In the realm of C++, various software tools like IDEs (Integrated Development Environments), compilers, and debuggers play a crucial role in enhancing productivity and streamlining the coding process.
Here's a simple code snippet demonstrating the use of a `for` loop in C++:
#include <iostream>
int main() {
for (int i = 0; i < 5; ++i) {
std::cout << "Hello, C++!" << std::endl;
}
return 0;
}
Integrated Development Environments (IDEs)
What is an IDE?
An Integrated Development Environment (IDE) is a comprehensive suite of tools that provides developers with an environment to write, test, and debug code. IDEs streamline the development process by integrating text editing, compiling, debugging, and project management functionalities into a single interface. In C++ development, using an IDE can significantly enhance productivity by providing features such as syntax highlighting, code completion, and integrated debugging tools.
Popular IDEs for C++
Microsoft Visual Studio
Microsoft Visual Studio is one of the most powerful IDEs available for C++ programming. It offers an extensive range of features, including:
- Smart Code Completion: Predicts the next code segment you might write.
- Integrated Debugging: Allows breakpoints, watch variables, and quick inspection.
Setting up Visual Studio is straightforward:
- Download and install from the [Microsoft Visual Studio website](https://visualstudio.microsoft.com/).
- Select “Desktop development with C++” during installation.
Here’s a simple example of creating a “Hello World” program in Visual Studio:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Pros: Versatile toolset, great debugging features, robust community support.
Cons: Resource-intensive, can be overwhelming for beginners.
Code::Blocks
Code::Blocks is another popular choice among C++ developers. It’s open-source and highly customizable. Key features include:
- Multi-compiler Support: Can work with various compilers (GCC, MSVC, etc.).
- Customizable Interface: Users can arrange panels according to their working style.
Installation steps for Code::Blocks:
- Download the installer from the [Code::Blocks website](http://www.codeblocks.org/).
- Choose the version that includes MinGW for a complete setup.
Sample code to print "Hello from Code::Blocks":
#include <iostream>
int main() {
std::cout << "Hello from Code::Blocks!" << std::endl;
return 0;
}
Pros: Lightweight, good documentation, simple user interface.
Cons: Limited features compared to other IDEs like Visual Studio.
CLion
Developed by JetBrains, CLion stands out for its intelligent code assistance, refactoring capabilities, and seamless integration with CMake.
Key features include:
- Smart code analysis to catch errors in real-time.
- Integrated terminal and version control.
To set up CLion:
- Download from the [JetBrains website](https://www.jetbrains.com/clion/).
- Configure CMake settings for your project.
Here’s a quick example for a “Hello CLion” program:
#include <iostream>
int main() {
std::cout << "Hello from CLion!" << std::endl;
return 0;
}
Pros: Powerful code analysis, supports various C++ standards, strong refactoring tools.
Cons: Requires a subscription for the latest features.
Comparing IDEs
When selecting an IDE for C++, consider comparing:
- Performance: How well it runs on your system.
- Ease of use: User interface and learning curve.
- Supported platforms: Compatibility with your operating system.
- Cost considerations: Whether it’s free, open-source, or requires a subscription.
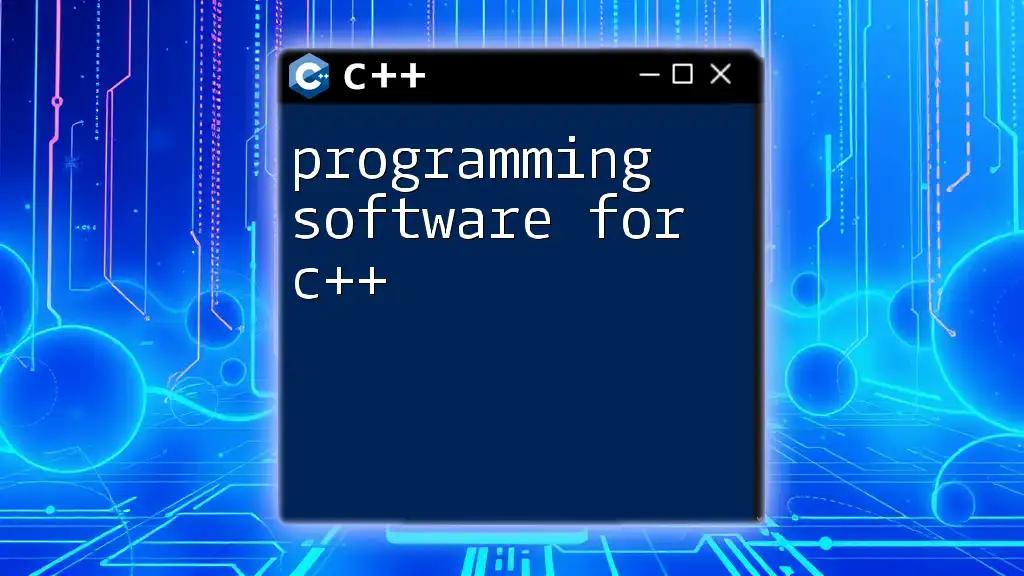
Text Editors for C++
What is a Text Editor?
