Engineering problem solving with C++ involves leveraging the language's powerful features to create efficient algorithms and data structures that address complex challenges in engineering tasks.
Here's a simple code snippet demonstrating a C++ function to calculate the factorial of a number, showcasing problem-solving through recursion:
#include <iostream>
unsigned long long factorial(int n) {
if (n <= 1) return 1;
return n * factorial(n - 1);
}
int main() {
int num = 5;
std::cout << "Factorial of " << num << " is " << factorial(num) << std::endl;
return 0;
}
Understanding the Importance of Problem Solving
In engineering, the ability to identify and resolve challenges is paramount. Effective problem solving enhances design integrity, reduces costs, and improves product quality. C++ is an exceptional tool in this process, offering a robust framework to develop solutions efficiently.
C++ is known for its speed and powerful object-oriented programming capabilities, making it suitable for technical applications ranging from simulation to real-time systems.
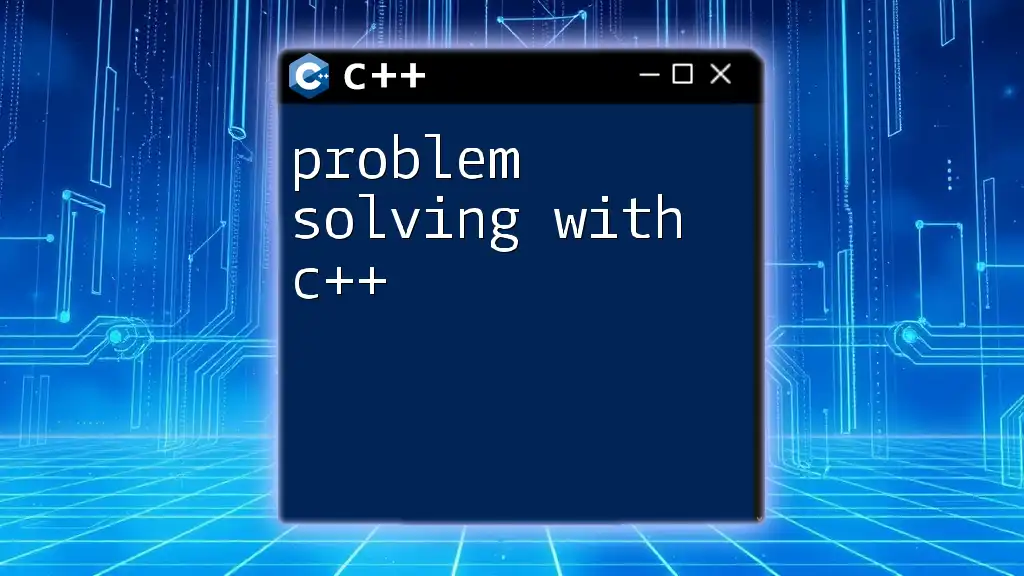
Identifying the Problem
The first step in the engineering problem-solving process is to clearly define the problem. This involves asking targeted questions to uncover the core issue. Techniques include:
- Root Cause Analysis: What is the underlying issue causing the problem?
- Stakeholder Interviews: What do users and engineers perceive as the challenges?
To illustrate, if an engineer is tasked with designing a new bridge, identifying concerns like load capacity, materials, and environmental impacts are vital.
