"Starting out with C++ 10th Edition provides beginners with a foundational understanding of programming concepts through clear examples and practical exercises, making it easier to grasp essential C++ commands."
Here's a simple code snippet that demonstrates basic input and output in C++:
#include <iostream>
int main() {
std::cout << "Hello, world!" << std::endl;
return 0;
}
Getting Started with C++ 10th Edition
Understanding the Basics of C++
What is C++?
C++ is a powerful general-purpose programming language that has been widely used for system/software development, game development, and embedded systems. It was initially developed as an extension of the C programming language and supports both procedural and object-oriented programming paradigms.
Why Learn C++?
Learning C++ can provide several career advantages. It is a foundational language in computer science and engineering, and many jobs in software development, game design, and systems programming require knowledge of C++. Moreover, C++ offers a blend of low-level capabilities and high-level abstractions, which makes it versatile for various applications.
Key Features of C++
One of the standout features of C++ is its support for Object-Oriented Programming (OOP) principles. This includes encapsulation, inheritance, and polymorphism, which help in organizing code and reusing it efficiently. C++ also allows low-level memory manipulation, giving programmers control over system resources.
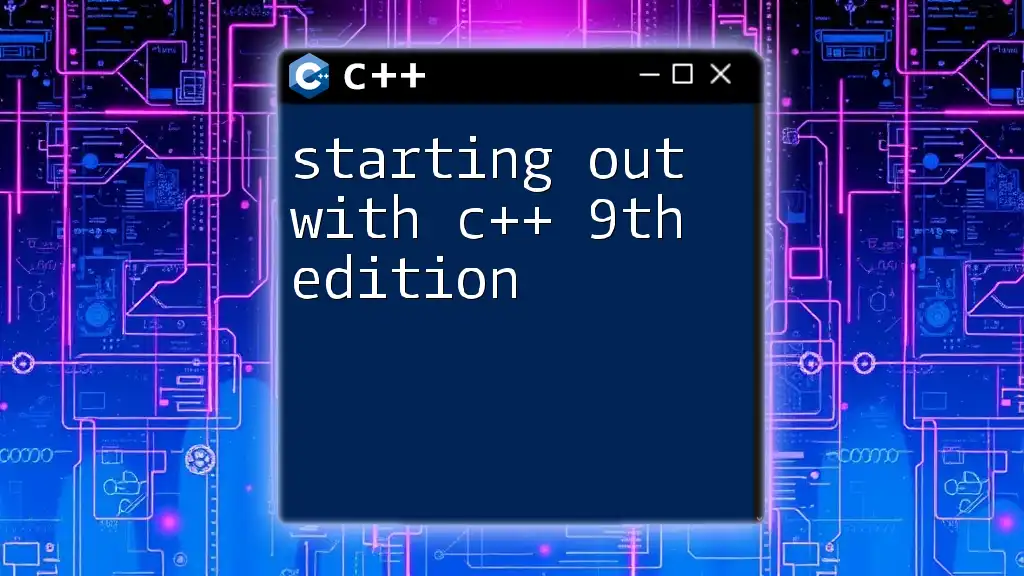
Setting Up Your Environment
Choosing an IDE and Compiler
Popular IDEs for C++
When starting out with C++ 10th edition, choosing the right Integrated Development Environment (IDE) can greatly enhance your coding experience. Some of the most popular IDEs for C++ include:
- Visual Studio: A feature-rich IDE ideal for Windows.
- Code::Blocks: A free, open-source IDE that is lightweight and supports multiple compilers.
- Eclipse: An extensible IDE that supports C/C++ development through the CDT (C/C++ Development Tooling) plugin.
Setting Up Your Development Environment
Installing the IDE typically involves downloading the installer from its official website and following the on-screen instructions. Whether you are using Windows, macOS, or Linux, the installation processes are fairly straightforward. After installation, configuring the environment for C++ development usually involves selecting the appropriate compiler (like GCC or Clang) and setting up a project folder.
Writing Your First Program
Hello, World! Example
Once your environment is set up, you can write your first C++ program. The classic "Hello, World!" program serves as the best starting point:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Explanation of the Code
- `#include <iostream>` is a directive that includes the Input/Output stream library, which enables the use of std::cout for outputting text to the console.
