In C++, returning `nullptr` from a function indicates that the function does not have a valid object to return, which is commonly used for pointer types.
Here’s an example of a C++ function that demonstrates returning `nullptr`:
#include <iostream>
int* findElement(int* arr, int size, int target) {
for (int i = 0; i < size; i++) {
if (arr[i] == target) {
return &arr[i]; // Return the address of the found element
}
}
return nullptr; // Return null if the target is not found
}
int main() {
int arr[] = {1, 2, 3, 4, 5};
int* result = findElement(arr, 5, 6);
if (result == nullptr) {
std::cout << "Element not found." << std::endl;
} else {
std::cout << "Element found: " << *result << std::endl;
}
return 0;
}
What Does 'Return Null' Mean in C++?
Defining Null in C++
In C++, the term "null" refers to a pointer that does not point to any valid memory location. Since C++11, the preferred way to represent a null pointer is using `nullptr`, which provides better type safety compared to the older `NULL` macro or the integer literal `0`. This distinction is crucial because using `nullptr` helps prevent ambiguous interpretations that might occur with `0`, particularly in overloaded functions.
Here's an example of defining a null pointer:
int* ptr = nullptr; // ptr is now a null pointer
The pointer `ptr` does not currently point to any valid memory location or object, clearly signifying its state as "null".
When to Use Null Returns
Returning null from a function is particularly useful in specific situations, as it serves as a clear indication of failure or the absence of a value:
- Indicating Failure: Functions that might not succeed should return nullptr to inform the caller of the failure.
- Non-existent Objects: When searching for an object (e.g., in a list or array) that might not exist.
Consider the following example for a function that searches for a node in a linked list:
Node* findNode(int value) {
if (/* node not found */) {
return nullptr; // indicate failure
}
return foundNode;
}
If `findNode` fails to locate the specified node, it returns `nullptr`, allowing the caller to know that no valid object is returned.
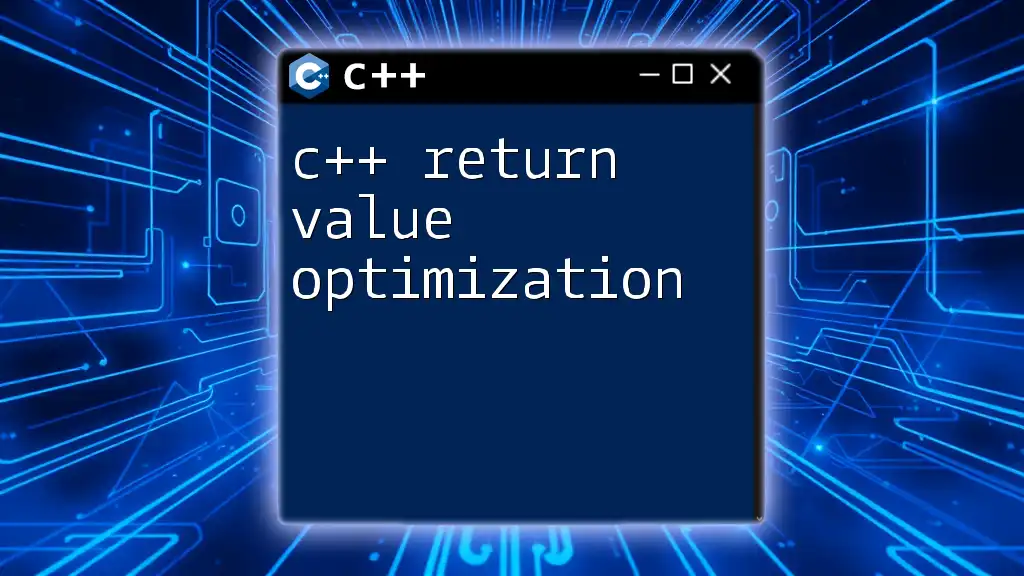
The Syntax of Returning Null
Returning null in C++ is straightforward. You simply use `nullptr` as the return value in your function. This can be applied in a variety of contexts, particularly when you're dealing with pointers.
