C++ Return Value Optimization (RVO) is a compiler optimization technique that eliminates the overhead of copying objects when returning a value from a function, promoting efficiency and performance.
#include <iostream>
class MyClass {
public:
MyClass() { std::cout << "Constructor called\n"; }
MyClass(const MyClass&) { std::cout << "Copy constructor called\n"; }
};
MyClass createObject() {
return MyClass(); // RVO avoids the copy constructor
}
int main() {
MyClass obj = createObject(); // RVO takes place here
return 0;
}
What is Return Value Optimization?
C++ Return Value Optimization, commonly referred to as RVO, is a compiler optimization technique that eliminates unnecessary copying of objects when they are returned from functions. This optimization becomes particularly significant in performance-sensitive applications where every cycle counts. RVO allows the compiler to construct the returned object directly into the memory space of the caller, avoiding expensive copy constructors or move constructors.
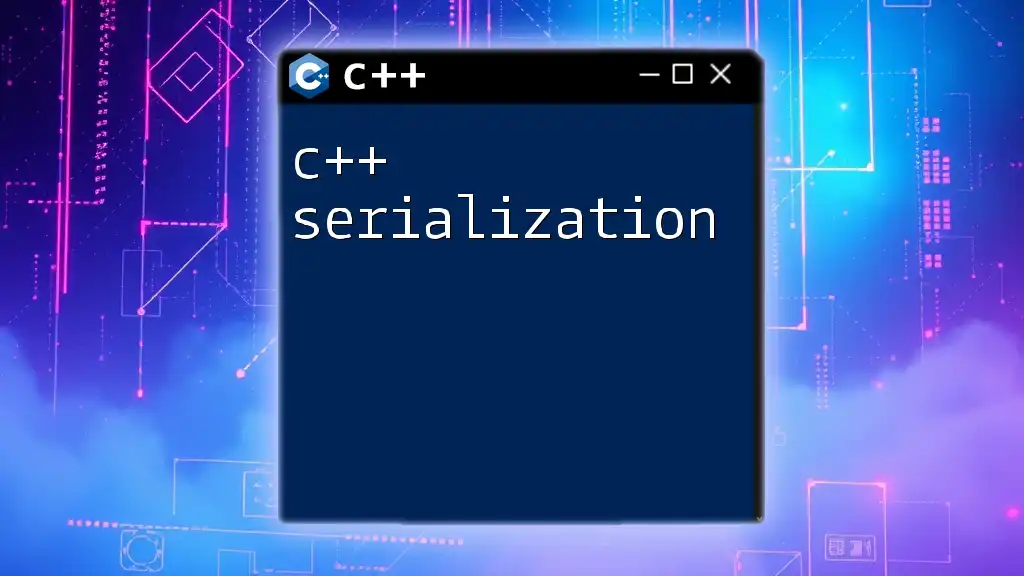
Why is RVO Important?
Understanding the overhead associated with copy construction is crucial for optimizing performance in C++. Each copy involves calling the copy constructor, which can be costly when dealing with larger objects. By utilizing RVO, developers are able to reduce the number of unnecessary copies, leading to more efficient and faster code execution. The benefits of RVO can manifest significantly in scenarios where large data structures, such as vectors or matrices, are returned from functions.
