C strings are arrays of characters terminated by a null character, while C++ strings are part of the Standard Template Library (STL) that provide more functionality and safety; for example, you can declare and manipulate them as follows:
#include <iostream>
#include <cstring> // For C strings
#include <string> // For C++ strings
int main() {
// C string
const char* cString = "Hello, C!";
// C++ string
std::string cppString = "Hello, C++!";
std::cout << cString << std::endl; // Output: Hello, C!
std::cout << cppString << std::endl; // Output: Hello, C++!
return 0;
}
Understanding C Strings
Definition of C Strings
A C string is a sequence of characters terminated by a null character (`\0`). It is essentially an array of characters, typically created and manipulated with primitive array operations. Due to this null-termination, functions from the C standard library can determine the end of the string.
Characteristics of C Strings
- Memory Management: One major aspect of C strings is manual memory management. Programmers must allocate and deallocate memory using functions like `malloc()` and `free()`, leading to potential memory leaks if not properly handled.
- Mutability: C strings can be modified, but this requires careful programming to avoid unsafe practices like buffer overflows, which can lead to security vulnerabilities.
Basic Syntax and Usage
Declaring a C string is straightforward. The syntax is as follows:
char myString[50]; // Declares an array of 50 characters
You can also initialize a C string like this:
char myString[] = "Hello, World!"; // Automatically allocating enough memory
To manipulate C strings, standard library functions are employed. For instance:
- `strcpy()` to copy strings,
- `strlen()` to find the length,
- `strcat()` to concatenate strings.
Here's how you might use these functions:
#include <cstring>
void exampleCSyntax() {
char dest[50];
char source[] = "Hello";
strcpy(dest, source); // Copying content from source to dest
strcat(dest, " World"); // Adding " World" to dest
}
Common Functions for C Strings
C string manipulation relies on a set of standard library functions defined in `<cstring>`. Some common functions include:
- `strlen()`: Returns the length of the C string.
- `strcpy()`: Copies one C string to another.
- `strcat()`: Concatenates two C strings.
Using these functions is essential for effective manipulation and management of C strings.
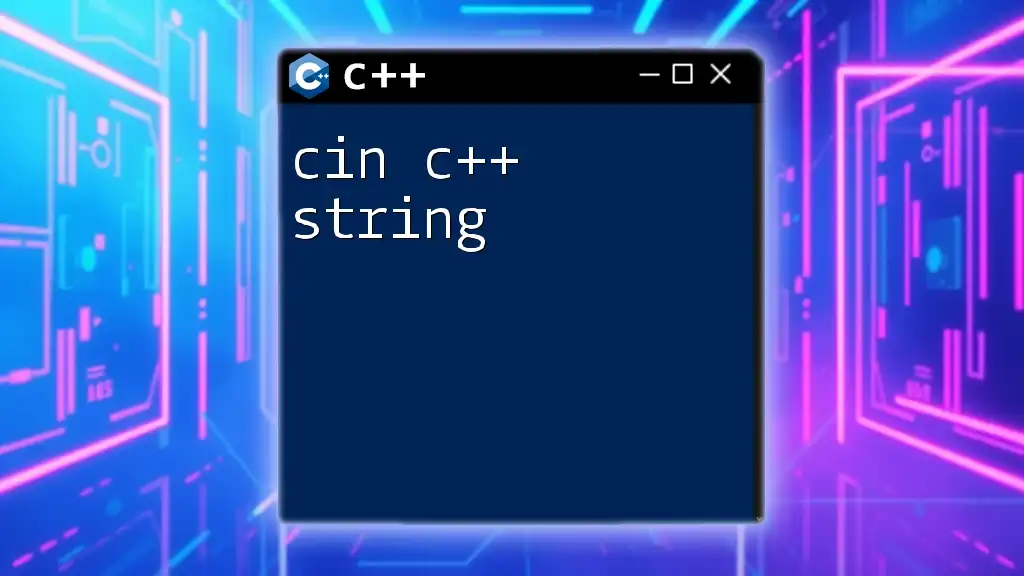
Understanding C++ Strings
Definition of C++ Strings
C++ strings, defined in the `<string>` library, provide a high-level, user-friendly way to manage and manipulate strings, abstracting away the complexities of memory management that C strings require.
Characteristics of C++ Strings
- Dynamic Memory Management: C++ strings automatically manage memory allocation and deallocation as strings grow or shrink, simplifying coding and reducing potential errors.
- Safety: C++ strings inherently guard against many common programming errors associated with C strings, such as buffer overflows, making them safer to use.
Basic Syntax and Usage
Declaring a C++ string is done simply as follows:
std::string cppString; // Default initialization
You can initialize and assign values like this:
std::string cppString = "Hello, World!";
Modifying C++ strings is straightforward. You might use functions such as:
- `append()` to add characters,
- `substr()` to extract sections of strings.
