Object-oriented programming in C++ enables developers to create modular and reusable code by defining classes and objects, which encapsulate data and behavior together.
Here's a simple example demonstrating the concept of a class and object in C++:
#include <iostream>
using namespace std;
class Car {
public:
string brand;
int year;
void displayInfo() {
cout << "Brand: " << brand << ", Year: " << year << endl;
}
};
int main() {
Car myCar;
myCar.brand = "Toyota";
myCar.year = 2021;
myCar.displayInfo();
return 0;
}
Understanding Object-Oriented Programming
What is Object-Oriented Programming?
Object-Oriented Programming (OOP) is a programming paradigm based on the concept of "objects", which can contain data in the form of fields (often known as attributes) and code in the form of procedures (often known as methods). This approach allows for a more organized structure by modeling real-world entities, which facilitates easier software development and maintenance.
Key Principles of OOP
OOP is founded on four main concepts that help build modular and maintainable software systems. Understanding these principles is essential for deciphering object-oriented programming with C++.
Encapsulation
Encapsulation is the bundling of the data with the methods that operate on that data, restricting direct access to some of the object's components. This creates a protective barrier that prevents outside interference and misuse of the methods and attributes.
- Benefits of Encapsulation:
- Control Over Data: You can control how the data is accessed or modified.
- Flexibility and Maintenance: Changes can be made inside the class without affecting external code.
Code Example: Demonstrating encapsulation in a class
class Account {
private:
double balance;
public:
void Deposit(double amount) {
balance += amount;
}
double GetBalance() const {
return balance;
}
};
In the example above, the `balance` attribute is private, ensuring it cannot be accessed directly from outside the class. Instead, operations on the balance are controlled through public methods like `Deposit` and `GetBalance`.
Inheritance
Inheritance is a mechanism through which one class (derived class) can inherit attributes and methods from another class (base class). This promotes code reusability and establishes a relationship between classes.
- Benefits of Inheritance:
- Reusability: No need to rewrite common functionalities.
- Improved Organization: Helps in managing and organizing code effectively.
Code Example: Simple inheritance demonstration
class Animal {
public:
void Speak() {
std::cout << "Animal speaks" << std::endl;
}
};
class Dog : public Animal {
public:
void Speak() {
std::cout << "Woof!" << std::endl;
}
};
Here, `Dog` inherits from `Animal` and can override the `Speak` method, allowing polymorphic behavior while still retaining access to the functionality defined in `Animal`.
Polymorphism
Polymorphism allows methods to do different things based on the object that it is acting upon. This can be implemented via function overriding and function overloading, enabling one interface to be used for a general class of actions.
- Compile-time vs. Run-time Polymorphism:
- Compile-time: Achieved through function overloading and operator overloading.
- Run-time: Achieved through method overriding with virtual functions.
Code Example: Demonstrating polymorphism with virtual functions
class Base {
public:
virtual void Show() {
std::cout << "Base class" << std::endl;
}
};
class Derived : public Base {
public:
void Show() override {
std::cout << "Derived class" << std::endl;
}
};
In this example, the `Show` method in `Derived` overrides the same method in `Base`, demonstrating how polymorphism lets the program decide at runtime which method to execute.
