Software architecture in C++ involves designing a robust structure for applications by effectively utilizing various design patterns and principles to ensure scalability, maintainability, and efficiency.
Here's a simple example illustrating the Factory Design Pattern in C++:
#include <iostream>
#include <memory>
// Product interface
class Shape {
public:
virtual void draw() = 0;
};
// Concrete products
class Circle : public Shape {
public:
void draw() override {
std::cout << "Drawing a Circle" << std::endl;
}
};
class Square : public Shape {
public:
void draw() override {
std::cout << "Drawing a Square" << std::endl;
}
};
// Factory
class ShapeFactory {
public:
std::unique_ptr<Shape> createShape(const std::string &shapeType) {
if (shapeType == "Circle") {
return std::make_unique<Circle>();
} else if (shapeType == "Square") {
return std::make_unique<Square>();
}
return nullptr;
}
};
// Client code
int main() {
ShapeFactory shapeFactory;
auto shape1 = shapeFactory.createShape("Circle");
shape1->draw(); // Outputs: Drawing a Circle
auto shape2 = shapeFactory.createShape("Square");
shape2->draw(); // Outputs: Drawing a Square
return 0;
}
Understanding Software Architecture
What is Software Architecture?
Software architecture refers to the high-level structure of a software system. It is the blueprint that outlines the system’s components, their interactions, and the principles governing their design and evolution. Software architecture plays a critical role in the software development life cycle, influencing both the technical and organizational aspects of a project.
The primary goals of software architecture include scalability, ensuring that the system can grow and handle increased loads; maintainability, allowing for easier updates and enhancements; performance, ensuring the system operates efficiently; and security, safeguarding data and resources from vulnerabilities.
Key Architectural Patterns
Layered Architecture
In a layered architecture, software is organized into layers, each responsible for a distinct role. The most common layers are:
- Presentation Layer: Handles UI and user interaction.
- Business Logic Layer: Contains the core functionality and business rules.
- Data Access Layer: Manages data storage and retrieval.
Example: A simple web application may be structured with a clear demarcation between these layers, ensuring that changes in one layer do not ripple through the others.
Here’s a brief code snippet that illustrates a possible C++ class structure for these layers:
class DataAccessLayer {
public:
void saveData(const std::string& data) {
// Code to save data
}
};
class BusinessLogicLayer {
DataAccessLayer dal;
public:
void processData(const std::string& input) {
// Process input data
dal.saveData(input);
}
};
class PresentationLayer {
BusinessLogicLayer bll;
public:
void userInput(const std::string& input) {
bll.processData(input);
}
};
Microservices Architecture
Microservices architecture breaks down a software application into smaller, manageable services that communicate over a network. This pattern enhances scalability and allows for independent deployment of services.
Benefits include:
- Flexibility: Each service can be developed, deployed, and scaled independently.
- Technology Diversity: Different services can utilize different technologies based on their needs.
However, it also presents challenges such as network latency and increased complexity in service management.
Example: Consider a simple e-commerce application where each function (user service, product service, order service) is a separate microservice.
Here's how a basic C++ service endpoint might look using the Pistache framework:
#include <pistache/endpoint.h>
using namespace Pistache;
class MyService : public Http::Handler {
public:
Http::Response onRequest(const Http::Request& request) override {
return Http::Response(Http::Code::Ok, "Service Response");
}
};
Client-Server Architecture
The client-server model organizes applications into two distinct components: the client, which requests resources, and the server, which provides them.
Advantages of client-server architecture include:
- Centralized data management.
- Enhanced security by isolating the data server from clients.
Example: A chat application could utilize this model where the client interface sends messages to a server that processes and relays them to other users.