Here is a basic syntax overview:
std::string* getString(bool returnNull) {
if (returnNull) {
return nullptr; // return a null string pointer
}
return new std::string("Hello, world!");
}
In this example, `getString` returns a `nullptr` when the input parameter `returnNull` is true. Otherwise, it creates and returns a new string. This demonstrates how `nullptr` can signify that no valid object exists.
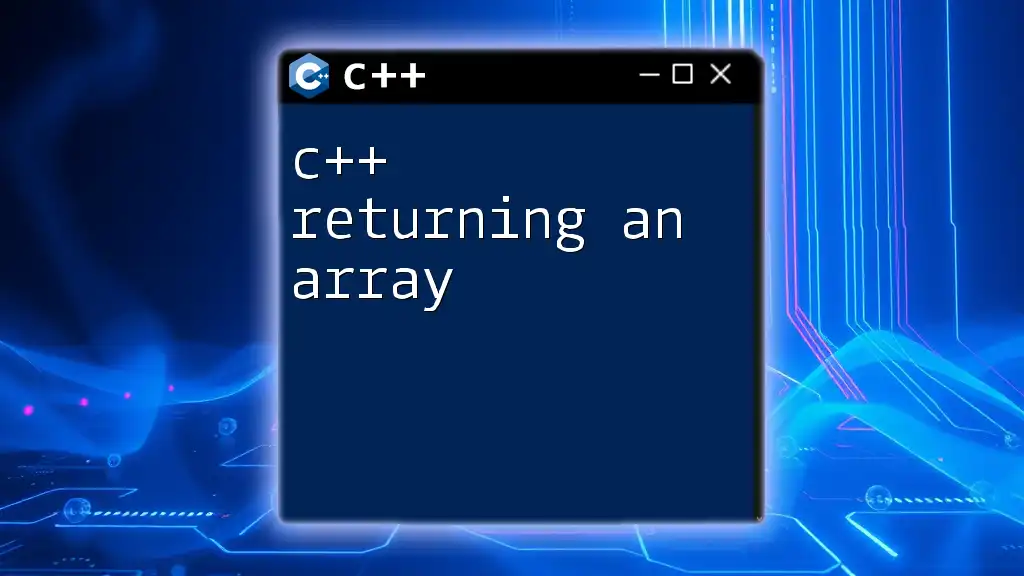
Common Mistakes When Returning Null
Forgetting to Handle Null Returns
One pitfall to avoid is neglecting to check whether a returned pointer is null before accessing its members. Failing to handle null returns can lead to dereferencing a null pointer, which results in undefined behavior or runtime crashes.
For instance, consider this erroneous function:
void processNode(Node* node) {
// Dangerous: assumes node is not null without a check
std::cout << node->value;
}
In the above snippet, if `node` is `nullptr`, dereferencing it will lead to a crash. Always ensure to check for null values before attempting to access members:
void processNode(Node* node) {
if (node != nullptr) {
std::cout << node->value;
} else {
std::cout << "Node is null!";
}
}
Returning Null from Void Functions
It's important to remember that void functions cannot return values, including null. A void function signifies that it doesn't return anything to the caller. Trying to return `nullptr` from such functions will result in a compilation error.
Here's an example that highlights this concept:
void printMessage(bool returnNull) {
if (returnNull) {
return; // No value to return; just exit
}
std::cout << "Hello, World!";
}
In this function, when `returnNull` is true, the function simply exits without returning any value, underscoring that returning null from a void function is inappropriate.