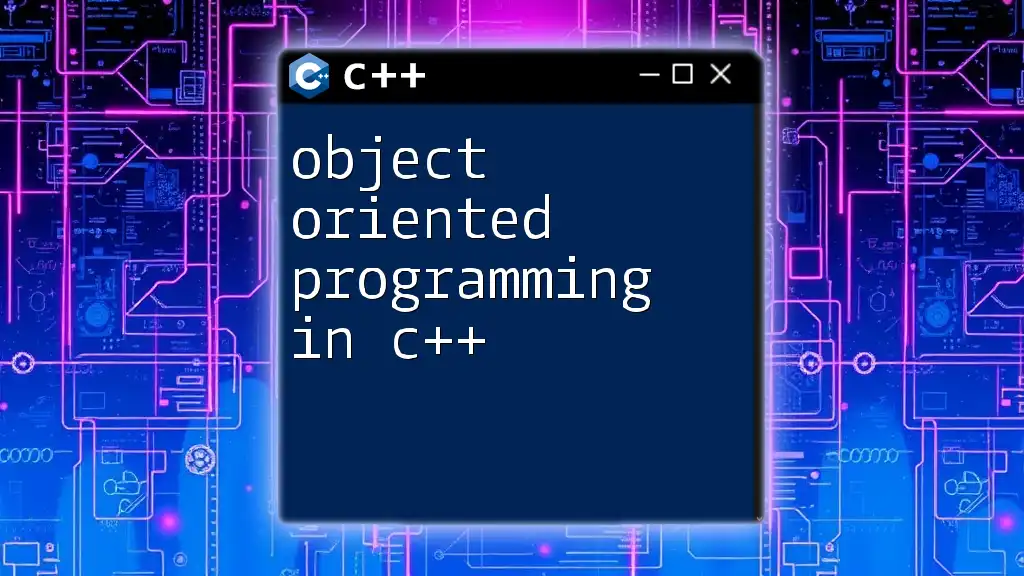
C++ Implementations of OOP
Classes and Objects
A class serves as a blueprint for creating objects, which are instances of classes. Classes specify the attributes the objects will have and implement the methods that define the behaviors.
Code Snippet: Simple class and object creation
class Car {
public:
string brand;
void Honk() {
std::cout << "Beep Beep!" << std::endl;
}
};
int main() {
Car myCar;
myCar.brand = "Toyota";
myCar.Honk();
}
In the code, `Car` acts as a blueprint, creating an object `myCar`. The `Honk` method associated with the `Car` class is called via the object.
Constructors and Destructors
Importance of Constructors
Constructors are special class functions that are called when an object is created. They are used to initialize member variables.
- Types of Constructors:
- Default constructor
- Parameterized constructor
Code Example: Using a constructor
class Rectangle {
public:
double length, width;
Rectangle(double l, double w) {
length = l;
width = w;
}
};
In this example, the `Rectangle` class uses a parameterized constructor to initialize the `length` and `width` attributes.
Destructors
A destructor is invoked when an object is destroyed, often used to free resources that the object may have acquired during its lifetime.
Code Example: Using a destructor
class Sample {
public:
Sample() {
std::cout << "Constructor called" << std::endl;
}
~Sample() {
std::cout << "Destructor called" << std::endl;
}
};
This example shows how a constructor and destructor provide lifecycle management for `Sample` objects.
Member Functions
Member functions are functions that are defined within a class and are designed to manipulate the class's data members.
- Access Specifiers: C++ provides three access specifiers:
- Public: Accessible from outside the class.
- Private: Accessible only within the class.
- Protected: Accessible in the class and in derived classes.
Code Example: Member functions in action
class Circle {
public:
double radius;
double Area() {
return 3.14159 * radius * radius;
}
};
Here, `Area()` is a member function that calculates the area of the circle based on its `radius`.
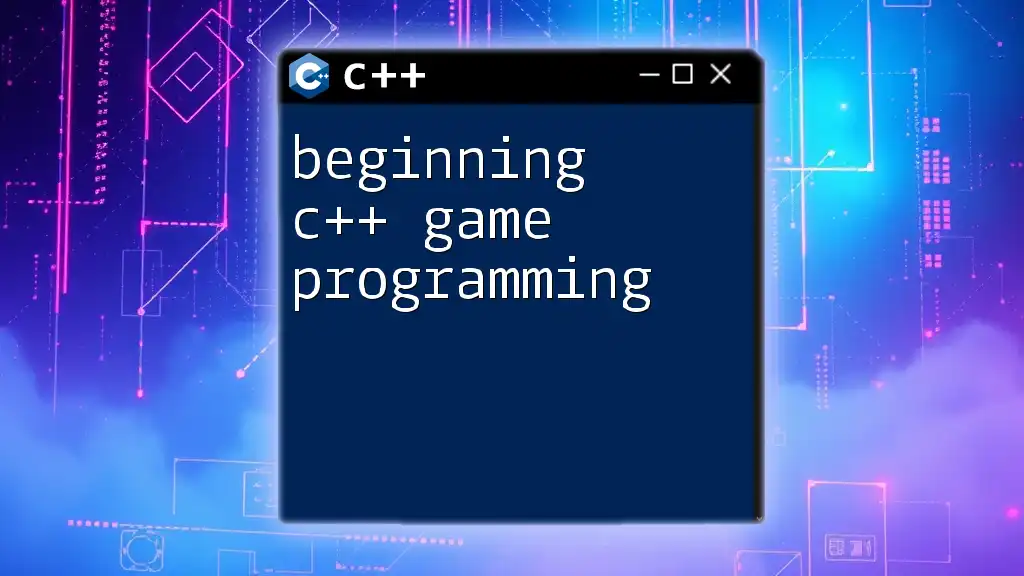
Advanced Concepts in OOP
Multiple Inheritance
Multiple Inheritance allows a class to inherit from more than one base class, which can lead to complex scenarios but also greater flexibility.