Here's a simple C++ socket programming example to illustrate client-server communication:
// Server Side
void startServer() {
int server_fd = socket(AF_INET, SOCK_STREAM, 0);
bind(server_fd, (struct sockaddr*)&address, sizeof(address));
listen(server_fd, 3);
int new_socket = accept(server_fd, (struct sockaddr*)&address, (socklen_t*)&addrlen);
}
// Client Side
void connectToServer(const char* ip, int port) {
int sock = socket(AF_INET, SOCK_STREAM, 0);
connect(sock, (struct sockaddr*)&server_address, sizeof(server_address));
}
Event-Driven Architecture
Event-driven architecture is a design paradigm that allows the system to react to events as they occur, making it especially useful for applications that require real-time processing.
Characteristics include:
- Asynchronous communication, enabling non-blocking operations.
- Flexible system architecture that can easily incorporate new events.
In C++, an event-driven design can be achieved using design patterns like Observer. Below is a simplistic example:
class Event {
// Event data
};
class Observer {
public:
virtual void onEvent(const Event& event) = 0;
};
class EventManager {
std::vector<Observer*> observers;
public:
void notify(const Event& event) {
for (auto observer : observers) {
observer->onEvent(event);
}
}
};
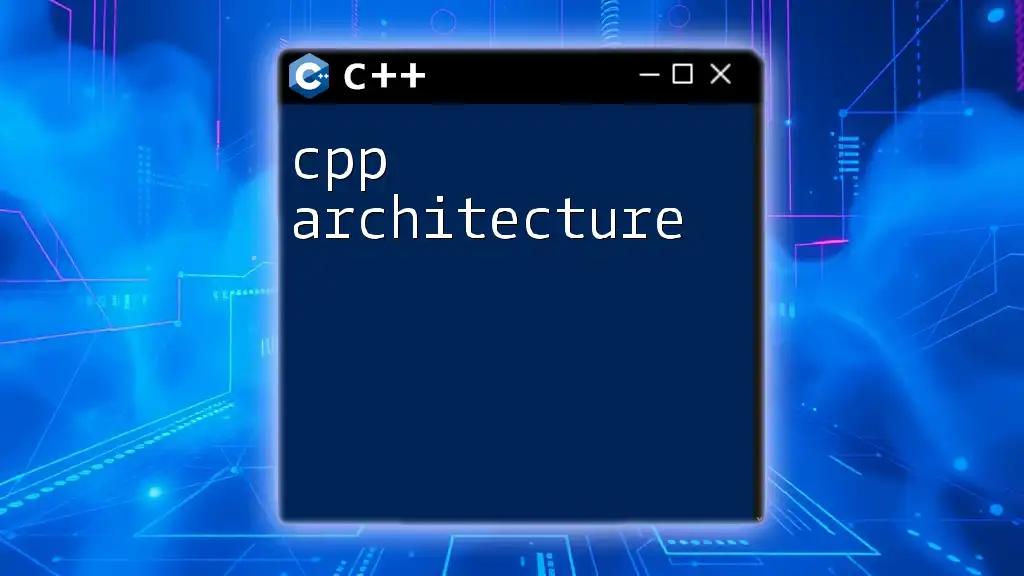
C++ Features Supporting Software Architecture
Object-Oriented Programming (OOP)
Object-oriented programming is fundamental to C++ and significantly influences software architecture.
The four core principles of OOP are:
- Encapsulation: Bundling data and methods that operate on the data.
- Inheritance: Creating new classes based on existing ones to promote code reuse.
- Polymorphism: Allowing methods to do different things based on the object it is acting upon.
Example: A C++ class utilizing these principles might look like:
class Shape {
public:
virtual double area() = 0; // Pure virtual function
};
class Circle : public Shape {
private:
double radius;
public:
Circle(double r) : radius(r) {}
double area() override {
return 3.14 * radius * radius;
}
};
class Square : public Shape {
private:
double side;
public:
Square(double s) : side(s) {}
double area() override {
return side * side;
}
};
Templates and Generic Programming
C++ templates enable the creation of functions and classes that operate with any data type. This feature is beneficial for building flexible and reusable components within an architecture.
Generic programming allows code to be written once and reused for any type, significantly reducing code duplication.
