The C++ Linux kernel refers to the development of the Linux operating system kernel using C++ programming language features, which can enhance code organization and modularity despite the kernel being primarily written in C.
Here’s a simple code snippet that demonstrates how to use C++ features within a Linux kernel module:
#include <linux/module.h>
#include <linux/kernel.h>
class HelloWorld {
public:
void greet() {
printk(KERN_INFO "Hello, World from C++ in the Linux kernel!\n");
}
};
static struct class *hello_class;
static struct device *hello_device;
static int __init hello_init(void) {
HelloWorld hw;
hw.greet();
return 0;
}
static void __exit hello_exit(void) {
printk(KERN_INFO "Goodbye, World!\n");
}
module_init(hello_init);
module_exit(hello_exit);
MODULE_LICENSE("GPL");
MODULE_DESCRIPTION("A simple Hello World kernel module with C++");
MODULE_AUTHOR("Your Name");
Understanding the Linux Kernel
What is the Linux Kernel?
The Linux Kernel is the core component of the Linux operating system, serving as a bridge between computer hardware and software applications. It is responsible for managing system resources, facilitating communication between hardware and software, and enabling multitasking. Unlike other operating systems that might have a fragmented or proprietary kernel structure, the Linux Kernel is open-source, meaning its source code can be inspected, modified, and distributed freely, fostering a vibrant community of developers and users.
The kernel is crucial in system architecture; it controls how hardware interacts with software. Through its design, it allows for efficient management of CPU time, memory allocation, and input-output (I/O) operations, ensuring the system works smoothly.
The Structure of the Linux Kernel
The Linux Kernel consists of various components organized into a structured hierarchy:
-
Monolithic Kernel vs. Microkernel: The Linux Kernel is typically a monolithic kernel, meaning all system services run in kernel space. This contrasts with microkernels, where only essential functions run in kernel space, enhancing modularity.
-
Key Subsystems: Several critical subsystems make up the kernel:
- Memory Management: Manages allocation and deallocation of memory resources.
- Process Management: Handles executing processes and managing states.
- File Systems: Manages how data is stored and retrieved.
- Device Drivers: Interfaces with hardware devices, abstracting their complexities from user applications.
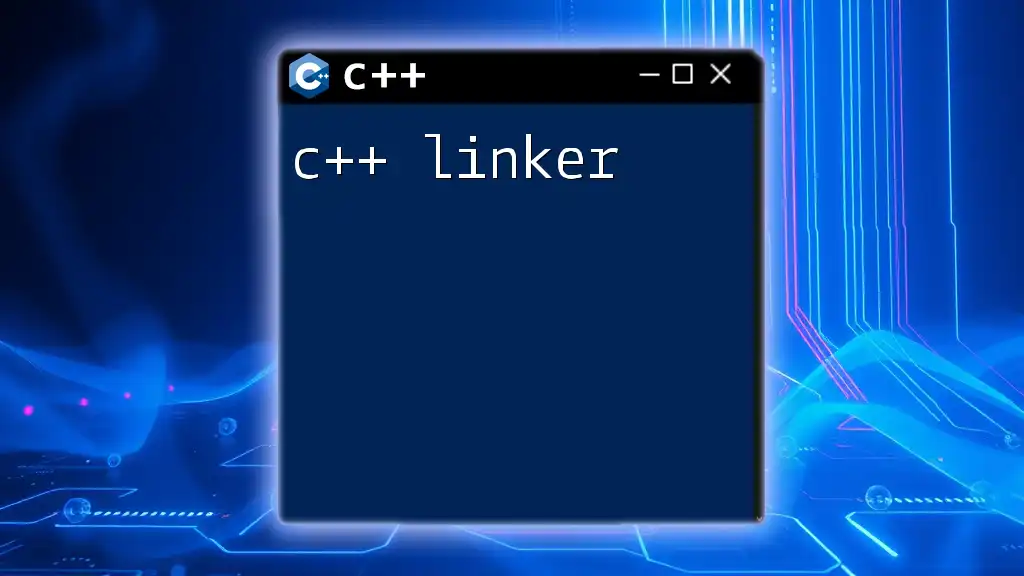
The Role of C++ in Linux Kernel Development
Why Use C++ for Kernel Development?
