Building low latency applications with C++ involves utilizing efficient algorithms and optimized data structures to minimize delay in processing and transmission of data.
Here's a simple example demonstrating how to use a busy-wait loop for low-latency polling in C++:
#include <iostream>
#include <chrono>
void busyWait() {
auto start = std::chrono::high_resolution_clock::now();
while (std::chrono::high_resolution_clock::now() - start < std::chrono::milliseconds(1)) {
// Busy waiting
}
std::cout << "Waited for 1 millisecond." << std::endl;
}
int main() {
busyWait();
return 0;
}
Understanding Latency
What is Latency?
Latency refers to the time it takes for data to travel from one point to another within a system. In the context of applications, particularly those that require speed and responsiveness, latency can significantly affect user experience and system performance. There are various types of latency, such as:
- Network Latency: The time taken for packets to travel over the network.
- Processing Latency: The time taken by the CPU to process data.
- Input/Output (I/O) Latency: The delay caused during read/write operations.
Metrics for measuring latency include round-trip time (RTT), one-way delay, and processing time. Monitoring these metrics is crucial for understanding and mitigating latency in applications.
Causes of Latency
Latency can arise from several sources, impacting the responsiveness of applications:
Hardware Limitations:
The processing speed of the CPU, the size and speed of RAM, and the type of storage (SSD vs. HDD) can all influence latency. For instance, slower CPUs may struggle with complex calculations, introducing delays.
Software Design:
Suboptimal algorithms and data handling can lead to excessive computational overhead. For example, nested loops or inefficient sorting routines can significantly increase processing time.
External Factors:
Network-related delays, such as packet loss, congestion, or unreliable connections, are often substantial contributors to network latency.
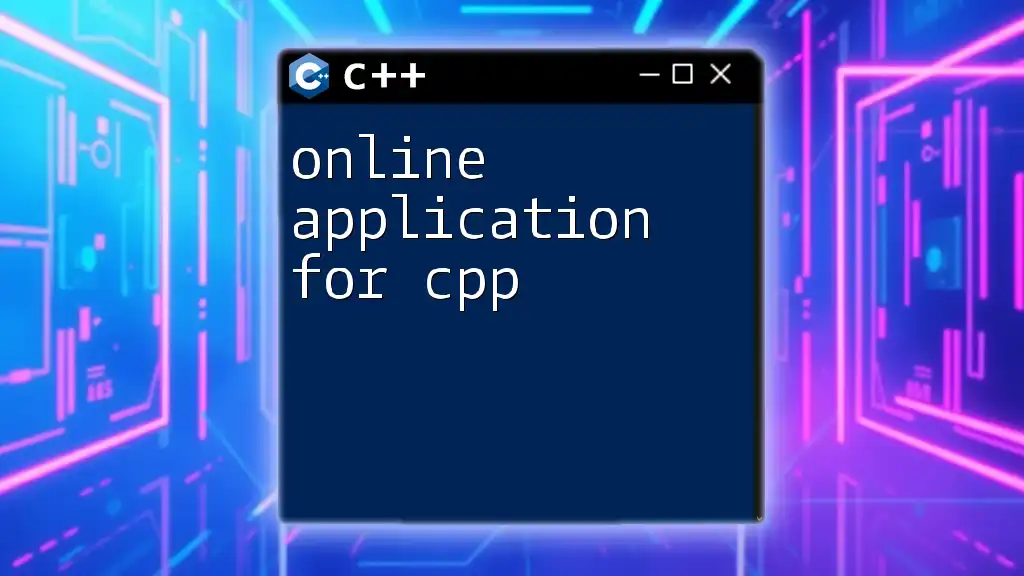
Best Practices for Building Low Latency Applications
Efficient Algorithms and Data Structures
Choosing the right algorithm is critical in minimizing latency. In applications where speed is paramount, opt for algorithms that have lower time complexity.
Examples of low-latency data structures include:
-
Queues: Ideal for scenarios where you need to manage tasks or messages with FIFO (First In First Out) logic.
#include <queue> std::queue<int> q; q.push(10); q.push(20); int front = q.front(); // Get front element q.pop(); // Remove front element
-
Heaps: Useful for managing dynamic priority tasks, heaps allow efficient inserts and deletions.
Memory Management Techniques
Dynamic Memory Allocation
Dynamic memory allocation can be a source of latency due to the overhead of allocating and deallocating memory on the heap. To minimize this, consider using fixed-size memory pools for frequently allocated objects.
