Llama-cpp-python is a Python wrapper for the Llama C++ library that facilitates the implementation of machine learning models, and on Windows, you can quickly install it using pip and run a simple example as follows:
import llama_cpp
model = llama_cpp.Model('path/to/your/model')
result = model.predict("Your input prompt here.")
print(result)
Understanding Llama-CPP
What is Llama-CPP?
Llama-CPP is a powerful and efficient library designed to facilitate the use of C++ commands within Python. It acts as a bridge, allowing developers to harness the performance of C++ while enjoying the simplicity and flexibility of Python. By utilizing Llama-CPP, you can handle computationally intensive tasks more efficiently, which is essential in fields like data science, machine learning, and software development.
Why Use Llama-CPP with Python?
The fusion of C++ and Python is particularly advantageous due to several key benefits:
- Performance: C++ is known for its speed and efficiency, making it ideal for resource-intensive tasks. By integrating it with Python, you can significantly enhance the performance of your applications.
- Simplicity: While C++ can be complex, Python provides an easy-to-read syntax which allows developers to focus on logic rather than boilerplate code.
- Compatibility: Many scientific and analytical libraries in Python require optimizations that can be accomplished using C++. Llama-CPP makes it possible to integrate these optimizations seamlessly.
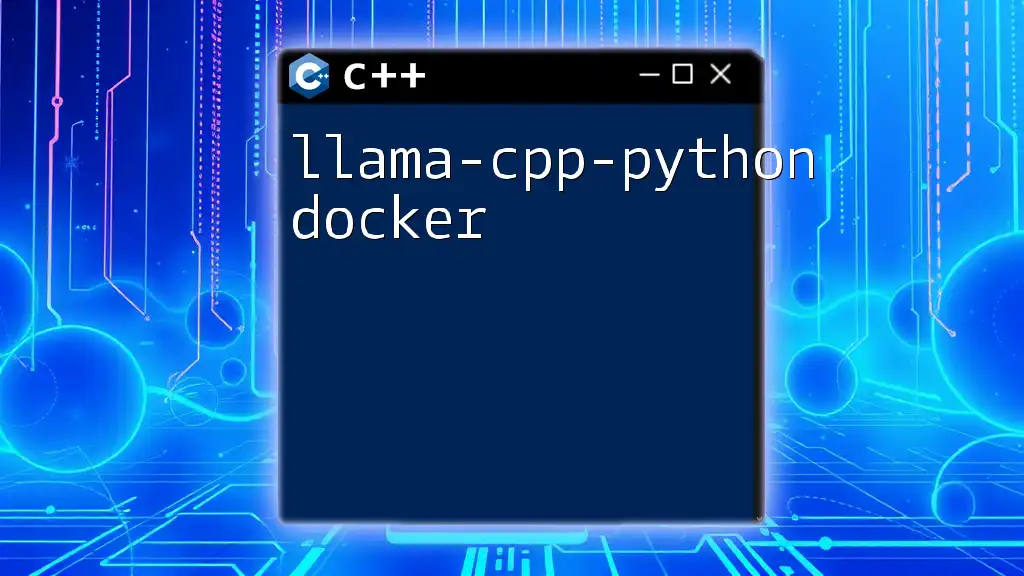
Setting Up Your Environment
Prerequisites
Before diving into Llama-CPP, ensure that you have the following software installed on your Windows machine:
- Python: Version 3.6 or higher.
- C++ Compiler: Such as MSVC or MinGW.
- pip: Python's package installer, which is bundled with Python installations.
Installing Llama-CPP on Windows
To get started with Llama-CPP, you need to download and install the library. Follow these steps:
-
Download Llama-CPP: You can download the latest version from its official repository (usually found on GitHub).
-
Install Llama-CPP: Use a terminal or command prompt with administrative rights to execute the following commands:
git clone https://github.com/your-repo/llama-cpp cd llama-cpp make install
-
Set Up Environmental Variables: If necessary, adjust your environmental variables to include the directories where the Llama-CPP executables are located.