While the Linux Kernel is predominantly written in C, using C++ can offer several advantages due to its features:
-
Object-Oriented Programming: C++ supports object-oriented principles such as classes, inheritance, and polymorphism, which can provide a more organized and modular code structure. This can be particularly useful when designing complex systems like device drivers or kernel modules.
-
Standard Template Library (STL): C++ brings with it the STL, which provides useful classes and functions for data structures and algorithms that may simplify certain tasks.
-
Real-World Use Cases: Some subsystems or projects might leverage C++ to enhance modularity and reduce complexity. Examples include network device drivers or specific system services that can benefit from C++ abstractions.
Limitations of C++ in Kernel Development
Despite its advantages, there are notable reasons why C remains the primary language for kernel development:
-
Complexity and Overhead: C++ can introduce additional overhead, especially with its more extensive features compared to C. Using these features might result in increased code complexity and performance impacts significant in kernel space.
-
Compatibility with Existing C APIs: The Linux Kernel is built around C APIs. Using C++ can lead to complications, as the need for interoperability can introduce potential errors. Developers must have a robust understanding of both C and C++ to navigate these challenges effectively.
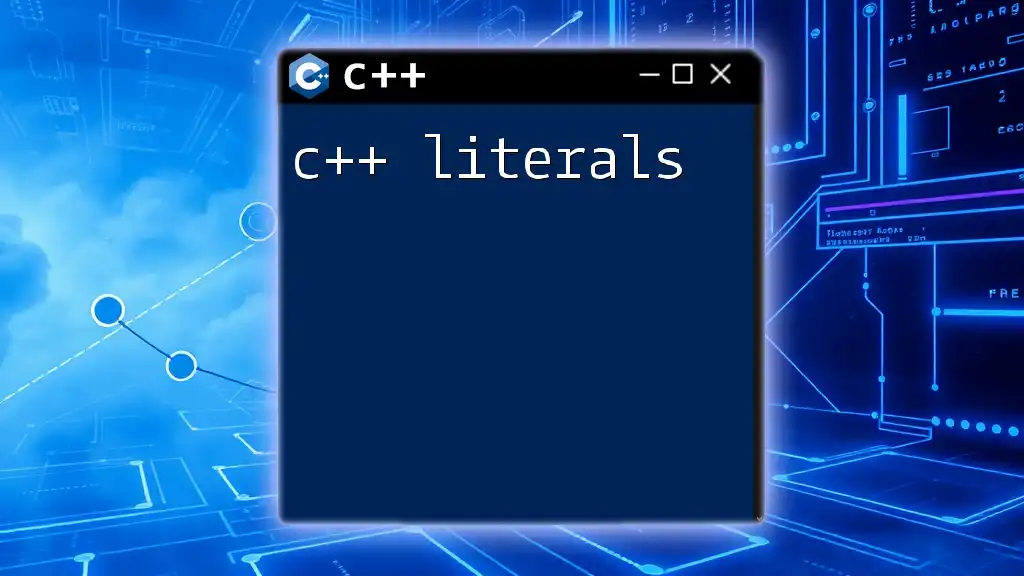
Setting Up Your Development Environment
Choosing the Right Tools
To effectively engage in C++ kernel development, selecting appropriate tools is essential:
-
IDEs and Editors: Recommended IDEs for C++ development include Visual Studio Code, Eclipse CDT, or CLion. Each of these provides comprehensive support for C++ syntax and code management.
-
Essential Build Tools: The GNU Compiler Collection (GCC) is instrumental in compiling C++ code for the kernel. Additionally, using Make helps manage build processes efficiently.
-
Configuring Debugging Tools: Debugging is critical for kernel development. Tools like GDB and specialized kernel debugging tools (like ftrace) offer insights into kernel behavior and assist in diagnosing issues.
Compiling the Linux Kernel
To start working with C++ in the kernel, you'll need to compile it. Here’s how to do it step-by-step:
- Download the Linux Kernel Source Code: Access the kernel repository.
- Navigate to the Kernel Directory:
git clone https://kernel.ubuntu.com/~kernel-ppa/mainline cd mainline
- Configure the Kernel: Use Make to configure the kernel options.
make menuconfig # Set your kernel options
- Compile the Kernel: Compile it using available CPU resources:
make -j$(nproc) # Use all available processors
This process prepares a new kernel build allowing for simple modifications in code.
