In this C++ game tutorial, you'll learn how to create a simple console-based guessing game using basic input and output commands.
#include <iostream>
#include <cstdlib>
#include <ctime>
int main() {
srand(static_cast<unsigned>(time(0))); // Seed for random number
int number = rand() % 100 + 1; // Random number between 1 and 100
int guess;
std::cout << "Guess the number (between 1 and 100): ";
do {
std::cin >> guess;
if (guess > number) {
std::cout << "Too high! Try again: ";
} else if (guess < number) {
std::cout << "Too low! Try again: ";
} else {
std::cout << "Congratulations! You guessed it!\n";
}
} while (guess != number);
return 0;
}
Setting Up Your Development Environment
Choosing the Right IDE
When delving into a C++ game tutorial, selecting the right Integrated Development Environment (IDE) is crucial for an effective learning experience. Popular choices include Visual Studio and Code::Blocks. Each provides robust tools that streamline coding, debugging, and compiling.
To install your chosen IDE:
- Visit the official website of the IDE.
- Download the installer suitable for your operating system.
- Follow the installation guidelines provided on the site to set it up properly.
Installing Essential Libraries and Tools
For game development with C++, libraries such as SFML (Simple and Fast Multimedia Library) and SDL (Simple DirectMedia Layer) provide essential features for graphics rendering, sound, and user input.
To install SFML, for instance, follow these steps:
- Visit the SFML download page.
- Choose the version compatible with your compiler (for example, “GCC” if you are using MinGW).
- Unzip the downloaded file and link the libraries in your project settings.
- You will need to include the SFML headers in your source files to start using them.
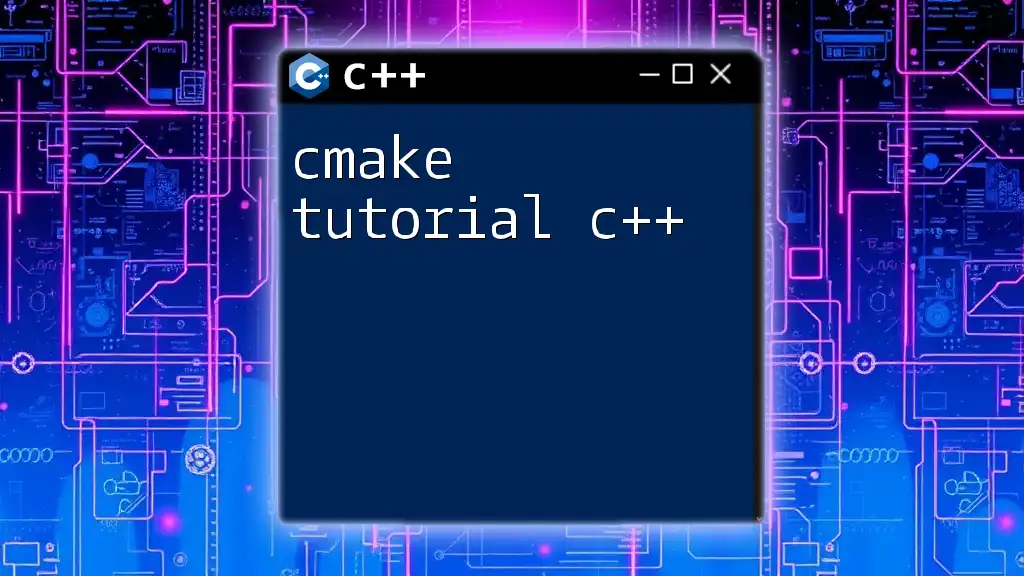
Basics of Game Development with C++
Understanding Game Loops
A game loop is the backbone of any game, responsible for maintaining the flow of the game. It continuously runs alongside the game’s logic, handling user input, updating game elements, and rendering graphics.
Here is a simple structure of a game loop using C++:
while (gameIsRunning) {
processInput();
updateGame();
render();
}
This entails:
- processInput(): Handling user inputs for actions such as movement or shooting.