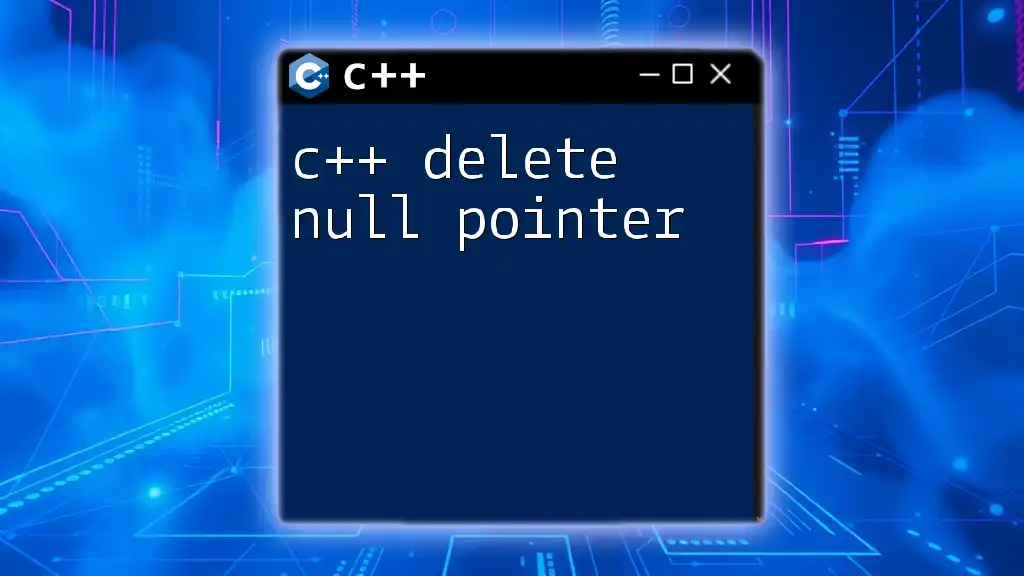
Best Practices for Using Null Returns
Always Document Function Behavior
When designing functions that can return null values, it is crucial to document this behavior. This documentation acts as a guide for users of the function, clarifying when they can expect a null return.
Here’s how you can document a function with Doxygen-style comments:
/**
* Find a node in a linked list.
* @param value The value to search for.
* @return A pointer to the node, or nullptr if not found.
*/
Node* findNode(int value);
By doing so, you clearly inform developers that they must handle the potential for a null return, fostering deeper understanding and more robust code.
Returning Smart Pointers Instead of Raw Pointers
Consider using smart pointers, such as `std::unique_ptr` and `std::shared_ptr`, instead of raw pointers. Smart pointers help manage memory automatically, minimizing the risk of memory leaks and dangling pointers.
Using smart pointers not only helps in resource management but also conveys the concept of ownership more clearly in your code. Here's an example showcasing the use of `std::unique_ptr`:
std::unique_ptr<Node> createNode() {
return std::make_unique<Node>();
}
In this case, `createNode` returns a smart pointer, which automatically manages its memory. This approach negates the need for manually returning null pointers since smart pointers will inherently handle object lifetimes and distinguish valid pointers from null pointers effectively.
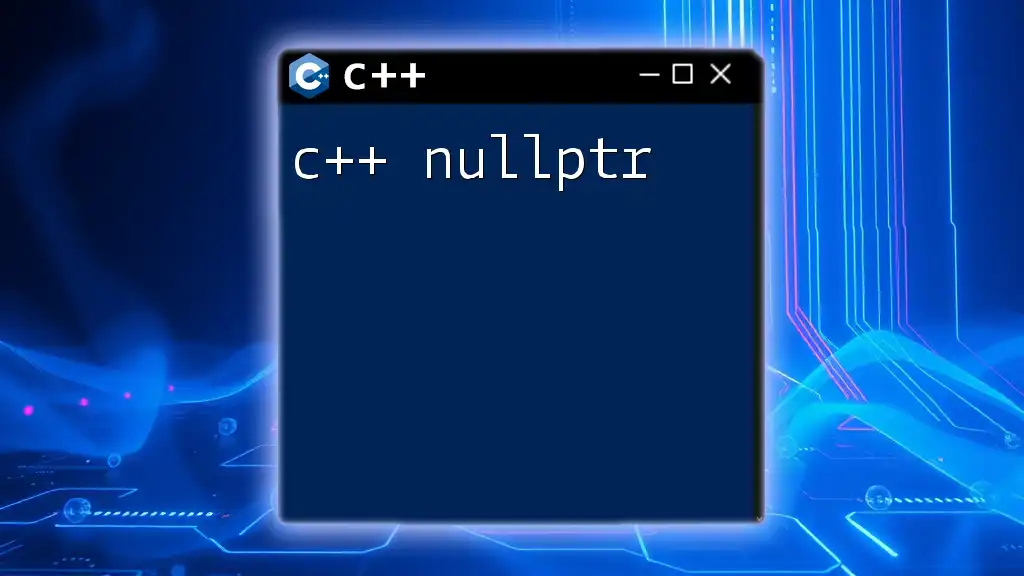
Real-World Applications of Returning Null
Dealing with Databases
When interacting with databases, functions that attempt to retrieve data might return null if the queried data does not exist. Consider the following scenario:
User* findUserById(int id) {
User* user = queryDatabase(id); // Hypothetical database query function
if (user == nullptr) {
// User with the given id does not exist
return nullptr; // Indicate not found
}
return user;
}
Here, `findUserById` returns a null pointer if no user is found, allowing the calling function to handle this case gracefully.
