In C++, the `private` access specifier restricts access to class members, allowing them to be accessed only within the class itself and not from outside or derived classes.
class MyClass {
private:
int secretNumber;
public:
void setSecret(int num) {
secretNumber = num;
}
int getSecret() {
return secretNumber;
}
};
Overview of C++ Accessibility Modifiers
Understanding Access Modifiers in C++
Access modifiers in C++ play a crucial role in defining the visibility of class members. They determine how and where these members can be accessed. This is fundamental for achieving encapsulation, which is a core concept in object-oriented programming (OOP). Encapsulation helps safeguard data by restricting direct access to some of an object's components.
The Three Main Access Specifiers
C++ offers three primary access specifiers: public, protected, and private.
-
Public: Members declared as public are accessible from anywhere in the code, meaning any part of the program can interact with these members.
-
Protected: Members defined as protected are accessible within their own class and by derived classes (subclasses) but not from outside the class hierarchy.
-
Private: Members marked as private are restricted to their own class. This means no other part of the code can directly access these members, promoting stricter control over data access.
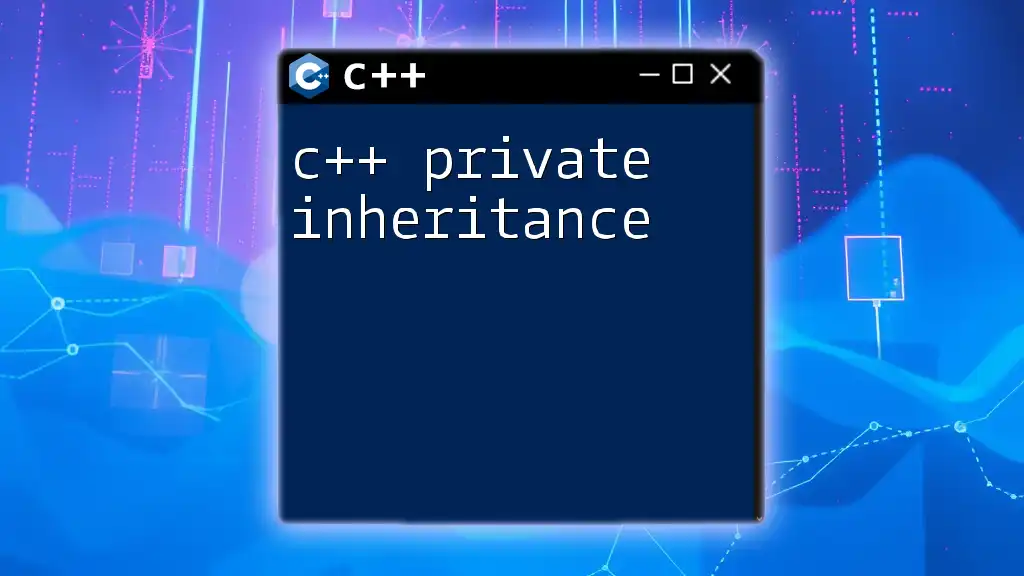
The Concept of Private Access in C++
What Does Private Mean in C++?
In C++, the term private refers to the accessibility level of class members. When a member is declared private, it is not accessible from any function or object outside its class. This is similar to having a secret room in a house—only the house's owner (the class itself) knows how to enter.
The Purpose of Private Members
The main goal of private members is to protect data integrity. They prevent unauthorized access and modification from outside the class. By encapsulating data, you eliminate the chances of unintended interactions that could disrupt the internal state of an object.
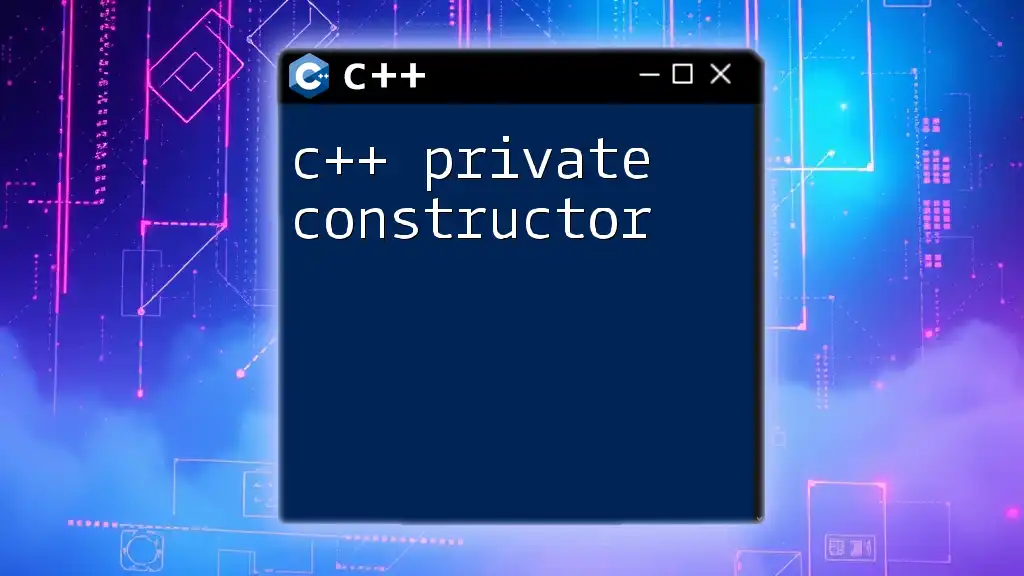
Using Private in Class C++
Declaring Private Members in a Class
To declare private members in a class, you simply use the `private` keyword before the member declarations. Here's an example illustrating how to do this:
class Sample {
private:
int secretNumber;
public:
void setSecret(int num) {
secretNumber = num;
}
int getSecret() {
return secretNumber;
}
};
In this example, `secretNumber` is a private member of the `Sample` class. It is only accessible through the public member functions `setSecret` and `getSecret`. This encapsulation prevents external entities from directly modifying `secretNumber`.
