"C++ How to Program, 10th Edition" provides a comprehensive guide to mastering C++ programming with practical examples and clear explanations, making it an essential resource for beginners and experienced programmers alike.
Here’s a simple code snippet demonstrating a basic C++ program that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Key Features of the 10th Edition
C++ How to Program 10th Edition by Deitel and Deitel presents a vital resource for both beginners and seasoned programmers. This edition has been updated to include the latest advancements in the C++ language, incorporating modern programming practices.
One of the standout features is the use of structured programming techniques, accompanied by real-world examples. This helps to bridge theoretical knowledge with practical applications, making the learning experience enriching and effective.
Additionally, this edition comes equipped with various learning tools, including video lectures and online resources, enabling learners to engage interactively with the material.
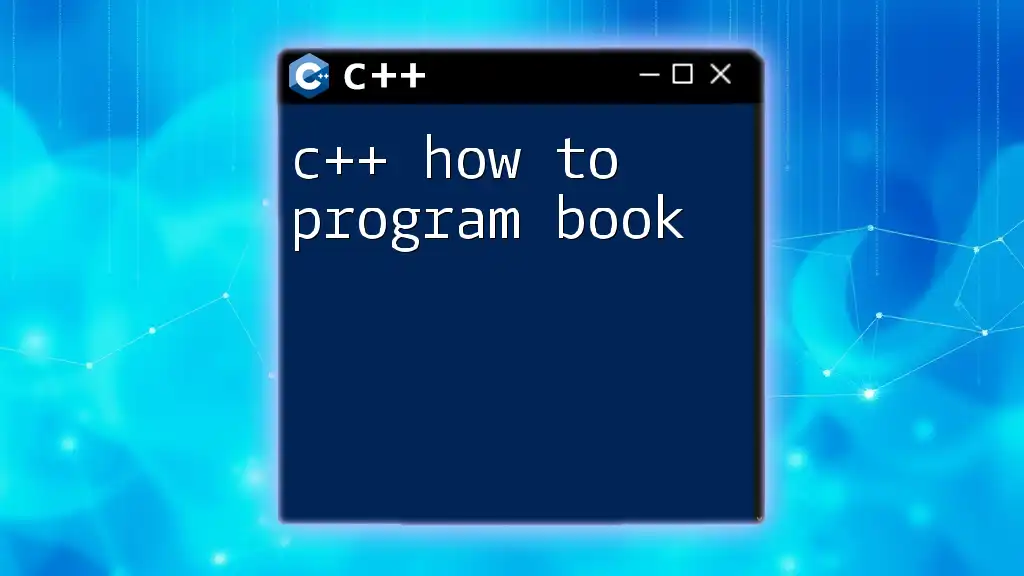
Getting Started with C++
Why C++?
C++ remains one of the most relevant programming languages in the tech industry today. It is known for its versatility and performance, capable of handling applications ranging from system/software development, game programming, to high-performance computing.
When you think of systems programming, C++ is often at the forefront due to its direct access to hardware and memory management features. This makes it essential in fields such as embedded systems or game development, where performance is critical.
Setting Up Your Environment
To start programming in C++, you first need to establish your development environment.
-
Installing a Compiler: Popular Integrated Development Environments (IDEs) include Visual Studio for Windows and Code::Blocks for a more lightweight option compatible with multiple operating systems.
-
Configuring Your First Project: Once your IDE is set up, create a new project. This usually involves selecting a project type (Console Application, for instance) and configuring any necessary settings such as project name and storage location.