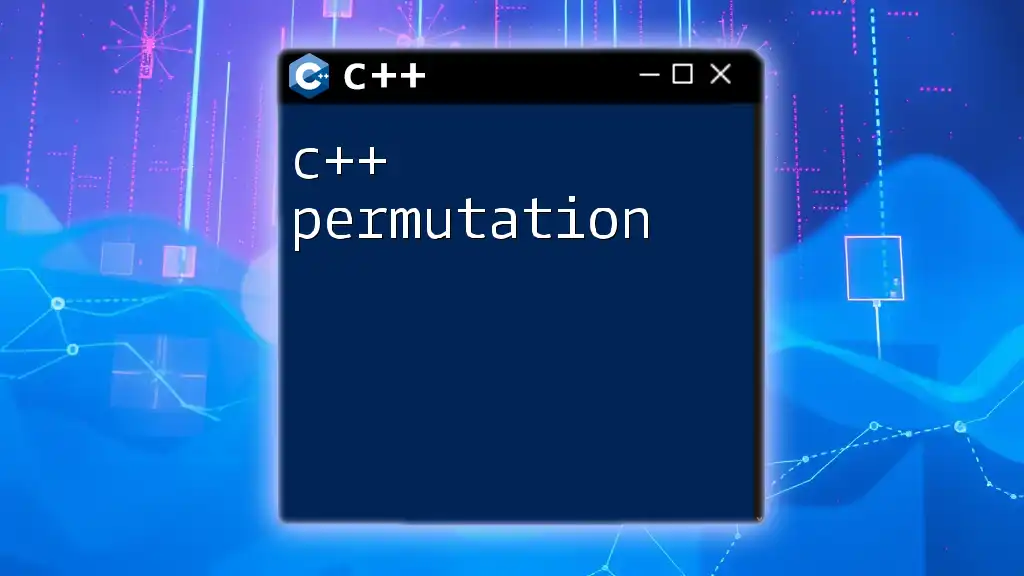
The Importance of Move Semantics in C++11 and Beyond
With the introduction of C++11, move semantics have changed the landscape of resource management in C++. Move semantics allow the resources of one object to be "moved" to another rather than being copied. This is when RVO becomes even more powerful, as it works seamlessly with move constructors to minimize both copy and move operations. By ensuring that temporary objects are constructed directly in the space where the return value is expected, RVO can lead to substantial performance improvements.
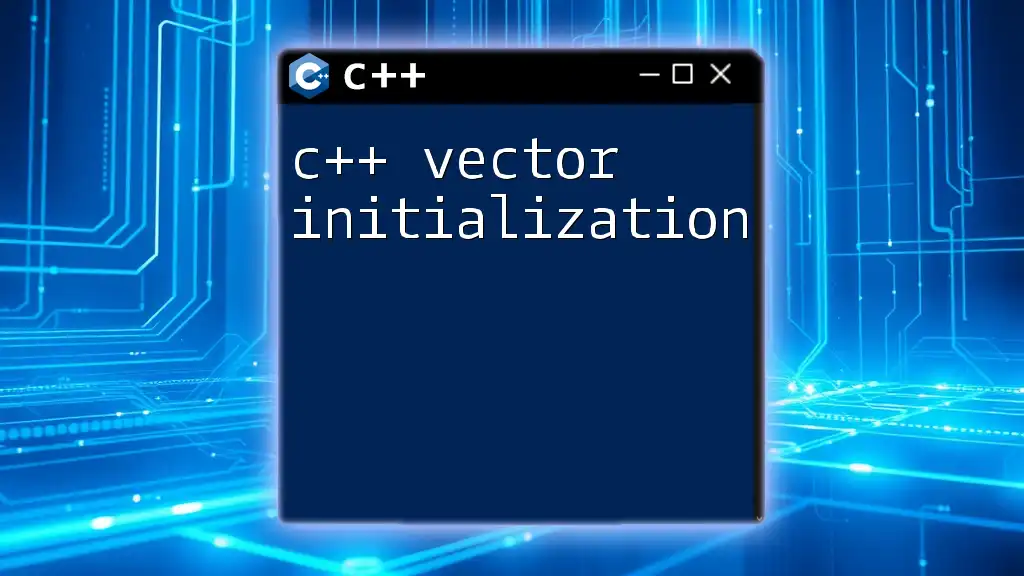
Copy Elision: What You Need to Know
Copy elision is a closely related concept where the compiler is allowed (or required, depending on the standard) to omit unnecessary copying of objects. Unlike RVO, which specifically deals with return values, copy elision can occur in other scenarios as well, such as in the initialization of temporaries. Both RVO and copy elision contribute to a cleaner, more efficient execution of C++ programs.
