Polymorphism in C++ allows methods to do different things based on the object that it is acting upon, typically achieved through function overriding and function overloading.
Here's a simple example demonstrating polymorphism through function overriding:
#include <iostream>
using namespace std;
class Animal {
public:
virtual void sound() {
cout << "Some generic animal sound" << endl;
}
};
class Dog : public Animal {
public:
void sound() override {
cout << "Bark" << endl;
}
};
class Cat : public Animal {
public:
void sound() override {
cout << "Meow" << endl;
}
};
int main() {
Animal* myDog = new Dog();
Animal* myCat = new Cat();
myDog->sound(); // Outputs: Bark
myCat->sound(); // Outputs: Meow
delete myDog;
delete myCat;
return 0;
}
What is Polymorphism in C++?
Definition of Polymorphism
Polymorphism in C++ refers to the ability of a single interface to represent different underlying forms (data types). It enables methods to do different things based on the object that it is acting upon, making the software more flexible and scalable. Polymorphism is a fundamental concept in Object-Oriented Programming (OOP) that allows for methods to be defined with the same name but behave differently based on the type of object calling them.
Types of Polymorphism
Polymorphism can be categorized into two main types: Compile-time Polymorphism and Runtime Polymorphism.
-
Compile-time Polymorphism occurs when the method to be invoked is determined at compile time. This is typically achieved through method overloading (defining multiple functions with the same name but different parameters) and operator overloading (providing custom behavior for operators).
-
Runtime Polymorphism, on the other hand, is resolved during runtime. This is generally implemented using virtual functions that allow derived classes to override base class methods.
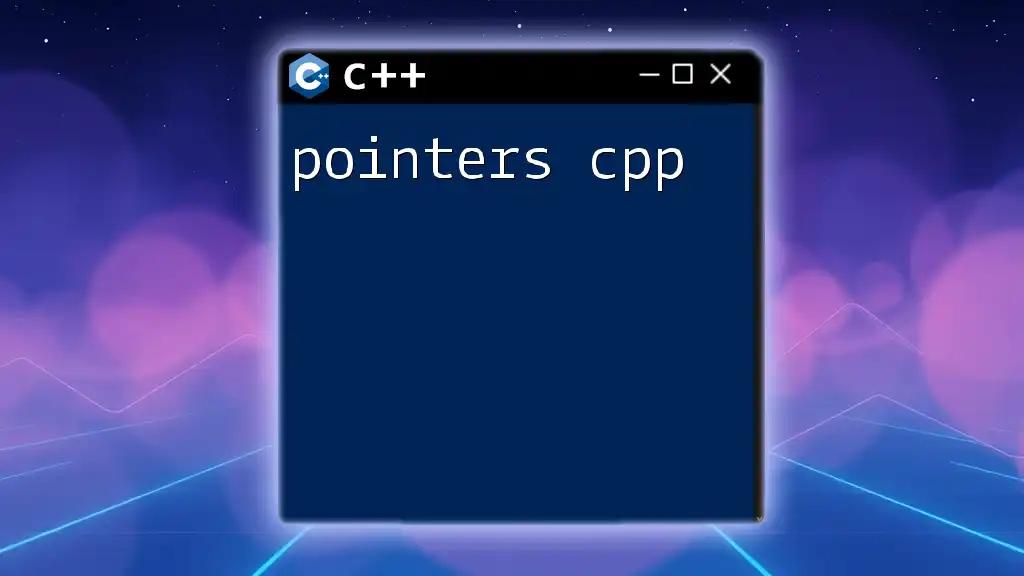
Why Use Polymorphism in C++?
Advantages of Polymorphism
Polymorphism contributes to better code organization and the facilitation of new features through code reusability, enabling developers to use existing code without modification. It provides flexibility in programming by allowing one interface for multiple implementations, thus adapting easily to change. Additionally, polymorphism helps simplify code maintenance, as changes to a method in the base class can propagate seamlessly through derived classes.
Real-World Applications of Polymorphism
In software engineering, polymorphism is widely used in design patterns such as the Strategy Pattern, where a family of algorithms is defined and selected at runtime. It is also used in creating frameworks that rely on extensibility, allowing developers to build on a common base while providing specialized functionalities.

Polymorphism in C++: Key Concepts
Inheritance and Polymorphism
Inheritance is crucial for achieving polymorphism. In C++, a base class can define virtual methods that derived classes can override. This mechanism allows a base pointer to point to a derived class object, and when a method is called using that pointer, the appropriate method for the derived class is executed.
Virtual Functions
Virtual functions are declared in a base class using the `virtual` keyword. When a derived class overrides a virtual function, the base class pointer will call the derived class's version of the function.
