The `fseek` function in C++ is used to reposition the file pointer to a specified location in a file, allowing for efficient navigation and access to different parts of the file.
#include <cstdio>
int main() {
FILE *file = fopen("example.txt", "rb");
if (file) {
fseek(file, 10, SEEK_SET); // Move the pointer to the 10th byte from the beginning
// Perform further file operations...
fclose(file);
}
return 0;
}
Understanding File Pointers
What are File Pointers?
File pointers are essential components of file handling in C++. They essentially act as references that allow the program to track the current location in a file stream. The ability to manipulate file pointers enables programmers to read from or write to specific locations within a file, making file operations more flexible and efficient.
How File Pointers Work
Every time you open a file in C++, a file pointer is created. This pointer represents the current position in that file. When you read or write to a file, the file pointer automatically advances, allowing you to sequentially access data. Understanding how to manipulate this pointer is key to effective file handling, especially when you need to revisit or skip parts of the file.

The fseek Function
Definition and Purpose
The fseek function is a powerful tool in C++ that allows developers to move the file pointer to a specific location within a file. This capability is crucial when working with large files or when you need to implement random access to data.
Syntax of fseek
The general syntax for the fseek function is as follows:
int fseek(FILE *stream, long offset, int whence);
Description of parameters:
- `FILE *stream`: A pointer to the file you are working with, which is typically obtained from functions like fopen.
- `long offset`: The number of bytes to move the pointer, which can be positive or negative depending on the desired direction of movement.
- `int whence`: This parameter determines the reference point for the offset.
Return Value of fseek
The fseek function returns 0 on success and -1 on failure. It is critical to check the return value of fseek to handle any potential errors in file operations. Mistakes in seeking could lead to unexpected behavior when reading from or writing to the file.
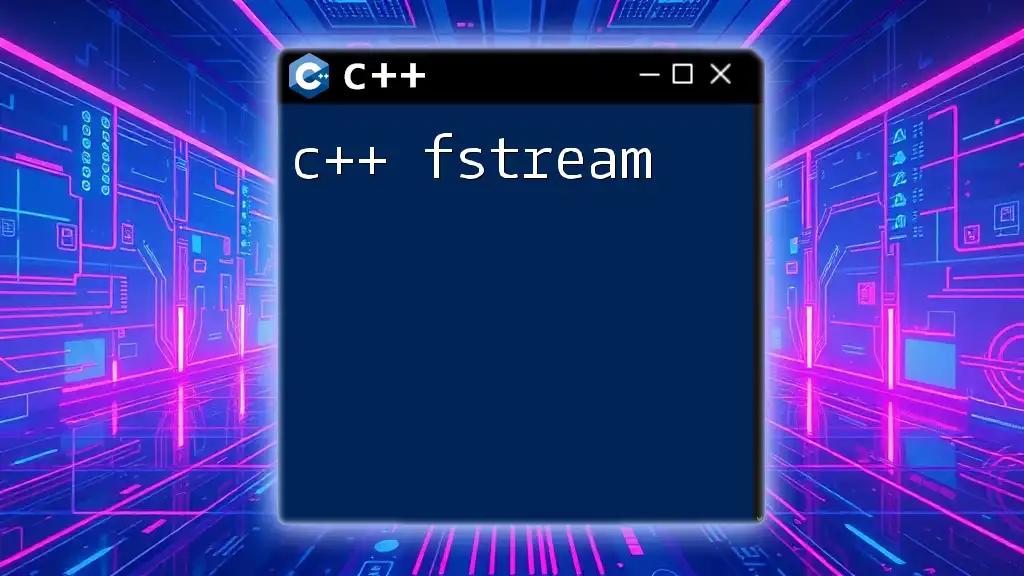
Using fseek in C++
Navigating Through Files
Using fseek effectively allows you to jump to varying locations within a file. For instance, if you want to reset the file pointer back to the beginning of the file, you can use:
fseek(filePointer, 0, SEEK_SET);
This command indicates that you want the file pointer to be set at the beginning, which is crucial for re-reading the file from its start.
Reading and Writing Data After fseek
After manipulating the file pointer with fseek, it is important to ensure data integrity during read/write operations. Here's an example that demonstrates reading data after seeking:
fseek(filePointer, 50, SEEK_SET);
char buffer[20];
fread(buffer, sizeof(char), sizeof(buffer), filePointer);
In this example, the file pointer is moved to the 50th byte so that the next read operation will pull data starting from that location.
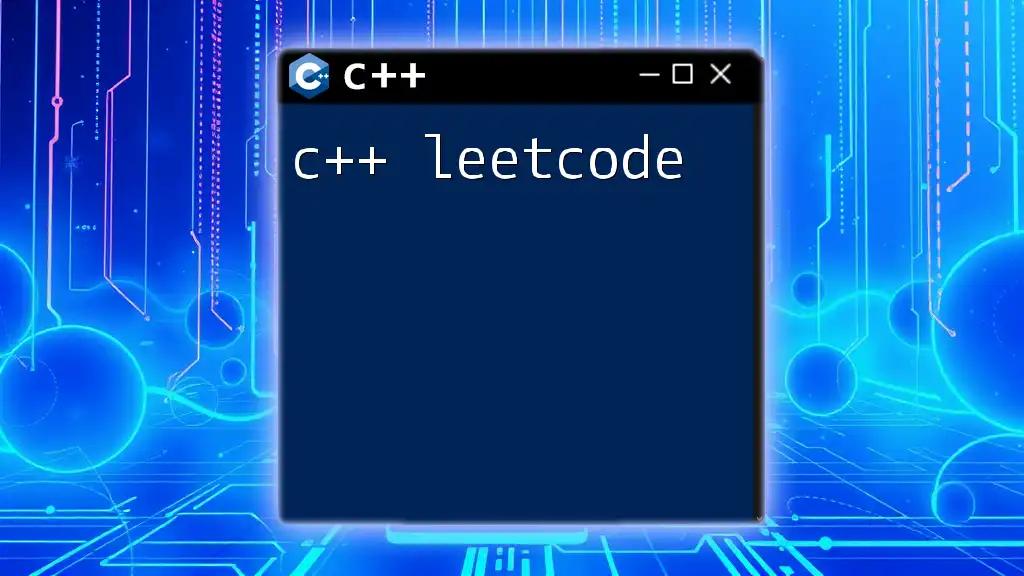
Practical Examples of fseek
Example 1: Moving to the End of a File
Sometimes, you may want to position the file pointer at the end of the file to append data. You can achieve this with:
fseek(filePointer, 0, SEEK_END);
This command places the pointer at the very end, allowing you to write new data without overwriting existing content.
Example 2: Custom File Navigation
In scenarios where you need more granular control, fseek can be used for custom navigation. The following snippet shows how to set the pointer back to the 100th byte in the file:
fseek(filePointer, 100, SEEK_SET); // Resetting pointer to the 100th byte
This flexibility in movement makes fseek a versatile function to have in your developers' toolkit.