Integrating with Python
Using pip to Install Llama-CPP
The simplest way to install Llama-CPP is through pip, which manages library installations for Python. Run the following command in your terminal:
pip install llama-cpp-python
After executing the command, you should verify the installation by importing the package in a Python shell:
import llama_cpp
If there are no errors, you have successfully installed Llama-CPP!
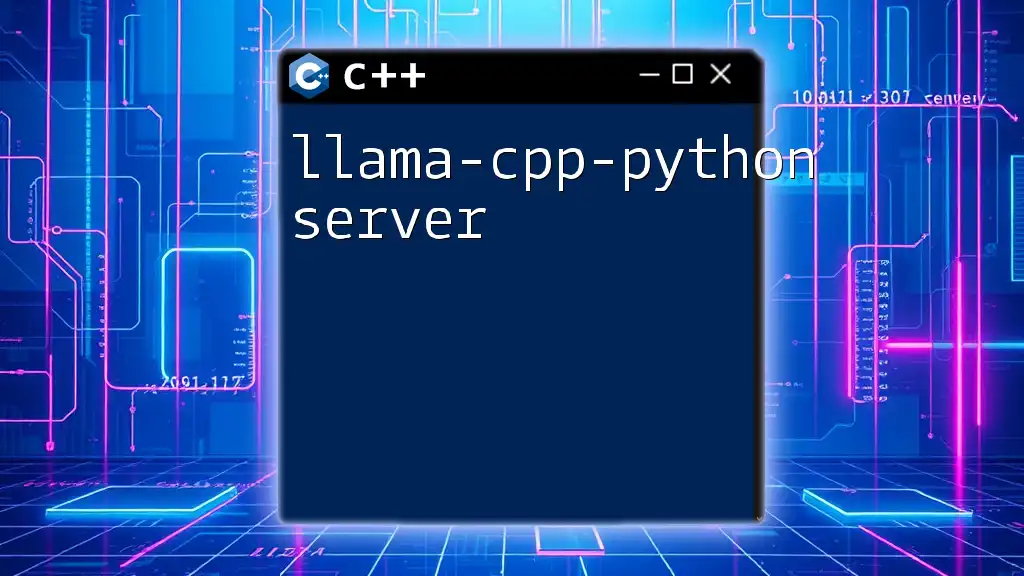
Getting Started with Llama-CPP in Python
Writing Your First Program
To illustrate how to use Llama-CPP commands, let’s write a simple program that demonstrates its functionality. Here’s a sample code snippet:
import llama_cpp
# Example function using llama_cpp
def hello_llama():
result = llama_cpp.perform_task() # Placeholder for an actual command
print(result)
if __name__ == "__main__":
hello_llama()
In this snippet:
- We import the llama_cpp module.
- Define a function, `hello_llama`, which calls a hypothetical C++ function `perform_task()`.
- Finally, we execute the function when the script runs.
Understanding Llama-CPP Commands
Llama-CPP provides several useful commands for accomplishing various tasks. Let’s explore a couple of them:
-
Command 1: `llama_cpp.command_name()`
- Description: This command interacts with a specific feature of Llama-CPP.
- Usage: Ideal for performing a particular task in a more efficient manner.
-
Command 2: `llama_cpp.another_command()`
- Description: This command serves another need, such as data manipulation.
- Usage: Use it to augment functionalities provided natively by Python.
