In C++, passing a vector by reference allows you to modify its contents without making a copy, improving performance and efficiency.
Here's a code snippet to demonstrate:
#include <iostream>
#include <vector>
void modifyVector(std::vector<int>& vec) {
for (int& num : vec) {
num *= 2; // Double each element in the vector
}
}
int main() {
std::vector<int> myVec = {1, 2, 3, 4, 5};
modifyVector(myVec);
for (int num : myVec) {
std::cout << num << " "; // Output: 2 4 6 8 10
}
return 0;
}
Understanding Vectors in C++
What is a Vector?
In C++, a vector is a dynamic array-like data structure found in the Standard Template Library (STL). Unlike traditional arrays, vectors allow for dynamic resizing, meaning you can add or remove elements without worrying about memory management. This flexibility makes vectors ideal for a variety of programming tasks where data size can change.
Vectors are defined in the `std::vector` class and can store elements of a given data type, ranging from primitive types (like int and char) to user-defined types.
Basic Vector Declaration and Initialization
To use a vector, you must include the vector header file:
#include <vector>
Here is a basic example of how to declare and initialize a vector:
std::vector<int> myVec = {1, 2, 3, 4, 5};
In this snippet, `myVec` is a vector of integers initialized with five values. Understanding this initialization process is fundamental as it sets the stage for further operations involving the vector.
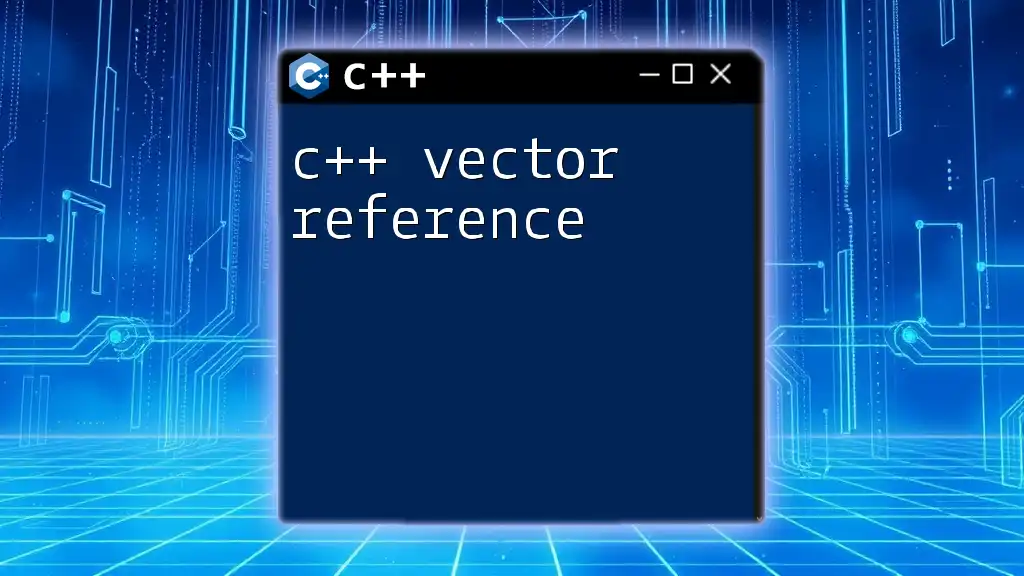
Passing Vectors in C++
By Value vs. By Reference
When you pass a vector to a function, C++ allows for two types of arguments: by value and by reference, each serving different purposes.
-
By Value: This method creates a copy of the vector. Any modifications done to it in the function do not affect the original vector. This can be memory- and resource-intensive, especially if the vector contains a large amount of data.
-
By Reference: This method allows the function to operate on the original vector. Changes made in the function reflect in the original vector, making it a memory-efficient choice.
Choosing by reference is particularly beneficial when dealing with large vectors or when you want to modify the original data.
