In C++, you can throw an exception using the `throw` keyword followed by an instance of an exception class, allowing you to handle errors gracefully.
#include <iostream>
#include <stdexcept>
int divide(int a, int b) {
if (b == 0) {
throw std::invalid_argument("Division by zero!");
}
return a / b;
}
int main() {
try {
std::cout << divide(10, 0) << std::endl;
} catch (const std::invalid_argument& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
return 0;
}
Understanding Exceptions in C++
Exceptions are a powerful mechanism in C++ that allow developers to handle errors and unexpected situations in a controlled manner. They enable a program to maintain flow and functionality even when issues arise, distinguishing between regular control flow and anomalous behavior. The importance of exception handling cannot be overstated; it enhances the robustness and reliability of software, allowing developers to manage errors without cluttering the main logic of their code.
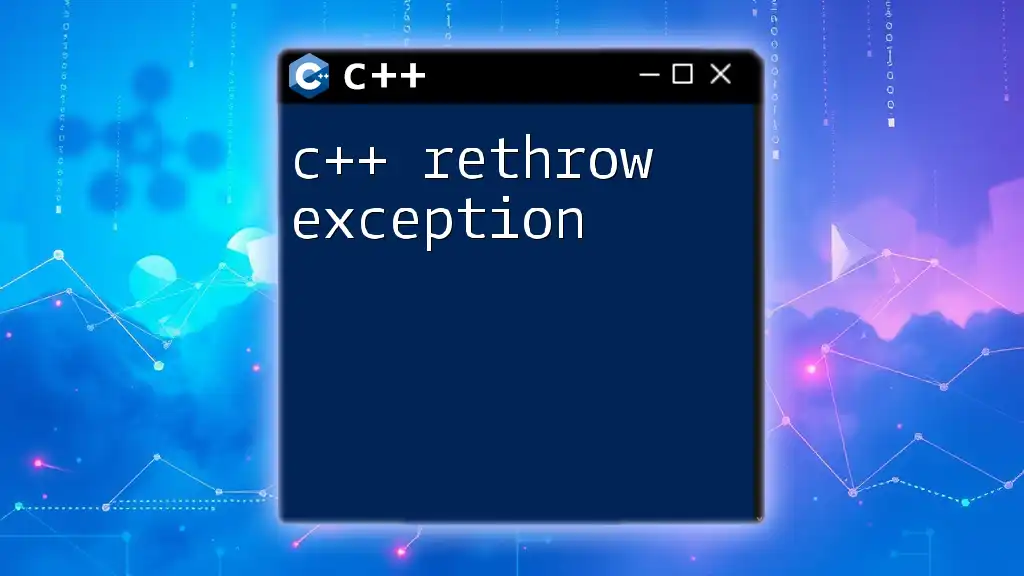
What is an Exception?
An exception in C++ is an event that disrupts the normal flow of a program’s execution. When certain conditions are encountered, such as invalid data or unexpected scenarios, exceptions can be "thrown." It’s essential to recognize that exceptions can be categorized into two types:
- Built-in Exceptions: Provided by the C++ Standard Library, these include various types of errors such as runtime errors, invalid arguments, or out-of-range accesses.
- User-defined Exceptions: Developers can create their own exceptions by deriving from existing exception classes, allowing customization for specific application needs.
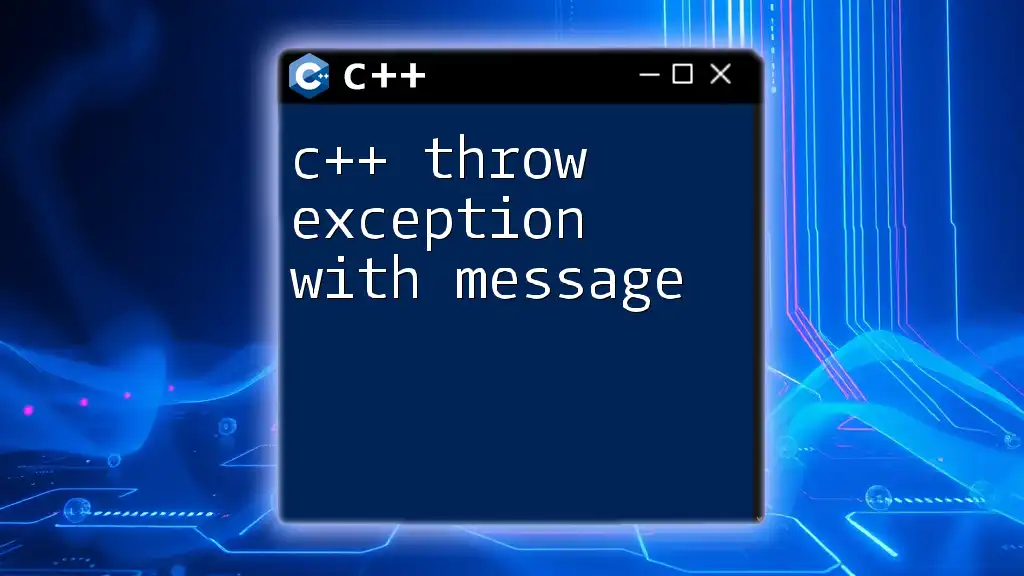
Throwing Exceptions in C++
Basic Syntax of the Throw Statement
In C++, the `throw` keyword is used to signal that an exception has occurred. The basic syntax involves using the throw keyword followed by an instance of an exception class. This provides context regarding the type of error that has occurred. A simple example would be:
throw std::runtime_error("An error occurred");
How to Throw an Exception in C++
Throwing an exception in C++ involves identifying conditions under which the exception should be raised. Let's examine how to do this step-by-step using a practical code snippet:
- Identify potential error scenarios.