For example:
class Base {
public:
virtual void show() {
std::cout << "Base Class Show Function" << std::endl;
}
};
class Derived : public Base {
public:
void show() override {
std::cout << "Derived Class Show Function" << std::endl;
}
};
void display(Base* b) {
b->show();
}
In this code snippet, if we pass a `Derived` object to the `display` function, it will invoke the `show` method of the `Derived` class, demonstrating runtime polymorphism.
Pure Virtual Functions and Abstract Classes
A pure virtual function is a virtual function that has no implementation in the base class and is marked by assigning `0` to it in the declaration. A class that contains at least one pure virtual function is referred to as an abstract class. This structure forms a protocol for derived classes, which must provide implementations for all pure virtual functions.
Example:
class AbstractBase {
public:
virtual void display() = 0; // Pure virtual function
};
class ConcreteDerived : public AbstractBase {
public:
void display() override {
std::cout << "Concrete Derived Display Function" << std::endl;
}
};
Here, `ConcreteDerived` must implement the `display` function, or it will remain abstract.
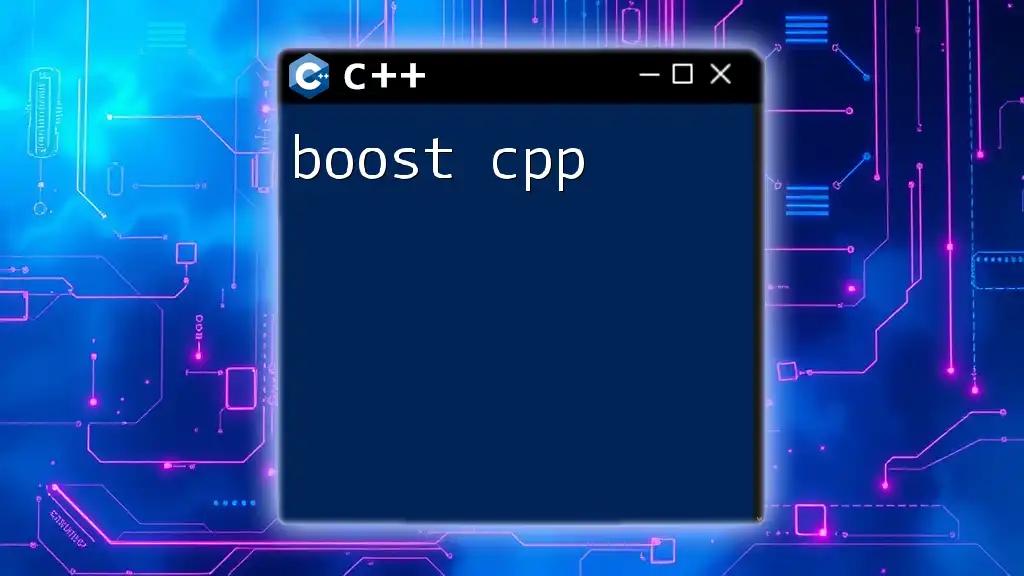
Exploring C++ Polymorphism with Examples
Example 1: Compile-time Polymorphism
Consider a simple example illustrating method overloading:
class Math {
public:
int add(int a, int b) {
return a + b;
}
double add(double a, double b) {
return a + b;
}
};
In this situation, the `add` function is overloaded for both `int` and `double` types, demonstrating compile-time polymorphism.
Example 2: Runtime Polymorphism
Building on the earlier example with runtime polymorphism, we can expand our `Base` and `Derived` class concept:
class Animal {
public:
virtual void sound() {
std::cout << "Some generic animal sound" << std::endl;
}
};
class Dog : public Animal {
public:
void sound() override {
std::cout << "Woof!" << std::endl;
}
};
class Cat : public Animal {
public:
void sound() override {
std::cout << "Meow!" << std::endl;
}
};
In this case, we can create an array of `Animal` pointers and invoke different sounds:
Animal* animals[] = {new Dog(), new Cat()};
for (int i = 0; i < 2; ++i) {
animals[i]->sound(); // Outputs: "Woof!" then "Meow!"
}
Example 3: Polymorphism in OOP Concepts
Polymorphism also integrates smoothly with other OOP principles. For instance, consider a scenario with multiple inheritance:
class Vehicle {
public:
virtual void drive() {
std::cout << "Driving a vehicle" << std::endl;
}
};
class Car : public Vehicle {
public:
void drive() override {
std::cout << "Driving a car" << std::endl;
}
};
class Bike : public Vehicle {
public:
void drive() override {
std::cout << "Riding a bike" << std::endl;
}
};
void testDrive(Vehicle* v) {
v->drive();
}
By passing different vehicle objects to `testDrive`, you can see which `drive` function gets called.

How to Implement Polymorphism in C++
Step-by-Step Guide
- Create a base class and declare virtual methods.
- Define derived classes and implement or override the virtual methods from the base class.
- Use pointers or references of the base class type to call the overridden methods in the derived classes.
Common Errors and Solutions
-
Error: Call to a non-virtual base class method.
