A C++ recursive lambda is a self-referential function that can call itself to perform repeated operations, which can be useful for tasks like calculating factorials or traversing data structures.
Here's an example of a recursive lambda in C++ that calculates the factorial of a number:
#include <iostream>
int main() {
auto factorial = [](auto&& self, int n) -> int {
return n <= 1 ? 1 : n * self(self, n - 1);
};
int result = factorial(factorial, 5); // Calculate factorial of 5
std::cout << "Factorial of 5 is: " << result << std::endl;
return 0;
}
Introduction to C++ Recursive Lambdas
What is a lambda function?
A lambda function in C++ is an anonymous function that can be defined inline. They provide a concise way to encapsulate small functions without the need for formal definitions. Comparatively, traditional function definitions require additional lines of code and are less flexible when it comes to capturing variables from the surrounding scope.
Importance of recursion in programming
Recursion is a fundamental concept in programming where a function calls itself to solve a problem. It often simplifies code and makes it easier to understand, particularly for problems that exhibit self-similarity, such as directories or tree structures.
Why use recursive lambdas in C++?
Utilizing recursive lambdas can enhance code clarity and conciseness. They integrate encapsulation with the power of recursion, making them especially useful in situations where you need a temporary function that doesn't require a full definition. For instance, recursive lambdas are particularly helpful in scenarios like calculating factorials or traversing complex data structures.
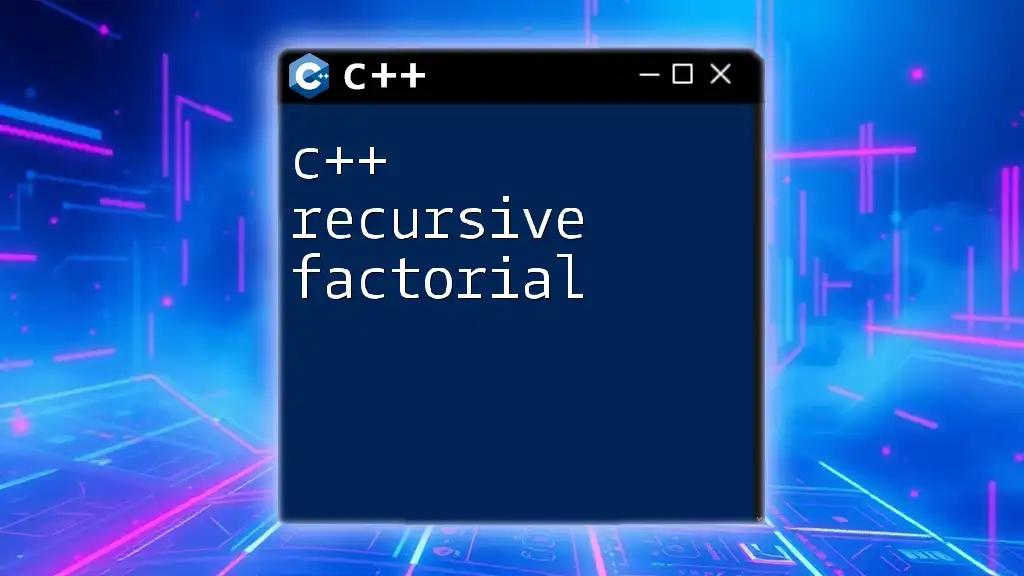
Understanding the Basics of Recursion
Definition of recursion
Recursion involves a function calling itself with a subset of the original problem until it reaches a base case that halts further calls. The base case serves as a stopping condition, while the recursive case handles the logic required to break the problem down.
Common pitfalls in recursion
While recursion is powerful, it can lead to problems such as stack overflow if not handled properly. This often occurs when the base case is not defined correctly, resulting in an infinite loop of function calls. Therefore, it is crucial to ensure that recursive calls eventually hit a base case.

Crafting Recursive Lambdas in C++
Syntax of lambda expressions
Lambda functions have a specific syntax in C++:
[ captures ] ( parameters ) -> return_type { body }
- Captures: This allows the lambda to use variables from its surrounding scope.
- Parameters: Similar to function parameters, lambdas can accept input.
- Return type: You may explicitly specify it, although it can often be inferred.
- Body: Contains the code that the lambda will execute.
Here’s an example of a simple lambda that adds two numbers:
auto add = [](int a, int b) -> int {
return a + b;
};
Understanding the capture clause
The capture clause is essential in lambdas because it defines how external variables are used. You can capture variables by reference (`&`) or by value (default). Using the correct capture method is vital to avoid unintended side effects, especially in concurrent or recursive contexts.