Accessing Private Members
To interact with private members, you must use public member functions that can be called from outside the class. The previous example demonstrates this approach:
Sample obj;
obj.setSecret(42);
std::cout << "Secret Number: " << obj.getSecret() << std::endl;
Here, `setSecret` allows you to set the value of the private `secretNumber`, and `getSecret` retrieves it. This method provides a controlled way to manage how private data is accessed and modified.
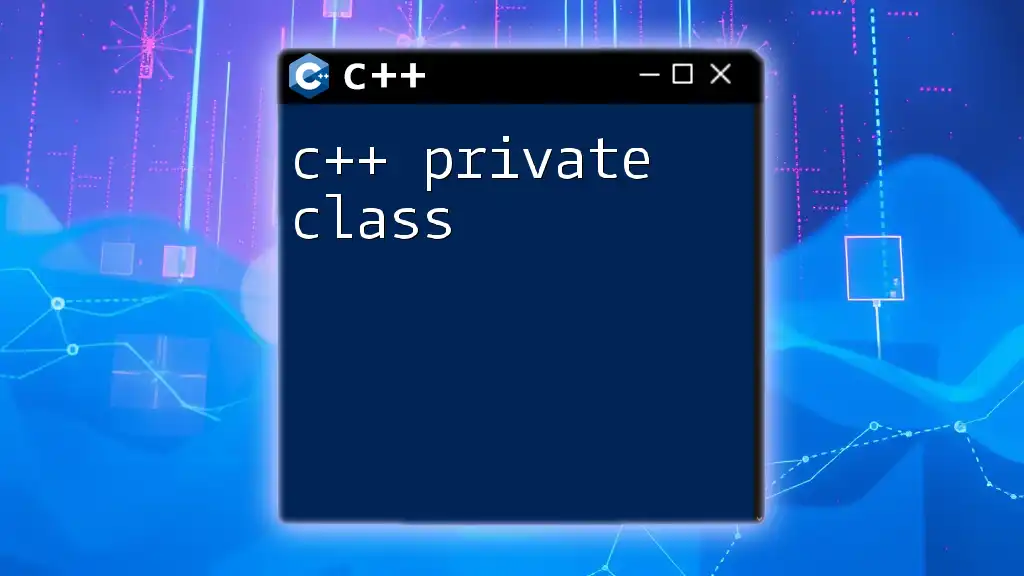
Private Class in C++
Defining a Class as Private
In addition to private members, C++ allows you to define entire classes as private within another class. This is often referred to as a private inner class. The syntax is as follows:
class Outer {
private:
class Inner {
public:
void display() {
std::cout << "Inner class method called." << std::endl;
}
};
};
In this example, the `Inner` class is declared private within the `Outer` class. As a result, instances of `Inner` cannot be directly accessed from outside the `Outer` class. This is useful for grouping functionalities that should not be exposed to the outside world.
Use Cases for Private Classes
Private classes are beneficial when you want to implement helper functionalities that aren't intended for public use. They allow for better organization of code while maintaining encapsulation. For instance, an internal data structure that supports the operations of the outer class without exposing itself to the users can be implemented as a private class.
