In C++, "call by reference" allows a function to modify the original variable passed to it by using a reference, enabling direct manipulation of the argument's value.
#include <iostream>
void modifyValue(int &num) {
num += 10; // Modifies the original variable
}
int main() {
int value = 5;
modifyValue(value);
std::cout << "Modified Value: " << value << std::endl; // Outputs: Modified Value: 15
return 0;
}
What is Call by Reference?
Call by reference is a method of passing arguments to functions in C++ where a reference (or alias) to the actual parameter is passed instead of a copy of the parameter. This allows functions to modify the actual argument variables within the calling function's context.
When we compare call by reference to call by value, the key difference lies in how data is handled. With call by value, a copy of the variable is made and passed to the function. Any changes made to the parameter within the function do not affect the original variable. In contrast, with call by reference, the function can modify the original variable directly, as it has access to the memory address of that variable. This method is particularly useful when dealing with large data structures or when you want to reflect changes back to the caller.

Syntax of Call by Reference in C++
To implement call by reference in C++, the syntax is straightforward. You declare parameters with an ampersand (&) preceding the parameter's type. This indicates that the function will receive a reference to the original variable.
Here's a basic example of using call by reference in a function:
void function(int ¶m) {
param += 10;
}
In this example, `param` is a reference to an integer variable, meaning any modification to `param` directly affects the original variable passed to the function.

Advantages of Call by Reference
Performance Benefits
One of the primary advantages of using call by reference is improved performance, especially when dealing with large data structures such as arrays, vectors, and user-defined classes. By passing a reference instead of a copy, the function avoids the overhead of copying potentially large amounts of data, which leads to faster execution.
Modifying Arguments
Another important aspect is that call by reference allows a function to modify the original arguments. This capability can be powerful when you need to apply changes to variables without returning values explicitly. It streamlines the process, making your code cleaner and more efficient.
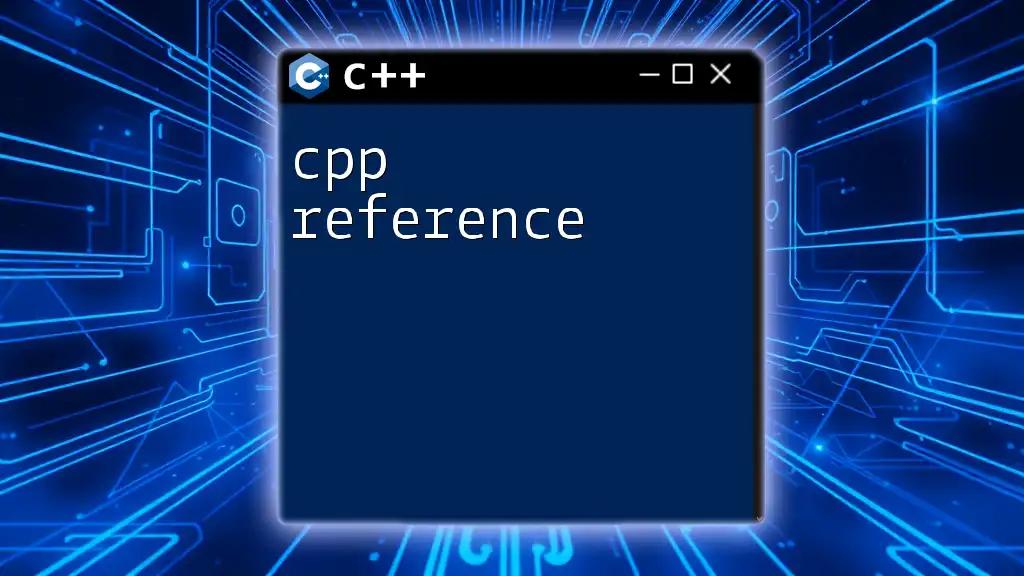
Disadvantages of Call by Reference
Despite its advantages, call by reference comes with some drawbacks.
Potential Side Effects
One significant concern is the potential for unintended side effects. When a function modifies its parameters, the caller is left unaware of these changes unless properly documented. This can lead to bugs that are difficult to trace, especially in large codebases.
Complexity in Debugging
Moreover, when multiple functions and variables are interacting through references, debugging can become more complex. You may encounter situations where you need to track down where a variable was modified, which can lead to confusion.