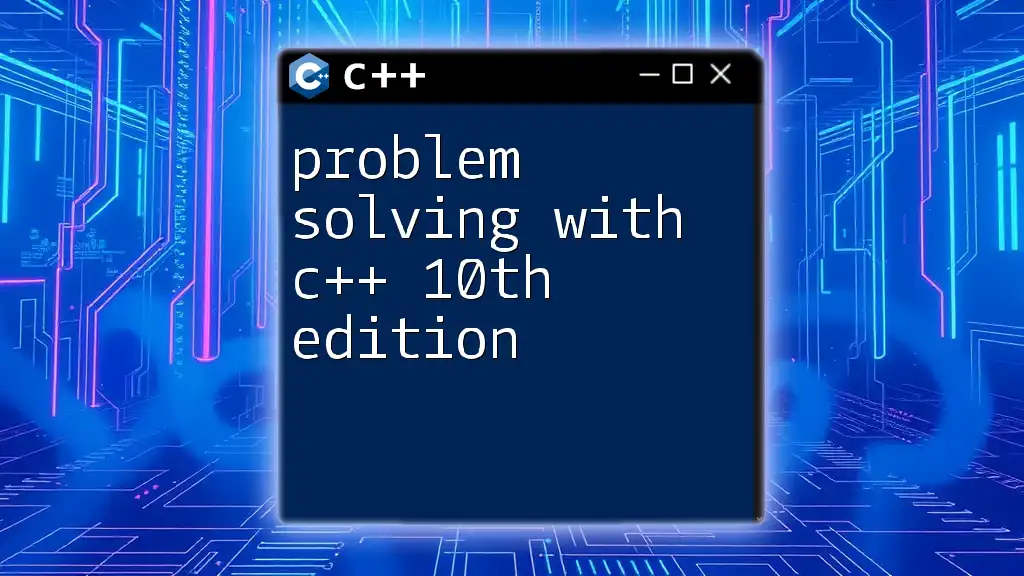
Analyzing and Understanding the Requirements
Once the problem is understood, it's essential to gather requirements and constraints. These could include:
- Functionality: What must the product do?
- Performance Metrics: How quickly must calculations be processed?
- Budget Limitations: What is the maximum expenditure allowed?
For instance, in structural design, the requirement may be to ensure a certain safety factor while minimizing material use.
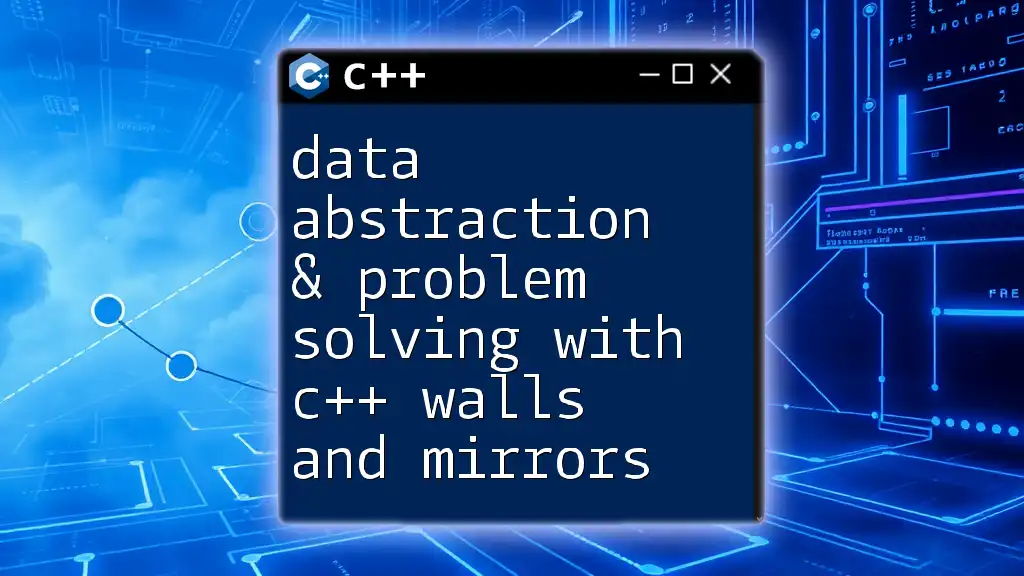
Developing the Plan
Creating a structured solution plan is crucial for effective problem-solving. Techniques like flowcharts and pseudocode can help visualize the process. For example, if developing a program to calculate a beam's deflection, one might outline the steps:
- Input beam specifications (length, material, loads)
- Calculate moment of inertia
- Compute deflection using applicable formulas
- Output results
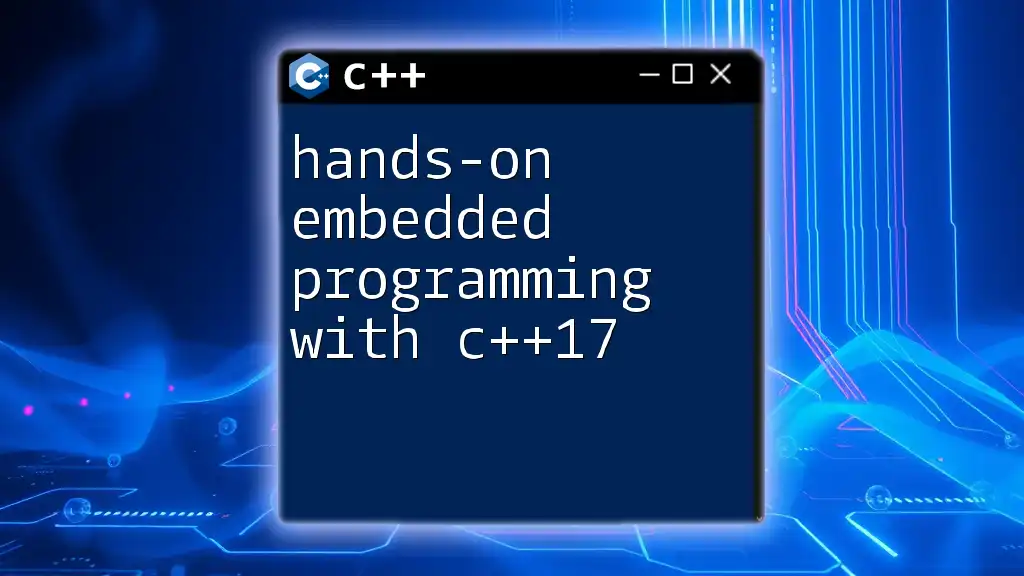
Implementing the Solution in C++
Basic C++ Constructs
Variables and Data Types
In C++, selecting appropriate data types can optimize performance. Consider the following code snippet demonstrating variable declarations:
int length = 10; // Length of the beam
double load = 15.5; // Applied load on the beam
bool isSafe = true; // Safety check
Control Structures