Text editors are programs designed to edit plain text, and while they lack the integrated features of IDEs, they can be lighter and faster for quick edits and simple coding tasks. They are particularly useful for those who prefer a minimalistic approach or need to make quick adjustments to their code.
Popular Text Editors
Visual Studio Code
Visual Studio Code is a lightweight, yet powerful editor favored by many C++ developers due to its extensive extension ecosystem. With the C/C++ extension installed, you can enjoy:
- Syntax highlighting and IntelliSense.
- Integrated terminal and Git version control.
To create a simple C++ application:
#include <iostream>
int main() {
std::cout << "Hello from Visual Studio Code!" << std::endl;
return 0;
}
Pros: Very lightweight, customizability through extensions, strong community support.
Cons: Requires additional setup for full C++ support.
Sublime Text
Sublime Text is known for its speed and efficiency. Its features—like multiple selections, command palette, and custom themes—make it a favorite among programmers.
For C++ development, you can use the following sample code:
#include <iostream>
int main() {
std::cout << "Hello from Sublime Text!" << std::endl;
return 0;
}
Pros: Fast, smooth performance, highly customizable.
Cons: Some features behind a one-time license fee.
Atom
Atom is an open-source text editor with a strong focus on hackability. The Teletype feature allows real-time collaboration with other developers.
Here’s a simple “Hello Atom” C++ program:
#include <iostream>
int main() {
std::cout << "Hello from Atom!" << std::endl;
return 0;
}
Pros: Community-driven packages, suitable for collaborative coding.
Cons: Can be slow for bigger projects compared to IDEs.
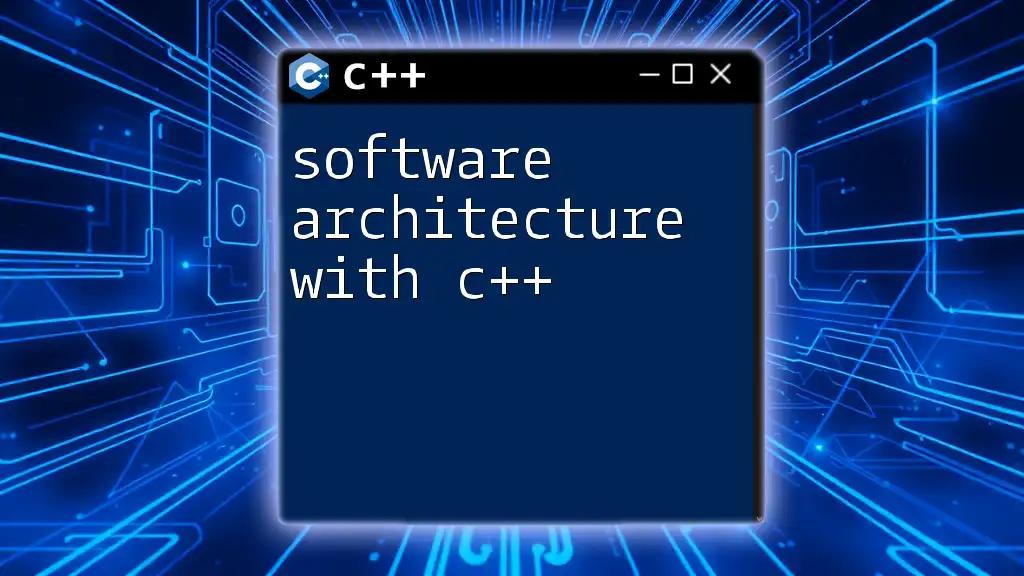
Compilers for C++
What is a Compiler?