- The `main()` function is the starting point of every C++ program. This is where execution begins, and it returns an integer value (typically 0) to indicate successful completion.
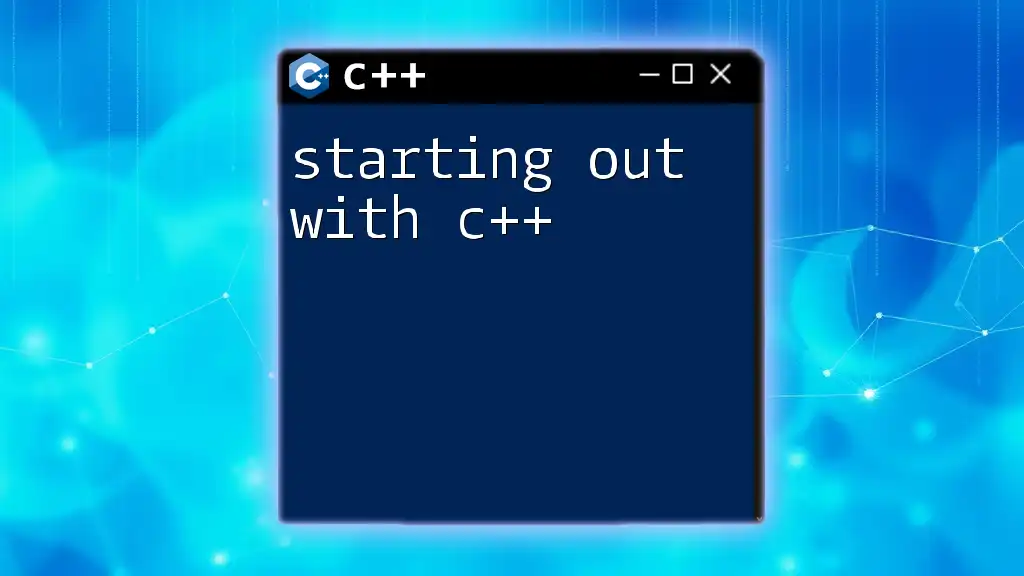
Fundamental Concepts in C++
Data Types and Variables
Understanding Data Types
C++ supports a rich variety of data types that programmers can use, including:
- Primitive Data Types: These include `int`, `float`, `double`, `char`, and `bool`. Each datatype has different properties and usage scenarios.
- User-defined Data Types: C++ allows for the creation of custom data types, such as structs and enums, enabling better organization and structure of code.
Declaring and Initializing Variables
Declaring variables in C++ is crucial as it defines their data type and initializes them as needed. For example:
int age = 25;
float salary = 50000.5;
char initial = 'A';
This snippet demonstrates the declaration of an integer, a floating-point number, and a character variable. Emphasizing descriptive variable names is essential for code readability and maintainability.
Control Structures
Conditional Statements
Control structures like conditions enable decision-making in code. Here’s an example of an if-else statement:
if (age > 18) {
std::cout << "Adult";
} else {
std::cout << "Minor";
}
This structure evaluates the condition and executes the corresponding block of code based on the result, demonstrating how C++ handles logical flow.
Loops
C++ also provides several looping structures, including for, while, and do-while loops, which allow code to run repeatedly. For instance, a simple for loop can be illustrated as follows:
for (int i = 0; i < 5; i++) {
std::cout << i << " ";
}
This code will output the numbers 0 to 4, showcasing the simplicity of iterating through sequences of data.
Functions in C++
Defining and Calling Functions
Functions are reusable code blocks that can be defined and called whenever needed. Here’s a basic function example:
void greet() {
std::cout << "Hello, User!";
}
int main() {
greet();
return 0;
}
In this case, the function `greet()` outputs a greeting. The main function then calls it, demonstrating how functions work in C++.
Function Parameters and Return Values
Functions can also take parameters and return values, which make them more versatile. For example:
int add(int a, int b) {
return a + b;
}
This function accepts two integers, adds them, and returns the sum. Emphasizing type safety in function parameters is key in C++.