Control structures are essential for directing the flow of your program. Using `if`, `switch`, and loops can enhance your code's effectiveness. Below, we demonstrate a simple `if` statement:
if (isSafe) {
std::cout << "The structure is safe." << std::endl;
} else {
std::cout << "The structure is not safe!" << std::endl;
}
Functions and Modularity
Defining Functions
Organizing code into functions can significantly improve readability and reusability. Here is a basic function example for calculating the area of a rectangle:
double calculateArea(double length, double width) {
return length * width;
}
Overloading and Templates
C++ allows the use of function overloading and templates. This flexibility is especially useful in engineering, where you might need similar functionality for different data types:
template <typename T>
T add(T a, T b) {
return a + b;
}
Data Structures
Arrays and Vectors
When managing collections of data, arrays and vectors are indispensable. Below we compare their uses with a simple example:
// Using an array
int loads[5] = {10, 15, 20, 25, 30};
// Using a vector
#include <vector>
std::vector<double> moments = {0.5, 1.0, 1.5, 2.0, 2.5};
Structures and Classes
Structures and classes facilitate organization of complex data. This is crucial in engineering applications. Here’s how to implement a simple `Point` class:
class Point {
public:
double x, y;
Point(double xCoord, double yCoord) {
x = xCoord;
y = yCoord;
}
void display() {
std::cout << "Point(" << x << ", " << y << ")" << std::endl;