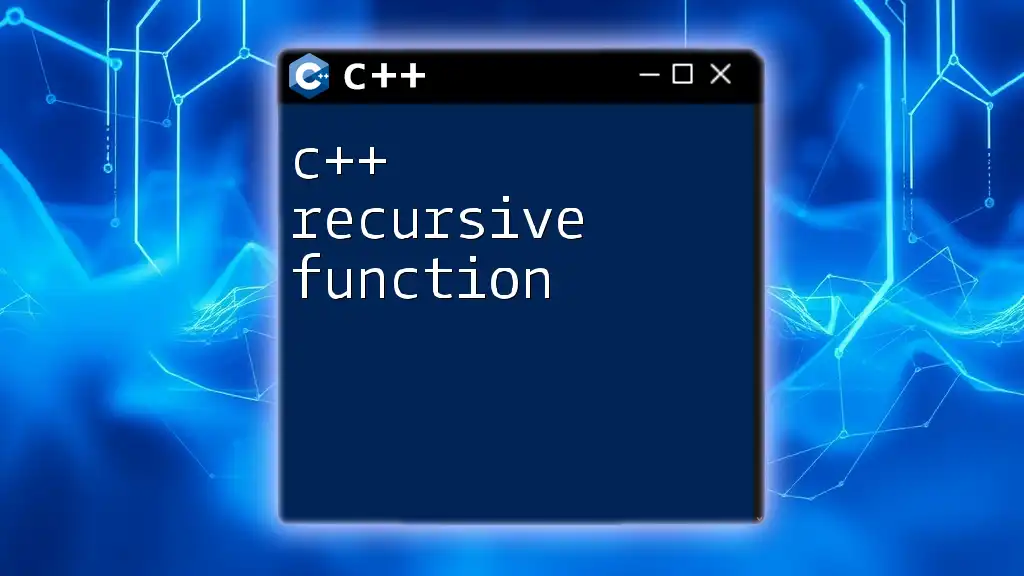
How RVO Works Behind the Scenes
The mechanics of RVO involve several intricate steps taken by the compiler:
- Function Identification: The compiler identifies functions that return large objects.
- Space Allocation: Instead of creating a temporary object that resides in its own space, the compiler allocates memory in the caller's stack frame.
- Direct Construction: The object is constructed directly in the allocated space, bypassing the intermediate temporary object.
This process ensures that the object is returned without the overhead of copying, thus improving performance without altering the programming model.
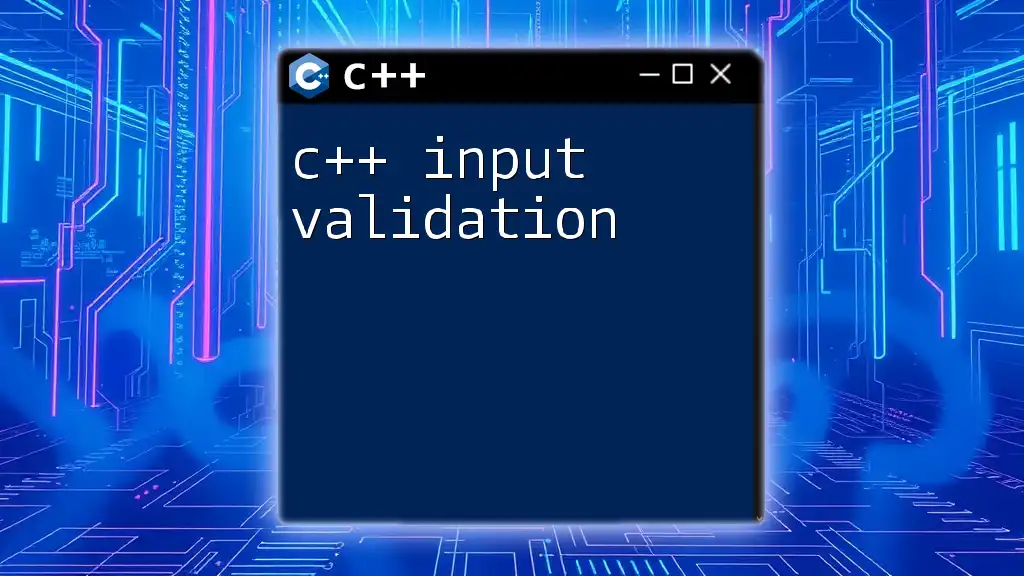
Compiler Optimizations
Different compilers may implement RVO or copy elision in slightly varying ways, but the underlying principle remains the same. For instance, the GCC compiler often performs aggressive optimizations with RVO during the compilation stage, while Clang and MSVC follow suit with their optimization strategies.
Consider the following example to illustrate this:
class MyClass {
public:
MyClass() { /* Constructor logic */ }
MyClass(const MyClass&) { /* Copy constructor logic */ }
MyClass(MyClass&&) noexcept { /* Move constructor logic */ }
};
MyClass createObject() {
return MyClass(); // RVO will likely eliminate the copy/move here
}
In the above example, if the compiler applies RVO, the `MyClass` object will be constructed directly in the memory allocated for the result of `createObject`, avoiding the invocation of the copy or move constructor.