Syntax of Passing a Vector by Reference
Using reference in a function definition is straightforward. Here’s the basic syntax:
void functionName(std::vector<int>& vec);
Here, the use of `&` indicates that `vec` is a reference to the original vector. This means any changes to `vec` inside the function will directly affect the vector passed from the calling function.
Benefits of Passing Vectors by Reference
Memory Efficiency
One of the most significant advantages of passing a vector by reference is memory efficiency. Since no copy is created, less memory is consumed, which is crucial when working with large vectors. Copying a large vector can lead to high overhead, which can slow down your program and increase the likelihood of running out of memory.
Performance Optimization
Passing a vector by reference enhances performance, particularly for large datasets. When you pass by value, C++ copies the entire data structure, which can be a costly operation. Here’s a simple comparison:
void processVectorByValue(std::vector<int> vec) {
// Processing something with 'vec'
}
void processVectorByReference(std::vector<int>& vec) {
// Processing something different with 'vec'
}
By passing `vec` by reference in the second function, the overhead of copying is eliminated, leading to improved speed. This is particularly evident if your vector contains thousands of elements.
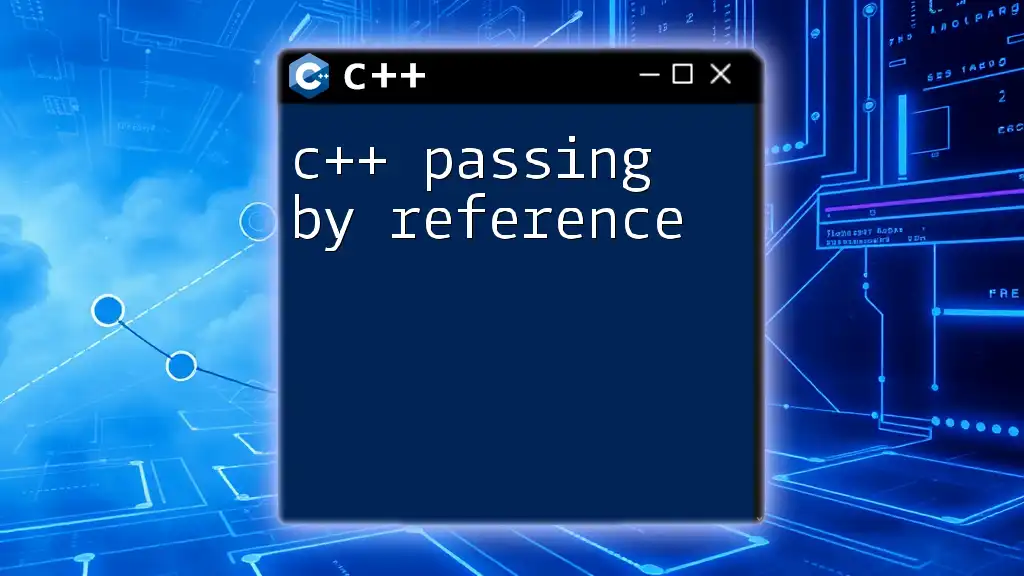
Code Examples
Simple Example: Modifying a Vector
Let’s consider a function that doubles each element in a vector. Passing the vector by reference allows us to modify the original data directly:
void modifyVector(std::vector<int>& vec) {
for(auto& element : vec) {
element *= 2; // Doubles each element
}
}
int main() {
std::vector<int> myVec = {1, 2, 3, 4};
modifyVector(myVec);
// myVec now contains {2, 4, 6, 8}
}
In this example, the `modifyVector` function doubles the value of each element in `myVec`. Because we passed the vector by reference, `myVec` is modified directly, demonstrating the power of this method.
Example: Aggregating Data
Let’s explore a scenario where you sum up the elements of a vector. Here, you’ll want to pass the vector by reference, ensuring efficiency, especially when handling large data sets:
int sumVector(const std::vector<int>& vec) {
int sum = 0;
for(int element : vec) {
sum += element; // Adds elements
}
return sum;
}
int main() {
std::vector<int> myVec = {1, 2, 3, 4};
int total = sumVector(myVec);
// total is 10
}
By using `const std::vector<int>&`, we ensure that the original vector isn’t modified, preserving its state. This showcases that you can comfortably pass large vectors without the fear of unintentional side effects.
