A function signature in C++ defines the function's name, return type, and parameters, providing a blueprint for how to call the function.
int add(int a, int b);
The Basics of C++ Function Signatures
What is a Function Signature?
A function signature in C++ serves as a declaration of a function, specifying its return type, name, and parameters. It tells the compiler what to expect from a function without defining the entire function body. Understanding function signatures is essential, as they allow for function calls and help maintain the structure and organization of your code.
Anatomy of a C++ Function Signature
A function signature consists of three fundamental components:
- Return Type: This indicates the data type of the value the function will return. Common return types in C++ include `int`, `float`, `void`, and more.
- Function Name: This is an identifier that uniquely names the function. It's crucial for readability and maintenance.
- Parameter List: This part may include one or more parameters that the function can take as input. Each parameter has a defined type and an identifier (name).
Example of a Function Signature
Here’s a simple example of a function signature:
int add(int a, int b);
In the above example:
- `int` is the return type.
- `add` is the function name.
- `(int a, int b)` is the parameter list, containing two integers.
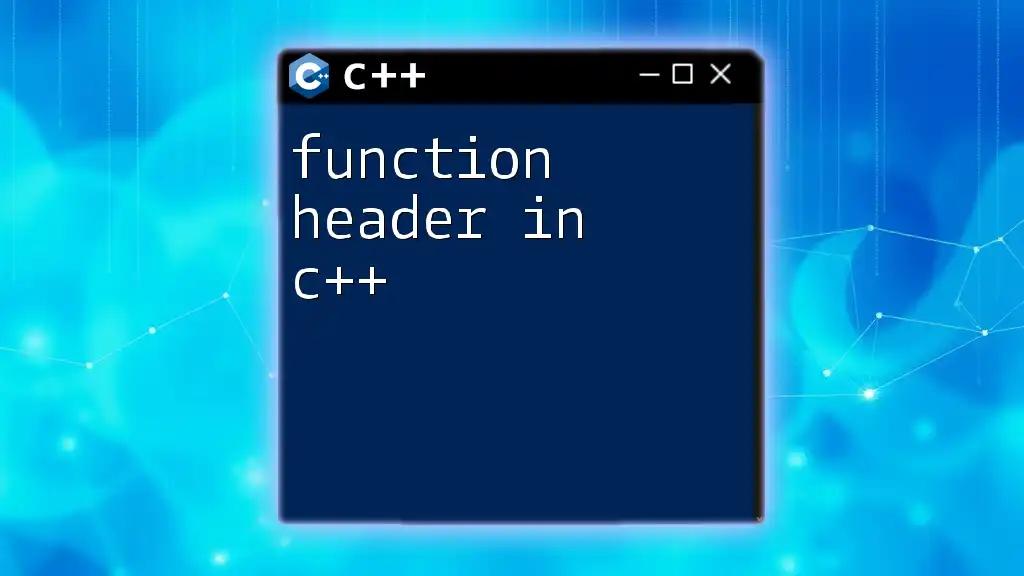
Writing C++ Function Signatures
Syntax of Function Signatures in C++
The general structure of a function signature in C++ appears like this:
<return_type> <function_name>(<parameter_type> <parameter_name>, ...);
It's important to adhere to this syntax to avoid confusion and errors.
Common Mistakes in Function Signatures
When writing function signatures, developers often make a few common mistakes:
- Forgetting the Return Type: Omitting the return type will lead to compilation errors, as the compiler won’t know what to expect from the function.
- Mismatched Parameter Types: Ensure that the types declared in the function signature match the expected types when the function is called.
- Using Keywords Incorrectly: Misusing access specifiers or data type keywords can lead to unexpected behavior or compilation errors.