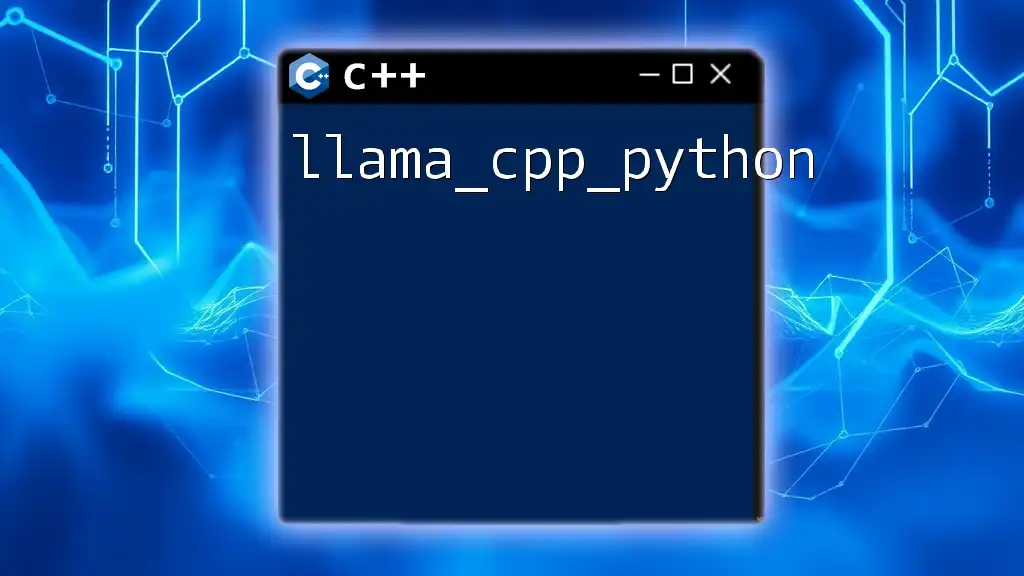
Advanced Features
Customizing Llama-CPP
One of the standout features of Llama-CPP is the ability to customize its functionality. Developers can easily define new commands that suit their needs. For instance:
// Example of a custom C++ function
extern "C" {
void custom_cpp_function() {
// Your C++ code here
}
}
Performance Optimization Tips
To maximize the efficiency of your applications using Llama-CPP, consider the following tips:
- Profile Your Code: Use profiling tools to identify bottlenecks in your Python and C++ code.
- Use Efficient Data Structures: Utilize C++ data structures that are optimized for your specific tasks.
- Leverage Multithreading: If applicable, C++ can handle multithreading more effectively than Python. Optimize performance by implementing concurrent processing.
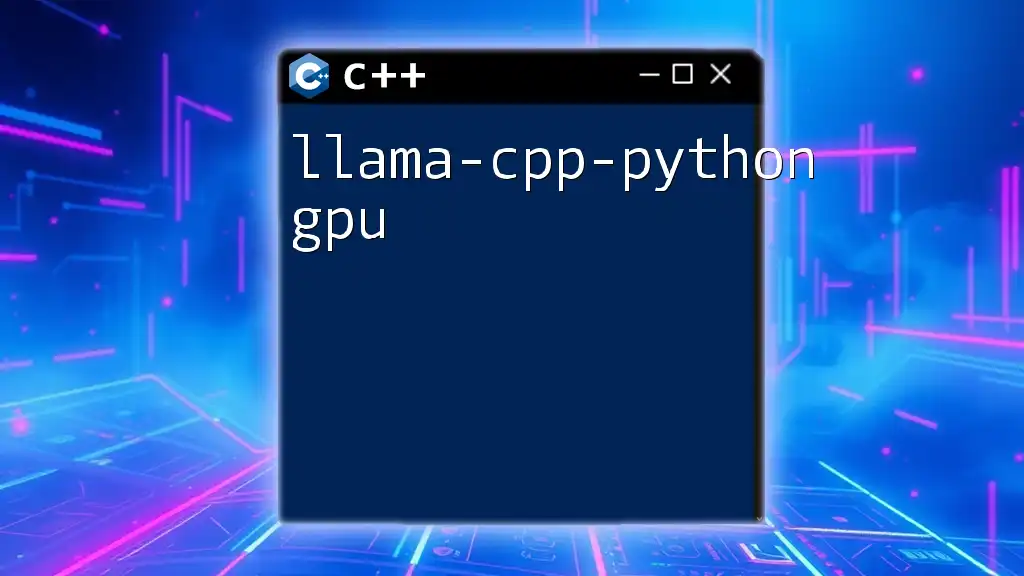
Troubleshooting Common Issues
Installation Problems
If you encounter issues during installation, common solutions include:
- Dependencies Missing: Ensure all required dependencies are installed.