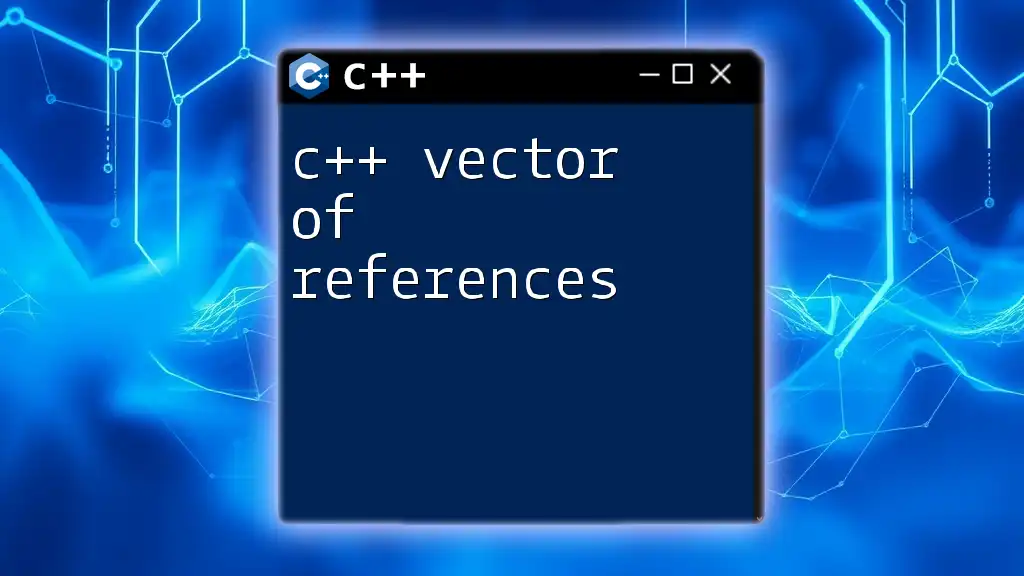
Real-World Applications
Leveraging Vectors in Algorithms
Vectors are widely used in algorithms due to their dynamic nature. When implementing complex algorithms like sorting or searching, passing vectors by reference reduces unnecessary overhead. For instance, algorithms in graph theory often need to modify large datasets, making reference passing a necessity for performance.
Passing Vectors in Object-Oriented Programming
In object-oriented programming (OOP), classes may require functions that accept vectors as class members or function parameters. Passing vectors by reference ensures that methods can efficiently manipulate internal data:
class DataAnalyzer {
public:
void analyze(std::vector<int>& data) {
// Perform analysis on data
}
};
In this scenario, `DataAnalyzer::analyze` efficiently works with the original `data` vector, allowing for real-time updates and modifications directly.
