In C++, a line break can be created in console output using the newline character `\n`, or alternatively by using `std::endl` to flush the output buffer.
#include <iostream>
int main() {
std::cout << "Hello, World!\n"; // Using \n for line break
std::cout << "Welcome to C++!" << std::endl; // Using std::endl
return 0;
}
Understanding Line Breaks in C++
What is a Line Break?
A line break in programming is a marker that indicates the end of a line of code and the beginning of a new one. In C++, line breaks play a crucial role in structuring your code, making it more readable and easier to understand.
Types of Line Breaks
It’s essential to differentiate between soft and hard line breaks:
- Soft Line Breaks: These occur naturally at the end of a line of code based on the developer's formatting. C++ ignores extra whitespace and newlines unless specified otherwise.
- Hard Line Breaks: These are created intentionally, often using escape sequences or formatting commands, to indicate a new line of output or a change in context.
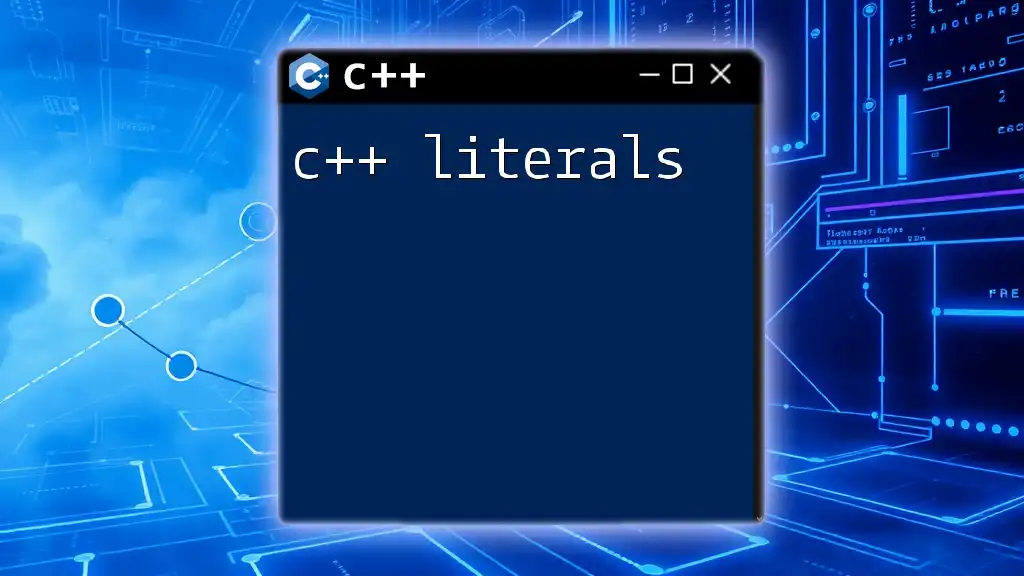
The Role of Line Breaks in C++
Syntax and Formatting
In C++, maintaining proper syntax is crucial for code execution and compilation. A well-placed line break can enhance the structure of the code, whereas a misallocated line break can lead to syntax errors. For instance, consider the following example:
#include <iostream>
int main()
{
std::cout << "Hello, ";
std::cout << "World!";
return 0;
}
In the example above, without appropriate line breaks and indentation, the code appears cluttered, making it more challenging to read. However, this changes dramatically with effective use of line breaks:
#include <iostream>
int main()
{
std::cout << "Hello, ";
std::cout << "World!";
return 0;
}
This layout is significantly easier to read and understand.
Enhancing Code Readability
Why Readability Matters
Readability is crucial in programming, especially when sharing code with others or revisiting your work. Code that is easy to read allows developers to quickly comprehend logic, spot errors, and make modifications. Poorly structured code can lead to misunderstandings and bugs, which can be frustrating and time-consuming to resolve.
Best Practices for Using Line Breaks
Effective use of line breaks can dramatically improve code readability. Here are some best practices:
- Break long lines of code into multiple lines where appropriate.
- Group related statements together using line breaks to create visual blocks of code.
- Insert line breaks before control structures (like `if`, `for`, and `while`) to enhance clarity.
