The Clang C++ standard refers to the set of C++ language specifications supported by the Clang compiler, allowing developers to write portable and efficient C++ code that adheres to specific versions of the C++ language, such as C++11, C++14, C++17, or C++20.
Here’s a simple example of a C++ command showing the use of a basic `main` function:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is Clang?
Clang is a powerful compiler front end for the C, C++, and Objective-C programming languages. It is part of the LLVM project and stands out due to its architecture, which facilitates modern compiler design and enables continuous improvements. Clang is known for its fast compilation, advanced diagnostics, and compatibility with GCC command-line tools.
The Importance of C++ Standards
C++ standards play a crucial role in ensuring that the language remains consistent, portable, and reliable. By adhering to established standards, developers can create code that is less dependent on specific compilers or platforms, leading to better maintainability and compatibility.
Since the inception of C++, various standards have been introduced:
- C++98: The first standardized version.
- C++03: A bug-fix release of C++98.
- C++11: Introduced significant features such as auto type deduction and lambda expressions.
- C++14, C++17, and C++20: Brought further improvements and modern programming techniques.
Understanding these standards is essential for any C++ developer, especially for users of the Clang compiler.
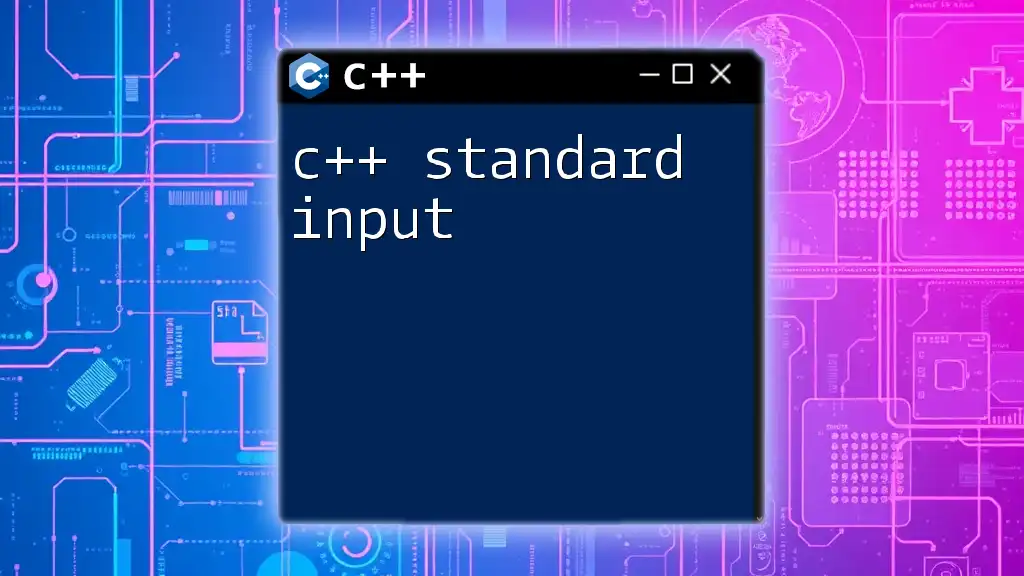
Clang's C++ Standard Support
Understanding Compiler Compliance
Compiler compliance indicates how well a compiler adheres to a specific C++ standard. Clang is renowned for its high level of compliance, making it a preferred choice for many developers.
Enabling C++ Standards in Clang
To compile C++ code using a specific standard in Clang, you can utilize the `-std` flag followed by the desired standard version. Here are some examples:
-
Using C++11:
clang++ -std=c++11 myfile.cpp -o myfile
-
Using C++17:
clang++ -std=c++17 myfile.cpp -o myfile
This flexibility allows developers to leverage the latest features while maintaining compatibility with older standards if necessary.