}
};
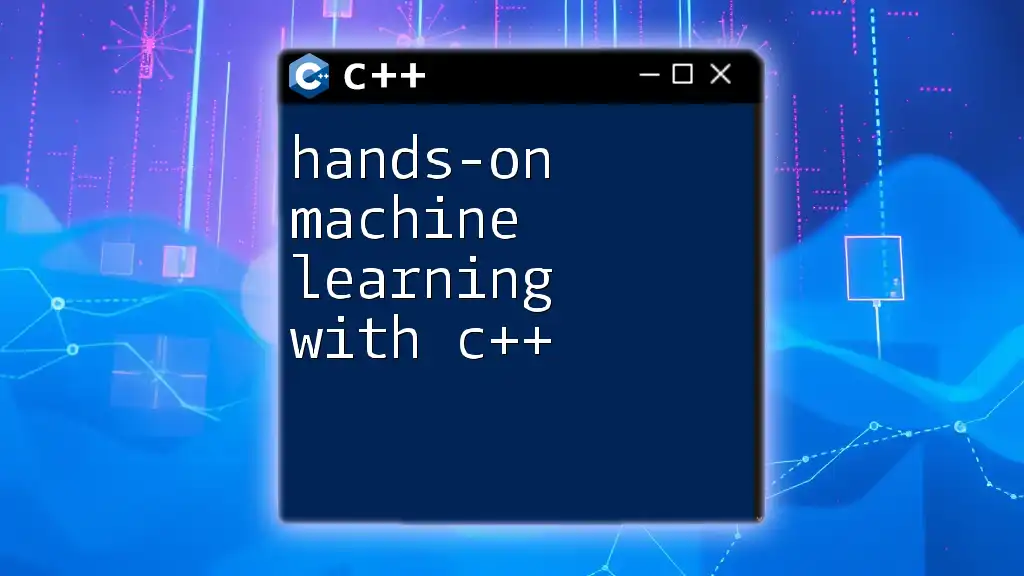
Applying Advanced Concepts
Object-Oriented Programming Principles
C++ embraces object-oriented programming (OOP) principles that can greatly simplify managing complexity in engineering applications. These include:
- Encapsulation: Keep data and functions safe within classes.
- Inheritance: Derive new classes from existing ones.
- Polymorphism: Allow methods to act differently based on the object invoking them.
For example, a simple shape hierarchy using inheritance could look like this:
class Shape {
public:
virtual void draw() = 0; // Pure virtual function
};
class Circle : public Shape {
public:
void draw() override {
std::cout << "Drawing a Circle" << std::endl;
}
};
Generic Programming with Templates
Templates in C++ facilitate generic programming, promoting code reusability. This is particularly advantageous when creating libraries for engineering applications:
template <typename T>
class Calculator {
public:
T add(T a, T b) {
return a + b;
}
};
Error Handling and Debugging Techniques
Handling errors gracefully is vital for robust applications. Employ `try`, `catch`, and assertions effectively in C++ for better reliability:
try {
if (load > maxLoad) {
throw std::runtime_error("Load exceeds maximum limit");
}
} catch (const std::exception& e) {
std::cerr << e.what() << std::endl;
}
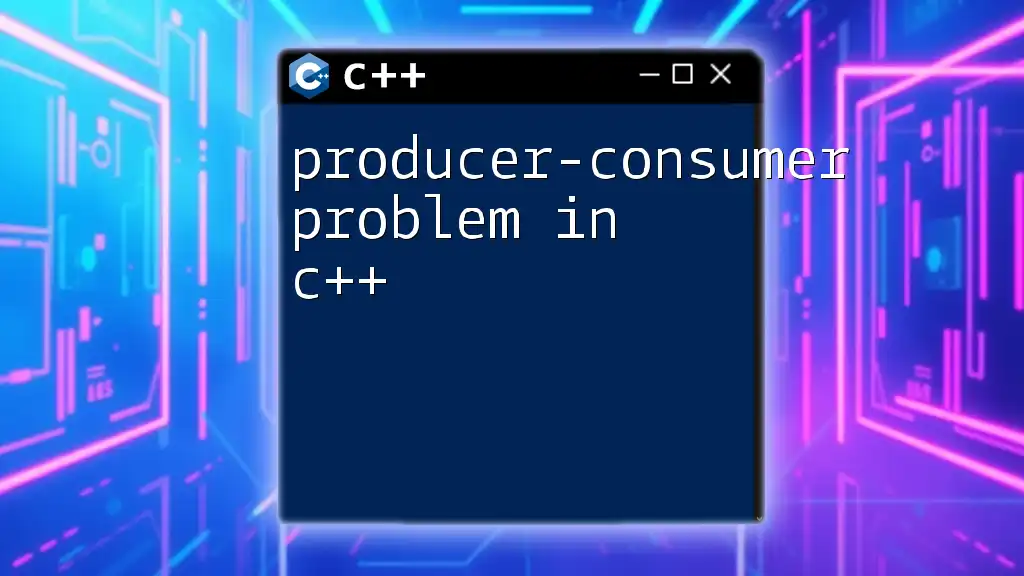
Real-World Engineering Problems and Their C++ Solutions