- Advantages:
- Enhanced functionality by inheriting features from multiple classes.
- Disadvantages:
- Increased complexity and potential for ambiguity (the "diamond problem").
Code Example: Demonstrating multiple inheritance
class Parent1 {
public:
void Function1() {}
};
class Parent2 {
public:
void Function2() {}
};
class Child : public Parent1, public Parent2 {};
In this case, `Child` inherits from both `Parent1` and `Parent2`, allowing it access to both sets of functionalities.
Abstract Classes and Interfaces
An Abstract Class is a class that cannot be instantiated and typically contains one or more pure virtual functions. These classes provide a base for derived classes to implement specific functionality.
Importance of Abstract Classes in OOP:
- They define a contract for classes that derive from them, enforcing a certain structure or behavior.
Code Example: Abstract classes and pure virtual functions
class AbstractAnimal {
public:
virtual void MakeSound() = 0; // Pure virtual function
};
In this example, `AbstractAnimal` cannot be instantiated directly, and any derived class must implement the `MakeSound` function.
Operator Overloading
Operator Overloading allows developers to redefine the way operators work for user-defined classes. This enhances code readability and functionality when dealing with objects.
Why Overload Operators?
- To make user-defined types behave like built-in types by enabling operations to be performed with them.
Code Example: Overloading the + operator
class Complex {
public:
double real, imag;
Complex operator+(const Complex& obj) {
return {real + obj.real, imag + obj.imag};
}
};
In this code, the `+` operator is overloaded to support addition of `Complex` objects, adding their respective real and imaginary parts.
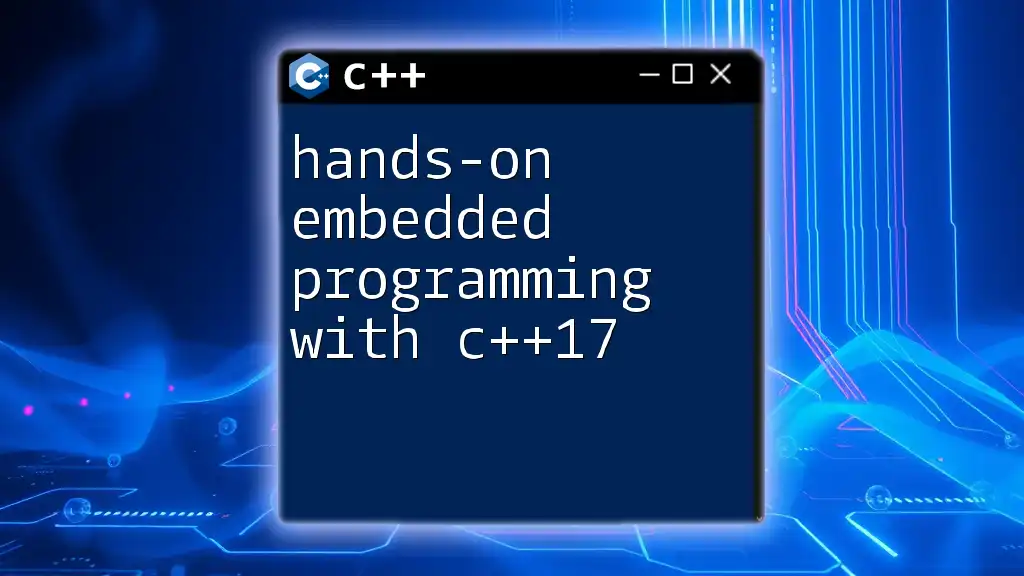
Practical Applications of OOP in C++
Use Cases of OOP
Object-oriented programming principles can be effectively applied across a wide range of domains, from simple applications to complex software systems. Industries such as finance, gaming, telecommunications, and healthcare increasingly leverage OOP in C++ to create maintainable and extendable software solutions.
