In C++, the keywords `false` and `true` represent the boolean values used in logical operations, where `false` indicates a condition that is not met and `true` indicates a condition that is met.
Here’s a simple example demonstrating their use in a conditional statement:
#include <iostream>
int main() {
bool condition = true; // or false
if (condition) {
std::cout << "Condition is true!" << std::endl;
} else {
std::cout << "Condition is false!" << std::endl;
}
return 0;
}
Understanding Boolean Values in C++
What Are Boolean Values?
In C++, the boolean data type is represented by two values: true and false. It plays a vital role in making decisions within a program. These values can be assigned to boolean variables, making it easy to store and check conditions.
Example:
bool isActive = true;
bool isFinished = false;
In this snippet, `isActive` is assigned a value of `true`, while `isFinished` has a value of `false`. This simplicity allows for straightforward logic in code.
The Role of Boolean in C++
Boolean values significantly influence control flow in C++. They are integral to various control statements like `if`, `else`, and `switch`. By evaluating conditions and returning either true or false, these values dictate which blocks of code should execute.
Code Snippet:
bool isRaining = false;
if (isRaining) {
printf("Take an umbrella!");
} else {
printf("Enjoy your day!");
}
In this example, since `isRaining` is false, the program will output "Enjoy your day!" without suggesting the use of an umbrella.
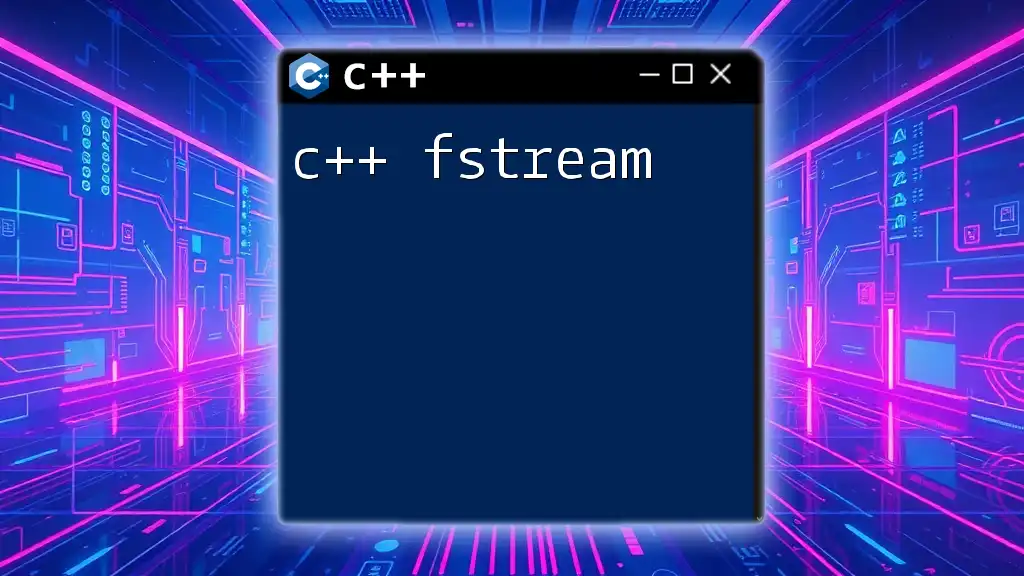
True and False in C++
Definition of True and False
In C++, the keywords true and false define the dual state of boolean variables. While the language relies on a binary system, C++ utilizes integers, where `0` corresponds to false, and any non-zero value corresponds to true. Understanding this binary representation is crucial for debugging logical errors.
Implicit vs Explicit Conversion
C++ allows for implicit and explicit conversions of non-boolean values to boolean. Implicit conversions take place automatically, while explicit conversions require a cast.
Code Snippet:
int a = 5;
bool isPositive = a; // Implicit conversion
bool isEqual = (a == 5); // Explicit check
In the first line, since `a` is non-zero, `isPositive` becomes true. Conversely, `isEqual` evaluates to true based on a direct comparison.
