In C++, you can use the `std::sort` function along with a lambda expression to sort a container based on custom criteria succinctly.
#include <algorithm>
#include <vector>
#include <iostream>
int main() {
std::vector<int> numbers = {4, 2, 3, 1, 5};
std::sort(numbers.begin(), numbers.end(), [](int a, int b) { return a < b; });
for (int n : numbers) std::cout << n << " "; // Output: 1 2 3 4 5
}
Understanding C++ Sorting
What is Sorting?
Sorting is the process of arranging the elements of a collection in a specified order, which can be ascending or descending. In programming, sorting is a fundamental operation, often a prerequisite for efficient searching and data analysis. As data sets grow larger, efficient sorting algorithms become increasingly important for performance optimization.
Introduction to C++ Sort
The C++ Standard Library provides a powerful sorting algorithm through the `std::sort` function, located in the `<algorithm>` header. This function uses the QuickSort algorithm, a highly efficient sorting method with an average time complexity of O(n log n).
Sorting is not just about ordering numbers; it has wide-reaching applications in software development, from organizing user inputs to preparing data for analysis—making it a vital skill for any C++ programmer.
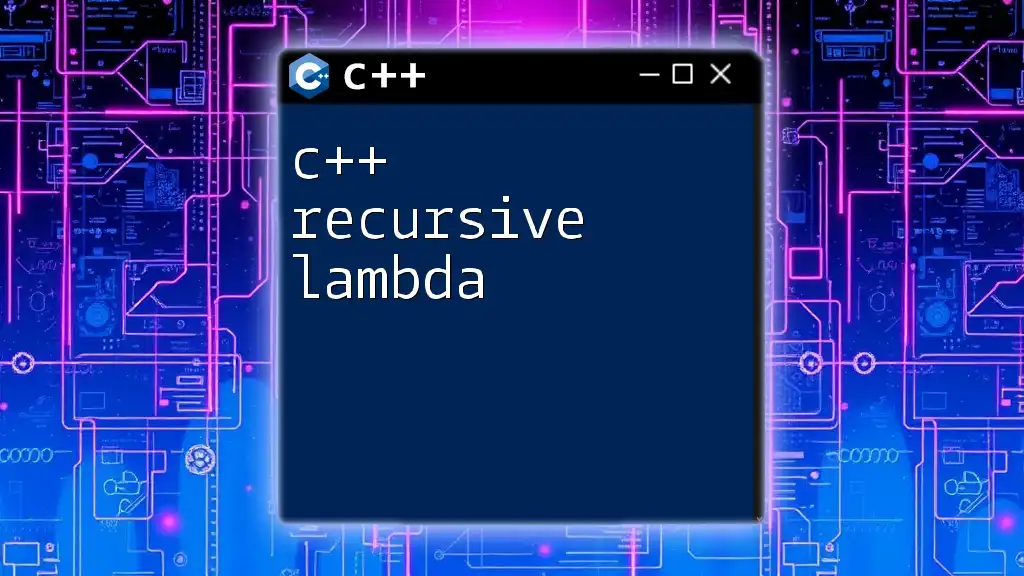
Using C++ Sort with Lambda
Basic Usage of `std::sort`
What is `std::sort`?
`std::sort` is a built-in function provided by the C++ Standard Library, designed to sort elements within a specified range. The function takes two iterator parameters that define the range and an optional comparator to specify the sorting order.
Example of Sorting with a Default Comparator
To demonstrate the basic usage, consider the following code snippet which sorts a vector of integers in ascending order using the default comparator:
#include <algorithm>
#include <vector>
#include <iostream>
int main() {
std::vector<int> numbers = {5, 1, 4, 2, 3};
std::sort(numbers.begin(), numbers.end());
for (int n : numbers) {
std::cout << n << " ";
}
return 0;
}
In this example, `std::sort` automatically arranges `numbers` in ascending order. The default behavior relies on the `<` operator, making it straightforward for simple types like `int`.
Custom Comparators with Lambda
Why Use Custom Comparators?