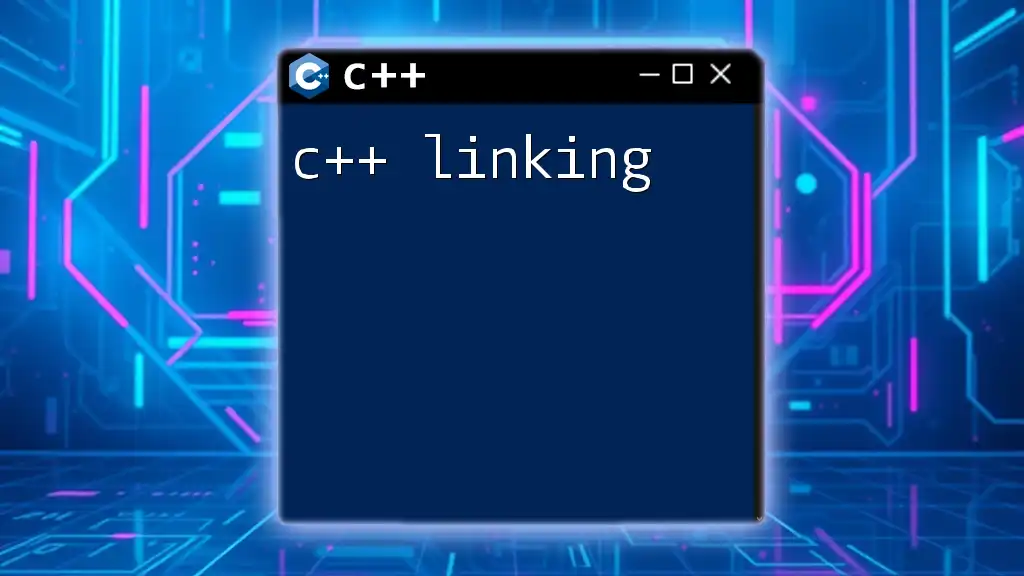
Writing C++ Code for the Linux Kernel
Key Concepts in C++ for Kernel Development
Incorporating C++ into kernel programming requires an understanding of unique paradigms:
- Memory Management in C++: Understanding pointers and references is crucial, particularly in a kernel environment where resource efficiency is paramount. Smart pointers, for instance, can automate memory management and help prevent memory leaks.
Code Snippets and Examples
A simple kernel module written in C++ might look like this:
extern "C" {
#include <linux/module.h>
#include <linux/kernel.h>
}
static int __init hello_init(void) {
printk(KERN_INFO "Hello, Linux Kernel from C++!\n");
return 0;
}
static void __exit hello_exit(void) {
printk(KERN_INFO "Goodbye, Linux Kernel from C++!");
}
module_init(hello_init);
module_exit(hello_exit);
MODULE_LICENSE("GPL");
This module demonstrates initializing and exiting a kernel module while using `printk` to log messages.
Advanced C++ Features
When delving deeper into C++, developers can take advantage of its language features. Here’s how you might create a basic device driver in C++ using classes:
class MyDevice {
public:
MyDevice(int id) : device_id(id) {}
void initialize() {
// Device initialization logic here
}
void cleanUp() {
// Clean up resources here
}
private:
int device_id;
};
In this example, MyDevice encapsulates the device's functionality and state, illustrating the object-oriented capabilities of C++.