- Use the `throw` keyword when these scenarios are met.
Example:
#include <iostream>
#include <stdexcept>
void exampleFunction() {
throw std::invalid_argument("Invalid argument provided");
}
This function will raise an `invalid_argument` exception if invoked. It’s crucial to ensure that the calling code is prepared to handle this.
Common Exception Types in C++
There are several standard exception types defined in the `<stdexcept>` header, which you can utilize in your applications:
- std::runtime_error: For errors that can only be detected during runtime.
- std::invalid_argument: Specifically when an argument to a function is invalid.
- std::out_of_range: Used when a request is made for an element outside the valid range.
User-defined Exceptions
Creating custom exception classes allows for greater flexibility and specificity in error reporting. When defining a user-defined exception, it’s best practice to inherit from `std::exception`. Here’s an example:
class MyException : public std::exception {
public:
const char* what() const noexcept override {
return "My custom exception occurred";
}
};
Throwing an Exception in C++: A Practical Example
Integrating throwing exceptions in your functions can improve code readability and error handling significantly. Below is a more comprehensive example that includes the throwing and catching of exceptions.
#include <iostream>
#include <stdexcept>
void checkValue(int value) {
if (value < 0) {
throw std::invalid_argument("Negative value not allowed");
}
}
int main() {
try {
checkValue(-1);
} catch (const std::invalid_argument& e) {
std::cerr << e.what() << std::endl;
}
return 0;
}
In this example, if `checkValue` is called with a negative integer, it throws an exception. The `main` function includes a try-catch block that captures this thrown exception, allowing the program to handle it gracefully rather than crashing.
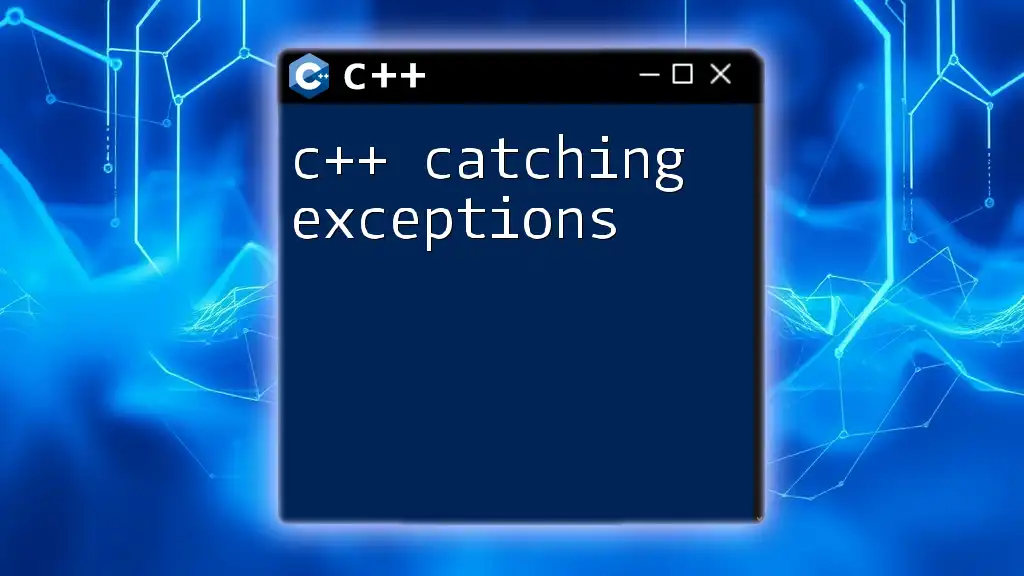
Catching Exceptions in C++
The Catch Block
When an exception is thrown, the control flow can be transferred to a catch block that handles the exception. The syntax for the catch block is:
catch (exception_type identifier) {
// Handle the exception
}
This allows developers to specify which type of exception they want to handle, enabling tailored responses.