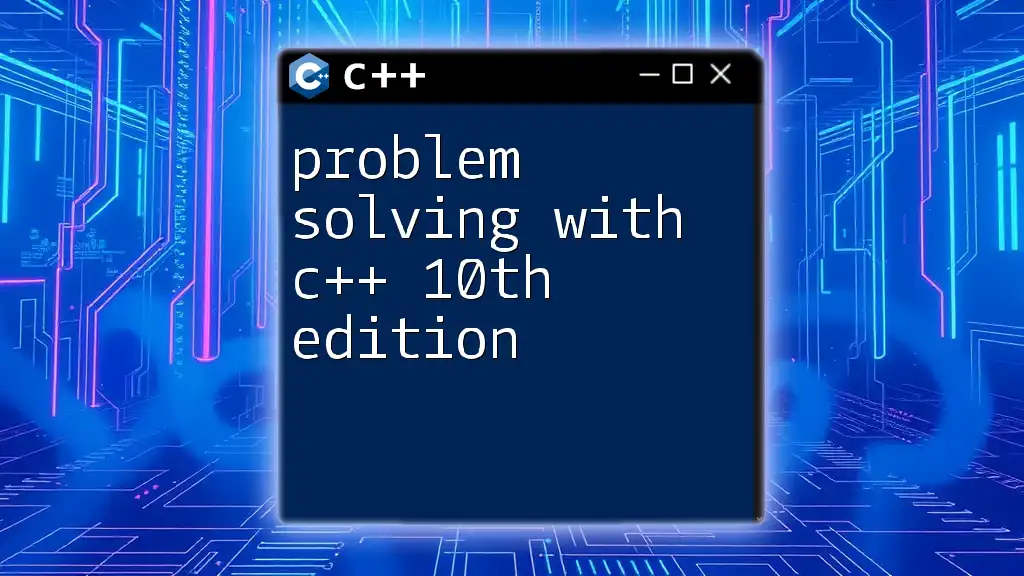
Object-Oriented Programming Concepts
Classes and Objects
What are Classes and Objects?
C++ supports Object-Oriented Programming (OOP), which revolves around the concepts of classes and objects. A class acts as a blueprint for creating objects, which are instances of classes.
Creating a Class
Here’s a simple example of a class definition:
class Car {
public:
std::string model;
void display() {
std::cout << "Car model: " << model << std::endl;
}
};
This `Car` class has a member variable `model` and a method `display()` that prints the model of the car. Using access specifiers (like `public`, `private`) ensures encapsulation, which is a core benefit of OOP.
Constructors and Destructors
Understanding Constructors
Constructors are special member functions called when an object of a class is instantiated. Here's how you can define a constructor in a class:
class Car {
public:
Car() {
std::cout << "Car created!" << std::endl;
}
~Car() {
std::cout << "Car destroyed!" << std::endl;
}
};
The constructor above displays a message upon object creation, while the destructor does the same when the object is destroyed. This usage enhances resource management in your applications.
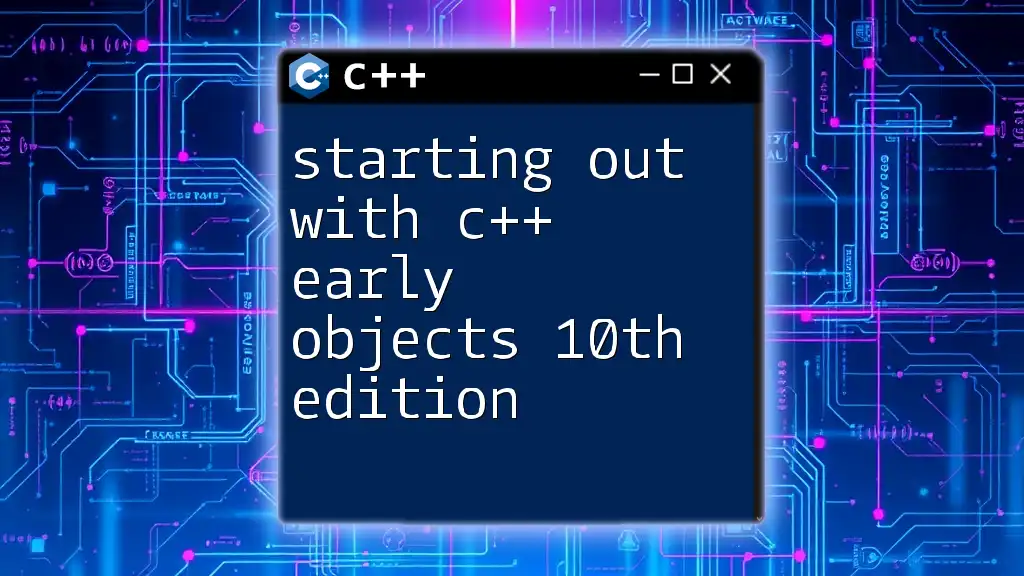
Advanced C++ Features
Templates and the Standard Template Library (STL)
Introduction to Templates
Templates allow functions and classes to operate with any data type. They make C++ extremely flexible and reusable. Here's a simple template function:
template <typename T>
T add(T a, T b) {
return a + b;
}
This function will work with any data type that supports the `+` operator.