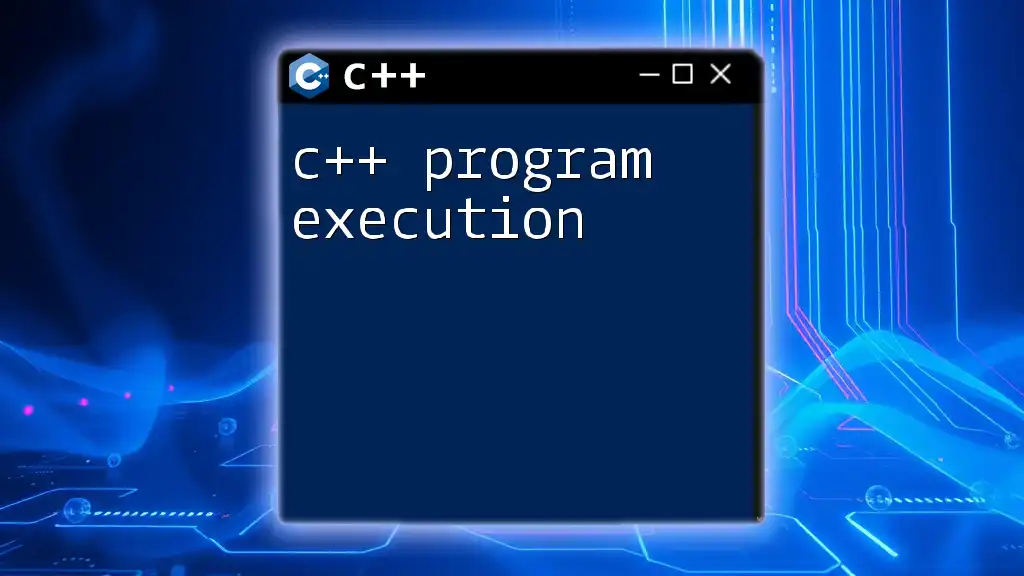
Fundamental Concepts
Basic Syntax and Structure
The basic syntax of C++ forms the foundation of all subsequent programming concepts. Understanding this is essential for writing effective code.
Consider the classic Hello World program, a staple of programming education:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Here, we include the iostream library to enable standard input and output. The main() function is where the execution begins. Inside the function, `std::cout` is used to print "Hello, World!" to the console, showcasing the use of standard output.
Variables and Data Types
C++ has a rich set of data types that caters to different programming needs. Start by understanding basic data types such as:
- int: Integer values
- float: Floating-point numbers
- char: Character values
- bool: Boolean values (true/false)
Variable Declaration and Initialization is crucial. Look at this example where we declare variables:
int age = 30;
float salary = 2500.50;
In this code, we declare an integer variable named age initialized to the value 30, and a floating-point variable salary set to 2500.50. Proper naming and initializing of variables is critical in maintaining code clarity and functionality.
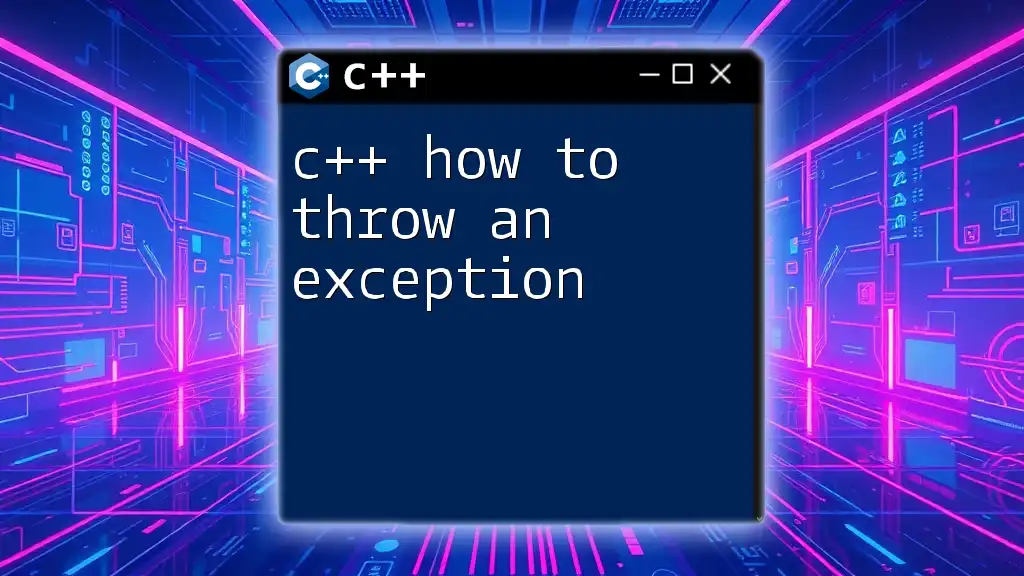
Control Structures
Conditional Statements
Conditional statements allow the program to execute different actions based on specific conditions.
Consider this example that uses if, else if, and else statements:
int age = 20;
if (age < 18) {
std::cout << "Minor";
} else {
std::cout << "Adult";
}
The program checks the age and prints "Minor" if the age is less than 18; otherwise, it prints "Adult."
