C++ libcurl is a powerful library used for transferring data with URLs, allowing developers to easily make HTTP requests and handle various network protocols.
Here’s a simple example of how to perform a GET request using libcurl in C++:
#include <iostream>
#include <curl/curl.h>
size_t WriteCallback(void* contents, size_t size, size_t nmemb, void* userp) {
((std::string*)userp)->append((char*)contents, size * nmemb);
return size * nmemb;
}
int main() {
CURL* curl;
CURLcode res;
std::string readBuffer;
curl = curl_easy_init();
if(curl) {
curl_easy_setopt(curl, CURLOPT_URL, "http://example.com");
curl_easy_setopt(curl, CURLOPT_WRITEFUNCTION, WriteCallback);
curl_easy_setopt(curl, CURLOPT_WRITEDATA, &readBuffer);
res = curl_easy_perform(curl);
curl_easy_cleanup(curl);
std::cout << readBuffer << std::endl; // Output the response
}
return 0;
}
What is libcurl?
libcurl is a powerful library that provides tools for transferring data with URLs. It supports a wide range of protocols, including HTTP, HTTPS, FTP, and more, making it an essential tool for any application that needs to perform network operations. In the context of C++, libcurl allows developers to incorporate network capabilities directly into their applications, enabling seamless communication with web services, data retrieval, and even file uploads.
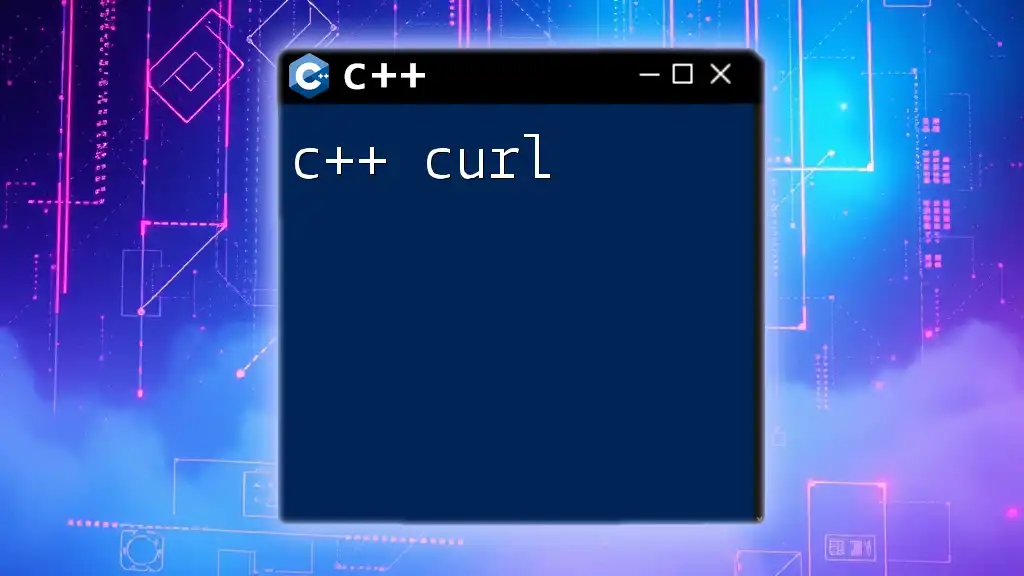
Why Use libcurl in C++?
Using libcurl in C++ is beneficial for several reasons:
- Versatility: libcurl supports numerous protocols, so developers can use it for various operations without switching libraries.
- Cross-Platform Support: Whether you are building applications for Windows, Linux, or macOS, libcurl functions consistently across these platforms.
- Asynchronous Operations: It allows for non-blocking operations, which are essential for improving the performance and responsiveness of applications.
