In C++, `long double` is a floating-point data type that provides extended precision for decimal values, typically offering more precision than the standard `double` type.
Here’s a simple code snippet demonstrating how to declare and use a `long double` variable:
#include <iostream>
#include <iomanip>
int main() {
long double pi = 3.141592653589793238462643383279502884L; // Declare long double with high precision
std::cout << std::setprecision(50) << "Value of pi: " << pi << std::endl; // Output pi with 50 digits of precision
return 0;
}
Understanding Data Types in C++
The Importance of Data Types
In programming, data types are crucial as they define the kind of data that can be stored and manipulated within a program. Choosing the correct data type directly affects both memory usage and the performance of your application. For instance, using a type that occupies more memory than necessary can slow down your program, while selecting a type that cannot accommodate your data can lead to unexpected behavior or errors.
Overview of Floating Point Types in C++
When dealing with real numbers in C++, you have a few basic floating-point types at your disposal: float, double, and long double. Each serves a different purpose based on precision and range requirements.
- float: Typically, a 32-bit representation providing about 7 decimal digits of precision.
- double: Usually a 64-bit type that offers approximately 15 decimal digits of precision.
- long double: This type’s size can vary but is generally more than double, offering greater precision than the standard double.
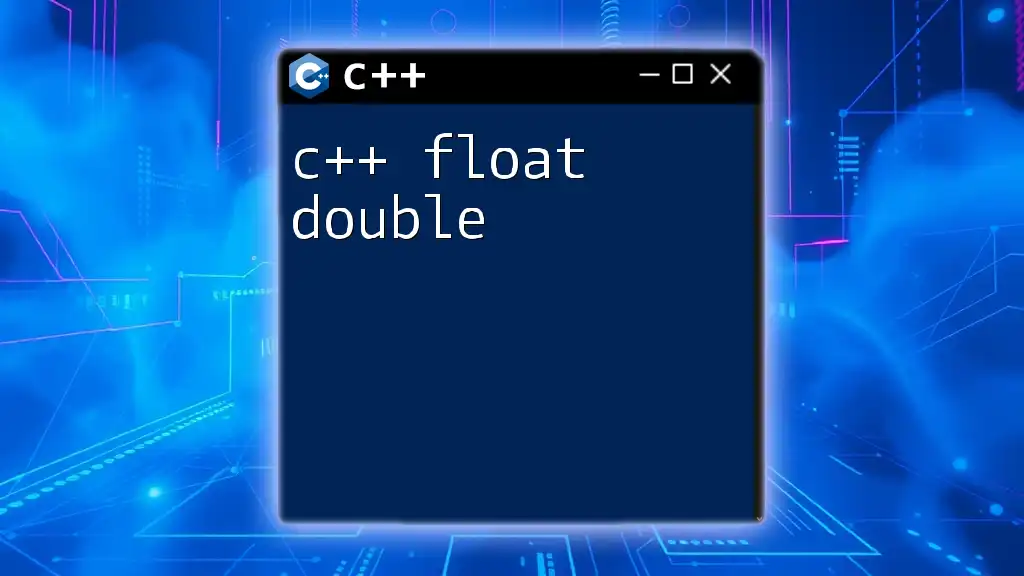
What is Long Double in C++?
Definition of Long Double
The long double data type in C++ is designed for floating-point computations that require higher precision than a regular double. The precise definition can vary by compiler, but it is generally intended for calculations that exceed the limitations of double precision.
Memory Size of Long Double
The size of the long double type typically depends on the implementation, often ranging from 80 bits (10 bytes) to 128 bits (16 bytes). This increased size allows for greater precision in calculations, making it preferable in scenarios where the precision of the result is paramount. However, be mindful that using long double may come with a performance tradeoff, especially in performance-critical applications.