#include <iostream>
#include <vector>
class MemoryPool {
private:
std::vector<int*> pool;
public:
int* allocate() {
if (!pool.empty()) {
int* ptr = pool.back();
pool.pop_back();
return ptr;
}
return new int; // If pool is empty, allocate new memory
}
void deallocate(int* ptr) {
pool.push_back(ptr);
}
~MemoryPool() {
for (int* ptr : pool) {
delete ptr; // Clean up remaining pointers
}
}
};
Cache Optimization
Understanding CPU cache structures is essential for writing latency-sensitive code. Optimizing your code to minimize cache misses can greatly reduce latency. For example, ensure data structures are contiguous to assist with better cache utilization.
Consider the following code example demonstrating a cache-friendly pattern:
#include <vector>
const int ARRAY_SIZE = 100000;
void accessArray(std::vector<int>& data) {
for (int i = 0; i < ARRAY_SIZE; ++i) {
data[i] = i * 2; // Accessing in a contiguous manner
}
}
Concurrency in C++
Multithreading Overview
Multithreading can be a powerful technique for reducing overall latency in applications by allowing multiple operations to occur simultaneously. However, it introduces complexity, especially concerning data consistency and race conditions.
C++ Threading Libraries
C++ provides several libraries for handling threads, such as `<thread>`, `<mutex>`, and `<condition_variable>`. Using these libraries makes it easier to implement effective multithreading strategies.
Here’s a simple code example to illustrate creating threads in C++:
#include <iostream>
#include <thread>
void task() {
std::cout << "Task running in thread: " << std::this_thread::get_id() << std::endl;
}
int main() {
std::thread t1(task);
std::thread t2(task);
t1.join();
t2.join();
return 0;
}
Networking Considerations
Choosing the Right Protocol
When dealing with networked applications, selecting the appropriate protocol is critical. For example, TCP is reliable but introduces overhead due to its connection-oriented nature and error-checking, while UDP is faster but offers no guarantees on packet delivery.
Asynchronous I/O
Implementing asynchronous I/O operations can minimize blocking calls and enhance performance in network-programming contexts. With libraries like Boost.Asio, you can achieve robust asynchronous networking, allowing your application to handle multiple connections efficiently.
Here's a brief code snippet to demonstrate async server operations using Boost.Asio:
#include <boost/asio.hpp>
void handleClient(boost::asio::ip::tcp::socket socket) {
// Handle incoming connection
}
int main() {
boost::asio::io_context io_context;
boost::asio::ip::tcp::acceptor acceptor(io_context, {boost::asio::ip::tcp::v4(), 12345});
while (true) {
boost::asio::ip::tcp::socket socket(io_context);
acceptor.accept(socket); // Accept a new connection
std::thread(handleClient, std::move(socket)).detach(); // Process in new thread
}
return 0;
}
Batch Processing
Reducing the number of round trips can significantly lessen latency. Instead of sending individual messages or data packets, consider batching them to transmit larger sets of data in a single request whenever possible.
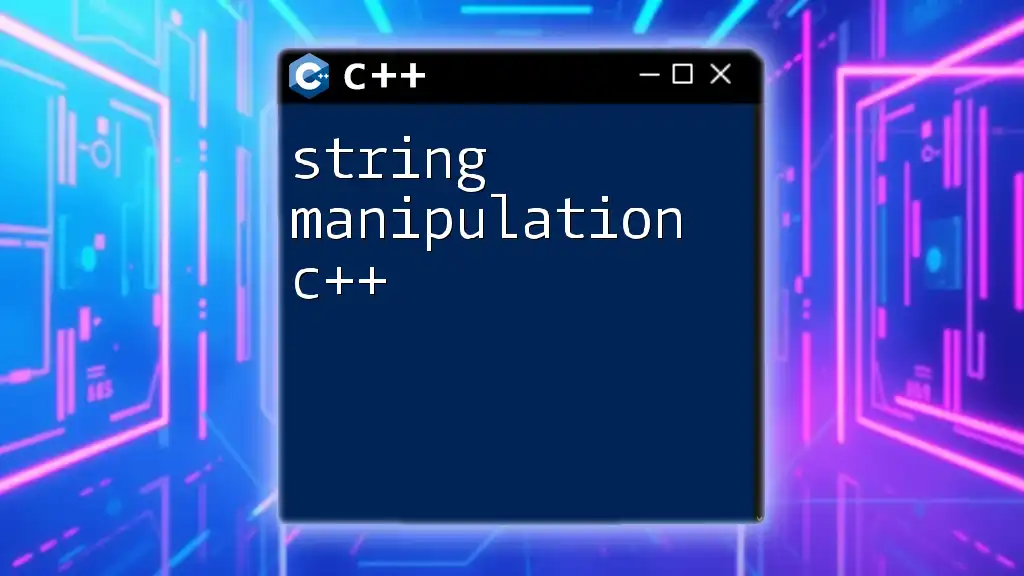
Advanced Techniques for Low Latency
Real-time Systems
Understanding real-time constraints is vital for applications needing consistent low-latency performance. Real-time operating systems (RTOS) are designed to process data as it comes in, typically used in systems where delays cannot be tolerated (e.g., embedded systems).