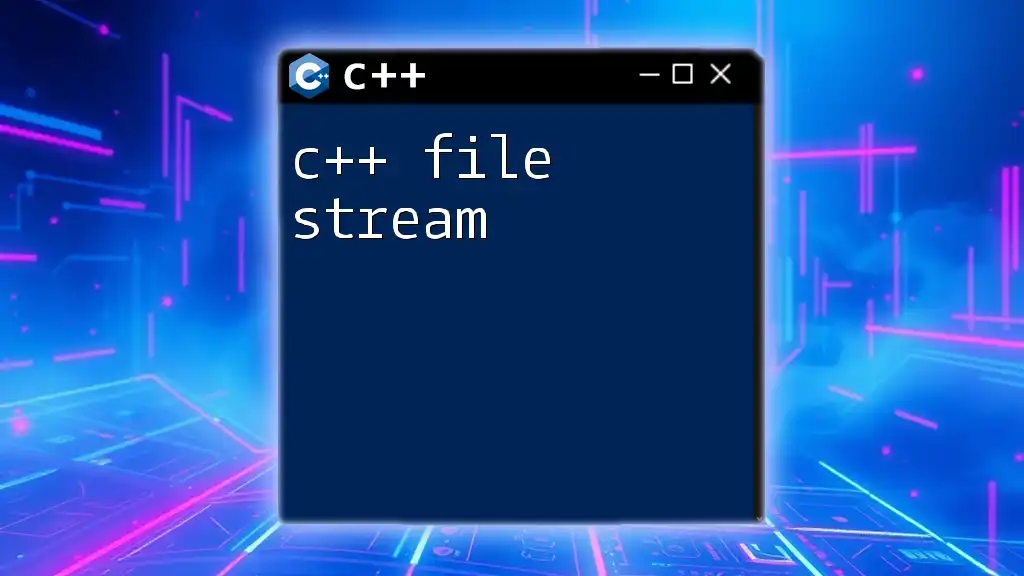
Using True and False in C++: Common Practices
Boolean Expressions
Boolean expressions are crucial for evaluation in decision-making scenarios. These expressions may involve one or more conditions leading to a boolean outcome.
Example:
int x = 10;
int y = 20;
bool isEqual = (x == y); // Using the equality operator
bool isGreater = (x > 5) && (y < 25); // Logical AND
In this example, `isEqual` evaluates to false, while `isGreater` evaluates to true.
Control Flow with True and False
True and false control the program's flow, especially in loops and conditional statements. Understanding their behavior enables efficient code execution.
Code Snippet:
bool continueRunning = true;
int count = 0;
while (continueRunning) {
count++;
if (count >= 5) {
continueRunning = false; // Set to false to stop the loop
}
}
printf("Loop executed %d times\n", count);
Here, the loop runs until `count` reaches 5, demonstrating how boolean values maintain control over repetitive actions.
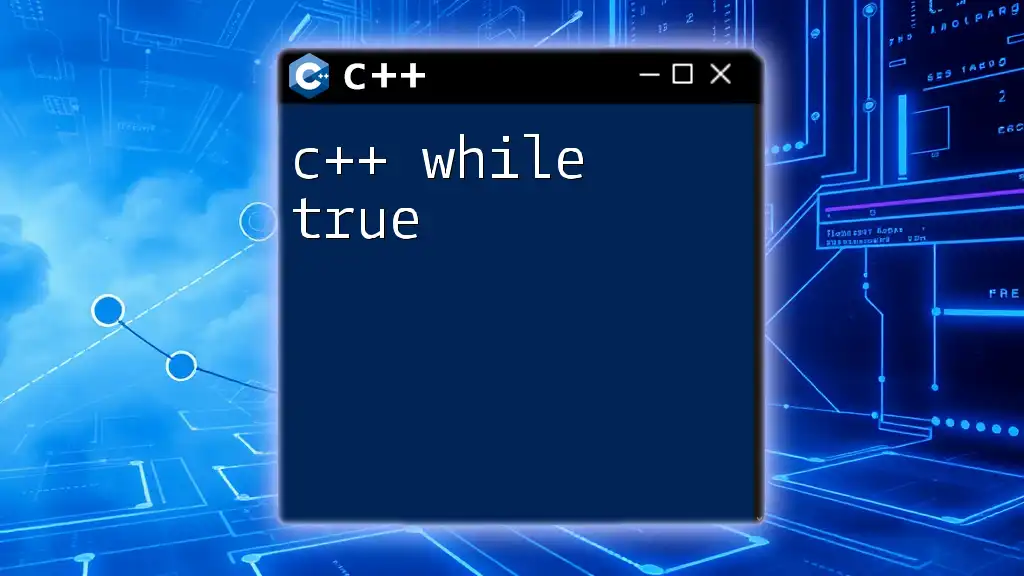
C++ True and False with Logical Operators
Logic Operations in C++
C++ provides logical operators: `&&` (AND), `||` (OR), and `!` (NOT), which manipulate boolean values to form complex expressions. Understanding these operators enhances your logical reasoning in programming.