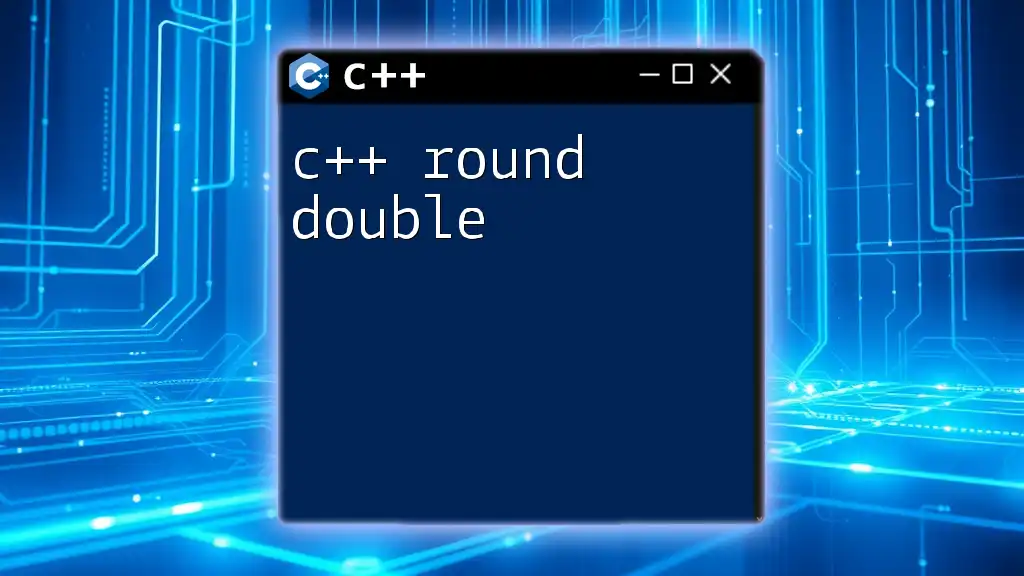
Characteristics of Long Double
Precision of Long Double
The primary distinguishing feature of long double is its higher precision compared to double. While a double can provide about 15 decimal digits, long double can provide up to 19 or more decimal digits of significant precision, depending on the compiler and architecture.
Range of Long Double
The range capability of long double can represent extremely small to extremely large values. The actual limits depend on the system, but long double often provides a range beyond that of traditional double types. For example, long double can handle values like 1.0e-4932 up to 1.0e+4932 in many implementations. This makes it beneficial in computational fields such as scientific simulations and financial calculations where exactness is critical.
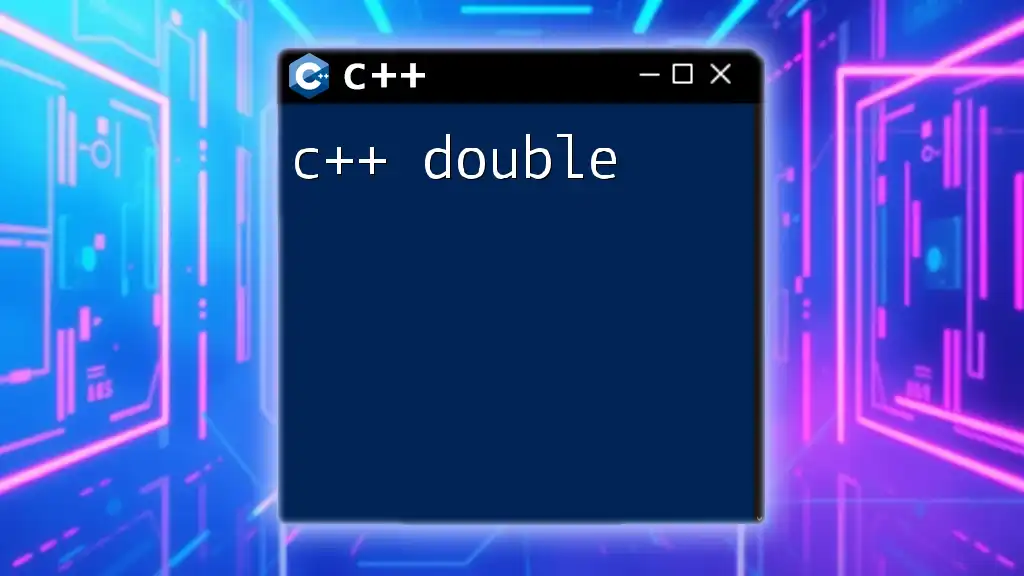
Working with Long Double in C++
Declaring Long Double Variables
To declare a long double variable, the syntax is very straightforward. Here’s an example:
long double myLongDouble = 3.14159265358979323846L;
Note the L suffix, indicating that the literal is of type long double.
Input and Output with Long Double
You can integrate long double seamlessly with standard input and output streams:
#include <iostream>
using namespace std;
int main() {
long double myValue;
cout << "Enter a long double value: ";
cin >> myValue;
cout << "You entered: " << myValue << endl;
return 0;
}
This snippet demonstrates how to read and display a long double value through the console easily.
Arithmetic Operations on Long Double
Arithmetic operations work straightforwardly with long doubles, similar to other types:
long double result = myLongDouble + 2.5L;
cout << "Result of addition: " << result << endl;
This operation maintains precision throughout the calculation, ensuring that results reflect the precision expected from a long double.
