An online application for C++ allows users to write, execute, and debug C++ code directly in their web browser, enhancing accessibility and convenience for learners.
Here's a simple code snippet to demonstrate a basic C++ program that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding CPP Online Applications
What is a CPP Online Application?
A CPP online application refers to software developed using C++ that operates via the internet. This type of application leverages the high performance and low-level capabilities of C++, making it suitable for tasks requiring speed and resource efficiency. By utilizing C++, developers can create a diverse range of online applications, from servers to sophisticated user interfaces.
The advantages of using C++ for online applications include:
- Performance: C++ delivers faster execution compared to many other high-level languages. This is crucial for applications dealing with heavy computations or real-time data processing.
- Control: C++ allows developers lower-level access to system resources, which can lead to more efficient usage of memory and processing power.
- Cross-Platform Compatibility: Applications written in C++ can be easily compiled to run on different platforms, making it versatile for web-based use.
Use Cases for CPP Online Applications
CPP online applications shine in several real-world scenarios:
- Game Development: Many online games utilize C++ for their engines due to the need for real-time performance and complex 3D graphics rendering.
- High-Performance Web Servers: C++ is often employed to create servers that require processing large volumes of requests swiftly, such as those used in cloud computing or web services.
- Financial Systems: Trading platforms or backend services for financial apps often use C++ for its performance in handling real-time data and calculations.
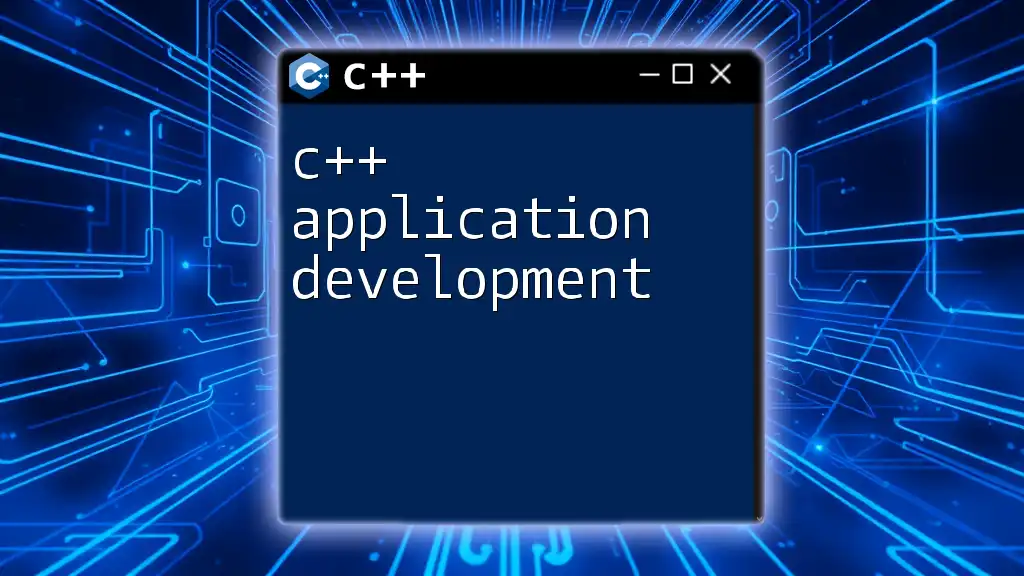
Setting Up Your Development Environment
Choosing the Right IDE for C++
Selecting the right Integrated Development Environment (IDE) is crucial for developing CPP online applications efficiently. Here are a few popular IDEs to consider:
- Visual Studio: Known for its comprehensive features, debugging capabilities, and easy integration with various tools.
- Code::Blocks: A free, open-source IDE that provides flexibility with plugins and configurations.
- CLion: A powerful IDE from JetBrains that offers intelligent code assistance and supports CMake.
Installing Necessary Tools
To begin developing CPP applications, it’s essential to set up the relevant tools:
- Compilers: Install a compiler such as GCC (GNU Compiler Collection) or Clang to translate your C++ code into machine code. Detailed installation guides can be found on their respective websites.
- Libraries: Depending on your project requirements, consider installing libraries such as Boost for providing additional functionality, and OpenSSL for secure networking. Libraries enhance your application's capabilities without the need to reinvent the wheel.