- updateGame(): Updating the status of all game elements (like player position, score, etc.).
- render(): Drawing the updated elements on the screen.
Creating a Simple Game Structure
Understanding classes and objects is key. They help organize your game code efficiently. Structuring your game might look like this:
- Main Menu: Handles the entry screen and user selections.
- Gameplay: The main loop where all the game action occurs.
- Game Over: Screen for when the game concludes.
Here’s a basic structure using classes in C++:
class Game {
void run();
void processInput();
void update();
void render();
};
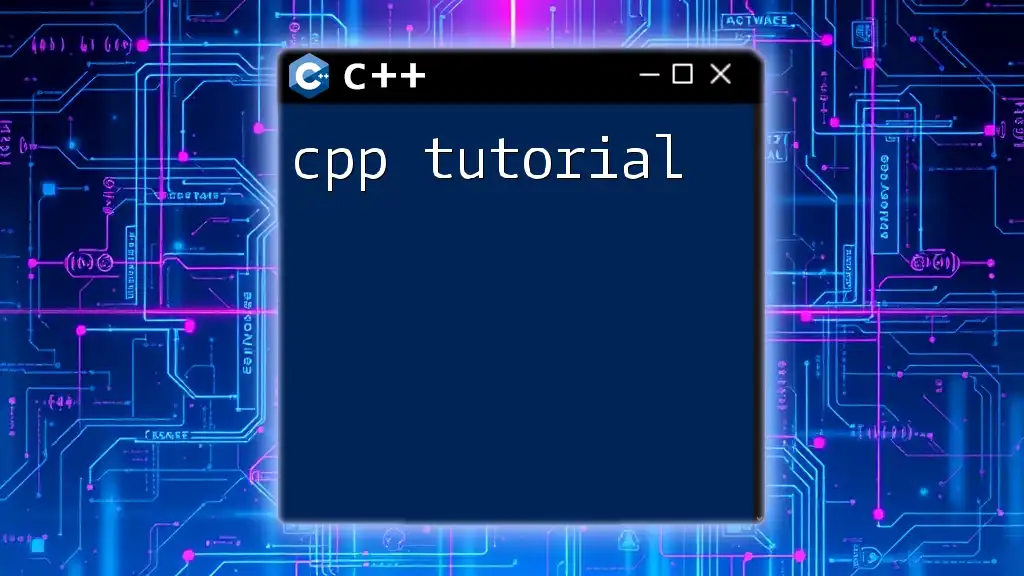
Rendering Graphics with C++
Introduction to Graphics Libraries
For modern game development, leveraging graphics frameworks like SFML or SDL makes rendering smooth and efficient.
Drawing Shapes and Textures
Using SFML, you can render shapes and textures with ease. For example, to create a circle, you would use the following code:
sf::CircleShape circle(50); // radius of the circle
circle.setFillColor(sf::Color::Green);
window.draw(circle); // draw shape on window
To load and display textures:
sf::Texture texture;
texture.loadFromFile("path_to_image.png");
sf::Sprite sprite(texture);
window.draw(sprite);
This illustrates how you can create visually engaging applications with minimal effort.