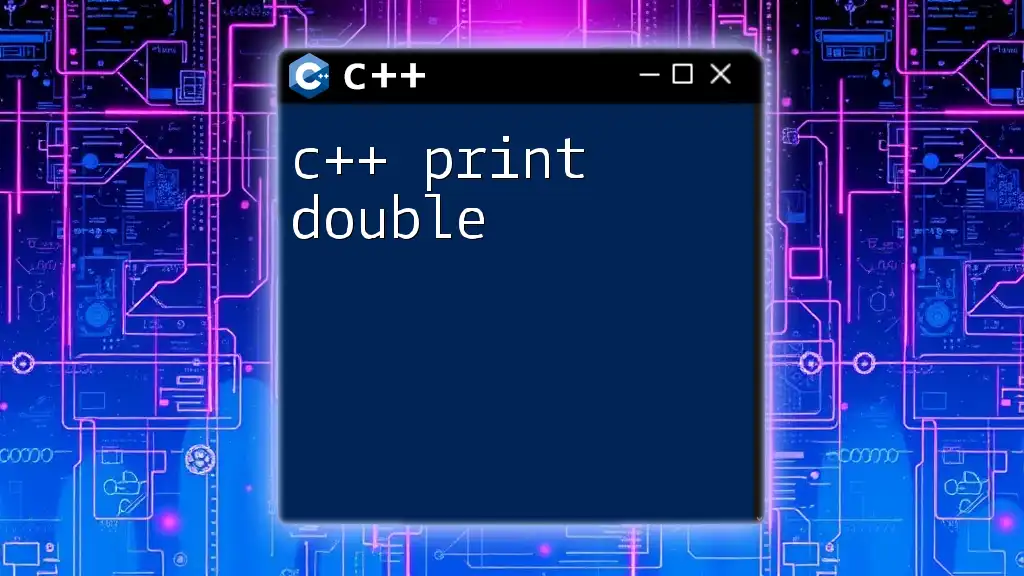
Best Practices for Using Long Double
When to Use Long Double
Before deciding to use long double, consider contexts where precision is critical. Such scenarios may include:
- Scientific computations: Simulations that model real-world phenomena with tight precision requirements.
- Financial applications: Calculations involving currency and finance that require a high level of accuracy to avoid significant discrepancies.
Performance Considerations
While long double offers advantages in precision, it also demands more memory and computing resources. Be cautious about using long double indiscriminately, particularly in performance-intensive operations. It is wise to benchmark performance in your specific use case to ensure that the precision gained justifies any computational overhead incurred.
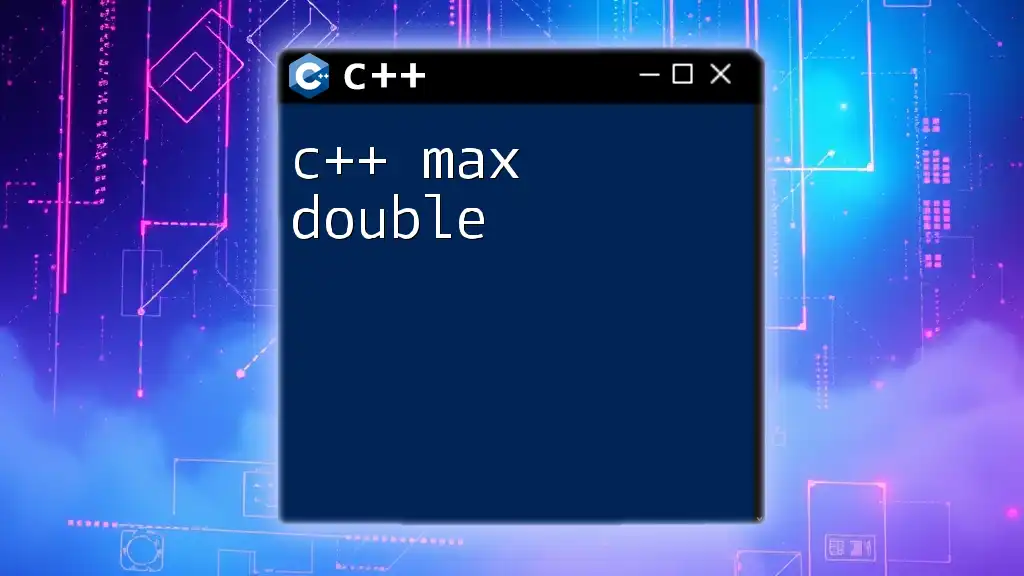
Common Pitfalls with Long Double
Implicit Type Conversions
In C++, implicit conversions between types can sometimes lead to unexpected results. For example:
int a = 5;
long double b = a; // Implicit conversion
While this works smoothly, always take care to ensure the integrity of your data, as auto-conversions may result in loss of precision in some contexts.
Comparing Long Double Values
One of the challenges with floating-point types, including long double, is comparing their values. Due to precision and rounding errors, directly comparing two long double values can yield misleading results.