Custom sorting is crucial when you need to apply unique rules beyond the natural order. A lambda expression can provide flexibility for defining such custom comparators inline without needing to create a separate function.
Example of a Custom Lambda Comparator
Here is how you can sort the same vector of integers in descending order using a lambda expression:
#include <algorithm>
#include <vector>
#include <iostream>
int main() {
std::vector<int> numbers = {5, 1, 4, 2, 3};
std::sort(numbers.begin(), numbers.end(), [](int a, int b) { return a > b; });
for (int n : numbers) {
std::cout << n << " ";
}
return 0;
}
In this example, the lambda expression `[](int a, int b) { return a > b; }` defines the sorting behavior, explicitly ordering the numbers in descending fashion. The use of lambdas keeps the code concise and expressive, allowing for quick adjustments to sorting logic.
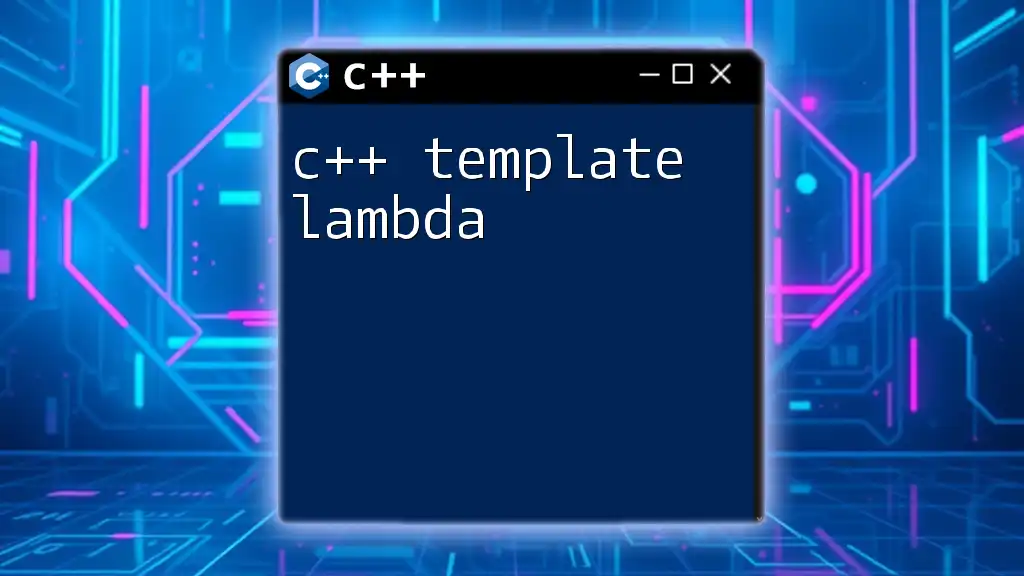
Advanced Lambda Usage with C++ Sort
Capturing Variables in Lambda Expressions
What is Variable Capture?
C++ lambda expressions are powerful not only for their inline definition but also for their ability to capture variables from the surrounding scope. This allows for greater flexibility and customization of the sorting criteria based on external parameters.
Example of Capturing Variables
If we want to sort the numbers based on a threshold value, we can capture that threshold variable with the lambda:
#include <algorithm>
#include <vector>
#include <iostream>
int main() {
int threshold = 3;
std::vector<int> numbers = {5, 1, 4, 2, 3};
std::sort(numbers.begin(), numbers.end(), [threshold](int a, int b) {
return (a > threshold) && (b <= threshold);
});
for (int n : numbers) {
std::cout << n << " ";
}
return 0;
}
In this case, the lambda captures `threshold`. The sorting condition prioritizes elements greater than the threshold before those less than or equal to it, showcasing a practical application of captured variables in custom sorting.
Using Multiple Conditions in Sorting with Lambda
Creating Complex Sort Conditions
In many real-world applications, sorting may require multiple criteria. Lambda expressions allow for such complexity without convoluted code.
