The C++ Standard Library provides a collection of pre-defined classes and functions that facilitate various programming tasks, allowing developers to write efficient and reusable code.
Here's a simple example demonstrating the use of the `vector` class from the C++ Standard Library:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
What is the C++ Standard Library?
The C++ Standard Library is a powerful set of pre-defined classes and functions that provide essential tools for C++ programming. It offers a wealth of utility components that facilitate various programming tasks, enabling developers to write efficient and reliable code without reinventing the wheel.
Components of the Standard Library
The library comprises several key components, including:
- Containers: These are data structures that store collections of objects. They help manage data efficiently.
- Algorithms: A collection of functions that perform operations on containers, such as sorting and searching.
- Iterators: These provide a method for traversing through the elements of a container.
- Utilities: This section includes various helper functions and classes, such as smart pointers.
- Input/Output (I/O) Streams: These handle data input and output, allowing for interaction with the user or files.
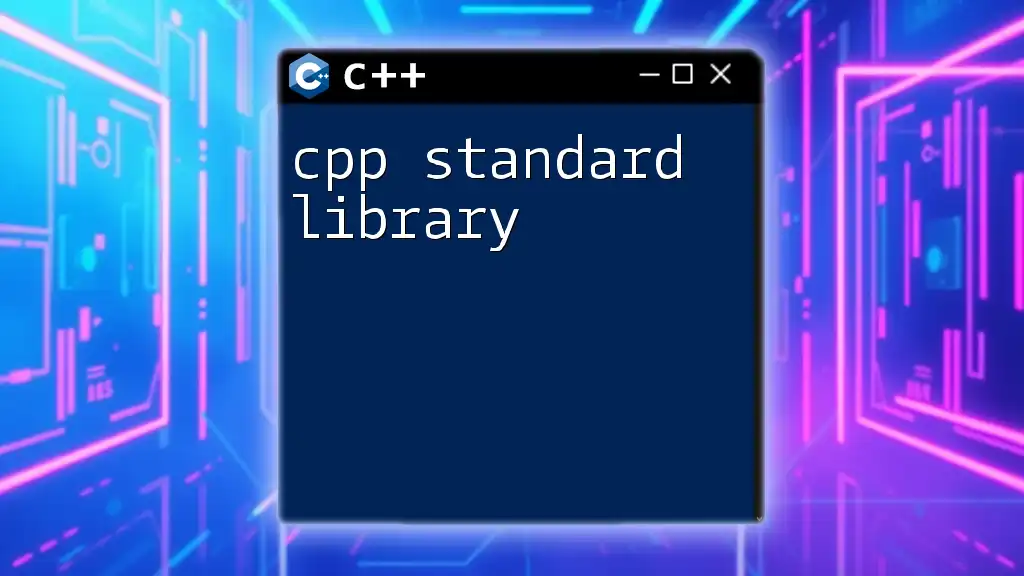
Getting Started with the Standard Library
To access the C++ Standard Library in your project, you include the relevant header files. You can either include the entire library or specific components depending on your needs. For example, you can include the headers for input-output streams and the vector container like this:
#include <iostream> // For standard input/output
#include <vector> // For using the vector container
This practice not only makes your code cleaner but also can improve compilation time by reducing unnecessary inclusions.