Example: A simple C++ class implementing a generic stack could look like this:
template<typename T>
class Stack {
std::vector<T> elements;
public:
void push(const T& element) {
elements.push_back(element);
}
T pop() {
T elem = elements.back();
elements.pop_back();
return elem;
}
};
Standard Template Library (STL)
The Standard Template Library (STL) is an essential component of C++ that provides a set of common data structures (like vectors, lists, and maps) and algorithms (like sorting and searching).
STL enhances software architecture by simplifying the implementation of common functionalities, promoting efficient and reliable coding practices.
Example: Using STL’s `std::vector` for dynamic arrays could improve the design of a program:
#include <vector>
class DataProcessor {
std::vector<int> data;
public:
void addData(int value) {
data.push_back(value);
}
};
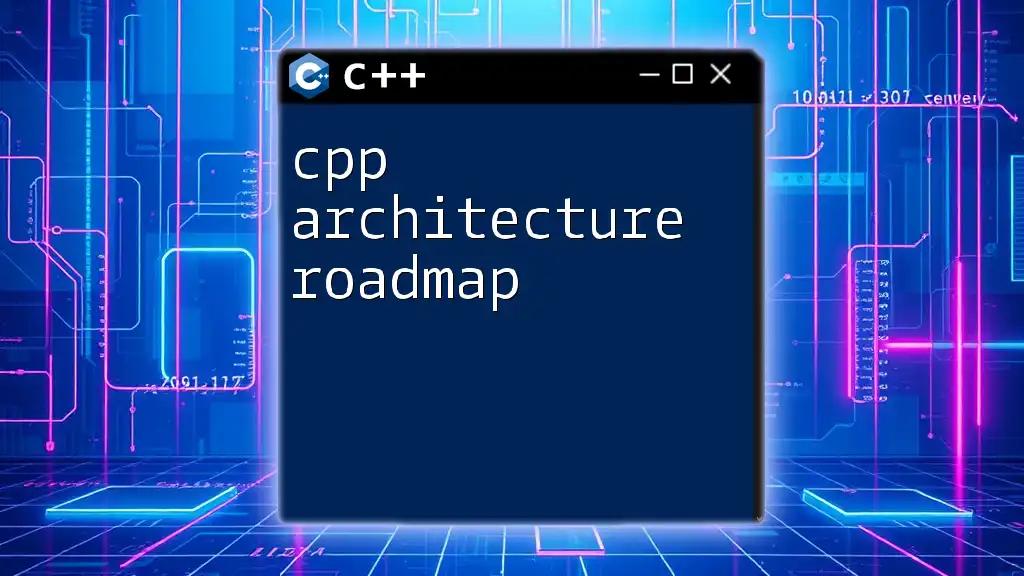
Designing a Software Architecture
Requirements Analysis
Understanding user needs is paramount for effective software architecture. Techniques like interviews, surveys, and use-case diagrams can aid in gathering comprehensive requirements.
Example: Creating user story maps helps visualize the relationships between tasks and user needs, ensuring all perspectives are considered during development.
Architectural Design Principles
SOLID Principles
The SOLID principles provide a set of guidelines for designing maintainable and scalable software. Each principle stands on its own, but they collectively enhance software architecture in C++. Here's a brief overview:
- Single Responsibility Principle: A class should only have one reason to change.
- Open/Closed Principle: Software entities should be open for extension but closed for modification.
- Liskov Substitution Principle: Subtypes must be substitutable for their base types.
- Interface Segregation Principle: Clients should not be forced to depend on interfaces they do not use.
- Dependency Inversion Principle: High-level modules should not depend on low-level modules; both should depend on abstractions.
Example: Applying these principles in a C++ project can prevent complex interdependencies and ease future enhancements.
DRY (Don't Repeat Yourself)
The DRY principle emphasizes the importance of minimizing duplication in code. This can be achieved through the use of functions, classes, and templates in C++.