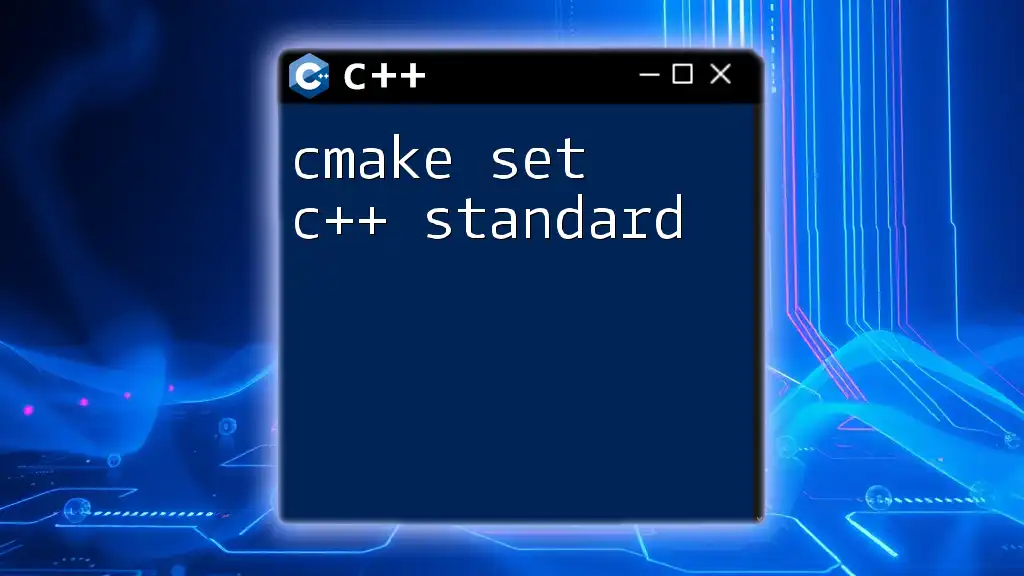
Key Features of Modern C++ Supported by Clang
Auto Type Deduction
The `auto` keyword simplifies type declarations by allowing the compiler to infer the type of an expression. This reduces verbosity and improves code readability.
Example:
auto x = 5; // x is type int
auto name = "John"; // name is type const char*
Range-based For Loops
Range-based for loops introduce a more intuitive way to iterate over collections. This feature minimizes boilerplate code and improves clarity.
Example:
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (auto n : numbers) {
std::cout << n << " ";
}
Lambda Expressions
Lambdas provide a concise syntax for defining anonymous functions, which is particularly useful for callbacks and functional programming styles.
Example:
auto add = [](int a, int b) { return a + b; };
std::cout << add(2, 3); // Outputs 5
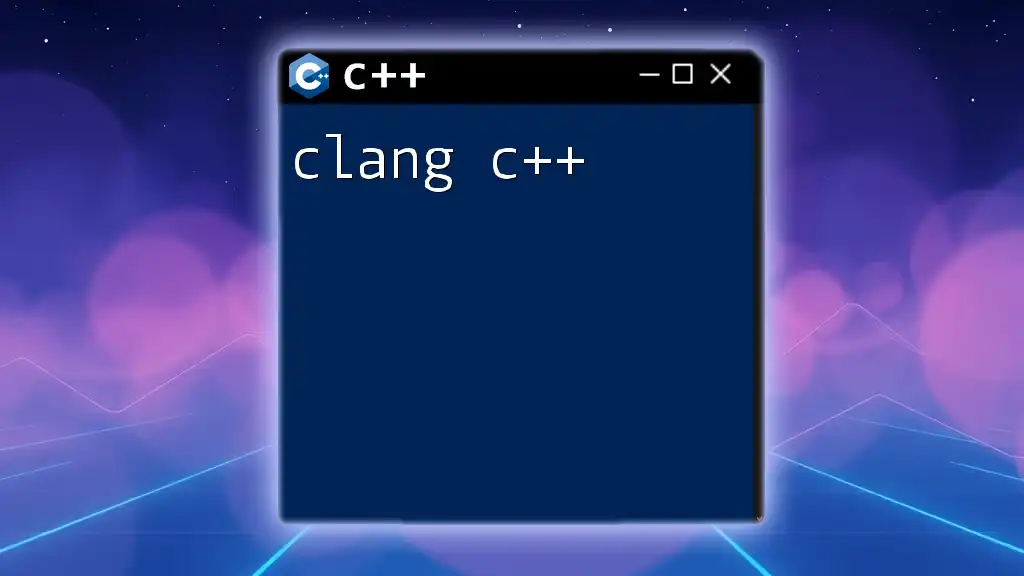
Using Clang with C++ Standards
Setting up Clang on Different Platforms
To leverage the features of the Clang compiler, setup can vary depending on your operating system:
-
Linux: Installation is straightforward via package managers like `apt` or `yum`.
-
Windows: Clang can be used through MinGW or integrated with Visual Studio.
-
macOS: Installation via Homebrew is recommended:
brew install llvm
Conventions for Writing Portable Code
Creating portable C++ code requires adherence to certain conventions, such as using header guards to prevent double inclusion:
#ifndef MY_HEADER_H
#define MY_HEADER_H
// Header content
#endif // MY_HEADER_H
Additionally, using version checks can help ensure compatibility with various C++ standards.