Lock-Free Programming
Lock-free programming eliminates the need for locks, thereby reducing thread contention and improving performance. Utilizing lock-free data structures allows multiple threads to operate on the same resource without interfering with each other.
Here's an example of a simple lock-free queue implementation:
#include <atomic>
#include <memory>
#include <iostream>
template<typename T>
class LockFreeQueue {
private:
struct Node {
T data;
Node* next;
Node(T const& value) : data(value), next(nullptr) {}
};
std::atomic<Node*> head;
std::atomic<Node*> tail;
public:
LockFreeQueue() {
Node* dummy = new Node(T());
head.store(dummy);
tail.store(dummy);
}
void enqueue(T const& value) {
Node* newNode = new Node(value);
Node* oldTail = tail.load();
oldTail->next = newNode;
tail.store(newNode);
}
T dequeue() {
Node* oldHead = head.load();
Node* nextHead = oldHead->next;
if (nextHead) {
head.store(nextHead);
T value = nextHead->data;
delete oldHead; // Free up the old head
return value;
}
throw std::runtime_error("Queue is empty"); // Handle empty case
}
~LockFreeQueue() {
while (Node* node = head.load()) {
head.store(node->next);
delete node;
}
}
};
Profiling and Optimization
Profiling Tools for C++
Profiling is crucial for identifying performance bottlenecks in your application. Tools such as Valgrind, gprof, and Visual Studio Profiler offer insights into function call durations, memory usage, and thread performance.
Optimization Techniques
After profiling, use insights gained to perform optimizations. Ensure that compiler optimizations, such as -O2 or -O3 flags in GCC, are enabled to generate more efficient machine code. Code optimization based on profiling results may involve rewriting algorithms or refactoring code to reduce overhead.
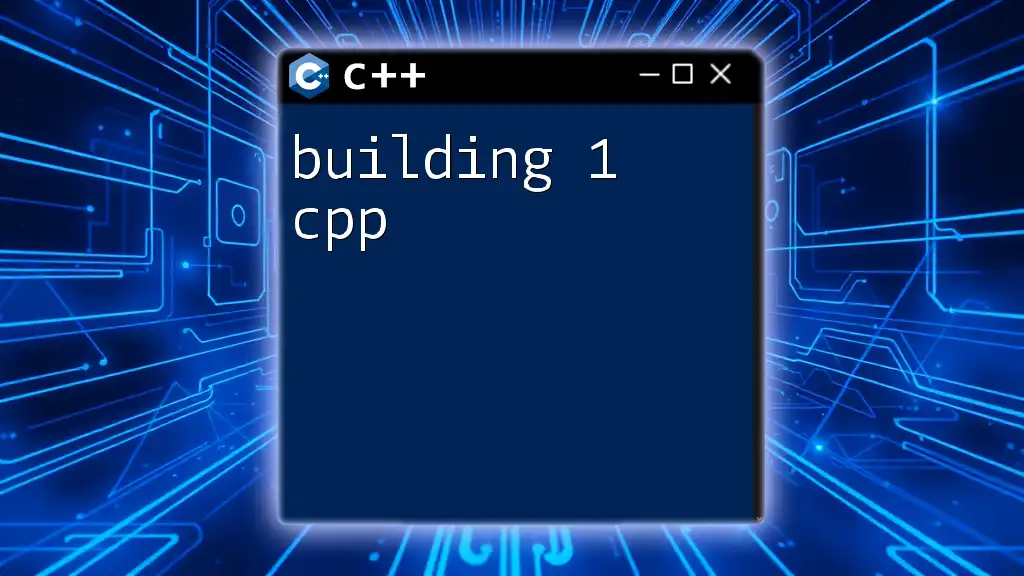
Case Studies
Financial Trading Systems
In environments like stock exchanges, maintaining low latency is vital. A trading algorithm must process buy and sell orders in milliseconds. Architectures often employ:
- In-memory data grids for rapid access.
- Low-level networking techniques to minimize protocol overhead.
- Direct connections to market databases to avoid intermediaries.
Online Gaming Engines
Multiplayer games demand low latency for smooth interaction among players. Techniques employed include:
- Client-side prediction to improve responsiveness.
- Lag compensation strategies to adjust inputs from players.
- Spatial partitioning to reduce the number of interactions processed each frame.
