To generate a random double value in C++, you can use the `<random>` library to create a uniform distribution between a specified range.
Here's a code snippet that demonstrates this:
#include <iostream>
#include <random>
int main() {
std::random_device rd; // Seed for random number generation
std::mt19937 gen(rd()); // Mersenne Twister engine
std::uniform_real_distribution<> dis(1.0, 10.0); // Range [1.0, 10.0]
double random_double = dis(gen); // Generate random double
std::cout << random_double << std::endl; // Output the random double
return 0;
}
Understanding Randomness in C++
What is Randomness?
In programming, randomness refers to the generation of numbers that cannot be accurately predicted. These numbers are essential in various applications, including gaming mechanics, simulations, cryptography, and statistical sampling. Having the ability to generate random values is crucial for creating more dynamic and less predictable programming environments.
Deterministic vs. Non-Deterministic Randomness
Random number generation can be categorized into two types: deterministic and non-deterministic randomness.
-
Deterministic randomness typically uses algorithms that produce repeatable sequences of numbers from an initial seed value. This means that if you start with the same seed, you will always generate the same sequence.
-
Non-deterministic randomness relies on unpredictable physical phenomena (like atmospheric noise) to generate numbers, making it less predictable. Each time you generate a number, the outcome can be different.
Using seed values effectively is crucial. They allow developers to reproduce the same sequence of random numbers for debugging purposes or testing.
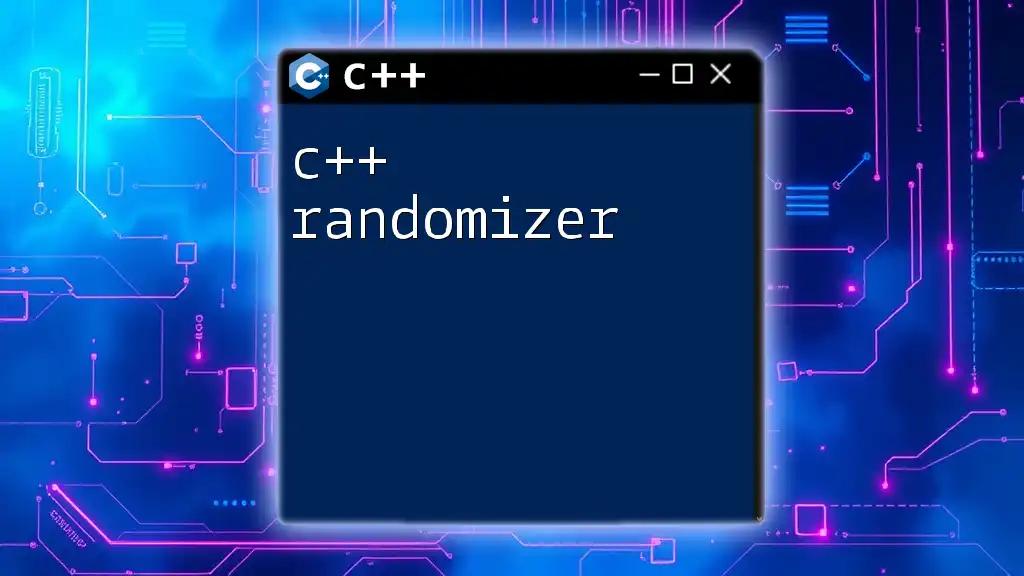
C++ Libraries for Random Number Generation
Standard Library Overview
C++ offers a comprehensive `<random>` header that provides robust tools for random number generation, allowing for more flexibility and better randomness compared to older methods like `rand()`. The use of the Standard Library is essential, as its implementations are tailored to avoid common pitfalls associated with random number generation.
Random Number Engines
Definition and Purpose
In C++, a random number engine is a complex algorithm designed to produce pseudo-random numbers. It’s the foundation upon which distributions operate, determining how the random numbers are generated.
Common Engines
Among the various engines available, two popular options are `std::default_random_engine` and `std::mt19937` (Mersenne Twister):
-
`std::default_random_engine`: A user-friendly option that employs a random number generation algorithm that optimally balances performance and quality.