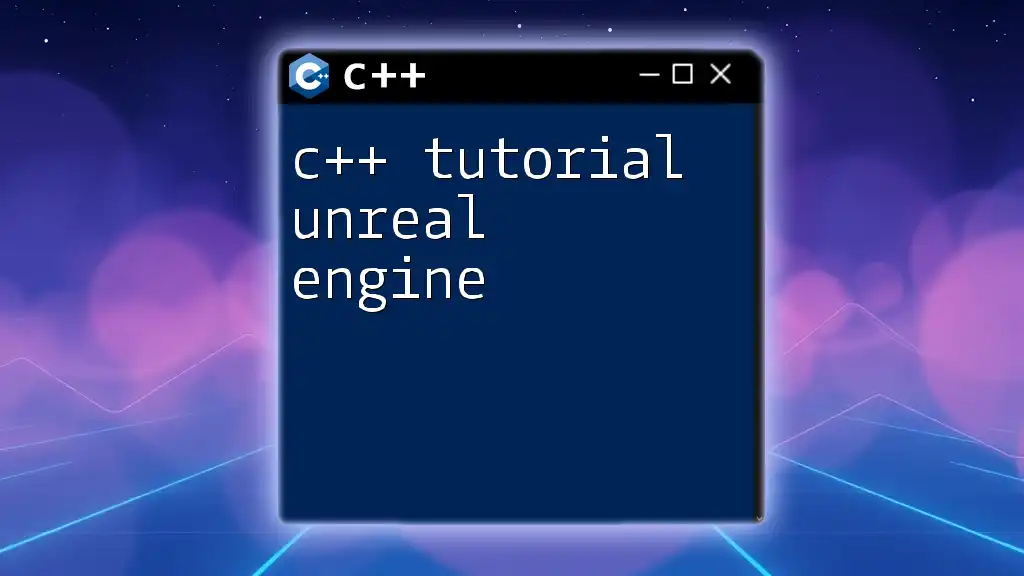
Handling User Input
Reading Keyboard and Mouse Input
Interactivity enhances player engagement, making input handling essential. SFML simplifies this process. For instance, handling keyboard input can be done as follows:
if (event.type == sf::Event::KeyPressed) {
if (event.key.code == sf::Keyboard::Up) {
player.move(0, -1); // move player up
}
}
Using Input for Game Mechanics
Initialize your character control mechanism to respond to user inputs effectively. By mapping certain keys to specific actions (like jumping or shooting), you will create a more immersive experience.
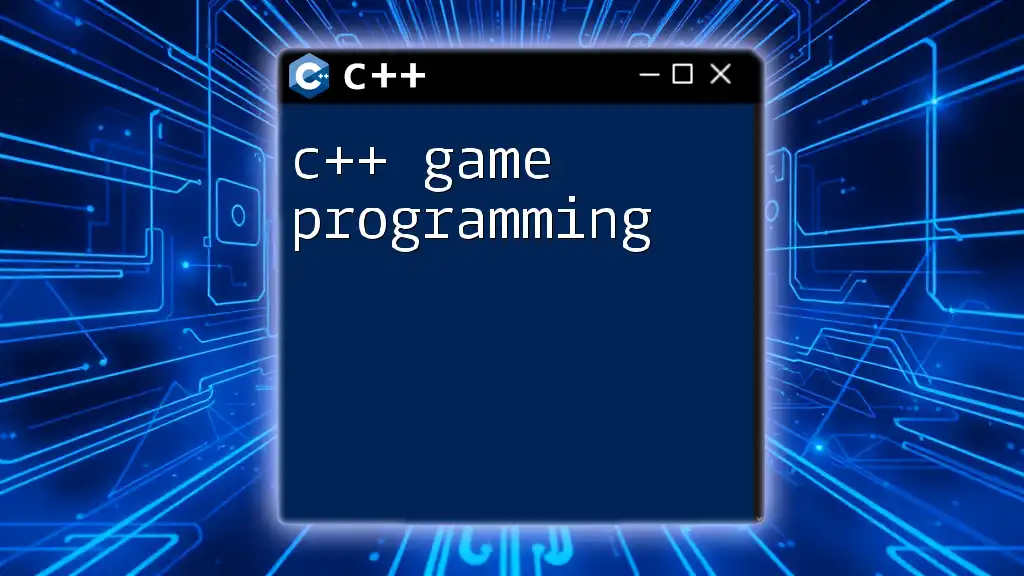
Implementing Game Logic
Creating Game Objects
To create a dynamic game, define game entities using classes. For example, a Player class might look like this:
class Player {
public:
void move(float deltaX, float deltaY);
void draw(sf::RenderWindow& window);
private:
sf::Sprite sprite;
float health;
};
Collision Detection and Response
To make the game realistic, incorporate collision detection. A simple approach is using bounding boxes to determine interactions between objects. Here’s a basic example of collision detection:
if (player.getGlobalBounds().intersects(enemy.getGlobalBounds())) {
// Handle collision
}
This code checks if the player and enemy objects overlap, prompting you to define a response (like reducing health).