Examples of Call by Reference in C++
Simple Example
Here's a simple function using call by reference to demonstrate the concept:
void increment(int &n) {
n++;
}
int main() {
int number = 5;
increment(number);
// number is now 6
}
In this example, the `increment` function takes a reference to `number`. When `increment` is called, it increments the value of `number` directly, demonstrating how call by reference allows modification of the original variable.
Practical Example: Swapping Values
A practical application of call by reference is in a swapping function. The following code demonstrates how two values can be swapped using references:
void swap(int &a, int &b) {
int temp = a;
a = b;
b = temp;
}
int main() {
int x = 10, y = 20;
swap(x, y);
// x is 20, y is 10
}
In this case, the function `swap` receives two integer references. The original variables `x` and `y` are modified directly, illustrating the utility of call by reference in practical coding scenarios.
Working with Objects
Call by reference is also applicable when working with objects. The following code shows how a function can modify an object's attributes via reference:
class Box {
public:
int volume;
Box(int v) : volume(v) {}
};
void changeVolume(Box &b, int newVolume) {
b.volume = newVolume;
}
int main() {
Box box1(100);
changeVolume(box1, 200);
// box1.volume is now 200
}
In this example, `changeVolume` modifies the `volume` property of the `Box` object `box1` directly, allowing changes to be reflected back in the `main` function.
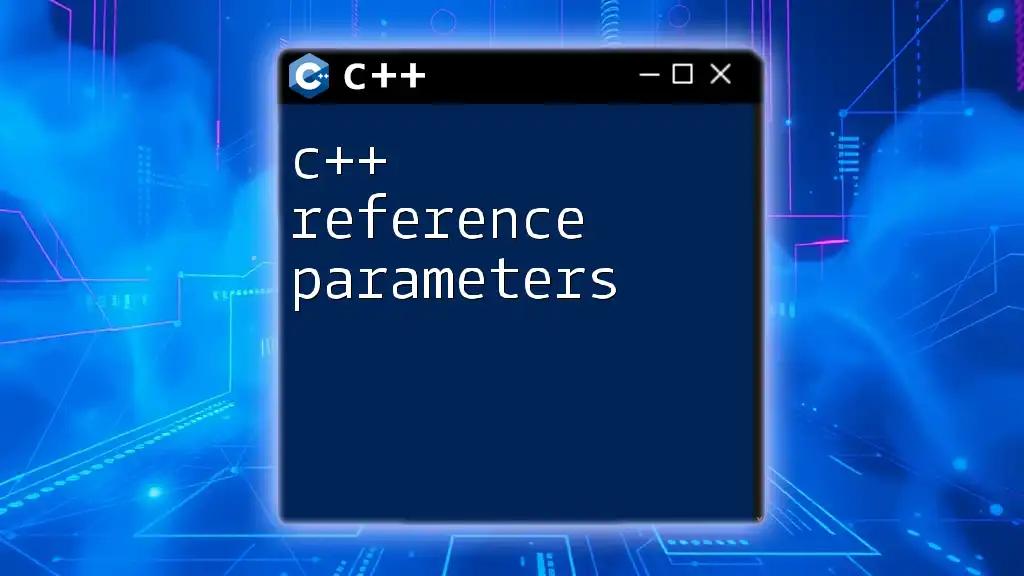
Call by Reference vs Call by Value
When considering whether to use call by reference or call by value, it's helpful to evaluate both approaches.
Aspect | Call by Reference | Call by Value |
---|---|---|
Performance | Faster, especially for large data | Slower due to data copying |
Safety | Risk of unintended modifications | Safer, as original data remains unchanged |
Use Case | Use when modifications are needed | Use for immutable or temporary data |
In scenarios where data safety is paramount, call by value is preferable. However, for large data structures or when direct modifications are required, call by reference is often the better choice.

Best Practices for Using Call by Reference
To maximize the benefits while minimizing risks, consider the following best practices:
-
Guidelines on Usage: Utilize call by reference when dealing with large structures or when you need to modify the caller's variable. For smaller, primitive types or when data integrity is crucial, prefer call by value.
-
Naming Conventions: Use clear and descriptive names for reference parameters to enhance code readability. For example, appending `&` to the variable name can signal to the reader that the parameter is a reference.
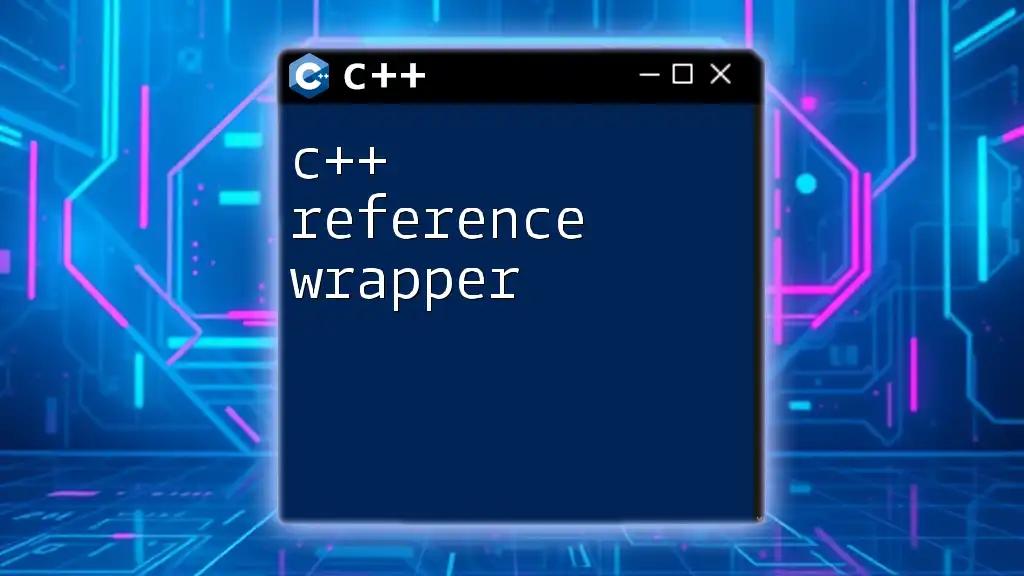
Common Mistakes When Using Call by Reference
While using call by reference can simplify your code, there are common pitfalls developers should avoid:
-
Forgetting to use the ampersand (&) when declaring function parameters can lead to the function treating variables as pass-by-value instead of as references.
-
Modifying read-only variables or constants can result in compilation errors, as references must point to modifiable entities.
-
Not considering the lifetime and scope of referenced variables can lead to dangling references and undefined behavior.

Conclusion
Understanding call by reference in C++ is crucial for effective programming. It provides a method to handle and manipulate data efficiently, but with great power comes great responsibility. By recognizing the advantages and disadvantages, developers can make informed choices about when to use this approach, thereby enhancing their code's performance and clarity. It's crucial to practice and experiment with these concepts to gain a deeper understanding and proficiency in C++.