File Handling Scenarios
File operations also often deal with null returns. For instance, consider a function that attempts to open a file:
File* openFile(const std::string& filename) {
if (!fileExists(filename)) {
return nullptr; // File does not exist
}
return new File(filename);
}
In this example, `openFile` returns `nullptr` if the specified file does not exist, making it clear to the caller that they must handle the case of a non-existent file.
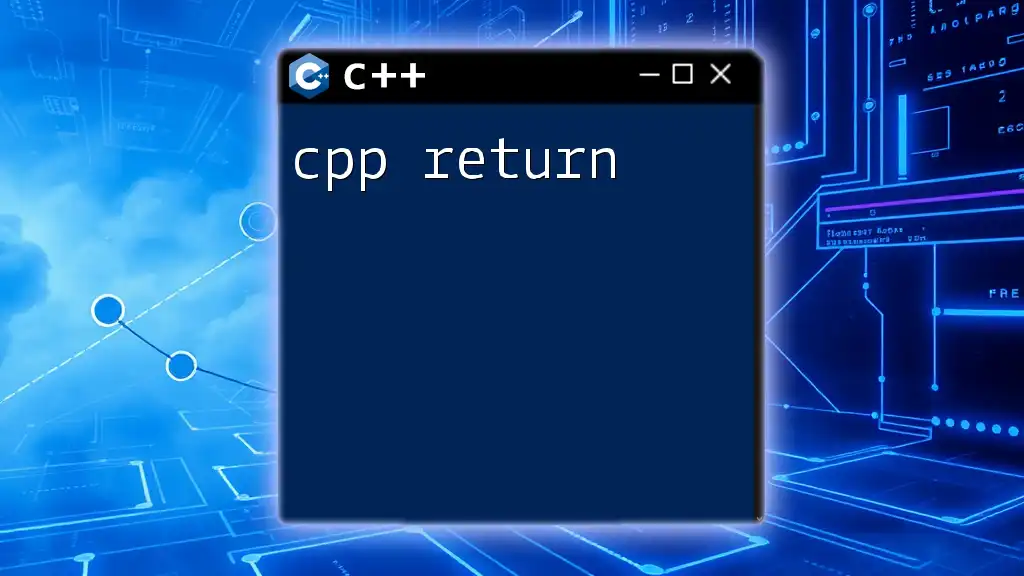
Conclusion
Understanding how to effectively utilize c++ return null is vital for creating robust applications. Proper handling of null return values not only prevents runtime errors but also enhances code readability and maintainability. Following the best practices highlighted in this article will help ensure that your C++ code is both safe and efficient.
Taking the time to implement these principles will equip you with a stronger grasp of managing pointers in your C++ applications and preparing you for more complex programming challenges.
