In C++, passing an array by reference allows you to modify the original array within a function without making a copy, as shown in the following example:
#include <iostream>
void modifyArray(int (&arr)[3]) {
for (int i = 0; i < 3; ++i) {
arr[i] *= 2; // Modifies the original array elements
}
}
int main() {
int myArray[3] = {1, 2, 3};
modifyArray(myArray);
for (int i : myArray) {
std::cout << i << " "; // Output: 2 4 6
}
return 0;
}
Understanding Arrays in C++
In C++, an array is a collection of elements, all of the same type, stored in contiguous memory locations. They are fundamental data structures that allow for efficient data manipulation and quick access to elements.
An array can be declared with a specific type and size. For example:
int myArray[5] = {1, 2, 3, 4, 5};
This declaration creates an array called `myArray` that can hold five integers.

Why Pass by Reference?
When it comes to passing arrays to functions, understanding the distinctions between pass by value and pass by reference is crucial.
-
Pass by Value: When an array is passed by value, a copy of the entire array is created. This approach consumes more memory and can lead to performance inefficiencies, especially with large arrays.
-
Pass by Reference: In contrast, passing an array by reference means that the function operates on the actual array, not a copy. This method is significantly more efficient in both memory usage and execution speed, particularly when manipulating large datasets. Moreover, any modifications made to the array within the function will reflect in the original array, which is a key advantage for developers aiming to modify data without additional copies.
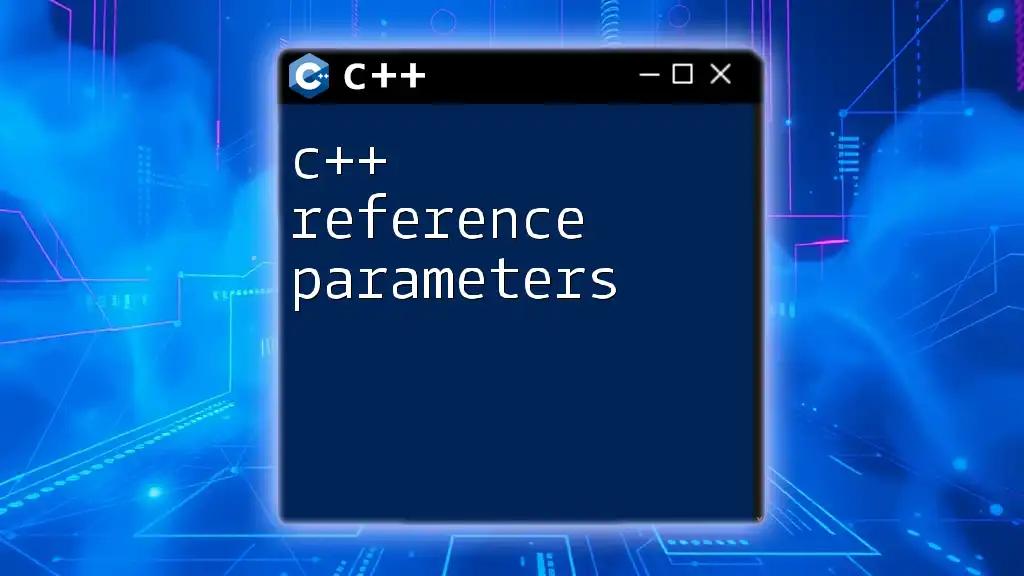
Passing Arrays by Reference in C++
Syntax for Passing Arrays by Reference
In C++, arrays can be passed to functions using the reference operator `&`. The syntax can be intimidating at first, but once understood, it simplifies the handling of arrays. Here’s how you can do this:
void modifyArray(int (&arr)[5]) {
for (int i = 0; i < 5; i++) {
arr[i] *= 2; // Each element in the array is doubled
}
}
In this example, the function `modifyArray` accepts a reference to an integer array of size 5. This allows the function to directly manipulate the original array passed in.
Example of Passing an Array to Function C++ by Reference
Here’s a complete example demonstrating how to pass an array by reference and output its elements:
#include <iostream>
using namespace std;
void displayArray(int (&arr)[5]) {
for (int i = 0; i < 5; i++) {
cout << arr[i] << " "; // Displays each element
}
cout << endl;
}
int main() {
int myArray[5] = {1, 2, 3, 4, 5};
displayArray(myArray); // Passing the array by reference
return 0;
}
In this code, `displayArray` receives `myArray` as a reference, allowing it to print the elements easily without creating a copy.
Differences Between Array References and Pointers
Understanding the differences between array references and pointers is fundamental in C++.
-
Array References: Array references allow easier syntax and function handling. They are fixed to the size specified during declaration, making them a safer choice for passing array parameters safely.
-
Pointers: C-style pointers are more flexible but require manual memory management and can lead to more complex code. They allow pointing to different sized arrays or even dynamic memory but increase the risk of memory-related errors (like dereferencing a null pointer).
Both can achieve similar results under the right conditions; however, using references can often lead to cleaner and more maintainable code.
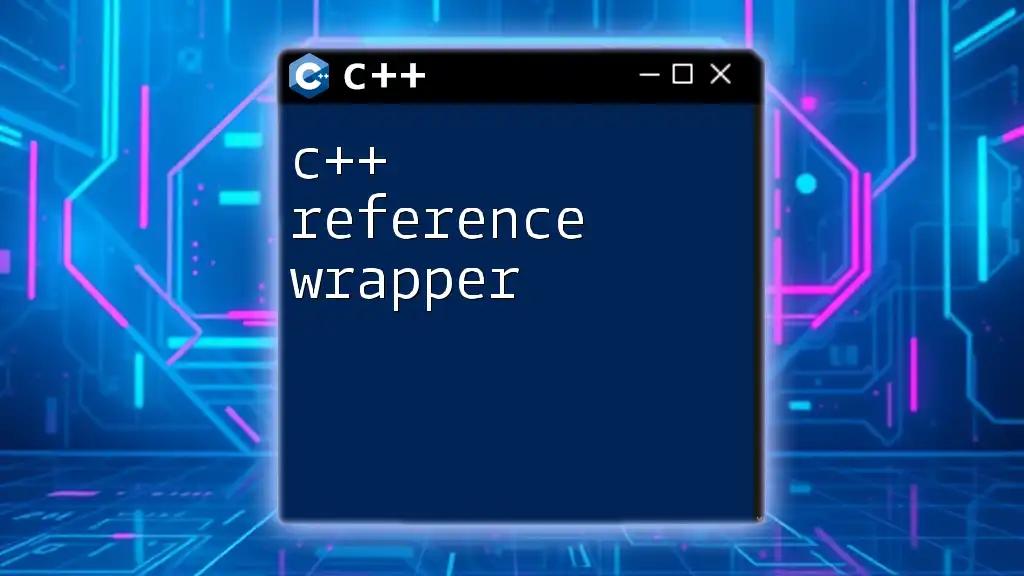
Passing Array to Function C++ by Reference: A Real-World Example
Example 1: Summing Elements of an Array
Consider the following example where we want to calculate the sum of all elements in an integer array:
int sumArray(int (&arr)[5]) {
int sum = 0;
for (int i = 0; i < 5; i++) {
sum += arr[i]; // Adding each element to the sum
}
return sum;
}
This function receives the array by reference and iteratively sums its elements to return the total.
Example 2: Finding Maximum Element in an Array
Another useful functionality might be finding the maximum value in an array:
int maxElement(int (&arr)[5]) {
int max = arr[0]; // Start with the first element
for (int i = 1; i < 5; i++) {
if (arr[i] > max) {
max = arr[i]; // Update max if a larger value is found
}
}
return max;
}
This straightforward function demonstrates how easy it is to find the maximum value in an array when passed by reference.