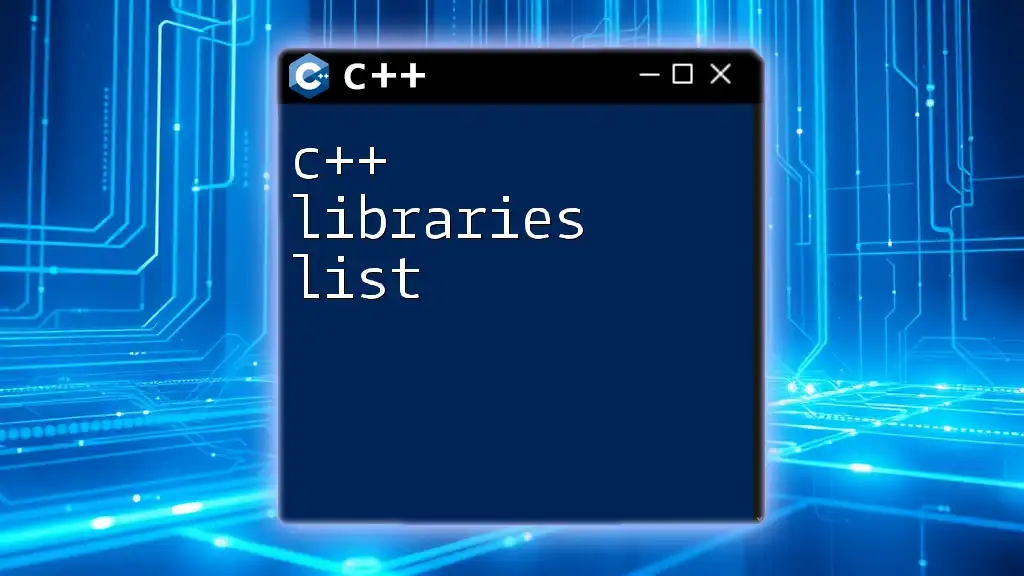
Installing libcurl
To get started with C++ libcurl, the first step is to install the library on your development environment.
Steps to Install libcurl
- For Windows: You can use a package manager like vcpkg or download the precompiled binaries from the official libcurl website.
- For Linux: Use your package manager. For example, on Ubuntu, run:
sudo apt-get install libcurl4-openssl-dev
- For macOS: The easiest way to install libcurl is via Homebrew:
brew install curl
Setting Up Your C++ Project
After installation, you need to link libcurl with your C++ project. If you are using CMake, you can add the following to your `CMakeLists.txt`:
find_package(CURL REQUIRED)
include_directories(${CURL_INCLUDE_DIRS})
target_link_libraries(your_project_name ${CURL_LIBRARIES})
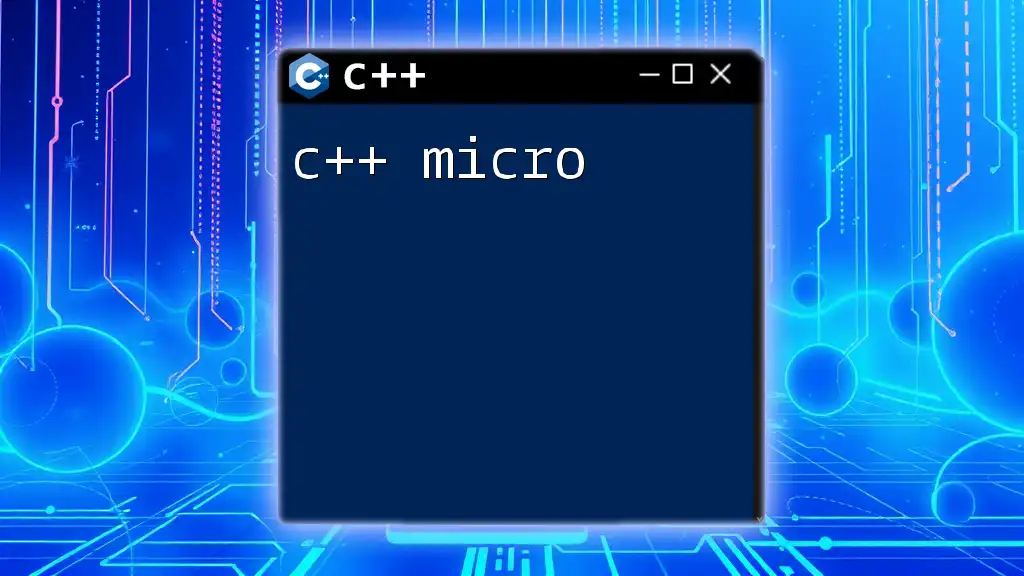
Basic Usage of libcurl in C++
Making a Simple GET Request
GET requests are the most common method for retrieving data from a server. Here’s how to make a simple GET request using C++ libcurl:
#include <curl/curl.h>
#include <iostream>
int main() {
CURL *curl;
CURLcode res;
curl = curl_easy_init();
if(curl) {
curl_easy_setopt(curl, CURLOPT_URL, "http://example.com");
res = curl_easy_perform(curl);
curl_easy_cleanup(curl);
}
return 0;
}
Explanation of the Code:
- `curl_easy_init()` initializes a CURL easy session.