Loops
Loops enable repetitive execution of a block of code. Understanding the difference between a for loop and a while loop is essential.
A typical for loop looks like this:
for (int i = 0; i < 5; i++) {
std::cout << "Iteration: " << i << std::endl;
}
This loop iterates five times, printing the iteration count each time.
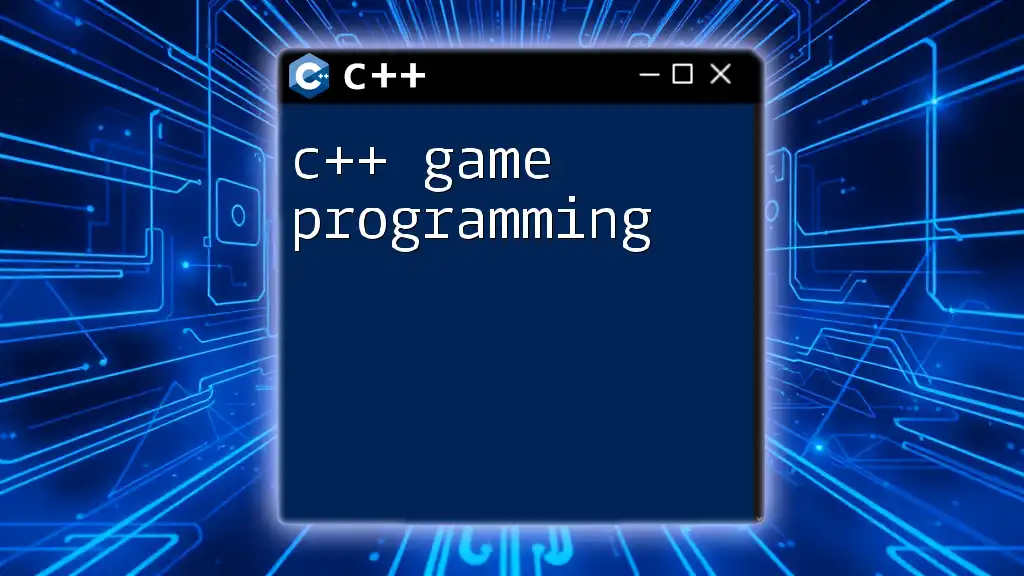
Functions
Defining and Calling Functions
Functions help in organizing code into reusable blocks. Here’s a simple function that adds two integers:
int add(int a, int b) {
return a + b;
}
In this function definition, you declare two integer parameters, a and b, and return their sum.
Scope and Lifetime of Variables
Understanding variable scope and lifetime is crucial. Local variables are those defined within functions, while global variables are defined outside any function and can be accessed anywhere in the program. The careful management of variable scope aids in creating cleaner and maintainable code.
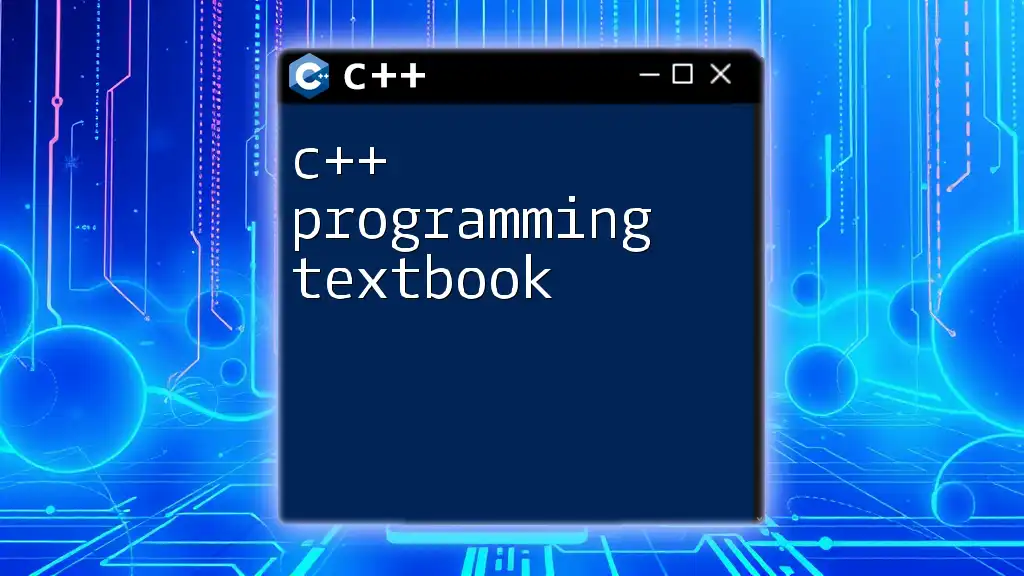
Object-Oriented Programming
Classes and Objects
C++ is well-known for its support of Object-Oriented Programming (OOP). OOP allows programmers to create self-contained modules known as classes. Here’s an example of a simple class:
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
Dog myDog;
myDog.bark(); // Call the bark method
In the Dog class above, the method bark() outputs "Woof!" whenever invoked. Class constructs allow for complex data management and functionality to be bundled together.