Best Practices for Pass Array By Reference C++
When working with arrays in C++, some best practices include:
-
Size Known at Compile Time: Always use fixed sizes when passing by reference for clarity and safety. If you need dynamic sizing, consider using `std::vector`.
-
Use Templates for Flexibility: If you need to handle arrays of varying sizes and types, employing templates can provide the versatility required without sacrificing performance.
-
Minimize Pointer Arithmetic: While pointers and references can be seductive due to their capability to manage memory directly, they can also lead to complex and error-prone code, especially in larger, scalable applications. Consider using array references unless pointers are explicitly required.

Conclusion
Understanding how to effectively use C++ pass by reference array techniques is invaluable for optimizing performance and memory usage in your programs. This method not only provides efficiency but also allows direct manipulation of array data within functions.
As you continue to explore C++, practice passing arrays by reference and experiment with different functions to build confidence in array manipulation. Doing so will enhance your programming skills and deepen your understanding of C++.
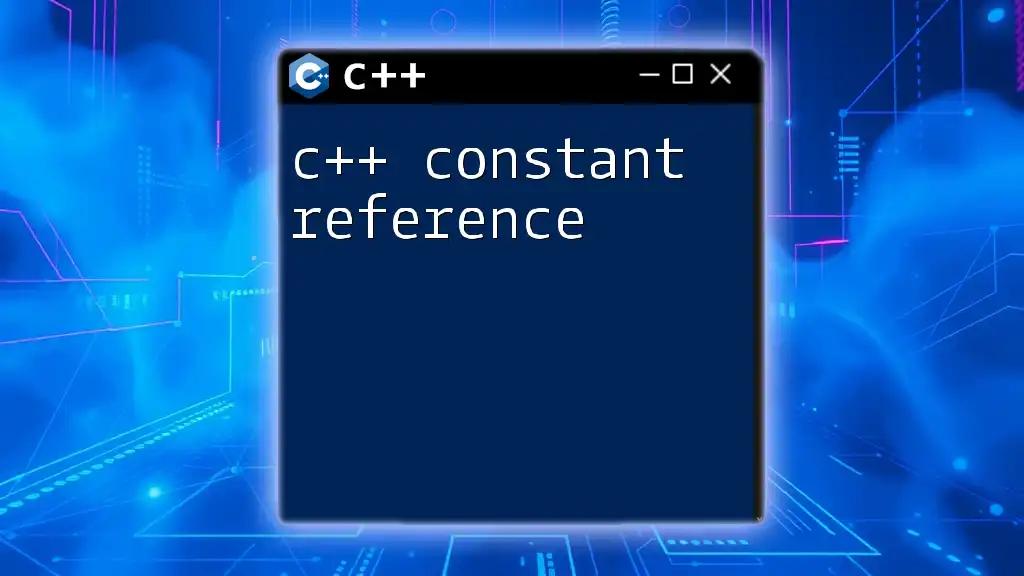
Further Reading
To further your knowledge on array manipulation and C++, consider exploring additional resources such as tutorials, online platforms, and textbooks that focus on both fundamental and advanced C++ topics. This foundation will enable you to utilize C++ arrays to their utmost potential in your programming endeavors.