A C++ floating point exception occurs when an invalid arithmetic operation, such as division by zero or overflow, is performed, leading to runtime errors.
Here’s an example code snippet that demonstrates a floating point exception by attempting to divide by zero:
#include <iostream>
int main() {
double numerator = 10.0;
double denominator = 0.0; // Division by zero
double result = numerator / denominator; // This will cause a floating point exception
std::cout << "Result: " << result << std::endl;
return 0;
}
What is a Floating Point Exception?
A floating point exception occurs when an operation on floating point types results in an error. Floating point numbers are used for representing real numbers that can have decimal places, and their representation in binary format makes them susceptible to specific issues during calculations. Understanding these exceptions is crucial as they can lead to unexpected behaviors in programs, impacting reliability and correctness.

Importance of Understanding Floating Point Exceptions
Grasping the concept of floating point exceptions is vital for developers as they play a significant role in numerical computing. Ignoring these can result in programs that behave unpredictably, leading to inaccuracies and potential vulnerabilities. Recognizing floating point exceptions allows developers to write safer, more robust code and ultimately improve the quality and performance of their applications.
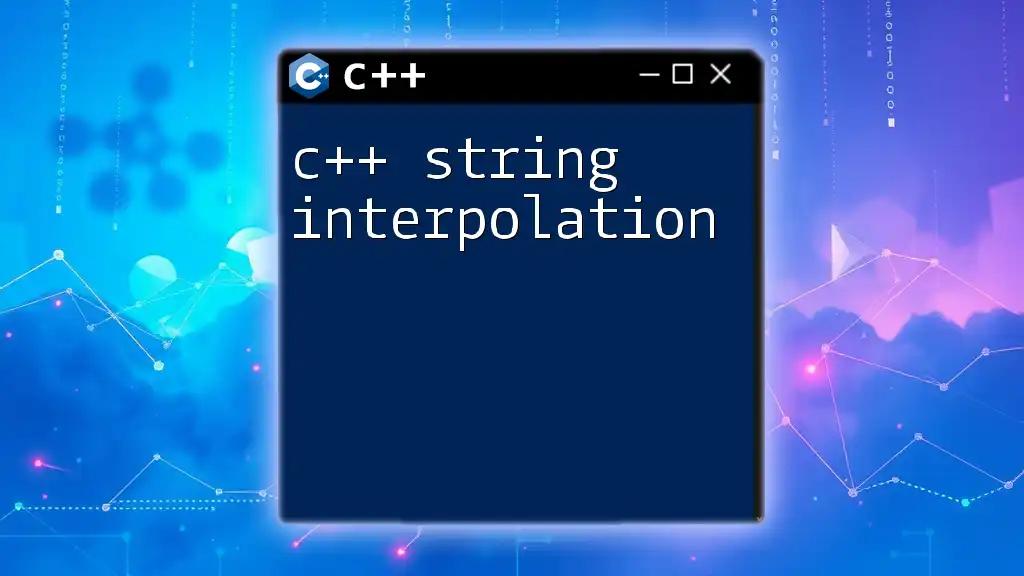
Types of Floating Point Exceptions in C++
Understanding the variety of floating point exceptions can help developers anticipate and handle issues more effectively. The most common types include:
Division by Zero
Division by zero is perhaps the most straightforward issue. When a floating point number is divided by zero, the operation may lead to undefined behavior, often resulting in special values such as positive or negative infinity.
Example of Division by Zero in C++
#include <iostream>
int main() {
float a = 5.0;
float b = 0.0;
float result = a / b; // Attempting to divide by zero leads to undefined behavior.
std::cout << "Result: " << result << std::endl; // Will not print a valid number.
return 0;
}
In this example, `result` becomes undefined due to the division by zero, demonstrating the necessity of careful checks before arithmetic operations.
Overflow and Underflow
Overflow occurs when a calculation produces a value larger than the maximum representable floating point number, while underflow happens when the result of an operation is too small and approaches zero, effectively losing precision.
Example of Overflow in C++
#include <iostream>
#include <limits> // For std::numeric_limits
int main() {
float largeNumber = std::numeric_limits<float>::max();
float result = largeNumber * 2; // This operation may cause overflow.
std::cout << "Result: " << result << std::endl; // Likely outputs 'inf'.
return 0;
}
Here, the multiplication leads to overflow, and 'inf' indicates the maximum possible value in floating point.
Example of Underflow in C++
#include <iostream>
#include <limits> // For std::numeric_limits
int main() {
float smallNumber = std::numeric_limits<float>::min();
float result = smallNumber / 2; // This operation may result in underflow.
std::cout << "Result: " << result << std::endl; // Likely outputs '0'.
return 0;
}