Here's an example of C++ string operations:
#include <string>
void exampleCPPSyntax() {
std::string cppString = "Hello";
cppString.append(" World"); // Appending " World" to cppString
std::string sub = cppString.substr(0, 5); // Extracting "Hello"
}
Common Functions for C++ Strings
C++ strings provide a rich set of member functions for string manipulation:
- `length()`: Returns the number of characters in the string.
- `append()`: Adds more characters to the end of the string.
- `find()`: Locates a substring within the string.
These functions make working with strings in C++ intuitive and powerful, allowing for complex string manipulations with minimal code.
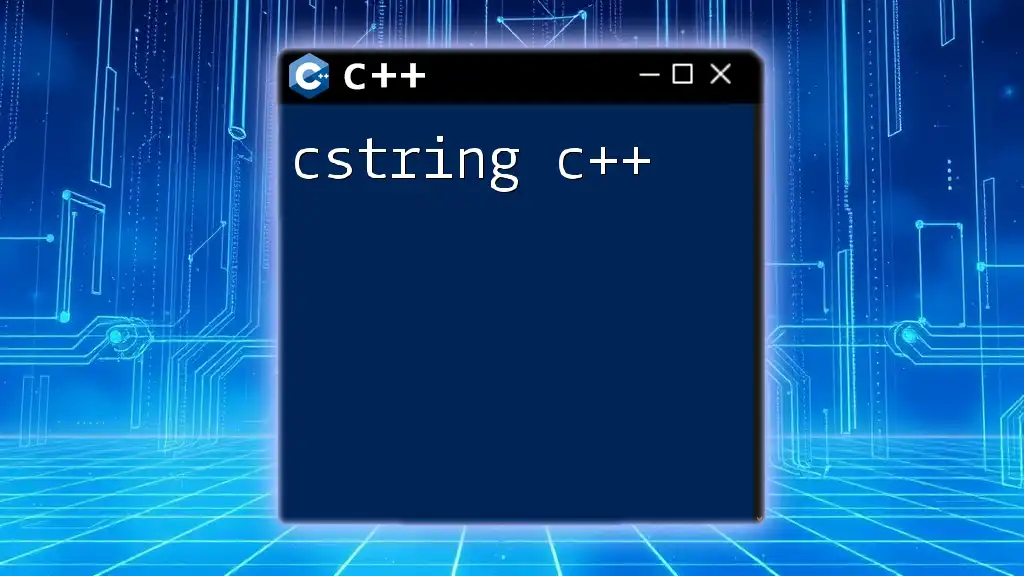
Key Differences Between C Strings and C++ Strings
Memory Management
The stark contrast between C strings and C++ strings lies in memory management. C strings require manual allocation/deallocation, increasing the chance of memory leaks. In contrast, C++ strings handle this automatically, reducing the risk of memory-related errors.
Safety and Error Handling
C++ strings are inherently safer. With automatic size adjustments and bounds checking, they help avoid common pitfalls in C string operations, like overwriting allocated memory.
Performance Considerations
In terms of performance, C strings might have an edge in certain low-level applications due to their straightforward implementation and the absence of overhead found in complex data structures. However, C++ strings offer better abstraction and ease of use, which can lead to faster development times and less bug-prone code.
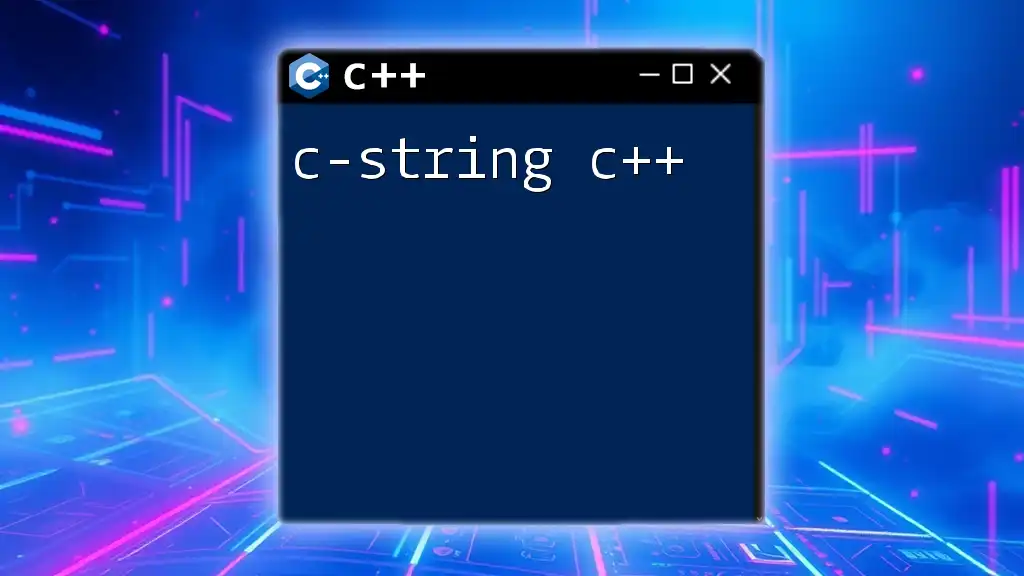
When to Use Each
Use Cases for C Strings
Despite the advantages of C++ strings, C strings are still relevant, especially when working with older C libraries or when performance is critical in specific situations, such as embedded systems.