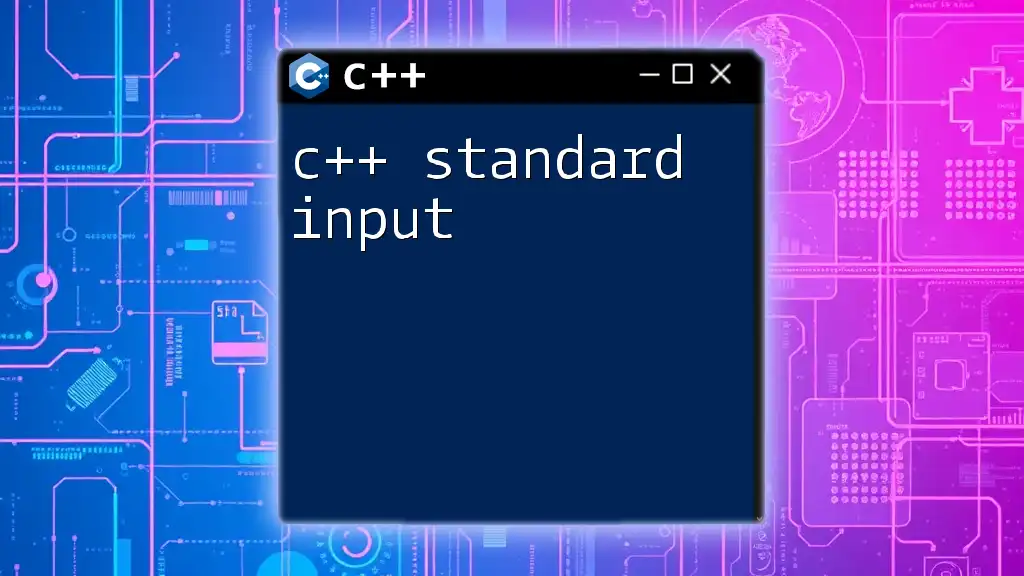
Commonly Used Components
Containers
Containers are vital for organizing and managing data efficiently. They come in various forms, each designed for different use cases:
- Sequence Containers: These allow elements to be stored in a linear sequence. Examples include:
- `std::vector`: A dynamic array that can grow and shrink as needed.
- `std::deque`: A double-ended queue allowing fast insertions and deletions at both ends.
- `std::list`: A doubly linked list ideal for scenarios requiring frequent insertions/deletions.
Example of Using `std::vector`:
#include <vector>
#include <iostream>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for(int n : numbers) {
std::cout << n << " ";
}
return 0;
}
In this example, a vector is created and initialized with values, demonstrating how to iterate through the container using a range-based for loop.
-
Associative Containers: These store key-value pairs, allowing for fast retrieval based on keys. Common examples are:
- `std::set`: A collection of unique elements organized in a specific order.
- `std::map`: A collection of key-value pairs, where each key is unique.
-
Unordered Containers: As the name suggests, they do not maintain any specific order. For instance:
- `std::unordered_set`: A collection of unique elements optimized for fast lookups.
- `std::unordered_map`: A hash table with key-value pairs, allowing for average-case constant time complexity in search operations.
Algorithms
The C++ Standard Library provides a rich set of algorithms that can be applied to the various containers. These algorithms simplify complex tasks like searching, sorting, and manipulating collections of data.
Two commonly utilized algorithms include:
- Sorting Algorithms: Functions such as `std::sort` allow you to sort elements in a container effortlessly.
Example of Using `std::sort`:
#include <algorithm>
#include <vector>
#include <iostream>
int main() {
std::vector<int> numbers = {5, 3, 2, 4, 1};
std::sort(numbers.begin(), numbers.end());
for (int n : numbers) {
std::cout << n << " ";
}
return 0;
}
In this case, the vector of integers is sorted in ascending order using `std::sort`, showcasing the simplicity of applying an algorithm from the Standard Library.
- Searching Algorithms: Functions like `std::find` and `std::binary_search` help to locate elements in containers quickly, depending on the container and your needs.
Iterators
Iterators play a crucial role in navigating through the elements stored in containers. They abstract the traversal mechanism, allowing you to write generic code that can work with any container type.
Types of Iterators:
- Input Iterators: These allow reading elements from a container (one read at a time).
- Output Iterators: These permit writing elements to a container.
- Forward Iterators: These allow multiple reads/writes in one direction.
- Bidirectional Iterators: These enable traversal in both directions (forward and backward).
- Random Access Iterators: These support direct access to any element, enabling complex navigational operations.