Using Multiple Catch Blocks
In cases where multiple types of exceptions may arise, you can utilize multiple catch blocks. This approach allows different responses based on the type of exception caught.
try {
// Code that may throw
} catch (const std::invalid_argument& e) {
// Handle invalid argument exception
std::cerr << e.what() << std::endl;
} catch (const std::runtime_error& e) {
// Handle runtime errors
std::cerr << e.what() << std::endl;
}
Catching General Exceptions
A catch-all handler can be used to catch any exceptions that do not match specific types. This is done with the ellipsis (`...`). It’s crucial to note that while this is convenient, it may obscure the specific nature of the error, so it should be used sparingly.
try {
// Code that may throw
} catch (...) {
std::cerr << "An unknown error occurred." << std::endl;
}
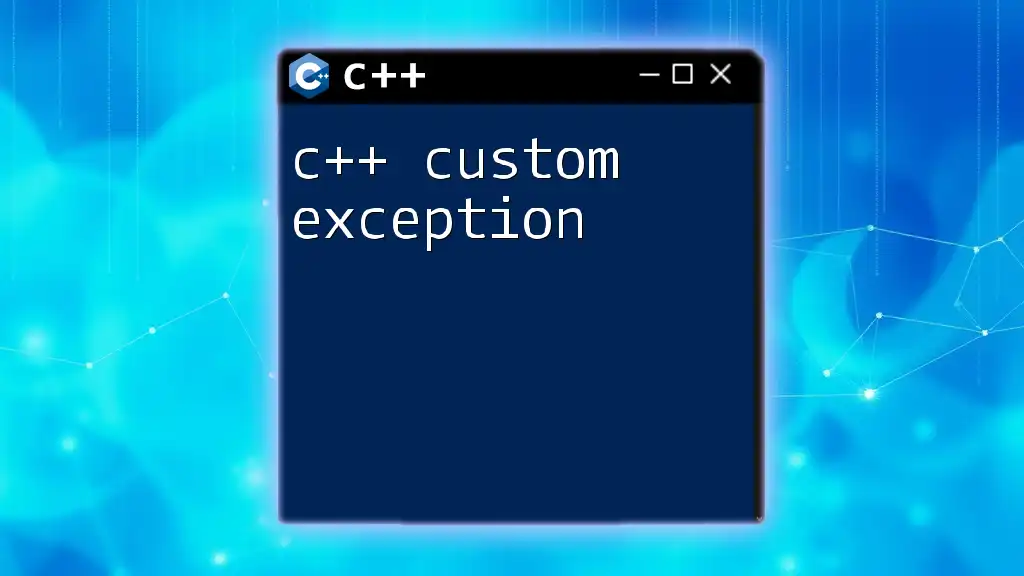
Best Practices for Throwing Exceptions in C++
When to Throw Exceptions
Understanding when to throw exceptions is vital for writing clean, maintainable code. Use exceptions for truly exceptional circumstances, such as invalid inputs or failed operations. Avoid overusing exceptions for regular control flow, as this can lead to performance hits and reduce code clarity.
Avoiding Common Pitfalls
Several common pitfalls can accompany exception handling:
-
Ignoring Exceptions: Failing to catch an exception can lead to program crashes. Always ensure your throw statements are paired with handle logic.
-
Resource Management: When an exception is thrown, it may interrupt code execution in the middle of resource allocation. Utilize RAII (Resource Acquisition Is Initialization) patterns or smart pointers to ensure resources are reclaimed correctly.
-
Nested Exceptions: Throwing exceptions from within catch blocks can complicate error handling. If this is necessary, ensure the outer catch handles the re-thrown exception properly.