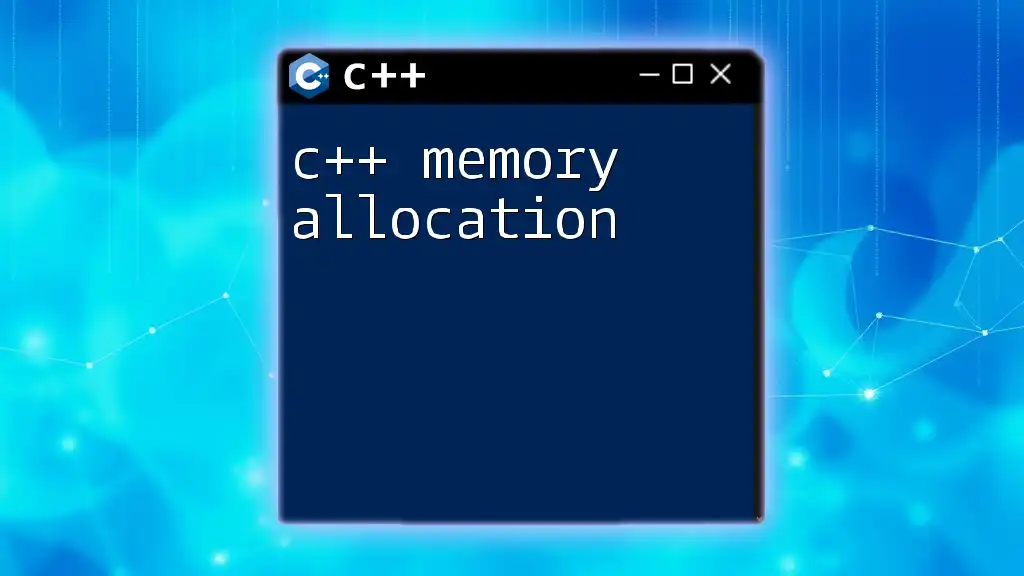
Best Practices for Leveraging RVO
To leverage RVO effectively, consider the following best practices:
- Return Objects by Value: Prefer returning objects by value rather than pointers or references to make the most out of RVO.
- Use Constructors Wisely: Ensure that your constructors are designed to allow for optimization.
- Minimize Complexity: Simpler code structures often lead to better optimization opportunities by the compiler.
When RVO May Not Apply
Despite its benefits, there are certain conditions where RVO is not guaranteed:
- Complex Expressions: If the returned value is part of a complex expression, RVO may be difficult for the compiler to apply.
- Dynamic Allocation: If you return an object through a pointer, RVO will not take effect since dynamic memory management does not allow direct construction in the caller.
For instance, in the below code, RVO would not be applied, and a copy would occur:
MyClass* createObjectWithPointer() {
return new MyClass(); // No RVO possible here
}
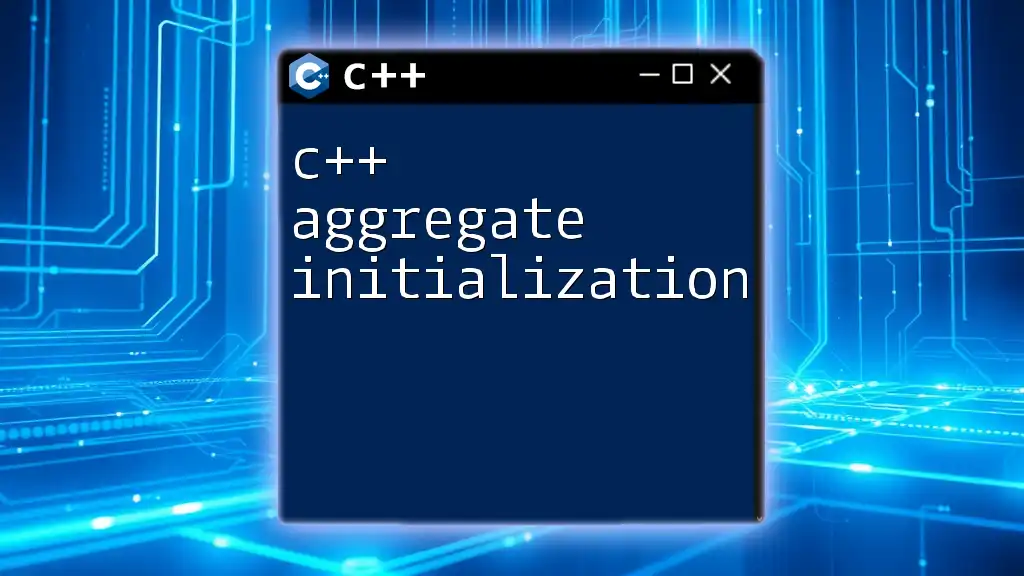
Compiler Differences and RVO
It is essential to be aware of how different compilers handle RVO. GCC, Clang, and MSVC have unique optimization behaviors and thresholds at which they apply RVO. To ensure RVO is effectively applied, it is essential to test and validate your code against various compilers, particularly if you are targeting multiple platforms.