- Permission Issues: Run the command prompt as an administrator.
Runtime Errors
Common runtime errors include:
- Import Errors: Verify that Llama-CPP was installed correctly and that the Python environment is configured properly.
- Function Not Found: Ensure that you are using the correct function names as defined in Llama-CPP.
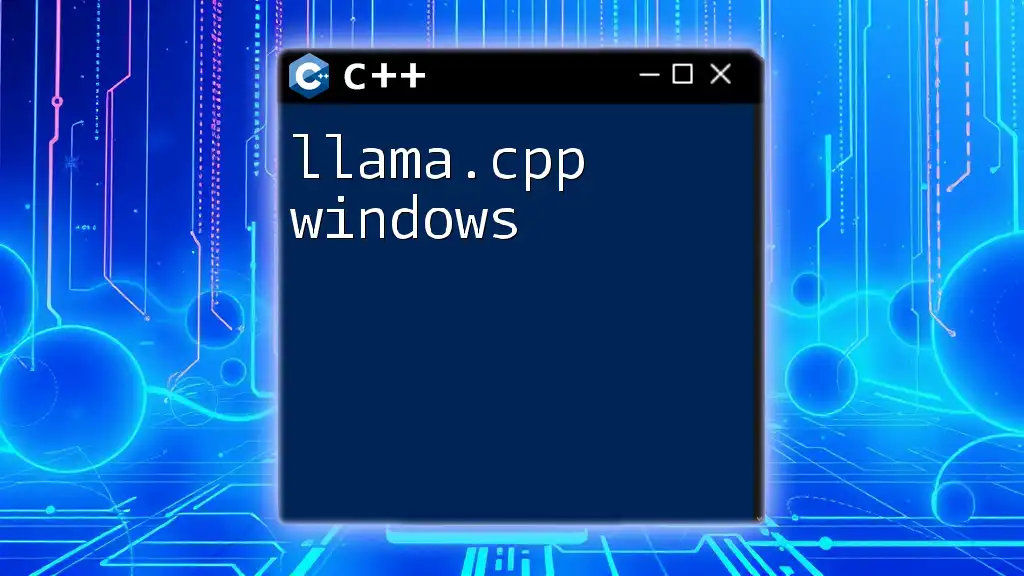
Best Practices
Writing Maintainable Code
When working with Llama-CPP, adhere to best coding practices such as:
- Clear Naming Conventions: Use descriptive names for functions and variables to enhance readability.
- Documentation and Comments: Document your code thoroughly for clarity.
Collaborating with Other Developers
Effective collaboration can be enhanced by:
- Using Version Control: Adopt Git for source code management to track changes and collaborate efficiently.
- Consistent Code Styles: Establish a unified style guide for code consistency across the development team.