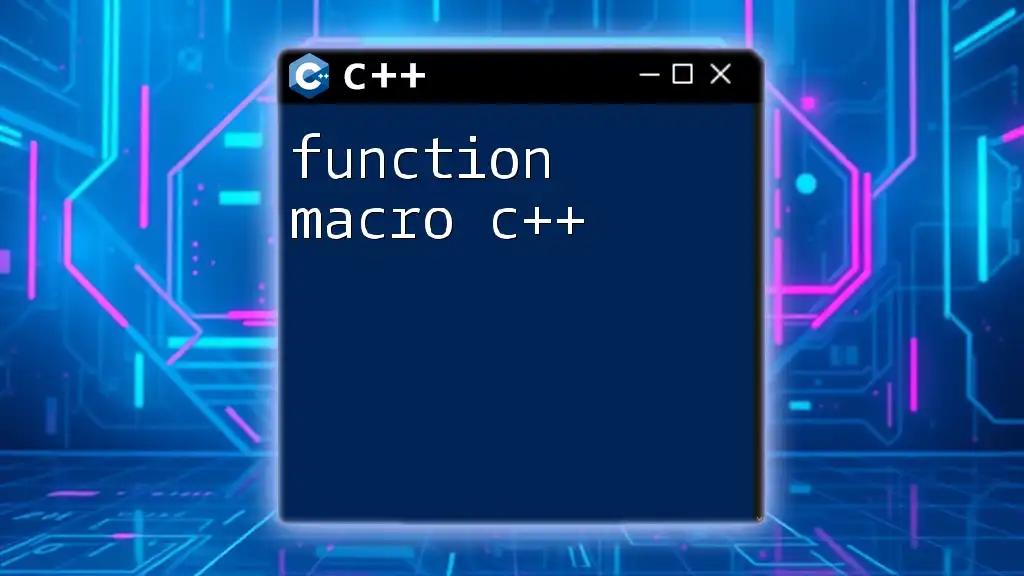
Types of Function Signatures
Simple Function Signatures
A simple function signature is straightforward and does not take any parameters. For example:
void displayMessage();
In this case, the return type is `void`, indicating that the function does not return a value.
Function Signatures with Parameters
Functions can accept parameters, which provide input data to work with. Here are some examples:
float calculateArea(float radius);
int sum(int a, int b, int c);
In these examples:
- `calculateArea` takes one parameter (a `float`), while `sum` takes three integers.
Function Signatures with Default Parameters
C++ allows default parameter values, which means you can call a function without specifying all arguments. For instance:
void logMessage(std::string message, int level = 1);
If the `level` is not provided during a call, it defaults to `1`, simplifying function calls while enhancing flexibility.
Overloaded Function Signatures
Function overloading allows multiple functions with the same name but different signatures. This helps create intuitive APIs. For example:
int add(int a, int b);
double add(double a, double b);
Both `add` functions serve different data types—`int` and `double`, respectively—yet can be called identically.
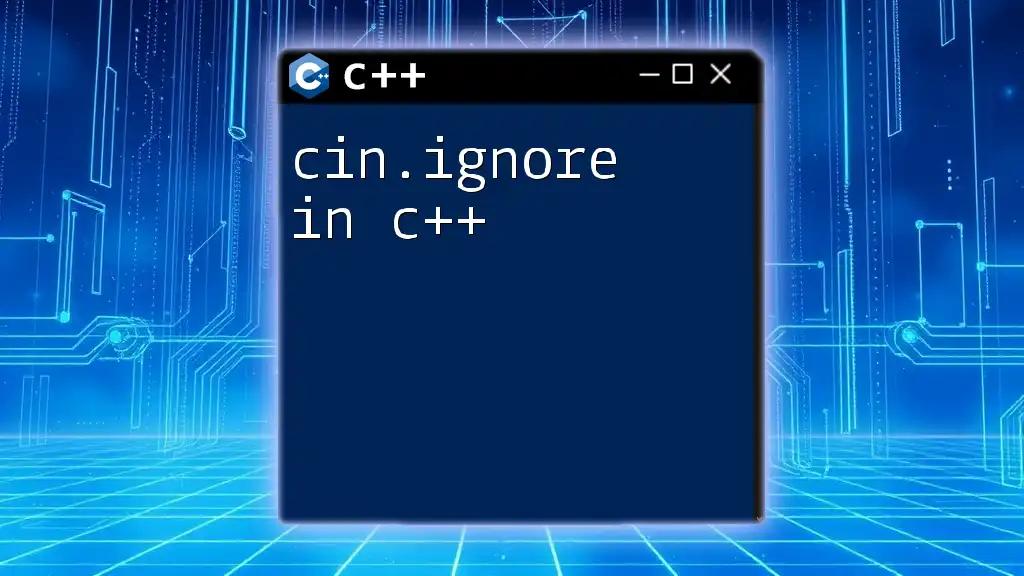
Advanced Topics in C++ Function Signatures
Function Pointer Signatures
Function pointers permit you to store addresses of functions. Its signature looks like:
void (*funcPtr)(int);
This declaration defines a pointer `funcPtr` that points to a function taking an `int` as its sole parameter and returning `void`. Practically, this enables dynamic function calls.