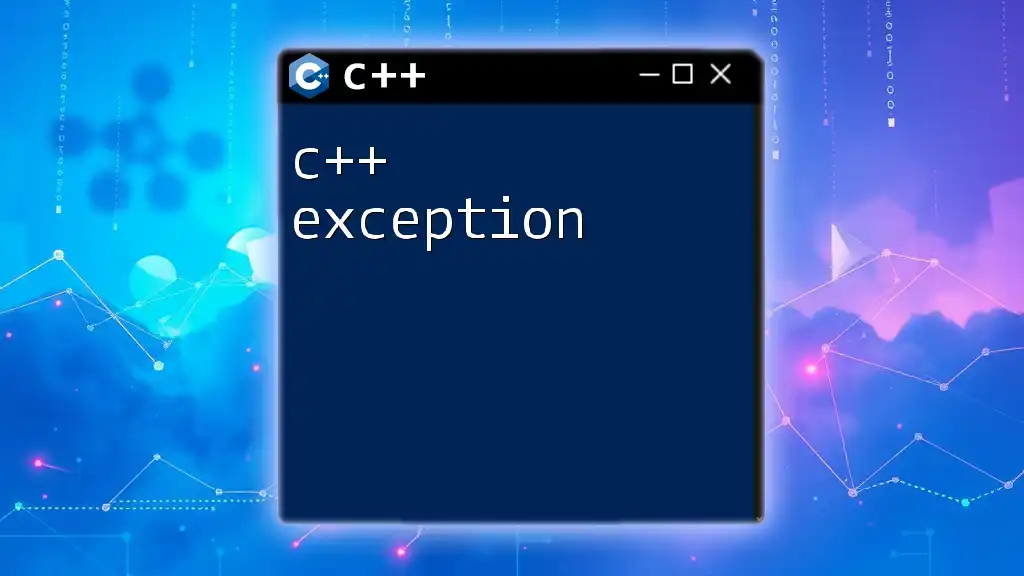
Conclusion
In conclusion, understanding C++ how to throw an exception is crucial to mastering effective error handling in your applications. Leveraging exceptions allows for cleaner, more manageable code that can respond gracefully to unexpected issues. By practicing the techniques discussed, including throwing and catching exceptions, creating custom exceptions, and following best practices, you can significantly enhance the reliability and maintainability of your C++ software.
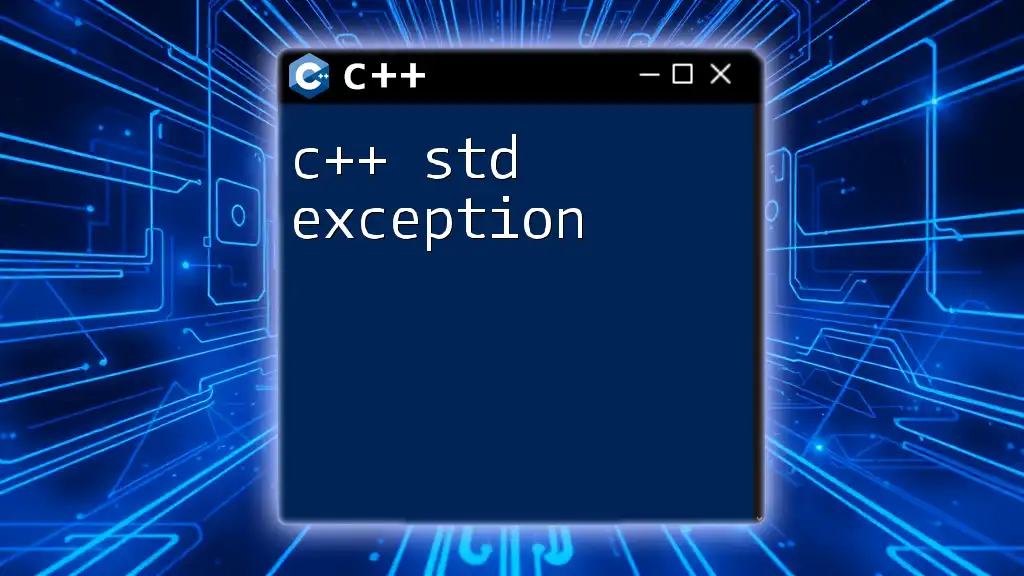
Additional Resources
To deepen your understanding of exception handling, consulting the official C++ documentation is beneficial. Additionally, consider looking into recommended books and tutorials focused on C++ programming and best practices.
FAQ Section (Optional)
-
What types of exceptions can I define? You can define exceptions derived from `std::exception` to create any type you want. Ensure you override the `what()` method for descriptive messages.
-
Should I use exceptions for every error? Use exceptions judiciously; they should represent truly exceptional conditions. Regular errors might be better handled with return codes or assertions.
By implementing these strategies and gaining hands-on experience with exception handling in C++, you can elevate your programming proficiency and solve complex problems more effectively.