In C++, the `<limits>` header provides a standardized way to define the characteristics of fundamental data types, such as their minimum and maximum values.
Here’s a code snippet demonstrating how to use it:
#include <iostream>
#include <limits>
int main() {
std::cout << "The maximum int value is: " << std::numeric_limits<int>::max() << std::endl;
std::cout << "The minimum int value is: " << std::numeric_limits<int>::min() << std::endl;
return 0;
}
Understanding C++ Limits
What Are C++ Limits?
C++ limits define the minimum and maximum values that can be held by various data types within the language. Understanding these limits is crucial for any developer, as it helps to ensure that programs run smoothly without unexpected behavior such as overflow or underflow. Different data types in C++, such as integers and floating-point numbers, have their own specific limits that must be considered during development.
Why C++ Limits Matter
Understanding C++ limits is essential for preventing common programming errors. When a variable exceeds its defined limit, it can lead to overflow (exceeding the maximum limit) or underflow (going below the minimum limit). Such issues can result in unpredictable behavior, incorrect results, or even program crashes. A firm grasp of limits enables developers to write more robust code and anticipate potential pitfalls.
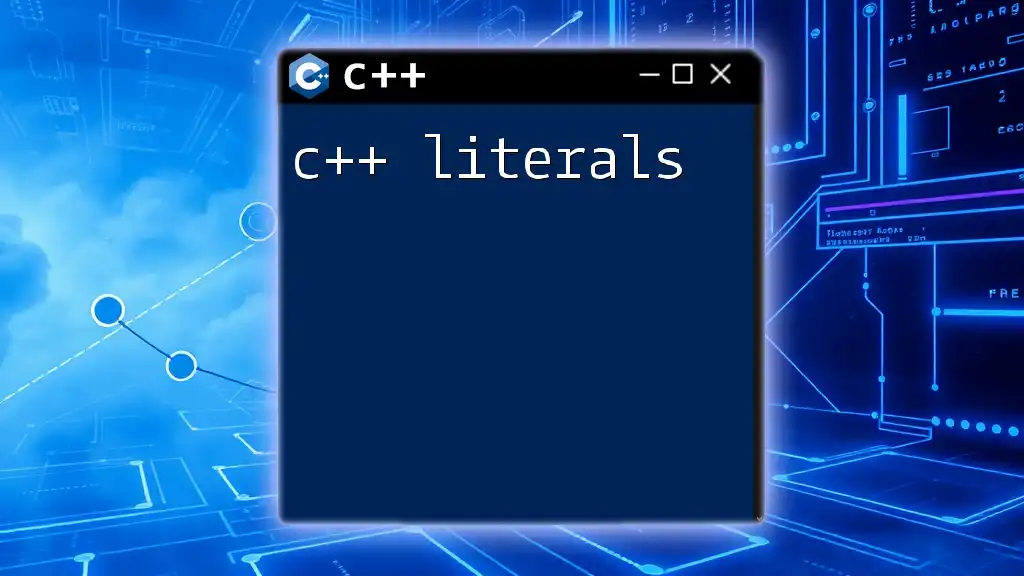
Data Type Limits in C++
Integral Types
Char Limits
The `char` data type typically represents a single byte of character data. Its limits can vary between signed and unsigned versions. In C++, the maximum value of a signed `char` is typically 127, while an unsigned `char` can reach 255. You can access these values using the `<climits>` library.
Example:
#include <iostream>
#include <climits>
int main() {
std::cout << "Char max: " << CHAR_MAX << std::endl;
std::cout << "Char min: " << CHAR_MIN << std::endl;
return 0;
}
Short Int Limits
The `short` data type is designed to hold small integers. Like `char`, it can be signed or unsigned. The typical range for a signed `short` is from -32,768 to 32,767.
Example:
#include <iostream>
#include <climits>
int main() {
std::cout << "Short max: " << SHRT_MAX << std::endl;
std::cout << "Short min: " << SHRT_MIN << std::endl;
return 0;
}
Int Limits
The `int` data type is one of the most commonly used in programming. The range for a signed integer usually spans from -2,147,483,648 to 2,147,483,647. This makes it a powerful choice for counting and indexing purposes.