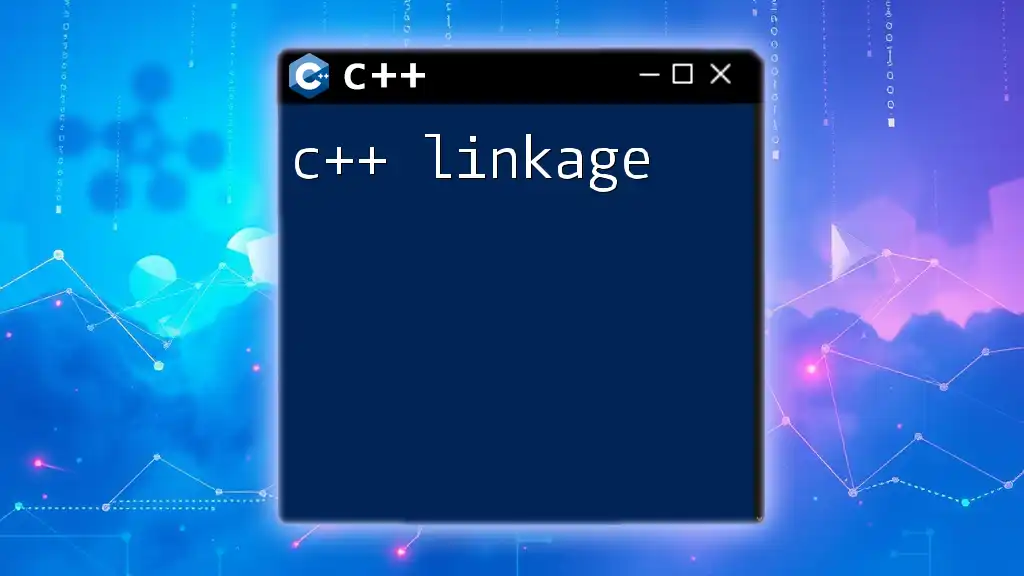
Integrating C++ with Existing Kernel Components
Working with C Libraries in the Kernel
Integrating existing C libraries into C++ code is common. Since the kernel primarily utilizes C, developers can call C functions within C++ code using `extern "C"` declarations to prevent name mangling.
Handling Compatibility Issues
To ensure your C++ code integrates smoothly with C kernel components, follow best practices such as maintaining consistent naming conventions, careful memory management, and minimal use of complex C++ features that may introduce performance overhead.
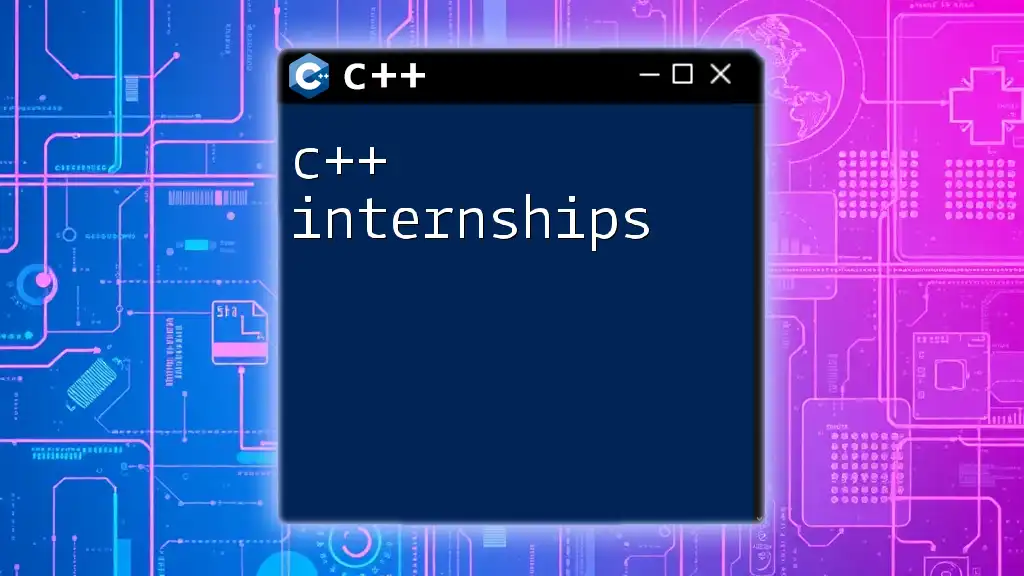
Testing and Debugging C++ Code in the Kernel
Testing Strategies for Kernel Code
Effective testing of kernel code can be categorized into unit testing and integration testing. Define test cases to validate the functionality of your kernel modules. For instance, simulate scenarios where your device drivers will be used, ensuring they handle all edge cases gracefully.
Debugging Techniques
Debugging is essential in kernel development. Here are techniques to leverage:
- Debugging with GDB: The GNU Debugger can be used to analyze kernel modules:
gdb vmlinux
- Utilizing Kernel Logs: Use print statements and monitor kernel logs via `dmesg` to understand your module's behavior and trace issues.