Combining Boolean Values
Combining multiple boolean conditions unveils the power of logic operations. You can create sophisticated checks that lead to precise decisions.
Code Snippet:
bool isSunny = true;
bool isWeekend = false;
if (isSunny && isWeekend) {
printf("Great day for a picnic!");
} else if (!isSunny) {
printf("Maybe stay indoors.");
} else {
printf("A regular day.");
}
The program evaluates the combination of two conditions; while `isSunny` is true, `isWeekend` is false, and thus, it prompts to "Maybe stay indoors."
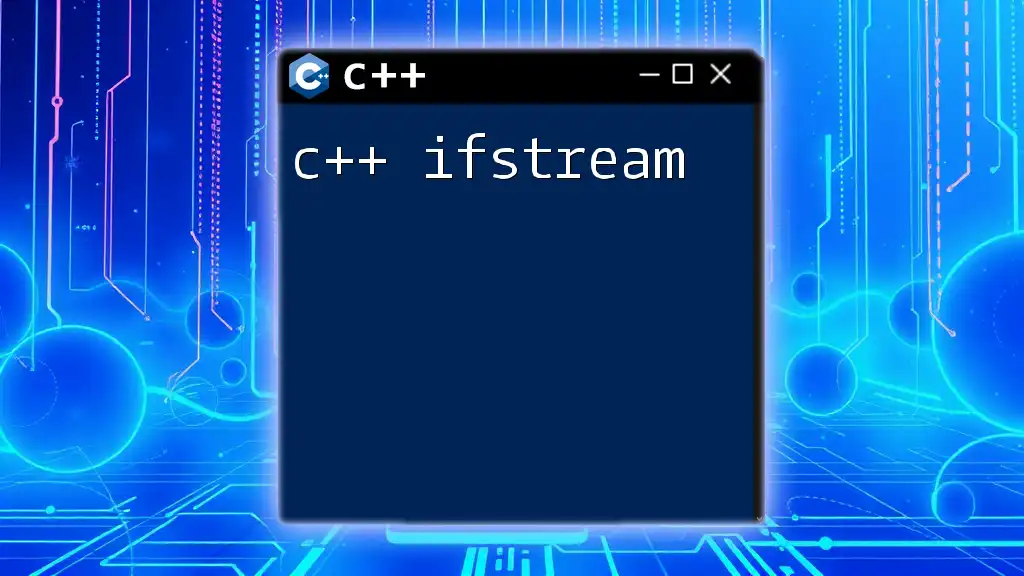
Common Mistakes with Boolean Values
Misunderstanding Boolean Context
A common pitfall is misunderstanding how boolean context functions, which may lead to unexpected outputs. Neglecting implicit conversions can also introduce bugs.
Example:
int num = 0;
if (num) {
printf("Number is non-zero");
} else {
printf("Number is zero"); // Correctly outputs "Number is zero"
}
Here, since `num` is zero, the output clearly indicates the conditional logic.
Value Comparison
Mistakes frequently occur when improperly using comparison operators. Ensuring the correct logic is vital to avoid logical errors.
Code Snippet:
bool isActive = (a > 0); // Correct
bool isActiveWrong = (a = 0); // Incorrect: assignment instead of comparison
In the second line, the programmer intended to check equality but mistakenly assigned a value instead. This can cause unwanted behavior in the code.