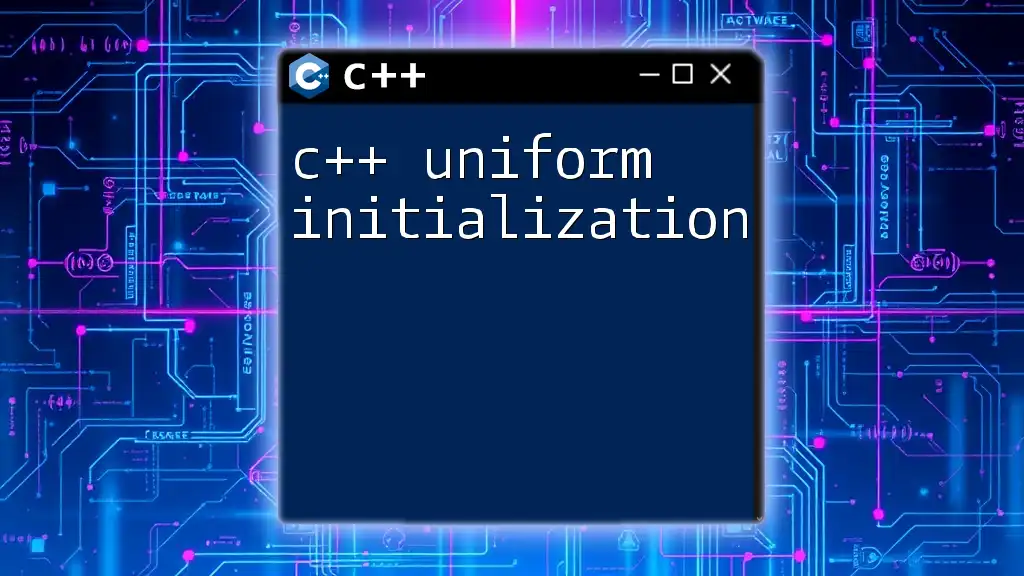
Practical Examples of RVO in Action
Basic Example of RVO
To understand RVO better, let’s start with a simple example:
class Point {
public:
Point(int x, int y) : x_(x), y_(y) {}
// Other member functions...
private:
int x_, y_;
};
Point createPoint() {
return Point(1, 2); // RVO can optimize away the temporary
}
In this example, the `Point` object can be constructed directly into the caller's stack frame, resulting in an efficient return.
Advanced Example with Move Semantics
Here's a more complex implementation that combines RVO with move semantics:
#include <vector>
class LargeData {
public:
LargeData(size_t size) : data_(size) {}
LargeData(LargeData&& other) noexcept : data_(std::move(other.data_)) {}
// Other member functions...
private:
std::vector<int> data_;
};
LargeData createLargeData() {
LargeData temp(1000); // Creates a large object
return temp; // RVO applied here
}
In the above example, not only does RVO optimize out unnecessary copy, but move semantics also ensure that if RVO does not apply (specific compiler optimizations), the move constructor is there as a fallback, further optimizing performance.