Case Study: Structural Analysis Software
Consider the challenge of evaluating a beam under a load. Utilizing C++, one could implement a program that computes deflection based on input parameters, employing earlier discussed concepts such as functions and classes:
double calculateDeflection(double load, double length, double momentOfInertia) {
return (load * length * length) / (48 * momentOfInertia);
}
Case Study: Simulation of Physical Systems
Simulations often require real-time calculations for system behavior. Using C++, you could create a simple model for particle movement:
class Particle {
public:
double x, y;
void move(double deltaX, double deltaY) {
x += deltaX;
y += deltaY;
}
};
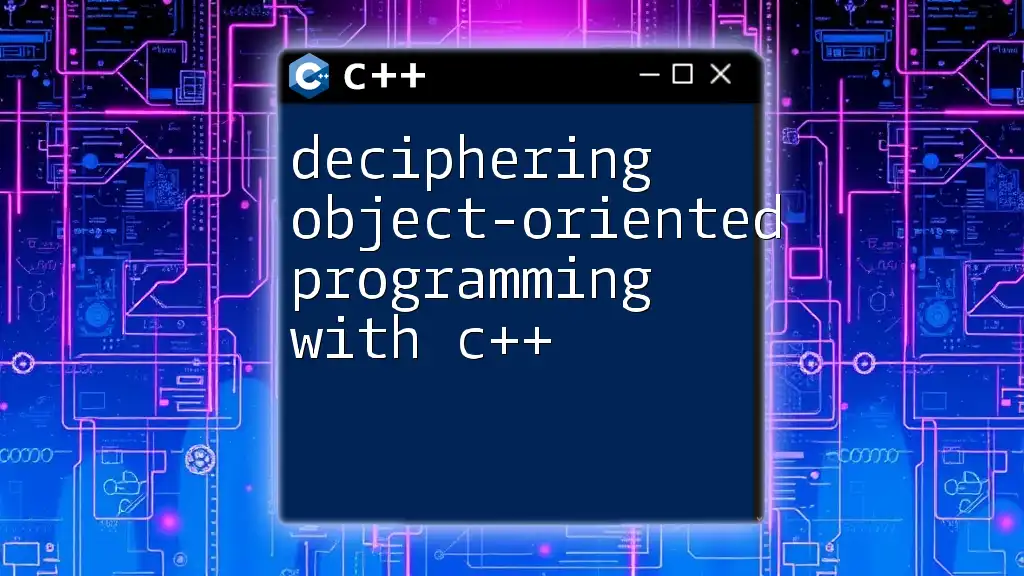
Performance Optimization in C++
Algorithm Efficiency
Understanding algorithm efficiency ensures the program scales and performs as required. Utilizing Big O notation helps in analyzing performance. For instance, compare a linear search to a binary search algorithm.
Memory Management
Proper memory management prevents leaks and ensures efficient execution. Utilizing pointers and smart pointers is crucial:
#include <memory>
std::unique_ptr<Point> p = std::make_unique<Point>(1.0, 2.0);
Profiling and Benchmarking Tools
Profiling tools such as Valgrind or gprof are vital for identifying bottlenecks. These tools help in interpreting the results to optimize performance effectively.
