In C++, you can return an array from a function by using a pointer, but it’s typically more effective to return a `std::vector` for better memory management and safety; here's an example using a pointer approach:
#include <iostream>
int* returnArray(int size) {
int* arr = new int[size]; // dynamically allocate array
for (int i = 0; i < size; i++) {
arr[i] = i * 10; // filling the array with values
}
return arr; // return pointer to the allocated array
}
int main() {
int size = 5;
int* myArray = returnArray(size);
for (int i = 0; i < size; i++) {
std::cout << myArray[i] << " "; // print elements
}
delete[] myArray; // free allocated memory
return 0;
}
Understanding Arrays in C++
What is an Array?
An array is a collection of elements that are stored in contiguous memory locations. These elements are typically of the same data type, allowing for efficient indexing. Arrays can be used to store data sets such as lists or tables and are essential for various algorithms and data processing tasks in C++.
Why Return an Array?
Returning an array from a function allows you to output multiple values without using global variables or requiring multiple return statements. This technique promotes code modularity and improves data handling. When dealing with complex data, returning arrays enables you to encapsulate logic and simplifies function calls in your code.
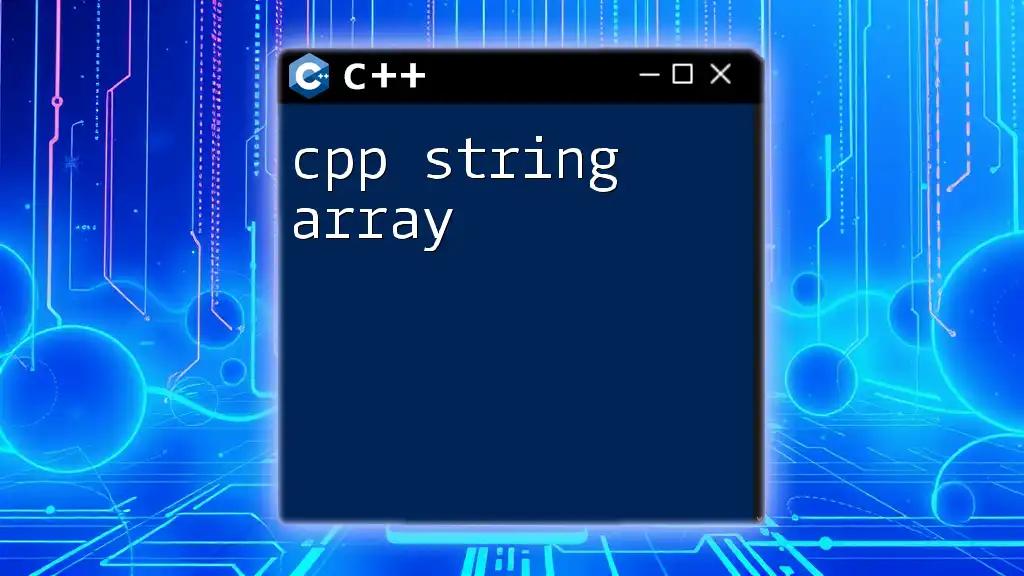
The Basics of Returning Arrays
How to Return an Array in C++
Returning an array in C++ requires an understanding of how memory is managed in the language. Directly returning an array from a function is not feasible due to how arrays are treated within the function scope. Instead, you generally return a pointer to the first element of the array.
Options for Returning Arrays
When it comes to returning arrays from functions, you have two primary approaches: returning pointers or encapsulating the array within a data structure like `std::array` or `std::vector`.
Returning a pointer allows for dynamic memory allocation but places the burden of memory management on the programmer. Alternatively, using standard libraries like `std::vector` simplifies memory management and extends the capabilities of arrays.

C++ Function That Returns an Array
Syntax of a Function Returning an Array
The syntax for a function that returns an array typically involves using a pointer type as the return type. This informs the compiler that the function will not return the array itself, but a reference to it.
returnType* functionName(parameters) {
// Function body
}
Example: A Simple Function Returning an Integer Array
Here's a straightforward example, where we create a function that generates and returns an integer array:
int* createArray(int size) {
int* arr = new int[size]; // Dynamically allocate memory for the array
// Fill the array with values
for (int i = 0; i < size; i++) {
arr[i] = i;
}
return arr; // Return a pointer to the first element
}
In this example, we allocate the memory for `arr` using `new`, which ensures that this array will persist outside the function scope. It's crucial to release the memory later using `delete[]` to prevent memory leaks.