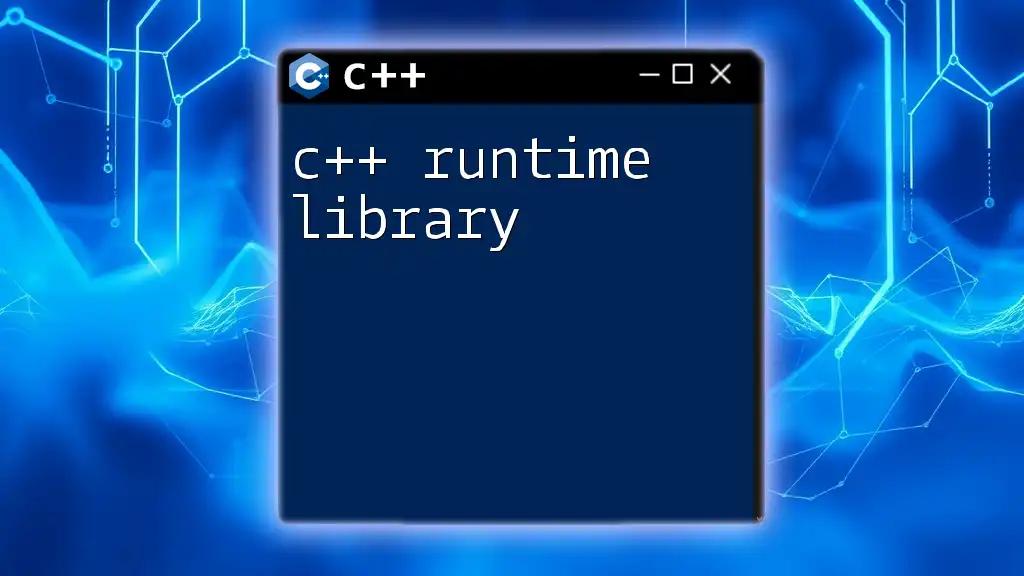
Implementing Recursive Lambdas
Recursive lambdas explained
To create a recursive lambda in C++, a common approach is to define the lambda inside a `std::function`. This allows it to call itself. Here’s an example of implementing a recursive lambda to calculate the factorial of a number:
#include <iostream>
#include <functional>
int main() {
std::function<int(int)> factorial = [](int n) -> int {
return (n <= 1) ? 1 : n * factorial(n - 1);
};
std::cout << "Factorial of 5: " << factorial(5) << std::endl; // Output: 120
return 0;
}
Example of a recursive lambda
The example above demonstrates how recursive lambdas can be elegantly defined, making them both concise and powerful. In this case, the lambda calculates the factorial of a number by calling itself recursively until it reaches the base case.
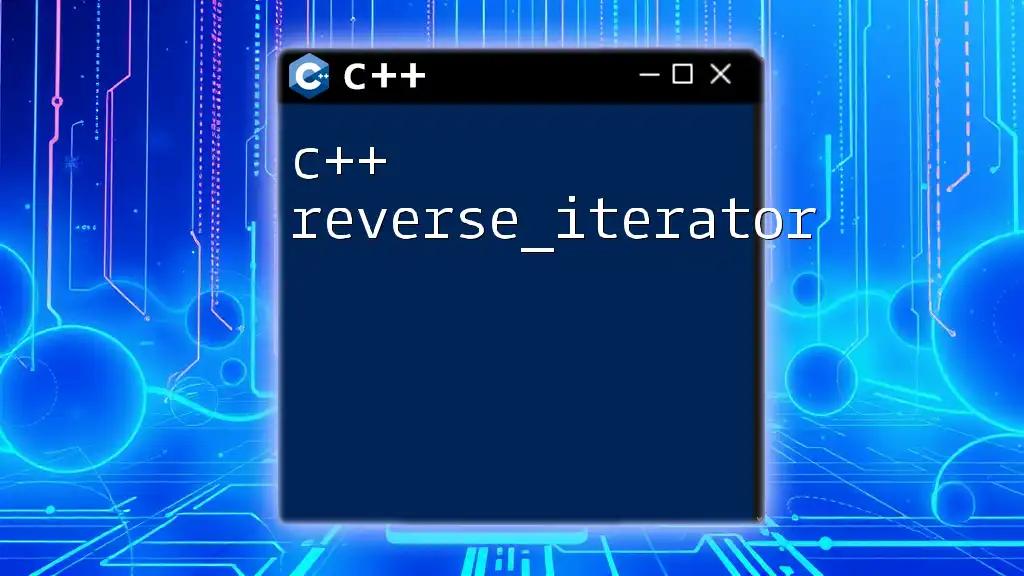
Advanced Use Cases for Recursive Lambdas
Calculating Fibonacci numbers
The Fibonacci sequence is another classic use case for recursion. In this context, each number is the sum of the two preceding ones. Below is an implementation of a Fibonacci calculation using a recursive lambda:
#include <iostream>
#include <functional>
int main() {
std::function<int(int)> fibonacci = [](int n) -> int {
return (n <= 1) ? n : fibonacci(n - 1) + fibonacci(n - 2);
};
std::cout << "Fibonacci of 6: " << fibonacci(6) << std::endl; // Output: 8
return 0;
}
Tree traversal using recursive lambdas
Recursive lambdas can also be employed to traverse tree structures, such as binary trees. By defining a recursive lambda that processes a node and its children, you can handle complex data structures fluidly.
