The bitwise NOT operator (`~`) in C++ inverts the bits of its operand, changing all 0s to 1s and all 1s to 0s.
#include <iostream>
int main() {
int num = 5; // In binary: 0000 0101
int result = ~num; // In binary: 1111 1010 (which is -6 in two's complement)
std::cout << "The bitwise NOT of " << num << " is " << result << std::endl;
return 0;
}
What is the Bitwise NOT Operator?
The Bitwise NOT operator is a unary operator that inverts the bits of its operand. In simpler terms, it changes every `1` to `0` and every `0` to `1`. This operator is an essential part of bitwise operations in C++, which allow for sophisticated manipulations of binary data. The Bitwise NOT operator is represented by the tilde symbol (`~`).
Unlike logical operations which evaluate conditions and return boolean values, bitwise operations work directly with the binary representations of integers.
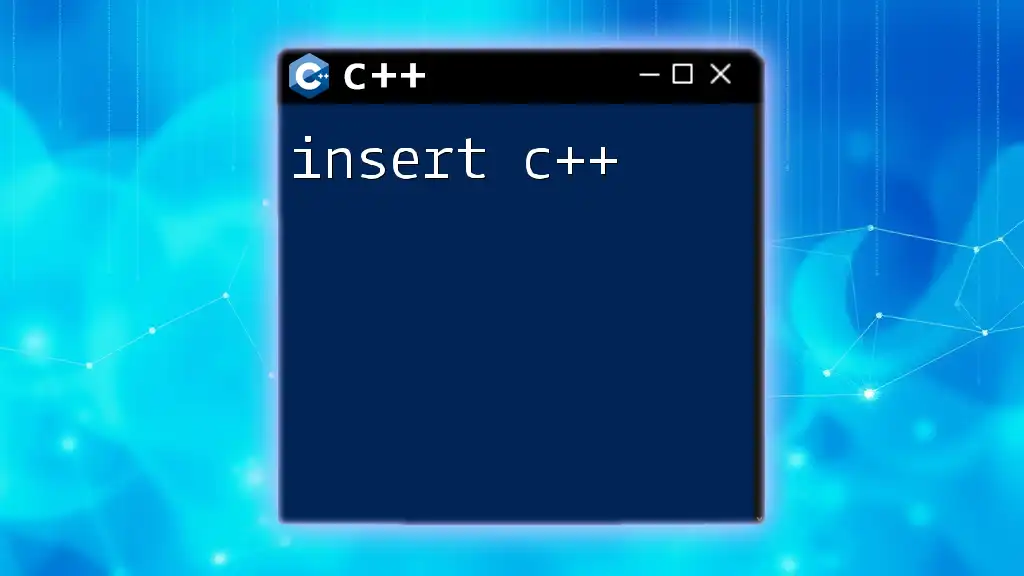
How to Use the Bitwise NOT Operator in C++
The syntax for using the Bitwise NOT operator is straightforward. Here’s how it appears in code:
int a = 5; // Binary: 00000000 00000000 00000000 00000101
int result = ~a; // The result is derived after inverting the bits
In this example, when `a` (which is `5` in decimal) is represented in binary, its value is `00000000 00000000 00000000 00000101`. By applying the Bitwise NOT operator, we get:
result = ~00000000 00000000 00000000 00000101
= 11111111 11111111 11111111 11111010 // Inverted bits
The result is `-6` in decimal, which, when viewed as a signed integer, represents the two's complement of the inverted binary value. When considering signed versus unsigned integers, the interpretation differs, so it's essential to understand the context in which you are working.
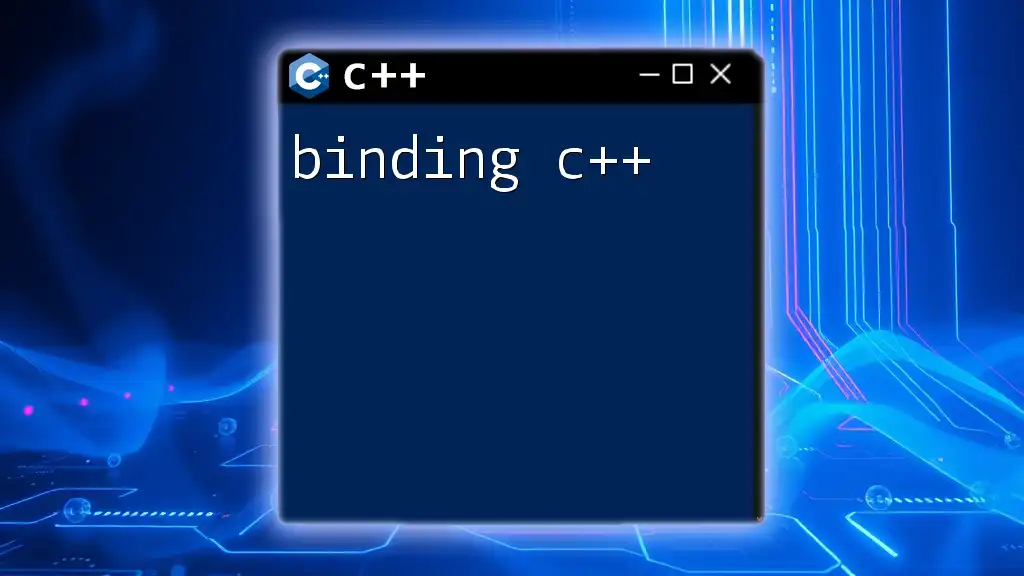
Practical Applications of Bitwise NOT
Data Manipulation
In programming, you often need to perform precise manipulations of data. The Bitwise NOT operator is valuable when you need to invert specific bits in your data. For example, if you receive a data packet where certain bits signify flags (on/off states), using Bitwise NOT can easily toggle these flags.
