The producer-consumer problem in C++ is a classic synchronization issue where one or more producers generate data that is consumed by one or more consumers, requiring a way to safely share the data between them to avoid race conditions.
Here’s a simple example using C++ with standard threading and a mutex:
#include <iostream>
#include <thread>
#include <queue>
#include <mutex>
#include <condition_variable>
#include <chrono>
std::queue<int> dataQueue;
std::mutex mu;
std::condition_variable condVar;
void producer(int id) {
for (int i = 0; i < 5; ++i) {
std::this_thread::sleep_for(std::chrono::milliseconds(100));
std::lock_guard<std::mutex> lock(mu);
dataQueue.push(i);
std::cout << "Producer " << id << " produced: " << i << std::endl;
condVar.notify_one();
}
}
void consumer(int id) {
for (int i = 0; i < 5; ++i) {
std::this_thread::unique_lock<std::mutex> lock(mu);
condVar.wait(lock, [] { return !dataQueue.empty(); });
int data = dataQueue.front();
dataQueue.pop();
std::cout << "Consumer " << id << " consumed: " << data << std::endl;
}
}
int main() {
std::thread prod1(producer, 1);
std::thread cons1(consumer, 1);
prod1.join();
cons1.join();
return 0;
}
Understanding the Producer-Consumer Problem
Definition and Concept
The producer-consumer problem in C++ involves two entities: producers and consumers. The producers generate data or resources and place them into a shared buffer, while consumers take data from that buffer for processing. This cycle creates a need for efficient synchronization to prevent resources from being wasted or corrupted.
To visualize the problem, think of a factory where workers (producers) create products and store them in a warehouse (buffer). Meanwhile, delivery trucks (consumers) pick up products to distribute. This system requires careful coordination to ensure that producers do not overwhelm the warehouse, and consumers timely pick up products to avoid delays.
Real-World Applications
The producer-consumer model has numerous applications in software systems. Here are some notable examples:
- Print Spooling: In printers, multiple documents are queued, and as one document is printed (consumed), the next can be sent (produced).
- Task Scheduling: Systems that manage tasks often need to queue tasks (produced) for processing by worker threads (consumed) in a controlled manner.
- Data Processing Pipelines: In data analytics, data is ingested (produced) and processed in batches (consumed) for analysis.

The Role of Synchronization
Why Synchronization is Necessary
In a concurrent environment, producers and consumers may access shared resources simultaneously. This can lead to race conditions, where the outcome depends on the sequence of events. Without synchronization, you risk data corruption and unexpected outputs. For example, if two producers attempt to add an item to a buffer simultaneously, it might lead to a scenario where both threads overwrite each other's data.
Common Synchronization Mechanisms in C++
Mutexes
A mutex (mutual exclusion) is a locking mechanism used to control access to shared resources. By utilizing mutexes, we can ensure that only one thread accesses a resource at a time.
To implement a mutex in C++, you can do the following:
#include <mutex>
std::mutex mtx; // protected shared resource
void producer() {
mtx.lock(); // Lock the mutex
// Produce item
mtx.unlock(); // Unlock the mutex
}
In this example, the mutex `mtx` is used to ensure that only one thread at a time can produce items, effectively preventing data corruption.
Condition Variables
Condition variables allow threads to wait until a particular condition is met, effectively signaling between producers and consumers. They are typically used in conjunction with a mutex.
For instance:
#include <condition_variable>
#include <queue>
std::queue<int> buffer;
std::mutex mtx;
std::condition_variable cv;
// Consumer function
void consumer() {
std::unique_lock<std::mutex> lock(mtx);
cv.wait(lock, [] { return !buffer.empty(); }); // Wait until buffer is not empty
int item = buffer.front();
buffer.pop();
lock.unlock();
}
In the consumer function, `cv.wait()` will block the thread until it is signaled that there is an item to consume. This avoids busy-waiting and is more efficient.