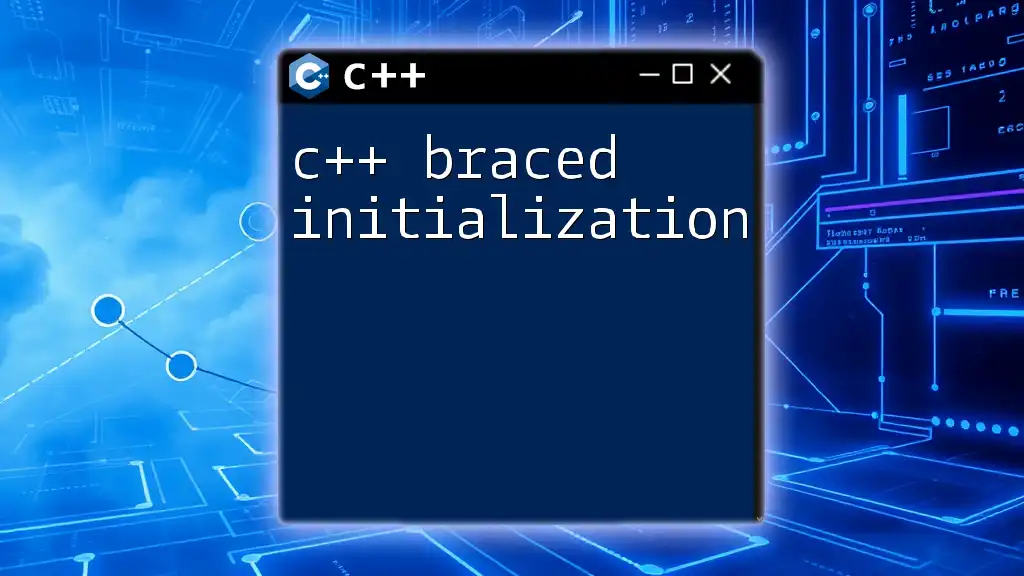
Measuring Performance Gains
To ascertain the performance benefits of RVO in your application, you can use profiling tools such as `gprof`, `valgrind`, or built-in high-resolution clock functions in C++. By comparing execution times, memory usage, and object construction counts, you can identify the differences between code with and without RVO.
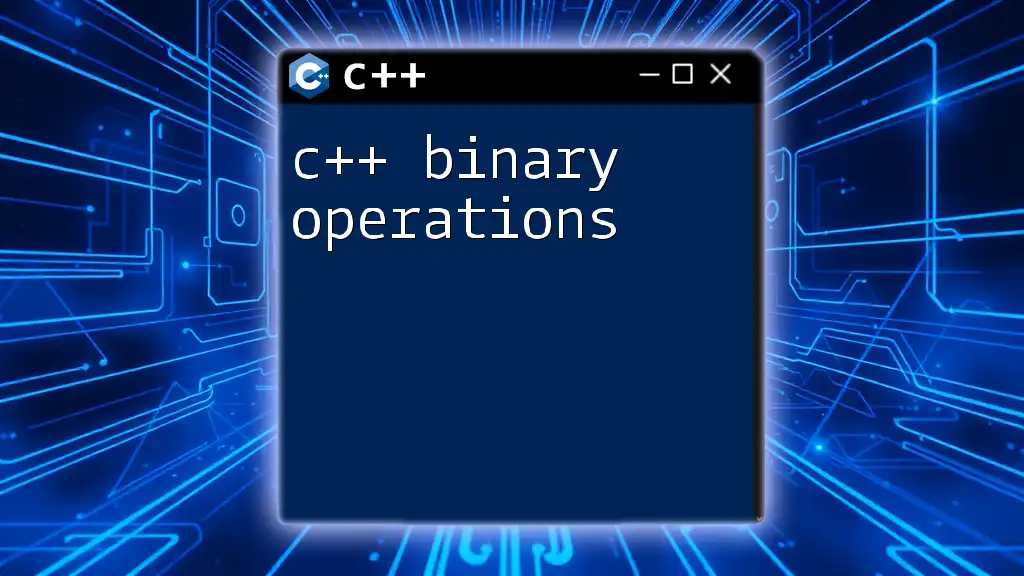
Real-World Application of RVO
In a real-world context, companies often rely on RVO to ensure their applications run efficiently. High-performance computing, game development, and large-scale data processing frequently optimize their C++ use cases by embracing RVO. Developers can accommodate RVO concerns in their algorithmic designs, leading to faster execution times and more efficient resource management.