Imagine a scenario in a graphical user interface where you have a list of enabled features represented by bits. Applying Bitwise NOT can modify these settings efficiently without needing complex structures.
Flags and Masks in Programming
Flags and masks are commonly used in programming for efficient state management. A flag is a binary variable that can be turned on or off (1 or 0). Masks are used in conjunction with bitwise operations to isolate certain bits.
Here’s a practical example that demonstrates how to use Bitwise NOT with flags:
int flags = 0b1110; // Current flag state: first three features on
flags = ~flags; // Flip all flag states
After the operation, if the original flags were `1110`, the new flag state becomes `0001`. This operation is crucial when toggling states, ensuring the system reflects the correct status.
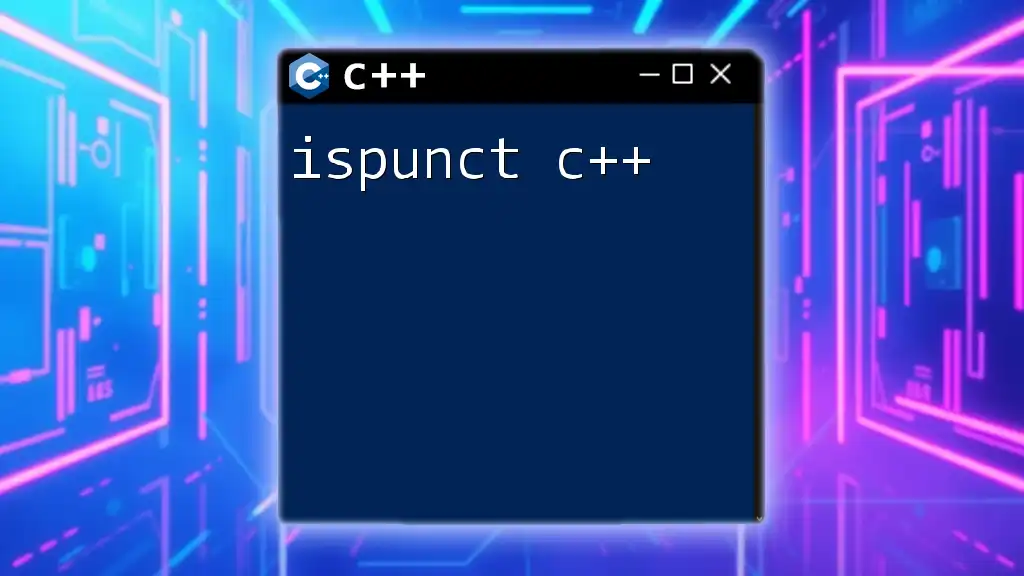
Bitwise NOT with Negative Numbers
When dealing with negative numbers, the behavior of Bitwise NOT can be uniquely insightful due to how negative integers are represented in binary, primarily using two's complement notation.
Consider the following example:
int b = -3; // Binary representation: 11111111 11111111 11111111 11111101
int not_b = ~b; // Resultant operation
In this case, applying the Bitwise NOT operator results in:
not_b = ~11111111 11111111 11111111 11111101
= 00000000 00000000 00000000 00000010
The result is `2`, illustrating that when you use Bitwise NOT on a negative number, it effectively recalibrates the sign through inversion.
Understanding the nuances of how Bitwise NOT interacts with negative values forms a cornerstone of effective low-level programming.