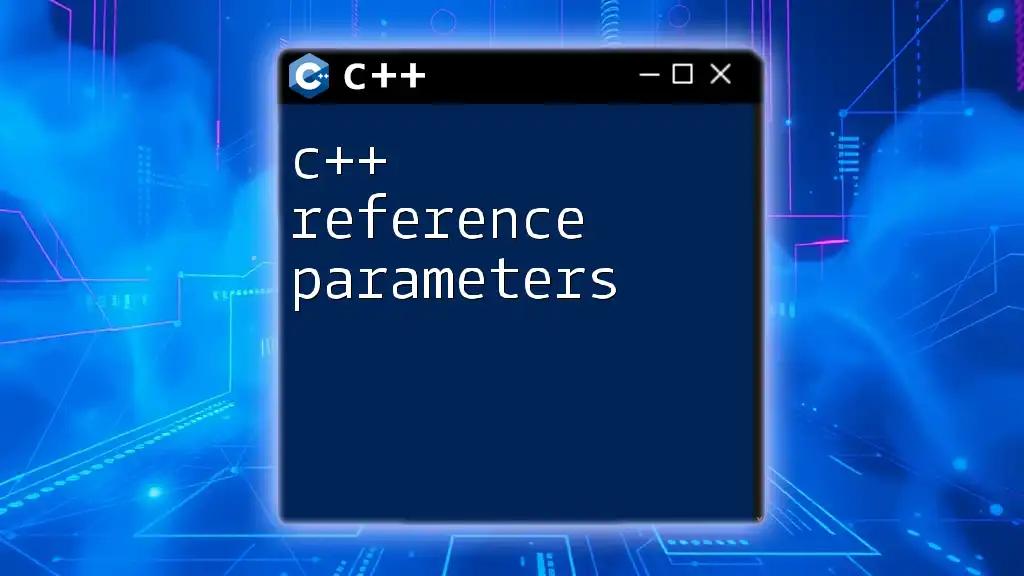
Performance Considerations
Efficiency of recursive lambdas
Analyzing the time complexity of recursive algorithms is crucial. For instance, the naive implementation of the Fibonacci calculation mentioned earlier has exponential time complexity, predominantly due to the overlapping subproblems. Considering iterative solutions or caching results (memoization) may enhance performance in practical applications.
Inlining and compiler optimizations
Modern C++ compilers can perform various optimizations on lambda functions. While `std::function` provides flexibility, it has overhead. For the best performance with recursive lambdas, consider directly using the lambda without encasing it in `std::function`, although it makes self-referencing trickier.