Example:
#include <iostream>
#include <climits>
int main() {
std::cout << "Int max: " << INT_MAX << std::endl;
std::cout << "Int min: " << INT_MIN << std::endl;
return 0;
}
Long and Long Long Limits
For larger integers, `long` and `long long` types come into play. The `long` data type often has a range from -2,147,483,648 to 2,147,483,647 on most systems, while `long long` extends this range even further, usually spanning -9,223,372,036,854,775,808 to 9,223,372,036,854,775,807.
Example:
#include <iostream>
#include <climits>
int main() {
std::cout << "Long max: " << LONG_MAX << std::endl;
std::cout << "Long long max: " << LLONG_MAX << std::endl;
return 0;
}
Floating-Point Limits
Floating-point types allow you to represent real numbers, complete with decimal points. These types—`float`, `double`, and `long double`—vary in terms of precision and the range of values they can handle.
Float, Double, and Long Double Limits
The `float` type usually provides about 7 digits of precision, while `double` offers around 15 digits. The `long double` type extends this further, with precision depending on the system's architecture.
Example:
#include <iostream>
#include <cfloat>
int main() {
std::cout << "Float max: " << FLT_MAX << std::endl;
std::cout << "Double max: " << DBL_MAX << std::endl;
return 0;
}
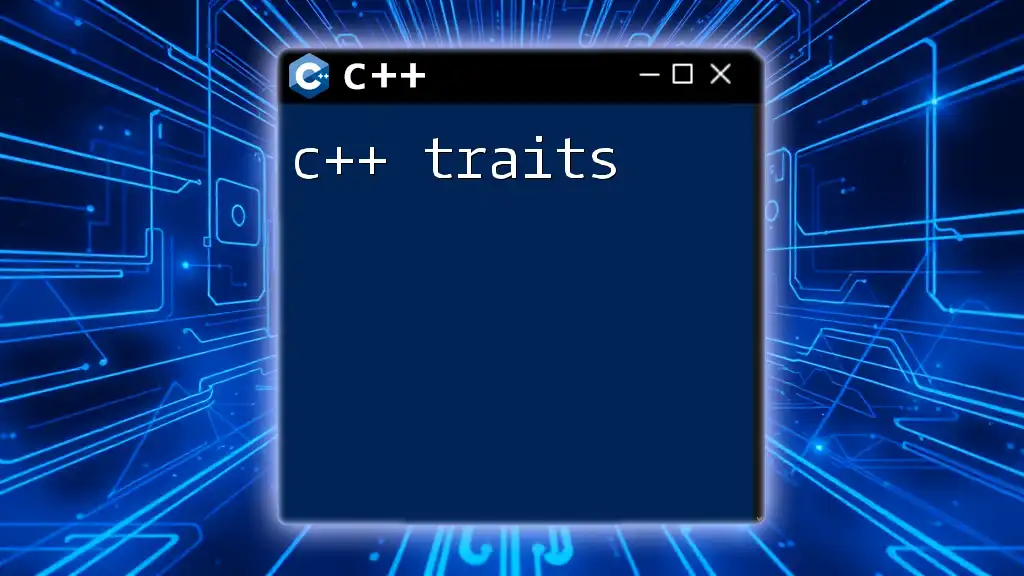
Handling Limits in C++
Detecting Overflow and Underflow
The impact of exceeding limits can be severe; therefore, it's essential to implement checks in your code. The `<limits>` header file provides constants that enable you to manage limits effectively.
For instance, checking if a value exceeds the `INT_MAX` limit can be done like this:
#include <iostream>
#include <limits>
int main() {
int value = INT_MAX + 1; // Simulate possible overflow
if (value > INT_MAX) {
std::cout << "Overflow detected!" << std::endl;
}
return 0;
}
Best Practices for Managing Limits
To prevent overflow or underflow, consider the following best practices:
- Input validation: Always validate user input before processing it to ensure it falls within acceptable limits.
- Using assertions: Employ assertions to catch errors during development.