Inheritance and Polymorphism
Inheritance allows a new class to inherit attributes and methods from an existing class, fostering code reuse. For example, if you create a class Animal with general attributes, you can create Dog and Cat classes that inherit from it.
Polymorphism, particularly function overriding, lets a derived class modify a method inherited from its base class, allowing for dynamic method resolution at runtime.
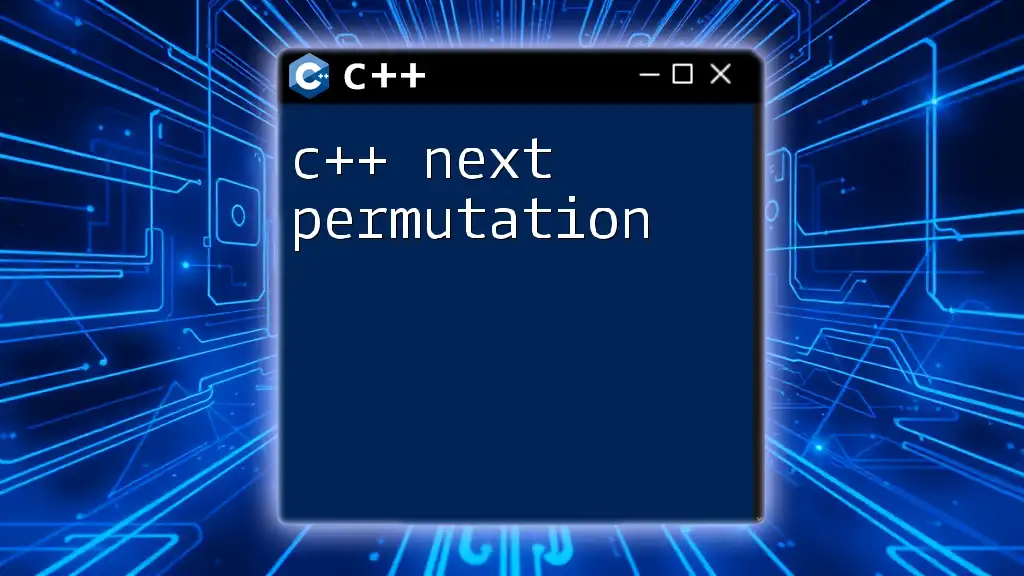
Standard Template Library (STL)
Introduction to STL
The Standard Template Library (STL) is a powerful feature in C++ that provides generic classes and functions, promoting code reusability. This library facilitates the use of data structures and algorithms seamlessly.
Common Containers
STL offers a variety of containers, such as vectors, lists, and maps. Vectors are dynamic arrays that can resize themselves, providing immense flexibility. Here's a sample usage of a vector:
std::vector<int> numbers = {1, 2, 3, 4, 5};
This line initializes a vector with five integer elements, showcasing STL's ability to handle dynamic data efficiently.