Case Study: Designing a Simple Banking System
Consider a simple banking system where we manage user accounts and transactions. In such a system, we could design classes to represent various entities like `User`, `Account`, and `Transaction`.
- Designing Classes:
- User: Contains information such as name and user ID.
- Account: Represents a bank account with balance and transaction history.
- Transaction: Represents various types of transactions (deposits, withdrawals).
Code Snippet: Evolving Design with OOP principles
class User {
public:
string name;
string userID;
// Additional user properties and methods
};
class Account {
private:
double balance;
User accountHolder;
public:
void Deposit(double amount) {
balance += amount;
}
// Additional account functionalities
};
class Transaction {
public:
void ExecuteTransaction(Account& acc, double amount) {
// Example logic for executing a transaction
}
};
The classes above illustrate how to encapsulate data related to users, accounts, and transactions while promoting clear structures and easy expansions as requirements change.
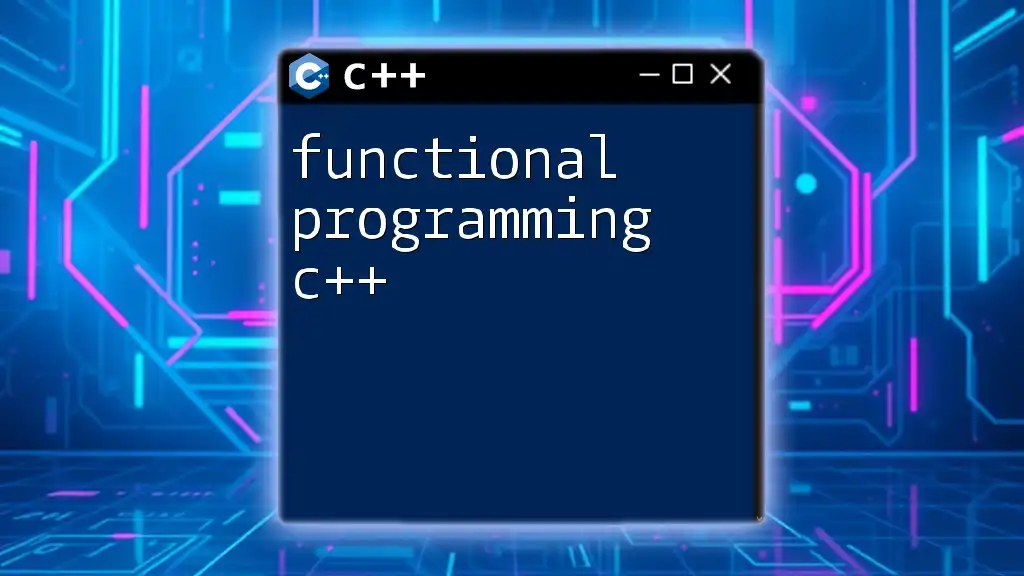
Conclusion
Understanding the essentials of deciphering object-oriented programming with C++ is critical for modern software development. By embracing the principles of encapsulation, inheritance, polymorphism, and other advanced OOP concepts, developers can create robust, modular, and maintainable software systems. As you continue your journey in C++, practice with these concepts and explore further reading to deepen your understanding. The effective application of OOP concepts will not only enhance your programming skills but also empower you to build sophisticated applications that mimic real-life entities and processes.