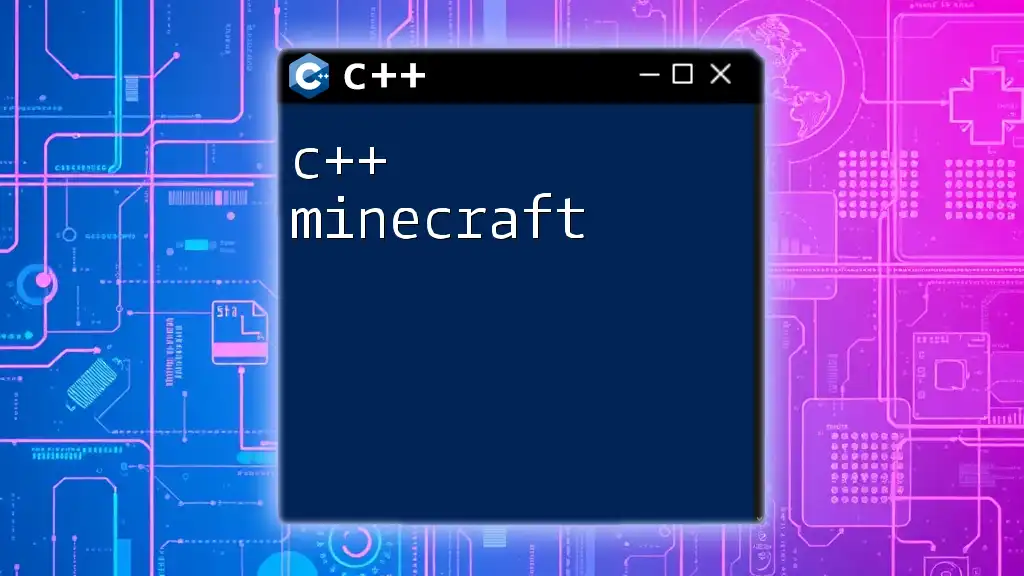
Implementing Line Breaks in C++
Using the Escape Sequence
One common way to create a line break in the output is by using the newline escape sequence, `\n`.
For example, consider the following code snippet:
#include <iostream>
int main() {
std::cout << "Hello, World!\nWelcome to C++ programming!";
return 0;
}
In this code, `\n` initiates a line break so that "Welcome to C++ programming!" appears on a new line when executed. The output will look like this:
Hello, World!
Welcome to C++ programming!
Using std::endl
Another method to introduce a line break is by using `std::endl`.
But what exactly is `std::endl`? It not only introduces a newline in the output but also flushes the output buffer. This means that the output is sent to the console immediately, which is particularly useful in debugging scenarios.
Here’s how you might use `std::endl` in your code:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
std::cout << "Welcome to C++ programming." << std::endl;
return 0;
}
While `std::endl` offers the benefit of flushing the output, it can introduce performance overhead, especially in scenarios with a large number of outputs. In contrast, using `\n` simply adds a line break without the flush.
Manual Line Breaks in Code
Breaking Long Code Lines
When dealing with lengthy expressions or statements, manually inserting line breaks can enhance readability significantly. This is especially true for calculations, long function calls, or extensive initializer lists:
double total = item1 + item2 + item3 +
item4 + item5 + item6; // Manual line break for clarity
In this example, breaking the expression across multiple lines makes it easier to understand which variables contribute to the total.
Combining Statements with Line Breaks
You can also use line breaks to delineate logical blocks of code, such as conditional statements. Consider the following example:
int a = 5;
int b = 10;
if (a < b)
{
std::cout << "a is less than b" << std::endl;
}
A clear line break before the `if` statement, combined with proper indentation within the block, enhances the visual structure of the code, making it easy for the reader to follow the logic.
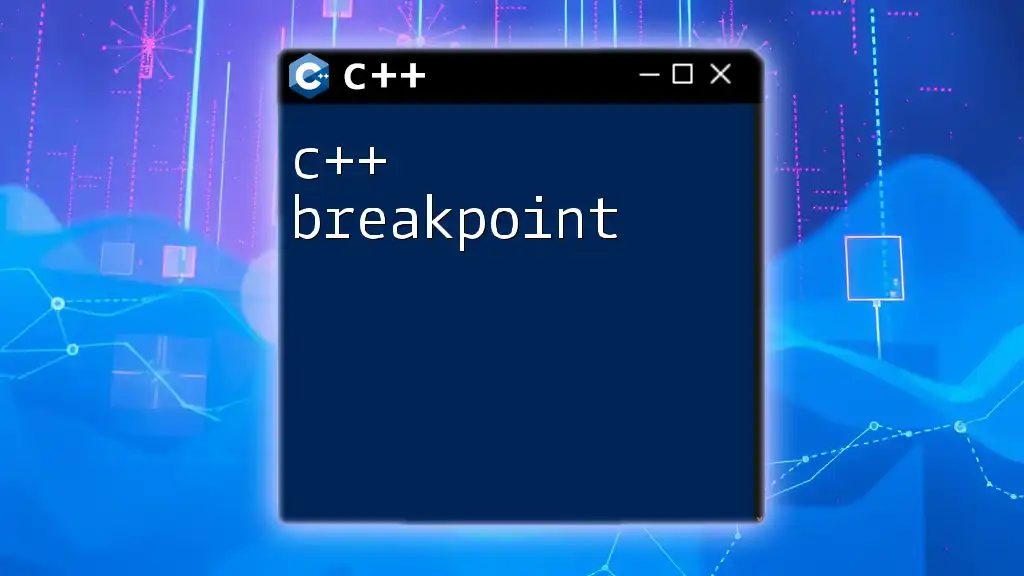
Common Mistakes and How to Avoid Them
Misusing Line Breaks
One prevalent mistake is misplacing line breaks, especially within statements that require continuity to function correctly. For instance, breaking a statement in the middle of an operation can lead to syntax errors. Always review your logic before adding line breaks.