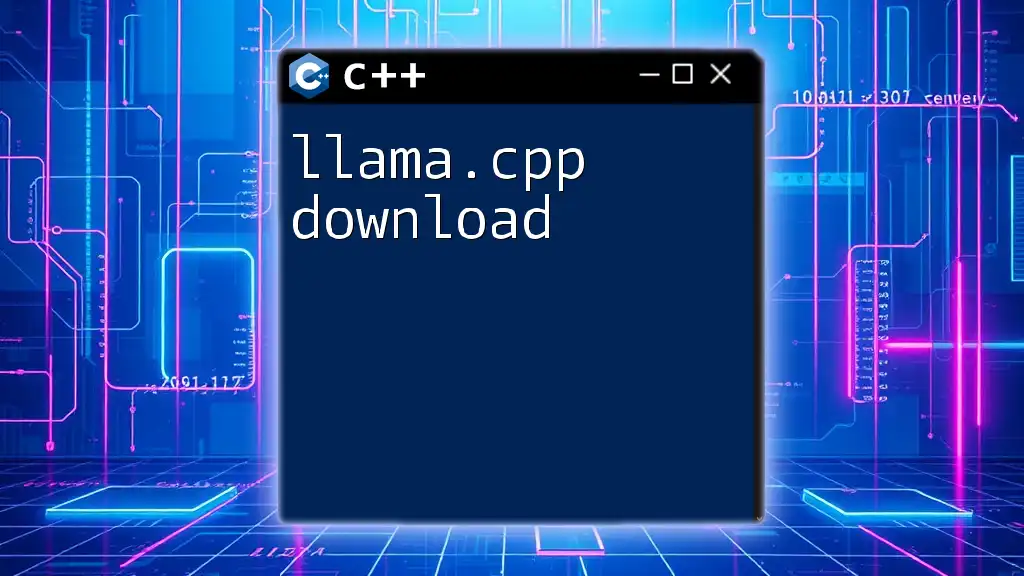
Conclusion
The integration of Llama-CPP in Python offers vast potential for performance enhancement and flexibility in software development. By following the guidance provided in this article, you are equipped to start leveraging C++ within your Python applications effectively. Explore further and share your experiences to help foster a community of innovators.
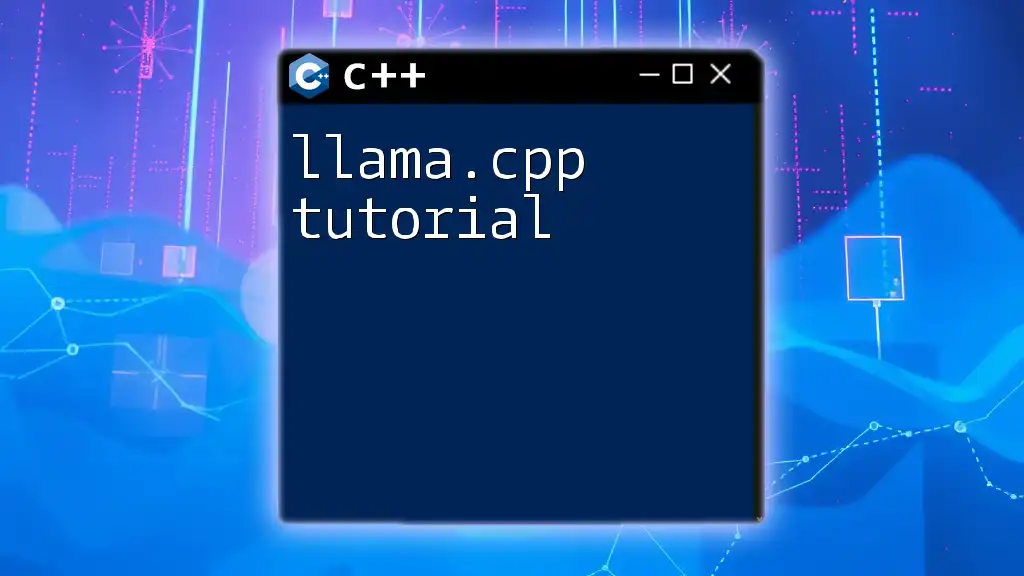
Additional Resources
For those looking to deepen their understanding, consider checking out the official Llama-CPP documentation and engaging with online communities. These resources provide invaluable support and insights that can enrich your development journey.