For instance, instead of using:
if (a == b) {
// Some logic
}
It’s better to check with a tolerance level:
const long double epsilon = 1e-10;
if (fabs(a - b) < epsilon) {
// Considered equal
}
This method acknowledges potential small differences that may arise from floating-point arithmetic.
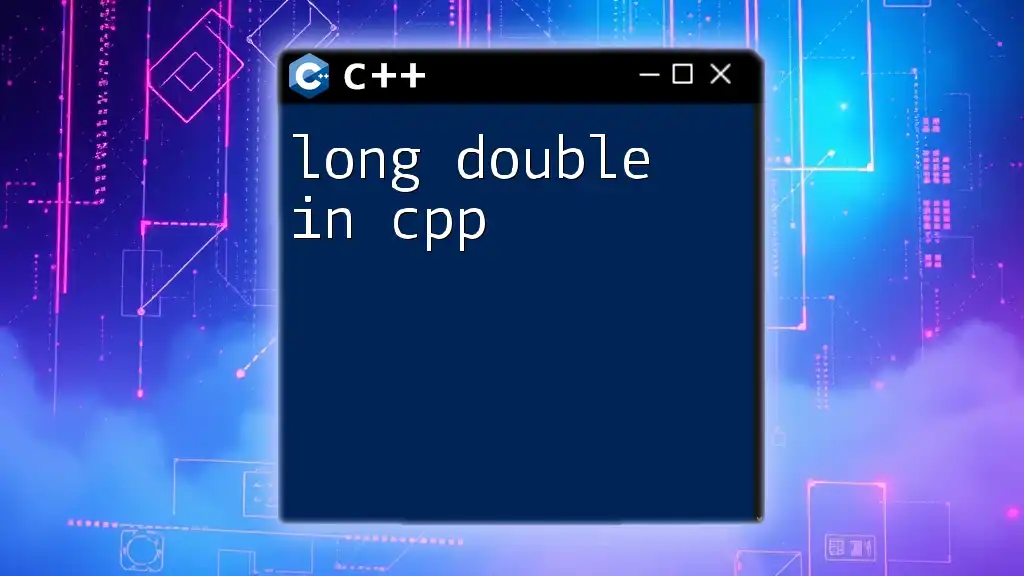
Conclusion
Recap of Long Double Benefits and Uses
In summary, the long double type in C++ is profoundly beneficial when demanding substantially high precision and a broad range of values. Where regular floating-point types might suffice in some cases, long double shines in computationally intensive fields requiring robust accuracy.
Resources for Further Learning
To deep dive into mastering C++ and floating-point types, several resources are available for additional study. Favor tutorials, books, and online courses that focus specifically on C++ to enhance your understanding and practical skills.
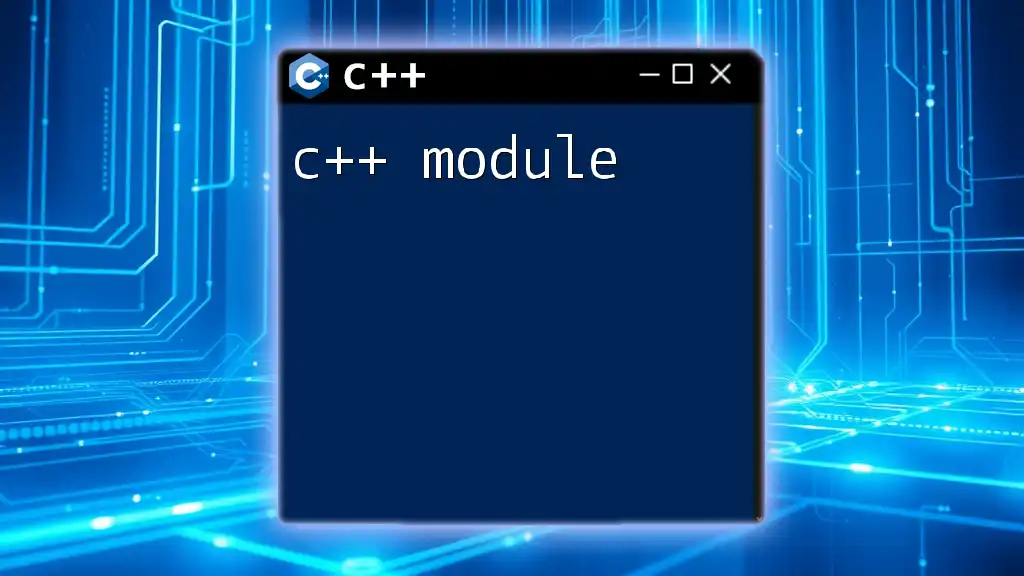
Call to Action
We invite you to join our community and explore more about C++ programming. Stay tuned for workshops and resources tailored to improve your competency with C++ commands and data types. Together, we can embark on the journey to mastering this powerful programming language!