A compiler translates your C++ source code into executable programs that your computer can run. Every C++ development environment requires a compiler, and the choice of which to use can affect performance and compatibility.
Popular C++ Compilers
GCC (GNU Compiler Collection)
GCC is a widely used compiler that supports multiple programming languages, including C++. It's powerful and available across various platforms.
Installation can be done through package managers or downloaded from the [GCC website](https://gcc.gnu.org/). Here’s how you compile a C++ program with GCC:
g++ -o hello hello.cpp
Pros: Open-source, cross-platform, highly optimized.
Cons: Command-line interface may be intimidating for beginners.
Clang
Clang is another open-source compiler, known for its modern architecture and focus on performance. It integrates seamlessly with various IDEs and has great support for C++ standards.
To compile a C++ file with Clang, use:
clang++ -o hello hello.cpp
Pros: Fast compilation, excellent diagnostics, modern features.
Cons: Availability on some older platforms may be limited.
Microsoft Visual C++ (MSC++)
MSC++ is the C++ compiler from Microsoft, primarily used with Visual Studio. It provides great support for Windows applications and excellent optimization features.
To compile a program using MSC++, the command might look like this in the Visual Studio Developer Command Prompt:
cl hello.cpp
Pros: Optimized for Windows, tight integration with Visual Studio.
Cons: Windows-only, licensing issues for commercial use.
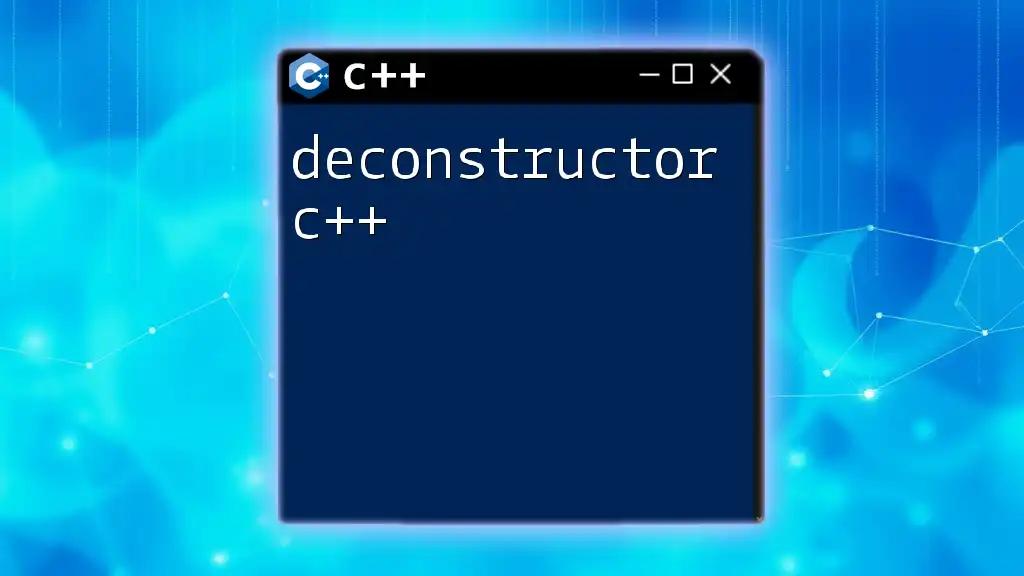
Debuggers for C++
Importance of Debugging
Debugging is a crucial phase in the development cycle, allowing programmers to identify and fix bugs or issues in their code. An effective debugger can provide insights into the program's execution, making it easier to analyze and understand runtime errors.
Popular Debugging Tools
GDB (GNU Debugger)
GDB is one of the most popular debugging tools for C++. It supports various platforms and can handle both local and remote debugging. With GDB, you can set breakpoints, inspect variables, and step through code.
A simple GDB session might look like this:
gdb ./hello
(gdb) run
(gdb) break main
(gdb) next
Pros: Powerful and flexible, supports remote debugging.
Cons: Command-line interface can be challenging for beginners.
LLDB
LLDB is part of the LLVM project and serves as an alternative to GDB. It provides similar functionalities but is optimized for modern C++ development, with better support for debugging C++11 and newer standards.
A GDB-like session in LLDB might look like this:
lldb ./hello
(lldb) run
(lldb) break set -n main
(lldb) next
Pros: More user-friendly than GDB, deep integration with LLVM tools.