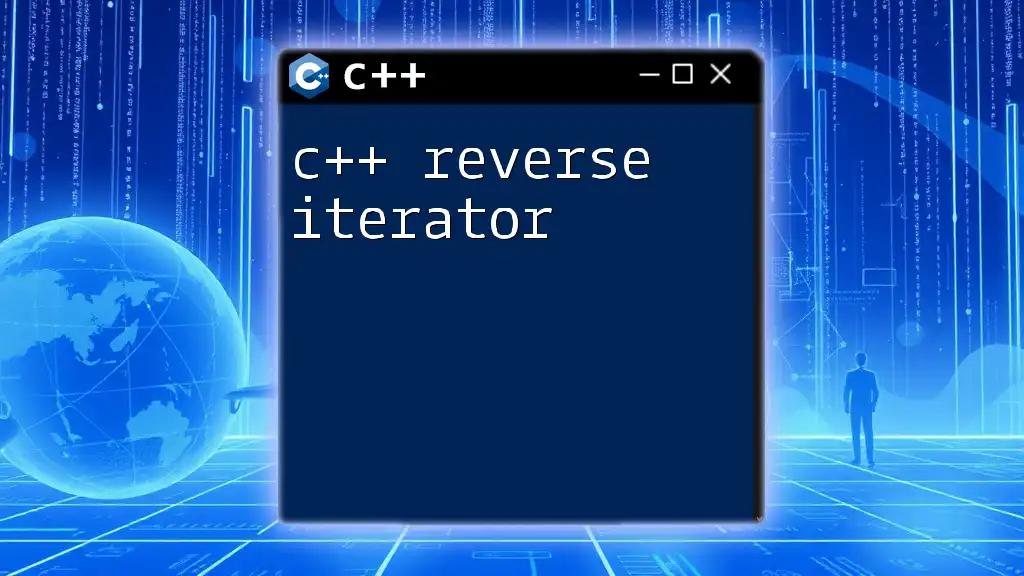
Error Handling with Recursive Lambdas
Common errors and debugging techniques
When working with recursive lambdas, one common error is hitting a stack overflow due to improper base cases. Ensure thorough testing of all paths in the recursive function to catch edge cases early. Utilizing tools like debuggers and logging can also aid in identifying the recursive calling patterns.
Using exceptions effectively in recursive lambdas
Implementing exception handling in recursive lambdas requires careful consideration. Here's an example of handling exceptions for invalid input within a recursive factorial lambda:
#include <iostream>
#include <functional>
#include <stdexcept>
int main() {
std::function<int(int)> factorial = [](int n) -> int {
if(n < 0) {
throw std::invalid_argument("Negative input not allowed");
}
return (n <= 1) ? 1 : n * factorial(n - 1);
};
try {
std::cout << "Factorial of -5: " << factorial(-5) << std::endl;
} catch (const std::invalid_argument& e) {
std::cout << "Error: " << e.what() << std::endl;
}
return 0;
}
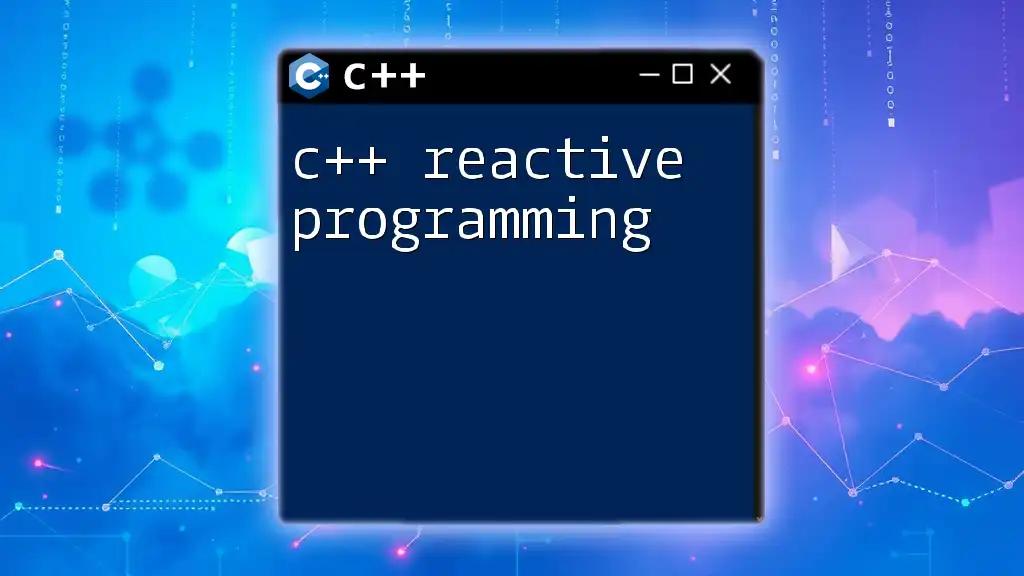
Conclusion
Recap of key concepts
C++ recursive lambdas combine the power of recursion with the expressive capabilities of lambdas, enabling developers to write concise and readable code. Understanding both recursion and lambda syntax is fundamental to leveraging their full potential.
Resources for further learning
To deepen your understanding of C++ and recursive lambdas, consider exploring comprehensive resources such as advanced C++ programming books, online tutorials, or engaging with the C++ community through forums and discussion groups.

Call to Action
Practice coding recursive lambdas to enhance your programming skills. Begin with exercises such as calculating factorials or Fibonacci numbers, and then challenge yourself to traverse more complex data structures. Don't hesitate to share your experiences, challenges, and questions as you embark on this exciting exploration of C++ recursive lambdas!