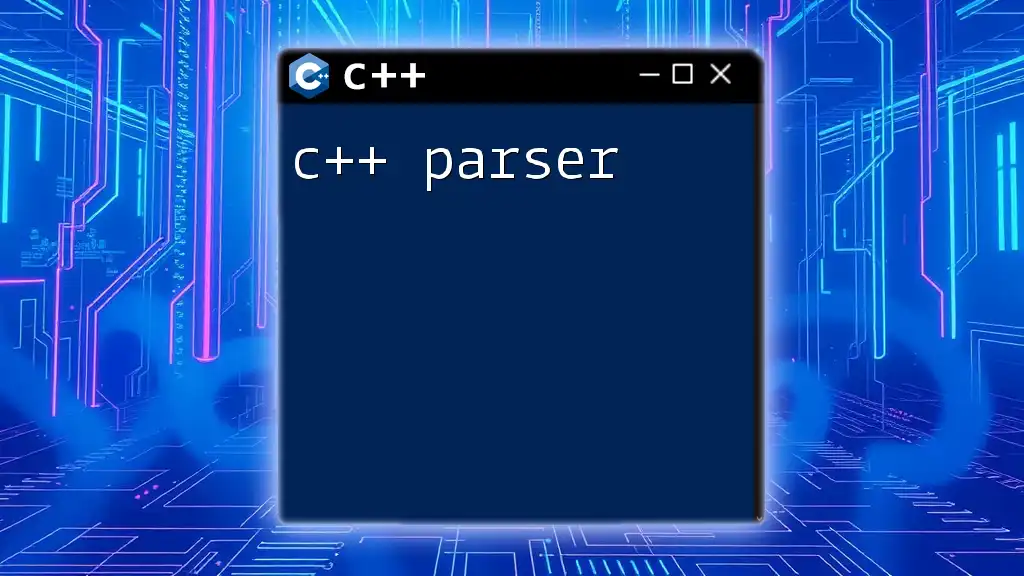
Advanced Topics
Boolean Functions
Creating boolean functions can simplify complex checks. They return true or false based on certain conditions, streamlining code readability.
Example:
bool isEven(int number) {
return (number % 2 == 0);
}
if (isEven(10)) {
printf("10 is even!");
}
The function `isEven` checks whether a number is even and allows for cleaner code by avoiding repetitive checks.
True and False in C++ Templates
Boolean values are also significant in template metaprogramming. They dictate types and control behavior at compile time.
Code Snippet:
template<bool condition>
void checkCondition() {
if (condition) {
printf("Condition is true!");
} else {
printf("Condition is false!");
}
}
// Usage
checkCondition<true>();
In this case, your function behaves differently based on the boolean template parameter passed.
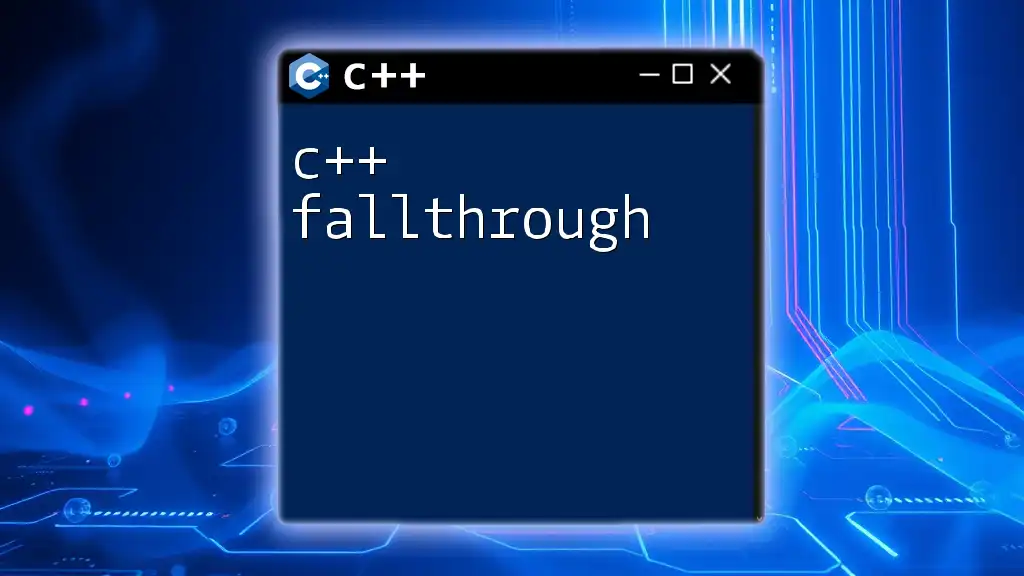
Conclusion
Understanding the nuances of true and false in C++ is imperative for programmers aiming to write efficient and effective code. Mastery of boolean logic and practices will bolster your programming skills and enhance your problem-solving abilities in various scenarios.