Common Mistakes When Using fseek
Misunderstanding whence Values
Using the wrong `whence` values can lead to unexpected results. The three options you can use are:
- `SEEK_SET`: Beginning of the file.
- `SEEK_CUR`: Current position of the file pointer.
- `SEEK_END`: End of the file.
It’s crucial to understand what each constant implies to prevent errors that arise from incorrect positioning.
Not Checking Return Values
Ignoring the return value of fseek may lead to unchecked errors. For example, failure to correctly adjust the file pointer can have severe implications for data accuracy. Always implement error handling like so:
if(fseek(filePointer, 0, SEEK_SET) != 0) {
perror("Error seeking in file");
}
This practice is essential to ensure robustness in your file operations.

Best Practices for Using fseek
When to Use fseek
fseek is advantageous when working with large files or when you need to access data points randomly rather than sequentially. Use it judiciously when performance and memory management are a priority, as it aids in optimizing file operations.
Performance Considerations
Frequent calls to fseek can impact performance, especially in large files. Aim to minimize the number of seek operations whenever possible. Group read/write operations to reduce overhead and improve efficiency throughout your file handling processes.

Conclusion
The fseek function is an indispensable part of file handling in C++. By mastering fseek alongside file pointers, developers can navigate files with agility and precision. Understanding its capabilities, syntax, and best practices allows for enhanced file manipulation in your programming endeavors. Embrace experimentation with the example code provided, visualizing new applications for fseek in your projects.