C++ low latency refers to techniques and practices that minimize the delay between input and output, achieving rapid response times crucial for real-time applications.
Here's a simple example demonstrating low latency by using a busy-wait loop to handle an event quickly:
#include <iostream>
#include <chrono>
#include <thread>
void lowLatencyFunction() {
auto start = std::chrono::high_resolution_clock::now();
while (true) {
// Simulate event checking without delay
if (/* condition met */) {
std::cout << "Event handled!" << std::endl;
break; // Exit loop after handling event
}
}
auto end = std::chrono::high_resolution_clock::now();
std::cout << "Event processing time: "
<< std::chrono::duration_cast<std::chrono::microseconds>(end - start).count()
<< " microseconds." << std::endl;
}
int main() {
lowLatencyFunction();
return 0;
}
Understanding Latency in Computing
Latency is one of the most critical performance metrics in computing, representing the time delay from the moment an instruction is initiated to the moment the result is available. In the realm of C++, latency can manifest in several forms including network latency, processing latency, and memory latency. Knowing where these delays occur is vital for optimizing systems, especially in applications where real-time performance is paramount.
Why Low Latency Matters
Low latency is essential across various domains, including high-frequency trading, online gaming, and telecommunications. In high-frequency trading, milliseconds can mean the difference between profit and loss. Similarly, in online gaming, even slight delays can disrupt the user experience and diminish the game's competitiveness.

Key C++ Features for Performance
Memory Management
Effective memory management is crucial for reducing latency in C++. Understanding the nuances of dynamic versus static allocation, as well as the differences between stack and heap usage, can profoundly impact performance.
Example: Efficient Memory Handling Techniques
Here’s a simple example that illustrates the difference between using stack and heap memory:
void stackAllocation() {
int arr[1000]; // Stack allocation
// do something with arr
}
void heapAllocation() {
int* arr = new int[1000]; // Heap allocation
// do something with arr
delete[] arr; // Don’t forget to free heap memory
}
In many cases, stack allocation is faster as it is automatically managed. However, it has limitations on size compared to heap allocation.
Minimizing Object Creation
Frequent object creation and destruction can add significant overhead, especially in performance-critical applications. Use techniques like object pooling to reuse instances, reducing allocation costs.
class ObjectPool {
public:
// Simplified example
Object* acquire() {
if (pool.empty()) {
return new Object();
} else {
Object* obj = pool.back();
pool.pop_back();
return obj;
}
}
void release(Object* obj) {
pool.push_back(obj);
}
private:
std::vector<Object*> pool;
};
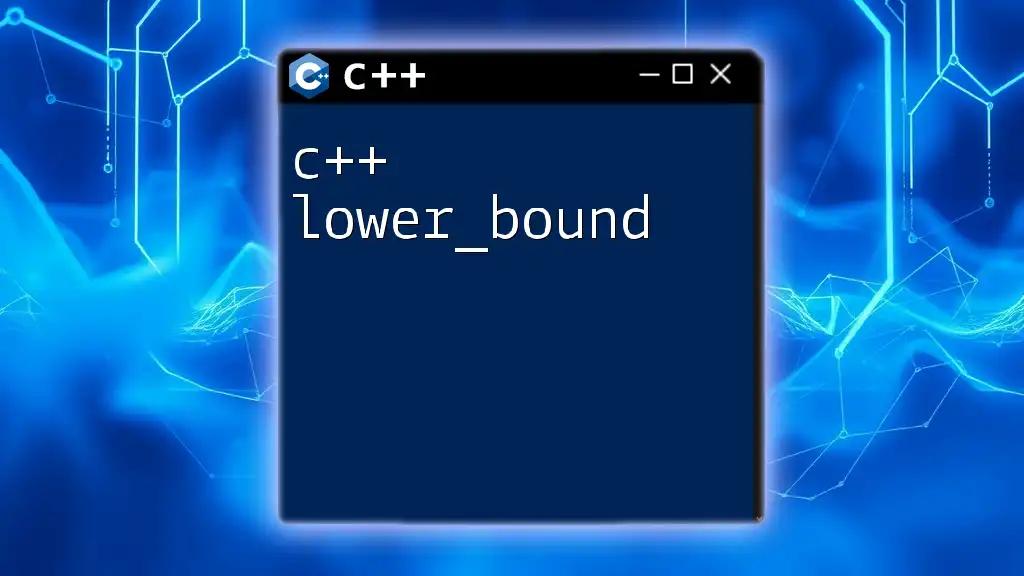
Techniques for Low Latency C++
Optimizing Compiler Settings
Optimizing compiler settings is a crucial step in achieving low latency in C++ applications. Appropriate compiler flags can dramatically enhance performance, enabling the compiler to optimize your code effectively.
