"C++ Primer" by Lippman is a comprehensive guide for beginners that covers essential C++ concepts and programming techniques through clear explanations and practical examples.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is "C++ Primer" by Lippman?
C++ Primer, authored by Stan Lippman, Josée Lajoie, and Barbara E. Moo, is widely recognized as one of the definitive texts for learning C++. The book serves both newcomers to programming and intermediate learners seeking to deepen their understanding of C++. It guides readers from basic syntax to more advanced topics in a clear and systematic manner, making it an essential resource for anyone serious about mastering the language.
Overview of the Book
Initially published in the early 1990s, "C++ Primer" has undergone multiple revisions to keep pace with the evolving C++ standards. Each edition enriches itself with updated content, new examples, and best practices, ensuring relevance for modern developers. The authors bring their extensive experience in both programming and teaching to this text, making complex topics accessible.
Target Audience
- Beginners: If you're new to programming or coming from another language, this book walks you through fundamental C++ concepts with clarity.
- Intermediate Learners: Those with some background in C++ can deepen their competency through the nuanced discussions and advanced features presented in the book.
- Educators: The structured approach and comprehensive content provide excellent material for teaching courses on C++.
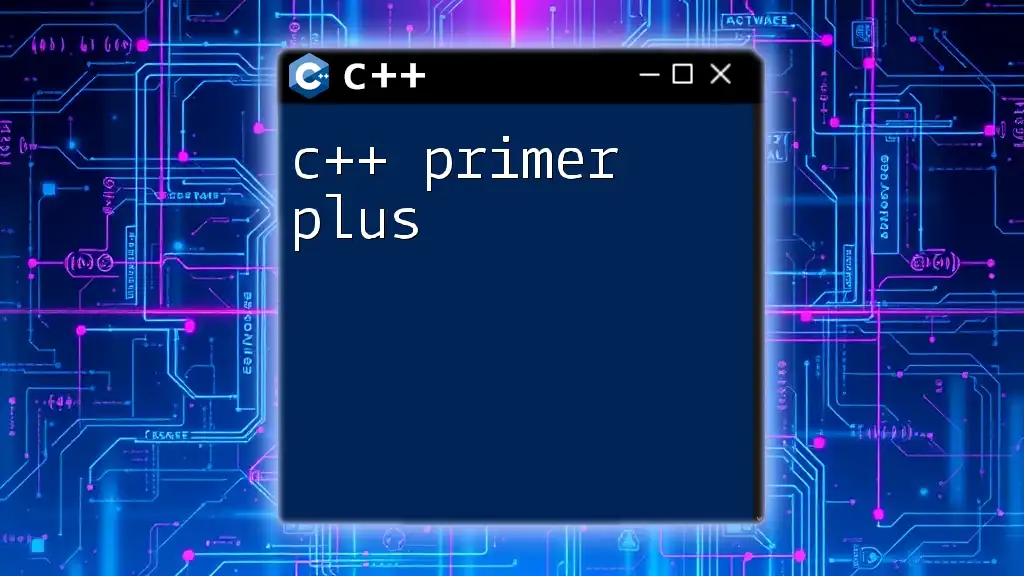
Structure of the Book
How the book is organized is crucial for understanding its effectiveness. It is structured into several parts, each focusing on different aspects of C++. From introductory concepts like basic syntax to advanced features such as templates and the Standard Template Library (STL), readers are guided step-by-step.
Learning Methodology
The authors emphasize a hands-on approach. Each chapter includes practical examples and exercises that reinforce concepts. This methodology encourages readers to apply what they've learned immediately, resulting in better retention and understanding of C++.