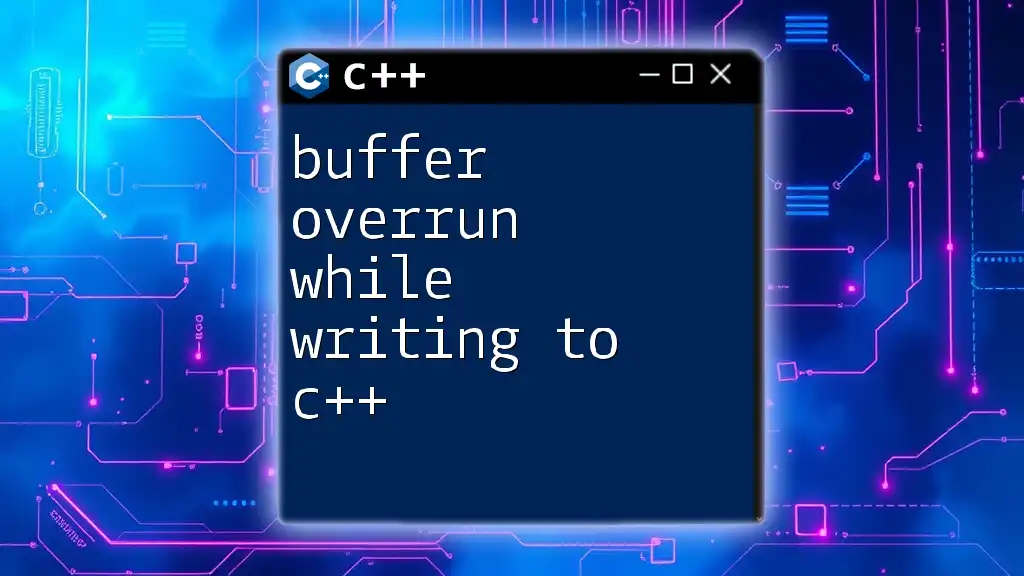
Summarizing the Importance of C++ in Engineering Problem Solving
C++ serves as a formidable ally in engineering problem solving due to its versatility, performance, and rich features. The capabilities of C++ empower engineers to tackle a wide range of challenges, ensuring solutions are both efficient and effective.
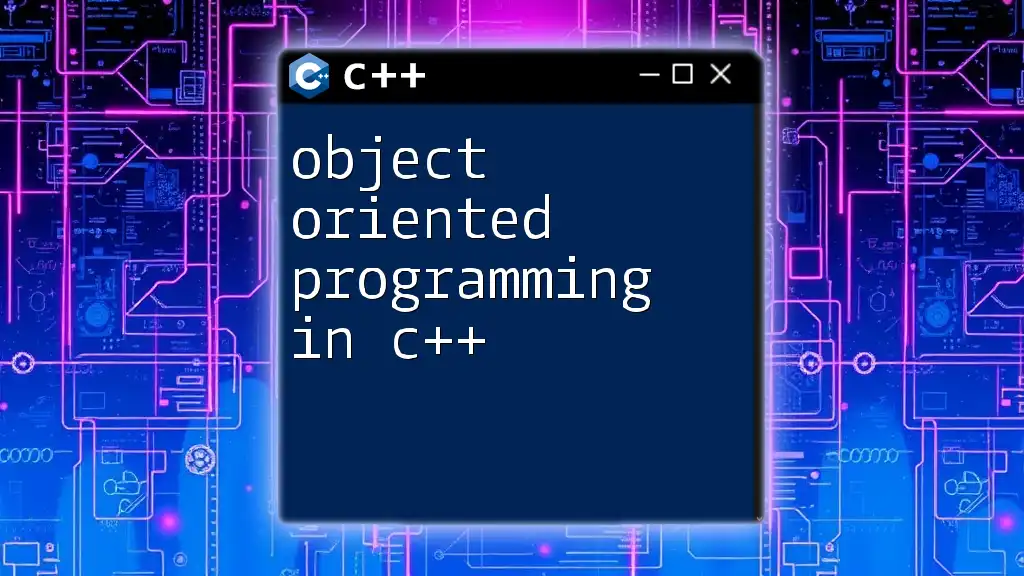
Encouraging Continuous Learning
To stay ahead in the field, continuous learning is crucial. Several resources can enhance your knowledge of C++ and its applications in engineering. Consider exploring books, online courses, and forums that cater specifically to C++ programming and engineering principles.