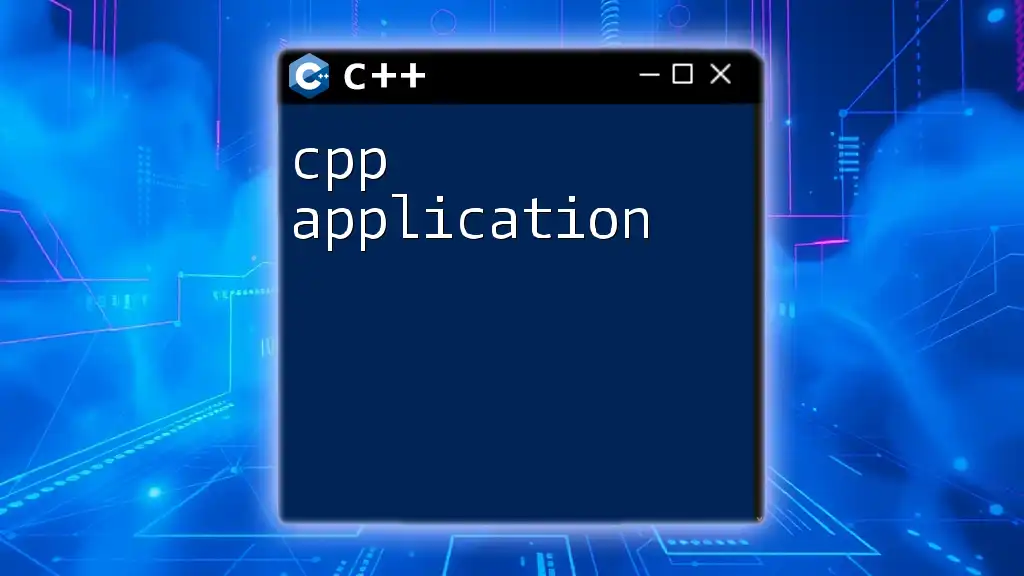
Key C++ Concepts for Online Applications
Object-Oriented Programming Fundamentals
C++ is rooted in Object-Oriented Programming (OOP), which is vital for developing maintainable and scalable applications. Key principles include:
- Encapsulation: Bundling data with methods that operate on that data leads to reduced complexity and increased modularity.
- Inheritance: This allows the creation of new classes based on existing classes, facilitating code reuse and extension.
- Polymorphism: Enables methods to do different things based on the object invoking them, making your application more flexible.
Memory Management Techniques
Effective memory management in C++ is critical, especially in online applications where resource optimization is paramount.
- Dynamic Memory: C++ provides operators like `new` and `delete` to manage dynamic memory. Allocating and freeing memory correctly helps avoid memory leaks and enhances performance.
Example of memory management:
int* numbers = new int[10]; // Allocating memory
// Use your numbers array
delete[] numbers; // Deallocating memory
Concurrent Programming in C++
Online applications often need to handle multiple tasks simultaneously. C++ supports concurrent programming through threads, which can significantly improve performance.
A simple multi-threaded application example:
#include <iostream>
#include <thread>
void sayHello() {
std::cout << "Hello from thread!\n";
}
int main() {
std::thread t1(sayHello); // Create a thread
t1.join(); // Wait for the thread to finish
return 0;
}
The above code illustrates how to create and manage threads in C++. Understanding threading will be invaluable for managing tasks like client requests or processing data in parallel.
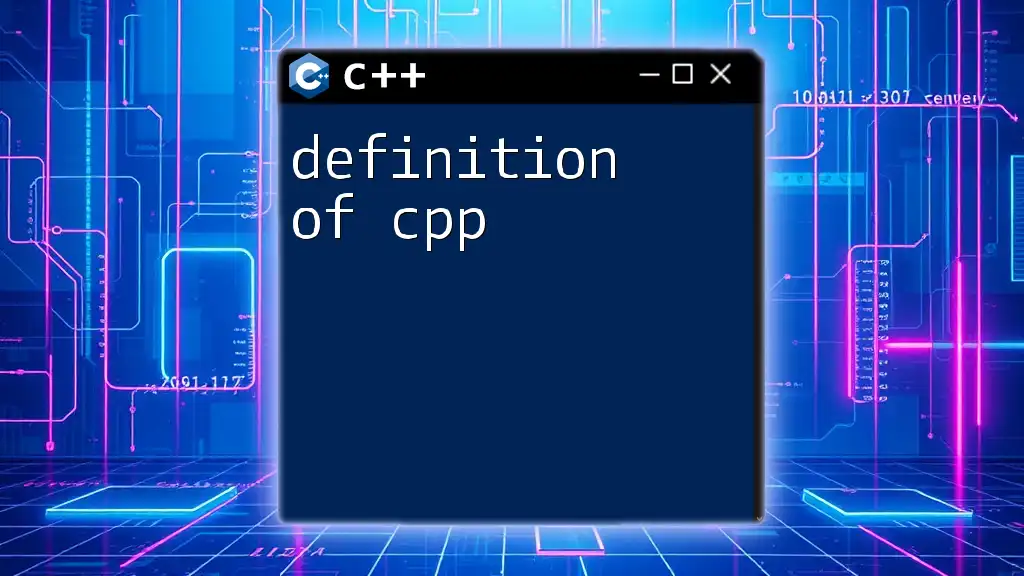
Building Your First CPP Online Application
Project Structure and Organization
Maintaining a well-organized project structure enhances navigation and maintenance. A suggested structure might look like this:
MyCPPApp/
├── src/ // Source files (.cpp)
├── include/ // Header files (.h)
├── lib/ // External libraries
├── build/ // Compiled files
└── README.md // Documentation
Writing Your First CPP Code
Let’s create a basic CPP program that takes user input and displays it. This is fundamental to building interactive online applications.