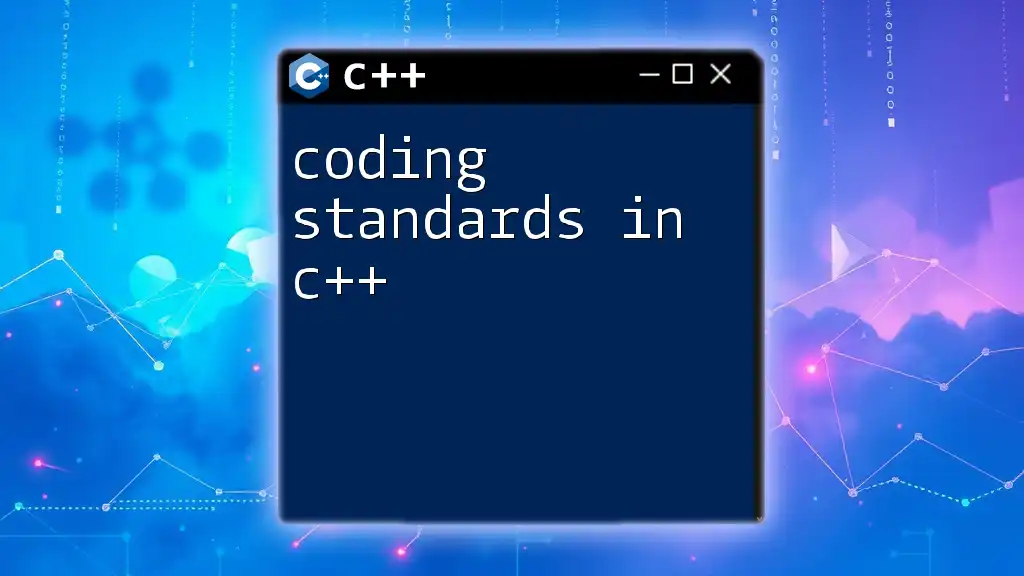
Advanced Features and Best Practices
C++17 and C++20 Features
Optional and Variant Types
C++17 introduced `std::optional` and `std::variant`, which enhance type safety and provide a more expressive approach to handling values that may or may not be present.
Example of `std::optional`:
std::optional<int> opt;
if (opt) {
std::cout << "Value: " << *opt;
} else {
std::cout << "No value present";
}
Coroutines in C++20
Coroutines simplify asynchronous programming, allowing functions to suspend execution and resume later. This feature helps manage state in a more maintainable way.
Example of using `co_await`:
#include <iostream>
#include <coroutine>
struct Coroutine {
struct promise_type {
Coroutine get_return_object() { return {}; }
auto initial_suspend() { return std::suspend_always(); }
auto final_suspend() noexcept { return std::suspend_always(); }
void unhandled_exception() {}
};
};
Coroutine example() {
co_await std::suspend_always{};
std::cout << "Coroutine resumed!";
}
Best Practices for Code Optimization in Clang
Writing efficient code requires attention to detail. Here are some best practices:
-
Use Optimization Flags: Clang provides several optimization flags such as `-O2` for general optimizations and `-O3` for aggressive optimizations. Use them to enhance runtime performance.
-
Profile-Guided Optimization (PGO): Collect data during test runs to refine optimization strategies.
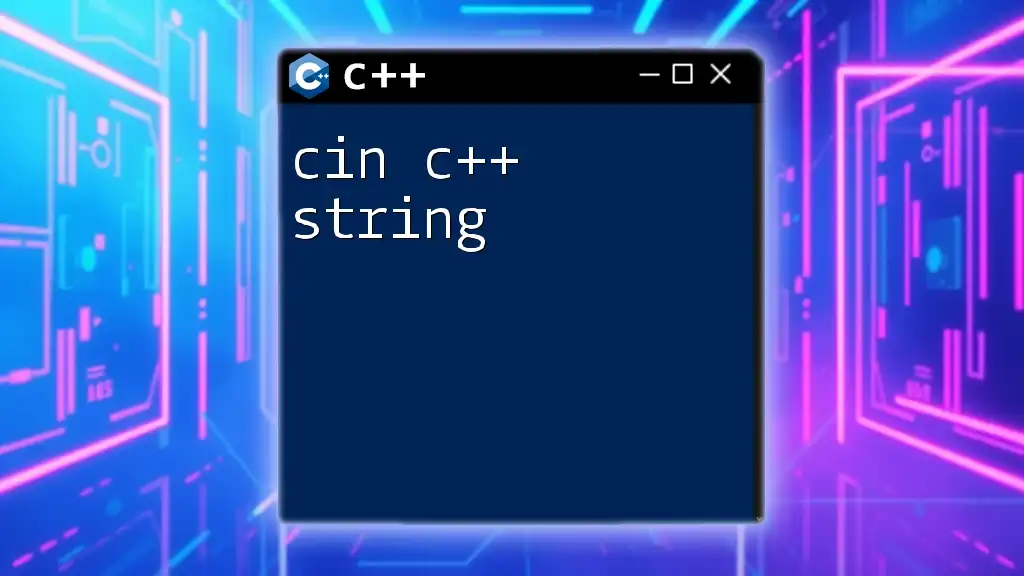
Troubleshooting Common Issues
Common Compilation Errors
When using Clang, understanding compiler messages is essential. Some common errors include missing headers or mismatched types. Reading the error message carefully can lead to quicker resolutions.