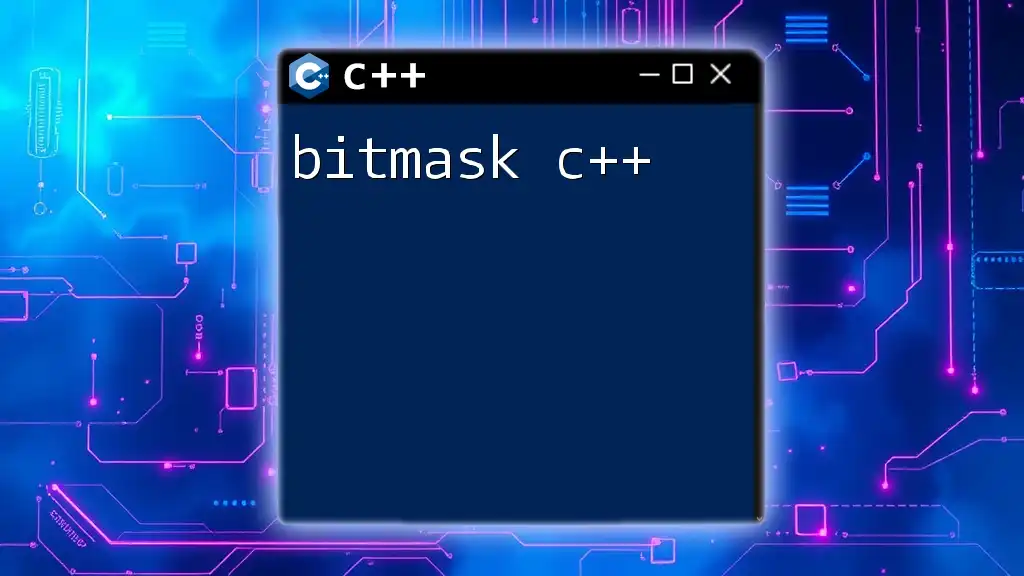
Common Mistakes and Debugging Tips
When working with bitwise operations, developers often encounter several common pitfalls. These include:
- Misunderstanding the output: It's vital to recognize that the output can differ based on whether you are dealing with signed or unsigned data types. Always check the data type's behavior in C++.
- Neglecting parentheses: When combining multiple bitwise operations in an expression, use parentheses to ensure the correct order of operations.
- Mismatch with decimal values: Results from bitwise operations may not yield intuitive decimal outputs. Familiarize yourself with binary representations to grasp results better.
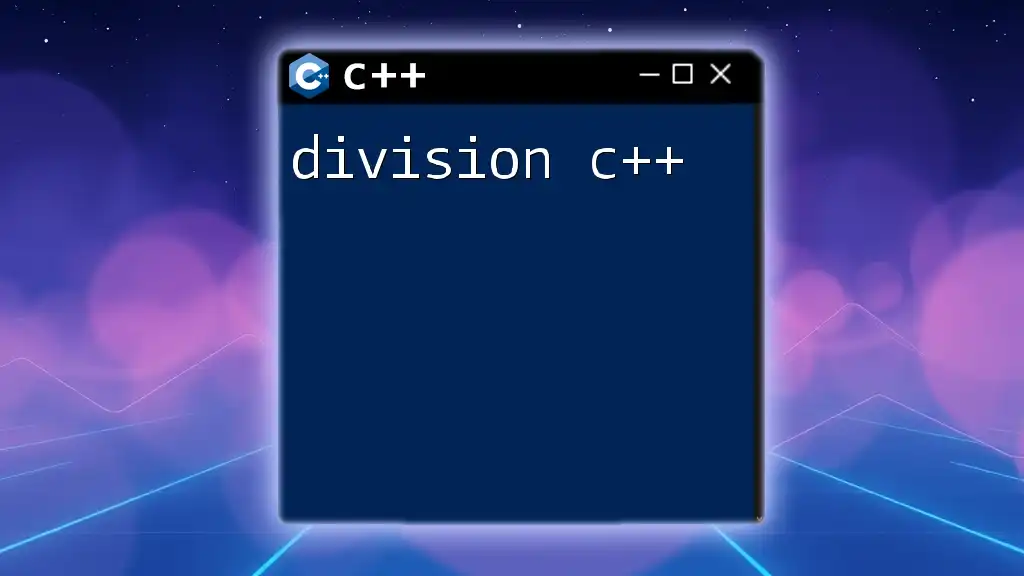
Conclusion
In summary, the Bitwise NOT operator in C++ is a powerful tool for manipulating binary data. Understanding how to invert bits allows you to engage with data at a fundamental level, offering solutions for various programming challenges. As you explore bitwise operations, consider using the Bitwise NOT operator to create efficient algorithms and improve the performance of your applications.