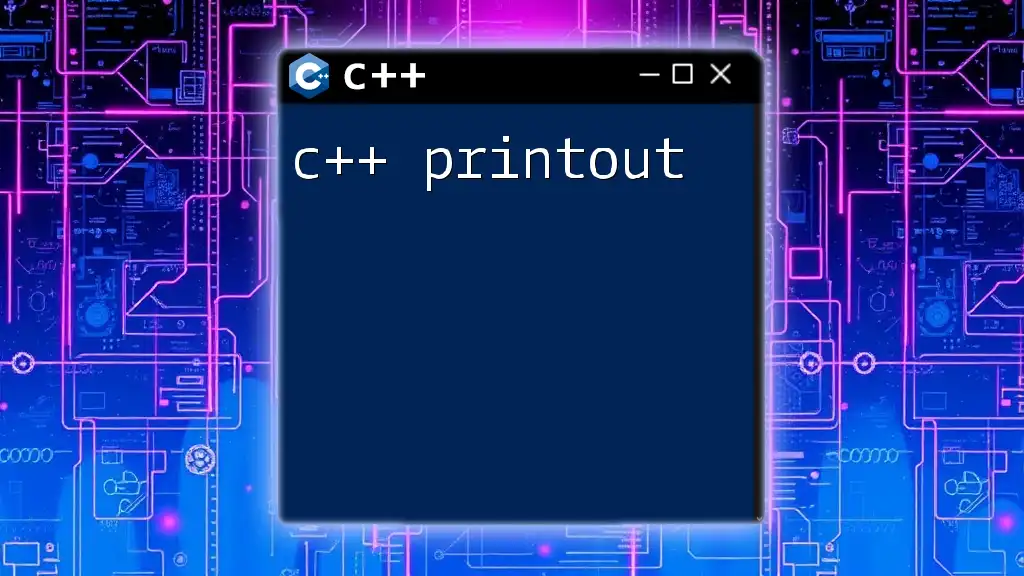
Best Practices for Using Private Members
Avoiding Excessive Use of Private Members
While private members are important for data protection, it's crucial to avoid overusing them. Excessively privatizing members can lead to an overly complex interface. Always consider the future extensibility of your class. Sometimes, using public or protected members may offer the required flexibility for subclassing or changes in functionality.
Balancing Encapsulation and Flexibility
Carefully balance the need for encapsulation with usability. Aim for a design where the private members are only as restrictive as necessary. For instance, if certain operations could facilitate better interactions without exposing data, consider using protected members or friend classes to promote flexibility while maintaining security.
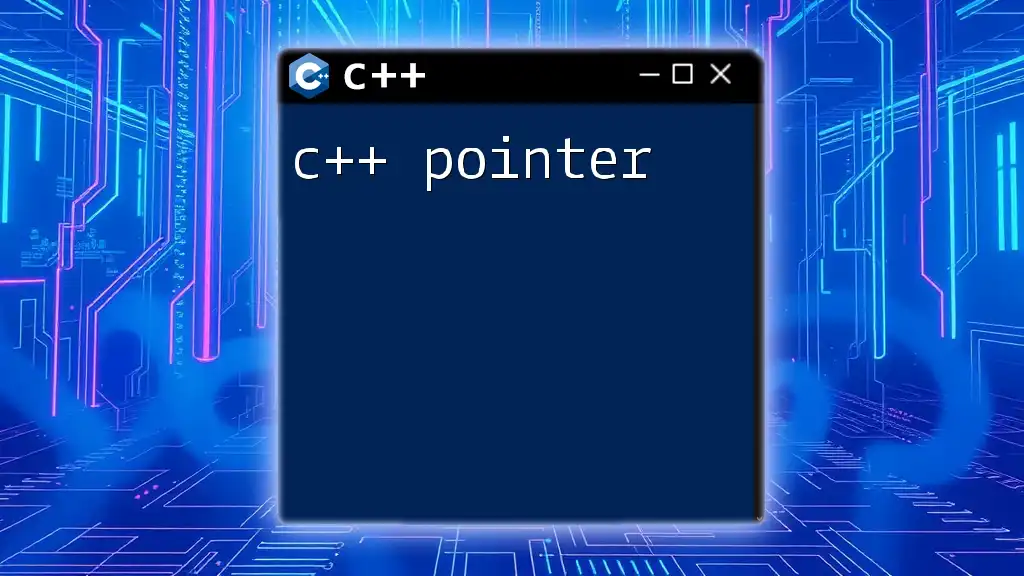
Common Mistakes with Private Members
Forgetting to Use Access Modifiers
One common mistake is neglecting to declare members with the appropriate access modifiers. This can lead to vulnerabilities as intended encapsulation is lost. Always strive to explicitly declare access modifiers, even if the default access (for classes it's private) seems adequate.
Misusing Private Access
Misusing private access can create situations where you inadvertently make a class too rigid and difficult to extend. For example, if you lock away too many members as private without proper public accessors or mutators, you may make it hard for subclasses to inherit and extend functionality.