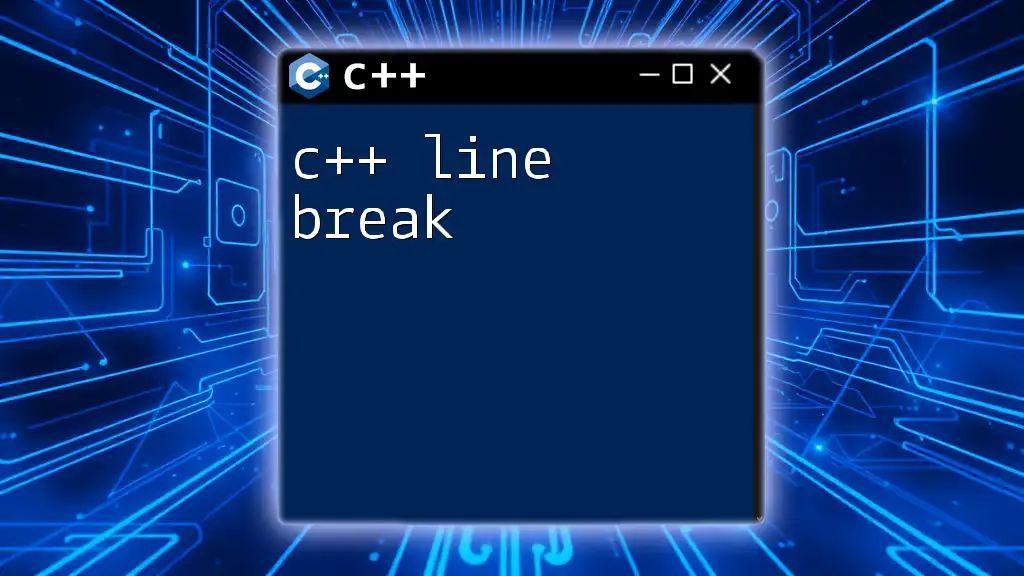
Conclusion
Using C++ for Linux Kernel development can present unique opportunities to enhance code structure and maintainability. However, it requires an understanding of both the power and limitations of C++ in a predominantly C-based environment. By exploring C++'s advanced capabilities while mastering kernel development fundamentals, you can contribute effectively to the open-source community.
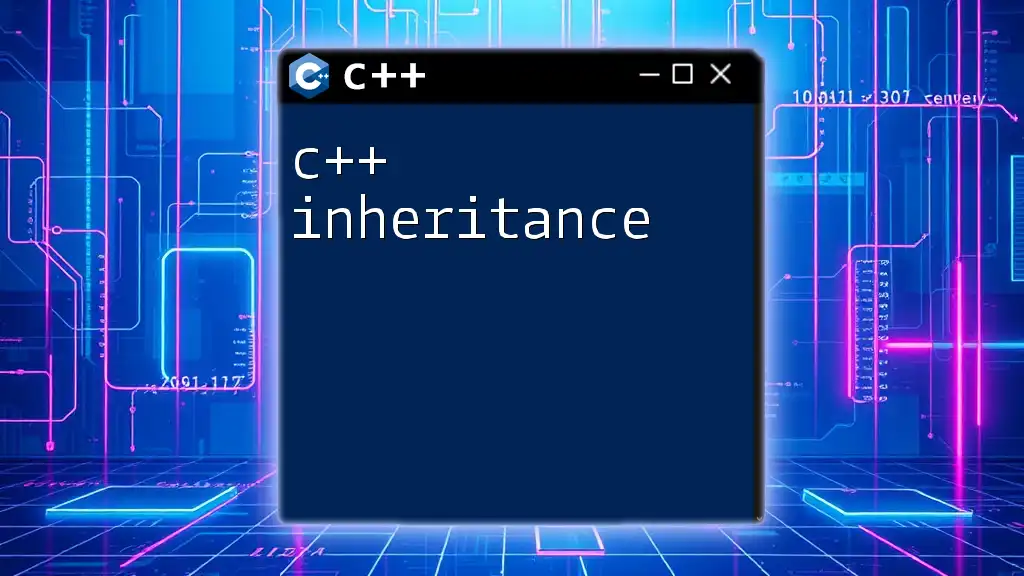
Additional Resources
To delve more into C++ and Linux Kernel development, consult the recommended books, online courses, and tutorials. The official Linux Kernel documentation is invaluable, as are community forums and groups offering support for individuals venturing into this exciting domain.