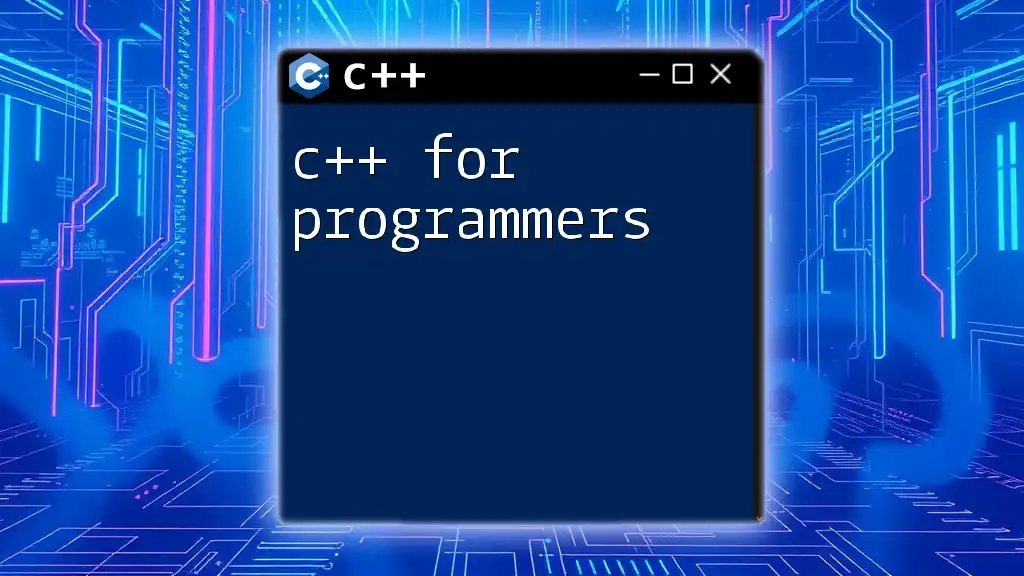
Best Practices and Tips
Writing Clean Code
Writing clean code is paramount in programming. Proper indentation, meaningful naming conventions, and judicious use of comments greatly enhance the readability and maintainability of your code. As a rule of thumb, aim for clarity, simplicity, and consistency in your coding style.
Debugging Techniques
Effective debugging techniques are essential for finding and fixing errors in your code. Use the debugging tools available in your IDE to step through your code, inspect variables, and observe the program flow. Developing a habit of using breakpoints and logging output can dramatically reduce your debugging time.
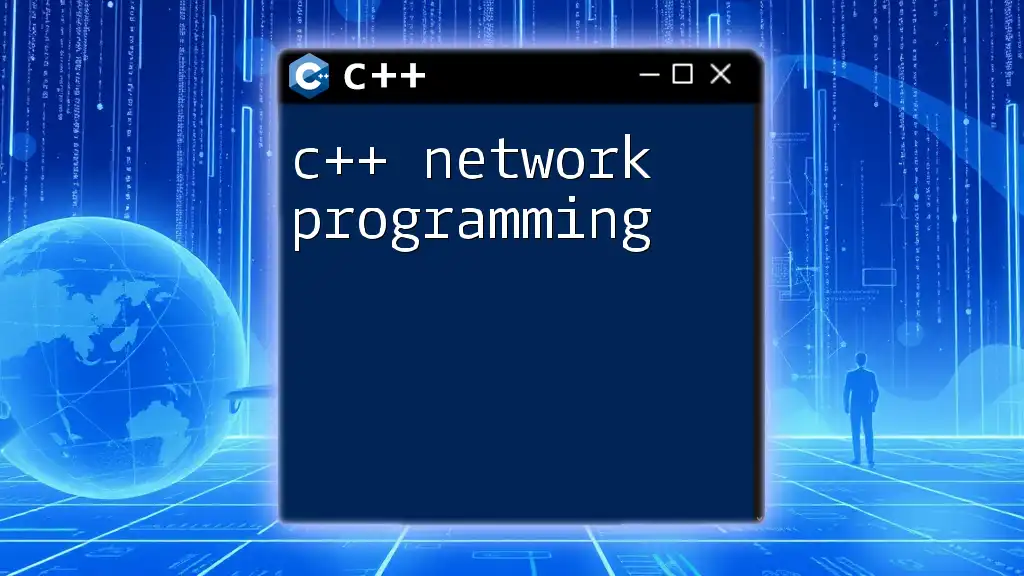
Conclusion
In summary, the concepts covered in C++ How to Program 10th Edition provide a solid foundation for any programmer's journey. From basic syntax to object-oriented principles, each element builds upon the last to equip you with valuable coding skills.
To truly master C++, consistent practice and exploration beyond this guide are vital. Keep pushing your boundaries, build projects, and continuously enhance your understanding of the language.