Example of Multi-Condition Lambda
Consider sorting a vector of custom objects based on multiple attributes. Below is an example using a `Person` struct, sorting primarily by age and secondarily by name:
#include <algorithm>
#include <vector>
#include <iostream>
struct Person {
std::string name;
int age;
};
int main() {
std::vector<Person> people = {{"Alice", 30}, {"Bob", 25}, {"Charlie", 30}};
std::sort(people.begin(), people.end(), [](const Person &a, const Person &b) {
return (a.age == b.age) ? (a.name < b.name) : (a.age < b.age);
});
for (const auto& person : people) {
std::cout << person.name << " " << person.age << std::endl;
}
return 0;
}
In this example, the lambda checks if two `Person` objects have the same age. If they do, it sorts them by name, otherwise, it sorts them by age. This demonstrates the power and flexibility of lambda expressions for defining custom sorting logic.
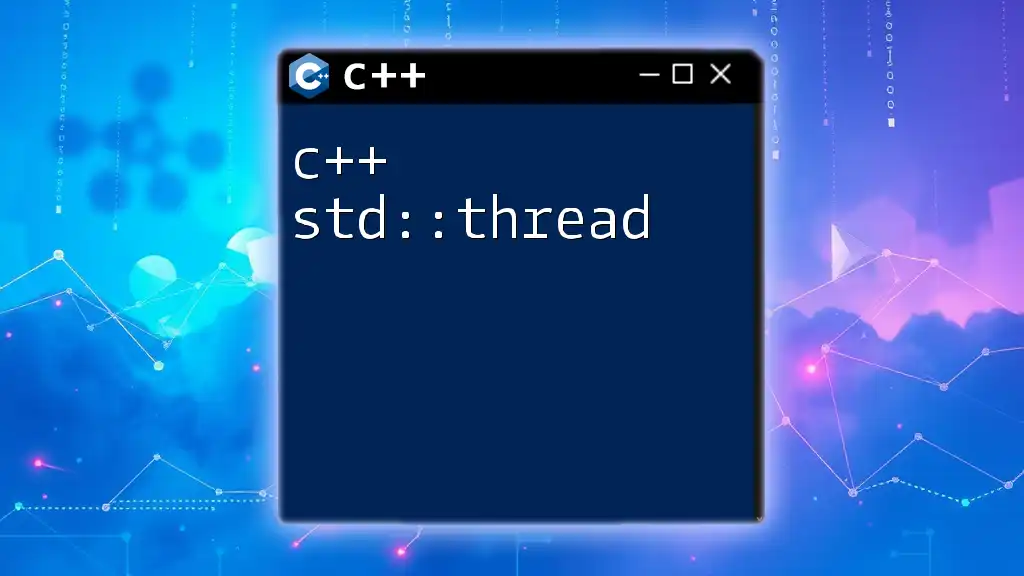
Performance Considerations
Efficiency of Lambda Expressions
One of the notable benefits of using lambda expressions is their efficiency. When using lambdas over traditional function pointers, the compiler can optimize the code better, often inlining the lambda calls directly into the sort algorithm. This can result in performance improvements, especially in complex sorting scenarios.
Memory Usage
Another key consideration is memory usage. When lambda expressions capture variables by value, they may incur additional memory costs. Understanding these implications is essential for optimizing performance in high-demand applications.