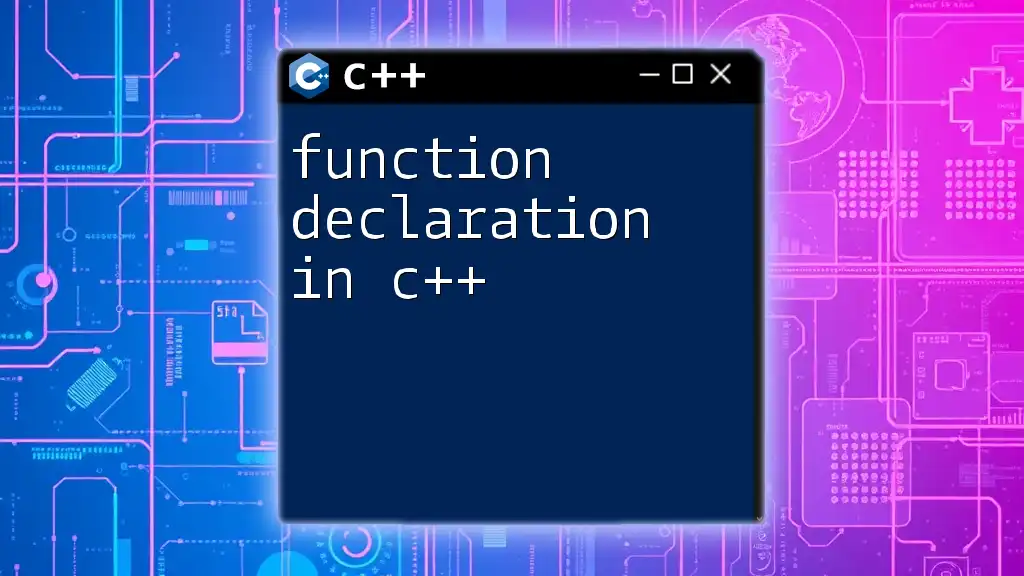
Tools and Libraries for Low Latency in C++
Specific libraries and frameworks are designed with low latency in mind, enhancing development efficiency and performance. They include:
- Boost.Asio: An asynchronous I/O library for networking.
- Google’s Protobuf: Efficient serialization of structured data.
- ZeroMQ: High-performance messaging library for building distributed applications.
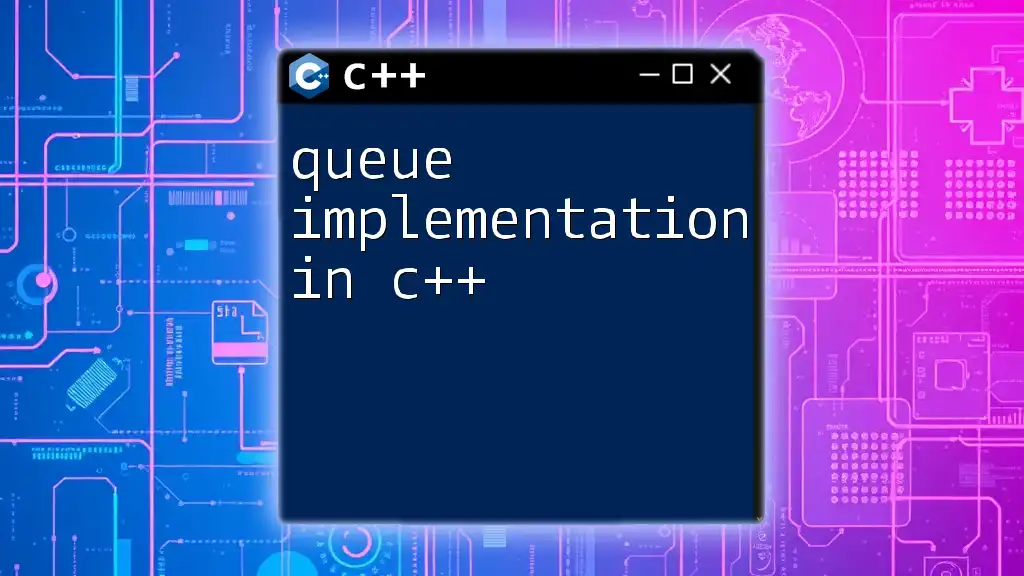
Conclusion
Building low latency applications with C++ requires a comprehensive understanding of both the underlying principles and practical strategies to implement them effectively. By leveraging efficient algorithms, optimizing memory use, embracing concurrency, and utilizing appropriate tools and libraries, developers can create robust applications that meet modern performance demands.
With continuous evolution in technology, developers are encouraged to stay informed and experiment with new paradigms and techniques to enhance the speed and responsiveness of their applications.