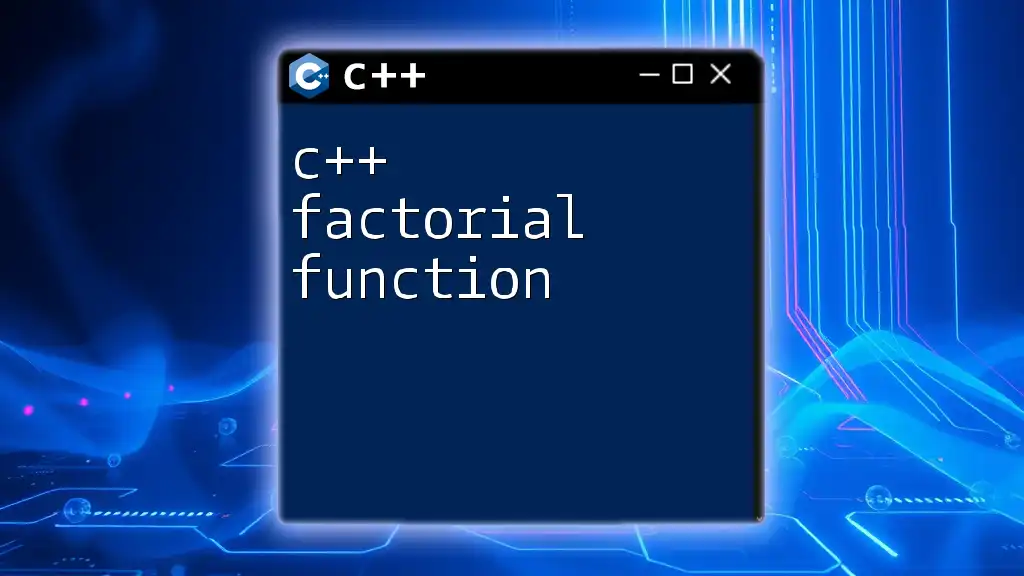
Adding Sound and Music
Incorporating Audio in Your Game
Audio adds depth to your gaming experience. Libraries such as OpenAL or FMOD can help integrate sound effectively. A simple example of playing background music might look like this:
sf::Music music;
music.openFromFile("background_music.ogg");
music.play(); // starts playing the music
Consider integrating sound effects for actions like shooting or jumping to enhance the experience.
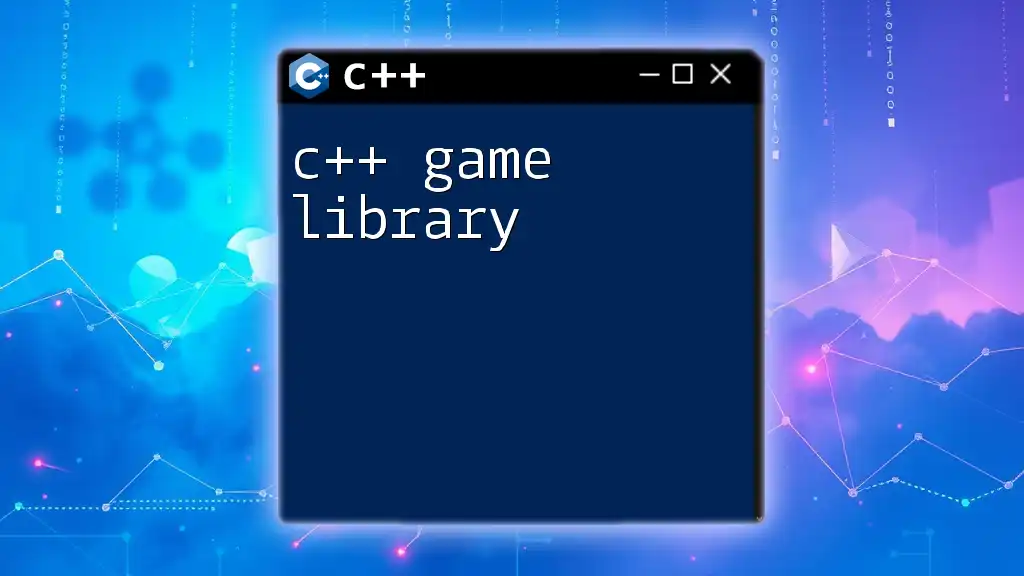
Developing a Complete Game Example
Combining All Concepts into a Simple Game
For an enticing C++ game tutorial, developing a simple game—like a basic shooter or platformer—will solidify your understanding. Commonly, this combines all learned elements, such as rendering graphics, processing input, managing game logic, and implementing audio.