#include <iostream>
#include <string>
int main() {
std::string userInput;
std::cout << "Enter your message: ";
std::getline(std::cin, userInput);
std::cout << "You entered: " << userInput << std::endl;
return 0;
}
In this simple program, we utilize standard input/output to interact with the user. Understanding how to handle input and output is essential for any online application.
Integrating with Online APIs
Communicating with online services or databases often means making HTTP requests. Libraries like cURL simplify this process.
Example of making a GET request using cURL:
#include <curl/curl.h>
int main() {
CURL *curl;
curl_global_init(CURL_GLOBAL_DEFAULT);
curl = curl_easy_init();
if(curl) {
curl_easy_setopt(curl, CURLOPT_URL, "https://api.example.com/data");
CURLcode res = curl_easy_perform(curl);
curl_easy_cleanup(curl);
}
curl_global_cleanup();
return 0;
}
This code initializes a cURL session, sets a target URL, and executes the request. Understanding API integration is essential for fetching data in online applications.
Deploying Your Application Online
Selecting a Hosting Service
Once your application is developed, the next step is deployment. Choose a hosting service that fits your requirements. Factors to consider include:
- Performance: Look for servers optimized for C++ applications.
- Scalability: Ensure that the provider allows you to scale resources as needed.
- Cost: Evaluate your budget against the offerings of different services.
Steps for Deployment
- Uploading Files: Use FTP/SFTP to transfer your application files to the server.
- Server Configuration: Set up necessary configurations, including firewalls and databases, ensuring that security settings are in place for a smooth operation.
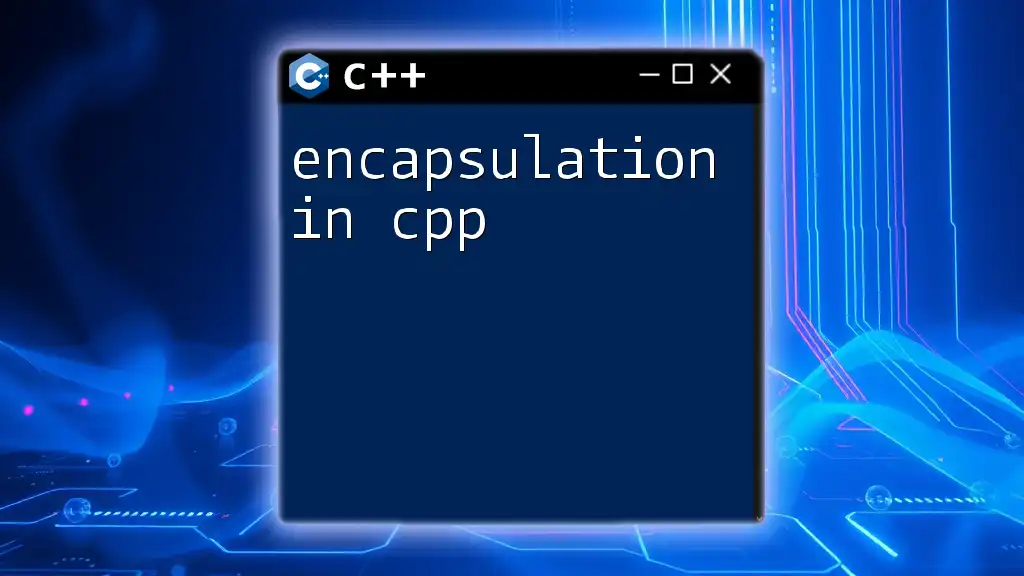
Testing and Debugging Your CPP Application
Importance of Testing in Application Development
Testing is a crucial component of the development lifecycle. It ensures that your application operates as intended and is free of critical bugs.
Common types of testing to incorporate include:
- Unit Testing: Isolate and test individual components for proper behavior.
- Integration Testing: Assess how well different parts of your application work together.
Debugging Techniques
Debugging is essential in identifying and resolving issues within your application. Utilize tools such as gdb (GNU Debugger) to diagnose problems:
Example of using gdb commands:
- To compile code with debugging info:
g++ -g my_program.cpp -o my_program
- To start debugging:
gdb ./my_program
Remember that effective debugging can significantly improve your application’s performance and reliability by identifying bottlenecks or faulty logic.