Example: A C++ codebase can be refactored to eliminate repetitive code by abstracting common functionalities into reusable components:
class Logger {
public:
void log(const std::string& message) {
std::cout << message << std::endl;
}
};
// Usage
Logger logger;
logger.log("This is a log message.");
Documentation and Communication
Effective documentation is central to successful software architecture. Clear documentation helps various stakeholders understand the system's design, reducing miscommunication and errors.
Tools/resources for documenting architecture include UML diagrams and architecture description languages (ADLs). Establishing a consistent documentation format can greatly enhance clarity and accessibility.

Testing and Maintaining Software Architecture
Testing Strategies
Implementing various types of testing—unit, integration, and system testing—is crucial for ensuring a robust software architecture. Automated testing allows for quick feedback on code changes, fostering rapid development cycles.
Example: Popular testing frameworks for C++ include Google Test and Catch2, which facilitate the creation and management of test cases.
Code Snippet: A simple unit test using Google Test:
#include <gtest/gtest.h>
TEST(SampleTest, TestAddition) {
EXPECT_EQ(2 + 2, 4);
}
Performance Optimization
Performance is a vital aspect of software architecture. Profiling and tuning the system helps identify bottlenecks, allowing developers to optimize critical paths in their C++ applications.
Tools available for performance tuning in C++ include `gprof`, `valgrind`, and `perf`. Understanding differences between pre-optimization and post-optimization analysis is key to effective improvements.
Example: Monitor an application to measure execution times before and after implementing optimizations.
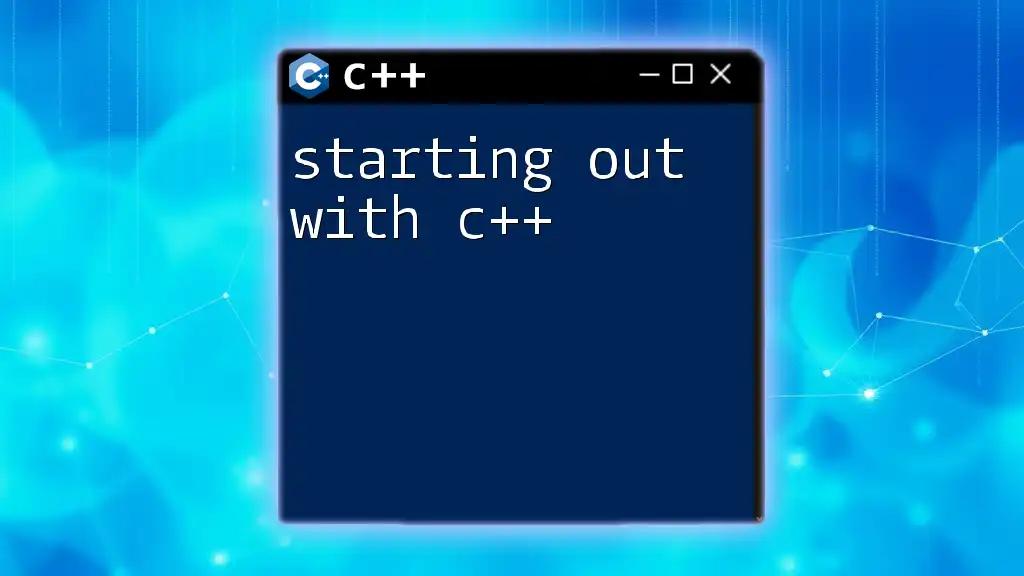
Conclusion
Software architecture with C++ is a multifaceted subject that combines principles of good design, C++ features, and effective processes to create scalable, maintainable, and efficient systems. By leveraging the strengths of C++, software architects can create robust systems tailored to meet user needs while future-proofing against evolution and change.