Solution: Always ensure that the base class methods intended to be overridden are declared as `virtual`. -
Error: Object slicing occurs when a derived class object is assigned to a base class object, losing the derived part.
Solution: Always use pointers or references to manage polymorphic classes.
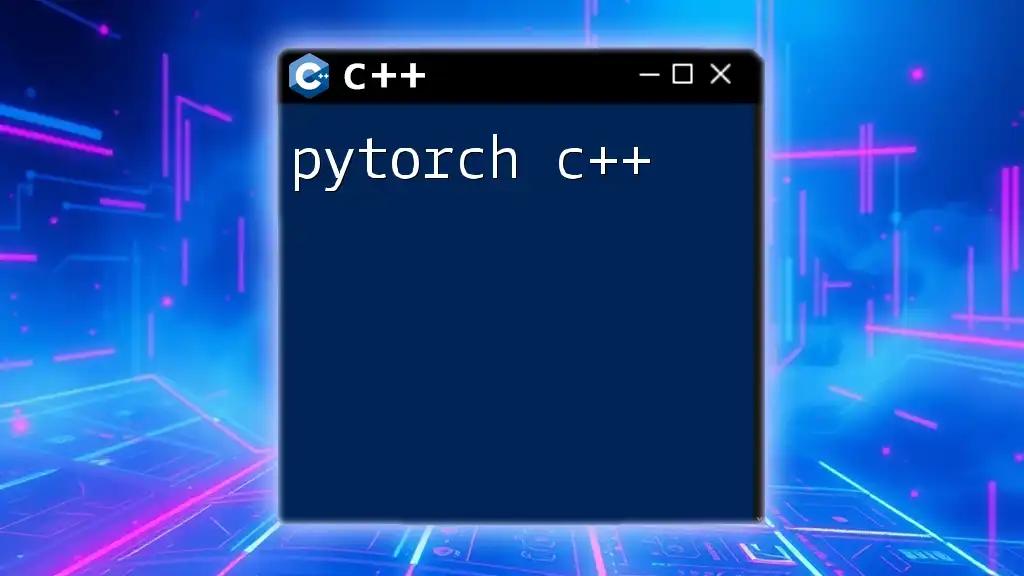
Best Practices for Using C++ Polymorphism
Guidelines for Effective Use
- Utilize polymorphism where it clarifies the design and implementation.
- Ensure that classes that implement polymorphic behavior are logically related.
Performance Considerations
Polymorphism—especially the runtime variant—can introduce overhead due to the use of virtual tables. It’s crucial to weigh the flexibility gained against potential performance impacts, particularly in resource-intensive applications.
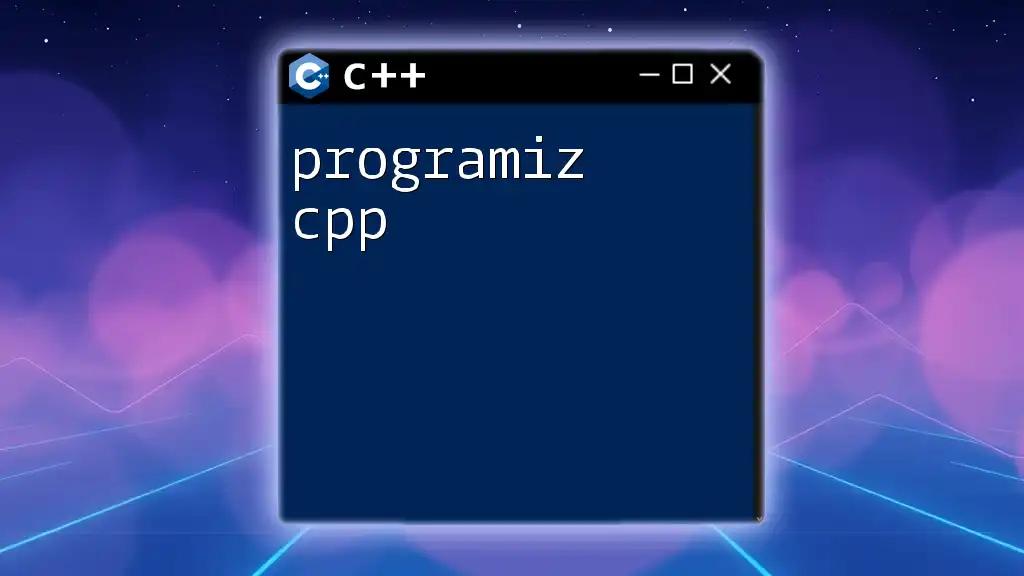
Conclusion
In summary, polymorphism in C++ is a powerful feature that enhances code flexibility and reusability. Through virtual functions, method overloading, and the use of abstract classes, programmers can create dynamic applications that are easier to maintain and extend. As you become comfortable with these concepts, you’ll be better equipped to handle complex coding challenges in C++.