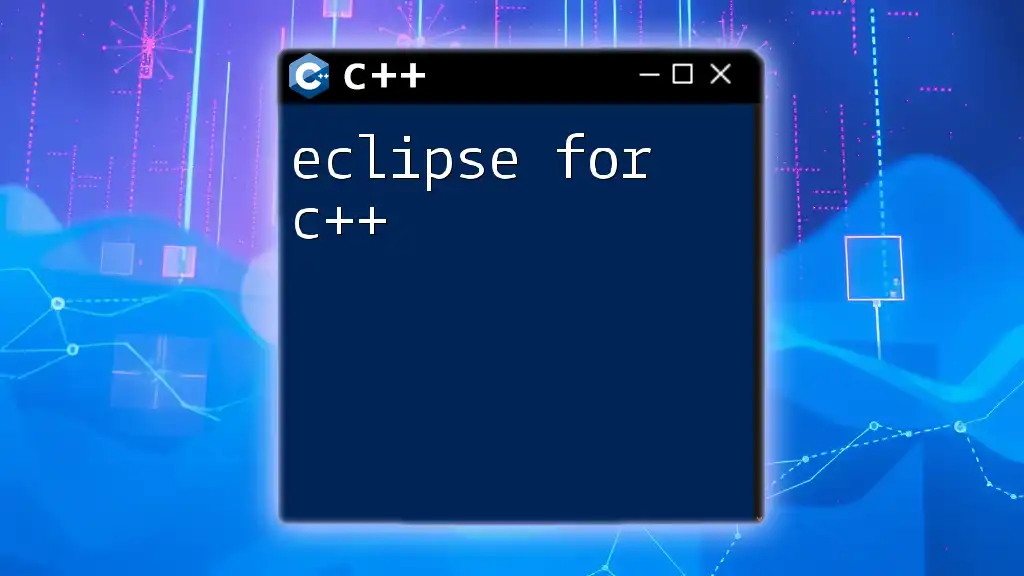
Additional Resources
For hands-on practice, try using online compilers that support C++ code execution to experiment with the Bitwise NOT operator. As you dive deeper into C++, don't hesitate to explore further reading on bitwise operations, as they are an essential part of any seasoned programmer’s toolkit.
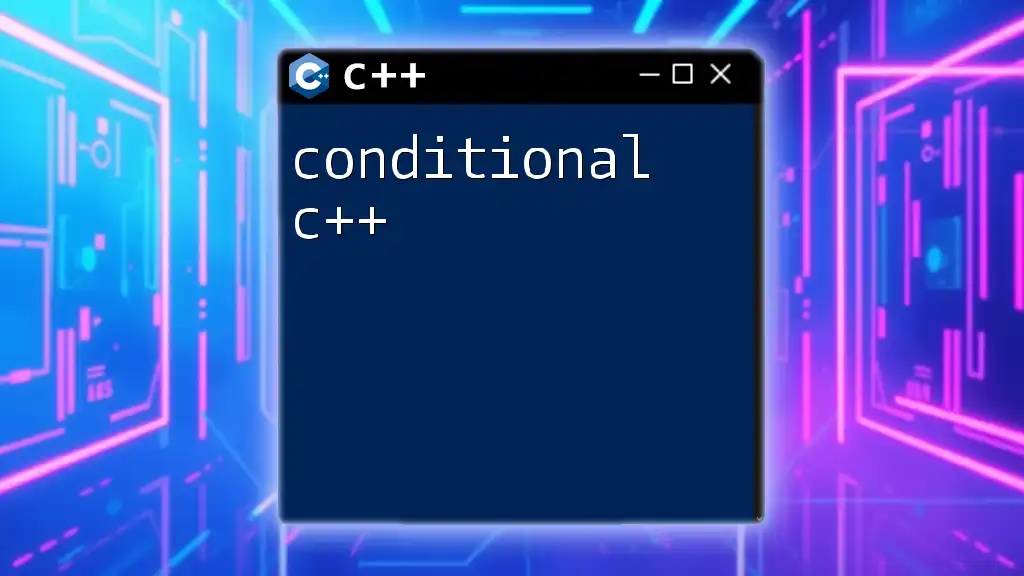
FAQs about Bitwise NOT in C++
-
What happens when you apply Bitwise NOT to a number?
Applying Bitwise NOT flips each bit of the number. Hence, each `1` becomes `0` and each `0` becomes `1`. -
How is Bitwise NOT different from logical NOT?
Bitwise NOT operates on the binary representation of numbers, while logical NOT evaluates boolean values. Bitwise NOT is used for manipulation of bits, while logical NOT is used for logical evaluations. -
Can Bitwise NOT be used with floating-point numbers?
No, Bitwise NOT is only applicable to integer types, as it operates at the bit level. To manipulate floating-point numbers, consider converting them to integer representations first.