Understanding STL
The Standard Template Library provides a collection of functionalities like algorithms and data structures (e.g., vectors, lists) that can simplify programming tasks. STL is efficient and helps in improving code reuse.
Exception Handling
Basics of Exception Handling
Exception handling allows your programs to respond gracefully to runtime errors. C++ provides constructs such as try, catch, and throw:
try {
throw std::runtime_error("An error occurred");
} catch (std::exception &e) {
std::cout << e.what();
}
The above code demonstrates how exceptions can be caught and handled, ensuring that programs do not terminate abruptly.
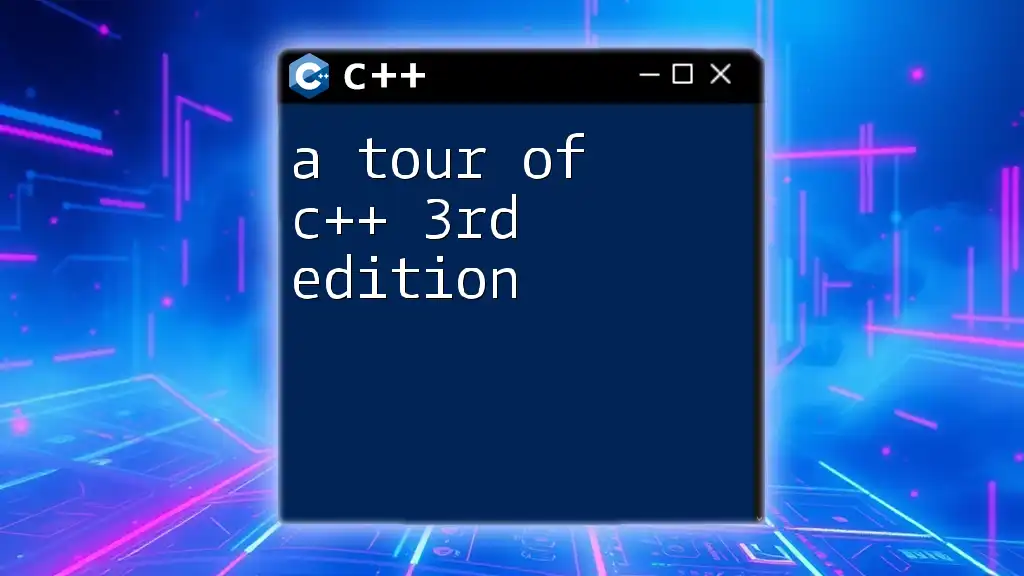
Best Practices for Writing C++ Code
Coding Standards
Adhering to coding standards is critical in C++. This includes:
- Writing readable and maintainable code.
- Utilizing consistent naming conventions for variables and functions.
- Adding comments to explain complex logic, which enhances future maintainability.
Debugging and Testing
Debugging tools integrated into your IDE can help identify and resolve issues efficiently. Furthermore, practicing unit testing in C++ using frameworks like Google Test can significantly improve code reliability and ensure that each component of your application functions as expected.