- `curl_easy_setopt()` is used to specify options for the CURL session, such as the URL to fetch.
- `curl_easy_perform()` executes the request.
- `curl_easy_cleanup()` frees up resources associated with the CURL handle.
Handling Responses from GET Requests
To retrieve and process the response from the server, you'll need to implement a callback function. Here’s how you can do that:
#include <curl/curl.h>
#include <string>
#include <iostream>
static size_t WriteCallback(void *contents, size_t size, size_t nmemb, void *userp) {
((std::string*)userp)->append((char*)contents, size * nmemb);
return size * nmemb;
}
int main() {
CURL *curl;
CURLcode res;
std::string readBuffer;
curl = curl_easy_init();
if(curl) {
curl_easy_setopt(curl, CURLOPT_URL, "http://example.com");
curl_easy_setopt(curl, CURLOPT_WRITEFUNCTION, WriteCallback);
curl_easy_setopt(curl, CURLOPT_WRITEDATA, &readBuffer);
res = curl_easy_perform(curl);
curl_easy_cleanup(curl);
}
std::cout << readBuffer << std::endl; // Display the response
return 0;
}
In this example, the `WriteCallback` function captures the data returned by the server and appends it to the `readBuffer` string. This approach allows you to process the response easily.
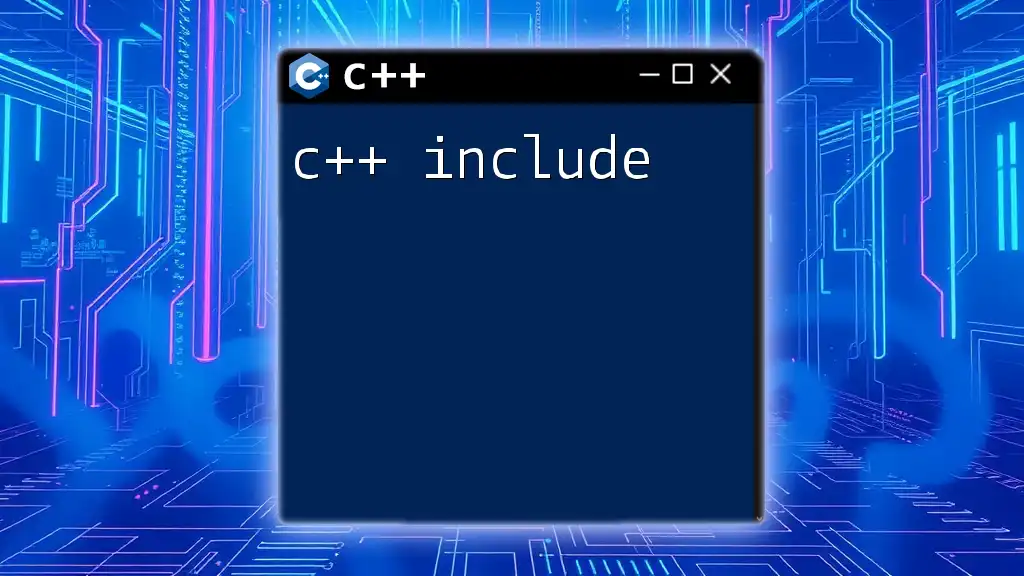
Advanced libcurl Functions
Making a POST Request
POST requests are typically used to send data to a server. Here is how you can perform a POST request using C++ libcurl:
curl_easy_setopt(curl, CURLOPT_POST, 1L);
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, "name=John&age=30");
This code sets the option to indicate that this is a POST request and specifies the data to be sent.