Lambda Expressions in Function Signatures
Lambda expressions offer a concise way to implement function-like behavior without a formal declaration. Here's an example:
auto add = [](int a, int b) { return a + b; };
This syntax creates an anonymous function that adds two integers, demonstrating flexibility in function definitions.
Templates and Function Signatures
Function templates allow you to define functions that operate with different data types, enhancing code reusability. Consider this template function signature:
template <typename T>
T add(T a, T b);
This template permits the `add` function to accept any data type specified during invocation, be it `int`, `float`, or custom types.
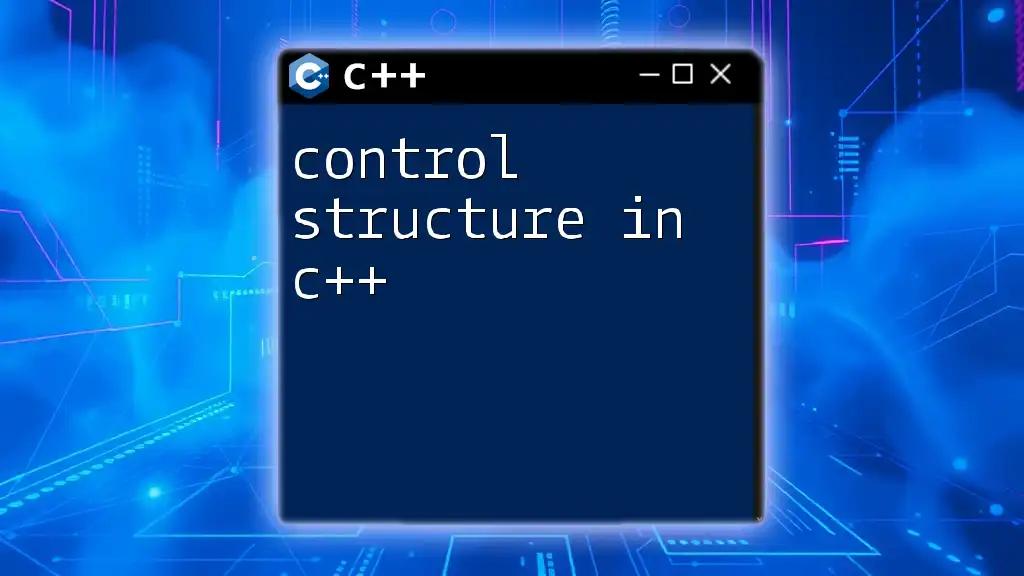
Best Practices for C++ Function Signatures
Maintainability and Readability
Creating clear signatures is fundamental for maintaining and understanding code. Use explanatory names and appropriate return types to ensure clarity. A well-structured function signature conveys the function's purpose even before inspecting its implementation.
Consistency Across Function Signatures
Consistency is vital in function signatures. Adopt uniform naming conventions and parameter types throughout your codebase. This aids maintainability and helps both current and future developers understand your code more readily.

Conclusion
In C++, the function signature is a critical aspect of functions, encapsulating essential information such as return type, name, and parameters. A solid understanding of function signatures not only facilitates better programming practices but also enables developers to write more maintainable and readable code. By mastering function signatures, you enhance your skills and enable smoother collaborations in your programming endeavors.

Additional Resources
For further learning, refer to the official C++ documentation, online tutorials, and relevant books that delve into function signatures and best programming practices. Regular practice will solidify these concepts and improve your coding proficiency.