Performance Considerations
While readability is essential, you should also be aware of performance implications. Although using line breaks generally does not affect performance, overusing commands like `std::endl` versus `\n` could. Prefer `\n` in performance-critical loops where immediate output flushing is not vital.
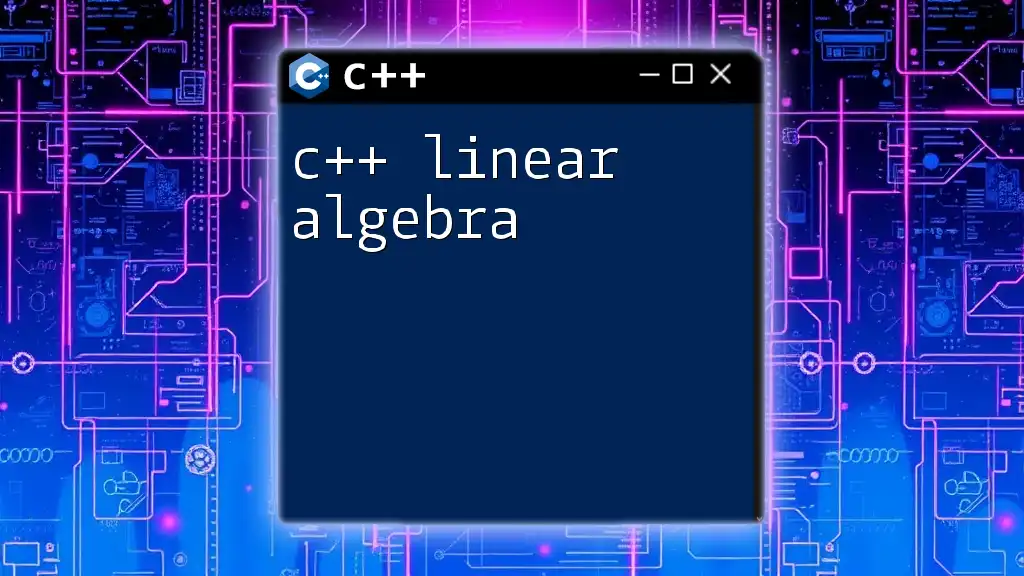
Conclusion
Mastering the use of C++ line breaks is essential for any developer aiming to write clean, efficient, and comprehensible code. By understanding the types of line breaks, their implications on syntax and readability, and the best practices for implementation, programmers can significantly enhance their code quality. Embrace the art of structuring your C++ code with line breaks—a small effort that pays off in clarity and maintainability.
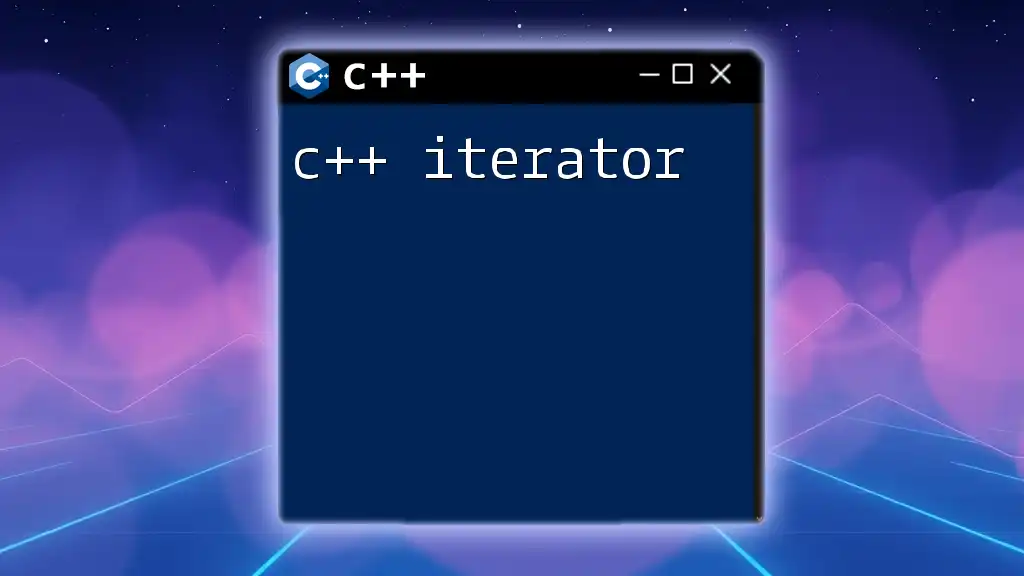
Additional Resources
For further reading, consider diving into the official C++ documentation, various coding style guides, and joining online communities focused on programming best practices. These resources will help reinforce your understanding and usage of line breaks in C++.