-
`std::mt19937`: This engine is based on the Mersenne Twister algorithm known for its excellent random number quality and long period. Mersenne Twister is widely used in statistical computations.
Both engines allow the developer to leverage the benefits of randomness while ensuring efficiency.
Distributions
What are Distributions?
Distributions define the manner in which random numbers are generated. They establish the range and frequency of values based on specified parameters, which can be vital for simulating real-world scenarios or ensuring randomness adheres to certain probabilistic rules.
Types of Distributions
There are several distribution types in the C++ Standard Library:
-
`std::uniform_real_distribution`: Generates random numbers uniformly over a specified range. Every number within the range has an equal chance of being generated.
-
Example of Uniform Distribution
To generate random doubles uniformly within a specific range, you can use the following code snippet:
#include <iostream>
#include <random>
int main() {
std::random_device rd; // Random device for seeding
std::mt19937 gen(rd()); // Mersenne Twister engine
std::uniform_real_distribution<> dis(0.0, 1.0); // Range (0, 1)
for (int n = 0; n < 10; ++n) {
std::cout << dis(gen) << ' '; // Generate random double
}
return 0;
}
-
`std::normal_distribution`: This generates numbers based on the normal (Gaussian) distribution, often used in simulations involving real-world data where most values cluster around a mean.
-
Example of Normal Distribution
If you wish to create random doubles that follow a normal distribution, the following example illustrates how this can be achieved:
#include <iostream>
#include <random>
int main() {
std::random_device rd;
std::mt19937 gen(rd());
std::normal_distribution<> dis(0.0, 1.0); // Mean 0, Std dev 1
for (int n = 0; n < 10; ++n) {
std::cout << dis(gen) << ' ';
}
return 0;
}
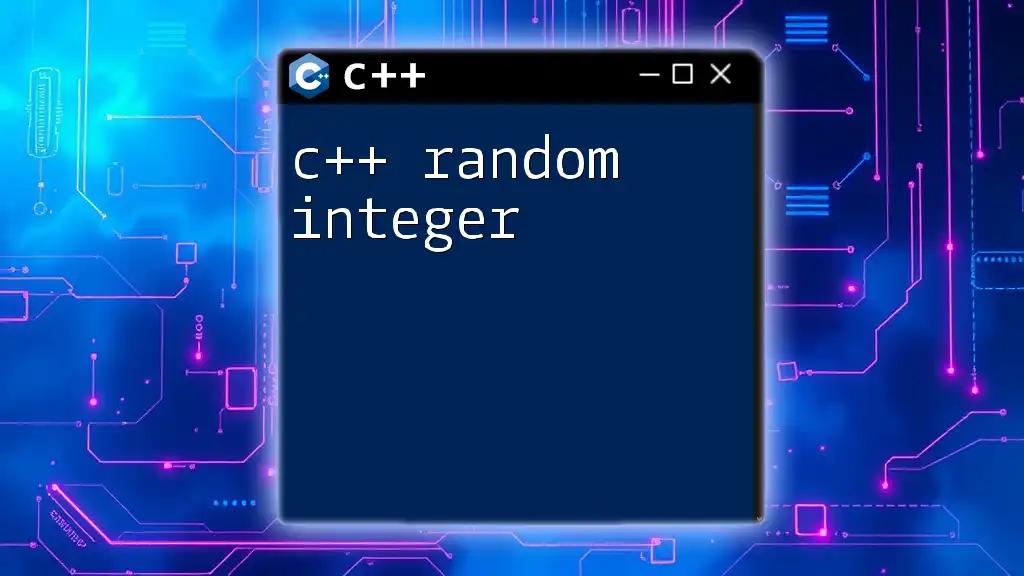
Generating Random Doubles in C++
Step-by-Step Process
To generate random doubles in C++, follow these steps:
- Setup: Include the necessary libraries by adding `#include <random>` and `#include <iostream>` for output.
- Choosing the Right Engine and Distribution: Decide on the appropriate random number engine and distribution for your needs.