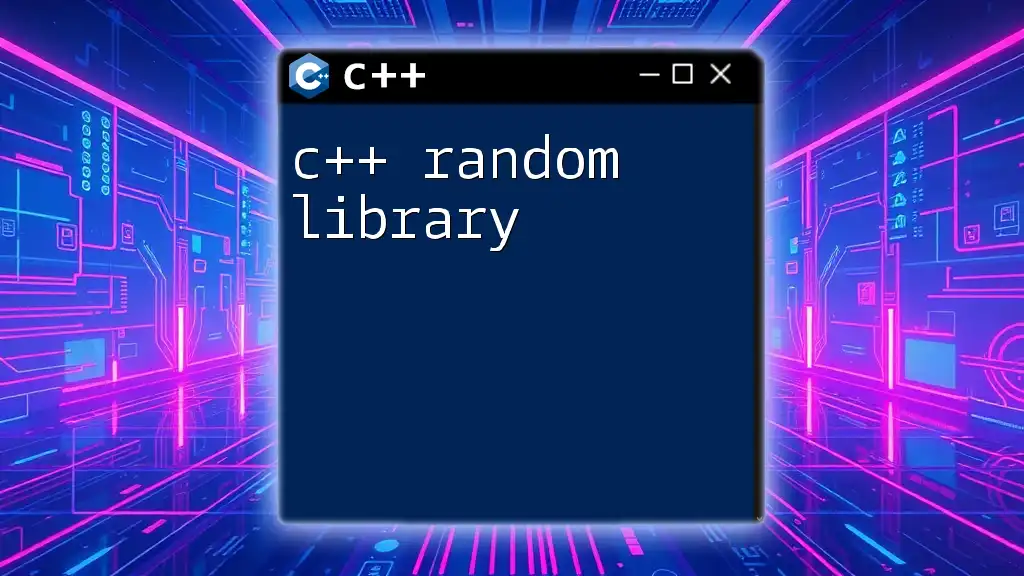
Detailed Exploration of Key Components
Containers
`std::vector`
`std::vector` is a dynamic array that can resize itself automatically. It provides various methods for adding, removing, and accessing elements, making it one of the most commonly used containers in C++. It is especially favored for its direct element access through indices.
`std::map`
`std::map` is ideal for scenarios where you need to store pairs of data, such as associating names with phone numbers. The keys in a map must be unique, and the map sorts the elements based on those keys.
`std::set`
`std::set` is useful for maintaining a collection of unique objects. It automatically orders the elements, which can also allow for quicker searches.
Algorithms
Sorting Algorithms
The `std::sort` function is just one example. Options like `std::stable_sort` maintain the relative order of equal elements.
Searching Algorithms
The library provides algorithms such as `std::find_if`, which can be used with lambda functions for customized searches, adding powerful search capabilities.