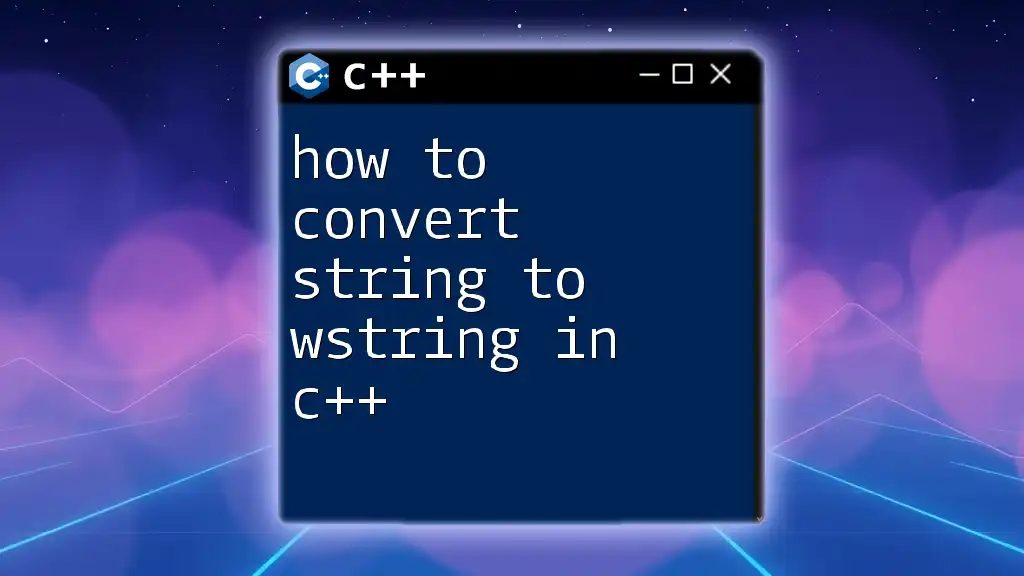
Additional Resources
Leverage recommended materials such as:
- Books: "The C++ Programming Language" by Bjarne Stroustrup, "Effective C++" by Scott Meyers.
- Online Courses: Udacity’s C++ Nanodegree, Coursera’s C++ for C Programmers.
- Libraries: Boost for generic programming tools, Eigen for linear algebra applications.
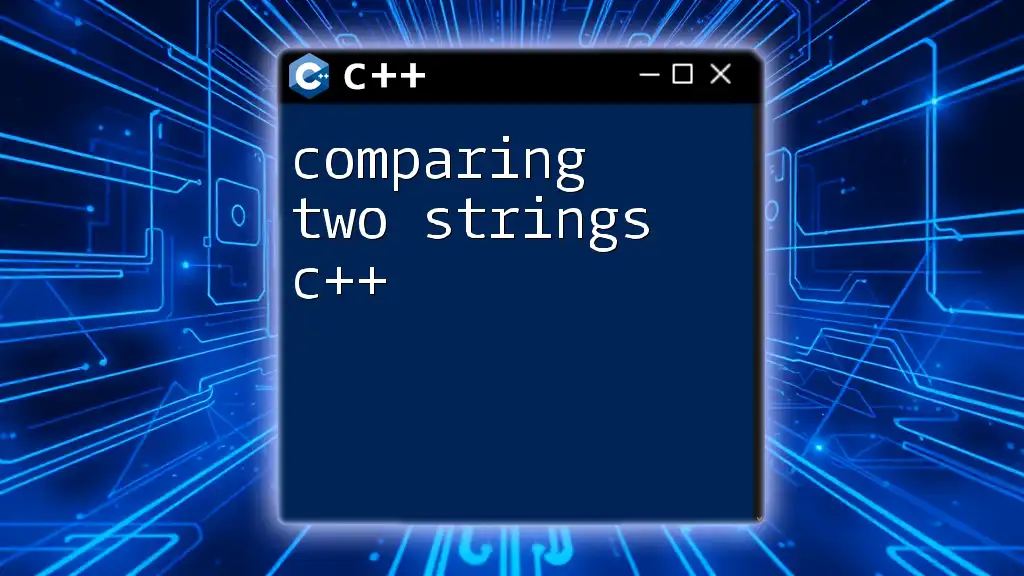
Call to Action
Now that you have an understanding of engineering problem solving with C++, empower yourself to harness this knowledge in your projects. Take the initiative to implement engineering challenges and share your findings with your community for feedback and growth.