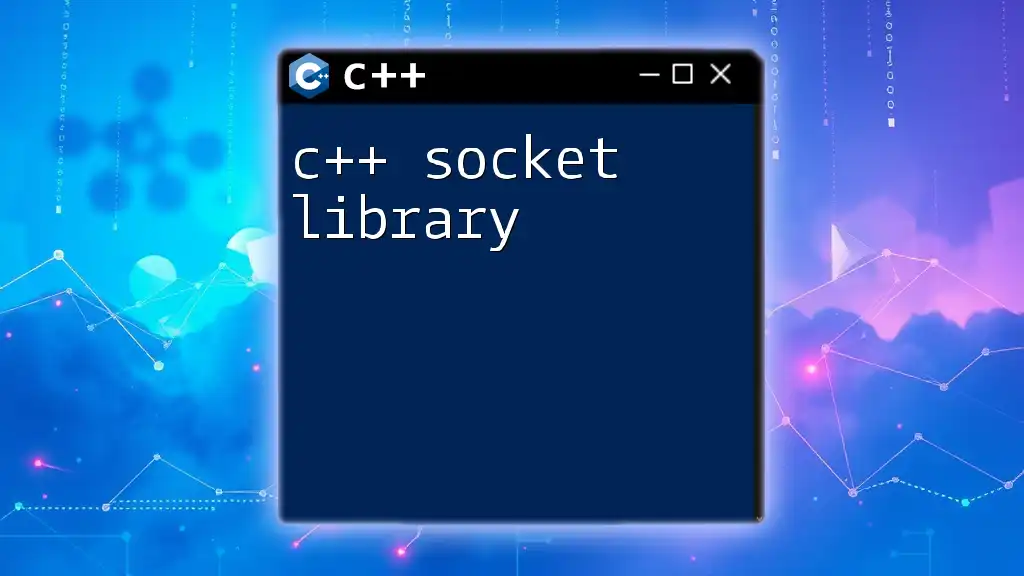
Conclusion
In summary, using C++ sort with lambda expressions provides powerful tools for sorting data efficiently and flexibly. This technique enables developers to create custom sorting rules inline, utilizing the full breadth of C++ capabilities while keeping code concise and organized.
Encouragement to Experiment with Sorting
I encourage you to take these concepts and explore various sorting scenarios in your C++ projects. Whether it's a simple case of ordering integers or more complex conditions defining how data is arranged, mastering `std::sort` with lambdas is a valuable skill that enhances your programming toolkit significantly.
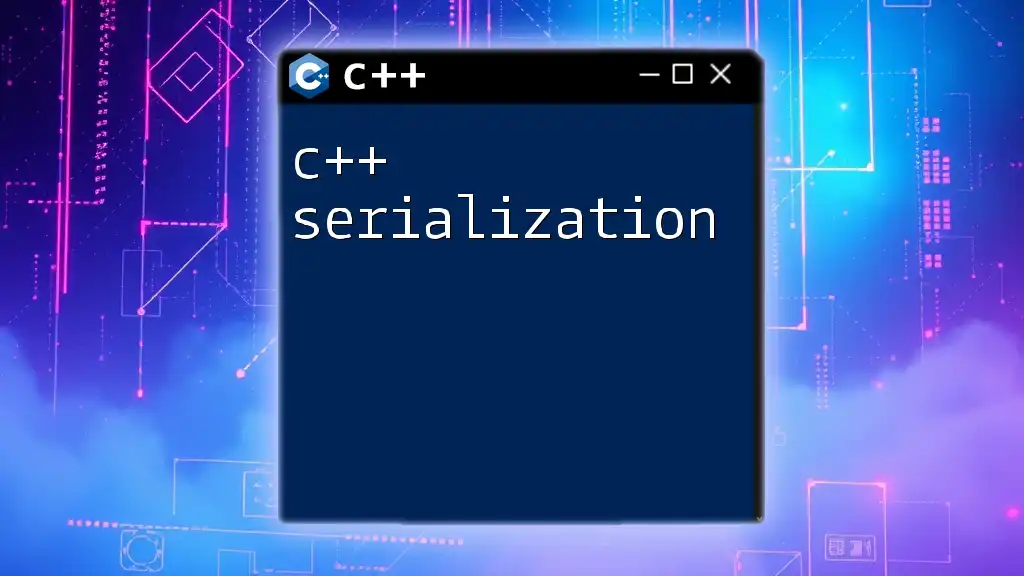
Additional Resources
Recommended Readings
- Consult the C++ reference documentation for `std::sort` to familiarize yourself with additional parameters and use cases.
- Explore additional articles and tutorials focused on lambda expressions in C++, which offer deeper insights into their applications and optimizations.
Tools and Libraries
Consider exploring tools that enhance your understanding of sorting algorithms, such as visualizers and libraries that implement various sorting techniques, thus broadening your capabilities in C++ programming.