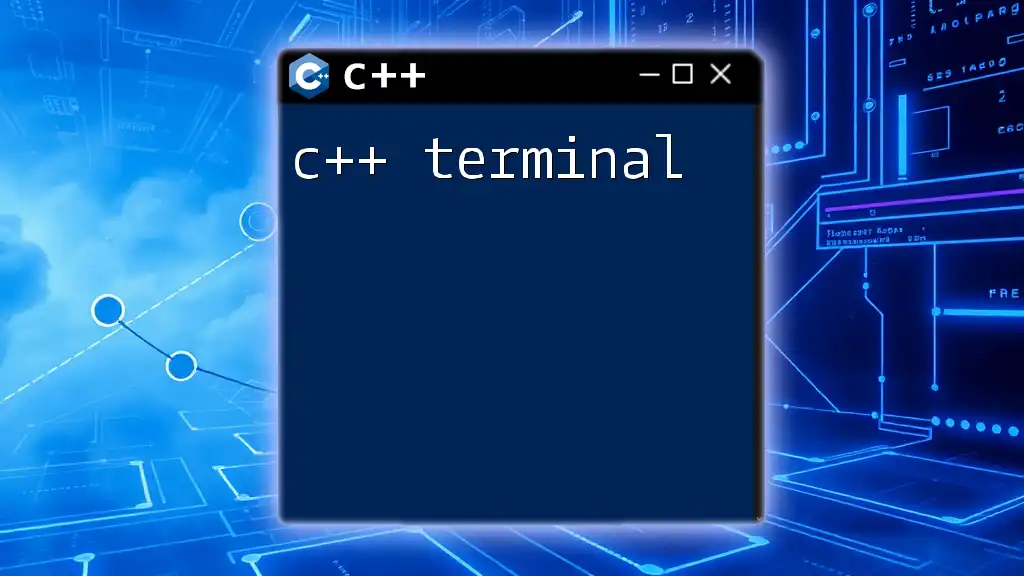
Further Reading
To delve deeper into C++ and related topics, consider exploring materials on C++ pointers, memory management, and best programming practices. These resources will enrich your understanding and enhance your coding prowess.
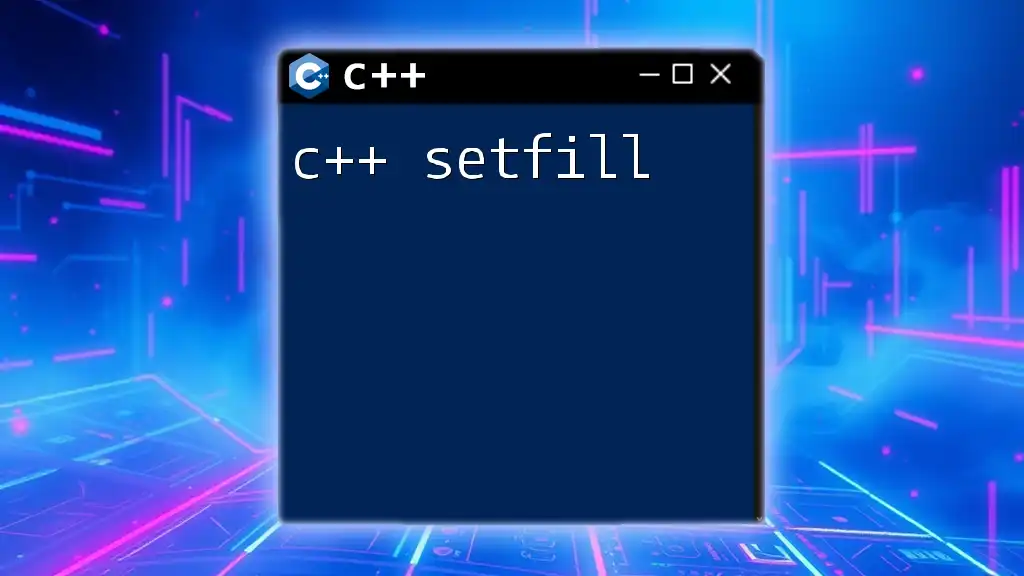
Call to Action
Ready to take your C++ skills to the next level? Explore more tutorials on C++ commands and don’t hesitate to share your experiences or questions in the comments!