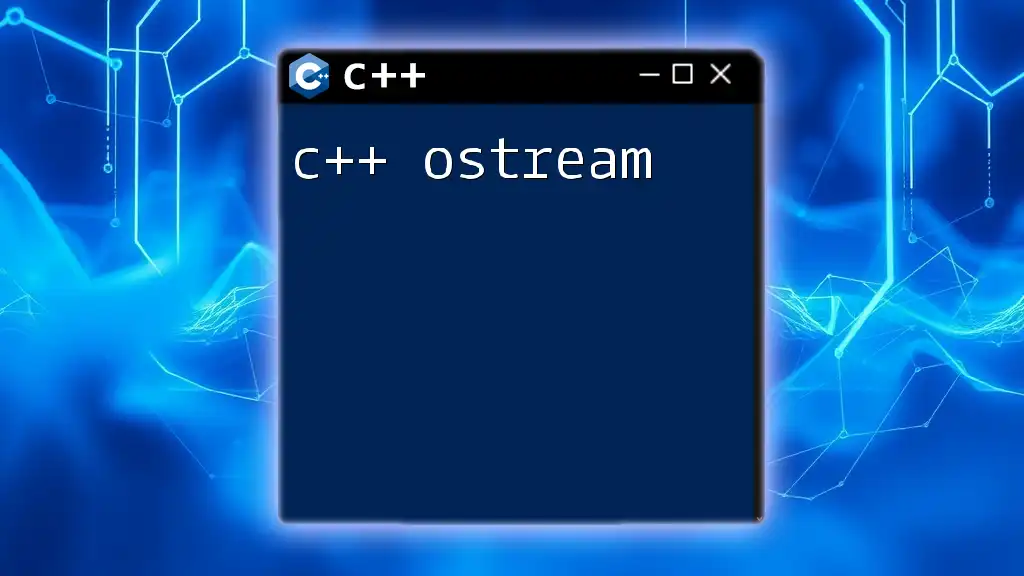
Call to Action
Engage further with our platform for more resources and tutorials aimed at strengthening your C++ knowledge. Dive into practical examples and coding challenges to enhance your understanding of C++ false true concepts!
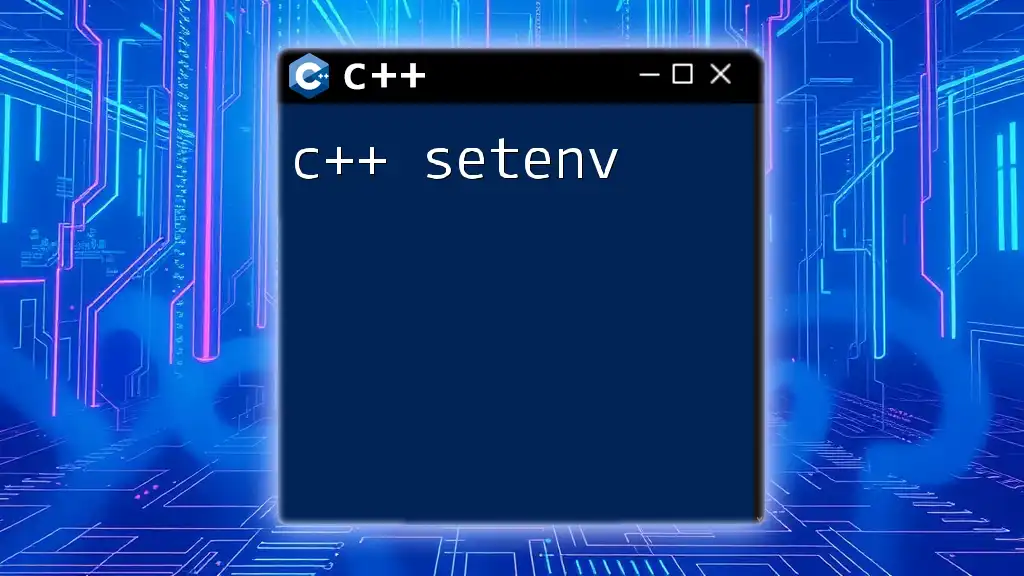
Additional Resources
Explore additional articles on C++ basics, boolean logic, and advanced programming techniques to support your journey in mastering C++.