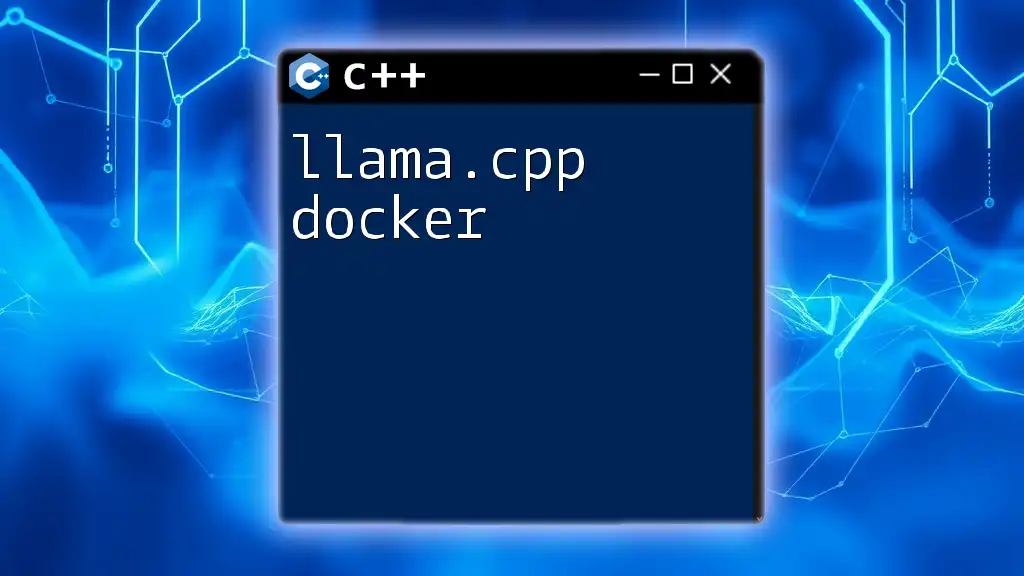
FAQs
Frequently Asked Questions
-
What is Llama-CPP? Llama-CPP is a library that enables the integration of C++ commands within Python, allowing for improved performance in computational tasks.
-
How do I install Llama-CPP on Windows? Use pip to install it with the command `pip install llama-cpp-python`, or build from source.
-
What are some common errors to expect? Errors may arise during installation or runtime, often related to missing dependencies or incorrect function names.
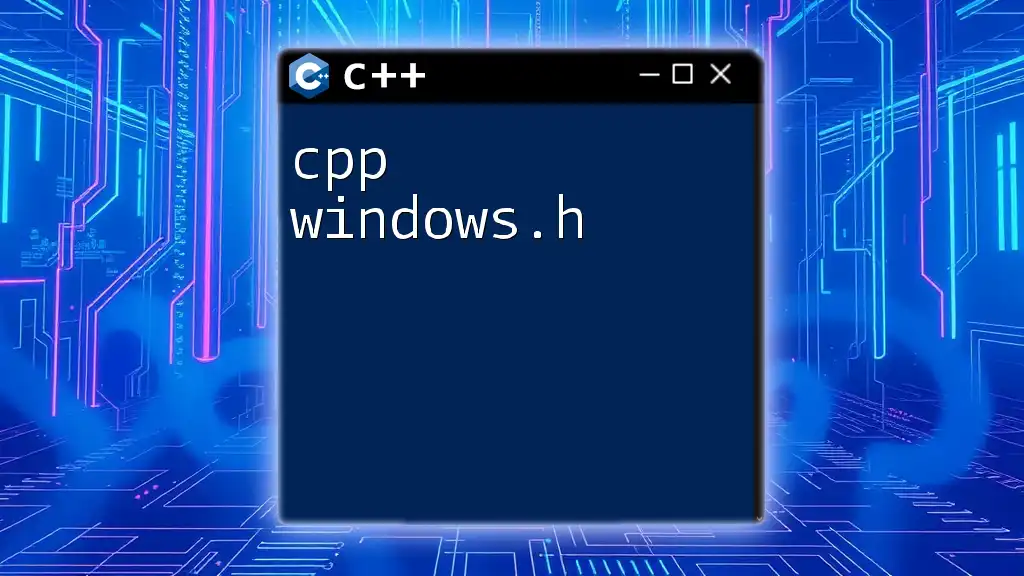
Final Thoughts
Emphasizing the importance of interoperability between C++ and Python can lead to enhanced performance and increased productivity in your projects. Take the plunge into using Llama-CPP to elevate your development capabilities, and don’t hesitate to experiment with the functionalities discussed!