In this case, the division by two results in a number so small that it effectively becomes zero, losing detail and precision.
Inexact Results
Due to the limitations of floating point representation, some operations may yield inexact results. This typically occurs because certain decimal fractions cannot be represented exactly as binary fractions.
Example of Inexact Results in C++
#include <iostream>
int main() {
float a = 0.1f;
float b = 0.2f;
float sum = a + b;
std::cout << "Sum of 0.1 and 0.2 is: " << sum << std::endl; // May not equal 0.3 exactly.
return 0;
}
The output of this example may not equal `0.3` due to floating point precision issues, demonstrating the necessity for caution when performing arithmetic with floating point numbers.
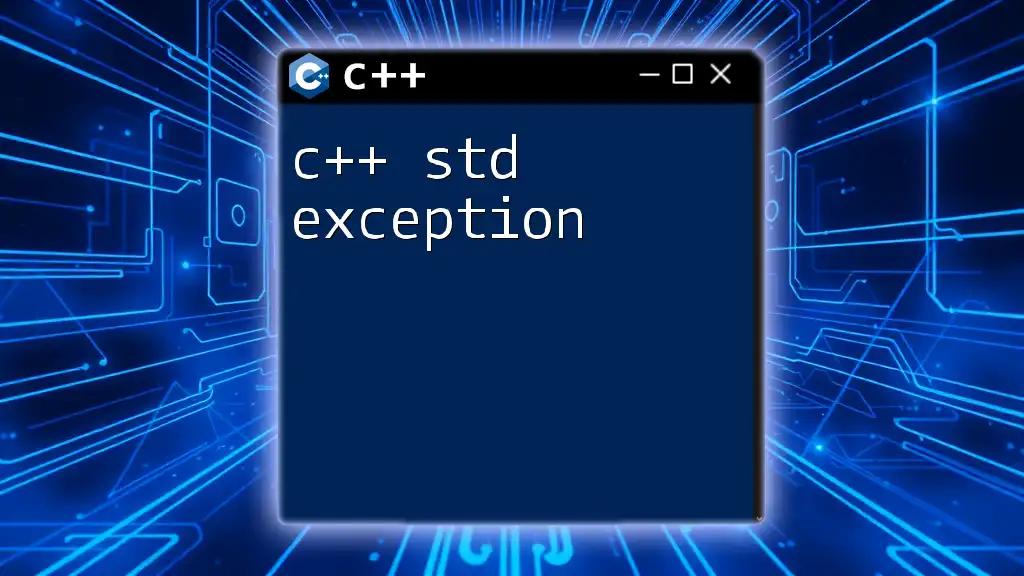
Handling Floating Point Exceptions in C++
Best Practices for Avoiding Floating Point Exceptions
To reduce the risk of floating point exceptions, developers can follow several best practices:
- Always validate inputs before performing calculations, especially for operations that could lead to division by zero.
- Prefer integer arithmetic where appropriate, and only use floating point types when necessary.
- Utilize higher precision types (e.g., `double`) to minimize rounding errors in critical calculations.
Error Handling Techniques
Proper error handling is crucial when dealing with floating point exceptions. C++ offers robust mechanisms to catch and manage exceptions, improving the resilience of applications.
Example of Error Handling
#include <iostream>
#include <stdexcept>
int main() {
float a = 5.0;
float b = 0.0;
try {
if (b == 0) {
throw std::runtime_error("Division by zero exception");
}
float result = a / b;
std::cout << "Result: " << result << std::endl;
} catch (const std::exception& e) {
std::cerr << e.what() << std::endl; // Catch and handle the floating point exception.
}
return 0;
}
In this code, a division by zero is anticipated and handled with an exception, ensuring the program does not crash unexpectedly.

Debugging Floating Point Exceptions
When floating point exceptions occur, proper debugging techniques can help identify the root cause.
Techniques for Debugging
- Trace back calculations: Examine the sequence of operations leading to the exception.
- Use assertions: Assert preconditions on inputs or postconditions on outcomes to catch potential floating point issues early.
- Monitor precision: Keep a close eye on the range of values your program is working with, and log operations extensively.
Common Debugging Tools for C++
Tools like GDB and Valgrind can be invaluable in pinpointing floating point exceptions. GDB can help track the execution flow, while Valgrind can identify memory issues and precision errors, allowing developers to address these issues proactively.

Conclusion
Understanding the intricacies of C++ floating point exceptions is instrumental for writing robust and precise numerical code. By acknowledging the potential for errors such as division by zero, overflow, underflow, and inexact results, developers can take proactive steps to manage these issues effectively. Implementing best practices, using error handling techniques, and employing debugging tools will enhance the reliability of software. Ultimately, familiarity with these concepts paves the way for more confident and capable programming in C++.