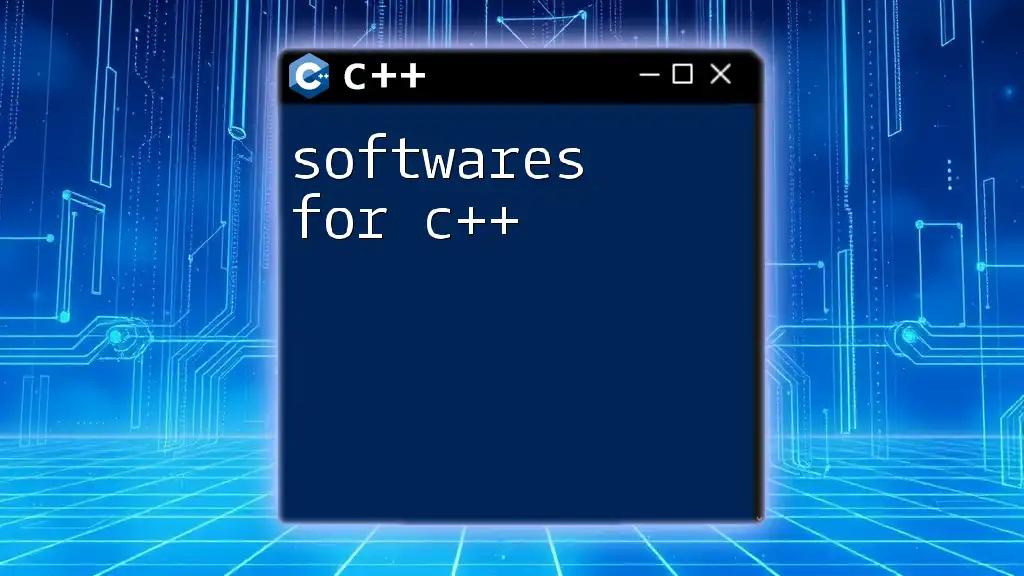
Additional Resources
For further exploration, consider diving into these books, articles, and online resources that delve deeper into best practices and advanced concepts in software architecture and C++ programming.
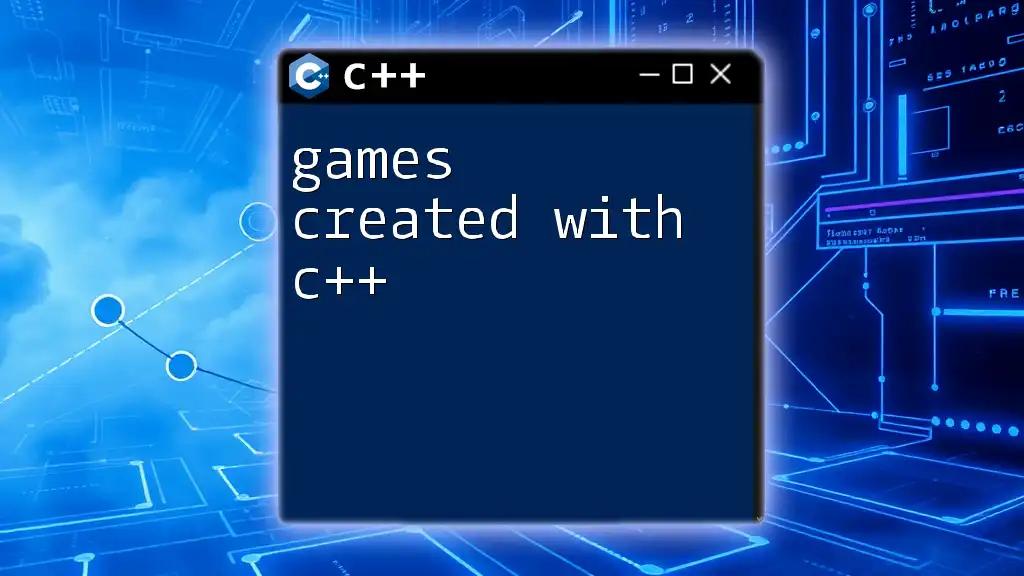
Call to Action
We invite you to share your experiences in implementing software architecture with C++. Join our community to exchange insights, ask questions, and stay updated on courses that will enhance your C++ skills!