Explanation of the Example
The `createArray` function uses dynamic memory allocation to handle arrays, thus allowing them to be created with a size determined at runtime. After using the array, remember to free the allocated memory like so:
delete[] arr; // Correctly freeing dynamic memory

C++ Array Return Techniques
Returning Arrays Via Pointers
When using pointers, you can manipulate the array elements directly by passing a pointer to the function. Here’s another example:
void fillArray(int* arr, int size) {
for (int i = 0; i < size; i++) {
arr[i] = i * 10; // Fill the array with multiples of 10
}
}
In this function, `arr` points to the first element of the original array passed from wherever `fillArray` is called. This allows direct modification of the contents of the original array based on the pointer.
Returning Arrays by Value Using std::array or std::vector
For modern C++ development, utilizing `std::array` or `std::vector` can greatly enhance the safety and usability of array handling. Using `std::vector` takes advantage of dynamic size limits and built-in memory management as shown below:
#include <vector>
std::vector<int> createVector(int size) {
std::vector<int> vec; // Initialize an empty vector
for (int i = 0; i < size; i++) {
vec.push_back(i); // Add values to the vector
}
return vec; // Return the vector by value
}
With `std::vector`, you no longer have to manage memory manually, as the vector class handles it for you. This feature makes your code cleaner and more maintainable.
Explanation of Using `std::vector`
Using `std::vector` provides several advantages, such as automatic resizing, built-in bounds checking, and reduced risk of memory leaks. By abstracting memory management, you can focus on other parts of your program and reduce potential errors.
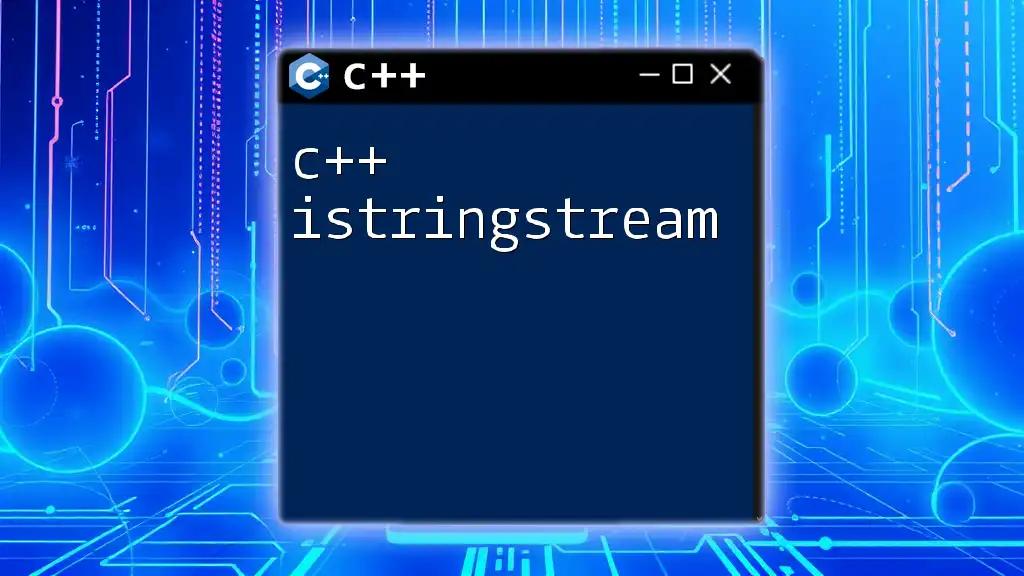
Function Return Array in C++ Best Practices
Managing Memory Efficiently
When returning arrays, particularly those created with `new`, memory management becomes paramount. If you allocate memory manually, always ensure to free that memory after its use. Utilize smart pointers (like `std::unique_ptr`) to automate memory management and reduce the risk of memory leaks.
Ensuring Safety and Avoiding Memory Leaks
Common pitfalls include:
- Dangling pointers: After freeing memory, any references to this memory become invalid.
- Memory leaks: Not freeing allocated memory leads to wastage and potential program failure.
To mitigate these issues, keep your array's lifetime clearly defined, ensure that you always deallocate memory when it's no longer needed, and prefer using safer alternatives like `std::vector`.