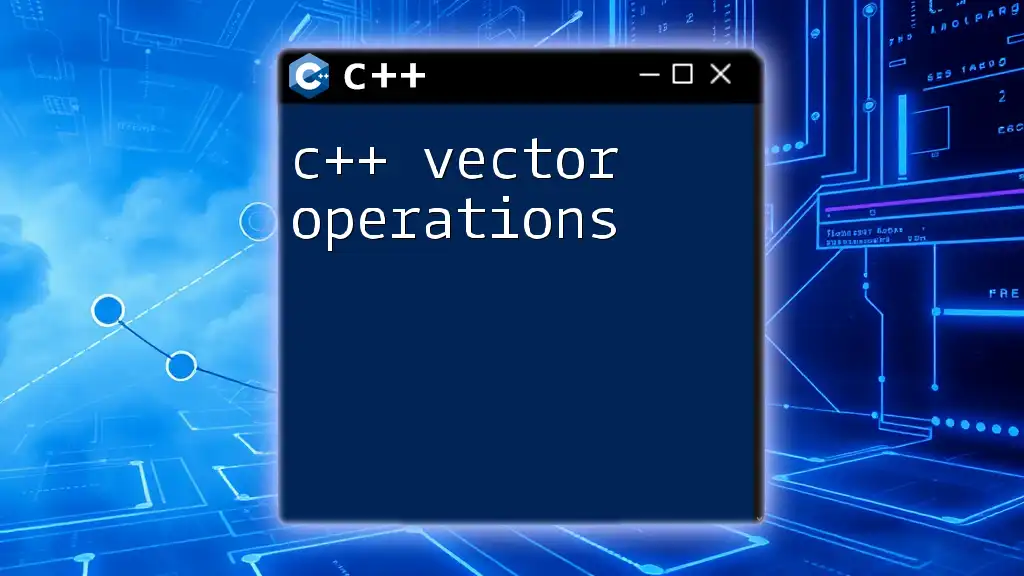
Recap of Key Takeaways
Throughout this exploration of C++ Return Value Optimization, we have observed its potential to dramatically improve performance by minimizing object copies. Understanding when and how RVO applies introduces developers to a powerful optimization tool within their coding arsenal.
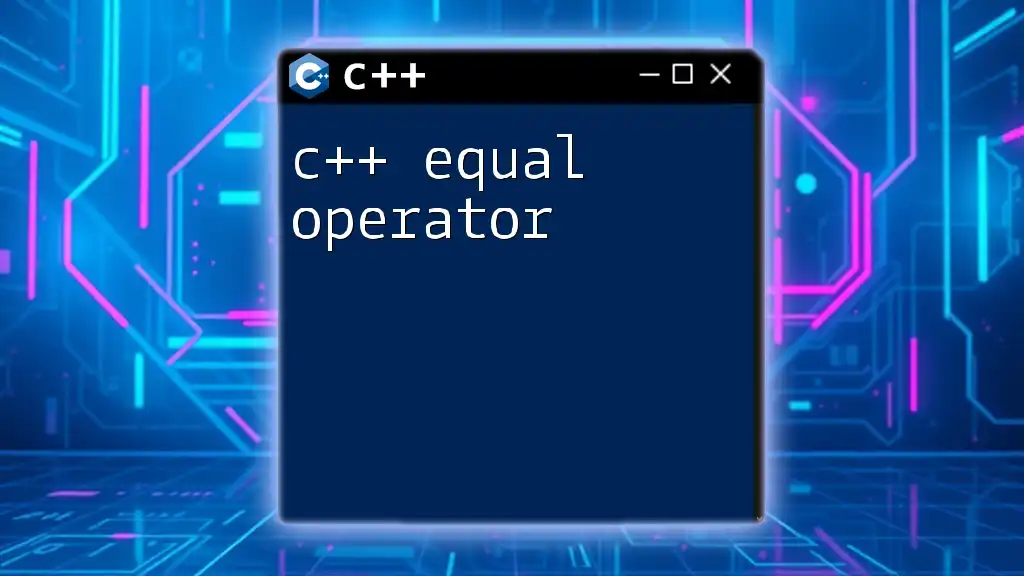
Final Thoughts on C++ Performance Optimization
In conclusion, C++ Return Value Optimization is a critical concept for accelerating applications and reducing overhead associated with object copying. By applying best practices, embracing modern C++ features, and leveraging compiler optimizations, developers can write cleaner and more efficient code, delivering improved performance.