Cons: Some features may be less mature compared to GDB.
Built-in Debuggers in IDEs
Most modern IDEs come with built-in debugging tools that offer features like variable inspection, call stack views, and live code editing, making debugging in an IDE intuitive and user-friendly.
In Visual Studio, for instance, you can easily set breakpoints and step through your code using a graphical interface.
Comparison: When selecting a debugger, consider ease of use, integration with your IDE, and community support.
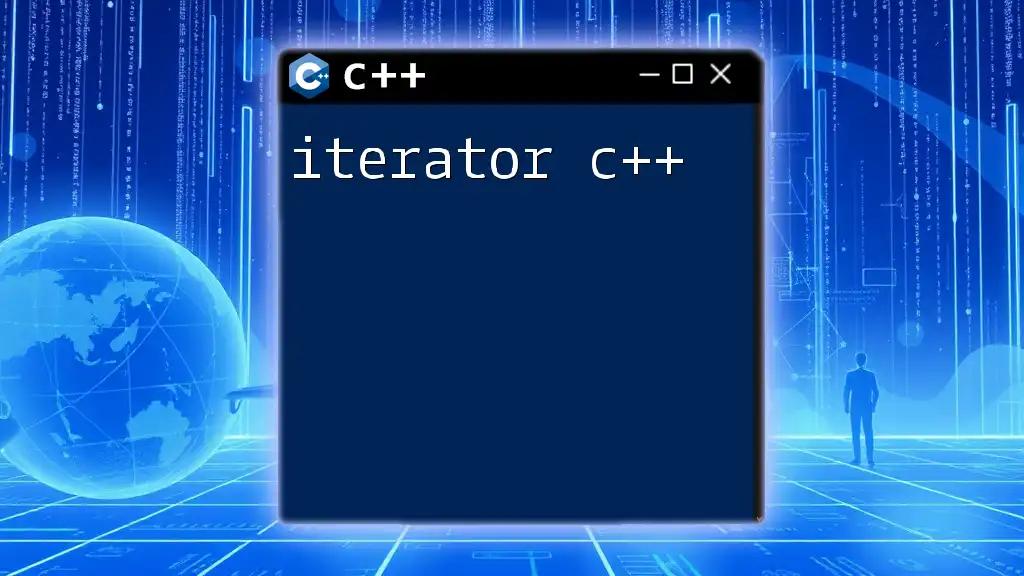
Version Control Software
Importance of Version Control
Version control systems track changes to code over time, helping developers manage and collaborate on projects efficiently. They enable teams to work simultaneously, keep a history of changes, and revert back to previous versions if necessary.
Popular Version Control Systems
Git
Git is the most widely used version control system in C++ development. Its decentralized nature means that every developer has a complete copy of the repository, allowing for flexible workflows.
Basic commands include:
git init # Initialize a new repository
git add . # Stage changes
git commit -m "message" # Commit changes
git push origin master # Push changes to a remote repository
Pros: Fast operations, robust branching and merging capabilities, strong community support.
Cons: Initial learning curve for beginners.
Subversion (SVN)
SVN is a centralized version control system that is still relevant for certain projects. It maintains a single central repository and allows users to check out files.
Basic SVN commands:
svn checkout URL # Check out a repository
svn commit -m "message" # Commit changes
Pros: Simplified model for projects, good scalability.
Cons: Less flexible than Git, single point of failure.