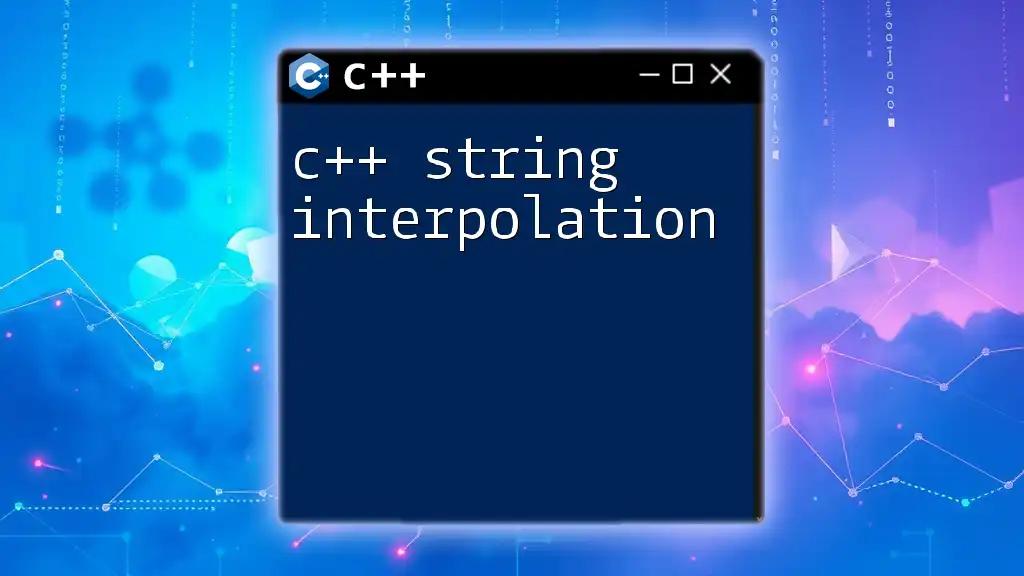
Conclusion
In this guide on C++ returning an array, we explored various methods and best practices for handling arrays in function returns. Understanding how to return arrays safely and effectively is crucial for efficient programming in C++. As you continue to learn, experimenting with these techniques will serve you well in mastering C++. Join our community for more insights on coding in C++ and enhancing your programming skills!
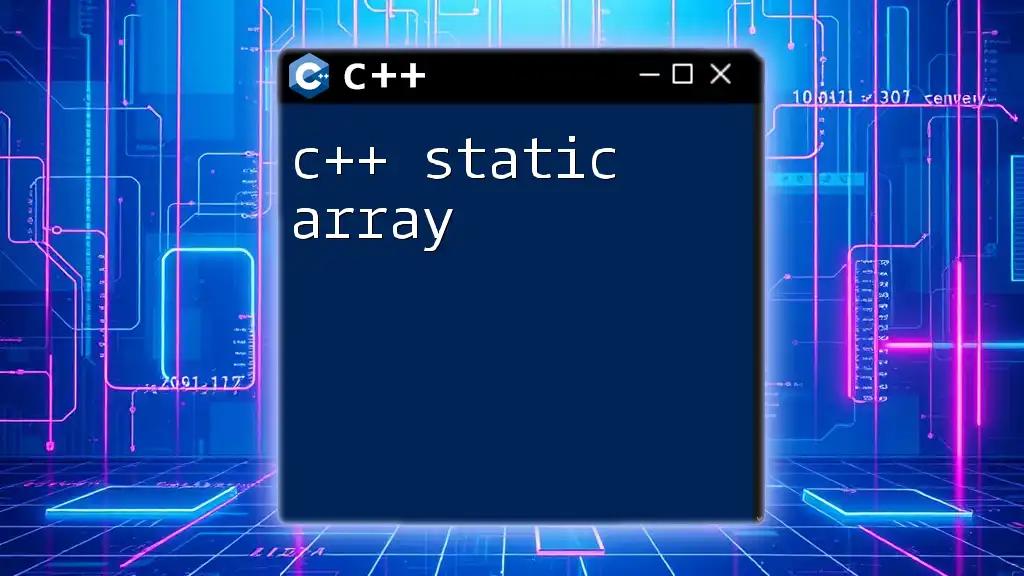
Additional Resources
Recommended Reading
For further learning, you might consider reading more about:
- C++ data structures and algorithms
- Memory management in C++
- Advanced C++ programming techniques
FAQs about Returning Arrays in C++
Common questions frequently arise about returning arrays:
- Can you return a local array from a function? No, because it goes out of scope when the function exits.
- How do I prevent memory leaks? Always use `delete[]` on dynamically allocated arrays and consider using smart pointers.
By understanding these principles, you will make significant strides toward becoming a proficient C++ programmer.