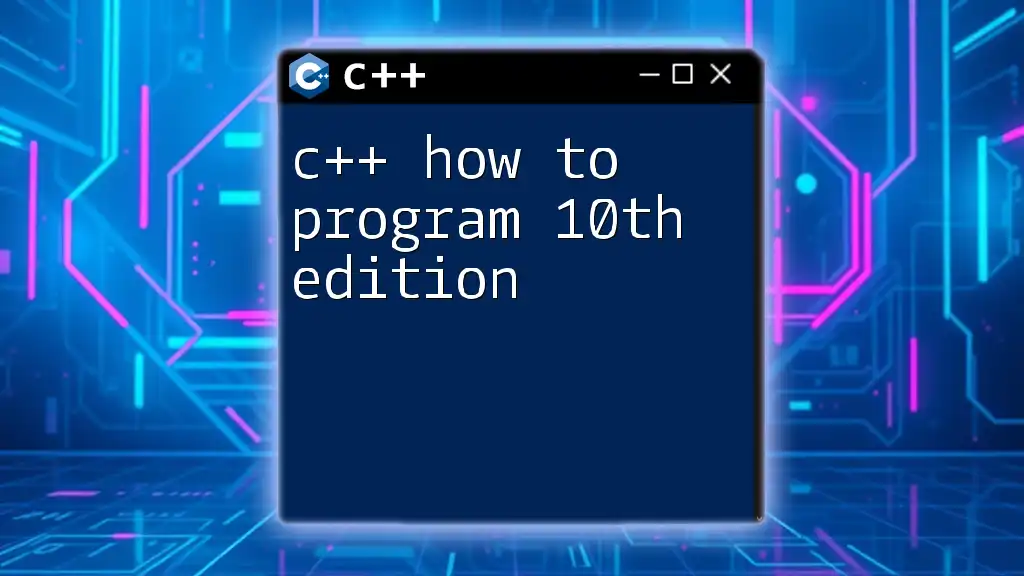
Resources for Learning C++
Recommended Books and Online Courses
To deepen your knowledge of C++, several resources are invaluable:
- Top Books for C++: "C++ Primer" and "Effective C++" are two highly recommended books that cover foundational and advanced concepts in detail.
- Online Learning Platforms: Websites like Coursera, Udemy, and edX offer excellent courses ranging from introductory to advanced C++ concepts.
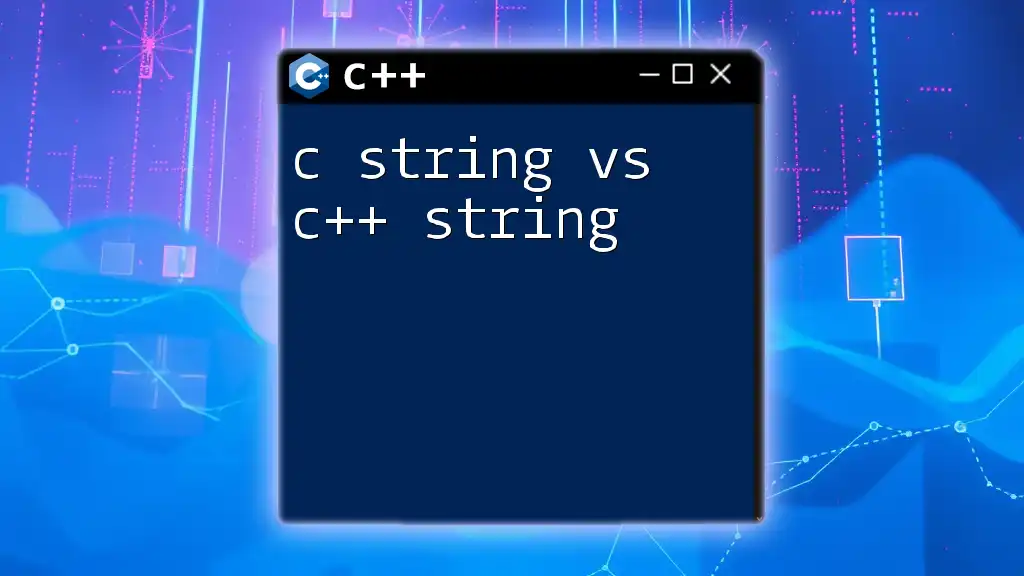
Conclusion
In summary, starting out with C++ 10th edition equips you with the skills necessary for a successful programming career. The language's blend of power, flexibility, and control makes it a pivotal tool for developers.