Iterators
You can leverage iterators to traverse through a container. Using iterators is not only more generic but also improves code readability. Below is an example of using iterators to traverse a vector:
#include <vector>
#include <iostream>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (auto it = numbers.begin(); it != numbers.end(); ++it) {
std::cout << *it << " ";
}
return 0;
}
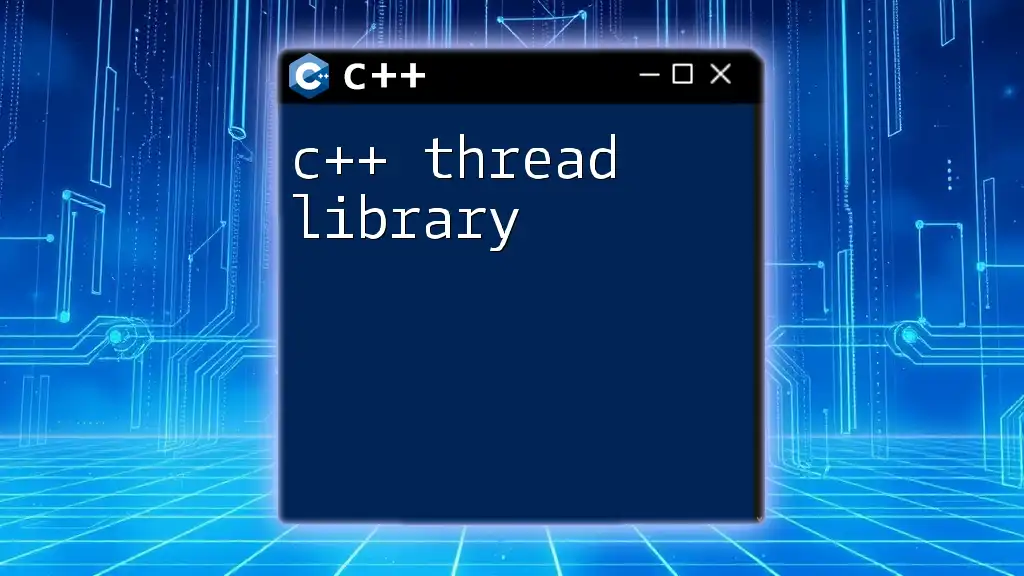
Input and Output Stream
C++ streams are essential for handling data input and output. The two primary objects used for this purpose are `cin` (standard input) and `cout` (standard output). Additionally, file streams such as `ifstream` and `ofstream` provide functionality to read from and write to files.
Manipulators
Stream manipulators allow you to format output easily. For example, using `std::fixed` and `std::setprecision` can help control how floating-point numbers are displayed.
Example:
#include <iostream>
#include <iomanip>
int main() {
double pi = 3.14159;
std::cout << std::fixed << std::setprecision(2) << pi << std::endl;
return 0;
}
In the example above, `std::fixed` is used to format the output to two decimal places for the value of Pi.
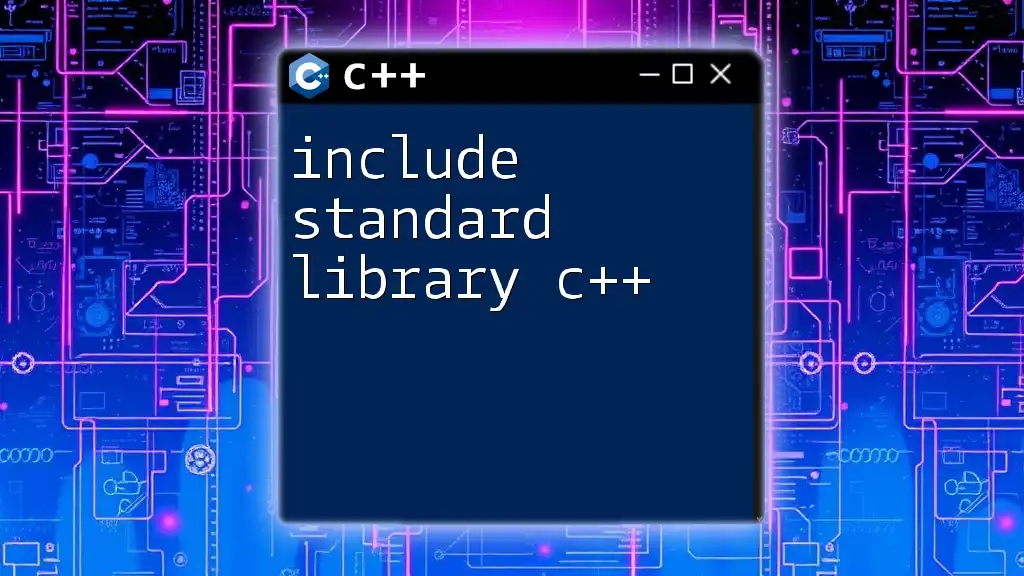
Utilities
Outside of containers and algorithms, the Standard Library includes several utility classes and functions. Smart pointers like `std::unique_ptr` and `std::shared_ptr` are critical in modern C++ for managing dynamic memory more safely. They automatically deallocate memory, preventing memory leaks.