Alternative Mechanisms
Other synchronization tools include semaphores and barriers, each serving different use cases. A semaphore can be used for controlling access to a specific number of resources, making it ideal for situations where a limited number of threads can access the shared resource concurrently.

Implementation of the Producer-Consumer Problem in C++
Setup
To implement the producer-consumer problem in C++, basic libraries like `<thread>`, `<mutex>`, and `<condition_variable>` are essential. You will also need a buffer—a shared queue where the items are stored.
#include <iostream>
#include <thread>
#include <mutex>
#include <condition_variable>
#include <queue>
std::queue<int> buffer;
std::mutex mtx;
std::condition_variable cv;
const int bufferSize = 10; // Limit of buffer size
Producer Function
The producer's role is to create items and store them in the buffer. Here's how to implement the producer function:
void producer(int id) {
for (int i = 0; i < 10; ++i) {
std::this_thread::sleep_for(std::chrono::milliseconds(100)); // Simulate production time
std::lock_guard<std::mutex> lock(mtx);
while (buffer.size() == bufferSize) { // Buffer is full
// Wait until there's space
}
buffer.push(i);
cv.notify_one(); // Notify a waiting consumer
std::cout << "Produced: " << i << std::endl;
}
}
In this code, the producer waits if the buffer is full before adding new items. The `cv.notify_one()` call wakes up one consumer thread, signaling it to start consuming.
Consumer Function
The consumer's function is straightforward—consume items from the buffer:
void consumer() {
while (true) {
std::unique_lock<std::mutex> lock(mtx);
cv.wait(lock, [] { return !buffer.empty(); }); // Wait for items
int item = buffer.front();
buffer.pop();
std::cout << "Consumed: " << item << std::endl;
lock.unlock();
}
}
Putting It All Together
Finally, to connect everything and create threads for the producers and consumers, use the following setup in the main function:
int main() {
std::thread p1(producer, 1);
std::thread c1(consumer); // Start consumer code here.
p1.join(); // Wait for producer to finish
c1.join(); // Wait for consumer to finish
return 0;
}
This main function initializes one producer thread and one consumer thread, managing their execution and ensuring clean exits.
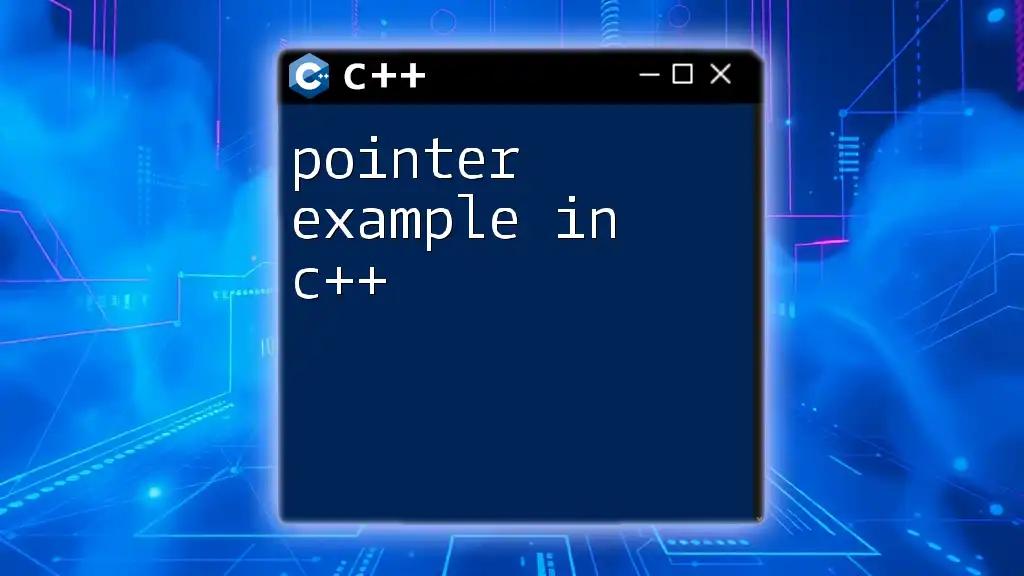
Common Pitfalls and Solutions
Deadlocks
A deadlock occurs when two or more threads are unable to proceed because they are waiting for each other to release resources. To prevent deadlocks:
- Use a consistent order when locking multiple resources.