Using libcurl with Different Content Types
For applications that work with various data formats, you may need to set specific content types. Here’s how to send a JSON payload in a POST request:
std::string jsonData = "{\"name\":\"John\", \"age\":30}";
curl_easy_setopt(curl, CURLOPT_POSTFIELDS, jsonData.c_str());
curl_easy_setopt(curl, CURLOPT_HTTPHEADER, headers);
Ensure you set the appropriate headers for content type, such as `application/json`, to inform the server about the type of data being sent.
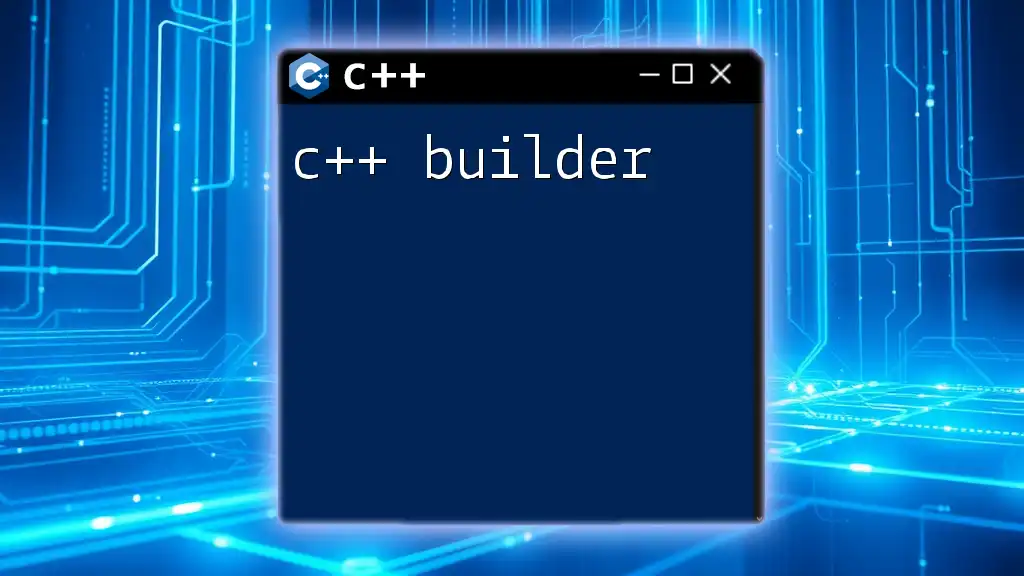
Error Handling in libcurl
Understanding curlcode and Error Management
When working with C++ libcurl, it's crucial to manage errors appropriately. The curlcode returned by functions like `curl_easy_perform()` indicates success or failure. Always check this code and handle errors gracefully:
if(res != CURLE_OK) {
std::cerr << "curl_easy_perform() failed: " << curl_easy_strerror(res) << std::endl;
}
Debugging libcurl Requests
To troubleshoot issues with requests, enabling verbose output can be incredibly helpful. You can activate verbose mode with the following line:
curl_easy_setopt(curl, CURLOPT_VERBOSE, 1L);
This will print detailed information about the request and response, which can help you diagnose problems.