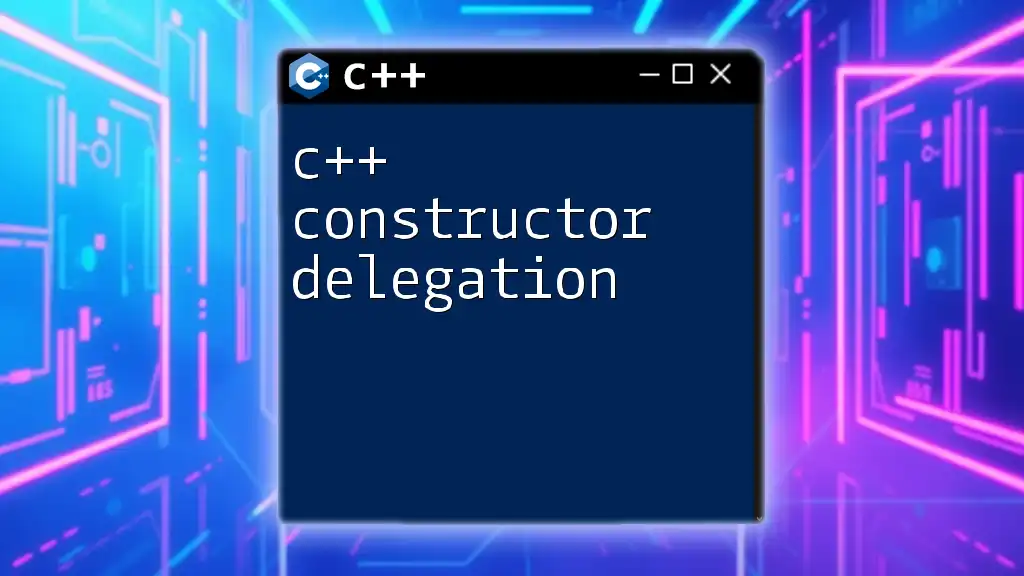
Additional Resources
To further support your learning journey, consider exploring additional books and tutorials that can deepen your understanding of C++. Online platforms and communities such as Stack Overflow or Reddit can be invaluable for networking and getting help as you navigate through your C++ programming adventure.
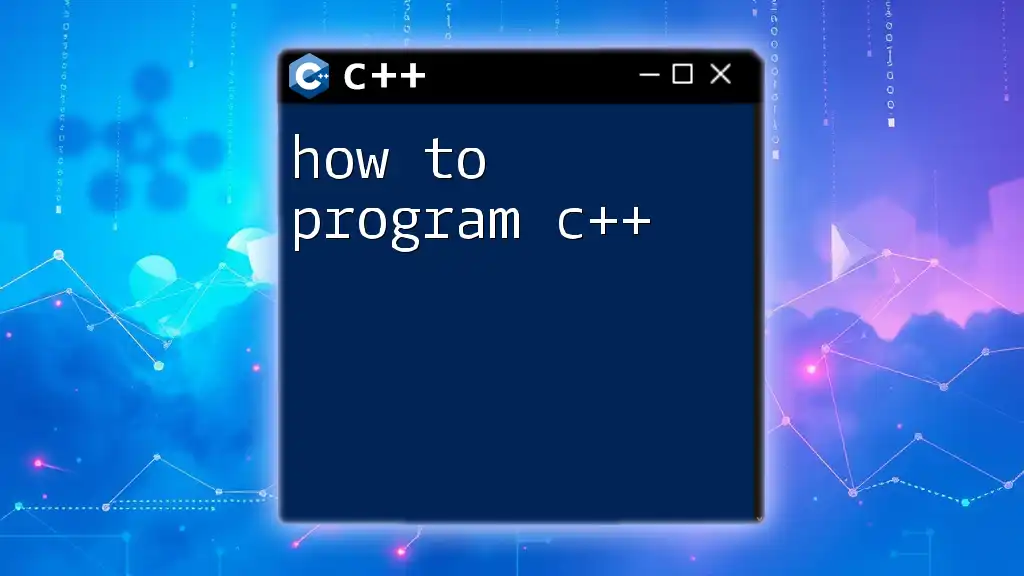
Call to Action
If you’re ready to dive deeper into C++ programming, consider enrolling in our courses, where we offer comprehensive training tailored for every skill level. Together, let’s make your programming journey rewarding and enjoyable!