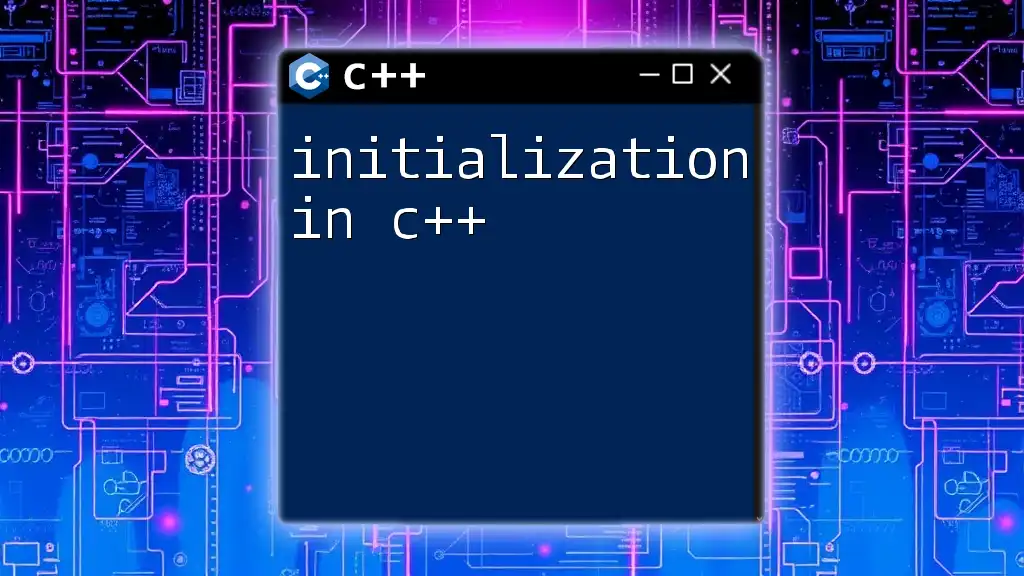
Enhancing Your CPP Online Application
Optimizing from a Performance Standpoint
Optimization is crucial for delivering a responsive online application. Here are practical strategies:
- Efficient Algorithms: Utilize optimal algorithms that reduce time complexity.
- Code Optimization: Streamline your code to minimize execution time. Profile your application using tools such as Valgrind to identify slow functions.
Implementing Security Features
Security is paramount when developing online applications. Basic measures include:
- Input Validation: Always validate user inputs to prevent malicious activities like SQL injection.
- Secure API Calls: Use HTTPS to encrypt API connections, ensuring data confidentiality.
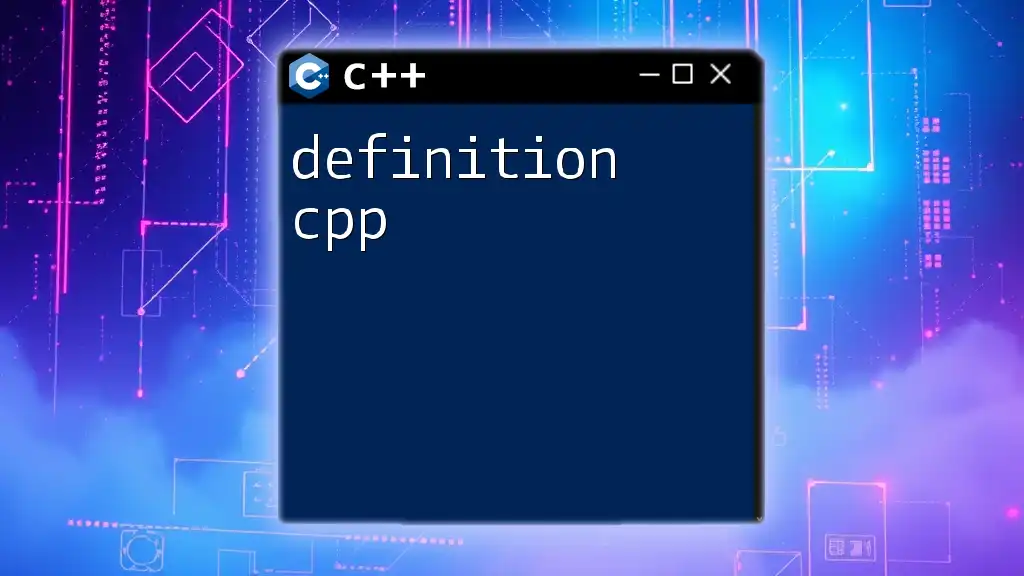
Conclusion
Throughout this article, we've explored what an online application for CPP entails, from understanding key concepts to building, deploying, and enhancing your application. C++ provides a robust foundation for creating high-performance applications, and as you continue to develop your skills, remember that practice and ongoing learning are key to success in the ever-evolving field of programming. Explore additional resources, engage with community forums, and keep challenging yourself to build increasingly complex applications.