Function Objects and Lambdas
Function objects (functors) and lambda expressions allow you to create anonymous functions that you can pass to algorithms. This feature adds flexibility to your code, enabling shorter and more expressive expressions.
Example of Using a Lambda:
#include <iostream>
#include <algorithm>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
std::for_each(numbers.begin(), numbers.end(), [](int &n) { n++; });
for (int n : numbers) {
std::cout << n << " ";
}
return 0;
}
Here, we're using `std::for_each` alongside a lambda function to increment each number in the vector.
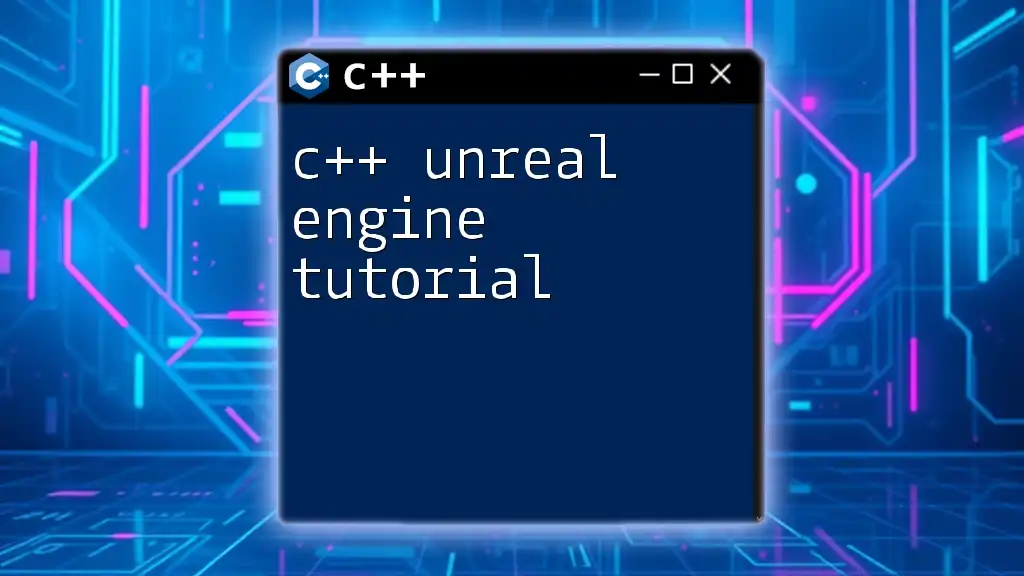
Best Practices for Using the C++ Standard Library
When utilizing the components of the C++ Standard Library, consider the following best practices for efficiency and reliability:
- Use the right container for the job. Each container has its strengths and weaknesses.
- Rely on algorithm functions instead of writing your own from scratch when possible. Library functions are optimized and thoroughly tested.
- Prefer iterators for traversing containers to enhance the readability and efficiency of your code.
Avoiding Common Pitfalls
One common mistake is misusing the lifecycle of dynamic objects managed by smart pointers. Always ensure that shared and unique pointers are properly managed to avoid dangling references or memory leaks.