Use Cases for C++ Strings
C++ strings are preferable in modern software development, where code maintainability, safety, and product development speed are prioritised. They suit applications that make extensive use of string operations, such as text processing and data management.
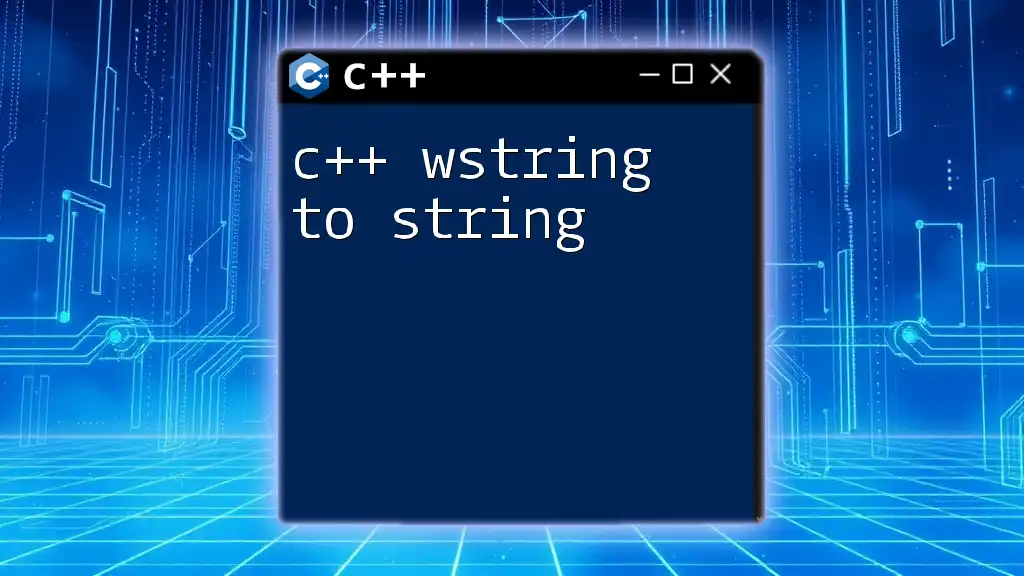
Conclusion
In the debate of C string vs C++ string, the choice largely depends on your specific application needs. While C strings provide essential low-level techniques, C++ strings present a more robust and user-friendly option for modern development practices. Understanding the strengths and limitations of each will empower programmers to make the right choice suitable for their projects.
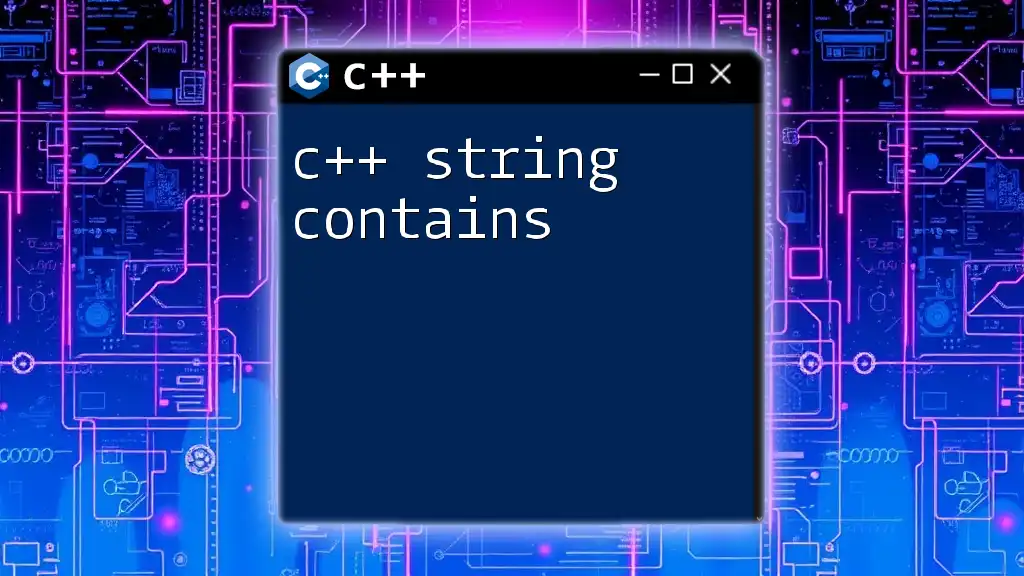
Additional Resources
To further explore the topic, consider reviewing the official C++ documentation and reputable programming books focusing on the Standard Template Library and string manipulation. These resources can provide deeper insights and practical examples to enhance your understanding and proficiency in using strings in C and C++.