- Implement a timeout mechanism to help a thread back off if it cannot acquire a lock in a given timeframe.
Buffer Overflow and Underflow
Managing the buffer size carefully is crucial to prevent overflow (when producers add items to a full buffer) and underflow (when consumers attempt to poll from an empty buffer). Always check the state of the buffer before adding or removing items and utilize condition variables to synchronize access properly.

Testing and Debugging the Producer-Consumer Model
Techniques for Testing
To ensure your implementation works, unit testing is essential. You can create tests for the producer and consumer functions, checking if they can handle different scenarios and buffer limits. Additionally, use stress tests to simulate a high number of producers and consumers.
Debugging Tips
When debugging concurrent applications:
- Use logging to track the state of the buffer and operations performed by producers and consumers.
- Consider tools like GDB (GNU Debugger) or specialized tools for multithreading issues.
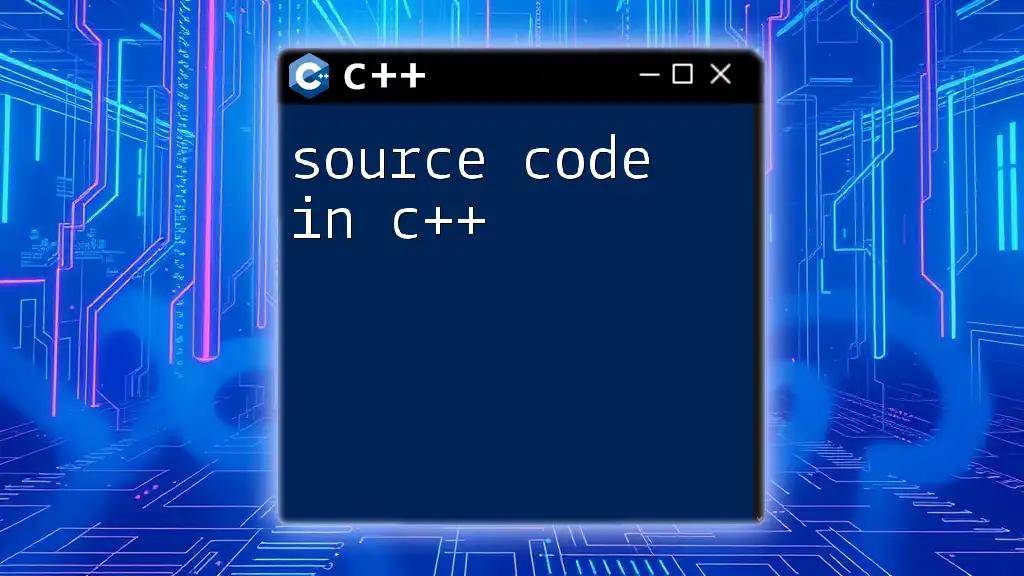
Conclusion
The producer-consumer problem in C++ exemplifies common challenges in concurrent programming, highlighting the need for synchronization mechanisms like mutexes and condition variables. By understanding this problem and implementing the provided strategies, you can build robust, multithreaded applications that handle resources effectively. Experimenting with the provided examples and extending them will deepen your understanding of C++ concurrency. Don't hesitate to dive into this fundamental aspect of programming—it will significantly enhance your skills!

Additional Resources
For those looking to dive deeper into the intricacies of concurrency and synchronization in C++, consider exploring official documentation, dedicated C++ tutorials, and books focused on multithreading practices.