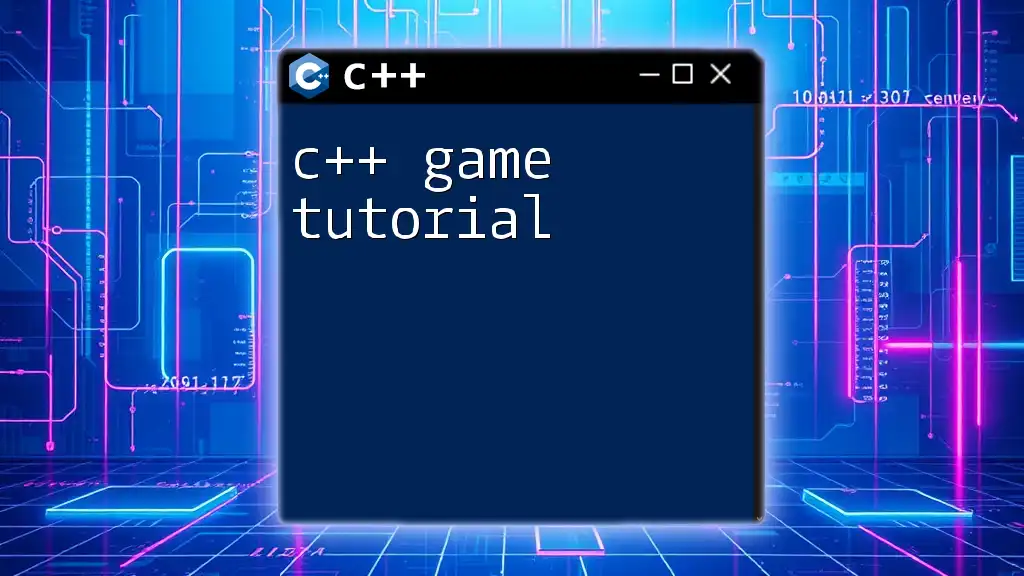
Conclusion
The C++ Standard Library is an indispensable tool in the C++ programmer's toolkit. Understanding how to effectively utilize its components—like containers, algorithms, and utility functions—will significantly enhance your programming efficiency and code quality.
By becoming familiar with the library's usage and best practices, you will be well on your way to becoming a proficient C++ developer. Don't hesitate to explore its vast functionalities, and leverage its power to streamline your coding experience.
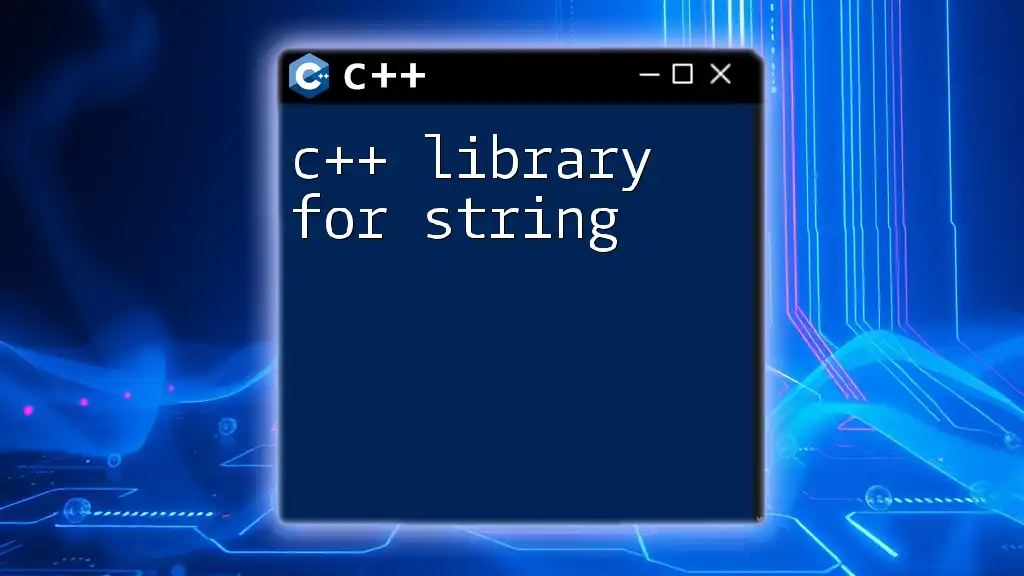
Additional Resources
As you delve deeper into the C++ Standard Library, consider consulting additional materials such as books, online courses, and the official documentation. These resources will provide you with further insights and advanced techniques for maximizing your use of the Standard Library.