Example: Compiler Flags for GCC or Clang
g++ -O2 -march=native -ffast-math your_code.cpp -o your_program
Flags like `-O2` optimize the code while `-march=native` tells the compiler to optimize the binary specifically for your machine's architecture.
Choosing the Right Data Structures
Data structures play a paramount role in the execution speed of C++ applications. The choice of data structure can significantly influence latency.
Example: Performance Comparison of Data Structures
Consider the performance differences when accessing elements in various data structures:
- Arrays provide O(1) access time but have a fixed size.
- Vectors offer dynamic resizing but come with O(n) time complexity for insertions at arbitrary positions.
- Linked Lists provide flexible sizing but have O(n) access time for indexed access.
Understanding these complexities allows developers to choose the optimal data structure for their specific use case.
Algorithm Efficiency
Understanding algorithm efficiency is crucial for minimizing latency. The faster your algorithms execute, the less time your application spends waiting.
Example: Comparing Search Algorithms
#include <vector>
#include <algorithm>
int linearSearch(const std::vector<int>& arr, int target) {
for(size_t i = 0; i < arr.size(); ++i) {
if (arr[i] == target) return i;
}
return -1;
}
int binarySearch(const std::vector<int>& arr, int target) {
auto it = std::lower_bound(arr.begin(), arr.end(), target);
if(it != arr.end() && *it == target) {
return it - arr.begin();
}
return -1;
}
In the example above, binary search is significantly faster than linear search for sorted arrays, demonstrating the importance of using efficient algorithms.
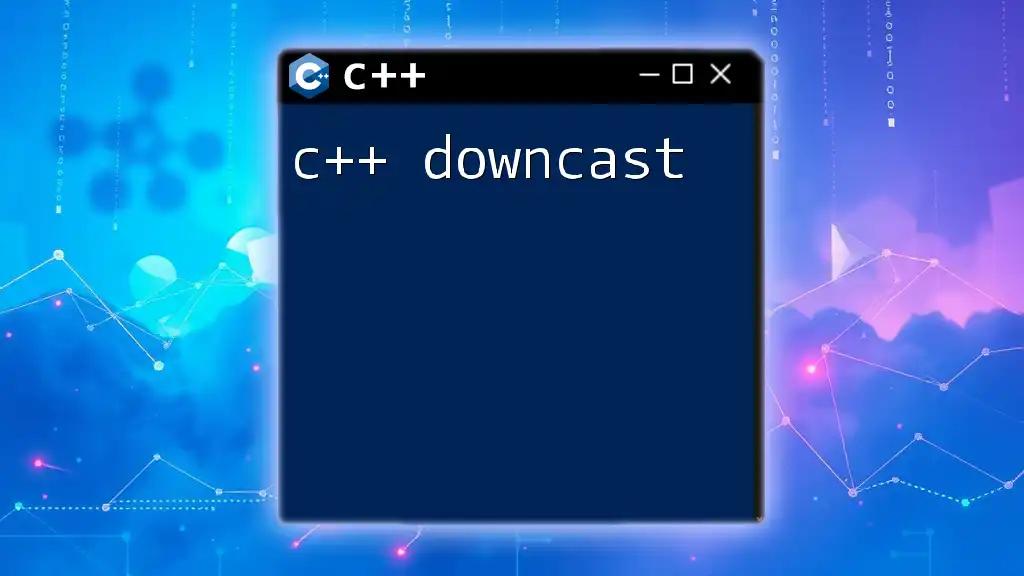
Best Practices for Writing Low Latency Code
Avoiding Unnecessary Complexity
In code design, simplicity can often lead to lower latency. Utilizing minimalistic design principles in C++ helps reduce the cognitive load on the compiler and optimizations can happen more effectively.