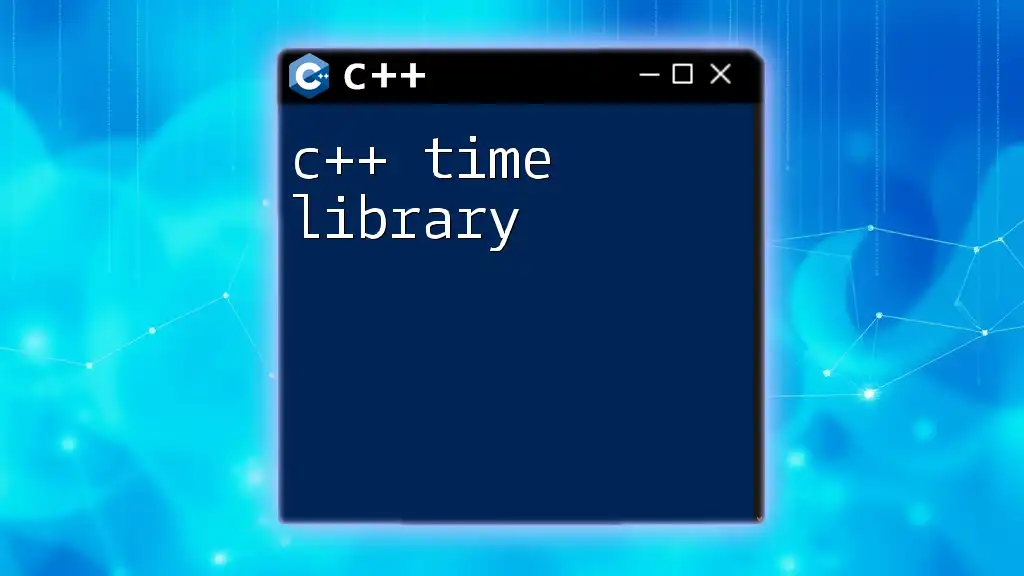
Key Concepts from "C++ Primer"
Basic Language Features
Understanding the basic syntax and semantics of C++ is critical. The book covers various topics including data types, variables, and operators in detail, illustrated with code snippets.
Example:
int main() {
int a = 5;
int b = 10;
std::cout << "Sum: " << (a + b) << std::endl;
return 0;
}
This simple code demonstrates variable declaration, arithmetic operations, and output, establishing the foundational skills needed in C++ programming.
Classes and Objects
An in-depth understanding of Object-Oriented Programming (OOP) is essential, and "C++ Primer" thoroughly covers these principles. The book explains how to create classes and objects, emphasizing encapsulation.
Example:
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
This example illustrates class definition and method creation, introducing the concept of objects and how they interact in C++.
Memory Management
Another critical area highlighted in the book is memory management, especially the differences between dynamic and static allocation. The text delves into the importance of using `new` and `delete` for efficient memory handling.
Example:
int* p = new int(5); // dynamically allocated
delete p; // proper deallocation
This snippet shows how to allocate and deallocate memory effectively, helping you to avoid memory leaks that can disrupt program execution.
Templates and Generic Programming
Templates are a powerful feature of C++ that allows for generic programming. The book introduces the concept and benefits of templates in enhancing code reusability and versatility.
Example:
template<typename T>
T add(T a, T b) {
return a + b;
}
This function template exemplifies how you can create functions that operate on any data type, making your code more adaptable.
STL (Standard Template Library)
The Standard Template Library (STL) is another focal point in "C++ Primer." It provides a rich set of generic classes and functions that implement common data structures and algorithms.
Example:
#include <vector>
std::vector<int> numbers = {1, 2, 3, 4, 5};
This snippet initializes a vector, showcasing how STL makes it easier to handle collections of data, further demonstrating the power and flexibility C++ offers.