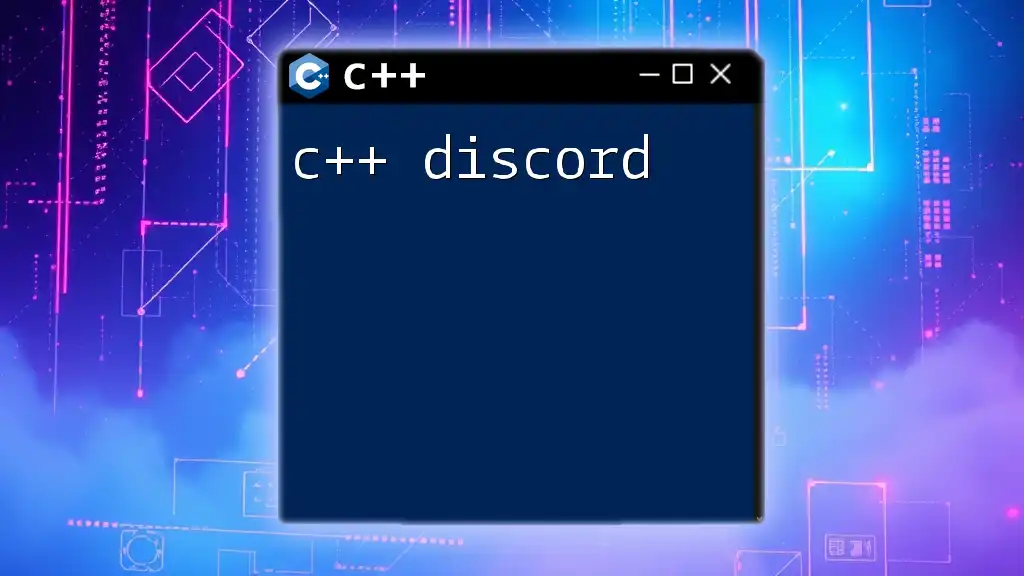
Integrating libcurl into Larger Projects
Structuring Your Code for Maintainability
When working with libcurl in larger projects, consider organizing your network operations into separate classes or functions. This makes your code more modular and easier to maintain. For example, encapsulate your GET and POST requests in dedicated classes.
Multi-threading with libcurl
Asynchronous and concurrent requests are vital for modern applications, especially when dealing with multiple URLs. You can leverage libcurl's multi interface to perform concurrent operations:
CURLM *multi_handle = curl_multi_init();
// add easy handles to the multi handle and perform requests
Using multi-threading can drastically reduce the time needed to fetch data from multiple endpoints.
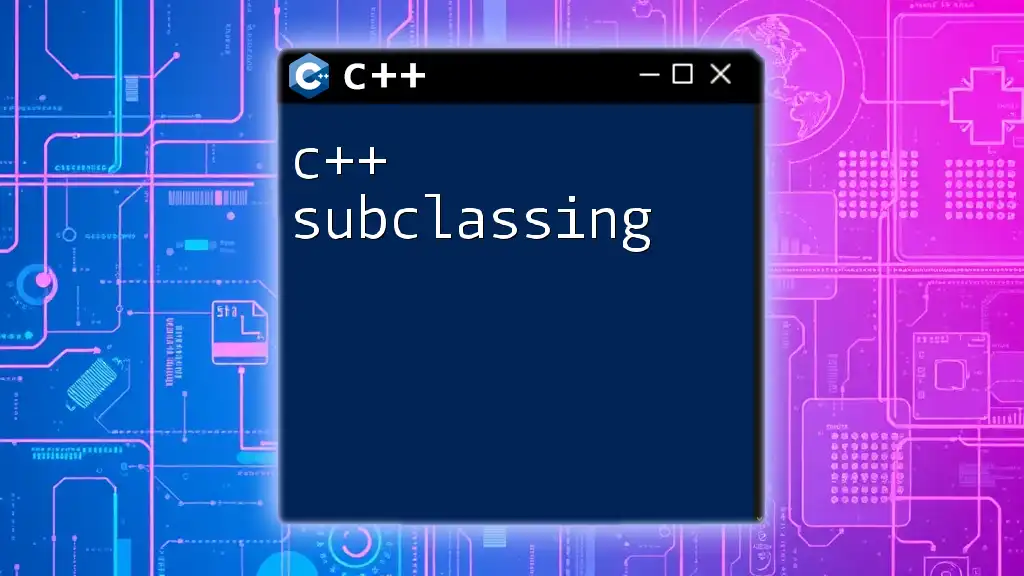
Conclusion
In this guide, we've explored the essentials of C++ libcurl, covering everything from installation to making GET and POST requests, handling responses, managing errors, and integrating libcurl into larger projects. The capabilities of libcurl empower developers to create robust applications that communicate efficiently over the web.
For those wishing to dive deeper, I encourage you to explore the official libcurl documentation and engage with community resources, enhancing your skills in C++ and libcurl!