Reducing Function Call Overhead
Function calls, while essential for good code structure, can introduce latency. By using the `inline` keyword, developers can suggest to the compiler to avoid function call overhead in simple functions.
Example: Performance of Inline vs. Non-Inline Functions
inline int add(int a, int b) {
return a + b;
}
int main() {
int result = add(3, 5); // Calls inline function
}
Inline functions can speed up performance considerably when used correctly, although indiscriminate use can lead to code bloat.
Concurrency Techniques
Multithreading for Latency Reduction
Leveraging multithreading can vastly improve performance by allowing multiple operations to run concurrently. C++ provides robust threading capabilities through the Standard Library.
#include <thread>
#include <iostream>
void task() {
std::cout << "Executing task in a separate thread." << std::endl;
}
int main() {
std::thread t1(task);
t1.join(); // Ensures main thread waits for t1 to complete
}
This simple multithreading example illustrates how to offload work to a new thread.
Lock-Free Programming
Understanding and implementing lock-free programming can provide a significant boost in applications requiring high throughput and low latency. The use of atomic operations ensures that multiple threads can operate without the need for locking mechanisms.
Example: Creating a Lock-Free Stack
#include <atomic>
#include <memory>
template<typename T>
class LockFreeStack {
public:
void push(T value) {
auto new_node = std::make_unique<Node>();
new_node->data = value;
new_node->next = head.load();
while (!head.compare_exchange_weak(new_node->next, new_node.get()));
new_node.release();
}
private:
struct Node {
T data;
Node* next;
};
std::atomic<Node*> head{nullptr};
};
This code demonstrates a lock-free stack implementation using atomic operations, which can significantly reduce wait times among threads competing for resources.
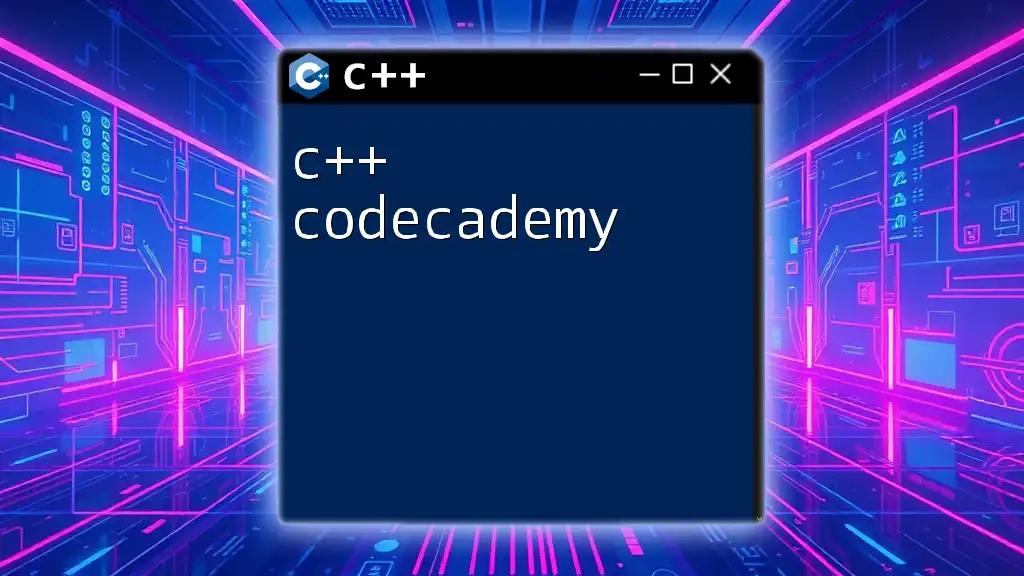
Tools and Libraries for Low Latency C++
Profiling and Benchmarking Tools
Utilizing profiling tools allows developers to identify bottlenecks within their code. Tools like Valgrind or gprof can aid in providing performance metrics and help analyze where latency issues lie.