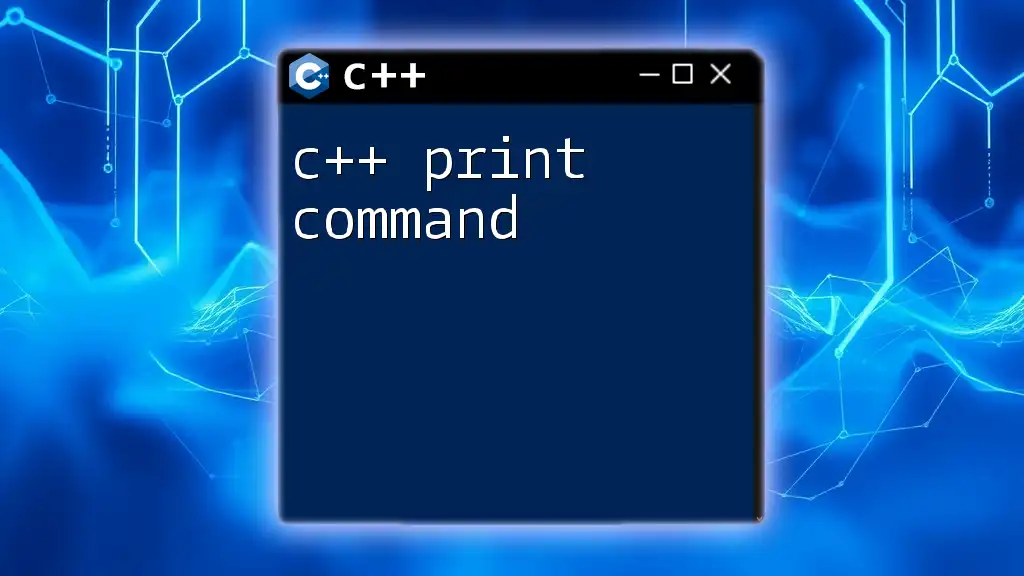
Practice Problems and Solutions
One significant highlight of learning from "C++ Primer" is the importance of practice. The book includes numerous exercises that challenge your understanding and application of concepts. By working through these problems, you reinforce your skills and deepen your grasp on complex topics.
Consider tackling problems ranging from basic syntax to designing classes and implementing algorithms. Solutions often accompany these exercises, providing an opportunity for self-assessment and ensuring that you can learn from your mistakes.
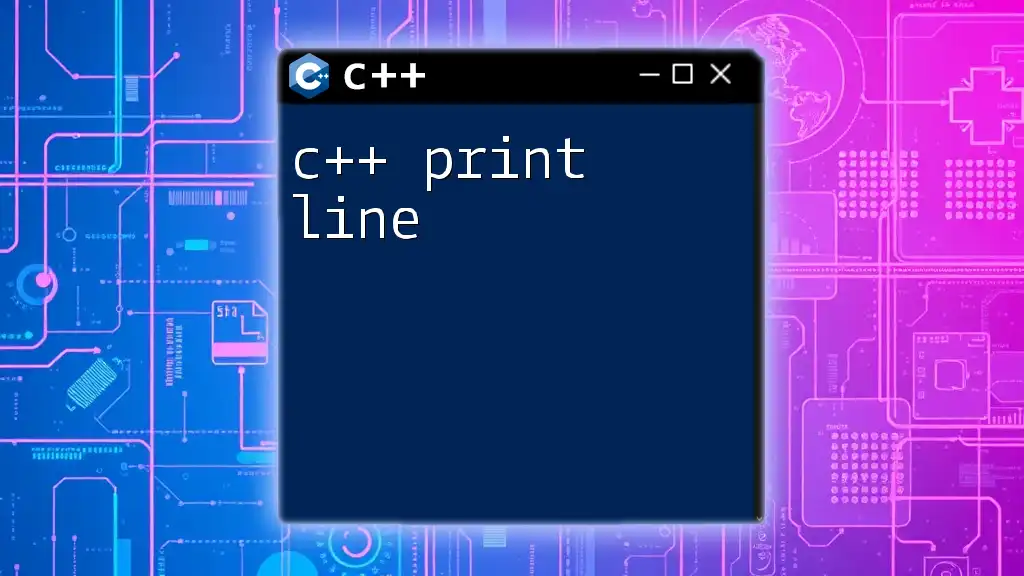
Tips for Getting the Most out of "C++ Primer"
Active Reading Techniques
To fully leverage "C++ Primer," employ active reading techniques:
- Note-taking: Capture critical concepts, examples, and code snippets as you progress.
- Annotation: Highlight areas of confusion and revisit them to ensure comprehension.
- Reviewing: Schedule time to go back over chapters to cement your understanding and retention.
Supplementary Resources
While "C++ Primer" is a comprehensive resource, supplemental materials can enhance your learning. Consider exploring:
- Additional programming books that focus on different aspects of C++.
- Online courses that offer guided instruction and hands-on projects.
- Engaging with C++ communities and forums where you can ask questions, share insights, and collaborate.