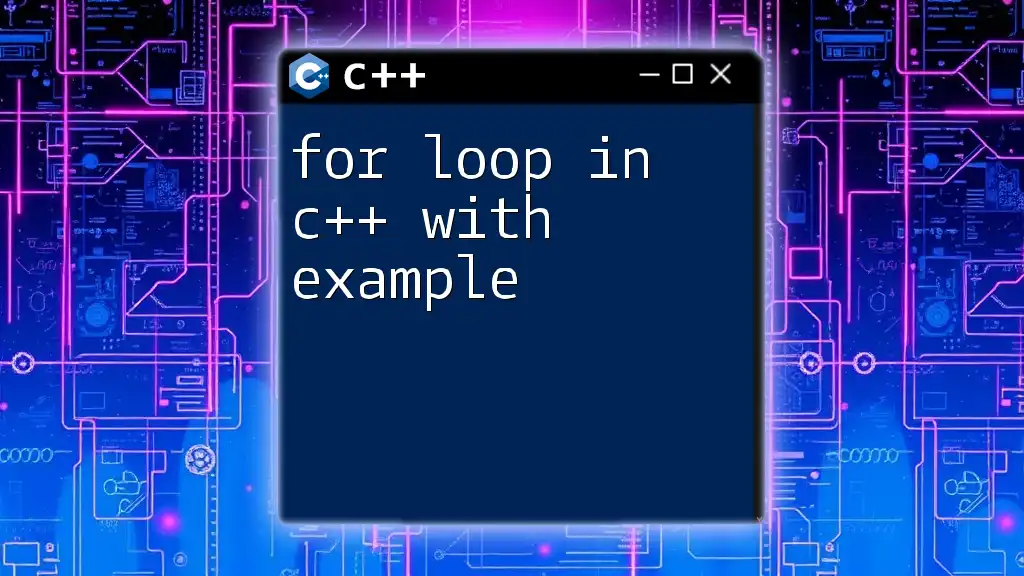
Call to Action
If you're interested in furthering your C++ knowledge and honing your skills, consider joining our C++ program. We offer comprehensive lessons and workshops tailored to help you master C++ effectively. Sign up for our newsletter to stay updated on upcoming sessions and resources.
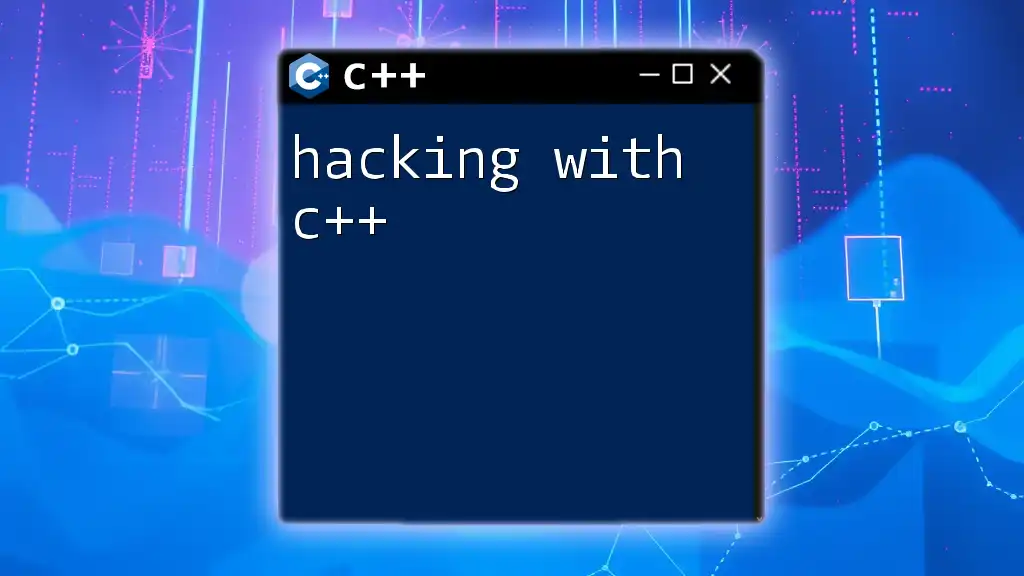
FAQs
Common Questions About Learning C++
-
Can I learn C++ without prior programming experience?
Yes! Many resources are designed for beginners and can help you grasp the fundamentals step by step. -
How long does it take to become proficient in C++?
Proficiency can vary; however, with consistent practice and study, you could gain a strong grasp in a few months.