Cross-Platform Issues
Differences in C++ library implementations can cause portability challenges. Always test your code on multiple platforms and utilize preprocessor directives to manage inconsistencies.
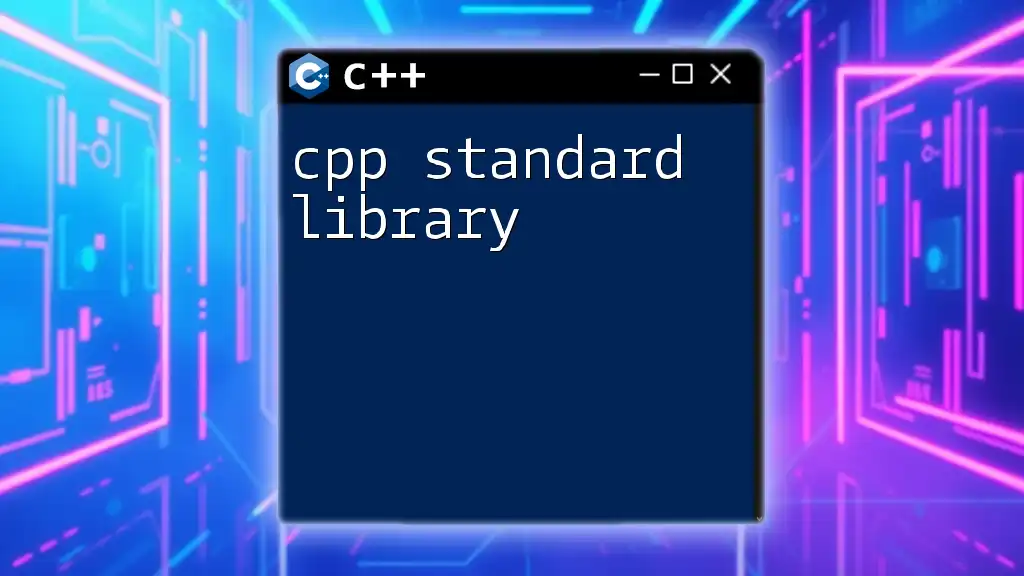
Conclusion
As C++ continues to evolve, so does Clang's implementation of its standards. By staying informed about the latest features and adhering to good practices, developers can leverage the full power of Clang to create robust and efficient applications. Exploring the documentation provided by Clang further enhances understanding and aids in mastering the essentials of the Clang C++ standard.
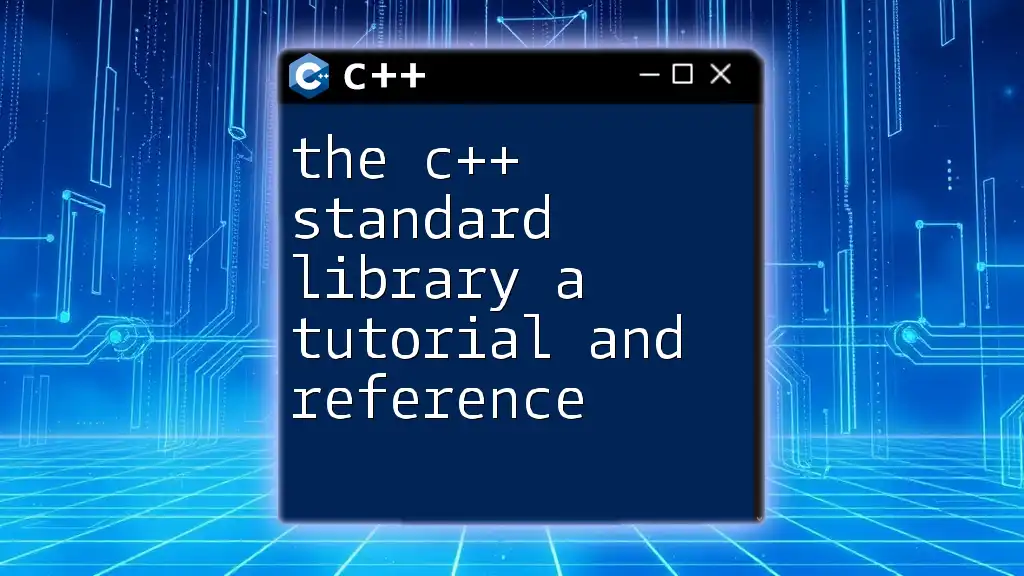
References
For further exploration, delve into these resources:
- [Clang Official Documentation](https://clang.llvm.org/docs/)
- [C++ Standards and Updates](https://en.cppreference.com/w/cpp/17)
- [LLVM Project](https://llvm.org/)