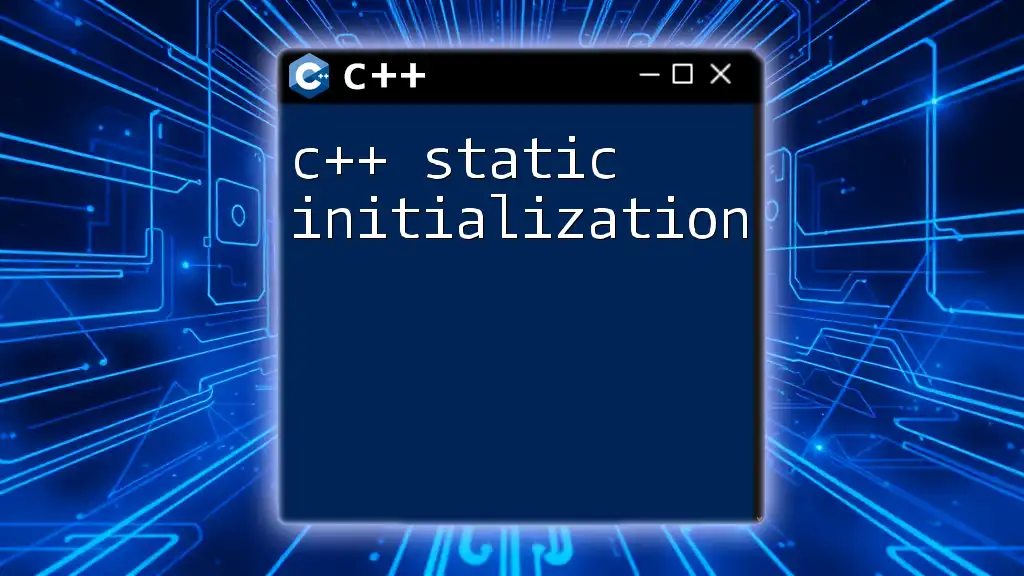
Further Reading and References
For those looking to deepen their understanding of C++ performance optimization, consider exploring authoritative texts and articles focusing on object-oriented programming, memory management, and compiler optimizations.
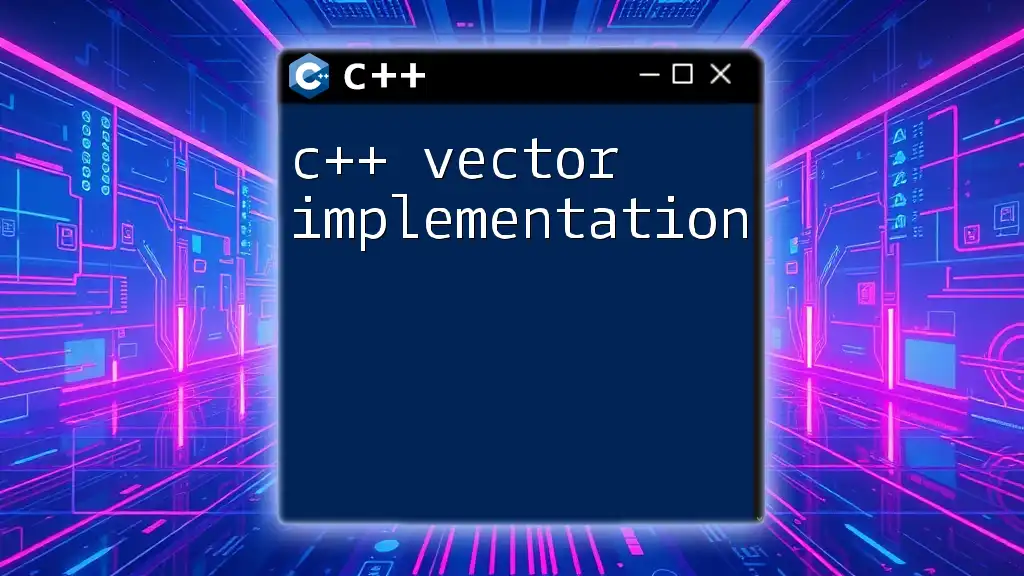
Related Topics for Continued Learning
- Move Semantics
- Performance Profiling Tools
- Effective C++ programming techniques