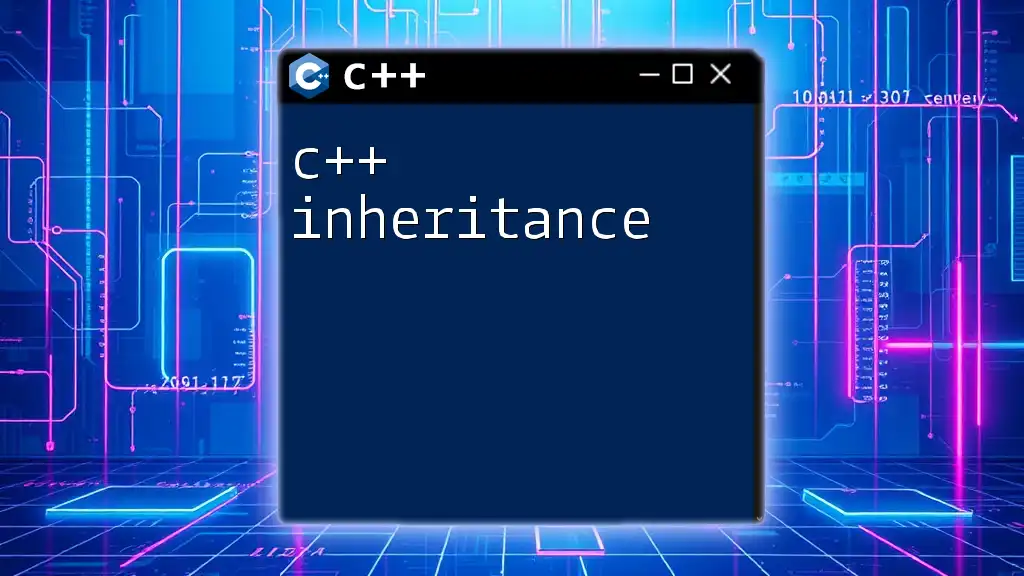
Conclusion
Mastering C++ is a journey, and "C++ Primer Lippman" is an invaluable companion on that path. Its structured approach, practical examples, and depth of content make it an essential tool for anyone serious about software development. By diving into its pages and practicing diligently, you pay the groundwork for a career in programming that could lead to exciting opportunities. Start reading, coding, and engaging with the materials today—your future self will thank you!
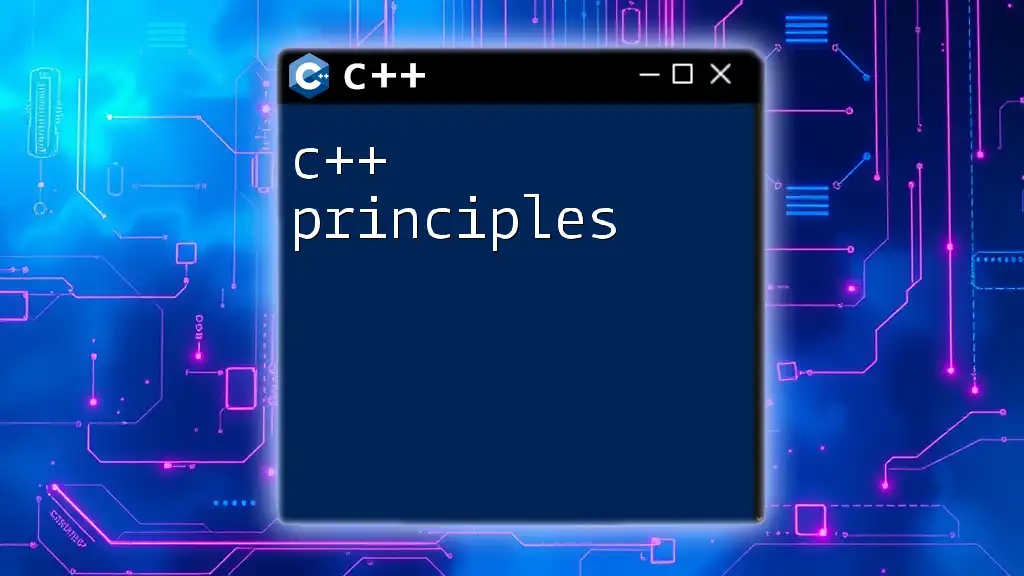
References
To maximize your learning experience, refer to additional resources, including documentation, online tutorials, and interactive coding platforms to complement your understanding gained from "C++ Primer."