Code Implementation
Here’s how to generate a random double within a specific range, say between 10.0 and 20.0:
#include <iostream>
#include <random>
int main() {
std::random_device rd; // Seed
std::mt19937 gen(rd()); // Mersenne Twister engine
std::uniform_real_distribution<> dis(10.0, 20.0); // Range (10.0, 20.0)
// Generate and print random doubles
for (int n = 0; n < 10; ++n) {
std::cout << dis(gen) << ' ';
}
return 0;
}
In this example, each time you run the code, it will print a list of random double numbers between 10.0 and 20.0. This flexibility is advantageous for diverse applications.
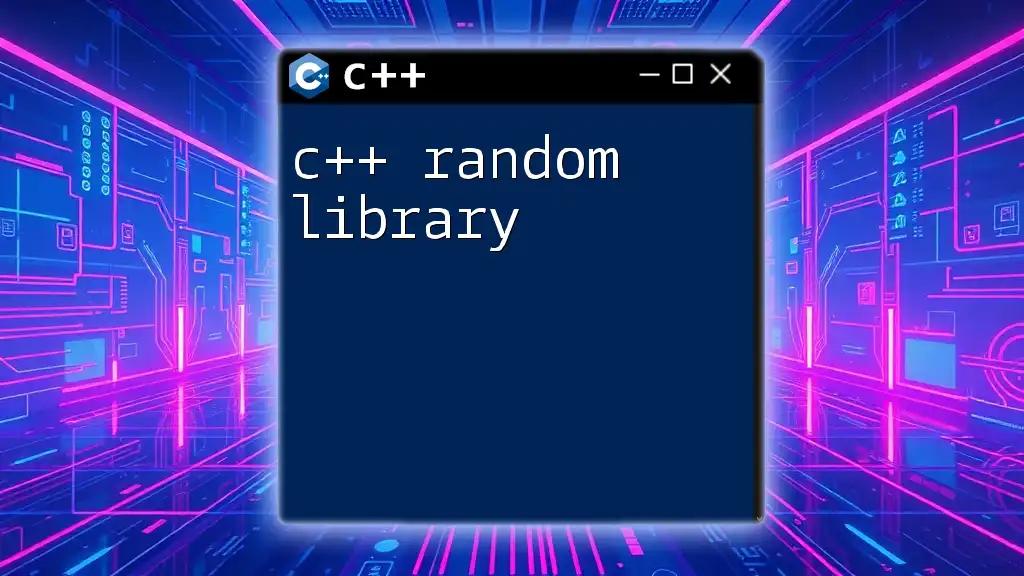
Best Practices for Random Number Generation
Seeding Strategies
To ensure that your randomness serves its purpose, unique seed values are essential. Use `std::random_device` to provide a high-quality seed, which can avoid generating predictable sequences. Reseeding might be beneficial in longer-running applications to maintain entropy.
Using Random Numbers Safely
To produce truly random outputs, it's important to avoid biases. For instance, if you require uniformly distributed values, carefully select and apply the appropriate distribution type, ensuring all segments of your range are equally probable.

Common Pitfalls to Avoid
Not Using the `<random>` Library
One of the most significant errors beginners make is relying on the outdated `rand()` function. This method lacks the quality and features offered by the `<random>` library, making it often unreliable for serious applications.
Poor Seed Management
Predictability can occur if you repeatedly use the same seed in random number generation. This restricts randomness, undermining the robustness needed in fields like cryptography and gaming.
Misunderstanding Distribution Types
Each distribution has its own mechanics and use cases. Using the wrong type can lead to incorrect assumptions about randomness. Familiarize yourself with the characteristics of each distribution to apply them effectively in your projects.

Conclusion
Mastering the generation of c++ random double values not only enhances your programming skills but also enriches the functionality of your applications. With the provided code snippets and explanations, you've learned how to integrate randomness meaningfully into your projects. Embrace these techniques and experiment with various distribution types to unlock new creative possibilities in your coding journey.

Additional Resources
For further reading and exploration, consider checking out the official C++ documentation on the `<random>` header and other resources that delve into advanced random number generation techniques. Happy coding!