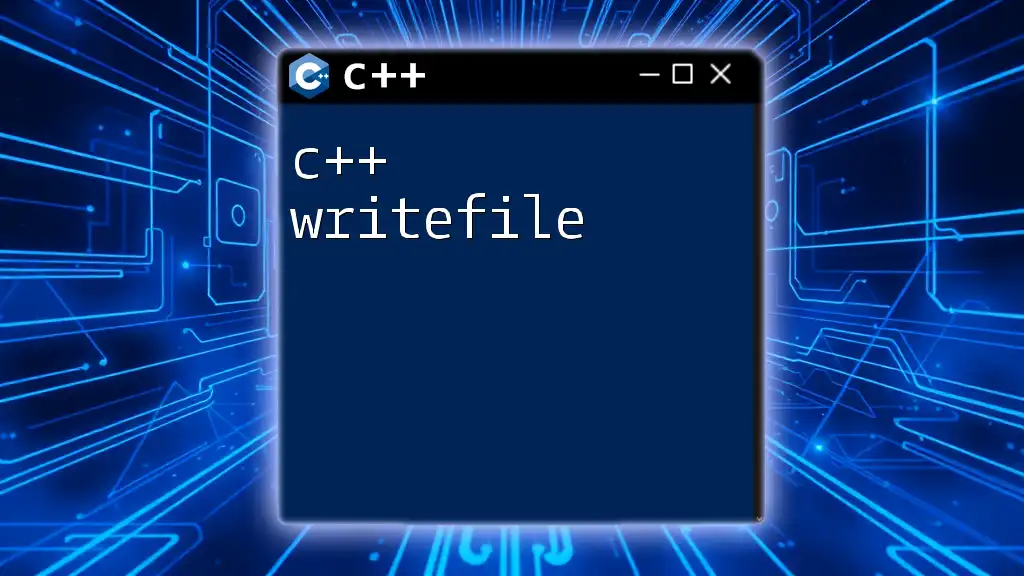
Conclusion
Recap on the Importance of Private in C++
In summary, the use of private access specifier in C++ plays a vital role in protecting data integrity and enforcing encapsulation. By implementing private members, you ensure that data can only be accessed and modified through controlled interfaces, ultimately leading to cleaner and more maintainable code.
Next Steps in Learning C++
As you progress in your C++ learning journey, it’s beneficial to explore further topics related to access control, such as inheritance and polymorphism, to fully grasp how encapsulation can influence design patterns.
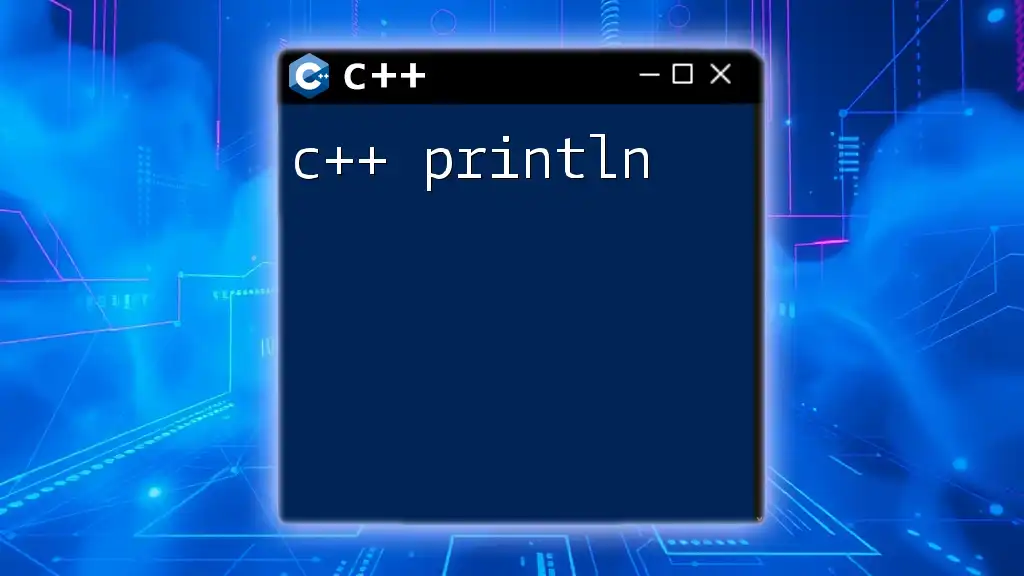
FAQ Regarding C++ Private
What Happens If I Don’t Use Private Access?
Failing to use private access can expose your class members to the outside, risking unintended modifications. This often results in buggy code or security issues.
Can Private Members Be Accessed in Derived Classes?
No, private members cannot be accessed directly in derived classes. Only public and protected members are accessible, ensuring a clear boundary between base and derived class interactions.
How Can I Test Private Members?
Testing private members can be a challenge. One common approach is using friend classes or functions, which grant access to private members specifically for testing purposes. This allows you to maintain encapsulation while ensuring thorough testing of class functionalities.