- Code example:
assert(value <= INT_MAX); // This will trigger if the condition is false
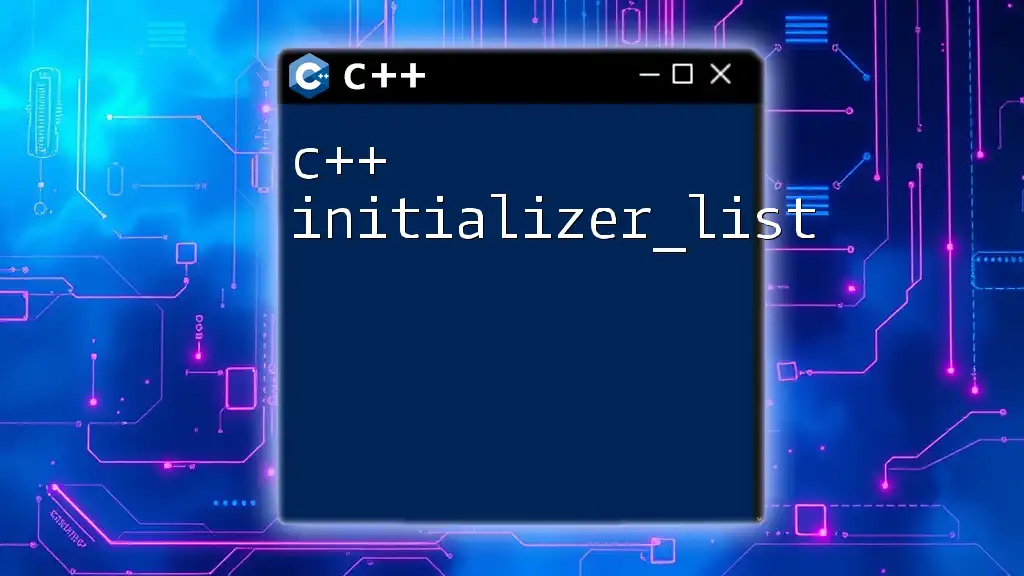
Beyond Basic Limits
Custom Limits in Classes
In addition to built-in data types, you can create custom classes with specific limits on their attributes. For example, consider a custom integer class that only allows values within the range of normal integers.
Example:
#include <cassert>
#include <iostream>
#include <limits>
class MyInt {
int value;
public:
void setValue(int val) {
assert(val <= INT_MAX);
value = val;
}
int getValue() const {
return value;
}
};
int main() {
MyInt myInt;
myInt.setValue(1000); // Valid
// myInt.setValue(INT_MAX + 1); // Uncommenting this line will trigger assertion failure
std::cout << "Value: " << myInt.getValue() << std::endl;
return 0;
}
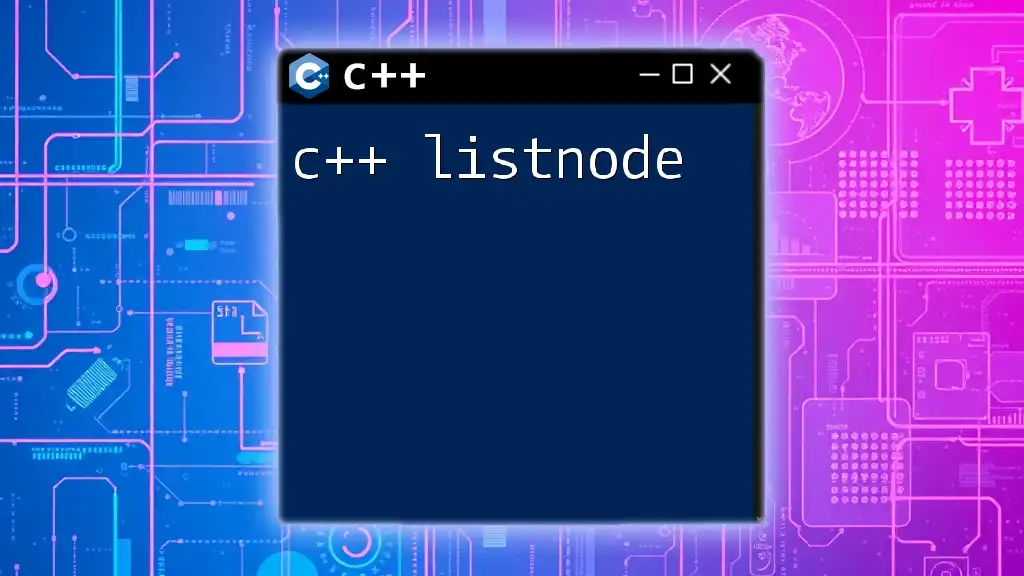
Conclusion
Understanding C++ limits is a fundamental aspect of writing efficient and error-free code. By familiarizing yourself with the range and behavior of various data types, leveraging built-in protections, and employing best practices, you will be better equipped to deal with potential pitfalls in your programming journey. Always remember that a robust understanding of limits not only enhances your code's reliability but also safeguards against common programming errors.