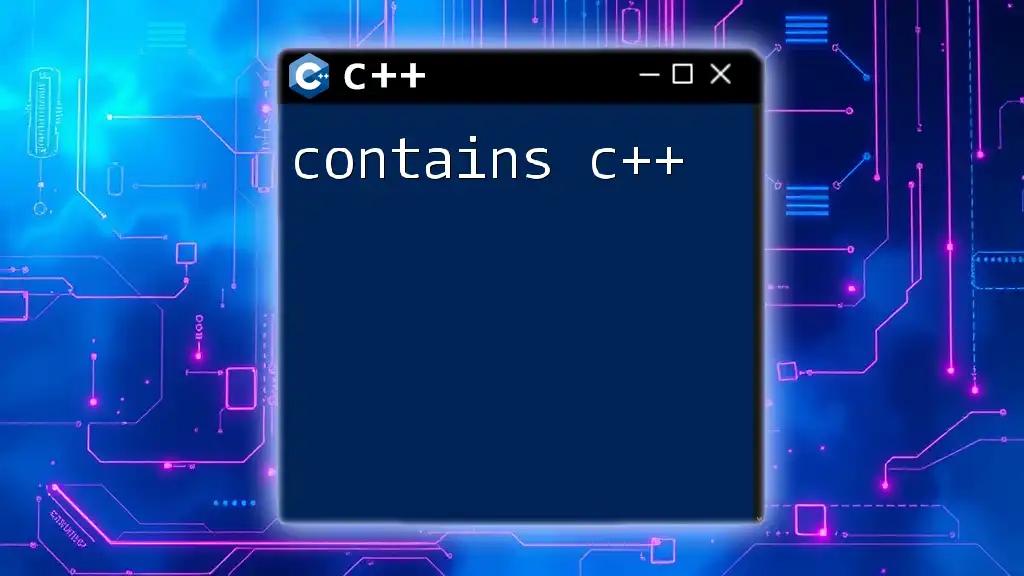
Build Automation Tools
Significance of Build Tools
Build automation tools help automate the tasks involved in building C++ applications, such as compiling source code, linking libraries, and packaging. Automating these processes can save significant time and reduce errors in larger projects.
Popular Build Automation Software
CMake
CMake is a powerful cross-platform build system that generates standard build files. It’s particularly useful for managing complex projects with multiple components.
Here’s an example `CMakeLists.txt` configuration for a simple project:
cmake_minimum_required(VERSION 3.10)
project(HelloWorld)
add_executable(helloworld main.cpp)
To build the project, navigate to the directory and run:
mkdir build
cd build
cmake ..
make
Pros: Cross-platform, handles complex projects with ease.
Cons: Configuration can be complex for simple projects.
Make
Make is one of the oldest build automation tools and is still widely used. It uses a simple `Makefile` that defines how to compile and link the program.
An example `Makefile` might look like this:
all: helloworld
helloworld: main.cpp
g++ -o helloworld main.cpp
clean:
rm helloworld
To build your project, simply run:
make
Pros: Simple for small projects, widely supported.
Cons: Can become complex for larger projects.
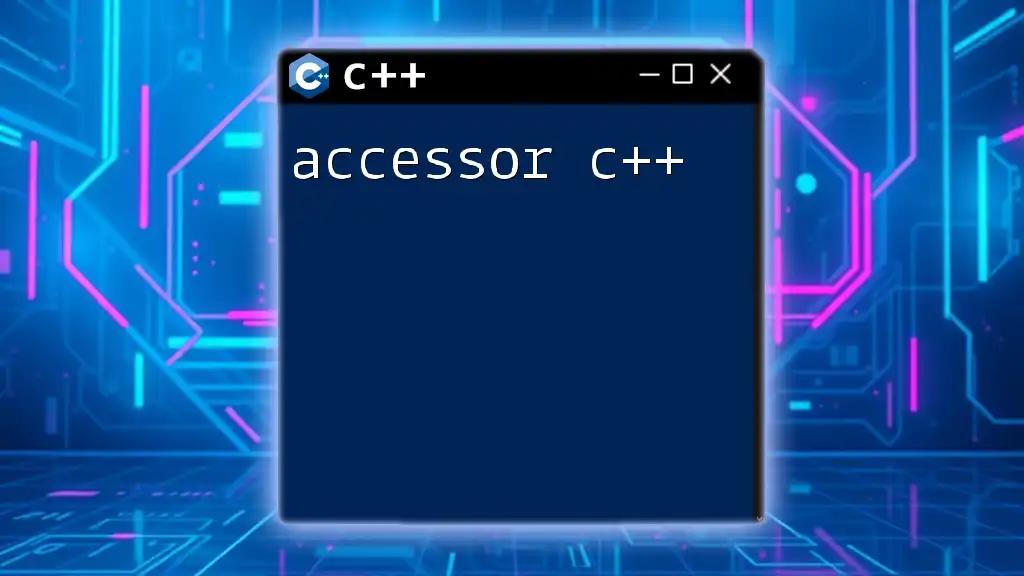
Conclusion
Choosing the right software tools for C++ development can significantly enhance productivity and coding efficiency. Whether you prefer a feature-rich IDE, a lightweight text editor, or any combination of tools, understanding their capabilities and limitations is key to successful programming.
