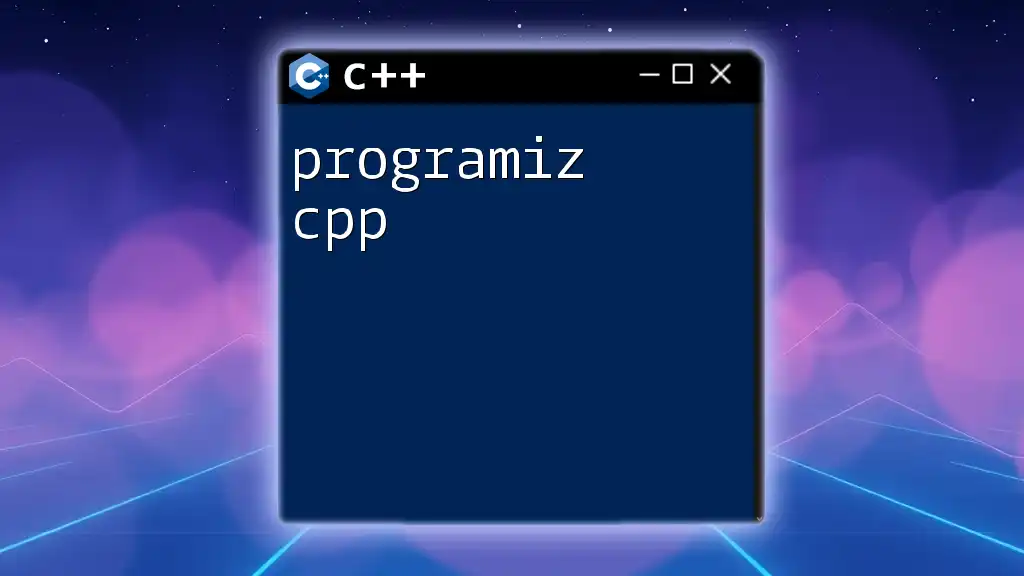
Call to Action
Now it's your turn! Try implementing Radix Sort in your own projects and see how it stacks up against other sorting methods. Don’t forget to share your insights or experiences using Radix Sort in the comments below—your feedback helps enrich the learning community!