Here’s an overview of how the complete game structure might look:
class SimpleGame {
public:
void run() {
while (gameIsRunning) {
processInput();
updateGame();
render();
}
}
// Other necessary methods here...
};
Full Code Example of the Game
int main() {
SimpleGame game;
game.run();
return 0;
}
This code serves as the entry point, illustrating how to organize your game effectively.
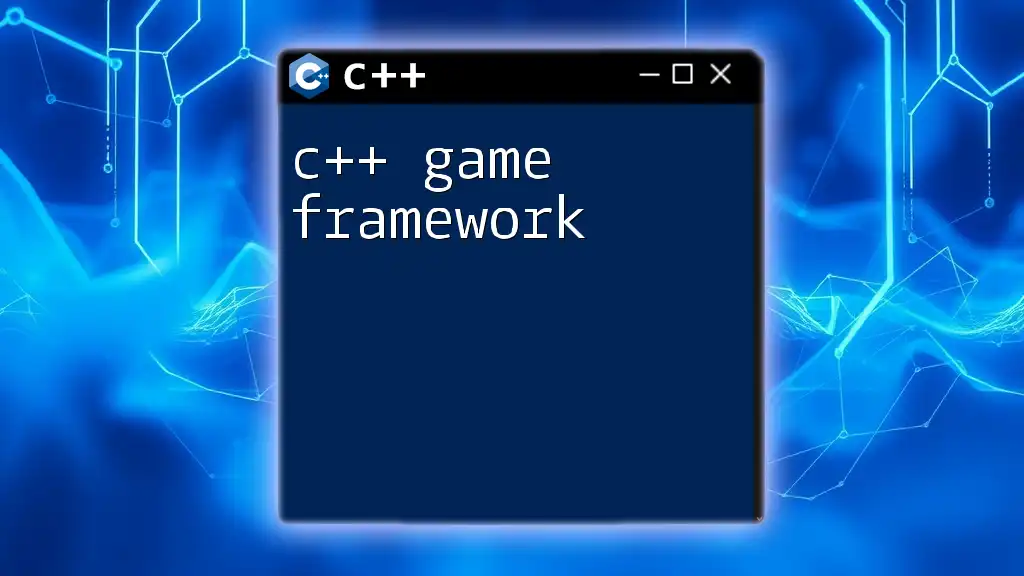
Debugging and Optimizing Your Game
Common Debugging Techniques
Debugging is essential in game development. Use the debugging tools available in your IDE to set breakpoints and step through your code. This will help you identify problematic sections quickly.
Performance Optimization Tips
To ensure your game runs smoothly:
- Optimize resource management: load assets once and reuse them rather than loading multiple times.
- Use appropriate data structures (like vectors) based on access patterns.
- Monitor performance overhead and eliminate bottlenecks.