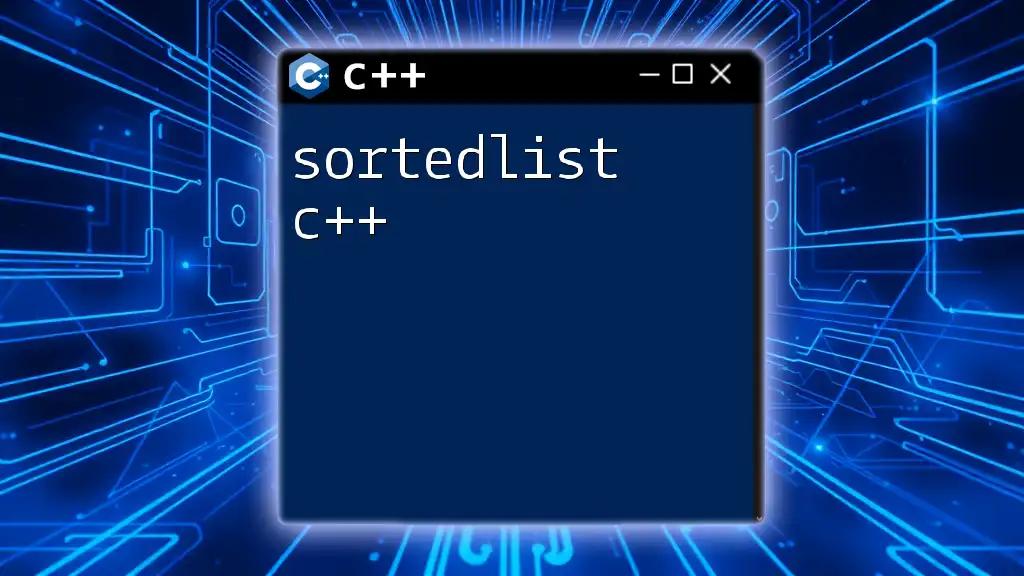
Call to Action
Explore the tools and resources mentioned in this guide and find what fits your workflow best. Don’t hesitate to provide feedback or share your experiences with different software for C++. Your input helps create a dynamic and supportive community for C++ developers!
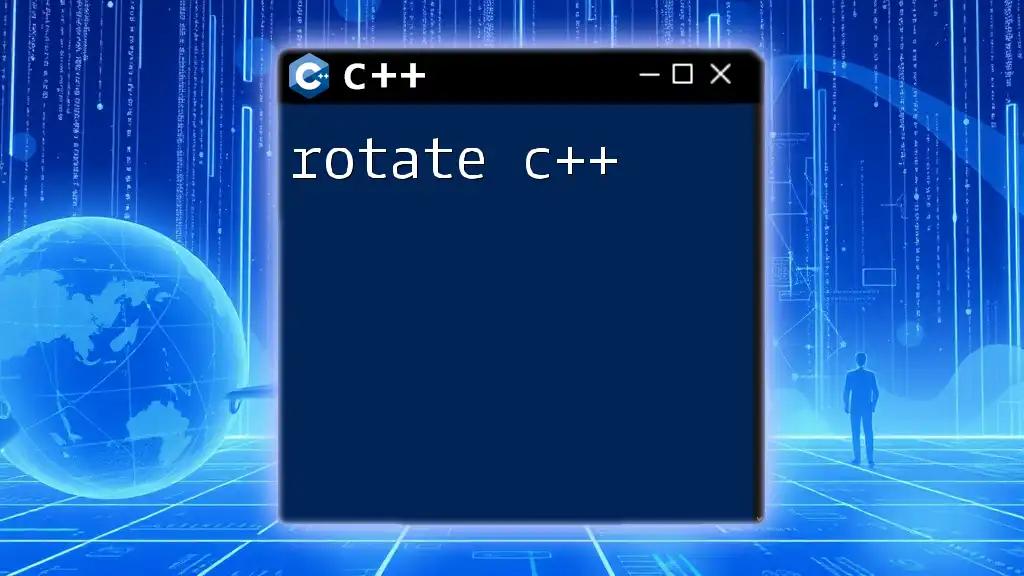
Additional Resources
For further learning, explore official documentation for the software presented, recommended books on C++ programming, and join online forums to engage with other developers.