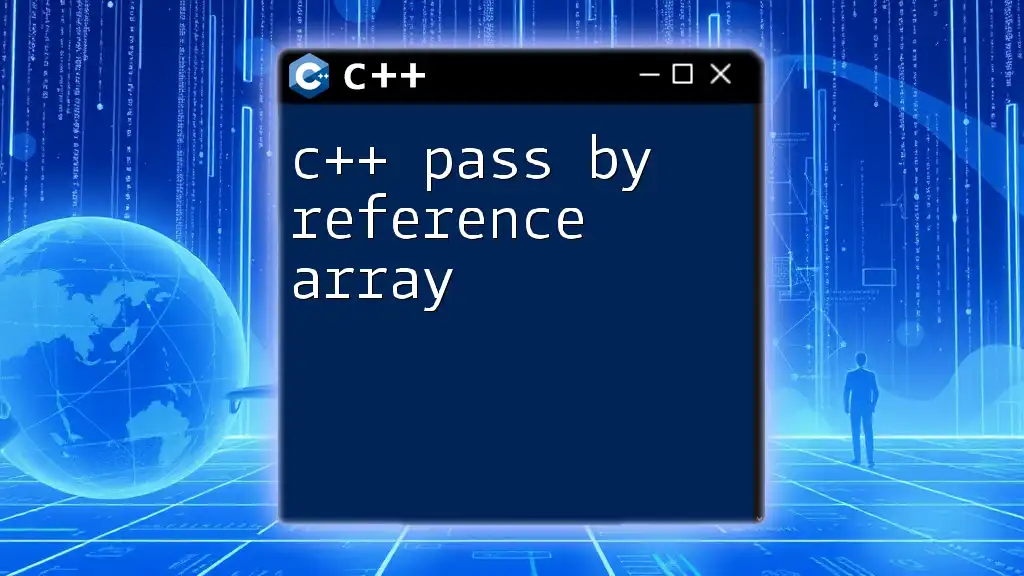
Common Mistakes
Not Using `&` for Reference
A common error when writing functions that accept vectors is forgetting to use the `&` for reference. This leads to the creation of a copy, which can cause unexpected behavior and inefficient memory usage. Always remember to declare function parameters using the `&` when you intend to pass by reference.
Modifying Original Data Intentionally vs. Unintentionally
When modifying vectors, be aware of the implications of passing by reference. If your function is meant solely for reading, prefer using `const` references to prevent unintentional changes. Mismanaging modifications can result in bugs that are difficult to track.
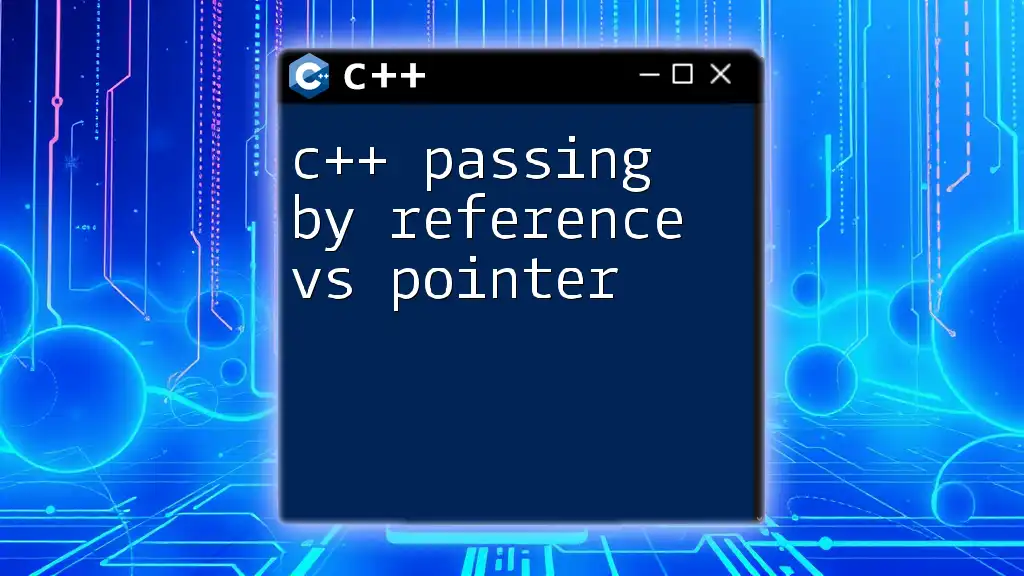
Conclusion
In summary, understanding how to c++ pass vector by reference is crucial for efficient programming. This technique not only enhances performance and memory efficiency but is also a best practice in handling complex data structures in C++. By practicing the examples provided and avoiding common pitfalls, you can develop a strong grasp on utilizing vectors effectively.
Embrace this knowledge and apply it to your projects; it’ll significantly improve the way you write and manage C++ code. Happy coding!