Example: Using gprof for Performance Analysis
Compile your code with profiling enabled:
g++ -pg your_code.cpp -o your_program
After running your program, use gprof to analyze the output:
gprof your_program gmon.out > analysis.txt
C++ Libraries for Low Latency Applications
Several libraries target low-latency applications effectively. Libraries like Boost, POCO, and ZeroMQ can help accelerate the development of high-performance applications.
Example: Implementing a Basic Client-Server Model with ZeroMQ
// Server
#include <zmq.hpp>
int main() {
zmq::context_t context(1);
zmq::socket_t socket(context, ZMQ_REP);
socket.bind("tcp://*:5555");
while (true) {
zmq::message_t request;
socket.recv(&request);
// process request
socket.send(zmq::message_t("Response"), zmq::send_flags::none);
}
}
In this example, we set up a basic server using ZeroMQ that can handle requests efficiently, showcasing its suitability for real-time applications.
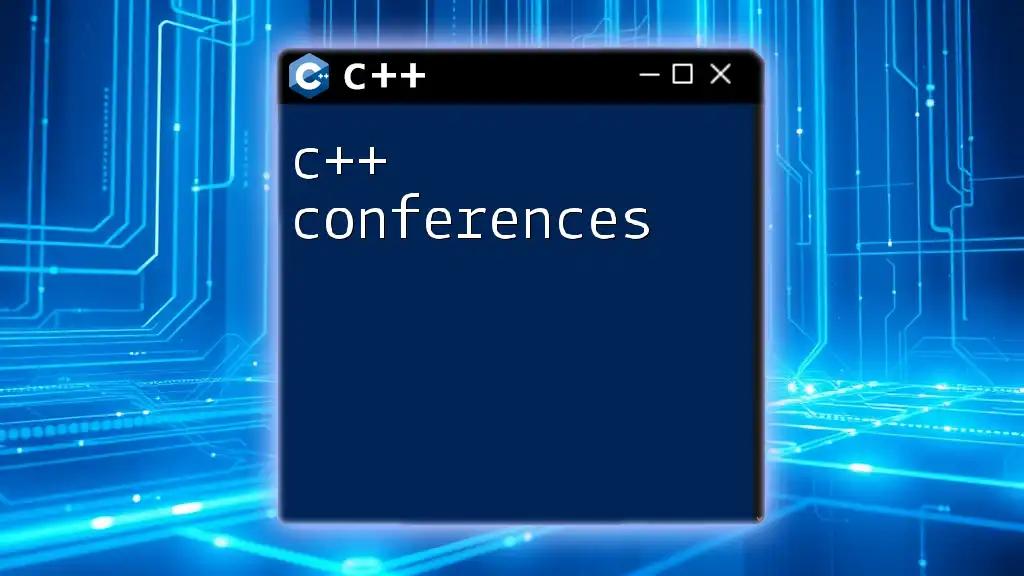
Real-World Applications of Low Latency C++
Case Study: High-Frequency Trading Systems
High-frequency trading (HFT) systems leverage low latency to capitalize on minute changes in market conditions. Key attributes of these systems include rapid data processing, efficient network communication, and robust error handling.
To achieve low latency, HFT systems often utilize specialized hardware and software stacks, including FPGA processing and optimized network stacks.
Low Latency in Gaming
In gaming, particularly in real-time multiplayer environments, low latency is critical to maintaining a fair playing field. Game engines must be designed to synchronize state across clients while minimizing delays that can impede performance or create a poor user experience.

Conclusion
In summary, achieving C++ low latency is a multifaceted endeavor that requires careful attention to detail, including efficient memory management, optimal use of data structures, and leveraging advanced programming techniques such as concurrency and lock-free programming. Each of these components contributes to building applications that can respond to user actions and external events with minimal delay.
As the field of software engineering evolves, staying informed about best practices and emerging trends in low-latency programming will be essential for developers aiming to excel in performance-critical applications. Exploring and implementing these techniques will enhance your ability to contribute to successful low-latency solutions in a range of applications.