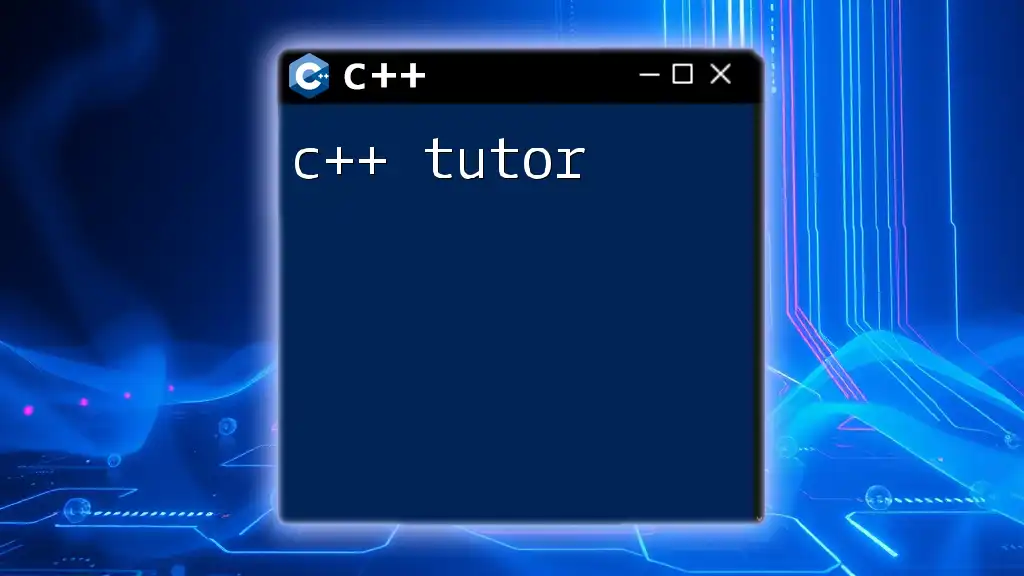
Conclusion
In this C++ game tutorial, we covered the foundational aspects of game development using C++. By understanding the structure of a game loop, rendering graphics, handling input, implementing game logic, and debugging, you now have the tools needed to create engaging gaming experiences.
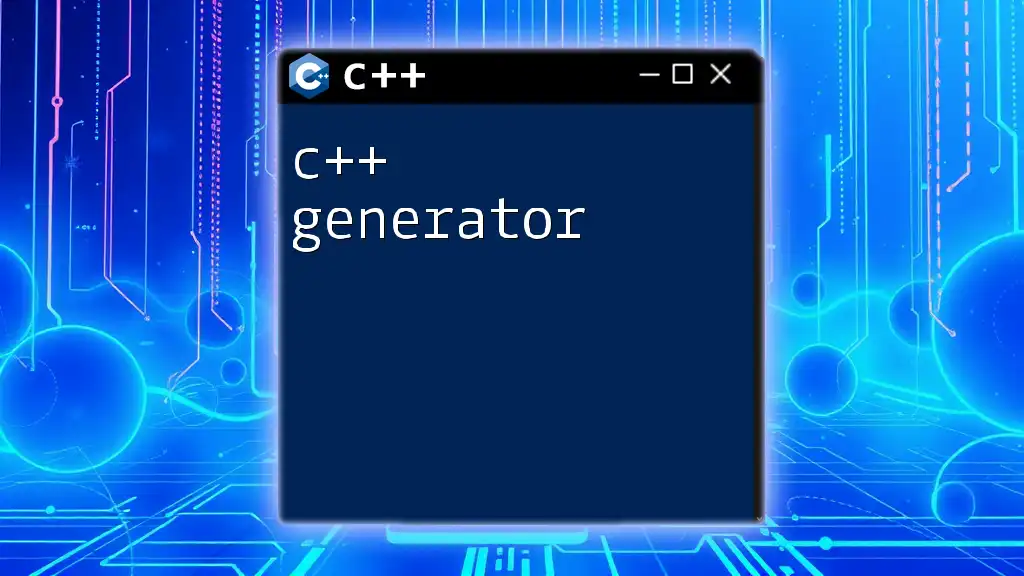
Resources and References
As you move forward, consider expanding your knowledge through additional resources like books, online courses, and community forums dedicated to C++ game development. Engaging with fellow developers can provide support and insights as you continue your journey.